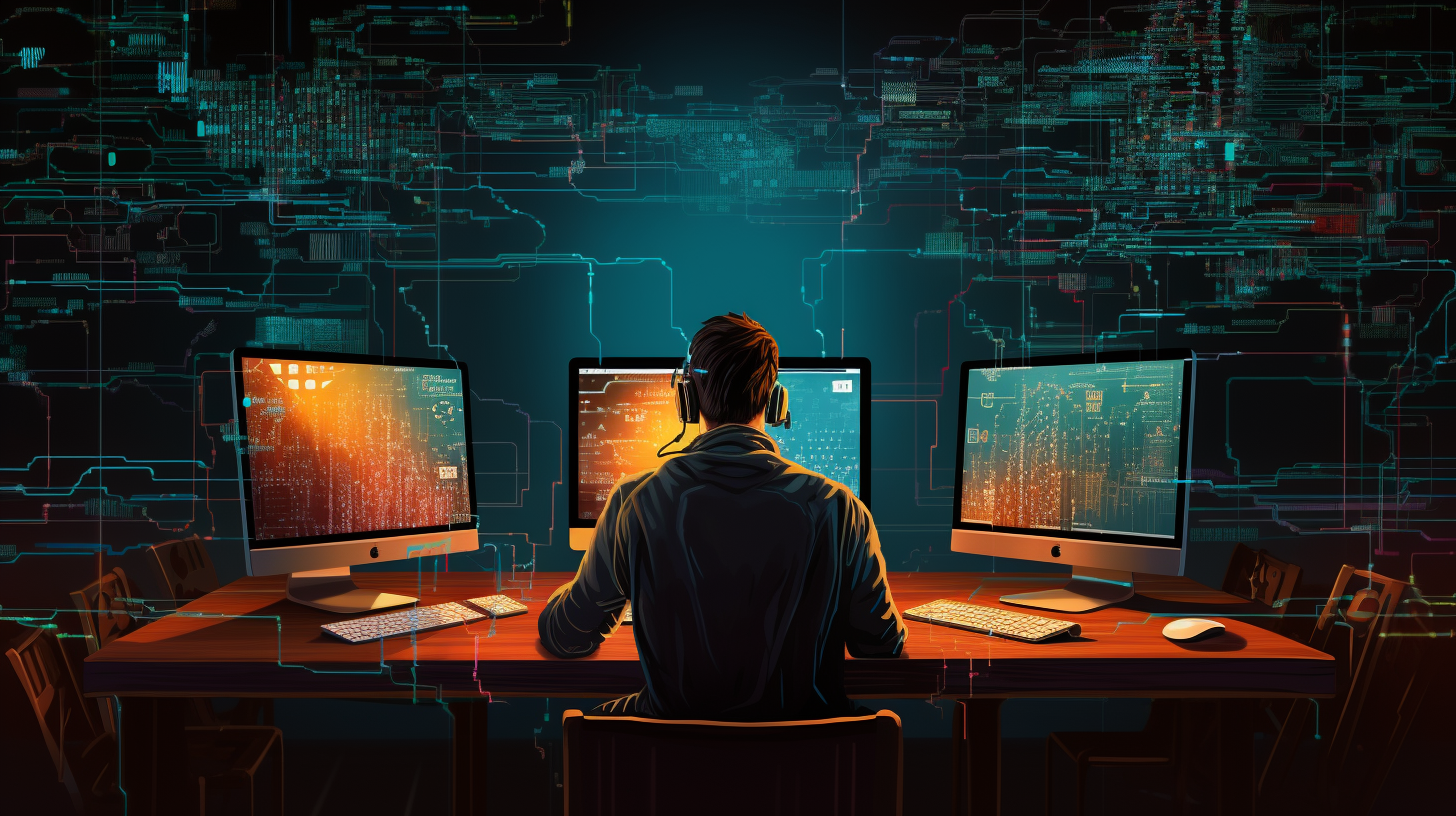
JavaScript and Personal Digital Assistants
JavaScript has emerged as a cornerstone technology in the development of Personal Digital Assistants (PDAs). Its flexibility and dynamism enable developers to create responsive and interactive applications that enhance user experience. JavaScript runs natively in web browsers, making it particularly well-suited for cross-platform development. This allows PDAs to operate seamlessly across various devices, from smartphones to tablets, ensuring that users have consistent access to their digital assistants regardless of their hardware.
One of the most significant roles of JavaScript in PDAs is its ability to facilitate real-time communication between the user and the assistant. By using asynchronous capabilities, JavaScript can handle multiple tasks at the same time, such as fetching data from APIs, processing user input, and updating the user interface without causing interruptions. This non-blocking nature leads to a smoother experience, which is essential for applications that rely on instant feedback.
