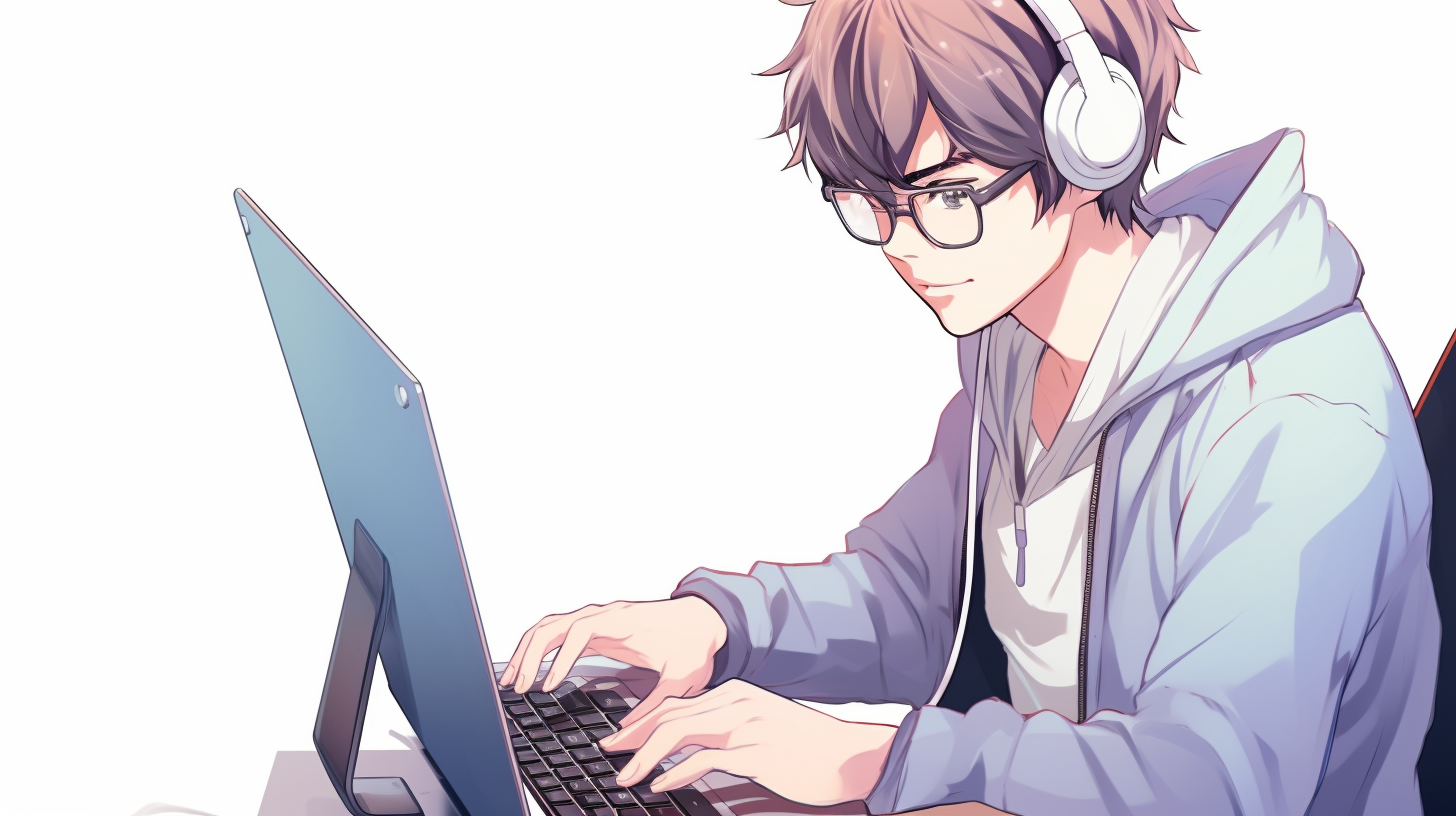
JavaScript and User Authentication
User authentication is a fundamental aspect of web applications that serves as a gatekeeper, ensuring that only authorized users can access certain resources or functionalities. At its core, user authentication is the process of verifying the identity of a user who attempts to access a system. This process typically involves the submission of a username and password, but can also encompass more advanced techniques, all of which contribute to the overall security framework of the application.
When a user attempts to log in, the application must validate the provided credentials against a stored dataset, usually in a database. If the credentials match, the user is authenticated and granted access. However, if they do not, the user is denied entry, and an appropriate error message is returned. The simplicity of this method belies the complexity of its secure implementation.
