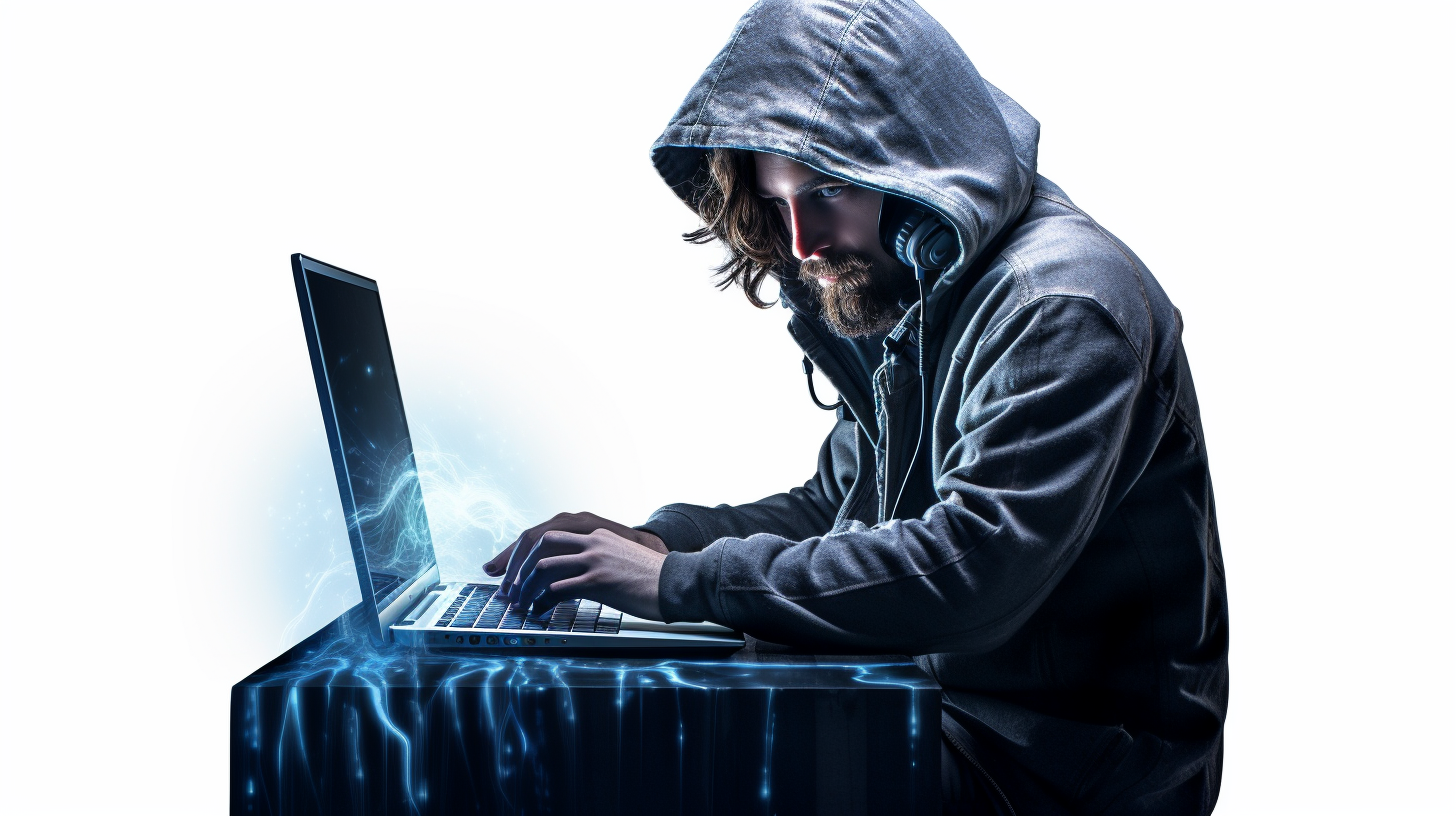
JavaScript Animation Basics
Animation in JavaScript is more than just moving elements around; it’s a carefully orchestrated pipeline that transforms your visual concepts into a reality on the screen. Understanding this pipeline is important for creating fluid, engaging animations that users will find captivating.
