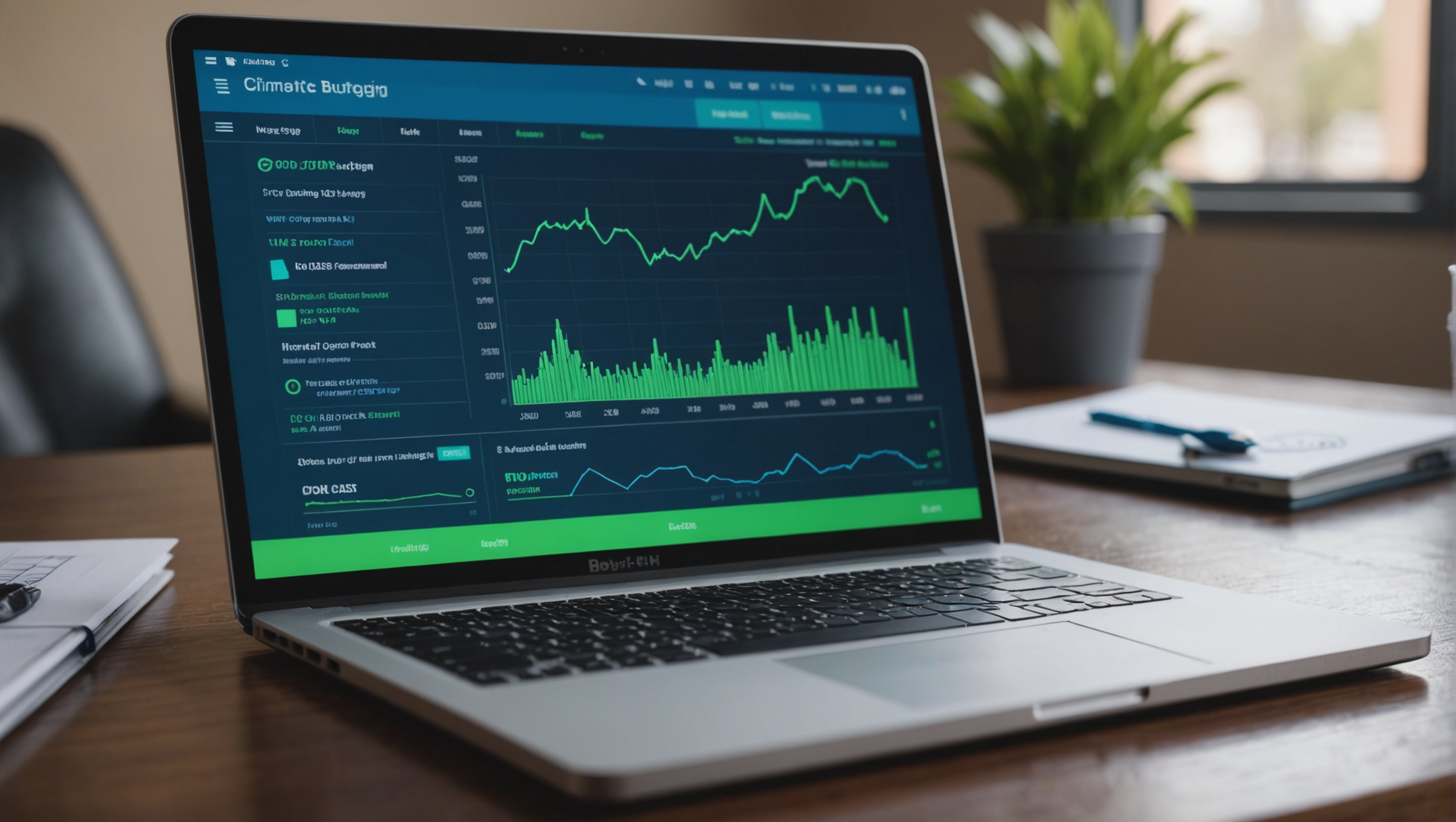
JavaScript and Personal Finance Tools
When it comes to managing personal finances, budgeting is a cornerstone activity. JavaScript, with its versatility and ease of integration into web applications, provides the perfect toolkit for building effective budgeting applications. These applications allow users to track their income, expenses, and savings in real-time, offering insights that can significantly improve financial decision-making.
