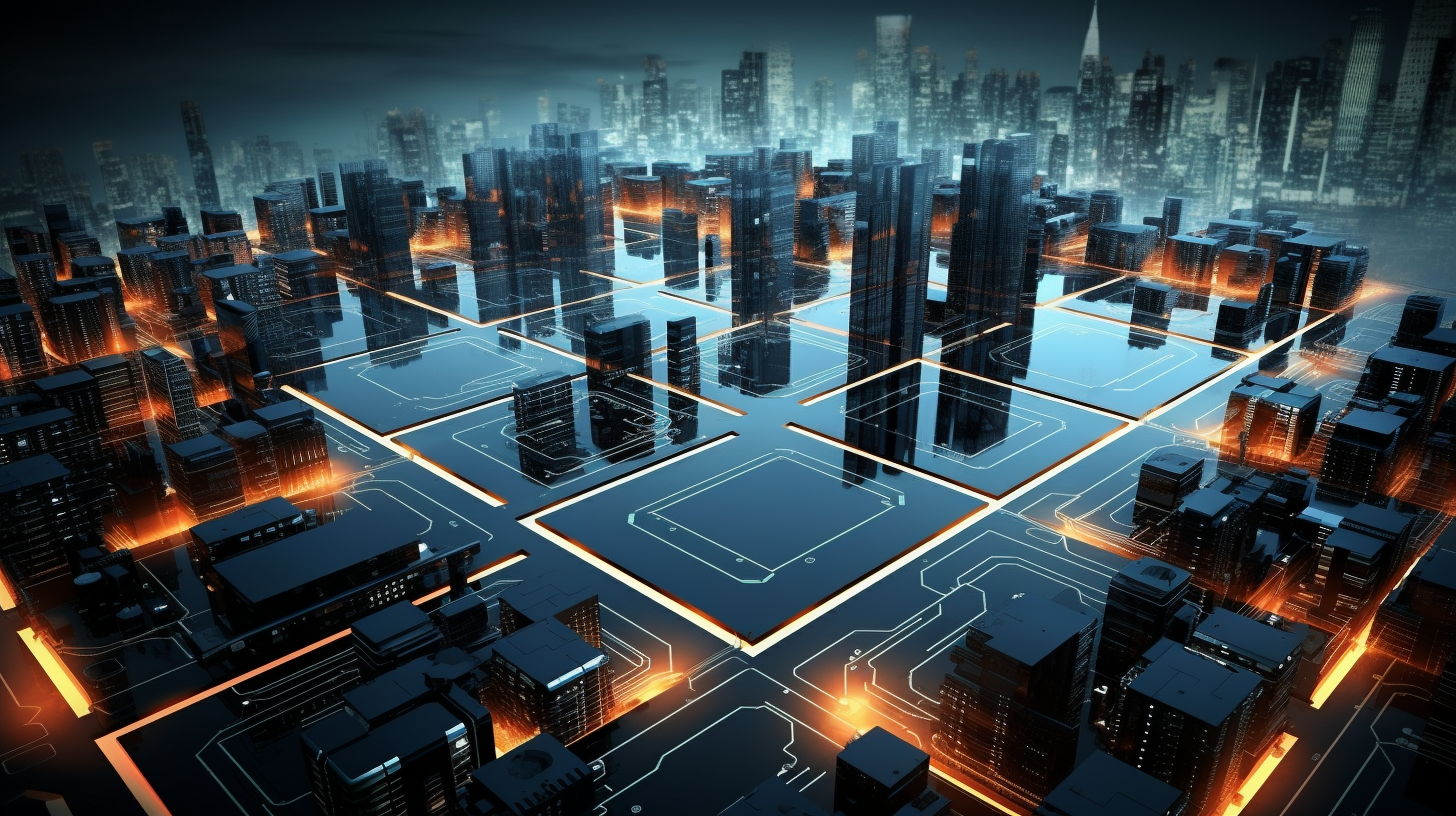
JavaScript and Social Media Integration
When it comes to integrating social media functionality into web applications, using JavaScript libraries can make the process significantly more efficient. These libraries provide pre-built functions and components that simplify the integration of various social media services, allowing developers to focus on building engaging user experiences rather than wrestling with API details.
