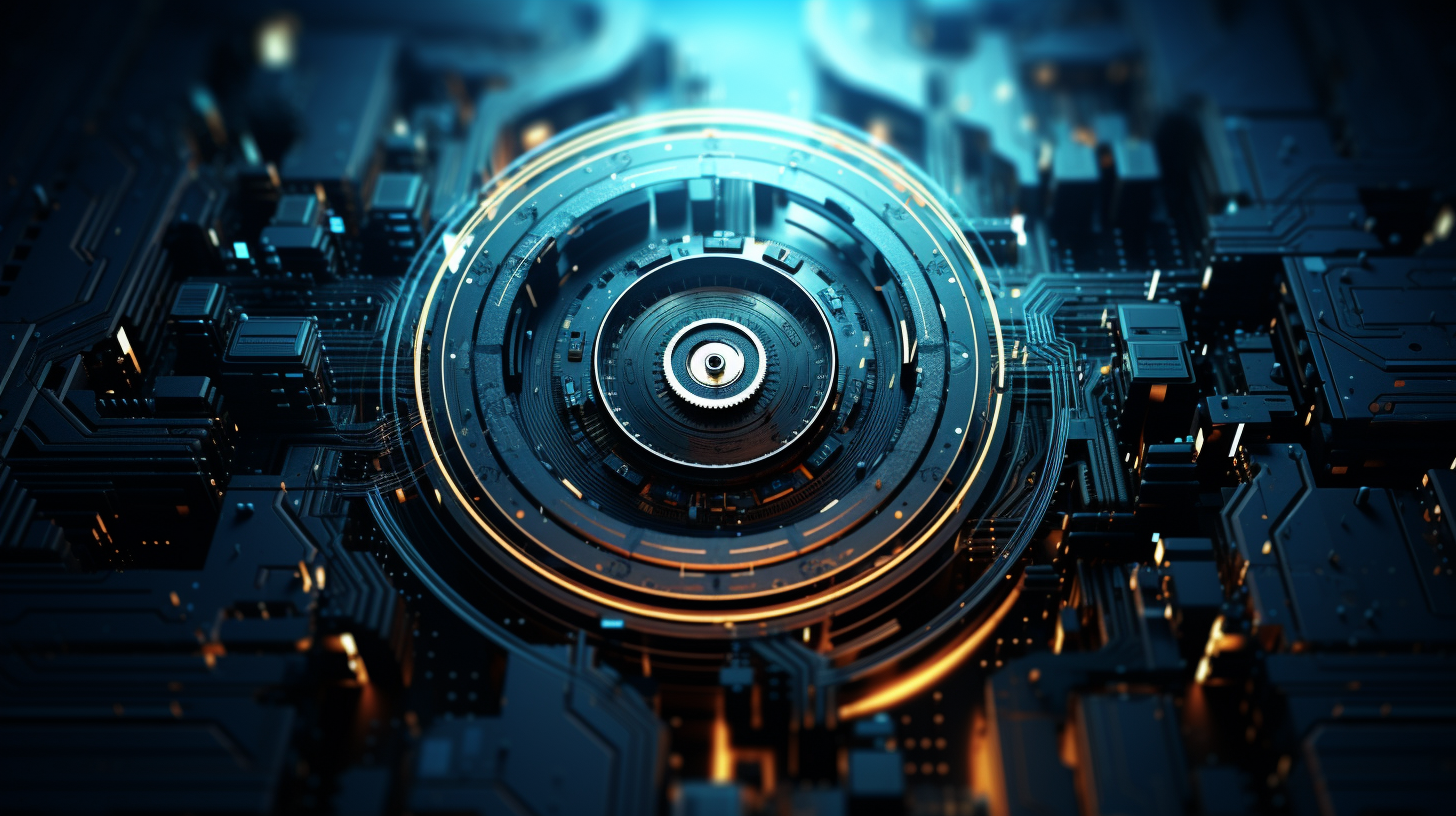
JavaScript and Web Crawling
Web crawling, at its essence, is the automated process of navigating the web to collect data. Think of it as the digital equivalent of an explorer charting new territories. Web crawlers, often called spiders or bots, systematically browse the vast expanse of the internet, following links from one page to another, gathering information, and indexing content for search engines.
The primary function of a web crawler is to discover and retrieve web pages. They start with a set of known URLs (often referred to as seeds) and use those as a launching point. By parsing the HTML of each page, crawlers identify hyperlinks and add them to their list of URLs to visit next. This recursive nature allows them to cover a significant portion of the web efficiently.
For developers, understanding how web crawlers function very important, particularly when it comes to ensuring that their websites are indexed properly. The fundamental principles of web crawling include:
- Crawlers discover new URLs through hyperlinks present in the pages they visit.
