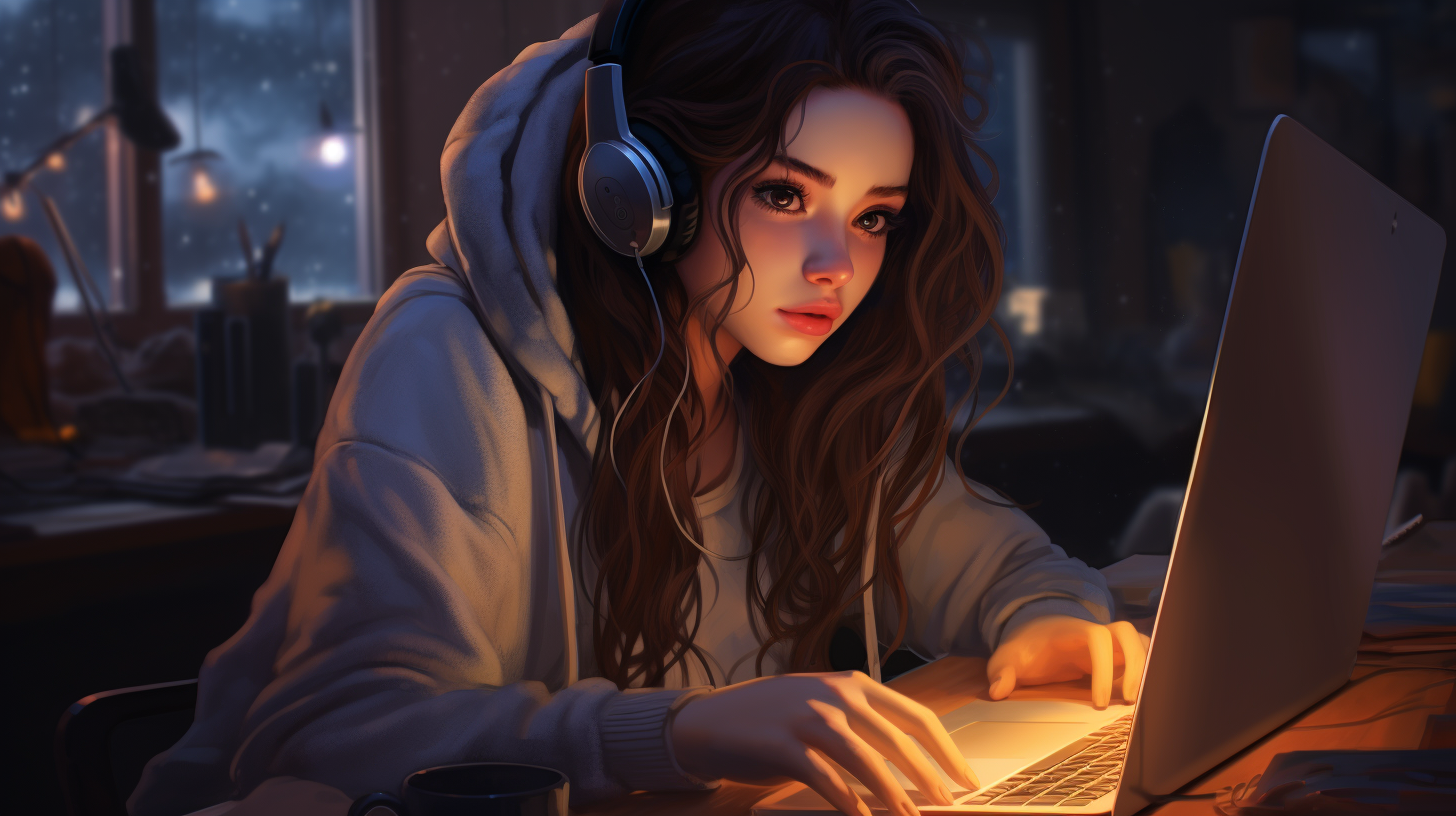
JavaScript Closures Explained
At its core, a closure in JavaScript is a powerful feature that allows a function to access variables from its outer (enclosing) scope, even after that outer function has finished executing. This behavior is fundamentally tied to the way JavaScript handles scoping and function execution.
