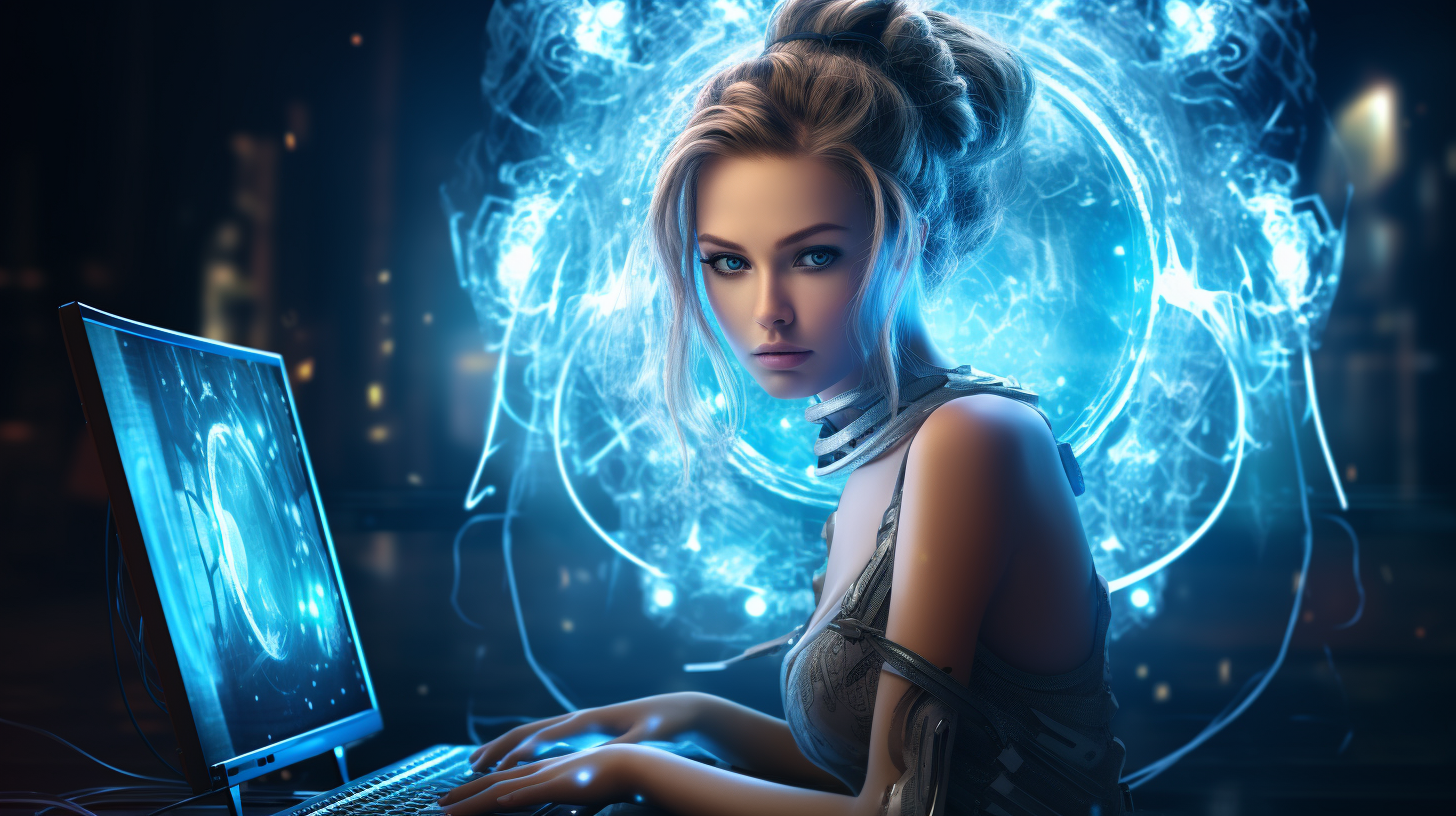
JavaScript and WebSockets for Real-Time Applications
WebSockets represent a revolutionary advancement in web communications, allowing for persistent, bidirectional communication channels between clients and servers. Unlike traditional HTTP requests, which are stateless and require a new connection for each interaction, WebSockets maintain a single open connection that can be used for multiple messages in both directions. This capability proves essential for real-time applications, such as online gaming, live chat, and collaborative tools.
One of the key advantages of WebSockets is the reduction in latency. Because the connection remains open, data can be sent and received almost instantaneously, which is a stark contrast to the delays introduced by repeated handshakes in HTTP requests. This feature makes WebSockets ideal for applications that demand quick and continuous updates, such as stock market tickers or real-time notifications.
Additionally, WebSockets minimize overhead. Each HTTP request carries headers that add to the payload size, while WebSocket frames are lightweight, resulting in less bandwidth consumption. For applications that need to convey a high volume of messages, such as gaming or live sports updates, this advantage translates into improved performance and responsiveness.
