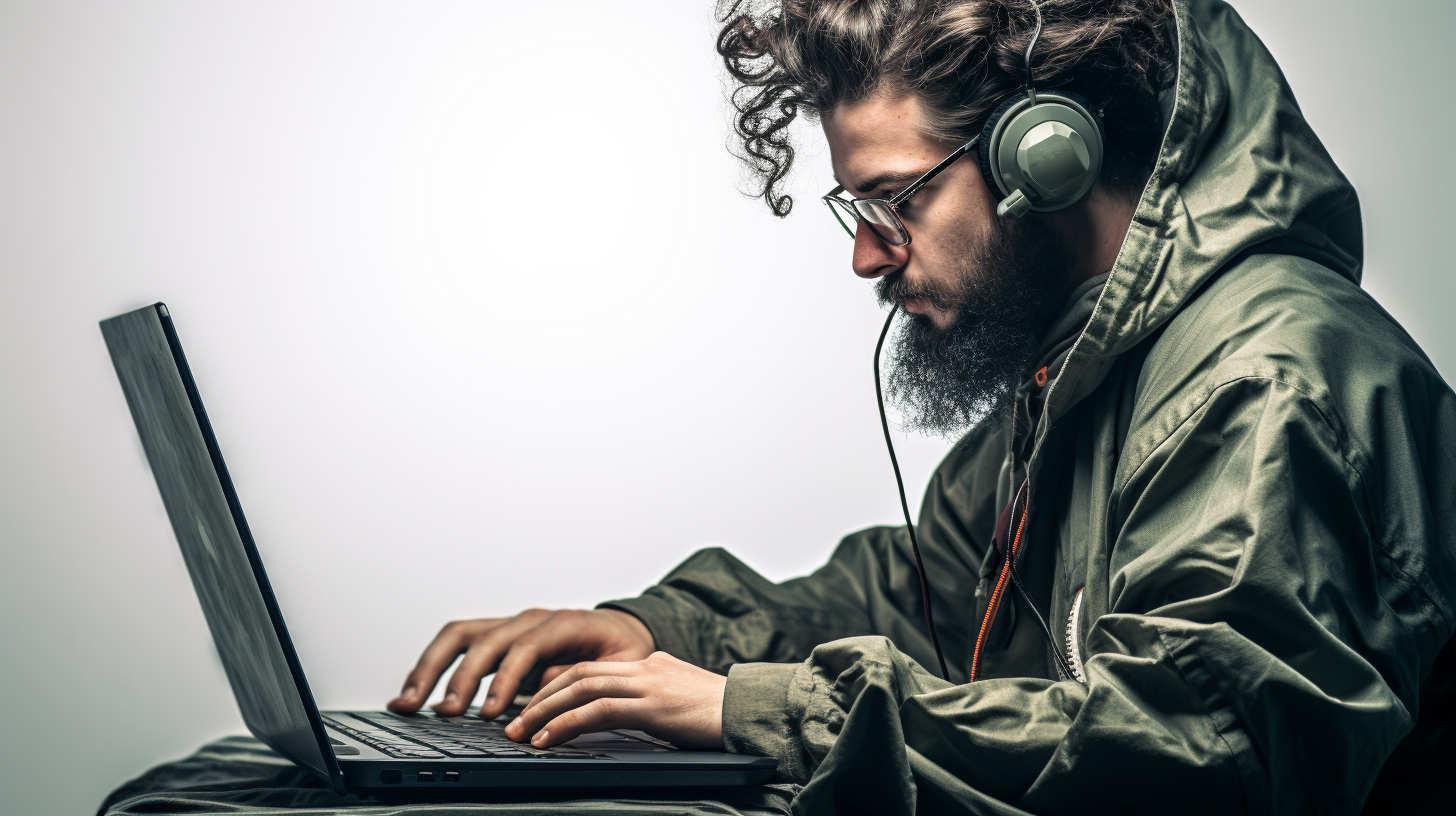
JavaScript and Voice Recognition
Voice recognition technology has traversed a remarkable journey from its nascent stages to the sophisticated systems we recognize today. In the early days, voice recognition attempts were rudimentary, relying heavily on analog signals and basic pattern recognition techniques. These systems struggled with the nuances of human speech, often requiring users to dictate words in a very controlled manner.
As computer processing power surged and algorithms advanced, the 1990s marked a significant leap forward. This era saw the introduction of more complex statistical models, such as Hidden Markov Models (HMMs), which enabled systems to learn from vast datasets of spoken language. This improvement allowed for more natural speech patterns and a broader vocabulary, making voice recognition more accessible to the general public.
The dawn of the 21st century brought with it the rise of machine learning and neural networks. These technologies further enhanced the accuracy of voice recognition systems. The advent of deep learning revolutionized the field, allowing systems to understand context and intonation significantly better. Companies like Google, Apple, and Amazon began to integrate voice recognition into their products, leading to the development of intelligent assistants like Siri, Google Assistant, and Alexa.
