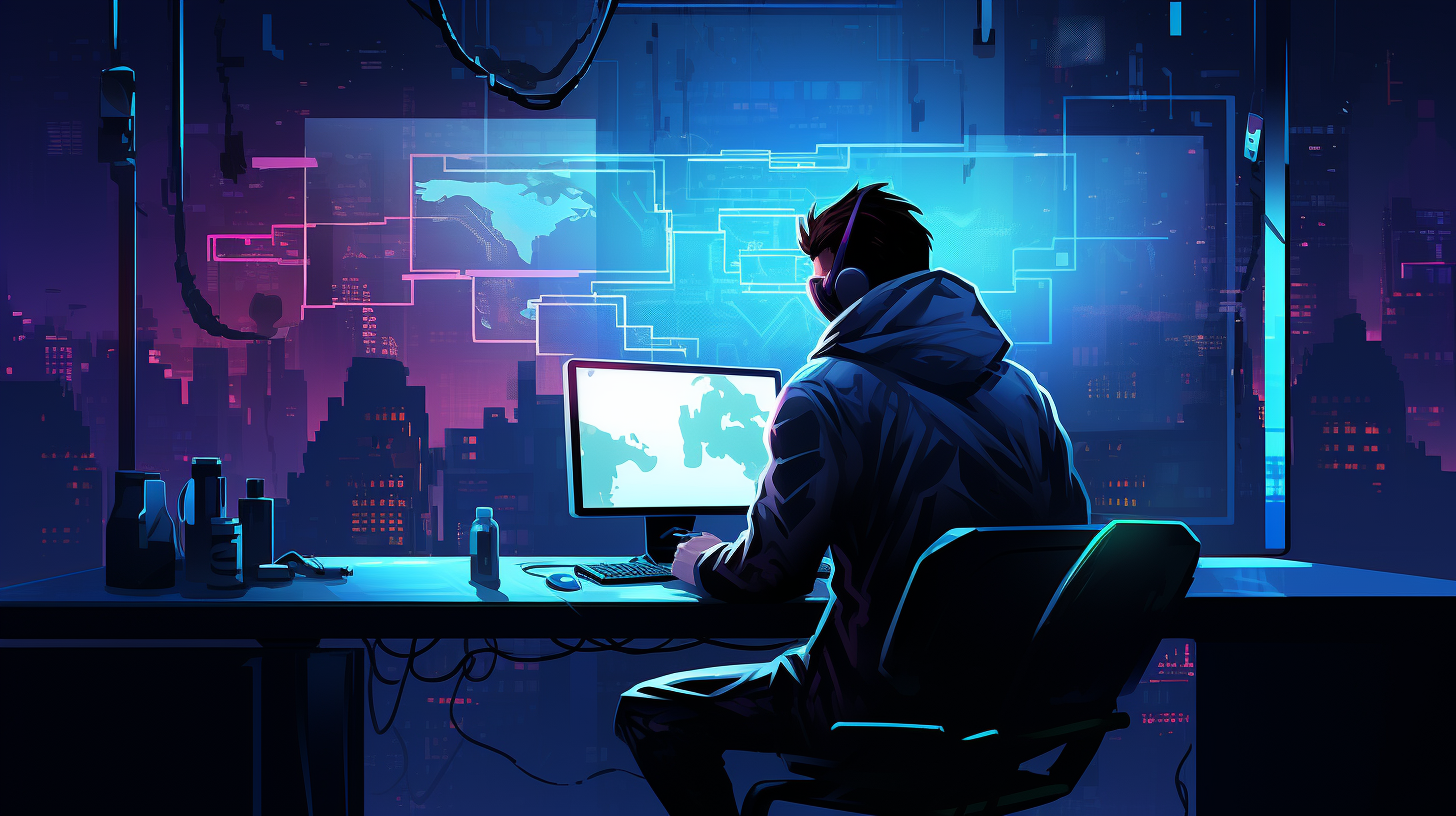
JavaScript and Recipe Websites
In the landscape of modern recipe websites, JavaScript serves as the backbone that transforms static content into a dynamic, engaging experience for users. With the increasing demand for interactivity, JavaScript has become essential in providing functionality that enhances the way users interact with culinary content.
