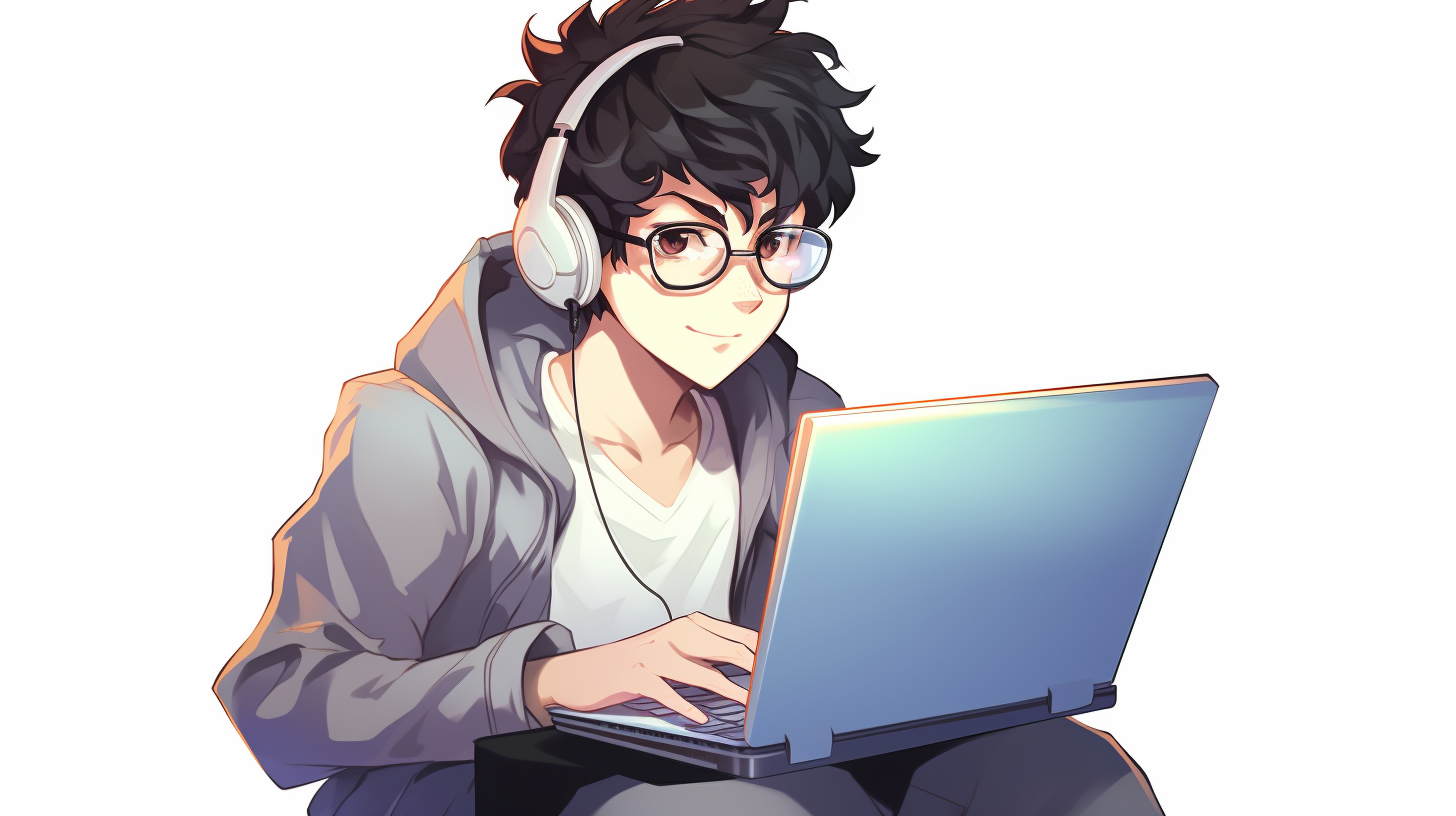
JavaScript and the Internet of Things (IoT)
JavaScript is often perceived as the language of the web, but its versatility extends far beyond browser-based applications. In the sphere of the Internet of Things (IoT), JavaScript plays a pivotal role in bridging the gap between hardware and software, enabling developers to create interconnected systems that communicate and collaborate seamlessly.
