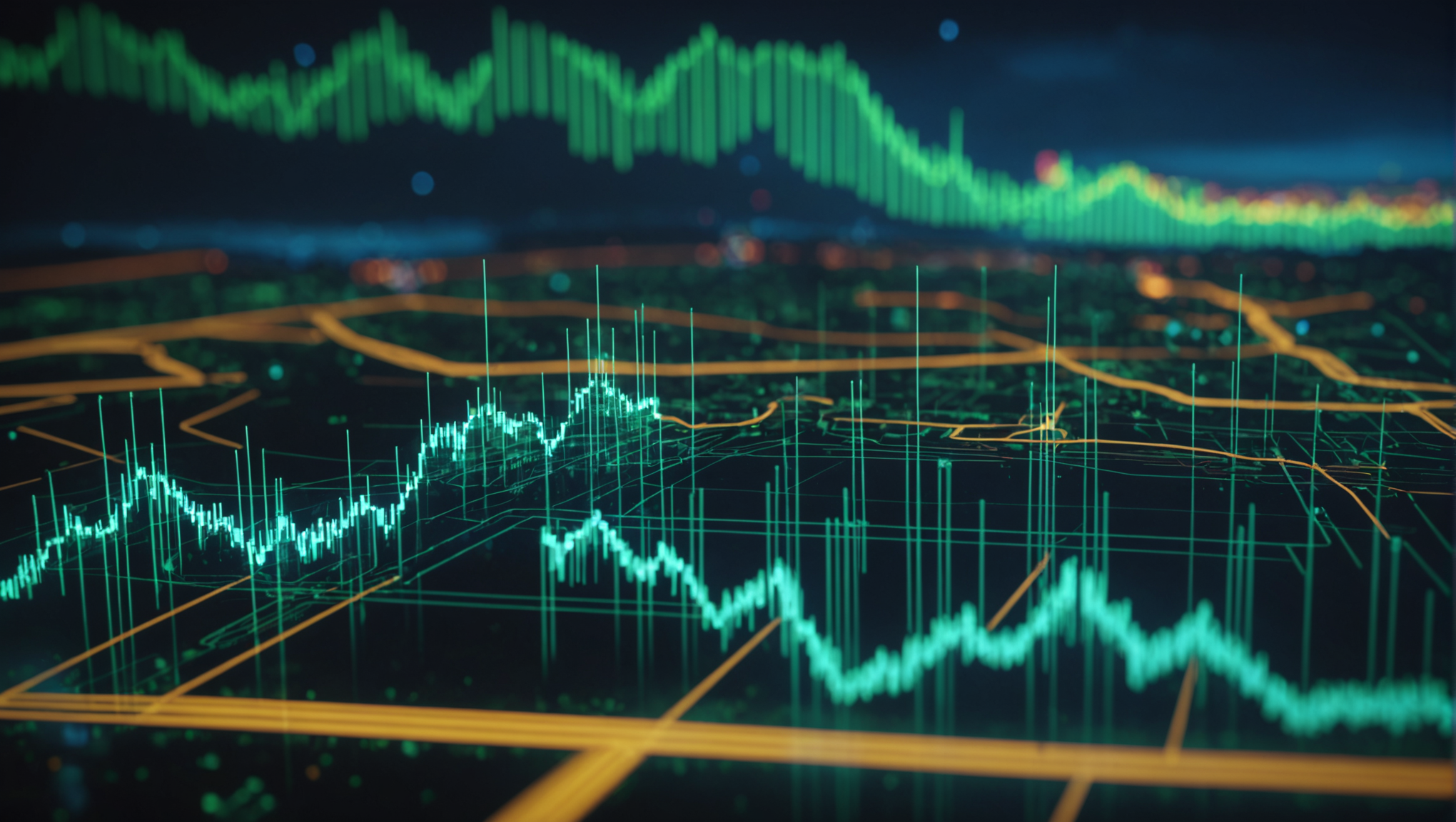
JavaScript and Serverless Architecture
Serverless architecture is a cloud computing model that allows developers to build and run applications without the complexities of managing server infrastructure. In this paradigm, cloud providers dynamically manage the allocation of machine resources. This means that rather than provisioning a specific number of servers, developers can focus solely on writing code. The cloud provider automatically handles the scaling, load balancing, and server management.
At its core, serverless architecture operates on the concept of functions as a service (FaaS). When an event occurs, such as an API request, a database trigger, or a user action, the serverless framework spins up short-lived functions to handle the request. These functions are stateless by design; they execute quickly and only when needed, which contributes to both efficiency and cost-effectiveness.
JavaScript, being a widely used language, fits seamlessly into this model. With its asynchronous and event-driven nature, JavaScript aligns well with the event-based architecture of serverless applications. For instance, using Node.js on the server side allows developers to write serverless functions that respond to events such as HTTP requests or messages from a queue.
Ponder the following example of a simple serverless function written in JavaScript using the AWS Lambda service:
exports.handler = async (event) => { const response = { statusCode: 200, body: JSON.stringify('Hello, World!'), }; return response; };
In this snippet, the function is designed to respond to incoming requests. When deployed in a serverless environment, this function can be triggered by various events without the need for dedicated server management.