At its core, the animation pipeline consists of several stages: the setup, the execution, and the rendering. Each stage plays a vital role in delivering a seamless animation experience.
Setup: This is where you define the parameters of your animation. You decide what elements will be animated, their starting and ending states, and any intermediary steps. This setup is akin to laying the groundwork for a performance before the curtain rises.
const element = document.getElementById('animateMe'); const startPosition = 0; const endPosition = 300; const duration = 1000; // in milliseconds
Execution: Once the setup is complete, the execution phase begins. This phase involves calculating the changes in position and state over time, which especially important for ensuring that the animation flows smoothly. Using a timer or the browser’s animation frame is essential here.
let startTime = null; function animate(timestamp) { if (!startTime) startTime = timestamp; const elapsed = timestamp - startTime; // Calculate the current state of the animation const progress = Math.min(elapsed / duration, 1); const currentPosition = startPosition + (endPosition - startPosition) * progress; // Apply the calculated position element.style.transform = `translateX(${currentPosition}px)`; if (progress < 1) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
Rendering: Finally, we have the rendering stage where the browser takes the calculated values and draws them on the screen. That’s where the magic happens—the visual representation of your animation comes to life. To achieve optimal rendering, it is essential to use methods like requestAnimationFrame
, which helps in synchronizing your animation with the display refresh rate of the browser.
Each of these stages is interconnected, and understanding how they work together allows you to create animations that not only look good but also perform well. Mastery of this pipeline sets the foundation for more complex animation techniques that can elevate your web applications to new heights.
The Role of CSS in JavaScript Animations
The integration of CSS into JavaScript animations is a game-changer, offering a powerful way to enhance the visual allure of your web applications. While JavaScript provides the logic and control to create animations, CSS complements this by allowing for smoother transitions, better performance, and a more simpler application of styles. The interplay between CSS and JavaScript can elevate your animations from simple movements to complex, visually striking experiences.
One of the critical roles of CSS in animations is its ability to handle transitions and transformations efficiently. CSS transitions allow you to define how property changes should occur over time without the need for scripting every single frame. By using CSS for certain aspects of your animations, you can reduce the work JavaScript has to do, resulting in better performance and smoother animations.
For instance, consider a scenario where you want an element to fade in and out. Instead of manually computing the opacity in JavaScript, you can take advantage of CSS transitions:
const fadeElement = document.getElementById('fadeMe'); fadeElement.style.opacity = 0; // Start hidden // Trigger the CSS transition setTimeout(() => { fadeElement.style.transition = 'opacity 1s ease-in-out'; fadeElement.style.opacity = 1; // Fade in }, 1000);
This approach allows the browser to optimize the rendering of the transition, as it can handle the changes internally. Furthermore, CSS animations can be hardware-accelerated, resulting in better performance on various devices, particularly mobile.
Additionally, CSS can facilitate complex animations using keyframes. With CSS animations, you can define multiple steps in your animation sequence, allowing for intricate movements without extensive JavaScript coding. For example:
@keyframes slideIn { from { transform: translateX(-100%); opacity: 0; } to { transform: translateX(0); opacity: 1; } } #slideMe { animation: slideIn 1s forwards; }
In the example above, the `@keyframes` rule creates a sliding effect that can be easily reused and modified. When combined with JavaScript, this enables dynamic control over when an animation starts or its specific parameters, allowing for a responsive user interface.
Another benefit of using CSS alongside JavaScript is the ability to separate concerns. By keeping your styling in CSS, you can maintain cleaner JavaScript code focused purely on logic and functionality. This separation enhances maintainability and scalability, particularly in larger projects where many developers may be involved.
However, it’s important to know when to use CSS versus JavaScript animations. For simple transitions and effects, CSS is often the best choice. When extensive control over the animation sequence is required or when you need to synchronize animations based on user input or other events, JavaScript is more appropriate.
Ultimately, mastering the roles of CSS and JavaScript in animations allows you to take full advantage of both technologies, leading to visually stunning and highly performant web applications. By carefully considering how to leverage CSS animations with JavaScript control, you can elevate your work to a level that captivates users and enhances their experience.