To start developing a basic budgeting application, one can leverage JavaScript to create an interactive interface that captures user inputs such as income and expenditure. Using the Document Object Model (DOM) allows for seamless updates of the budget display without requiring page reloads.
const budget = { income: 0, expenses: 0, savings: 0, updateIncome(newIncome) { this.income = newIncome; this.calculateSavings(); }, updateExpenses(newExpenses) { this.expenses = newExpenses; this.calculateSavings(); }, calculateSavings() { this.savings = this.income - this.expenses; this.displayBudget(); }, displayBudget() { document.getElementById('income').textContent = this.income; document.getElementById('expenses').textContent = this.expenses; document.getElementById('savings').textContent = this.savings; } }; // Example usage: budget.updateIncome(5000); budget.updateExpenses(2000);
In the above example, we define a simple budget object that handles income, expenses, and savings. By encapsulating related functionalities within this object, we ensure a clean and maintainable codebase. Whenever the user updates their income or expenses, the respective methods are called, recalculating the savings and updating the UI accordingly.
For an enhanced user experience, incorporating event listeners can be beneficial. By reacting to user inputs dynamically, the application can provide immediate feedback. Here’s how you can add input fields for users to enter their income and expenses:
document.getElementById('incomeInput').addEventListener('change', (event) => { const newIncome = parseFloat(event.target.value) || 0; budget.updateIncome(newIncome); }); document.getElementById('expensesInput').addEventListener('change', (event) => { const newExpenses = parseFloat(event.target.value) || 0; budget.updateExpenses(newExpenses); });
This code snippet listens for changes in the input fields and updates the budget object accordingly. Notice how the use of parseFloat
allows us to handle non-numeric inputs gracefully by defaulting to zero.
In addition to basic functionalities, incorporating features such as monthly expense categorization can provide deeper insights into spending habits. By extending the budget object to include categories, users can track where their money goes, enabling more informed financial decisions.
budget.categories = {}; budget.addExpense = function(category, amount) { if (!this.categories[category]) { this.categories[category] = 0; } this.categories[category] += amount; this.updateExpenses(this.totalExpenses()); }; budget.totalExpenses = function() { return Object.values(this.categories).reduce((acc, val) => acc + val, 0); }; // Example usage: budget.addExpense("Groceries", 150); budget.addExpense("Entertainment", 100);
This additional layer of functionality allows users to categorize their expenses, making it easier to see which areas may need adjustment. With JavaScript at the helm, the possibilities for creating a robust budgeting application are virtually limitless.
Building Investment Trackers with JavaScript
Building an investment tracker with JavaScript follows a similar philosophy to creating a budgeting application, but with a focus on managing assets, monitoring market trends, and calculating potential returns. An investment tracker enables users to input their investments, view their performance over time, and make data-driven decisions about their financial future. By using JavaScript, developers can create a responsive and interactive tool that provides real-time updates on investment performance.
To begin, the core functionality of an investment tracker can be encapsulated within an object that maintains a list of investments, their corresponding values, and the ability to calculate total returns. Here’s a foundational setup:
const investmentTracker = { investments: [], addInvestment(name, amount, currentValue) { this.investments.push({ name, amount, currentValue }); }, calculateTotalValue() { return this.investments.reduce((total, investment) => total + investment.currentValue, 0); }, calculateTotalReturns() { return this.investments.reduce((total, investment) => total + (investment.currentValue - investment.amount), 0); }, displayInvestments() { const investmentList = document.getElementById('investmentList'); investmentList.innerHTML = ''; this.investments.forEach(investment => { const investmentItem = document.createElement('li'); investmentItem.textContent = `${investment.name}: $${investment.currentValue} (Original: $${investment.amount})`; investmentList.appendChild(investmentItem); }); this.updateTotals(); }, updateTotals() { document.getElementById('totalValue').textContent = this.calculateTotalValue(); document.getElementById('totalReturns').textContent = this.calculateTotalReturns(); } }; // Example usage: investmentTracker.addInvestment("Tech Stocks", 1000, 1500); investmentTracker.addInvestment("Real Estate", 50000, 55000); investmentTracker.displayInvestments();
The above code establishes an investment tracker object with methods to add investments, calculate total values and returns, and display the investments in the UI. The use of the reduce method allows for concise calculations of the total value and returns from the investments array.
To make this functionality interactive, we can create a simple form for users to input their investment details. Each time they add a new investment, we can update the display. Here’s how you might set up the event listeners for a form:
document.getElementById('addInvestmentForm').addEventListener('submit', (event) => { event.preventDefault(); const name = event.target.elements['investmentName'].value; const amount = parseFloat(event.target.elements['investmentAmount'].value) || 0; const currentValue = parseFloat(event.target.elements['currentValue'].value) || 0; investmentTracker.addInvestment(name, amount, currentValue); investmentTracker.displayInvestments(); });
This snippet listens for the submission of an investment form, retrieves user inputs, and updates the investment tracker accordingly. It allows for dynamic interaction with the investment data without requiring a page refresh, providing instant feedback to the user.