Each method of authentication operates on a unique flow and requires careful consideration of security implications. For example, storing passwords in plaintext is a critical vulnerability. Instead, developers must employ hashing algorithms, such as bcrypt, to securely store passwords. The hashing process transforms the password into a fixed-size string that cannot be reversed, thereby safeguarding the user’s data even if the database is compromised.
In a typical web application, the authentication process can be broken down into several key steps:
// Example of a simple login function async function loginUser(username, password) { const user = await findUserByUsername(username); if (user && await verifyPassword(password, user.hashedPassword)) { // Generate a session token or JWT const token = generateToken(user.id); return { success: true, token: token }; } return { success: false, message: "Invalid username or password." }; }
After successful authentication, the application often establishes a session or issues a token, which is then used for subsequent requests. This token serves as proof that the user has been authenticated and can access protected routes. The use of tokens, especially in single-page applications (SPAs), simplifies the user experience by reducing the need for frequent reauthentication.
However, merely authenticating a user does not complete the security picture. It’s essential to implement measures that protect against common vulnerabilities such as Cross-Site Scripting (XSS) and Cross-Site Request Forgery (CSRF). These attacks can manipulate authenticated sessions and lead to unauthorized access if not adequately addressed.
Understanding user authentication in web applications is not just about the mechanics of username and password validation; it encompasses a broader spectrum of security practices and protocols necessary for building robust and secure applications. In this ever-evolving threat landscape, developers must remain vigilant and adapt their authentication strategies to safeguard user data effectively.
Common Authentication Methods in JavaScript
Within the scope of JavaScript, there are several authentication methods that developers commonly employ to protect user data and ensure secure access. Each method has its nuances, advantages, and potential vulnerabilities. Below, we delve into some of the most prevalent authentication methods used in JavaScript applications today.
1. Basic Authentication
Basic authentication is one of the simplest methods available, relying on the HTTP protocol. Users provide their credentials (username and password) encoded in Base64, which are then sent in the HTTP headers. While easy to implement, it lacks security since credentials are typically transmitted unencrypted, making it susceptible to interception by malicious actors.
const username = "user"; const password = "pass"; const credentials = btoa(username + ":" + password); fetch("https://api.example.com/protected-resource", { method: "GET", headers: { "Authorization": "Basic " + credentials } }).then(response => { if (response.ok) { return response.json(); } throw new Error("Authentication failed."); });
2. Token-Based Authentication
Token-based authentication improves upon basic authentication by issuing a token to the user upon successful login. This token can be stored in local storage or a cookie and is sent in the Authorization header for subsequent requests. JSON Web Tokens (JWT) are a popular choice due to their compact size and self-contained nature, allowing for easy verification of user identity.
async function authenticateUser(username, password) { const response = await fetch("/api/auth/login", { method: "POST", headers: { "Content-Type": "application/json" }, body: JSON.stringify({ username, password }) }); if (response.ok) { const { token } = await response.json(); localStorage.setItem('authToken', token); return true; } return false; }
3. OAuth 2.0
OAuth 2.0 offers a robust framework for third-party authentication, enabling users to log in using credentials from platforms like Google or Facebook. This method enhances convenience and security by offloading credential management to trusted providers. Integrating OAuth in JavaScript typically involves redirecting users to the provider for authentication, then handling the access token upon their return.
function redirectToProvider() { const clientId = "YOUR_CLIENT_ID"; const redirectUri = "YOUR_REDIRECT_URI"; const scope = "email profile"; window.location.href = `https://provider.com/oauth/authorize?client_id=${clientId}&redirect_uri=${redirectUri}&scope=${scope}`; }
4. Session-Based Authentication
With session-based authentication, the server creates a session for each authenticated user, storing their session data on the server-side. A session ID is then sent to the client as a cookie. Subsequent requests from the client include this cookie, allowing the server to identify the user. While effective, managing sessions can introduce scalability challenges, particularly in distributed systems.
async function startSession(username, password) { const response = await fetch("/api/sessions", { method: "POST", headers: { "Content-Type": "application/json" }, body: JSON.stringify({ username, password }) }); if (response.ok) { const sessionId = response.headers.get("Set-Cookie"); document.cookie = `sessionId=${sessionId}; path=/`; return true; } return false; }
Each of these methods has its place in the developer’s toolkit, depending on the specific requirements of the application, user experience considerations, and security needs. The choice of authentication method requires a careful balance between usability and security, and understanding these common approaches is important for building secure JavaScript applications.