- Once a page is accessed, its content is analyzed to extract relevant information and links.
- The information gathered is stored in a structured format, often in a database, for further analysis or indexing.
- Crawlers should respect the rules set in a website’s
robots.txt
file, which indicates allowable paths for crawling and those that should be ignored.
The typical lifecycle of a web crawler can be summarized as follows:
const crawler = { seeds: ['http://example.com'], visited: new Set(), async crawl(url) { if (this.visited.has(url)) return; this.visited.add(url); const content = await fetch(url).then(res => res.text()); const links = this.extractLinks(content); for (const link of links) { await this.crawl(link); } }, extractLinks(html) { const linkRegex = /<a href="([^"]+)"/g; let links = []; let match; while ((match = linkRegex.exec(html)) !== null) { links.push(match[1]); } return links; } }; crawler.crawl(crawler.seeds[0]);
This rudimentary implementation of a web crawler fetches a given URL, extracts hyperlinks, and recursively crawls each link, ensuring that it does not revisit any URLs. However, real-world web crawling requires much more sophistication, including handling different protocols, managing request rates, and dealing with errors.
The foundational aspects of web crawling revolve around URL management, data retrieval, and content parsing, all while adhering to ethical standards set by the web community. As the internet continues to evolve, so too do the techniques and technologies that support effective web crawling.
JavaScript’s Role in Dynamic Content Loading
JavaScript plays a pivotal role in the modern web, especially when it comes to dynamic content loading. With the advent of single-page applications (SPAs) and JavaScript frameworks like React, Angular, and Vue.js, more and more content is being generated and displayed on-the-fly, rather than being loaded as static HTML. This shift necessitates a nuanced approach to web crawling since traditional crawlers may struggle with content that’s rendered after the initial page load.
Dynamic content loading typically occurs through AJAX calls or WebSocket connections, where data is fetched asynchronously in response to user actions or application state changes. Consequently, crawlers need the capability to execute JavaScript and wait for content to fully load before scraping it. That is where headless browsers come into play, allowing developers to simulate a real user’s experience in a browser environment.
When a crawler encounters a JavaScript-heavy webpage, it needs to wait for the JavaScript to execute and for the content to load completely. Tools like Puppeteer or Playwright can be leveraged to automate browser interactions and extract content effectively. These libraries provide the ability to control headless instances of browsers, enabling crawlers to navigate through complex applications just like a user would.
const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('http://example.com'); // Wait for a specific element to load await page.waitForSelector('.dynamic-content'); // Extract HTML content const content = await page.content(); console.log(content); await browser.close(); })();
In this example, the crawler uses Puppeteer to launch a headless browser, navigate to a given URL, and wait for the element containing dynamic content to load. By ensuring that the necessary content is present before extraction, the crawler can capture data that would otherwise be missed.
Moreover, it’s essential to understand the timing and order of events in JavaScript applications. Many frameworks employ patterns such as lazy loading, where content is loaded as the user interacts with the page. Thus, a well-designed crawler must be able to simulate these user interactions—scrolling, clicking buttons, or navigating through the site structure—to ensure that all relevant data is gathered.
await page.evaluate(() => { window.scrollBy(0, window.innerHeight); }); // Perform actions like clicking a button await page.click('#loadMoreButton');
This code snippet demonstrates how the crawler can programmatically scroll down the page and interact with a button to load more content. Understanding these interactions can significantly enhance the effectiveness of a web crawler working with JavaScript-rendered pages.
As web developers continue to embrace JavaScript for crafting user experiences, the challenge for crawlers is to keep pace with these dynamic environments. Equipped with the right tools and techniques, JavaScript can be used to create sophisticated crawlers capable of navigating and extracting meaningful data from even the most complex web applications.
Techniques for Web Crawling with JavaScript
Within the scope of web crawling, especially when using JavaScript, there are several techniques that developers can implement to effectively navigate and extract data from websites. These techniques help in crafting robust crawlers capable of handling various challenges posed by modern web architectures.
1. Asynchronous Crawling
JavaScript inherently supports asynchronous programming, which especially important for web crawling. By using JavaScript’s asynchronous features, such as async/await
and Promises
, crawlers can initiate multiple requests simultaneously, significantly reducing the time taken to gather data from multiple sources.
async function fetchUrls(urls) { const fetchPromises = urls.map(url => fetch(url).then(response => response.text())); return await Promise.all(fetchPromises); }
2. Rate Limiting
To prevent being blocked by a target website, implementing rate limiting within the crawler is paramount. This technique involves controlling the frequency of requests sent to a server, ensuring that the crawler operates within acceptable bounds while still progressing efficiently.