In addition, an investment tracker can benefit from integrating real-time market data through APIs, allowing users to see live performance updates. For example, by using the Fetch API, developers can pull current stock prices or cryptocurrency values dynamically. Here’s a rudimentary example of how you might implement this:
async function fetchCurrentValue(stockSymbol) { const response = await fetch(`https://api.example.com/stock/${stockSymbol}`); const data = await response.json(); return data.currentPrice; } // Example usage: fetchCurrentValue('AAPL').then(currentValue => { investmentTracker.addInvestment("Apple Stocks", 1200, currentValue); investmentTracker.displayInvestments(); });
In this example, an asynchronous function fetches the current stock price from an API and updates the investment tracker with this value. This integration of real-time data not only enriches the user experience but also enhances the accuracy of the investment tracking process.
With these capabilities, an investment tracker built with JavaScript can become a powerful personal finance tool, enabling users to monitor their investments, analyze performance, and make informed decisions based on current market data. The flexibility and responsiveness of JavaScript empower developers to create robust applications that adapt to the evolving needs of their users.
Creating Interactive Financial Calculators
Creating interactive financial calculators using JavaScript can significantly enhance the user’s understanding of their financial situation. These calculators can help users estimate loan payments, calculate savings growth, or project investment returns, all based on user-defined variables. By delivering real-time feedback, these calculators empower users with immediate insights, allowing them to make informed decisions without complex calculations.
To develop a loan payment calculator, we can start by establishing a function that calculates the monthly payment based on the loan amount, interest rate, and loan term. Here’s a simple example:
function calculateLoanPayment(principal, annualRate, years) { const monthlyRate = annualRate / 100 / 12; const numberOfPayments = years * 12; const monthlyPayment = (principal * monthlyRate) / (1 - Math.pow(1 + monthlyRate, -numberOfPayments)); return monthlyPayment.toFixed(2); } // Example usage: const loanAmount = 20000; // Principal amount const interestRate = 5; // Annual interest rate in percentage const loanTerm = 5; // Loan term in years const payment = calculateLoanPayment(loanAmount, interestRate, loanTerm); console.log(`Monthly Payment: $${payment}`);
This function uses the formula for monthly payments on an amortizing loan, which is essential for users looking to understand how much they will owe each month. The function takes three parameters: the principal amount, the annual interest rate, and the number of years for the loan. The resulting payment is formatted to two decimal places for clarity.
To make this calculator interactive, we can set up an HTML form where users can input their loan details, and then attach an event listener to handle the calculation:
document.getElementById('loanCalculatorForm').addEventListener('submit', (event) => { event.preventDefault(); const principal = parseFloat(event.target.elements['principal'].value) || 0; const annualRate = parseFloat(event.target.elements['annualRate'].value) || 0; const years = parseInt(event.target.elements['years'].value) || 0; const monthlyPayment = calculateLoanPayment(principal, annualRate, years); document.getElementById('result').textContent = `Monthly Payment: $${monthlyPayment}`; });
The above code sets up an event listener for the form submission, preventing the default form action. It retrieves values entered by the user, invokes the calculateLoanPayment
function, and updates the UI with the calculated monthly payment.
Beyond loan calculations, we can also create a savings calculator to project future savings based on monthly contributions and interest rates. Here’s a basic implementation:
function calculateFutureSavings(initialAmount, monthlyContribution, annualRate, years) { let futureValue = initialAmount; const monthlyRate = annualRate / 100 / 12; const numberOfPayments = years * 12; for (let i = 0; i < numberOfPayments; i++) { futureValue = futureValue * (1 + monthlyRate) + monthlyContribution; } return futureValue.toFixed(2); } // Example usage: const initialAmount = 5000; // Initial savings const monthlyContribution = 500; // Monthly contribution const savingsRate = 4; // Annual interest rate const savingsYears = 10; // Time in years const futureValue = calculateFutureSavings(initialAmount, monthlyContribution, savingsRate, savingsYears); console.log(`Future Savings: $${futureValue}`);
This function iterates over the number of months, compounding interest monthly and adding the contributions. This loop provides users with a realistic projection of their savings growth over time.