JavaScript enables a seamless integration of features that allow users to not only read recipes but also engage with them. For example, a simple JavaScript function can create an interactive checklist for users as they prepare their dishes. This fosters a sense of involvement and helps users keep track of their progress while cooking.
function toggleChecklist(item) { item.classList.toggle('checked'); }
Moreover, JavaScript plays a pivotal role in managing user inputs and preferences. By capturing data on user behavior, recipe websites can personalize the content that is presented to each visitor. For instance, if a user frequently searches for vegan recipes, JavaScript can dynamically update the homepage to showcase vegan options, using localStorage to retain user preferences across sessions.
function saveUserPreference(preference) { localStorage.setItem('recipePreference', preference); }
Another crucial aspect of JavaScript in recipe websites is the implementation of interactive features like timers and ingredient scaling. A JavaScript-based timer can enhance the cooking experience by allowing users to know exactly how long they need to wait for each step of the recipe, minimizing the risk of overcooking or undercooking.
let timer; function startTimer(duration) { let time = duration, minutes, seconds; timer = setInterval(() => { minutes = parseInt(time / 60, 10); seconds = parseInt(time % 60, 10); seconds = seconds < 10 ? "0" + seconds : seconds; console.log(minutes + ":" + seconds); if (--time < 0) { clearInterval(timer); } }, 1000); }
Finally, the power of JavaScript lies in its ability to create a responsive and adaptive user interface. By using frameworks and libraries like React or Vue.js, developers can craft interfaces that respond fluidly to user interactions, ensuring that recipes are not only easy to follow but also visually appealing and accessible across various devices.
In essence, JavaScript is the secret ingredient that elevates recipe websites from mere collections of cooking instructions to vibrant, interactive platforms that cater to the needs and preferences of modern users. Its ability to combine functionality with a user-centric design makes it an indispensable tool for anyone looking to create an engaging culinary experience online.
Enhancing User Experience with Interactive Features
One of the most appealing aspects of modern recipe websites is the ability to create a highly interactive environment. JavaScript enables developers to implement features that allow users to manipulate content in real-time, enhancing their cooking journey. For instance, a common interactive feature is ingredient scaling, which allows users to adjust serving sizes with a simple input field. This not only empowers users but also reduces food waste by helping them prepare the right amount of food.
function scaleIngredients(scaleFactor) { const ingredients = document.querySelectorAll('.ingredient'); ingredients.forEach(ingredient => { const originalAmount = parseFloat(ingredient.dataset.amount); ingredient.textContent = (originalAmount * scaleFactor).toFixed(2) + ' ' + ingredient.dataset.unit; }); }
Additionally, implementing interactive sliders for portion sizes can significantly improve user engagement. By adjusting a slider, users can see the ingredient amounts change in real-time. This visual feedback reinforces the users’ sense of control over their cooking process, making it not just a task, but an enjoyable experience.
const slider = document.getElementById('portion-slider'); slider.addEventListener('input', function() { const scaleFactor = this.value; scaleIngredients(scaleFactor); });
JavaScript can also enhance the overall aesthetics of recipe websites through animations and transitions. For example, as users navigate through different recipe steps, smooth transitions can be implemented to guide them through the cooking process. This reduces cognitive load by presenting information in a digestible manner. Libraries like GSAP (GreenSock Animation Platform) can be utilized to create eye-catching animations that make the cooking process feel dynamic and exciting.
gsap.from('.recipe-step', {duration: 0.5, opacity: 0, y: -50, stagger: 0.2});
Furthermore, implementing interactive quizzes or polls can engage users by allowing them to share their cooking experiences or preferences. Such features not only foster community engagement but also provide valuable feedback that can help tailor the content to better suit user needs.
document.getElementById('submit-poll').addEventListener('click', function() { const selectedOption = document.querySelector('input[name="recipe-feedback"]:checked').value; alert('Thank you for your feedback: ' + selectedOption); });
All of these interactive features not only improve the user experience but also increase the likelihood of users returning to the website. By creating a sense of community and engagement, recipe websites can transform the act of cooking into a shared adventure, making every user feel like they’re a part of something bigger.
Dynamic Recipe Filtering and Search Capabilities
Dynamic recipe filtering and search capabilities are pivotal in enhancing the user experience on modern recipe websites. With a plethora of recipes available online, users are often overburdened with the choices presented to them. JavaScript empowers developers to implement sophisticated filtering and search functionalities that allow users to quickly find recipes that match their preferences, dietary restrictions, and cooking skills.