With the rise of the internet and cloud computing, voice recognition technology has become even more powerful. Real-time processing of voice commands became feasible through cloud-based solutions, which allow for quick access to vast amounts of data. This evolution has empowered developers to create applications that can seamlessly integrate voice capabilities, enhancing user experiences across various platforms.
Integrating Voice Recognition with JavaScript
Integrating voice recognition into JavaScript applications opens up a world of possibilities for developers looking to provide users with more interactive and intuitive experiences. The Web Speech API is at the forefront of this integration, providing a practical interface for incorporating speech recognition and synthesis directly into web applications.
To get started, the first step is to check if the Web Speech API is supported in the user’s browser. Not all browsers may support speech recognition, so it’s crucial to implement a fallback mechanism or inform users if their browser does not support this feature.
const SpeechRecognition = window.SpeechRecognition || window.webkitSpeechRecognition; if (!SpeechRecognition) { console.error("Speech Recognition API not supported in this browser."); } else { const recognition = new SpeechRecognition(); recognition.lang = 'en-US'; // Set the language for recognition recognition.interimResults = false; // Set to true to get interim results recognition.maxAlternatives = 1; // Maximum number of alternatives to return }
Once the API is confirmed to be available, the next step is to configure the recognition settings. You can specify the recognition language, whether to receive interim results, and how many alternative interpretations of the speech should be provided. The configuration allows for nuanced control over the user experience.
Starting the speech recognition process is simpler. You invoke the start
method on the recognition object. This begins capturing audio input from the user’s microphone. It’s also essential to handle various events such as onresult
, onerror
, and onend
to manage the application’s behavior based on the speech recognition results.
recognition.onstart = () => { console.log("Voice recognition activated. Try speaking into the microphone."); }; recognition.onresult = (event) => { const transcript = event.results[0][0].transcript; console.log("You said: " + transcript); // Handle the recognized speech (e.g., process commands, search queries) }; recognition.onerror = (event) => { console.error("Error occurred in speech recognition: " + event.error); }; recognition.onend = () => { console.log("Speech recognition service disconnected"); }; // Start recognition recognition.start();
To create a more engaging experience, you may want to trigger specific actions based on the recognized text. For instance, if the user says “play music,” you could integrate a function to start playing audio. This requires parsing the transcript and mapping it to corresponding actions in your application.
function handleCommand(command) { switch(command.toLowerCase()) { case 'play music': playMusic(); break; case 'stop music': stopMusic(); break; // Add more commands as needed default: console.log("Command not recognized."); } } // Call this function with the recognized transcript handleCommand(transcript);
Key Libraries and APIs for Voice Recognition
In the sphere of voice recognition with JavaScript, using key libraries and APIs can dramatically enhance the functionality and user experience of web applications. The most notable among these is the Web Speech API, which provides developers with both speech recognition and speech synthesis capabilities. This API is a potent tool designed to bridge the gap between human communication and machine understanding, allowing for seamless integration of voice commands into web interfaces.
When you ponder of voice recognition in the context of JavaScript, the Web Speech API immediately comes to mind. This API is particularly attractive due to its native support in modern browsers, fostering accessibility without the need to rely on external libraries. It consists of two primary components: the SpeechRecognition interface for recognizing spoken language and the SpeechSynthesis interface for generating spoken output from text.
Here’s a basic example of how to use the SpeechRecognition interface:
const SpeechRecognition = window.SpeechRecognition || window.webkitSpeechRecognition; if (SpeechRecognition) { const recognition = new SpeechRecognition(); recognition.lang = 'en-US'; recognition.interimResults = false; recognition.maxAlternatives = 1; recognition.onstart = () => { console.log("Voice recognition activated. Try speaking."); }; recognition.onresult = (event) => { const transcript = event.results[0][0].transcript; console.log("You said: " + transcript); handleCommand(transcript); }; recognition.onerror = (event) => { console.error("Error: " + event.error); }; recognition.onend = () => { console.log("Voice recognition has stopped."); }; // Start recognition recognition.start(); } else { console.error("Speech Recognition API is not supported in this browser."); }
In addition to the Web Speech API, several other libraries can extend the functionality of voice recognition within JavaScript applications. Libraries such as annyang and responsivevoice.js simplify the integration of voice commands and speech synthesis, respectively. Annyang is particularly useful for developers who want to implement voice commands with minimal boilerplate code.
Here’s a simple usage example with annyang:
if (annyang) { // Define the commands const commands = { 'hello': () => { console.log('Hello!'); }, 'play music': () => { playMusic(); }, 'stop music': () => { stopMusic(); }, // Add more commands as needed }; // Add the commands to annyang annyang.addCommands(commands); // Start listening annyang.start(); } else { console.error("Annyang is not supported in this browser."); }
These libraries not only reduce the complexity of working with the Web Speech API but also provide a more effortless to handle interface for defining and managing voice commands. In an increasingly interactive web environment, these tools empower developers to create applications that respond to voice inputs more naturally.