Using requestAnimationFrame for Smooth Animations
When it comes to crafting smooth animations in JavaScript, the requestAnimationFrame method stands as an essential tool in your arsenal. This method provides a way to create animations that are not only visually appealing but also optimized for performance, taking full advantage of the browser’s refresh rate.
The primary advantage of using requestAnimationFrame over traditional methods like setTimeout or setInterval lies in its ability to synchronize your animation with the browser’s paint cycle. By doing so, you reduce the chance of flickering and stuttering during animations, leading to a more fluid user experience.
To utilize requestAnimationFrame, you define an animation loop function that updates the properties you wish to animate. This function is called before the browser repaints, ensuring that your updates are smooth and in sync with the display. Here’s a simple example to illustrate this:
const box = document.getElementById('animatedBox'); let position = 0; // Start position const targetPosition = 500; // Target position const speed = 2; // Speed of the animation function animate() { // Update position if (position < targetPosition) { position += speed; box.style.transform = `translateX(${position}px)`; // Request the next frame requestAnimationFrame(animate); } } // Start the animation requestAnimationFrame(animate);
In this example, we have a box that moves horizontally across the screen. The animate function updates the position of the box and requests the next animation frame using requestAnimationFrame. As long as the current position is less than the target position, the animation will continue running.
One crucial aspect of using requestAnimationFrame is its ability to pause and resume animations efficiently. If the user navigates away from the page, the browser can automatically suspend the animations, saving processing power and improving overall performance. This means that animations can resume without any jarring jumps once the user returns, as the timing will remain consistent with the frame rate.
Another thing to ponder is that requestAnimationFrame provides a timestamp as an argument to the callback function, which can be leveraged for more complex animations that require timing calculations. For instance, if you want to create a more nuanced animation where the speed varies or you need to calculate easing effects, this timestamp can be invaluable:
let lastTimestamp = null; const duration = 2000; // Duration in milliseconds function animateWithEasing(timestamp) { if (!lastTimestamp) lastTimestamp = timestamp; const elapsed = timestamp - lastTimestamp; // Calculate the progress in terms of the easing function const progress = Math.min(elapsed / duration, 1); const easeInOutQuad = progress < 0.5 ? 2 * progress * progress : -1 + (4 - 2 * progress) * progress; // Update the position based on the eased progress position = easeInOutQuad * targetPosition; box.style.transform = `translateX(${position}px)`; if (progress < 1) { lastTimestamp = timestamp; // Update last timestamp requestAnimationFrame(animateWithEasing); } } // Start the animation requestAnimationFrame(animateWithEasing);
In this code, we utilize an easing function to create a more fluid and natural movement, which enhances the visual feedback for users. By adjusting the way the progress is calculated, animations can feel more organic and engaging.
In summary, mastering requestAnimationFrame is essential for any JavaScript developer looking to create smooth animations. Its efficiency and synchronization capabilities provide a robust foundation for building visually captivating experiences that respond dynamically to user interactions.
Creating Simple Animations with JavaScript
Creating simple animations with JavaScript involves manipulating the properties of DOM elements over time to produce visual effects that can enhance user experience. At its core, the process boils down to defining a target state for your elements and calculating the intermediate frames that lead to that state. Let’s delve into the mechanics of building a simpler animation using vanilla JavaScript.
To illustrate, think an example where we want to animate a box moving across the screen. The first step is to set up our HTML and CSS for the animated element:
With the box in place, we can now write the JavaScript that will drive the animation. We’ll define the starting position, the target position, the duration of the animation, and an update function that adjusts the box’s position over time.
const box = document.getElementById('box'); let startPosition = 0; const endPosition = 500; const duration = 2000; // in milliseconds const startTime = performance.now(); function animate(currentTime) { const elapsed = currentTime - startTime; const progress = Math.min(elapsed / duration, 1); const currentPosition = startPosition + (endPosition - startPosition) * progress; box.style.transform = `translateX(${currentPosition}px)`; if (progress < 1) { requestAnimationFrame(animate); } } requestAnimationFrame(animate);
In this script, we define a function named animate that’s called repeatedly by requestAnimationFrame. We calculate the elapsed time since the animation started and determine how far along we are in the animation based on the specified duration. The current position of the box is updated using the progress value, which dictates how much of the distance to the target position has been covered.