One of the most effective ways to implement dynamic filtering is through the use of event listeners that respond to user input. For example, ponder a scenario where users want to filter recipes based on specific criteria such as cuisine type, preparation time, or main ingredients. By using JavaScript, we can create a responsive interface that updates the displayed recipes in real-time as users make selections from dropdown menus or checkboxes.
const filterRecipes = () => { const selectedCuisine = document.querySelector('select[name="cuisine"]').value; const selectedTime = document.querySelector('select[name="prep-time"]').value; const recipes = document.querySelectorAll('.recipe-card'); recipes.forEach(recipe => { const matchesCuisine = recipe.dataset.cuisine.includes(selectedCuisine) || selectedCuisine === 'all'; const matchesTime = recipe.dataset.time <= selectedTime || selectedTime === 'all'; recipe.style.display = (matchesCuisine && matchesTime) ? 'block' : 'none'; }); }; document.querySelector('select[name="cuisine"]').addEventListener('change', filterRecipes); document.querySelector('select[name="prep-time"]').addEventListener('change', filterRecipes);
In the example above, the filterRecipes
function is called whenever a user changes their selection in the cuisine or preparation time dropdowns. Each recipe is evaluated against the selected filters, and only those that match the criteria are displayed. This real-time feedback ensures that users feel in control of their search, providing a more engaging experience.
Moreover, incorporating a search bar with autocomplete suggestions can significantly enhance the filtering process. JavaScript can be utilized to filter through the list of available recipes as users type, presenting them with relevant suggestions on-the-fly. This functionality not only saves users time but also reduces frustration, allowing them to find their desired recipes with minimal effort.
const searchRecipes = () => { const query = document.querySelector('input[name="search"]').value.toLowerCase(); const recipes = document.querySelectorAll('.recipe-card'); recipes.forEach(recipe => { const title = recipe.querySelector('.recipe-title').textContent.toLowerCase(); recipe.style.display = title.includes(query) ? 'block' : 'none'; }); }; document.querySelector('input[name="search"]').addEventListener('input', searchRecipes);
This searchRecipes
function evaluates the user’s input against the titles of the recipes, dynamically showing or hiding recipes based on the search term. Not only does this feature streamline the recipe discovery process, but it also encourages users to explore more content on the site, potentially increasing engagement and time spent browsing.
Additionally, to improve the filtering and search capabilities further, integrating tags or categories for each recipe can provide users with a comprehensive way to navigate through different culinary options. Using JavaScript to implement tag-based filtering allows users to click on tags that interest them, instantly updating the displayed recipes to show those that match the selected tags.
const tagFilter = (tag) => { const recipes = document.querySelectorAll('.recipe-card'); recipes.forEach(recipe => { const hasTag = recipe.dataset.tags.split(',').includes(tag); recipe.style.display = hasTag ? 'block' : 'none'; }); }; document.querySelectorAll('.tag').forEach(tag => { tag.addEventListener('click', () => { tagFilter(tag.textContent); }); });
Through these mechanisms, JavaScript not only simplifies the process of finding the right recipe for users but also transforms recipe websites into intuitive platforms that respond to the specific needs of their audience. The implementation of dynamic filtering and search functionalities ensures that users can navigate the culinary landscape with ease, thus enhancing their overall experience on the site.
Integrating APIs for Ingredient Substitutions
Integrating APIs for ingredient substitutions is a game-changing feature for modern recipe websites. It allows users to customize their culinary experiences, accommodating dietary restrictions, allergies, or simply the unavailability of certain ingredients. JavaScript plays an important role in this integration, enabling real-time suggestions and modifications that enhance user interaction and satisfaction.
Imagine a scenario where a user is cooking a recipe that calls for a specific ingredient, say, “buttermilk.” If the user doesn’t have buttermilk on hand, a well-implemented API can suggest suitable alternatives. For this functionality, developers can utilize a simple JavaScript function that queries a substitution API and updates the recipe in real-time based on user input.
const fetchSubstitutes = async (ingredient) => { const response = await fetch(`https://api.example.com/substitutes?ingredient=${ingredient}`); const data = await response.json(); return data.substitutes; }; const updateSubstitutions = async () => { const ingredientInput = document.getElementById('ingredient-input').value; const substitutes = await fetchSubstitutes(ingredientInput); displaySubstitutes(substitutes); }; const displaySubstitutes = (substitutes) => { const substitutesContainer = document.getElementById('substitutes-list'); substitutesContainer.innerHTML = ''; // Clear previous suggestions substitutes.forEach(sub => { const listItem = document.createElement('li'); listItem.textContent = sub; substitutesContainer.appendChild(listItem); }); }; document.getElementById('ingredient-input').addEventListener('input', updateSubstitutions);
This code snippet demonstrates how to fetch ingredient substitutes using an API and dynamically display them on the webpage. When a user types an ingredient into the input field, the `updateSubstitutions` function triggers an API call to retrieve possible substitutes. The results are then rendered in the `substitutes-list` container, providing immediate feedback to the user, which enhances the overall cooking experience.