function rateLimit(fn, limit) { let lastCall = 0; return function(...args) { const now = Date.now(); if (now - lastCall >= limit) { lastCall = now; return fn(...args); } }; }
3. User-Agent Rotation
Websites often use the User-Agent string to determine the source of traffic. By rotating User-Agents, crawlers can mimic requests from different browsers or devices, making them less detectable and reducing the chance of getting blocked.
const userAgents = ['Mozilla/5.0', 'Chrome/91.0', 'Safari/537.36']; function getRandomUserAgent() { return userAgents[Math.floor(Math.random() * userAgents.length)]; }
4. Handling JavaScript Execution
Many modern websites utilize JavaScript frameworks that render content dynamically. To handle this, crawlers can use headless browsers, such as Puppeteer or Playwright, which allow JavaScript to execute in a controlled environment. This ensures that all dynamically loaded content is captured during the crawling process.
const puppeteer = require('puppeteer'); async function crawlWithPuppeteer(url) { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto(url); const content = await page.content(); await browser.close(); return content; }
5. Using APIs Where Available
Many websites offer APIs that provide structured data in a more accessible format than scraping HTML. Whenever possible, using these APIs can not only simplify the data retrieval process but also ensure compliance with the site’s terms of service.
async function fetchFromApi(apiUrl) { const response = await fetch(apiUrl); const data = await response.json(); return data; }
By applying these techniques, developers can enhance their web crawlers, making them more efficient, respectful of server limits, and capable of obtaining the data they require from a wide array of web applications. Embracing the dynamic capabilities of JavaScript allows for the creation of intelligent crawlers that can adapt to the complexities of modern web content.
Handling JavaScript-Rendered Pages
Handling JavaScript-rendered pages can pose a unique set of challenges for web crawlers. Traditional crawlers that solely parse HTML at the initial request might miss content this is dynamically loaded through JavaScript after the page has been rendered. In modern web applications, particularly those built with frameworks like React, Angular, or Vue.js, much of the content is generated on the fly. This means that as a crawler, merely fetching the static HTML might not provide the complete picture.
To effectively crawl JavaScript-rendered pages, a common approach is to utilize headless browsers. These tools can simulate a full web browser environment, executing JavaScript and allowing the crawler to retrieve the final rendered HTML. Popular libraries such as Puppeteer and Playwright provide the capability to automate browser actions, making it easier to navigate through pages and extract content.
Here’s an example of how you can use Puppeteer to handle JavaScript-rendered pages:
const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('http://example.com'); // Wait for a specific element to load await page.waitForSelector('.dynamic-content'); // Extract the rendered HTML const content = await page.content(); console.log(content); await browser.close(); })();
In this example, we launch a headless browser with Puppeteer, navigate to a given URL, and wait for a specific element that is expected to be dynamically loaded. After the element is present, we retrieve the complete HTML content of the page, which now includes the dynamically rendered sections.
Another effective technique is to use a JavaScript library like Axios to fetch the data directly from the APIs that power these dynamic pages. Many modern web applications rely on backend APIs to fetch data, and by understanding how these APIs work, you can bypass the rendering process entirely and get the data you need more efficiently.
Here is a simple example of using Axios to fetch JSON data from an API:
const axios = require('axios'); (async () => { try { const response = await axios.get('http://example.com/api/data'); const data = response.data; console.log(data); } catch (error) { console.error('Error fetching data:', error); } })();
This method of extracting data directly from APIs can be significantly faster than rendering the entire page, especially if the target data is available through a dedicated endpoint. However, it’s important to be aware of the site’s terms of service and robots.txt rules before proceeding with this approach.
Overall, handling JavaScript-rendered pages requires a deeper understanding of how modern web applications function. By using headless browsers or directly querying APIs, you can effectively gather the content necessary for your crawling needs, ensuring that no valuable data is left behind.
Best Practices for Ethical Web Crawling
As we delve into the ethical considerations surrounding web crawling, it becomes essential to establish a framework that not only adheres to legal standards but also fosters respect for the resources and rights of website owners. Ethical web crawling is about striking a delicate balance between data collection and responsible behavior. Here are some best practices to guide developers in their crawling endeavors:
1. Respect Robots.txt Files:
Every web crawler should honor the directives outlined in a website’s robots.txt
file. This file informs crawlers of which parts of the site are off-limits. Ignoring these guidelines can lead to legal repercussions and server overloads. Before initiating a crawl, it is prudent to check this file.
fetch('http://example.com/robots.txt') .then(response => response.text()) .then(text => console.log(text));
2. Limit Request Rates:
To avoid overwhelming a server, implement throttling to limit the frequency of requests. This consideration not only protects the server but also prevents your crawler from being perceived as malicious. A common practice is to introduce a delay between requests.