At the core of IoT architecture, JavaScript functions in both the client and server domains. On the client side, JavaScript enables the development of user interfaces that can control devices, visualize data, and respond in real-time to events from sensors or other devices. On the server side, JavaScript, particularly with the Node.js runtime, allows for efficient handling of asynchronous I/O operations, making it well-suited for processing the streams of data generated by IoT devices.
Device Communication
One of the key responsibilities of JavaScript in IoT architecture is managing device communication. This often involves using protocols such as MQTT (Message Queuing Telemetry Transport) or WebSockets, which facilitate lightweight and real-time communication between devices and servers. For instance, a temperature sensor could send data to a server using MQTT, which would then notify a web client to update the UI with the latest reading.
const mqtt = require('mqtt'); const client = mqtt.connect('mqtt://broker.hivemq.com'); client.on('connect', () => { client.subscribe('home/temperature', (err) => { if (!err) { console.log('Subscribed to temperature topic'); } }); }); client.on('message', (topic, message) => { // Message is a Buffer console.log(`New temperature reading: ${message.toString()}`); });
Data Processing
In addition to communication, JavaScript excels at data processing. When IoT devices generate data, it’s often necessary to filter, aggregate, and analyze this information before sending it to a user interface or database. JavaScript’s asynchronous capabilities allow developers to write code that processes incoming data streams without blocking the execution of other operations, which is important in an environment where timely responses are essential.
const processTemperatureData = (data) => { const tempValue = parseFloat(data); if (tempValue > 30) { console.log('Warning: High temperature detected!'); } // Further processing can be done here }; // Simulating incoming data stream setInterval(() => { const mockData = (Math.random() * 40).toString(); processTemperatureData(mockData); }, 1000);
Integration with Cloud Services
JavaScript also acts as a powerful tool for integrating IoT devices with cloud services. With the rise of cloud computing, many IoT applications rely on cloud platforms for data storage, processing, and analytics. JavaScript can be used to create REST APIs or to interact with cloud services directly, allowing devices to send and retrieve data efficiently. For example, a smart home device might send updates to an AWS DynamoDB database using JavaScript’s fetch API.
const updateToCloud = async (temperature) => { const response = await fetch('https://api.example.com/updateTemperature', { method: 'POST', headers: { 'Content-Type': 'application/json', }, body: JSON.stringify({ temperature }), }); if (response.ok) { console.log('Temperature updated successfully'); } else { console.error('Failed to update temperature'); } }; // Example of sending temperature data updateToCloud(22.5);
JavaScript’s event-driven architecture, along with its rich ecosystem of libraries and frameworks, positions it as a formidable ally in the IoT architecture landscape. Its ability to handle simultaneous connections, process large amounts of data, and integrate with various services solidifies its role as a cornerstone in the development of modern IoT applications.
Key JavaScript Frameworks and Libraries for IoT Development
When it comes to developing IoT solutions that leverage JavaScript, several frameworks and libraries stand out, each providing unique features that simplify the process of building applications for connected devices. Understanding these tools is essential for any developer looking to harness the full potential of JavaScript in IoT development.
Node.js is arguably the most significant framework in this landscape. As a server-side runtime environment built on Chrome’s V8 JavaScript engine, Node.js allows developers to write scalable network applications. Its non-blocking, event-driven architecture is ideally suited for handling the asynchronous nature of IoT devices, which often generate streams of data. With Node.js, developers can create servers that manage multiple device connections at the same time, significantly improving performance and responsiveness.
const http = require('http'); const server = http.createServer((req, res) => { res.writeHead(200, {'Content-Type': 'text/plain'}); res.end('IoT Server is runningn'); }); server.listen(3000, () => { console.log('Server running at http://localhost:3000/'); });
Johnny-Five is another popular framework tailored specifically for IoT applications. It offers a JavaScript framework for robotics and IoT, making it easier to control hardware components like sensors, lights, and motors. With Johnny-Five, developers can write code that interacts with various hardware platforms, such as Arduino, Raspberry Pi, and others, allowing for rapid prototyping and experimentation.
