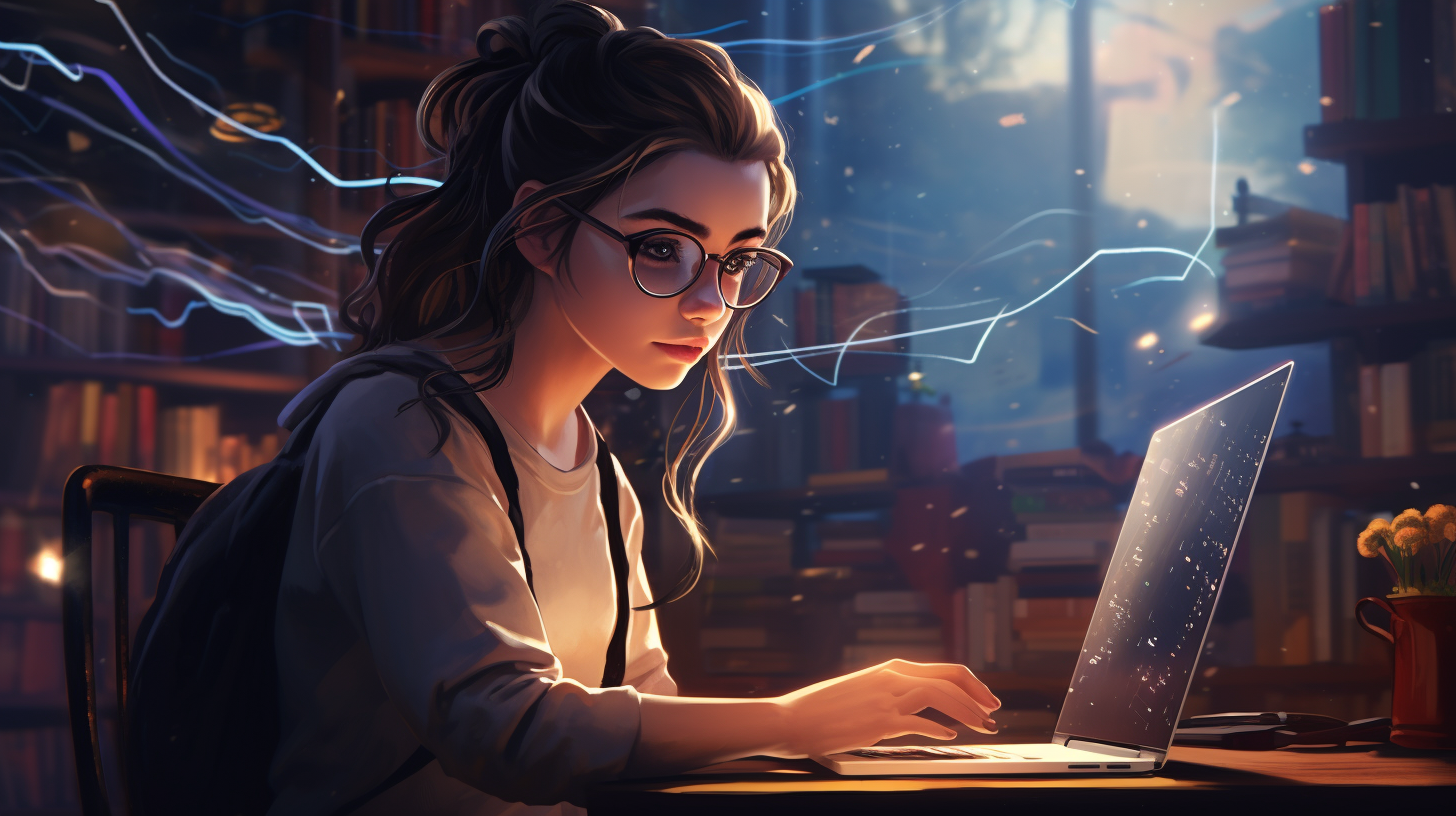
JavaScript Arrays and Their Methods
JavaScript arrays are a fundamental aspect of the language, efficiently storing multiple values in a single variable. They’re dynamic, meaning they can grow and shrink as needed, providing flexibility this is highly valued in modern development. At their core, arrays are a special type of object that allow for the storage of ordered collections of data.