One popular library is Socialite.js, a lightweight library that abstracts the complexities of social media APIs. With Socialite.js, you can easily implement login features and share content across multiple platforms. Here’s a simple example of using Socialite.js to enable a Facebook login:
// Import Socialite.js import Socialite from 'socialite.js'; // Initialize Socialite const socialite = new Socialite(); // Set up Facebook login socialite.on('facebook', (response) => { console.log('User logged in:', response); }); // Trigger Facebook login document.getElementById('facebook-login').addEventListener('click', () => { socialite.login('facebook'); });
Another powerful library is ShareThis, which provides a simple way to add share buttons to your website. With just a few lines of code, you can allow users to share content across various platforms, enhancing your site’s reach and engagement. Here’s how you can embed ShareThis into your project:
// Load ShareThis script (function() { var st = document.createElement('script'); st.type = 'text/javascript'; st.async = true; st.src = 'https://platform-api.sharethis.com/js/sharethis.js#property=YOUR_PROPERTY_ID&product=inline-share-buttons'; var s = document.getElementsByTagName('script')[0]; s.parentNode.insertBefore(st, s); })(); // Initialize ShareThis buttons document.addEventListener('DOMContentLoaded', () => { window.__sharethis__.initialize(); });
In addition, OAuth.io provides a seamless way to handle OAuth authentication across multiple social media platforms. This library simplifies the inclusion of login features, allowing developers to authenticate users without delving deep into each platform’s authentication protocols. Below is a code snippet to configure OAuth.io for Twitter authentication:
// Include OAuth.io SDK OAuth.initialize('YOUR_PUBLIC_KEY'); // Trigger Twitter login document.getElementById('twitter-login').addEventListener('click', () => { OAuth.popup('twitter').done((result) => { console.log('Logged in with Twitter:', result); }).fail((err) => { console.error('Error during Twitter login:', err); }); });
These libraries not only save development time but also help ensure that your application adheres to best practices when interacting with social media APIs. By using these tools, developers can create a more robust and effortless to handle experience, allowing users to connect and share simply across their favorite platforms.
Using APIs for Social Media Features
Using APIs for social media features is essential for creating rich and interactive user experiences that leverage the power of these platforms. Most social media networks provide APIs that allow developers to access data and functionalities such as posting content, retrieving user information, and managing OAuth authentication. Understanding how to utilize these APIs effectively can significantly enhance your web application’s interactivity and engagement.
One of the primary tasks when using social media APIs is to perform API calls that fetch or push data. For instance, if you want to allow users to post updates to their Twitter feed directly from your application, you would need to interact with Twitter’s API. Below is an example of how to implement a basic function to post a tweet using the Fetch API and Twitter’s POST statuses/update endpoint:
async function postTweet(accessToken, tweetContent) { const response = await fetch('https://api.twitter.com/1.1/statuses/update.json', { method: 'POST', headers: { 'Authorization': `Bearer ${accessToken}`, 'Content-Type': 'application/json' }, body: JSON.stringify({ status: tweetContent }) }); if (response.ok) { const jsonResponse = await response.json(); console.log('Tweet posted successfully:', jsonResponse); } else { console.error('Error posting tweet:', response.statusText); } } // Example usage const userAccessToken = 'YOUR_ACCESS_TOKEN_HERE'; postTweet(userAccessToken, 'Hello Twitter! That's a tweet from my app.');
For Facebook integration, you can utilize the Facebook Graph API to manage user data and perform actions such as posting to a user’s timeline. Here’s an example that demonstrates how to use the Graph API to share a link:
function shareOnFacebook(accessToken, link) { fetch(`https://graph.facebook.com/me/feed`, { method: 'POST', headers: { 'Authorization': `Bearer ${accessToken}`, 'Content-Type': 'application/json' }, body: JSON.stringify({ link: link }) }) .then(response => { if (response.ok) { return response.json(); } else { throw new Error('Error sharing on Facebook'); } }) .then(data => { console.log('Successfully shared on Facebook:', data); }) .catch(error => { console.error('Error:', error); }); } // Example usage const fbAccessToken = 'YOUR_FACEBOOK_ACCESS_TOKEN'; shareOnFacebook(fbAccessToken, 'https://example.com');
Using these APIs not only enriches your application’s functionality but also provides users with a seamless experience. Furthermore, error handling and user feedback are vital parts of API interactions. You’ll want to ensure that your application gracefully handles any issues that arise during API calls, such as expired tokens or network errors.
It’s also important to familiarize yourself with the documentation of each social media platform, as they often provide comprehensive guides and examples on how to use their APIs effectively. This knowledge will allow you to implement advanced features such as user authentication, data retrieval, and content sharing, enhancing the overall user experience of your web application.
Enhancing User Experience with Social Sharing
Enhancing user experience with social sharing involves not just the fundamental integration of social media features but also the thoughtful design of how these features are presented and utilized within your application. The goal is to create a seamless and intuitive process for users, encouraging them to engage with your content and share it across their networks without friction.
