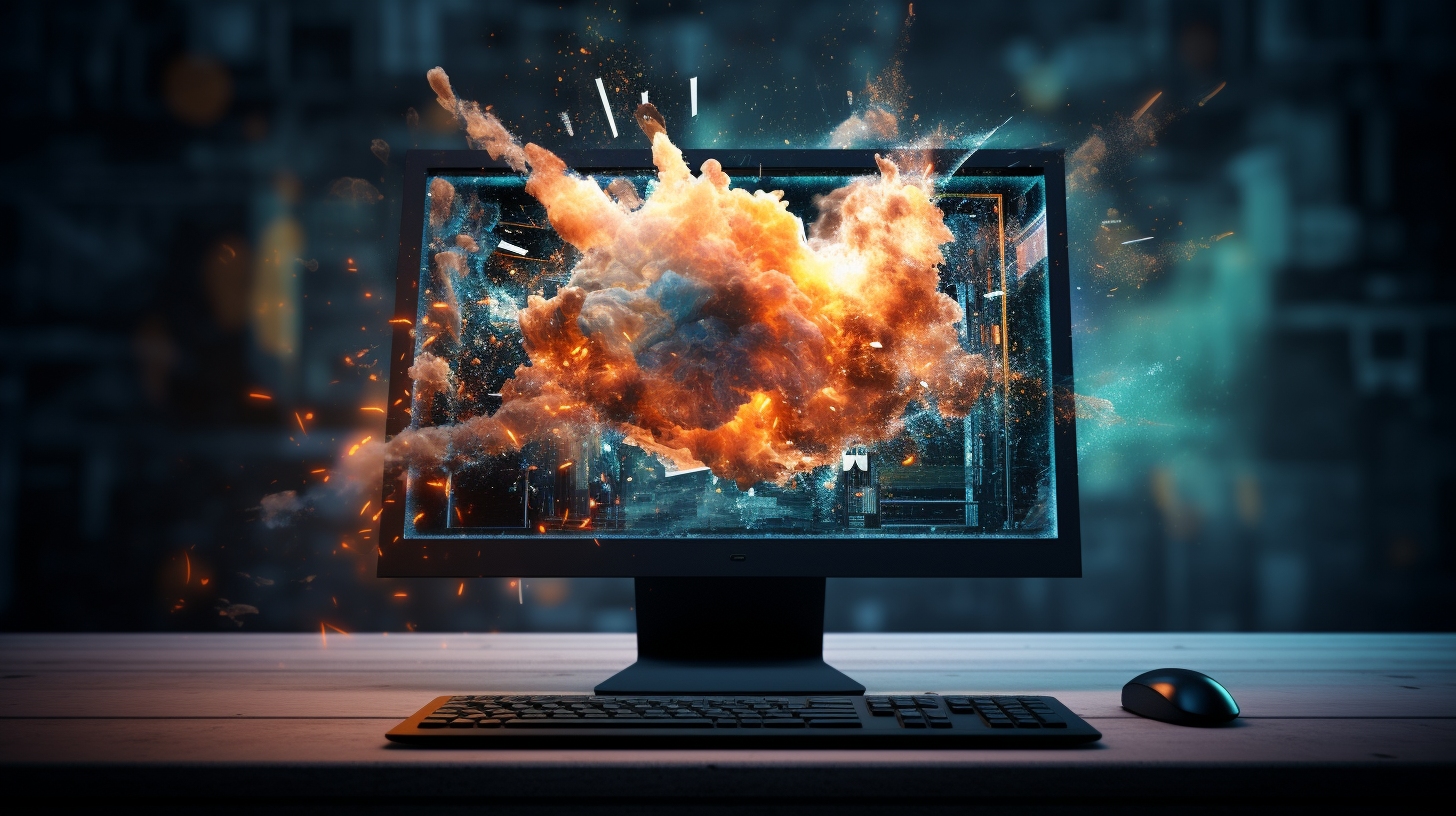
JavaScript Design Patterns
Design patterns are an important aspect of software development, serving as tried-and-true solutions to common problems encountered in programming. In JavaScript, design patterns help developers write cleaner, more efficient, and more maintainable code. To grasp the significance of design patterns in JavaScript, one must first understand what constitutes a design pattern.
A design pattern is essentially a template for solving a problem in a particular context. These patterns are not code per se but are rather guidelines or best practices that can be fleshed out in any programming language. They promote reusability and are instrumental in improving code readability and maintainability.
In JavaScript, due to its prototypal inheritance and first-class functions, certain patterns emerge that leverage these unique features of the language. Understanding these patterns not only helps in writing better code but also in communicating effectively with other developers, as these patterns often become a common language for discussing design.
There are three primary categories of design patterns: creational, structural, and behavioral patterns. Each serves a distinct purpose:
- Creational patterns deal with object creation mechanisms, optimizing code for various scenarios, such as when the creation process is complex.
- Structural patterns focus on composing classes or objects to form larger structures, ensuring flexibility and efficiency.
- Behavioral patterns manage algorithms and communication between objects, promoting loose coupling and enhancing code maintainability.