Creating an array in JavaScript can be done using the array literal syntax or the Array constructor. The array literal syntax involves enclosing a comma-separated list of values in square brackets, while the Array constructor allows for more dynamic array creation.
// Array literal syntax const fruits = ['apple', 'banana', 'cherry']; // Array constructor const numbers = new Array(1, 2, 3, 4, 5);
Arrays in JavaScript are zero-indexed, meaning the first element is accessed using the index 0. This characteristic very important when traversing or manipulating arrays. Accessing an element is straightforward:
console.log(fruits[0]); // Outputs: apple console.log(numbers[2]); // Outputs: 3
JavaScript arrays can hold any type of data, including numbers, strings, objects, and even other arrays, allowing for the creation of complex data structures. This flexibility is a double-edged sword; while it enables a wide variety of use cases, it can also lead to unexpected behavior if not managed carefully.
To check the length of an array, which indicates how many elements it contains, you can simply access the length property:
console.log(fruits.length); // Outputs: 3
One of the unique features of JavaScript arrays is that they can also be sparse. This means that you can have “gaps” in the indices. For example, you can define an array with a specific index without filling in the earlier indices:
const sparseArray = []; sparseArray[5] = 'hello'; console.log(sparseArray.length); // Outputs: 6 console.log(sparseArray[0]); // Outputs: undefined
This dynamic nature of arrays makes them incredibly useful for a variety of programming tasks such as data management and manipulation. Understanding the basics of JavaScript arrays is essential for using their full potential in your JavaScript applications.
Common Array Methods
JavaScript arrays come equipped with a rich set of built-in methods that simplify the manipulation and interaction with array data. These methods enhance functionality, allowing developers to perform operations efficiently without needing to reinvent the wheel. Here’s a deeper dive into some of the most common array methods that every JavaScript programmer should be familiar with.
One of the most frequently used methods is push(), which adds one or more elements to the end of an array and returns the new length of the array. This is particularly useful when you want to dynamically expand your array by adding new items:
const colors = ['red', 'green']; const newLength = colors.push('blue'); console.log(colors); // Outputs: ['red', 'green', 'blue'] console.log(newLength); // Outputs: 3
Conversely, the pop() method removes the last element of an array and returns that element. If the array is already empty, it returns undefined
. This pair of methods allows for easy management of an array as a stack:
const fruits = ['apple', 'banana', 'cherry']; const lastFruit = fruits.pop(); console.log(fruits); // Outputs: ['apple', 'banana'] console.log(lastFruit); // Outputs: cherry
In addition to adding and removing elements from the end of an array, you can also manipulate the beginning of an array using unshift() and shift(). The unshift() method adds one or more elements to the start of an array, while shift() removes the first element:
const numbers = [2, 3, 4]; numbers.unshift(1); console.log(numbers); // Outputs: [1, 2, 3, 4] const firstNumber = numbers.shift(); console.log(numbers); // Outputs: [2, 3, 4] console.log(firstNumber); // Outputs: 1
For those times when you need to know whether an array contains a particular element, the includes() method is invaluable. It checks if a specified value exists within the array and returns a boolean:
const pets = ['cat', 'dog', 'parrot']; console.log(pets.includes('dog')); // Outputs: true console.log(pets.includes('fish')); // Outputs: false
Another useful method is slice(), which returns a shallow copy of a portion of an array into a new array object. It takes two parameters: the start index (inclusive) and the end index (exclusive). This method is particularly useful for extracting portions of data without modifying the original array:
const animals = ['cat', 'dog', 'parrot', 'frog']; const someAnimals = animals.slice(1, 3); console.log(someAnimals); // Outputs: ['dog', 'parrot'] console.log(animals); // Outputs: ['cat', 'dog', 'parrot', 'frog']
On the other end of the spectrum, if you need to modify an array in place, splice() is your go-to method. It can add or remove items from any position in the array. The first argument specifies the index at which to start changing the array, the second argument specifies how many elements to remove, and any subsequent arguments specify elements to add:
const snacks = ['chips', 'cookies', 'candy']; snacks.splice(1, 1, 'cake', 'fruit'); console.log(snacks); // Outputs: ['chips', 'cake', 'fruit', 'candy']
These common array methods form the backbone of many operations you will perform with arrays in JavaScript. By mastering these functions, you will find it much easier to manipulate data and build more complex structures in your applications.