One key point to note is the use of requestAnimationFrame. This method ensures that our animation runs smoothly by aligning it with the browser’s refresh rate. This compatibility not only enhances visual performance but also minimizes the load on the CPU, leading to a more responsive experience.
To make this animation more engaging, we could add some easing effects. Instead of a linear transition, we can modify the progress calculation to include a simple easing function. Here’s how you could implement an ease-in-out effect:
function easeInOutQuad(t) { return t < 0.5 ? 2 * t * t : -1 + (4 - 2 * t) * t; } function animateWithEasing(currentTime) { const elapsed = currentTime - startTime; const progress = Math.min(elapsed / duration, 1); const easedProgress = easeInOutQuad(progress); const currentPosition = startPosition + (endPosition - startPosition) * easedProgress; box.style.transform = `translateX(${currentPosition}px)`; if (progress < 1) { requestAnimationFrame(animateWithEasing); } } requestAnimationFrame(animateWithEasing);
Here, we’ve introduced the easeInOutQuad function, which modifies the way we perceive the speed of the animation. With easing, the box will start slowly, gain speed in the middle, and then slow down as it approaches the end position, creating a more natural look.
Creating simple animations in JavaScript involves a combination of DOM manipulation, time-based calculations, and using the browser’s rendering capabilities. By mastering these techniques, you can elevate the interactivity of your web applications, providing users with compelling visual experiences that draw them in and keep them engaged.
Using Libraries for Advanced Animations
Using libraries for advanced animations can significantly enhance your JavaScript projects, providing a wealth of pre-built functionality that can simplify complex animation tasks and improve performance. While writing animations from scratch gives you fine-grained control, libraries can accelerate development time and help you achieve sophisticated effects with less effort. Let’s explore some popular animation libraries and their benefits.
One of the most widely used libraries is GSAP (GreenSock Animation Platform). GSAP is known for its power and flexibility, allowing for high-performance animations that work consistently across browsers. Its syntax is intuitive, making it accessible for beginners while still offering advanced features for seasoned developers. Here’s a basic example of animating a box using GSAP:
gsap.to("#box", { duration: 2, x: 500, opacity: 0.5 });
In this example, we use GSAP’s `to` method to animate the `#box` element, moving it 500 pixels to the right while gradually changing its opacity to 0.5 over 2 seconds. The beauty of GSAP lies in its ability to chain multiple animations and manage complex sequences efficiently.
Another notable library is Anime.js. It’s lightweight and offers a simple interface for creating animations with a variety of effects including transforms, CSS properties, SVG, and more. Its capabilities for handling animations in a timeline and providing easing functions make it an appealing choice. Below is an example demonstrating how to animate an element’s scale and rotation using Anime.js:
anime({ targets: '#box', scale: [0, 1], rotate: '1turn', duration: 2000, easing: 'easeInOutExpo' });
This code animates the `#box` from a scale of 0 to 1 while performing a full rotation. The `easing` function adds a smooth transition effect, further enhancing the user experience.
For developers looking to work with SVG animations, Snap.svg is an excellent choice. It provides a powerful API for manipulating SVG graphics and can be combined with other libraries for more comprehensive animations. Here’s how you could use Snap.svg to animate a circle:
var s = Snap("#svg"); var circle = s.circle(50, 50, 40); circle.attr({ fill: "#bada55" }); circle.animate({ r: 70, fill: "#ff0000" }, 1000, mina.bounce);
In this snippet, we create a circle and animate its radius and color over one second using a bounce easing effect. The simplicity of Snap.svg makes it easy to create intricate SVG animations without delving deeply into the SVG markup.
Using libraries for animation not only saves time but also brings a collection of best practices and optimizations that may not be immediately apparent when building from scratch. These libraries often handle browser inconsistencies, ensuring that your animations look the same across different environments, which can be a daunting task if you’re doing everything manually.