Moreover, JavaScript enables the integration of various libraries and frameworks that further enhance the functionality of digital assistants. For instance, using libraries like React or Vue.js allows for the creation of dynamic user interfaces that can respond to user interactions in real time. This very important for PDAs, as users often expect immediate responses to their queries.
const assistantResponse = async (userInput) => { try { const response = await fetch(`https://api.digitalassistant.com/query?input=${userInput}`); const data = await response.json(); displayResponse(data.answer); } catch (error) { console.error('Error fetching data:', error); } }; const displayResponse = (response) => { document.getElementById('response').innerText = response; };
This code snippet illustrates how JavaScript can handle user input and dynamically display responses from a digital assistant’s backend. The function assistantResponse
is asynchronous, ensuring that the user interface remains responsive while waiting for the API call to complete.
Furthermore, JavaScript plays an important role in integrating third-party services, enhancing the capabilities of PDAs. For example, by using JavaScript to interact with external APIs for weather, traffic, or news, PDAs can provide up-to-date information tailored to the user’s needs. This level of personalization is essential for creating a truly intelligent assistant that can anticipate users’ requirements.
JavaScript’s versatility and power make it an ideal choice for developing personal digital assistants. Its capability to manage real-time data, integrate with various services, and create dynamic interfaces positions JavaScript at the forefront of PDA technology, driving innovation and enhancing user satisfaction.
Integrating Voice Recognition Technologies
As digital assistants evolve, integrating voice recognition technologies becomes a paramount focus for developers. JavaScript, with its robust ecosystem and support for various libraries, provides the tools necessary to implement effective voice recognition in PDAs. Using Web Speech API, developers can build applications that allow users to interact with their digital assistants through voice commands, transforming the way users engage with technology.
The Web Speech API consists of two main components: the Speech Recognition API, which allows speech input to be recognized, and the Speech Synthesis API, enabling the assistant to respond with spoken words. This capability not only enriches user interaction but also makes technology more accessible to a wider audience, including those with disabilities.
Implementing voice recognition in a PDA application involves setting up a speech recognition instance and defining event handlers to process the recognized speech. The following example demonstrates how to initiate speech recognition and handle the recognized results:
const recognition = new (window.SpeechRecognition || window.webkitSpeechRecognition)(); recognition.onstart = () => { console.log('Voice recognition started. Speak now!'); }; recognition.onresult = (event) => { const transcript = event.results[0][0].transcript; console.log('Recognized speech:', transcript); processUserInput(transcript); }; recognition.onerror = (event) => { console.error('Error occurred in recognition: ', event.error); }; const startRecognition = () => { recognition.start(); }; const processUserInput = (input) => { // Here we can call the assistantResponse function to handle the input assistantResponse(input); };
In this snippet, a new instance of SpeechRecognition is created, and event handlers are defined to log recognized speech and handle errors. The startRecognition function can be linked to a button in the user interface, allowing users to initiate voice input easily.
Once the speech is recognized, it is processed by the assistantResponse function, which communicates with the backend to fetch relevant information and deliver a response. This seamless integration of voice recognition elevates the user experience, allowing for a more natural interaction with the digital assistant.
Furthermore, combining voice recognition with JavaScript’s capabilities allows developers to create features such as voice commands for controlling smart home devices or retrieving information hands-free. This not only makes the digital assistant more functional but also aligns with the growing trend of voice-first technology.
Incorporating voice recognition into PDAs using JavaScript not only enhances user engagement but also broadens the scope of what digital assistants can achieve, paving the way for a future where voice commands are as commonplace as text input.
Enhancing User Interaction with JavaScript
Enhancing user interaction with JavaScript goes beyond simple input and output mechanisms; it encompasses creating an engaging environment where users feel empowered as they interact with their digital assistants. One of the critical aspects of achieving that is through responsive design and interactive feedback mechanisms that JavaScript enables. By using JavaScript’s event-driven capabilities, developers can create rich user experiences that respond to user actions in real time.
Consider the use of subtle animations and transitions to provide feedback when a user interacts with the digital assistant. These elements not only make the interface visually appealing but also guide the user through their interactions. For instance, changing button states or providing visual cues when a command is recognized can significantly enhance the user’s experience. Here’s a simple code example demonstrating how to implement button feedback using JavaScript:
const button = document.getElementById('submit-button'); button.addEventListener('click', () => { button.classList.add('active'); // Simulate waiting for response setTimeout(() => { button.classList.remove('active'); }, 200); });
This snippet adds an ‘active’ class to a button when it’s clicked, providing immediate visual feedback to the user. After a short delay, the class is removed to revert the button to its original state. Such feedback reinforces the user’s actions and keeps them informed about the assistant’s processing state.