When a function is created in JavaScript, it forms a lexical environment that includes any variables from its surrounding context. This environment is preserved in memory, allowing the inner function to continue to access those outer variables even once the outer function has returned.
Ponder the following example:
function outerFunction() { let outerVariable = 'I am outside!'; function innerFunction() { console.log(outerVariable); } return innerFunction; } const closureFunction = outerFunction(); closureFunction(); // Outputs: I am outside!
In this snippet, outerFunction
defines a variable outerVariable
and returns innerFunction
. When we invoke outerFunction
, it returns a reference to innerFunction
while retaining access to its lexical scope. When we call closureFunction
, it correctly logs outerVariable
, demonstrating the closure in action.
Closures enable a range of programming techniques, including data encapsulation and the creation of private variables. This allows developers to shield internal state from the outside world, providing a cleaner and more manageable code structure.
As you delve deeper into closures, you will encounter various nuances and capabilities that can enhance your JavaScript programming. Understanding how closures operate at a fundamental level will empower you to leverage them effectively across your applications.
How Closures Work in Practice
To grasp how closures operate in practice, it is essential to recognize their role in preserving state across function calls. Closures allow inner functions to “remember” the environment in which they were created, leading to some interesting and often powerful programming patterns.
Ponder a scenario where you want to create a simple counter function that increments a value each time it is called. Using closures, you can encapsulate the counter variable within a function scope, effectively creating a private state that cannot be accessed directly from the outside. Here’s how it can be implemented:
function createCounter() { let count = 0; // This variable is private to createCounter return function() { count += 1; // Increment the count variable return count; // Return the updated count }; } const counter = createCounter(); console.log(counter()); // Outputs: 1 console.log(counter()); // Outputs: 2 console.log(counter()); // Outputs: 3
In the example above, the createCounter
function initializes a variable count
. Each time the returned function is invoked, it can access and modify count
thanks to the closure, which keeps count
alive even after createCounter
has finished executing. This encapsulation ensures that count
cannot be accessed or modified from outside the createCounter
scope, effectively simulating private variables.
Closures also play a significant role in asynchronous programming, especially in scenarios involving callbacks or event handlers. For instance, ponder the following example where we set up a timeout:
function delayedGreeting(name) { setTimeout(function() { console.log('Hello, ' + name + '!'); // Accessing the outer variable name }, 1000); } delayedGreeting('Alice'); // Outputs: Hello, Alice! (after 1 second)
Here, the delayedGreeting
function takes a parameter name
and sets up a timeout to log a greeting message. The inner function used as a callback retains access to the name
variable, showcasing how closures extend the lifetime of variables across asynchronous calls.
Understanding how closures work in these practical contexts can significantly enhance your ability to write efficient, robust, and clean JavaScript code. By using closures effectively, you can craft functions that maintain their own state and respond intelligently to function calls, leading to a more modular and organized codebase.
Common Use Cases for Closures
Closures are invaluable in a multitude of scenarios, particularly when it comes to maintaining state, encapsulation, and creating more modular code. Their versatility can be observed in several common use cases that developers encounter regularly.
One prominent use case for closures is in event handling. When dealing with events in JavaScript, closures can help maintain access to specific data points that were present at the time an event handler was defined. For instance, ponder a scenario where we wish to generate a series of buttons that each log their index when clicked:
function createButtons() { const buttons = []; for (let i = 0; i < 5; i++) { buttons[i] = document.createElement('button'); buttons[i].innerText = 'Button ' + i; buttons[i].addEventListener('click', function() { console.log('Button ' + i + ' clicked'); }); document.body.appendChild(buttons[i]); } } createButtons();
In this example, each button is assigned a click event listener that references the index variable i
. Thanks to the closure created by the event listener, each button retains its own specific value of i
at the moment the button was created, allowing the correct button index to be logged when clicked.