const five = require('johnny-five'); const board = new five.Board(); board.on('ready', () => { const led = new five.Led(13); led.blink(500); // Blink an LED every 500ms });
Socket.io is another powerful library that enables real-time, bidirectional communication between clients and servers. This capability is pivotal in IoT applications where devices need to send and receive data instantly. For example, a smart thermostat can use Socket.io to communicate temperature changes in real-time to a web interface, ensuring that users are always informed about their home environment.
const io = require('socket.io')(3000); io.on('connection', (socket) => { console.log('New client connected'); socket.on('temperatureUpdate', (temp) => { console.log(`Temperature updated: ${temp}`); }); });
Express is a minimal and flexible Node.js web application framework that provides a robust set of features for building web and mobile applications. In the context of IoT, Express can be used to create RESTful APIs that enable devices to interact with each other or with applications hosted on cloud platforms. That’s important for implementing functionalities such as data retrieval and command execution.
const express = require('express'); const app = express(); const PORT = 3000; app.use(express.json()); app.post('/temperature', (req, res) => { console.log(`Received temperature: ${req.body.temp}`); res.status(200).send('Temperature received'); }); app.listen(PORT, () => { console.log(`Server running on port ${PORT}`); });
Lastly, IoT.js is a lightweight JavaScript framework specifically designed for IoT devices. It aims to run on small footprint devices, enabling developers to deploy JavaScript applications on resource-constrained hardware. IoT.js supports various communication protocols and provides a set of APIs to interact with hardware resources, making it a solid choice for low-power IoT devices.
The JavaScript ecosystem provides a rich selection of frameworks and libraries tailored for IoT development. From the asynchronous capabilities of Node.js to the hardware-focused features of Johnny-Five, these tools allow developers to create sophisticated and efficient applications that can communicate, process, and act on data in real-time. As IoT continues to expand, mastering these frameworks will be essential for building the next generation of connected solutions.
Real-World Applications of JavaScript in IoT Solutions
Real-world applications of JavaScript in IoT solutions are diverse and reflect the language’s adaptability to various hardware and software environments. From smart homes to industrial automation, JavaScript’s role is pivotal in creating responsive and interconnected systems. Let’s explore some compelling use cases.
Smart Home Automation
In smart homes, JavaScript is employed to manage a myriad of devices, such as lights, thermostats, and security cameras. Using frameworks like Node.js and libraries like Johnny-Five, developers can create applications that enable homeowners to control their devices remotely. For instance, a simple JavaScript application can allow users to adjust their home temperature from a web interface.
const five = require('johnny-five'); const board = new five.Board(); board.on('ready', () => { const thermostat = new five.Thermometer({ pin: 'A0' }); thermostat.on("data", function() { console.log(`Current Temperature: ${this.celsius}°C`); }); // Simulate temperature adjustment const adjustTemperature = (desiredTemp) => { console.log(`Adjusting temperature to ${desiredTemp}°C`); // Logic to control heating/cooling devices }; });
This example demonstrates how a temperature sensor can publish its readings, allowing users to monitor and adjust their home environment through a centralized app.
Wearable Health Devices
JavaScript also finds applications in wearable health devices. By using technologies like Bluetooth Low Energy (BLE) and libraries such as Noble for Node.js, developers can create applications that collect and analyze health metrics in real-time. For example, a fitness tracker might utilize JavaScript to send heart rate data to a mobile app or a cloud service for storage and analysis.
const noble = require('@abandonware/noble'); noble.on('stateChange', (state) => { if (state === 'poweredOn') { noble.startScanning(); } else { noble.stopScanning(); } }); noble.on('discover', (peripheral) => { console.log(`Discovered device: ${peripheral.advertisement.localName}`); // Further processing to connect and read data });
This code snippet illustrates how to scan for nearby BLE devices, paving the way for more sophisticated interactions with health tracking devices.