Furthermore, when considering speech synthesis, the SpeechSynthesis interface complements the recognition capabilities, allowing applications to ‘speak’ back to users. This can be particularly useful in applications such as virtual assistants or educational platforms where feedback and interaction are key.
For example, implementing speech synthesis is as simpler as following this snippet:
const utterance = new SpeechSynthesisUtterance('Hello, how can I assist you today?'); speechSynthesis.speak(utterance);
Practical Applications of Voice Recognition in Web Development
Voice recognition technology has found its way into numerous practical applications in web development, transforming user interactions and enhancing accessibility. By enabling hands-free control and voice commands, developers can create more intuitive interfaces that respond to natural language inputs. This not only improves user experience but also opens up new avenues for engaging with applications.
One of the most common practical applications of voice recognition is in the context of virtual assistants. These applications allow users to perform tasks simply by talking. For instance, an e-commerce website can integrate voice commands to facilitate product searches, add items to the cart, and even complete purchases. This kind of functionality not only streamlines the shopping process but also caters to users with disabilities, increasing overall usability.
Ponder the following implementation where a voice-activated search feature is integrated into a web application:
const SpeechRecognition = window.SpeechRecognition || window.webkitSpeechRecognition; if (SpeechRecognition) { const recognition = new SpeechRecognition(); recognition.lang = 'en-US'; recognition.interimResults = false; recognition.onresult = (event) => { const transcript = event.results[0][0].transcript; console.log("You said: " + transcript); performSearch(transcript); // Function to handle search logic }; recognition.start(); } else { console.error("This browser does not support speech recognition."); } function performSearch(query) { // Logic to perform search based on the voice input console.log("Searching for: " + query); // Implement search functionality here }
Another fascinating application lies within the realm of accessibility. Voice recognition can significantly aid users with limited mobility or those who prefer voice commands over traditional input methods. By incorporating voice-to-text functionality, developers can enable users to dictate messages or documents directly into text fields, enhancing productivity and ease of use.
Here’s a simple implementation of a voice-to-text feature that captures user speech and displays it in a text area:
const recognition = new SpeechRecognition(); recognition.lang = 'en-US'; recognition.interimResults = false; recognition.onresult = (event) => { const transcript = event.results[0][0].transcript; document.getElementById("textArea").value += transcript + ' '; // Append to text area }; recognition.start();
Moreover, educational platforms have also harnessed voice recognition technology to create interactive learning environments. For instance, language learning applications can incorporate speech recognition to help users practice pronunciation. By listening to the user’s spoken words and providing real-time feedback, these applications can enhance learning experiences and outcomes.
Imagine a simple educational app that evaluates a user’s pronunciation:
const evaluatePronunciation = (expected, actual) => { // Basic evaluation logic return expected === actual ? "Correct!" : "Try again."; }; recognition.onresult = (event) => { const userInput = event.results[0][0].transcript; console.log(evaluatePronunciation("hello", userInput)); // Replace "hello" with the expected phrase };
Finally, incorporating voice commands in gaming applications has also started gaining traction. Players can control game actions using their voice, creating a more immersive experience. By mapping specific commands to in-game actions, developers can engage users on a deeper level.
As a practical example, consider how a voice-activated gaming command might be implemented:
const commands = { 'jump': () => { gameCharacter.jump(); }, 'run': () => { gameCharacter.run(); }, // Additional commands can be added here }; recognition.onresult = (event) => { const command = event.results[0][0].transcript.toLowerCase(); if (commands[command]) { commands[command](); // Execute the corresponding action } };
Challenges and Limitations of Voice Recognition in JavaScript
While the integration of voice recognition technology into JavaScript applications provides remarkable advantages, it isn’t without its challenges and limitations. Understanding these hurdles is essential for developers aiming to create robust applications that use voice interaction effectively.
One significant challenge is the inherent variability in human speech. Accents, dialects, and speech patterns differ widely from person to person, which can lead to recognition errors. For instance, a single word may be pronounced differently by speakers from various regions, and the speech recognition algorithms must be capable of adapting to this diversity. Even with advanced machine learning models, achieving perfect recognition across a broad spectrum of users remains a complex task.
Another limitation stems from background noise. Voice recognition systems, particularly those relying on microphone input, can struggle to discern speech from ambient sounds. In noisy environments, the accuracy of the recognition can significantly degrade. Developers must consider incorporating noise-cancellation techniques or prompting users to speak in quieter settings to mitigate this issue.