Implementing JWT (JSON Web Tokens) for Secure Sessions
Implementing JSON Web Tokens (JWT) for secure sessions is a powerful strategy in modern web applications, particularly those built with JavaScript. JWTs offer a way to represent claims securely between two parties, enabling a stateless authentication mechanism that alleviates some of the burdens associated with traditional session management.
A JWT is composed of three parts: the header, the payload, and the signature. The header typically consists of the token type (JWT) and the signing algorithm (e.g., HMAC SHA256 or RSA). The payload contains the claims, which can include user information and other metadata. Finally, the signature is computed using the header, the payload, and a secret key or a private key, ensuring that the token has not been tampered with.
Here’s a simple structure of a JWT:
// Example of a JWT structure const jwt = { header: { alg: "HS256", typ: "JWT" }, payload: { sub: "1234567890", name: "Albert Lee", iat: 1516239022 }, signature: "HMACSHA256(base64UrlEncode(header) + '.' + base64UrlEncode(payload), secret)" };
To implement JWT in your application, you’ll start by creating a JWT upon successful authentication. This typically occurs after verifying user credentials, as shown in the login function below:
// Example of generating a JWT after user authentication const jwt = require('jsonwebtoken'); const secretKey = 'your-256-bit-secret'; async function loginUser(username, password) { const user = await findUserByUsername(username); if (user && await verifyPassword(password, user.hashedPassword)) { // Generate a token const token = jwt.sign({ id: user.id, username: user.username }, secretKey, { expiresIn: '1h' }); return { success: true, token: token }; } return { success: false, message: "Invalid username or password." }; }
Once the token is generated, it can be sent back to the client, who stores it (usually in local storage or a cookie). For subsequent requests to protected endpoints, the client includes the JWT in the Authorization header:
// Example of sending a JWT in a request async function fetchProtectedResource() { const token = localStorage.getItem('authToken'); const response = await fetch("https://api.example.com/protected-resource", { method: "GET", headers: { "Authorization": "Bearer " + token } }); if (response.ok) { const data = await response.json(); console.log(data); } else { console.error("Failed to fetch protected resource."); } }
On the server-side, when a request arrives with a JWT, the application must verify the token to ensure it’s valid. This involves checking the signature and decoding the payload to extract the user information. Here’s how you can implement this verification:
// Example of JWT verification middleware in an Express.js application const express = require('express'); const jwt = require('jsonwebtoken'); const app = express(); app.use((req, res, next) => { const token = req.headers['authorization']?.split(' ')[1]; if (!token) return res.status(403).send('Token required.'); jwt.verify(token, secretKey, (err, decoded) => { if (err) return res.status(401).send('Invalid token.'); req.user = decoded; // Store user info in request next(); }); }); // Protected route app.get('/protected', (req, res) => { res.send(`Hello ${req.user.username}, you have access!`); });
By using JWTs, developers can build applications that not only authenticate users efficiently but also scale effectively without the need to maintain session state server-side. The stateless nature of JWTs allows for easy horizontal scaling, making them an attractive option in cloud-based architectures. However, it is important to handle JWTs securely, ensuring they are properly encrypted, have appropriate expiration times, and are validated correctly to thwart common vulnerabilities.
Using OAuth 2.0 for Third-Party Authentication
OAuth 2.0 is a widely adopted authorization framework that provides a secure and efficient way for users to log into applications using their existing credentials from trusted third-party providers, such as Google, Facebook, or GitHub. This is not merely a convenience; it’s a significant enhancement in terms of both user experience and security. By using OAuth 2.0, developers can delegate the authentication responsibility to these trusted providers, reducing the risk associated with managing credentials directly.
The OAuth 2.0 flow usually begins with the client application redirecting the user to the authorization server of the third-party provider. The user then logs in and grants permission for the client application to access their information. Upon successful authentication and authorization, the user is redirected back to the client application with an authorization code or an access token.