To achieve this, using visually appealing social sharing buttons is essential. These buttons should be easily accessible and strategically placed to capture user attention right when they engage with your content. Ponder using floating share buttons that follow the user as they scroll or placing them at the end of articles to prompt sharing after content consumption. Here’s an example of a simple implementation using HTML and JavaScript to create a share popup:
function openSharePopup(url) { const shareUrl = `https://www.facebook.com/sharer/sharer.php?u=${encodeURIComponent(url)}`; window.open(shareUrl, 'Share', 'width=600,height=400'); } // Attach event listener to the share button document.getElementById('share-button').addEventListener('click', () => { openSharePopup('https://example.com'); });
Moreover, presenting users with pre-filled messages can significantly improve their likelihood of sharing your content. You can use Twitter’s sharing URL to create a tweet with a predefined message or hashtags. Below is an example of how to set this up:
function shareOnTwitter(text, url) { const twitterUrl = `https://twitter.com/intent/tweet?text=${encodeURIComponent(text)}&url=${encodeURIComponent(url)}`; window.open(twitterUrl, 'Share', 'width=600,height=400'); } // Example usage document.getElementById('twitter-share-button').addEventListener('click', () => { shareOnTwitter('Check out this awesome article!', 'https://example.com'); });
In addition to functionality, consider the user’s context when integrating social sharing. Implement features that allow users to share content easily while they’re still engaged. For instance, using modal dialogues that appear after a user performs a specific action, such as completing a purchase or reading an article, can prompt them to share their experience. Here’s a simple modal implementation:
function showShareModal() { const modal = document.getElementById('share-modal'); modal.style.display = 'block'; } function closeModal() { const modal = document.getElementById('share-modal'); modal.style.display = 'none'; } // Example to show modal on button click document.getElementById('show-share-modal').addEventListener('click', showShareModal); document.getElementById('close-modal').addEventListener('click', closeModal);
Furthermore, incorporating social proof elements like share counts can motivate users to participate in sharing activities. Displaying how many times the content has been shared can create a sense of community and encourage others to join in. Use API calls to fetch share counts from platforms like Facebook and Twitter and update your UI accordingly. Here’s a hypothetical example of how to fetch and display share counts:
async function fetchShareCounts(url) { const response = await fetch(`https://api.example.com/share-counts?url=${encodeURIComponent(url)}`); const data = await response.json(); document.getElementById('share-count').innerText = `Shared ${data.count} times`; } // Fetch share counts on page load document.addEventListener('DOMContentLoaded', () => { fetchShareCounts('https://example.com'); });
Ultimately, enhancing user experience with social sharing is about creating an environment where users feel encouraged and empowered to share content efficiently. By using JavaScript for integrating functionality, designing intuitive interfaces, and providing context-aware prompts, developers can significantly increase user engagement and interaction with social media features on their platforms.
Managing User Authentication through Social Platforms
Managing user authentication through social platforms is an important aspect of modern web applications. It allows users to log in easily without the need to create a new account and remember another password. By using social media authentication, developers can streamline the registration and login processes, enhancing user experience while also improving security. The most common methods for managing user authentication include using OAuth protocols, which are supported by major social media platforms like Facebook, Google, and Twitter.
To implement social authentication, developers typically use libraries and SDKs provided by the social platforms. For example, Facebook offers the Facebook JavaScript SDK, which makes it simpler to handle user login, logout, and account management. Here’s a simple implementation demonstrating how to set up Facebook authentication:
// Load the Facebook SDK window.fbAsyncInit = function() { FB.init({ appId : 'YOUR_APP_ID', cookie : true, xfbml : true, version : 'v11.0' }); }; // Load the SDK asynchronously (function(d, s, id){ var js, fjs = d.getElementsByTagName(s)[0]; if (d.getElementById(id)) {return;} js = d.createElement(s); js.id = id; js.src = "https://connect.facebook.net/en_US/sdk.js"; fjs.parentNode.insertBefore(js, fjs); }(document, 'script', 'facebook-jssdk')); // Facebook login function function facebookLogin() { FB.login(function(response) { if (response.authResponse) { console.log('Welcome! Fetching your information.... '); FB.api('/me', function(response) { console.log('Good to see you, ' + response.name + '.'); }); } else { console.log('User cancelled login or did not fully authorize.'); } }); } // Attach event listener to the login button document.getElementById('fb-login-button').addEventListener('click', facebookLogin);
In addition to Facebook, Google also provides a robust authentication system through the Google Sign-In API. This service allows users to sign in to your application using their Google accounts easily. Here’s an example of how to implement Google Sign-In:
// Load the Google API client function onLoad() { gapi.load('auth2', function() { auth2 = gapi.auth2.init({ client_id: 'YOUR_CLIENT_ID', cookiepolicy: 'single_host_origin', }); }); } // Google login function function googleLogin() { auth2.signIn().then(function(googleUser) { var profile = googleUser.getBasicProfile(); console.log('ID: ' + profile.getId()); console.log('Name: ' + profile.getName()); console.log('Image URL: ' + profile.getImageUrl()); }); } // Attach event listener to the login button document.getElementById('google-login-button').addEventListener('click', googleLogin);
Both of these methods not only simplify the login process but also provide developers with access to a wealth of user data, should the user grant permission. However, it is essential to handle authentication securely. Always ensure the tokens received after login are stored securely and implement proper validation on your server-side to prevent unauthorized access.