Moreover, integrating APIs for nutritional information can further elevate the user experience. Consider a scenario where users want to know not just about ingredient substitutes but also their nutritional values. By using a nutritional API, recipe websites can display relevant information alongside ingredient substitutions. This empowers users to make informed decisions and align their cooking with their dietary goals.
const fetchNutritionalInfo = async (ingredient) => { const response = await fetch(`https://api.example.com/nutrition?ingredient=${ingredient}`); const data = await response.json(); return data.nutrition; }; const updateNutritionalInfo = async () => { const ingredientInput = document.getElementById('ingredient-input').value; const nutrition = await fetchNutritionalInfo(ingredientInput); displayNutrition(nutrition); }; const displayNutrition = (nutrition) => { const nutritionContainer = document.getElementById('nutrition-info'); nutritionContainer.innerHTML = `Calories: ${nutrition.calories}, Protein: ${nutrition.protein}g, Carbs: ${nutrition.carbs}g`; }; document.getElementById('ingredient-input').addEventListener('input', updateNutritionalInfo);
By incorporating nutritional information into the recipe workflow, developers can enhance the cooking experience significantly. This comprehensive approach not only caters to users’ immediate needs regarding ingredient substitutions but also provides valuable context about the food they’re preparing.
Furthermore, the synergy between JavaScript and APIs allows for robust error handling and user feedback. For instance, if a user inputs an ingredient that does not exist in the API’s database, JavaScript can provide a friendly message or suggest alternative actions, enhancing the overall user experience.
const updateSubstitutions = async () => { const ingredientInput = document.getElementById('ingredient-input').value; try { const substitutes = await fetchSubstitutes(ingredientInput); displaySubstitutes(substitutes); } catch (error) { console.error('Error fetching substitutes:', error); alert('Sorry, we could not find any substitutes for that ingredient.'); } };
This level of interactivity and responsiveness not only enriches the user experience but also fosters a sense of community among users who may share similar dietary restrictions or preferences. As recipe websites continue to evolve, the integration of APIs for ingredient substitutions stands out as an essential feature that turns cooking from a rigid task into a flexible and enjoyable journey.
Using JavaScript Frameworks for Responsive Design
In today’s digital age, the importance of responsive design cannot be overstated, particularly for recipe websites that cater to a wide audience with varying devices and screen sizes. Using JavaScript frameworks such as React, Vue.js, or Angular can dramatically improve the user experience by ensuring that content is displayed effectively, irrespective of the platform being used. These frameworks allow developers to create dynamic, responsive layouts that adjust to the user’s device, making recipes not only accessible but also visually appealing.
JavaScript frameworks excel in creating single-page applications (SPAs) where users can navigate through recipes without the need for constant page reloads. This capability is particularly beneficial for recipe websites, where users frequently browse multiple recipes. For instance, a recipe card can be dynamically loaded into the view as users scroll, providing a seamless experience that keeps them engaged.
const loadRecipe = async (recipeId) => { const response = await fetch(`https://api.example.com/recipes/${recipeId}`); const recipe = await response.json(); displayRecipe(recipe); }; const displayRecipe = (recipe) => { const recipeContainer = document.getElementById('recipe-container'); recipeContainer.innerHTML = `${recipe.title}
${recipe.description}
- ${recipe.ingredients.map(ing => `
- ${ing} `).join('')}
This exemplary code snippet illustrates how a recipe can be dynamically loaded and displayed using an asynchronous function to fetch data from an API. By doing so, users can click on recipe links, and the relevant information will appear without the jarring interruption of a full page reload. This approach enhances user satisfaction and encourages prolonged engagement with the content.
Moreover, JavaScript frameworks allow for the implementation of responsive design principles that adapt to various screen sizes. Using CSS-in-JS solutions such as styled-components in React not only allows developers to manage styles more effectively but also enables the creation of responsive layouts that change in real-time as the device size alters.
import styled from 'styled-components'; const RecipeCard = styled.div` width: 100%; max-width: 300px; margin: 10px; border: 1px solid #ddd; border-radius: 5px; padding: 10px; @media (max-width: 600px) { max-width: 100%; } `;
This styled-component dynamically adjusts the recipe card’s width based on the screen size, ensuring that it looks good on both mobile devices and larger screens. Such techniques enhance the visual presentation of recipes, ensuring that users have a pleasant viewing experience regardless of how they access the site.
In addition to aesthetics, JavaScript frameworks facilitate the creation of interactive components that can respond to user actions. For example, using Vue.js, developers can build a recipe filtering component that updates in real time as users select different options. This interactivity not only engages users but also simplifies their search for the perfect recipe.
new Vue({ el: '#app', data: { recipes: [], selectedCuisine: 'all' }, computed: { filteredRecipes() { return this.recipes.filter(recipe => { return this.selectedCuisine === 'all' || recipe.cuisine === this.selectedCuisine; }); } } });
By using computed properties in Vue.js, this example allows users to filter recipes based on their selected cuisine seamlessly. As the user interacts with the dropdown, the displayed recipes update automatically, reinforcing a responsive and interactive environment.