To integrate OAuth 2.0 into a JavaScript application, you’ll need to follow several key steps:
function initiateOAuthFlow() { const clientId = "YOUR_CLIENT_ID"; const redirectUri = "YOUR_REDIRECT_URI"; const scope = "email profile"; const authorizationUrl = `https://provider.com/oauth/authorize?client_id=${clientId}&redirect_uri=${redirectUri}&scope=${scope}`; window.location.href = authorizationUrl; // Redirect to provider }
Once the user grants access, they are redirected back to your specified redirect URI with an authorization code. Your application can then exchange this code for an access token by making a request to the provider’s token endpoint. This token is then used to authenticate requests to the provider’s API on behalf of the user.
async function fetchAccessToken(authorizationCode) { const tokenResponse = await fetch("https://provider.com/oauth/token", { method: "POST", headers: { "Content-Type": "application/x-www-form-urlencoded" }, body: new URLSearchParams({ code: authorizationCode, client_id: "YOUR_CLIENT_ID", client_secret: "YOUR_CLIENT_SECRET", redirect_uri: "YOUR_REDIRECT_URI", grant_type: "authorization_code" }) }); if (tokenResponse.ok) { const { access_token } = await tokenResponse.json(); return access_token; } throw new Error("Failed to fetch access token."); }
With the access token in hand, you can now make authenticated requests to the provider’s API to retrieve user data, such as their email address or profile information, which can then be used to create or update a user account in your application:
async function fetchUserProfile(accessToken) { const profileResponse = await fetch("https://provider.com/user", { method: "GET", headers: { "Authorization": `Bearer ${accessToken}` } }); if (profileResponse.ok) { const userProfile = await profileResponse.json(); console.log(userProfile); return userProfile; } throw new Error("Failed to fetch user profile."); }
While OAuth 2.0 significantly simplifies user authentication and enhances security, it’s essential to implement it carefully to mitigate potential risks. For instance, always validate the access token upon receipt, check the token’s expiration, and ensure that sensitive operations occur only over HTTPS to safeguard against token interception. Moreover, maintain a clear understanding of the scopes you request, as excessive permissions can lead to privacy concerns for users.
Overall, using OAuth 2.0 not only improves security by reducing the need for your application to handle passwords but also enhances user experience, as users can quickly log in using familiar credentials from trusted sources. This integration positions your application to be more secure, effortless to handle, and compliant with contemporary authentication standards.
Best Practices for Secure User Authentication
Implementing best practices for secure user authentication is paramount in protecting sensitive user data and ensuring the integrity of your web applications. A single vulnerability can lead to catastrophic breaches, making it essential to adopt a multifaceted approach. Here are several best practices when it comes to user authentication in JavaScript applications.
1. Use Strong Password Policies
Establishing a robust password policy is the first line of defense against unauthorized access. Encourage users to create complex passwords that include a mix of uppercase letters, lowercase letters, numbers, and special characters. Additionally, ponder implementing a minimum password length (at least 8-12 characters) and enforcing password expiration and renewal policies.
// Example of password validation function function isPasswordStrong(password) { const regex = /^(?=.*[a-z])(?=.*[A-Z])(?=.*d)(?=.*[@$!%*?&])[A-Za-zd@$!%*?&]{8,}$/; return regex.test(password); }
2. Implement Two-Factor Authentication (2FA)
Two-factor authentication adds an extra layer of security by requiring users to provide a second form of verification, typically something they have (like a mobile device). This drastically reduces the chances of unauthorized access, as an attacker would need both the password and the second factor (e.g., a one-time code sent via SMS or generated by an authenticator app).
// Example of sending a 2FA code via SMS async function sendTwoFactorCode(phoneNumber) { const code = generateRandomCode(); // Implement your random code generation await sendSMS(phoneNumber, code); // Use your SMS API to send the code return code; }
3. Secure Password Storage
As previously mentioned, it is critical to never store passwords in plaintext. Use secure hashing algorithms, such as bcrypt, to hash passwords before storing them in your database. This ensures that even if the database is compromised, the passwords remain secure.
// Example of hashing a password const bcrypt = require('bcrypt'); async function hashPassword(password) { const saltRounds = 10; const hashedPassword = await bcrypt.hash(password, saltRounds); return hashedPassword; }
4. Use HTTPS for Secure Communication
Always serve your application over HTTPS. This encrypts the data transmitted between the client and server, protecting it from interception and man-in-the-middle attacks. Modern browsers often warn users when a site is not secure, which can deter them from using your application.
5. Implement Rate Limiting and Account Lockout
To mitigate brute-force attacks, implement rate limiting on your authentication endpoints. This limits the number of login attempts from a single IP address over a specified time period. Additionally, think locking user accounts after a defined number of failed attempts, notifying users of the lockout and providing a mechanism for recovery.