In addition to visual feedback, sound effects can also play a pivotal role in enhancing interactions. JavaScript can easily integrate audio feedback, such as confirmation sounds when a task is completed or alert tones for errors. This multi-sensory feedback approach can help users feel more connected to their digital assistants.
const playSound = (soundFile) => { const audio = new Audio(soundFile); audio.play(); }; // Example of playing a sound on successful command processing if (commandProcessedSuccessfully) { playSound('success.mp3'); } else { playSound('error.mp3'); }
JavaScript also allows for the development of customizable user interfaces that adapt based on user preferences and behaviors. By tracking user interactions and modifying the display accordingly, developers can create a more personalized environment. A common technique involves using local storage to save user settings or preferences:
const saveUserPreference = (key, value) => { localStorage.setItem(key, value); }; const loadUserPreference = (key) => { return localStorage.getItem(key); }; // Example usage saveUserPreference('theme', 'dark'); const userTheme = loadUserPreference('theme'); document.body.classList.toggle('dark-theme', userTheme === 'dark');
In this example, user preferences for a theme are stored in local storage, allowing for a consistent experience across sessions. This personalization elevates the interaction by tailoring the interface to the user’s liking, making it feel more intuitive and responsive.
Moreover, incorporating JavaScript libraries such as jQuery or GSAP can simplify the implementation of complex animations and interactions. They provide a wealth of pre-built functions and capabilities that enhance the interactive components of digital assistants, allowing developers to focus on crafting a compelling user experience rather than getting bogged down in the details of CSS and JavaScript intricacies.
Ultimately, the goal of enhancing user interaction with JavaScript in digital assistants is to create a seamless, engaging, and responsive environment. By integrating effective feedback mechanisms, personalizing user experiences, and using powerful libraries, developers can build PDAs that not only serve functional purposes but also delight users with their interactivity and responsiveness.
Data Management and Personalization
Data management and personalization stand out as pivotal elements in developing effective Personal Digital Assistants (PDAs). The ability of a digital assistant to understand and respond to user needs relies heavily on how well it can manage data and tailor interactions based on individual preferences. JavaScript provides a robust framework for handling and processing user data in real-time, which is important for delivering personalized experiences.
At the core of data management in PDAs is the concept of collecting and analyzing user data to enhance interactions. JavaScript can access various data sources, whether local or remote, allowing developers to create a more responsive and personalized assistant. One common technique is to store user preferences and interaction history in local storage, enabling the assistant to learn from past interactions and refine its responses accordingly.
const userPreferences = { language: 'en', theme: 'light', favoriteCommands: [] }; const saveUserPreferences = () => { localStorage.setItem('userPreferences', JSON.stringify(userPreferences)); }; const loadUserPreferences = () => { const preferences = JSON.parse(localStorage.getItem('userPreferences')); if (preferences) { Object.assign(userPreferences, preferences); } }; // Save and load preferences saveUserPreferences(); loadUserPreferences();
This code snippet demonstrates how JavaScript can be utilized to manage user preferences by saving them in the browser’s local storage. By retrieving this data whenever the assistant starts, it can adjust its behavior and interface based on established preferences, enhancing user satisfaction through personalization.
Furthermore, JavaScript enables the integration of external data sources, such as APIs, that provide relevant information tailored to the user’s context. For example, if a user frequently asks about the weather, a digital assistant can fetch live weather data from a third-party API and present it in a personalized manner. Here’s how you might implement this:
const fetchWeatherData = async (location) => { try { const response = await fetch(`https://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q=${location}`); const data = await response.json(); displayWeather(data); } catch (error) { console.error('Error fetching weather data:', error); } }; const displayWeather = (data) => { const weatherInfo = `Current temperature in ${data.location.name}: ${data.current.temp_c}°C`; document.getElementById('weather').innerText = weatherInfo; }; // Example usage fetchWeatherData('New York');
This example showcases how to retrieve weather data using JavaScript and dynamically display it in the user interface. By customizing the request based on user location or preferences, the assistant becomes capable of delivering highly relevant information, thereby enriching the interaction.