As we delve into JavaScript design patterns, it’s essential to ponder how these patterns can resolve typical issues developers face. For example, the Singleton pattern ensures a class has only one instance while providing a global point of access to it. This can be particularly useful in managing shared resources or configurations.
class Singleton { constructor() { if (!Singleton.instance) { Singleton.instance = this; } return Singleton.instance; } } const instance1 = new Singleton(); const instance2 = new Singleton(); console.log(instance1 === instance2); // true
In the above code, the singleton pattern prevents multiple instances of the Singleton class from being created, demonstrating how design patterns can enforce structure and predictability in JavaScript applications.
Ultimately, grasping the essence of design patterns equips JavaScript developers with the tools to tackle complex problems more efficiently, enhancing both their coding proficiency and the overall quality of the software they produce.
Common Design Patterns and Their Implementations
Continuing our exploration of common design patterns, let’s delve deeper into specific examples, illustrating their implementation in JavaScript.
Factory Pattern
The Factory Pattern is a creational design pattern that provides an interface for creating objects in a superclass, but allows subclasses to alter the type of objects that will be created. This pattern is particularly useful when the exact type of the object to be created isn’t known until runtime.
class Car { constructor(make, model) { this.make = make; this.model = model; } } class CarFactory { static createCar(make, model) { return new Car(make, model); } } const myCar = CarFactory.createCar('Toyota', 'Corolla'); console.log(myCar); // Car { make: 'Toyota', model: 'Corolla' }
In the example above, the CarFactory
class encapsulates the creation of Car
objects, allowing for greater flexibility and separation of concerns in our code.
Observer Pattern
The Observer Pattern is a behavioral design pattern that establishes a one-to-many relationship between objects, so when one object changes state, all its dependents are notified and updated automatically. That’s particularly useful for implementing event handling systems.
class Subject { constructor() { this.observers = []; } subscribe(observer) { this.observers.push(observer); } unsubscribe(observer) { this.observers = this.observers.filter(obs => obs !== observer); } notify(data) { this.observers.forEach(observer => observer.update(data)); } } class Observer { update(data) { console.log(`Observer received data: ${data}`); } } const subject = new Subject(); const observer1 = new Observer(); const observer2 = new Observer(); subject.subscribe(observer1); subject.subscribe(observer2); subject.notify('Hello Observers!'); // Observer received data: Hello Observers! // Observer received data: Hello Observers!
This implementation allows for dynamic subscription and notification, making it simple to manage and scale the communication within the application.
Module Pattern
The Module Pattern is a structural design pattern that helps in organizing code in a way that encapsulates private variables and functions while exposing only the public API. This is particularly beneficial in JavaScript due to its function scope.
const Module = (function() { let privateVar = 'I am private'; function privateMethod() { console.log(privateVar); } return { publicMethod: function() { privateMethod(); } }; })(); Module.publicMethod(); // I am private
Here, privateVar
and privateMethod
are inaccessible from outside the module, thus preserving encapsulation while allowing controlled access through the public API.
Decorator Pattern
The Decorator Pattern is a structural design pattern that allows behavior to be added to individual objects, either statically or dynamically, without affecting the behavior of other objects from the same class. This can be extremely useful for extending functionality in a flexible manner.
class Coffee { cost() { return 5; } } class MilkDecorator { constructor(coffee) { this.coffee = coffee; } cost() { return this.coffee.cost() + 1; } } const myCoffee = new Coffee(); const myCoffeeWithMilk = new MilkDecorator(myCoffee); console.log(myCoffeeWithMilk.cost()); // 6
In this example, MilkDecorator
enhances the original Coffee
class by adding new behavior without modifying its structure, showcasing the power of the decorator pattern.
As we continue to explore the vast landscape of design patterns in JavaScript, the implementations outlined here are crucial stepping stones. Each pattern addresses specific challenges and enhances our ability to navigate complex codebases efficiently, fostering a culture of clean and maintainable code in the JavaScript ecosystem.
Benefits of Using Design Patterns in JavaScript
Incorporating design patterns into JavaScript development yields a multitude of benefits that extend beyond mere code organization. At the heart of using these patterns lies the promise of a more streamlined development process, making it easier to handle both simple and complex applications alike.
Improved Code Readability and Maintainability: One of the foremost advantages of employing design patterns is the enhancement of code readability. When developers implement familiar patterns, even those new to a project can quickly grasp the intent and structure of the code. For example, using the Observer pattern for event handling provides a clear and consistent methodology that makes tracking changes across objects simpler. As a result, maintenance becomes less daunting, as developers can easily identify where changes need to occur without deciphering convoluted logic.
Facilitating Better Collaboration: In a team environment, design patterns serve as a common vocabulary. When developers use widely recognized patterns, they can communicate ideas and solutions more effectively, reducing misunderstandings. This shared understanding among team members enables smoother collaboration, as everyone is on the same page regarding the architectural decisions made throughout the codebase.