Additionally, serverless architecture often comes with built-in integrations for services such as databases, message queues, and APIs. This means developers can streamline workflows and reduce the time spent on setting up and maintaining infrastructure.
Furthermore, since serverless functions are invoked in response to events, they scale automatically. For instance, if a sudden surge of traffic occurs, the cloud provider will instantiate multiple instances of the function to handle the load, ensuring that performance remains steady without any additional configuration from the developer.
Key Benefits of Using JavaScript in Serverless Environments
JavaScript offers a multitude of benefits when utilized in serverless environments, making it a compelling choice for developers looking to harness the efficiency and scalability of serverless architecture. One of the primary advantages is the language’s inherent asynchronous capabilities, which allow developers to write non-blocking code. This aligns perfectly with the event-driven nature of serverless computing, where functions are triggered by various events, such as HTTP requests or database changes. The ability to handle multiple events simultaneously without waiting for one to complete before starting another is a game changer in improving application responsiveness.
Moreover, JavaScript’s lightweight nature contributes to faster function execution. In serverless environments, cold starts—where functions must be initialized before execution—can impact performance. However, JavaScript, particularly in its Node.js incarnation, tends to have faster initialization times than some other languages. This leads to quicker responses for end-users, enhancing the overall user experience.
Another key benefit is JavaScript’s extensive ecosystem. The language boasts a rich array of libraries and frameworks that can significantly expedite development. For example, using libraries like Axios for HTTP requests or Lodash for data manipulation can streamline the coding process, allowing developers to focus on building features rather than reinventing the wheel. This ecosystem also facilitates easy integration with various services, making it simpler to set up databases, authentication, and other functionalities.
const axios = require('axios'); exports.handler = async (event) => { try { const response = await axios.get('https://api.example.com/data'); return { statusCode: 200, body: JSON.stringify(response.data), }; } catch (error) { return { statusCode: 500, body: JSON.stringify({ message: 'Error fetching data' }), }; } };
Flexibility is another significant advantage of using JavaScript in serverless applications. Developers can easily write functions to handle various tasks without worrying about the underlying infrastructure. This means that a single application can encompass different functionalities, such as API endpoints, background processing, and real-time data handling, all written in JavaScript. This versatility enables teams to maintain consistent coding practices across their entire stack.
Additionally, the development and deployment processes are simplified in serverless environments. With tools like the Serverless Framework or AWS SAM, developers can define their serverless architecture as code. This allows for version control, easier collaboration, and rapid iteration cycles, which are essential in today’s fast-paced development landscape.
Lastly, the cost-effectiveness of serverless architecture is enhanced when using JavaScript. Since functions are executed on-demand, you pay only for the compute time you consume. This can lead to significant savings, especially for applications with variable workloads. By combining JavaScript’s efficiency with the pay-as-you-go model of serverless services, developers can optimize both performance and costs.
Popular Serverless Platforms for JavaScript Developers
When it comes to selecting a serverless platform that integrates well with JavaScript, several options stand out, each offering unique features and advantages tailored for JavaScript developers. Understanding these platforms can significantly enhance a developer’s ability to build and deploy efficient serverless applications.
AWS Lambda is one of the most popular serverless platforms, particularly among JavaScript developers. It allows you to run your Node.js applications without provisioning or managing servers. AWS Lambda supports multiple triggers from AWS services, enabling developers to create complex applications with minimal effort. By writing functions in JavaScript using Node.js, developers can quickly respond to events such as changes in an S3 bucket or API Gateway requests. Here’s a concise example of an AWS Lambda function that processes an incoming API request:
exports.handler = async (event) => { // Log the incoming event console.log("Received event:", JSON.stringify(event, null, 2)); // Process the event and return a response return { statusCode: 200, body: JSON.stringify({ message: 'Request processed successfully' }), }; };
Azure Functions is another robust option for JavaScript developers. It provides an event-driven serverless compute platform that scales automatically. Azure Functions seamlessly integrates with various Azure services and supports a variety of programming languages, including JavaScript. The platform offers a rich set of binding options, allowing developers to interact easily with various data sources and services through simple function signatures. Here’s a basic example of an Azure Function that processes an HTTP request:
module.exports = async function (context, req) { const name = (req.query.name || req.body.name || 'Guest'); context.res = { body: `Hello, ${name}!` }; };
Google Cloud Functions is another excellent platform for JavaScript developers seeking to build serverless applications. It enables developers to create single-purpose functions that are triggered by events from Google Cloud services, HTTP requests, or Firebase services. Google Cloud Functions supports Node.js, making it easy for JavaScript developers to get up and running quickly. Here’s an example of a Google Cloud Function that responds to an HTTP request:
const functions = require('firebase-functions'); exports.helloWorld = functions.https.onRequest((request, response) => { response.send("Hello from Google Cloud Functions!"); });
IBM Cloud Functions, based on Apache OpenWhisk, is also worth mentioning. It provides an open-source serverless platform that supports JavaScript and is designed for event-driven applications. IBM Cloud Functions encourages developers to use a serverless architecture to build applications that respond to events from various sources, including HTTP requests and cloud services. Below is a simple IBM Cloud Function example:
function main(params) { return { message: `Hello, ${params.name || 'World'}` }; }
Best Practices for Developing Serverless Applications
When developing serverless applications, adhering to best practices can significantly enhance the application’s performance, maintainability, and cost-effectiveness. Here are some essential strategies to consider:
1. Design for Statelessness: Since serverless functions are stateless, each invocation should be independent. Avoid relying on the local state and instead use external storage solutions like databases or object storage to maintain the application’s state. This ensures that functions can scale seamlessly and handle multiple concurrent requests efficiently.