Just like with the loan calculator, we can create an interactive form for users to input their savings parameters, thereby prompting a calculation based on their entries:
document.getElementById('savingsCalculatorForm').addEventListener('submit', (event) => { event.preventDefault(); const initialAmount = parseFloat(event.target.elements['initialAmount'].value) || 0; const monthlyContribution = parseFloat(event.target.elements['monthlyContribution'].value) || 0; const annualRate = parseFloat(event.target.elements['annualRate'].value) || 0; const years = parseInt(event.target.elements['years'].value) || 0; const futureValue = calculateFutureSavings(initialAmount, monthlyContribution, annualRate, years); document.getElementById('savingsResult').textContent = `Future Savings: $${futureValue}`; });
Incorporating these interactive financial calculators into a web application not only enhances user engagement but also serves as a valuable resource for individuals aiming to comprehend their financial landscape better. By using JavaScript’s capabilities, developers can create tools tailored to the unique needs of users, fostering a deeper understanding of personal finance.
Integrating APIs for Real-Time Financial Data
Integrating APIs into financial applications can elevate their functionality, providing users with real-time data that influences their financial decisions. JavaScript, with its asynchronous capabilities, is particularly well-suited for this task, allowing developers to fetch and utilize data from various external sources without interrupting the user experience.
When building a financial application, one of the most valuable types of data to integrate is real-time market information. For instance, an investment tracker can benefit greatly from live stock prices or cryptocurrency values. By using APIs, developers can keep users informed about the latest trends and changes in their investments.
Here’s a simple example of how to use the Fetch API to retrieve stock prices:
async function fetchStockPrice(symbol) { const response = await fetch(`https://api.example.com/stock/${symbol}`); if (!response.ok) { throw new Error(`Error fetching stock data: ${response.statusText}`); } const data = await response.json(); return data.currentPrice; } // Example usage: fetchStockPrice('AAPL').then(price => { console.log(`Current price of AAPL: $${price}`); }).catch(error => { console.error(error); });
In this example, the fetchStockPrice function sends a request to a hypothetical API endpoint to retrieve the current stock price for a given symbol. The response is checked for errors and then parsed into JSON format. Using async/await allows clean handling of asynchronous operations, making the code more readable. When invoking this function, you can handle the result or any potential errors seamlessly, ensuring that the user experience remains robust.
Moreover, you can integrate multiple APIs to diversify the data you present to users. For instance, if you want to provide both stock data and economic indicators, you could do something like this:
async function fetchFinancialData(stockSymbol, economicIndicator) { const stockResponse = fetch(`https://api.example.com/stock/${stockSymbol}`); const indicatorResponse = fetch(`https://api.example.com/economic/${economicIndicator}`); const [stockData, indicatorData] = await Promise.all([stockResponse, indicatorResponse]); if (!stockData.ok || !indicatorData.ok) { throw new Error('Error fetching financial data'); } const stockPrice = await stockData.json(); const economicData = await indicatorData.json(); return { stockPrice: stockPrice.currentPrice, economicIndex: economicData.indexValue, }; } // Example usage: fetchFinancialData('AAPL', 'GDP').then(data => { console.log(`AAPL Price: $${data.stockPrice}, GDP Index: ${data.economicIndex}`); }).catch(error => { console.error(error); });
This fetchFinancialData function retrieves both stock prices and economic indicators at once using Promise.all, allowing both requests to be sent in parallel. This method not only reduces loading time but also provides users with comprehensive financial insights simultaneously.
For real-time updates, ponder using WebSocket connections if your API supports them. This method allows you to receive data updates live without polling the server continuously. Here’s a basic implementation:
const socket = new WebSocket('wss://api.example.com/realtime'); socket.onopen = () => { console.log('Connected to WebSocket'); socket.send(JSON.stringify({ type: 'subscribe', symbol: 'AAPL' })); }; socket.onmessage = (event) => { const message = JSON.parse(event.data); console.log(`Updated price for ${message.symbol}: $${message.price}`); }; socket.onerror = (error) => { console.error(`WebSocket error: ${error.message}`); }; socket.onclose = () => { console.log('WebSocket connection closed'); };
With this WebSocket setup, users will receive real-time updates on stock prices as they occur, enhancing the interactivity and responsiveness of the application. The integration of APIs, whether through RESTful calls or WebSocket connections, transforms a static financial tool into a dynamic, user-responsive application.
Using JavaScript to integrate APIs offers the potential to create more informed and engaged users, as they can access the latest financial data and trends with ease. This not only fosters trust in the application but also empowers users to make better financial decisions based on real-time information.