Another significant benefit lies in the ease of implementation. Most modern web browsers support WebSockets, and establishing a connection is simpler. The WebSocket API provides a simple interface for both creating connections and handling events, which allows developers to focus more on application logic than on the intricacies of network communication.
Here’s a basic example of how to create a WebSocket connection in JavaScript:
const socket = new WebSocket('ws://localhost:8080'); // Event listener for when the connection is open socket.addEventListener('open', function (event) { console.log('WebSocket is connected!'); }); // Event listener for receiving messages socket.addEventListener('message', function (event) { console.log('Message from server: ', event.data); }); // Sending a message to the server socket.send('Hello, Server!');
In this example, the WebSocket connection is established to a server running on localhost at port 8080. The connection triggers a message when opened, and it listens for incoming messages. This setup is the foundation for building more complex real-time interactions.
Furthermore, WebSockets play well with existing web technologies. Since they use the same underlying protocols as HTTP, developers can leverage their current knowledge while integrating real-time features into applications. This not only accelerates development time but also provides users with a seamless experience that complements the static content of traditional web pages.
WebSockets offer significant advantages over traditional web communication methods, enabling efficient, real-time data exchange with minimal overhead and latency. Their simplicity and compatibility with existing web technologies make them an excellent choice for developers aiming to enrich their applications with instantaneous interactivity.
Setting Up a WebSocket Server with JavaScript
Setting up a WebSocket server in JavaScript is a simpler process, thanks to the built-in capabilities provided by the Node.js runtime. To get started, ensure you have Node.js installed on your machine. If you haven’t done so, download and install Node.js from the official website.
Once you have Node.js ready, you can create a new project directory and initialize a new Node.js application using the following command:
mkdir websocket-server cd websocket-server npm init -y
Next, you’ll need the `ws` package, which is a simple and efficient WebSocket library for Node.js. Install it by running:
npm install ws
With the `ws` library installed, you can create a basic WebSocket server. Below is a simple implementation that listens for connections and handles incoming messages:
const WebSocket = require('ws'); // Create a new WebSocket server const wss = new WebSocket.Server({ port: 8080 }); // Listen for connection events wss.on('connection', (ws) => { console.log('New client connected'); // Listen for messages from clients ws.on('message', (message) => { console.log(`Received: ${message}`); // Echo the message back to the client ws.send(`Server received: ${message}`); }); // Send a welcome message to the newly connected client ws.send('Welcome to the WebSocket server!'); }); // Log when the server is up and running console.log('WebSocket server is running on ws://localhost:8080');
In this example, the WebSocket server listens on port 8080. When a client connects, it logs a message to the console. The server is also set to listen for incoming messages from the client, which it echoes back after logging the received message. Additionally, a welcome message is sent to the new client upon connection.
To run the WebSocket server, use the following command in your terminal:
node server.js
Make sure to replace `server.js` with the name of your JavaScript file, if you choose a different one. After starting your server, you can test the connection using a simple WebSocket client, such as the browser’s JavaScript console or a dedicated tool.
Using this basic setup, you can build upon the server to include more complex features, such as broadcasting messages to all connected clients, handling user authentication, or integrating with other APIs. The WebSocket server acts as the backbone of your real-time application, opening up a world of possibilities for interactive and engaging user experiences.
Implementing Real-Time Communication in Web Applications
Implementing real-time communication in web applications using WebSockets requires a clear understanding of how to effectively utilize the connection established between the client and server. By using the persistent connection that WebSockets provide, developers can create applications that respond to user actions and events in near real-time.
Once you’ve set up your WebSocket server, the next step is to enable communication between the server and multiple clients. This can involve broadcasting messages to all connected clients, handling private messages, or managing user sessions. Below is an example that showcases how to implement basic broadcasting functionality.
const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 8080 }); wss.on('connection', (ws) => { console.log('New client connected'); // Listen for messages from the client ws.on('message', (message) => { console.log(`Received: ${message}`); // Broadcast the message to all clients wss.clients.forEach(client => { if (client.readyState === WebSocket.OPEN) { client.send(message); } }); }); // Send a welcome message to the newly connected client ws.send('Welcome to the WebSocket server!'); }); // Log when the server is up and running console.log('WebSocket server is running on ws://localhost:8080');
In this implementation, whenever a message is received from one client, the server echoes that message to all connected clients. This is accomplished by iterating over `wss.clients`, which holds all active connections. Each client’s `readyState` is checked to ensure it’s open before sending the message, preventing errors when trying to communicate with disconnected clients.