Finally, combining JavaScript frameworks with mobile-first design practices ensures that recipe websites remain accessible and uncomplicated to manage. Features such as touch gestures, adaptive layouts, and optimized loading times all contribute to a robust user experience that accommodates the needs of today’s users. As the culinary world continues to grow online, using JavaScript frameworks for responsive design will remain a cornerstone of effective recipe website development.
Optimizing Performance for Recipe Loading Times
Optimizing performance for recipe loading times is a fundamental aspect of creating a seamless user experience on recipe websites. With users expecting instant access to content, employing JavaScript to enhance loading speeds can significantly impact user retention and satisfaction. When users encounter slow-loading pages, they’re likely to abandon the site, resulting in lost opportunities for engagement and conversions.
One effective strategy for improving loading times is implementing lazy loading for images and other media assets. By loading only the content that is visible in the viewport, we can reduce the initial payload and speed up the rendering of the page. JavaScript can be utilized to detect when elements come into view and load them dynamically, ensuring a smooth browsing experience.
const lazyLoadImages = () => { const images = document.querySelectorAll('img[data-src]'); const options = { root: null, rootMargin: '0px', threshold: 0.1 }; const observer = new IntersectionObserver((entries, observer) => { entries.forEach(entry => { if (entry.isIntersecting) { const img = entry.target; img.src = img.dataset.src; // Load the image img.classList.remove('lazy'); // Remove the lazy class observer.unobserve(img); // Stop observing the loaded image } }); }, options); images.forEach(image => { observer.observe(image); }); }; document.addEventListener('DOMContentLoaded', lazyLoadImages);
This snippet employs the Intersection Observer API to monitor when images enter the viewport. By deferring the loading of images until they are about to be seen, we can drastically cut down on the initial load time and provide users with immediate access to text content while images load in the background.
In addition to lazy loading, using asynchronous data fetching is important in optimizing performance. For instance, when displaying a list of recipes, we can fetch the data asynchronously and render it only after it has been retrieved. This method allows the page to load quickly while the recipe data is fetched in parallel.
const fetchRecipes = async () => { try { const response = await fetch('https://api.example.com/recipes'); const recipes = await response.json(); displayRecipes(recipes); } catch (error) { console.error('Error fetching recipes:', error); } }; const displayRecipes = (recipes) => { const recipeContainer = document.getElementById('recipe-list'); recipes.forEach(recipe => { const recipeElement = document.createElement('div'); recipeElement.textContent = recipe.title; recipeContainer.appendChild(recipeElement); }); }; document.addEventListener('DOMContentLoaded', fetchRecipes);
In this example, the `fetchRecipes` function retrieves the recipe data asynchronously and then populates the HTML only after the data is ready. This ensures that the user has minimal delay before they can start interacting with the available recipes.
Another technique for improving loading times is minimizing the size of JavaScript files. By employing techniques such as minification and bundling, developers can reduce the number of HTTP requests and the overall size of scripts loaded by the browser. Tools like Webpack can automate this process, allowing developers to focus on writing cleaner, more maintainable code.
// Example Webpack configuration for minification const path = require('path'); module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') }, mode: 'production', // This enables minification automatically optimization: { minimize: true } };
By setting the mode to ‘production’ in Webpack, the JavaScript files will be automatically minified, significantly reducing their size and improving loading speeds. Furthermore, by using code splitting, we can ensure that only the necessary code is loaded for each page, further enhancing performance.
Lastly, implementing caching strategies through service workers can dramatically boost the loading times for repeat visitors. By caching static assets and API responses, users experience quicker load times on subsequent visits, creating a frictionless browsing experience.
if ('serviceWorker' in navigator) { window.addEventListener('load', () => { navigator.serviceWorker.register('/service-worker.js').then(registration => { console.log('Service Worker registered with scope:', registration.scope); }).catch(error => { console.error('Service Worker registration failed:', error); }); }); }
This code registers a service worker which can handle caching strategies defined in the accompanying service-worker.js file. By caching important resources, we can serve them instantly on subsequent loads, thus enhancing user satisfaction and engagement.
Incorporating these strategies not only optimizes the performance of recipe websites but also establishes a solid foundation for a uncomplicated to manage experience. As users navigate through recipes with ease and speed, they are more likely to return, share, and engage with the culinary content presented to them.