Another powerful use case of closures is in the creation of factory functions. These functions can generate multiple instances of similar objects while encapsulating shared state. Here’s an illustration of how that can work:
function createPerson(name) { return { getName: function() { return name; // Closure preserves the name variable }, greet: function() { console.log('Hello, ' + name + '!'); } }; } const alice = createPerson('Alice'); const bob = createPerson('Bob'); alice.greet(); // Outputs: Hello, Alice! bob.greet(); // Outputs: Hello, Bob!
In this case, createPerson
acts as a factory that generates person objects. Each object retains access to its own name
through closures, enabling them to maintain distinct identities and behaviors even after the factory function has executed.
Moreover, closures are frequently used in functional programming patterns, particularly for creating higher-order functions. These are functions that return other functions or accept functions as arguments. A classic example is implementing a function that generates a multiplier:
function makeMultiplier(factor) { return function(x) { return x * factor; // factor is preserved through closure }; } const double = makeMultiplier(2); console.log(double(5)); // Outputs: 10
Here, makeMultiplier
returns a new function that multiplies its input by a fixed factor. The closure allows the inner function to access factor
even after makeMultiplier
has completed execution, demonstrating the utility of closures in creating flexible and reusable functions.
Lastly, closures are also beneficial in creating memoized functions—functions that cache results to improve performance by avoiding duplicate calculations:
function memoizedFib() { const cache = {}; // Cache for storing previously computed results return function fib(n) { if (n in cache) { return cache[n]; // Return cached result if available } if (n <= 1) { return n; // Base case for Fibonacci } const result = fib(n - 1) + fib(n - 2); cache[n] = result; // Save result to cache return result; }; } const fib = memoizedFib(); console.log(fib(10)); // Outputs: 55
In this memoization example, the inner function fib
can access the caching mechanism defined in the outer function, thus optimizing the repeated computation of Fibonacci numbers.
Closures serve as a cornerstone for several advanced programming concepts in JavaScript. Their ability to encapsulate state, maintain access to outer variables, and facilitate powerful design patterns renders them an essential tool in the arsenal of any proficient JavaScript developer.
Avoiding Pitfalls with Closures
{ return n; } const result = fib(n - 1) + fib(n - 2); cache[n] = result; // Store computed result in cache return result; }; } const fib = memoizedFib(); console.log(fib(10)); // Outputs: 55 console.log(fib(50)); // Outputs: 12586269025 (computed quickly due to caching)
In this memoized Fibonacci implementation, the closure maintains a cache of previously computed Fibonacci numbers, significantly improving performance by preventing recalculations. By using closures in this way, developers can write efficient algorithms that save time and resources.
Despite their a high number of benefits, closures can also lead to potential pitfalls if not managed carefully. One common issue arises when closures inadvertently retain references to variables that are expected to change. This can lead to unexpected behavior—particularly in asynchronous operations.
Ponder the following example:
function countdown(num) { for (var i = num; i >= 0; i--) { setTimeout(function() { console.log(i); // i is shared across all closures }, (num - i) * 1000); } } countdown(3); // Outputs: 4, 4, 4, 4 (after 0, 1, 2, 3 seconds)
In this snippet, the countdown function attempts to log the value of i at each second. However, because var creates a function-scoped variable, the closure retains a reference to the same i variable for all iterations. By the time the timeout functions execute, the loop has completed, resulting in the output of 4 for each timer.
To avoid this pitfall, you can use let instead of var, as let creates a block-scoped variable. This ensures each iteration maintains its own scope, thus preserving the correct value of i:
function countdown(num) { for (let i = num; i >= 0; i--) { setTimeout(function() { console.log(i); // Each closure retains its own i }, (num - i) * 1000); } } countdown(3); // Outputs: 3, 2, 1, 0 (as expected)
By understanding these potential pitfalls and employing best practices, you can harness the full power of closures without falling into common traps. With a solid grasp of how closures work and their implications, you can implement them effectively in your JavaScript applications, leading to more robust and maintainable code.