Latency is also a pressing concern. Real-time speech recognition requires quick processing and response times, but delays can occur due to network issues, especially when using cloud-based services. Such latency can disrupt user experience, leading to frustration and disengagement. Developers should optimize their applications to handle these scenarios, possibly by implementing local recognition when feasible.
Furthermore, privacy and security issues are paramount when dealing with voice data. Users may be hesitant to engage with applications that process their voice commands due to concerns about data collection and misuse. It’s important for developers to communicate transparently about how voice data is used and stored, ensuring users feel secure while using their applications.
Additionally, the dependency on web browsers to support the Web Speech API presents another limitation. Not all browsers offer the same level of support for voice recognition features, which can lead to inconsistent experiences across platforms. Developers must implement fallback mechanisms or alternative solutions when users access their applications with unsupported browsers.
Lastly, the technical complexity involved in creating a seamless voice interface can be daunting. Developers may face challenges not only in the speech recognition aspect but also in integrating these features confidently into existing applications. This requirement for additional expertise can be a barrier, particularly for smaller teams or individual developers.
To illustrate, think the following potential structure for handling errors and ensuring compatibility:
const recognition = new (window.SpeechRecognition || window.webkitSpeechRecognition)(); if (!recognition) { alert("Speech Recognition is not supported in this browser."); } else { recognition.onerror = (event) => { switch (event.error) { case 'no-speech': console.error("No speech detected. Please try again."); break; case 'audio-capture': console.error("Audio capture failed. Ensure your microphone is working."); break; case 'not-allowed': console.error("Permission to use microphone is denied."); break; default: console.error("Error occurred in speech recognition: " + event.error); } }; }
The Future of Voice Interaction in Web Applications
The future of voice interaction in web applications is poised for a radical transformation, driven by continued advancements in artificial intelligence, natural language processing, and user interface design. As we look ahead, several trends and innovations are on the horizon that promise to redefine how we interact with technology through voice.
One significant trend is the increasing accuracy and contextual understanding of voice recognition systems. Using the power of deep learning and neural networks, future applications will likely be able to comprehend not just words but the intent behind them. This capability will enable more natural conversations, allowing users to interact with applications in a fluid and conversational manner. The integration of context-aware processing will allow these systems to remember user preferences and past interactions, tailoring responses to individual users.
Moreover, the proliferation of smart devices and the Internet of Things (IoT) will further integrate voice interaction into everyday life. As homes and workplaces become smarter, voice commands will be at the heart of navigating everything from lighting to appliances. This shift will redefine web applications as integral hubs that not only respond to commands but also intelligently anticipate user needs based on patterns of behavior and context.
const commands = { 'turn on the lights': () => { smartHomeSystem.lights.on(); }, 'set the thermostat to 72 degrees': () => { smartHomeSystem.thermostat.setTemperature(72); }, 'play my favorite playlist': () => { musicApp.playPlaylist('Favorites'); } };
Additionally, advancements in multilingual support will broaden the accessibility of voice interactions. Applications will increasingly support multiple languages and dialects, breaking down barriers for non-English speakers and making technology more inclusive. This push will also involve understanding cultural nuances and adapting speech recognition models accordingly.
Another exciting development is the rise of emotion-aware voice recognition. Future systems may use sentiment analysis to gauge users’ emotions based on their tone and speech patterns. This capability could enable applications to respond empathetically, adjusting interactions based on whether a user is frustrated, happy, or confused. In customer service, for example, an application could detect frustration in a user’s voice and escalate the issue to a human representative, enhancing user satisfaction.
const analyzeEmotion = (transcript) => { const emotion = sentimentAnalysis(transcript); // Hypothetical function for sentiment analysis if (emotion === 'frustration') { escalateToHumanSupport(); } else { handleCommand(transcript); } };
Moreover, the integration of voice interaction with augmented reality (AR) and virtual reality (VR) technologies will create immersive experiences that blend physical and digital realms. Users will be able to control virtual environments using voice commands, providing a more intuitive way to navigate these digital landscapes. This integration will open new avenues for gaming, education, and training simulations where voice commands enhance the user experience in profound ways.
Security and privacy will also take center stage in the future of voice recognition. As concerns about data privacy grow, applications will be designed with robust security measures, ensuring that voice data is processed and stored securely. Innovations such as on-device processing, where voice recognition occurs locally rather than in the cloud, will minimize data exposure and enhance user trust.
const recognition = new (window.SpeechRecognition || window.webkitSpeechRecognition)(); recognition.onresult = (event) => { const transcript = event.results[0][0].transcript; processLocally(transcript); // Hypothetical function for local processing };
Lastly, as developers continue to explore the potential of voice interaction, the tools and libraries available will evolve to facilitate integration and create richer user experiences. New APIs and frameworks will emerge, designed specifically for voice user interfaces, making it easier for developers to implement sophisticated voice recognition capabilities without deep expertise in the underlying technologies.