Manipulating Arrays: Adding and Removing Elements
Manipulating arrays in JavaScript is a critical skill for any developer, as it allows for flexible handling of data. Adding and removing elements from arrays can be done in various ways, each suited for different scenarios. Understanding these methods empowers you to manage your data effectively.
First, let’s delve into adding elements to an array. The push() method is commonly used to append one or more elements to the end of an array. This method modifies the original array and returns the new length of the array, which can be helpful if you need to know how many elements are currently in the array after the operation:
const fruits = ['apple', 'banana']; const newLength = fruits.push('cherry'); console.log(fruits); // Outputs: ['apple', 'banana', 'cherry'] console.log(newLength); // Outputs: 3
If you need to add elements to the start of an array, the unshift() method comes into play. Similar to push(), this method modifies the original array and returns the new length:
const vegetables = ['carrot', 'lettuce']; const updatedLength = vegetables.unshift('spinach'); console.log(vegetables); // Outputs: ['spinach', 'carrot', 'lettuce'] console.log(updatedLength); // Outputs: 3
On the flip side, when it comes to removing elements, pop() is your go-to method for removing the last element of an array. This method returns the removed element, so that you can work with it if necessary:
const snacks = ['chips', 'cookies', 'soda']; const lastSnack = snacks.pop(); console.log(snacks); // Outputs: ['chips', 'cookies'] console.log(lastSnack); // Outputs: 'soda'
To remove the first element, shift() is the equivalent of pop(). It removes the first element of the array and returns it:
const numbers = [1, 2, 3]; const firstNumber = numbers.shift(); console.log(numbers); // Outputs: [2, 3] console.log(firstNumber); // Outputs: 1
For more controlled manipulation of an array, the splice() method is invaluable. It can be used to add or remove elements from any position in the array. You specify the index to start from, the number of elements to remove, and any new elements to add:
const fruits = ['apple', 'banana', 'cherry']; fruits.splice(1, 1, 'orange', 'grape'); console.log(fruits); // Outputs: ['apple', 'orange', 'grape', 'cherry']
In this example, we started at index 1, removed one element (banana), and added two new elements (orange and grape) in its place. The splice() method modifies the original array, making it a powerful tool for real-time data manipulation.
For cases where you want to extract elements without modifying the original array, the slice() method is ideal. It creates a shallow copy of a portion of the array based on the specified start and end indices:
const colors = ['red', 'green', 'blue', 'yellow']; const primaryColors = colors.slice(0, 3); console.log(primaryColors); // Outputs: ['red', 'green', 'blue'] console.log(colors); // Outputs: ['red', 'green', 'blue', 'yellow']
By mastering these methods for adding and removing elements, you can manipulate arrays dynamically and efficiently, making it easier to handle complex data structures in your JavaScript applications.
Iterating Through Arrays
Iterating through arrays is an essential skill in JavaScript, allowing developers to access and manipulate each element in an array seamlessly. Given the dynamic nature of JavaScript arrays, there are a high number of techniques available to traverse them, from traditional loops to modern higher-order functions.
One of the most simpler methods for iterating over an array is using a simple for
loop. This gives you complete control over the iteration process, so that you can specify the starting index, the end condition, and the increment step:
const numbers = [1, 2, 3, 4, 5]; for (let i = 0; i < numbers.length; i++) { console.log(numbers[i]); // Outputs each number }
In this example, we loop through the numbers
array, accessing each element by its index. While this approach is effective, it can become cumbersome for larger arrays or more complex operations.
Another common way to iterate through arrays is by using the forEach
method. This higher-order function provides a more elegant syntax and abstracts away the manual index management:
const fruits = ['apple', 'banana', 'cherry']; fruits.forEach((fruit) => { console.log(fruit); // Outputs each fruit });
The forEach
method takes a callback function that’s executed for each element in the array, passing the current element as an argument. This results in cleaner and more readable code, especially when performing actions on each item.
For situations where you need to create a new array based on the original, the map
method is a powerful tool. It allows you to apply a function to each element and return an array of the results:
const numbers = [1, 2, 3, 4, 5]; const doubled = numbers.map((num) => num * 2); console.log(doubled); // Outputs: [2, 4, 6, 8, 10]
The map
method is particularly useful for transforming data, whether you’re performing calculations, formatting strings, or extracting properties from objects.