const AWS = require('aws-sdk'); const dynamoDB = new AWS.DynamoDB.DocumentClient(); exports.handler = async (event) => { const item = { id: event.id, data: event.data, }; await dynamoDB.put({ TableName: 'MyTable', Item: item, }).promise(); return { statusCode: 200, body: JSON.stringify(item) }; };
2. Minimize Cold Start Impact: Cold starts occur when a function is triggered for the first time or after a period of inactivity, leading to latency. To mitigate this, keep your functions lightweight and only include necessary dependencies. Additionally, use techniques like provisioned concurrency in AWS Lambda to maintain a minimum number of warm instances.
3. Optimize Function Performance: Monitor the performance of your functions and optimize them for speed. This can involve choosing the right runtime, minimizing package size, and using efficient algorithms. Profiling tools can help identify bottlenecks and areas for improvement.
exports.handler = async (event) => { const data = event.data.map(item => processData(item)); // Process data in parallel return { statusCode: 200, body: JSON.stringify(data) }; }; function processData(item) { // Perform processing logic return item * 2; }
4. Implement Error Handling and Retries: Since serverless applications can encounter transient errors, incorporate robust error handling and retry logic. Use mechanisms provided by serverless platforms to automatically retry failed invocations. This can greatly improve the resilience of your applications.
exports.handler = async (event) => { try { // Your processing logic here } catch (error) { console.error("Error processing event:", error); throw new Error("Function failed, will be retried"); // Ensure the error is thrown for retries } };
5. Monitor and Log Effectively: Implement comprehensive monitoring and logging to gain insights into function performance and health. Use tools like AWS CloudWatch, Azure Monitor, or other third-party solutions to track metrics and logs, enabling you to detect issues and optimize function behavior.
exports.handler = async (event) => { console.log("Event received:", JSON.stringify(event, null, 2)); // Your function logic here console.log("Processing complete."); };
6. Use Infrastructure as Code (IaC): Manage your serverless architecture using IaC tools such as AWS CloudFormation, Terraform, or Serverless Framework. This approach allows you to version control your infrastructure, automate deployments, and maintain consistent environments across different stages of development.
service: my-service provider: name: aws runtime: nodejs14.x functions: helloWorld: handler: handler.helloWorld events: - http: path: hello method: get
7. Secure Your Functions: Apply proper security practices by using identity and access management (IAM) to restrict permissions for your functions. Ensure that sensitive information, such as API keys and database credentials, are stored securely using environment variables or secret management services.
const AWS = require('aws-sdk'); const secretsManager = new AWS.SecretsManager(); exports.handler = async (event) => { const secret = await secretsManager.getSecretValue({ SecretId: 'MySecret' }).promise(); const apiKey = JSON.parse(secret.SecretString).apiKey; // Use apiKey securely };
Real-World Use Cases of JavaScript in Serverless Architecture
Real-world use cases of JavaScript in serverless architecture demonstrate the versatility and efficiency that this combination can offer. From handling web applications to processing data in real-time, JavaScript’s role in serverless environments is integral and impactful.
One prominent use case is the development of serverless APIs. JavaScript, particularly with Node.js, allows developers to create lightweight, scalable APIs that respond quickly to client requests. For example, ponder a simple RESTful API that serves user data. By using AWS Lambda and API Gateway, developers can easily build endpoints without managing any servers. Here’s a basic implementation:
exports.handler = async (event) => { const users = [ { id: 1, name: 'Alice' }, { id: 2, name: 'Bob' } ]; return { statusCode: 200, body: JSON.stringify(users), }; };
This function fetches a static list of users and returns it as a JSON response. The beauty of serverless architecture shines through here, as the developer can focus on the function’s logic rather than the underlying infrastructure. The API can scale automatically with incoming traffic, effortlessly handling sudden spikes in user requests.
Another compelling use case is data processing in real-time. For instance, businesses often need to process large amounts of streaming data, such as logs or events from IoT devices. JavaScript can be employed in conjunction with serverless functions to analyze and manage this data efficiently. Think a scenario where data from an IoT device is sent to an AWS Kinesis stream, which triggers a Lambda function for processing:
exports.handler = async (event) => {
const records = event.Records.map((record) => {
const payload = Buffer.from(record.kinesis.data, 'base64').toString('utf-8');
return JSON.parse(payload);
});// Process records
console.log("Processing records:", records);