function delay(ms) { return new Promise(resolve => setTimeout(resolve, ms)); } async crawlWithDelay(url) { await delay(1000); // wait 1 second between requests // perform the crawl logic here }
3. Identify Your Crawler:
Transparency is key in ethical web crawling. By setting a User-Agent string that accurately identifies your crawler, you foster trust with website administrators. This string should clearly state the purpose of your crawler and provide contact information.
const options = { method: 'GET', headers: { 'User-Agent': 'MyCrawler/1.0 (http://mycrawler.example.com/contact)' } }; fetch('http://example.com', options) .then(response => response.text()) .then(data => console.log(data));
4. Avoid Collecting Sensitive Information:
When crawling, it’s crucial to avoid accessing pages that contain sensitive data, such as login forms, personal user data, or any other information that could infringe on privacy. Crawlers should only target publicly available data.
5. Provide Value to the Community:
Whenever possible, aim to contribute positively to the web ecosystem. This can take the form of sharing insights from your crawls, providing data in an accessible manner, or even notifying website owners about broken links or issues found during your crawls.
6. Monitor for Changes:
Websites evolve, and so too should your crawling strategy. Regularly revisit the robots.txt
file and be mindful of any changes to the website’s structure or policies. This vigilance ensures that your crawling practices remain ethical over time.
By adhering to these best practices, developers can engage in web crawling in a manner that’s both effective and respectful, minimizing the risk of backlash while maximizing the potential for collaboration and insight generation across the web.
Tools and Libraries for JavaScript Web Crawling
In the context of web crawling using JavaScript, various tools and libraries can drastically simplify the process of creating efficient and effective crawlers. These tools not only provide robust functionalities but also help manage the complexities that come with navigating and extracting data from web pages. Below are some of the most prominent tools and libraries that developers can leverage for their web crawling endeavors.
Puppeteer is a powerful library developed by the Google Chrome team. It provides a high-level API to control headless Chrome or Chromium over the DevTools Protocol. Puppeteer is particularly useful for web scraping and crawling JavaScript-heavy websites, where traditional methods may fail. With Puppeteer, you can simulate user interactions, take screenshots, generate PDFs, and even execute JavaScript on the pages you crawl.
const puppeteer = require('puppeteer'); (async () => { const browser = await puppeteer.launch(); const page = await browser.newPage(); await page.goto('http://example.com'); const content = await page.content(); // Extract data const data = await page.evaluate(() => { return Array.from(document.querySelectorAll('h1')).map(element => element.textContent); }); console.log(data); await browser.close(); })();
Cheerio offers another avenue for web crawling, particularly when combined with Node.js. It’s a fast, flexible, and lean implementation of jQuery designed for the server. While Cheerio itself does not support JavaScript execution, it excels at parsing HTML and traversing the DOM. Thus, it’s best utilized for crawling static pages or when you have already fetched the HTML content using other methods like Axios or request.
const axios = require('axios'); const cheerio = require('cheerio'); (async () => { const { data } = await axios.get('http://example.com'); const $ = cheerio.load(data); const titles = []; $('h1').each((i, elem) => { titles.push($(elem).text()); }); console.log(titles); })();
Scrapy is a widely used Python framework for web scraping, but it can be effectively integrated with JavaScript for those who prefer the Node.js environment. By using it in conjunction with the ScrapyJS middleware, you can scrape JavaScript-rendered pages by managing a headless browser within the Scrapy architecture. This setup allows for sophisticated crawling strategies while still taking advantage of Python’s robust data-processing capabilities.
Axios, while primarily an HTTP client, is essential for any web crawler looking to make requests to endpoints and retrieve HTML content. Its promise-based architecture makes it relatively easy to work with asynchronous operations, ensuring that your crawler can fetch and process content without blocking the event loop.
const axios = require('axios'); async function fetchData(url) { try { const response = await axios.get(url); console.log(response.data); } catch (error) { console.error('Error fetching data:', error); } } fetchData('http://example.com');
Playwright is another modern library for browser automation that supports multiple browser engines, including Chromium, Firefox, and WebKit. It is particularly useful for crawling sites that require more advanced interactions, such as handling multiple tabs or capturing network requests. Playwright’s API is comparable to Puppeteer’s but offers additional features like auto-waiting for elements to be ready before performing actions.
const { chromium } = require('playwright'); (async () => { const browser = await chromium.launch(); const page = await browser.newPage(); await page.goto('http://example.com'); const content = await page.content(); console.log(content); await browser.close(); })();
With these tools and libraries at your disposal, the task of building a web crawler becomes significantly more approachable. Choosing the right tool largely depends on the specific requirements of your project, such as the type of content you need to scrape, the complexity of the webpages, and the need for rendering JavaScript. By using the strengths of these libraries, developers can tap into the vast resources of the web with grace and efficiency.