// Example of rate limiting with express-rate-limit const rateLimit = require('express-rate-limit'); const loginLimiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 5, // Limit each IP to 5 login attempts per windowMs message: "Too many login attempts, please try again later." }); app.post('/login', loginLimiter, (req, res) => { // Authentication logic here });
6. Regularly Update Dependencies
In an ever-evolving security landscape, it is vital to keep your dependencies up to date. Outdated libraries can harbor vulnerabilities that attackers can exploit. Regularly review your project dependencies for updates and security vulnerabilities, and apply patches as necessary.
7. Monitor and Log Authentication Events
Implement logging and monitoring for authentication activities. This includes logging successful and failed login attempts, password changes, and 2FA verifications. Monitoring these events can help identify suspicious activities early and enable timely responses to potential breaches.
// Example of logging authentication events const fs = require('fs'); function logAuthEvent(event) { const logEntry = `${new Date().toISOString()}: ${event}n`; fs.appendFile('auth.log', logEntry, (err) => { if (err) console.error("Could not log event", err); }); }
8. Educate Users
User education is another vital aspect of securing user authentication. Help users understand the importance of password security, the risks of phishing, and how to recognize suspicious activities. Providing resources and guidance can empower users to take an active role in protecting their accounts.
By adhering to these best practices for secure user authentication, developers can significantly enhance the security posture of their applications. It is a continuous process that requires vigilance, adaptability, and a proactive approach to addressing emerging threats within the scope of web security.
Handling User Sessions and State Management in JavaScript
Handling user sessions and state management in JavaScript is a critical aspect of maintaining secure and efficient web applications. After a user has successfully authenticated, the application must manage the user’s session to ensure that their state is preserved across different parts of the application. This involves not only tracking user state but also ensuring the integrity and security of that state.
In a typical web application, sessions can be managed in various ways. The most common methods include cookie-based sessions and token-based sessions. Each method has its pros and cons, and the choice depends on the application’s architecture and security requirements.
Cookie-based sessions are established when the server generates a session ID after user authentication. This session ID is sent to the client as a cookie and is included in subsequent requests. The server can then use this session ID to identify the user and retrieve their session data stored on the server. While this method is simpler, it can lead to scalability issues, especially in distributed systems, where session data must be consistently accessible across multiple servers.
// Example of setting a cookie for session management function setSessionCookie(sessionId) { document.cookie = `sessionId=${sessionId}; path=/; secure; HttpOnly; SameSite=Strict`; }
Token-based sessions, on the other hand, utilize JSON Web Tokens (JWTs) or similar mechanisms, allowing for a stateless approach. After user authentication, the server issues a token that the client stores (typically in local storage or a cookie). This token is then sent with each subsequent request, allowing the server to authenticate the user without maintaining session state. This approach simplifies scalability, as the server does not need to track session data for each user.
// Example of storing a JWT in local storage async function storeToken(token) { localStorage.setItem('authToken', token); }
Regardless of the session management method chosen, it is vital to ensure that sessions are secure. This includes implementing mechanisms to handle session expiration and invalidation. Sessions should have a limited lifetime—either by setting an expiration date on cookies or defining a token expiration time. When a session expires, the user should be required to re-authenticate, thereby reducing the risk of unauthorized access.
// Example of checking token expiration function isTokenExpired(token) { const payload = JSON.parse(atob(token.split('.')[1])); return Date.now() >= payload.exp * 1000; }
Another critical aspect of session management is protecting against Cross-Site Request Forgery (CSRF) attacks, which can exploit authenticated sessions. To mitigate this risk, developers can implement CSRF tokens that are validated on the server side. This token should be included in requests that modify state, ensuring that the request is legitimate and originates from an authenticated user.
// Example of including a CSRF token in a request async function submitForm(data) { const csrfToken = getCsrfToken(); // Function to get the CSRF token await fetch('/api/submit', { method: 'POST', headers: { 'Content-Type': 'application/json', 'X-CSRF-Token': csrfToken }, body: JSON.stringify(data) }); }
In addition to security, effective state management involves maintaining a consistent user experience. This includes implementing logic to check session validity before allowing access to protected routes and providing users with feedback when their session is about to expire. This proactive approach can lead to a smoother user experience and reduce confusion over unexpected logouts.
Lastly, ponder employing libraries and frameworks that provide built-in support for session management. Tools like Redux for state management in React applications can be configured to facilitate user session handling, allowing developers to focus on building features rather than managing session complexity.
Handling user sessions and state management in JavaScript applications is a nuanced task that requires a keen understanding of both security considerations and user experience. By choosing appropriate session management strategies, implementing strong security measures, and ensuring a seamless user experience, developers can create robust applications that effectively meet the needs of their users.