When you need to filter out elements based on a condition, the filter
method is your best friend. It creates a new array containing only elements that satisfy the provided condition:
const ages = [12, 18, 21, 16, 25]; const adults = ages.filter((age) => age >= 18); console.log(adults); // Outputs: [18, 21, 25]
By providing a function that returns true or false, you can easily select which items to keep, enhancing your ability to manage data collections.
For cases where you need to find a specific element in an array, the find
method performs a similar function but returns the first element that meets the criteria:
const products = [ { name: 'apple', price: 1 }, { name: 'banana', price: 0.5 }, { name: 'cherry', price: 2 } ]; const expensiveFruit = products.find((product) => product.price > 1); console.log(expensiveFruit); // Outputs: { name: 'cherry', price: 2 }
Finally, if you are looking to reduce an array to a single value, the reduce
method is invaluable. This method applies a function against an accumulator and each element in the array (from left to right) to reduce it to a single value:
const numbers = [1, 2, 3, 4]; const sum = numbers.reduce((accumulator, currentValue) => accumulator + currentValue, 0); console.log(sum); // Outputs: 10
In this example, we accumulate the total sum of the elements in the numbers
array, showcasing how reduce
can be leveraged for various operations, from summing values to flattening arrays.
Each of these iteration techniques offers unique advantages, enabling developers to process and manipulate JavaScript arrays effectively. By mastering these methods, you can unlock the full potential of arrays in your applications, making your code more efficient and expressive.
Advanced Array Methods and Techniques
// Advanced array methods offer a plethora of functionalities for developers looking to harness the full power of JavaScript arrays. These methods provide ways to perform complex operations with ease, allowing for more efficient and expressive code. Let's explore some of these advanced techniques that can elevate your JavaScript programming skills. One such powerful method is reduceRight(). This method works similarly to reduce(), but it processes the array from right to left. This can be particularly useful in cases where the order of operations matters. const numbers = [1, 2, 3, 4]; const reversedSum = numbers.reduceRight((accumulator, currentValue) => accumulator + currentValue, 0); console.log(reversedSum); // Outputs: 10 Another advanced method is the flat() method, which is handy when dealing with multi-dimensional arrays. It creates a new array with all sub-array elements concatenated into it recursively up to the specified depth. const nestedArray = [1, 2, [3, 4, [5, 6]]]; const flatArray = nestedArray.flat(2); console.log(flatArray); // Outputs: [1, 2, 3, 4, 5, 6] If you need to execute a function on each element and return a promise for each, Promise.all() in combination with map() can be a game changer. This allows you to handle asynchronous operations on arrays efficiently. const urls = ['url1', 'url2', 'url3']; const fetchData = async (url) => { const response = await fetch(url); return response.json(); }; Promise.all(urls.map(fetchData)).then((results) => { console.log(results); // Outputs an array of JSON data from all the URLs }); For scenarios requiring sorting, the sort() method provides a way to sort the elements of an array in place and return the sorted array. Custom sorting logic can be implemented by providing a comparison function. const fruits = ['banana', 'apple', 'cherry']; fruits.sort((a, b) => a.localeCompare(b)); console.log(fruits); // Outputs: ['apple', 'banana', 'cherry'] The some() and every() methods allow you to test whether at least one or all elements in an array pass a specific test, respectively. This can streamline validation processes in your code. const ages = [15, 20, 17, 22]; const hasAdult = ages.some((age) => age >= 18); console.log(hasAdult); // Outputs: true const allAdults = ages.every((age) => age >= 18); console.log(allAdults); // Outputs: false Lastly, the fill() method can be utilized to change all elements in a static array to a static value, which is particularly useful for initializing arrays. const emptyArray = new Array(5).fill(0); console.log(emptyArray); // Outputs: [0, 0, 0, 0, 0] By incorporating these advanced array methods into your JavaScript toolkit, you can achieve more complex and efficient data manipulation, leading to cleaner and more maintainable code. Understanding when and how to use these methods will undoubtedly enhance your programming prowess and enable you to tackle a wider range of challenges with ease.