Furthermore, maintaining a state for each connected user can enhance your application’s interactivity. You may want to implement user identification, enabling personalized messaging or notifications. Storing user information, such as usernames, can be done using a simple object to map socket connections to user data. Here’s an example:
let users = {}; wss.on('connection', (ws) => { ws.on('message', (message) => { const data = JSON.parse(message); if (data.type === 'register') { users[ws] = data.username; ws.send('User registered: ' + data.username); } else if (data.type === 'message') { // Broadcast message to all users const username = users[ws]; const msg = `${username}: ${data.content}`; wss.clients.forEach(client => { if (client.readyState === WebSocket.OPEN) { client.send(msg); } }); } }); });
In this snippet, a `users` object is created to map each WebSocket connection to a username. When a client sends a message of type `register`, their username is stored. For messages of type `message`, the server retrieves the associated username and broadcasts the formatted message to all clients. This allows for a more personalized communication experience.
Another common requirement in real-time applications is handling disconnections gracefully. You can achieve this by adding an event listener for the `close` event on the WebSocket connection:
ws.on('close', () => { console.log('Client disconnected: ', users[ws]); delete users[ws]; // Remove user from the users map });
By listening to the `close` event, you can clean up resources or notify other users when a client disconnects. The `delete` operation ensures that the disconnected user is removed from the user mapping.
To improve the user experience further, ponder implementing error handling. Using the `error` event on the WebSocket can help manage unexpected issues:
ws.on('error', (error) => { console.error('WebSocket error observed:', error); });
By following these principles, you can implement robust real-time communication in your web applications using WebSockets. The capability to broadcast messages, manage user sessions, and handle connections dynamically opens up a realm of possibilities for interactive applications, leading to a more engaging user experience.
Best Practices for WebSocket Performance and Security
When working with WebSockets, ensuring optimal performance and security is critical to creating a reliable and robust real-time application. The persistent nature of WebSocket connections introduces unique challenges that require careful consideration, particularly when it comes to scalability, data integrity, and user safety. Adopting best practices in these areas can significantly enhance your application’s reliability and user experience.
Performance Optimization
One of the primary concerns when implementing WebSockets is managing performance, especially when your application scales to handle many concurrent connections. Here are some key strategies to achieve optimal performance:
1. Connection Management: Reuse WebSocket connections whenever possible. Frequent opening and closing of connections can lead to unnecessary overhead and latency. Instead, establish a connection once and maintain it for the application’s duration.
2. Load Balancing: For applications expecting high traffic, think using load balancers to distribute WebSocket connections across multiple servers. This approach not only improves performance but also provides redundancy in case one of the servers fails.
3. Message Compression: Reducing the payload size can enhance performance, especially when sending large amounts of data. Implementing message compression over WebSocket connections can help decrease bandwidth usage. The permessage-deflate
extension can be used for this purpose.
const WebSocket = require('ws'); const server = new WebSocket.Server({ port: 8080, perMessageDeflate: true }); server.on('connection', (socket) => { console.log('Client connected'); // Other connection logic... });
4. Batching Messages: If your application frequently sends updates, ponder batching multiple messages into a single WebSocket frame. This reduces the overhead associated with processing each frame separately and can lead to improved efficiency.
5. Monitor Connection State: Create mechanisms to monitor WebSocket connection states. Implementing heartbeat messages or ping-pong frames can help detect unresponsive clients and clean up connections that are no longer active.
Security Considerations
Security is paramount when dealing with real-time applications that involve user data. Below are several best practices to enhance the security of your WebSocket implementation:
1. Use Secure WebSockets (WSS): Always use the WSS protocol to encrypt the data transmitted between the client and server. This protects against eavesdropping and man-in-the-middle attacks.
const wss = new WebSocket.Server({ port: 8080, perMessageDeflate: true }); // Always prefer WSS over WS const socket = new WebSocket('wss://yourdomain.com/path');
2. Authentication and Authorization: Implement robust authentication mechanisms to ensure that only authorized users can establish a WebSocket connection. Using tokens or session IDs can help verify users and maintain their privileges throughout the session.
3. Input Validation: Always validate and sanitize incoming messages from clients. This is critical to prevent injection attacks or malicious data from corrupting your application state.
ws.on('message', (message) => { const sanitizedMessage = sanitizeInput(message); // Process the sanitized message... });
4. Rate Limiting: Implement rate limiting on WebSocket connections to prevent abuse, such as denial-of-service attacks. This can be achieved by limiting the number of messages a client can send in a given timeframe.
5. Origin Checking: Validate the origin of incoming WebSocket connections to ensure they are coming from trusted sources. This helps mitigate cross-origin attacks.
const allowedOrigins = ['https://trusteddomain.com']; wss.on('connection', (ws, req) => { const origin = req.headers.origin; if (!allowedOrigins.includes(origin)) { ws.close(); // Reject the connection } });
By adhering to these best practices, you can create a WebSocket-based application this is both efficient and secure. The combination of performance optimization and security measures ensures that your application is not only responsive but also resilient against potential threats, thereby providing a safe environment for your users to engage in real-time interactions.