Another essential aspect of data management in PDAs is ensuring user privacy and data security. JavaScript can implement various measures to protect user data, such as encryption and secure transmission protocols. For instance, when sending user data to a server, developers can utilize the Fetch API to ensure communication is conducted securely:
const sendUserData = async (userData) => { try { const response = await fetch('https://api.digitalassistant.com/userdata', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify(userData), }); const result = await response.json(); console.log('User data sent successfully:', result); } catch (error) { console.error('Error sending user data:', error); } }; // Example usage const userData = { name: 'John', preferences: userPreferences }; sendUserData(userData);
By implementing secure data handling methods, developers can build trust with users, emphasizing the importance of privacy in the digital assistant experience.
The ability to analyze user data not only facilitates personalization but also enables predictive features. By employing machine learning algorithms alongside JavaScript, developers can create assistants that anticipate user needs based on historical interactions. This proactive approach to assistance can significantly enhance user engagement and satisfaction.
Ultimately, JavaScript’s capabilities in data management and personalization empower developers to create intelligent, responsive digital assistants. By using effective data storage, integrating external APIs, securing user information, and using predictive analytics, PDAs can deliver tailored experiences that resonate with users, making technology more intuitive and accessible.
Future Trends in JavaScript for Digital Assistants
As we gaze into the future of Personal Digital Assistants (PDAs), the role of JavaScript is poised to expand dramatically. Emerging trends indicate a shift towards more intelligent, responsive, and integrated digital assistants, driven by advancements in both technology and user expectations. As JavaScript continues to evolve, it will undoubtedly adapt to meet these demands, ensuring that PDAs become increasingly capable and intuitive.
One prominent trend is the move towards more sophisticated natural language processing (NLP). As NLP technologies improve, JavaScript will serve as a vital tool for creating interfaces that can interpret and respond to nuanced user queries. By using libraries and APIs designed for NLP, developers can enable PDAs to understand context, sentiment, and even subtleties in conversation, leading to more meaningful interactions.
const nlpResponse = async (userInput) => { const nlpAPI = 'https://api.nlp-service.com/analyze'; try { const response = await fetch(nlpAPI, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ text: userInput }), }); const data = await response.json(); handleNLPResponse(data); } catch (error) { console.error('Error in NLP processing:', error); } };
This example shows how JavaScript can send a user input to an NLP service for analysis, showcasing the potential for enhanced understanding and response generation in PDAs.
Another critical trend is the integration of AI and machine learning capabilities directly into JavaScript frameworks. With the rise of libraries such as TensorFlow.js, developers can implement machine learning models directly in the browser or on serverless platforms. This capability allows PDAs to learn from user interactions and improve over time without relying solely on external servers.
import * as tf from '@tensorflow/tfjs'; const model = await tf.loadLayersModel('path/to/model.json'); const predictUserIntent = async (userInput) => { const inputTensor = tf.tensor2d([userInputData]); const prediction = model.predict(inputTensor); const intent = prediction.argMax(-1).dataSync()[0]; return intent; };
This code snippet illustrates how a machine learning model can be used to predict user intent, enhancing the assistant’s ability to respond appropriately based on learned patterns.
Moreover, the future of PDAs will see a significant emphasis on privacy and data security. As users become more aware of their digital footprints, JavaScript will play a critical role in implementing privacy-first design principles. Techniques such as client-side encryption and secure data handling will become standard practices, ensuring that user interactions with digital assistants remain confidential.
const encryptData = (data) => { const encrypted = btoa(JSON.stringify(data)); // Simple base64 encoding for demonstration return encrypted; }; const secureSendData = async (userData) => { const encryptedData = encryptData(userData); // Send encrypted data... };
This example demonstrates a rudimentary approach to encrypting data before transmission, highlighting the importance of safeguarding user information in the evolving landscape of PDAs.
Finally, the future of JavaScript in PDAs will likely involve deeper integration with IoT devices, allowing for seamless control and interaction across multiple platforms. By using APIs that connect with smart home devices, wearables, and other connected technologies, developers can create comprehensive ecosystems where digital assistants serve as the central hub for managing user environments.
const controlSmartDevice = async (deviceId, action) => { const apiUrl = `https://api.smartdevice.com/control`; try { const response = await fetch(apiUrl, { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ id: deviceId, command: action }), }); const result = await response.json(); handleDeviceResponse(result); } catch (error) { console.error('Error controlling device:', error); } };
This snippet demonstrates how JavaScript can be utilized to send commands to smart devices, showcasing the potential for holistic user experiences within the smart ecosystem.
As we look toward the horizon of digital assistants, JavaScript is set to drive innovation through enhanced natural language processing, integrated AI, robust privacy measures, and comprehensive IoT connectivity. With these advancements, PDAs will not only become more efficient but also more aligned with human behaviors and expectations, revolutionizing the way we interact with technology.