Encouragement of Reusability: Design patterns promote reusability, allowing developers to leverage existing solutions for common problems rather than reinventing the wheel. For instance, the Factory pattern can be reused in various parts of an application whenever object creation is needed. This not only reduces code duplication but also ensures consistency across the application, which very important for debugging and refining features as projects evolve.
Facilitating Scalability: The modular nature of many design patterns, such as the Module Pattern, supports scalability. By encapsulating functionality, developers can add new features or modify existing ones without impacting the entire system. This scalability is essential for larger applications that require ongoing enhancements and adaptations.
Enhanced Flexibility: Patterns like the Strategy pattern allow developers to define a family of algorithms and make them interchangeable. This flexibility permits the application to evolve without extensive rewrites, as behaviors can be changed at runtime. For example, ponder a sorting algorithm that can be swapped out based on user preference, thus providing a more dynamic user experience.
class Sorter { constructor(strategy) { this.strategy = strategy; } sort(data) { return this.strategy.sort(data); } } class QuickSort { sort(data) { // Quick sort implementation } } class BubbleSort { sort(data) { // Bubble sort implementation } } const sorter = new Sorter(new QuickSort()); sorter.sort([5, 3, 8, 1]); // Uses QuickSort sorter.strategy = new BubbleSort(); sorter.sort([5, 3, 8, 1]); // Now uses BubbleSort
Performance Optimization: Some patterns, especially creational patterns, can lead to performance enhancements. By optimizing object creation and management, applications can minimize resource consumption, which is vital in environments where performance is a critical factor.
Choosing to implement design patterns in JavaScript is not merely an academic exercise; it is a strategic decision that can significantly improve the development lifecycle. By embracing these established solutions, developers are empowered to create robust, efficient, and maintainable code, ultimately leading to a higher quality of software that can adapt to future demands.
Real-World Applications of JavaScript Design Patterns
Real-world applications of JavaScript design patterns manifest in various scenarios, from web development to server-side applications. Understanding how to implement these patterns effectively can provide structure and robustness to projects, ultimately leading to higher quality outcomes.
Consider the MVC (Model-View-Controller) pattern, a structural design pattern that has become a mainstay in web application development. This pattern separates concerns by dividing the application into three interconnected components. The Model manages the data, the View presents the data to the user, and the Controller handles user input. This separation facilitates easier testing and maintenance, as each component can be developed and modified independently.
class Model { constructor() { this.data = []; } addItem(item) { this.data.push(item); this.notify(); // Notify observers (views) } notify() { // Logic to update views } } class View { constructor(model) { this.model = model; this.model.subscribe(this); } update() { console.log('View updated with new data:', this.model.data); } } class Controller { constructor(model) { this.model = model; } addItem(item) { this.model.addItem(item); } } const model = new Model(); const view = new View(model); const controller = new Controller(model); controller.addItem('New Item'); // View updated with new data: ['New Item']
In this example, the MVC pattern promotes a clean separation of logic, which leads to easier maintenance and a more organized codebase. Each component has a distinct responsibility, allowing teams to collaborate more effectively on different parts of the application.
Another example is the Command pattern, a behavioral design pattern that encapsulates a request as an object. This pattern enables users to parameterize clients with queues, requests, and operations. It also provides support for undoable operations, making it a powerful tool in applications that require transaction-like features, such as editor applications.
class Command { execute() {} } class AddCommand extends Command { constructor(receiver, item) { super(); this.receiver = receiver; this.item = item; } execute() { this.receiver.addItem(this.item); } } class Editor { constructor() { this.items = []; } addItem(item) { this.items.push(item); console.log('Item added:', item); } } const editor = new Editor(); const addCommand = new AddCommand(editor, 'Hello World'); addCommand.execute(); // Item added: Hello World
By encapsulating the logic of adding an item into a command object, you gain the advantage of reusability and the ability to extend functionality without modifying existing code. The Command pattern showcases the flexibility and power of design patterns when dealing with user interactions and operations.
Furthermore, the use of the Facade pattern can provide a simplified interface to a complex subsystem. That is particularly useful in applications with multiple APIs, where developers need to interact with several services concurrently. By creating a facade, you can abstract the complexity and present a cleaner API to the user, thus improving the overall usability of the application.
class API { fetchData() { return 'Data fetched from API'; } fetchAdditionalInfo() { return 'Additional info fetched'; } } class Facade { constructor(api) { this.api = api; } fetchAllData() { return `${this.api.fetchData()} and ${this.api.fetchAdditionalInfo()}`; } } const api = new API(); const apiFacade = new Facade(api); console.log(apiFacade.fetchAllData()); // Data fetched from API and Additional info fetched
In this case, the Facade pattern facilitates interaction with the API by providing a unified interface to the client. It prevents the client from dealing with the complexities of the subsystem, thus resulting in a cleaner and more manageable codebase.
Ultimately, the real-world application of design patterns in JavaScript not only enhances the structure of the code but also enables developers to maintain flexibility and adaptability as projects evolve. By using the power of these established patterns, teams can build robust applications that stand the test of time, while also streamlining their development process.