Industrial IoT (IIoT)
In industrial settings, JavaScript plays a vital role in monitoring and controlling machinery. By using platforms like Node-RED, which allows for the visual programming of IoT applications, developers can create workflows that integrate various sensors and actuators. This setup can facilitate predictive maintenance by analyzing data from equipment sensors and sending alerts when anomalies are detected.
const express = require('express'); const app = express(); app.post('/machine-status', (req, res) => { const { machineId, status } = req.body; console.log(`Machine ${machineId} status: ${status}`); // Logic to handle machine status updates res.send('Status received'); }); app.listen(3000, () => { console.log('IIoT server running on port 3000'); });
This example highlights how JavaScript can be used to receive and process status updates from industrial machines, enabling proactive management and decision-making in the workplace.
Smart Agriculture
Moreover, in agriculture, JavaScript is used to develop systems that monitor soil moisture levels, weather conditions, and crop health. By employing sensors connected via IoT, farmers can optimize irrigation and ensure their crops receive the right amount of nutrients. Using JavaScript, these systems can provide valuable insights through data visualization tools.
const mqtt = require('mqtt'); const client = mqtt.connect('mqtt://broker.hivemq.com'); client.on('connect', () => { client.subscribe('farm/moisture', (err) => { if (!err) { console.log('Subscribed to soil moisture topic'); } }); }); client.on('message', (topic, message) => { const moistureLevel = parseFloat(message.toString()); console.log(`Current soil moisture level: ${moistureLevel}%`); // Logic to trigger irrigation systems based on moisture level });
This code example depicts how moisture levels can be monitored in real time, allowing farmers to make informed decisions regarding irrigation.
These real-world applications demonstrate the immense potential of JavaScript in the IoT space. By enabling seamless interaction between devices and users, JavaScript becomes an important element in the development of innovative IoT solutions that enhance everyday life and optimize industrial processes.
Challenges and Future Trends of JavaScript in the IoT Landscape
As the landscape of the Internet of Things (IoT) continues to evolve, developers face a multitude of challenges when using JavaScript for IoT solutions. One prominent issue is the fragmentation of platforms and devices. With a vast array of hardware, protocols, and standards in use, ensuring interoperability can be daunting. JavaScript developers must navigate these complexities to create solutions that can communicate across diverse systems, which requires a deep understanding of various communication protocols, such as MQTT, CoAP, and WebSockets.
Another challenge lies in resource constraints. Many IoT devices are designed to be low-power and low-cost, leading to limitations in processing power and memory. While JavaScript, particularly in the Node.js ecosystem, excels at handling asynchronous operations, it can be less efficient on resource-constrained devices compared to more lightweight languages like C. Consequently, developers may need to optimize their JavaScript code rigorously or adopt specialized frameworks like IoT.js that are tailored for low-resource environments.
Security is also a major concern within the IoT landscape. As devices become increasingly interconnected, the potential attack surface expands, making them prime targets for cyber threats. JavaScript applications must implement robust security measures, such as encryption and authentication, to safeguard data in transit and at rest. Additionally, developers must stay informed about the latest security practices and vulnerabilities, ensuring that their applications remain resilient against emerging threats.
Despite these challenges, the future trends in JavaScript for IoT development point towards greater interoperability and enhanced performance. The rise of standards such as Web of Things (WoT) aims to bridge the gap between different devices and services, allowing for more seamless integration. This shift towards standardized protocols can help mitigate some of the fragmentation currently faced by developers.
Moreover, as hardware capabilities improve and compute resources become more accessible, we can expect to see a surge in edge computing solutions. This will allow for more complex data processing and analysis to occur closer to the source, reducing latency and bandwidth usage. JavaScript will play an important role in these edge computing scenarios, enabling real-time data processing right at the device level.
Furthermore, advancements in machine learning and artificial intelligence are beginning to permeate the IoT space, and JavaScript is no exception. With libraries like TensorFlow.js, developers can implement machine learning models directly on IoT devices, enabling smarter applications that can learn from the data they collect. This capability opens up exciting possibilities for predictive maintenance, anomaly detection, and personalized user experiences.
As we look ahead, the evolution of JavaScript within the IoT landscape promises to be dynamic and impactful. With ongoing improvements in frameworks, libraries, and standards, JavaScript is well-positioned to meet the demands of tomorrow’s interconnected world.