Furthermore, integrating logout functionality is equally important. Users should be able to disconnect from the application at any time. Here’s how to implement logout for both Facebook and Google:
// Facebook logout function function facebookLogout() { FB.logout(function(response) { console.log('User logged out from Facebook.'); }); } // Google logout function function googleLogout() { auth2.signOut().then(function() { console.log('User logged out from Google.'); }); } // Attach event listeners to logout buttons document.getElementById('fb-logout-button').addEventListener('click', facebookLogout); document.getElementById('google-logout-button').addEventListener('click', googleLogout);
By implementing these authentication solutions, developers can enhance user convenience while maintaining a secure application environment. Moreover, as social media platforms evolve, staying updated with their SDKs and best practices is vital for successful integration.
Analytics and Tracking Social Media Engagement
When it comes to analytics and tracking social media engagement, JavaScript plays a pivotal role in gathering data that can be crucial for refining marketing strategies and understanding user behavior. By using the various APIs and SDKs provided by social media platforms, developers can implement functionalities that not only track user interactions but also provide insights into how content is shared and consumed.
One of the most effective ways to track social media engagement is through event tracking. Google Analytics, for instance, allows you to track specific events on your website, such as when a user shares content on platforms like Facebook or Twitter. Implementing event tracking with JavaScript is simpler. Here’s an example of how to set it up:
// Function to track social share events function trackShareEvent(platform, url) { gtag('event', 'share', { 'event_category': 'Social Media', 'event_label': platform, 'value': url }); } // Attach event listeners for share buttons document.getElementById('facebook-share-button').addEventListener('click', () => { trackShareEvent('Facebook', 'https://example.com'); }); document.getElementById('twitter-share-button').addEventListener('click', () => { trackShareEvent('Twitter', 'https://example.com'); });
In this snippet, the `trackShareEvent` function sends an event to Google Analytics whenever a user shares content on Facebook or Twitter. You can customize the `event_category`, `event_label`, and `value` parameters to fit your tracking needs. This level of detail allows you to determine which platforms are driving the most engagement.
Another crucial aspect of tracking engagement is understanding how users interact with your content before sharing. Implementing scroll tracking can provide insights into how far down a page users scroll before deciding to share. Below is an example of how to set up scroll tracking:
// Function to track scroll depth function trackScrollDepth() { const scrollDepth = Math.round(window.scrollY / document.body.scrollHeight * 100); gtag('event', 'scroll_depth', { 'event_category': 'Engagement', 'event_label': `${scrollDepth}% scrolled` }); } // Attach event listener to track scroll window.addEventListener('scroll', trackScrollDepth);
The `trackScrollDepth` function calculates the percentage of the page that the user has scrolled and sends this data to Google Analytics. By understanding scroll behavior, you can make informed decisions about where to place your social sharing buttons and how to optimize your content.
Additionally, tracking user interactions with specific social media features, such as likes and comments, can provide valuable feedback. For example, if you have integrated a Facebook comment plugin on your site, you can capture insights using the Facebook Graph API. Here’s how to retrieve comments for a particular post:
// Function to fetch comments from Facebook Graph API async function fetchFacebookComments(postId, accessToken) { const response = await fetch(`https://graph.facebook.com/${postId}/comments?access_token=${accessToken}`); const data = await response.json(); console.log('Comments:', data); } // Example usage const postId = 'YOUR_POST_ID'; const accessToken = 'YOUR_FACEBOOK_ACCESS_TOKEN'; fetchFacebookComments(postId, accessToken);
By gathering comments, you can analyze user sentiment and engagement, which can inform your content strategy moving forward. Moreover, it allows you to respond promptly to user feedback, enhancing the overall user experience.
Lastly, it’s important to visualize the data you gather. Integrating tools like Chart.js or D3.js can help you create insightful visualizations of engagement metrics over time. By turning raw data into charts and graphs, you provide stakeholders with clear insights into how social media is impacting your site’s performance.
// Sample code to visualize share events using Chart.js const ctx = document.getElementById('engagementChart').getContext('2d'); const engagementChart = new Chart(ctx, { type: 'bar', data: { labels: ['Facebook', 'Twitter', 'Instagram'], datasets: [{ label: 'Shares', data: [12, 19, 3], // Sample data for shares backgroundColor: ['rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)'], borderColor: ['rgba(255, 99, 132, 1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)'], borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } } } });
Incorporating robust analytics and tracking mechanisms enables developers to measure the impact of social media integrations effectively. Understanding user behavior and engagement patterns not only helps in refining current strategies but also in predicting future outcomes, making analytics an indispensable part of any social media integration project.