Moreover, many animation libraries come with extensive documentation and community support, making it easier to troubleshoot issues and learn new techniques. They often include features such as timelines, sequencing, and callbacks, which can help you manage complex animations effectively.
As you explore the world of advanced animations, consider integrating these libraries into your projects. They not only enhance the visual allure and interactivity of your web applications but also allow you to focus on creating compelling user experiences without getting bogged down in the intricacies of animation calculations. Mastering these tools can transform your animations from simple effects into engaging narratives that captivate your audience.
Optimizing Performance in JavaScript Animations
When it comes to optimizing performance in JavaScript animations, a disciplined approach can yield significant improvements in both visual fluidity and resource management. Animation can be resource-intensive, and if not handled correctly, it can lead to janky frames, increased CPU usage, and a generally poor user experience. Therefore, understanding and applying performance optimization techniques is critical for any developer aiming to create smooth animations.
One fundamental principle of performance optimization is to minimize the number of DOM manipulations. Each interaction with the DOM can be expensive, so batching changes wherever possible is advisable. Instead of manipulating styles directly within the animation loop, consider calculating the needed changes and applying them in a single operation. For example:
const box = document.getElementById('box'); let position = 0; const endPosition = 500; const duration = 2000; function animate() { const startTime = performance.now(); function step(timestamp) { const elapsed = timestamp - startTime; const progress = Math.min(elapsed / duration, 1); position = progress * endPosition; // Apply all style changes at once box.style.transform = `translateX(${position}px)`; if (progress < 1) { requestAnimationFrame(step); } } requestAnimationFrame(step); } animate();
In the code above, the `box.style.transform` property is updated only once per frame, which reduces the overhead of multiple style recalculations. Additionally, using the `requestAnimationFrame` method not only allows for smoother updates but also lets the browser optimize the rendering effectively.
Another significant consideration is the use of CSS for animations where possible. CSS animations and transitions are often hardware-accelerated, meaning they can leverage the GPU for rendering, which is generally faster than CPU-based animations done with JavaScript. For instance, animating properties like `transform` and `opacity` in CSS can lead to better performance compared to animating properties like `width`, `height`, or `left`, which may cause layout recalculations.
.box { transition: transform 0.5s ease-in-out; } .box.animate { transform: translateX(500px); }
In this case, applying a simple CSS class to trigger the animation can yield a much smoother effect. This delegation of work to the browser’s rendering engine allows for more efficient use of resources, enabling animations to run at the same time without blocking the main thread.
Moreover, it is essential to be mindful of the frequency of your animations. Excessively high frame rates can lead to unnecessary CPU usage and battery drain, particularly on mobile devices. A general rule of thumb is to maintain a frame rate around 60 frames per second (FPS), which is the typical refresh rate of most displays. Using the `requestAnimationFrame` method helps keep your animations aligned with this standard, as it automatically optimizes the timing of animations to match the refresh rate.
In addition, consider using throttling or debouncing techniques for animations triggered by user input. For instance, if you have animations that respond to scrolling or window resizing, wrapping your animation calls in a debounce function can prevent excessive triggering, which can help maintain a fluid experience.
function debounce(func, timeout = 300) { let timer; return (...args) => { clearTimeout(timer); timer = setTimeout(() => { func.apply(this, args); }, timeout); }; } window.addEventListener('resize', debounce(() => { // Trigger animation animate(); }));
Lastly, performance profiling is invaluable when optimizing animations. Tools like Chrome DevTools allow you to analyze frame rates, memory usage, and CPU load. By examining the Timeline panel, you can identify bottlenecks in your animations and make data-driven decisions to enhance performance.
Optimizing performance in JavaScript animations requires a strategic approach that balances visual fidelity with resource management. By using techniques such as minimizing DOM manipulation, using CSS animations, ensuring optimal frame rates, and employing profiling tools, you can create animations that are not only visually stunning but also performant and responsive. Mastering these techniques will empower you to craft engaging user experiences that captivate and retain your audience.