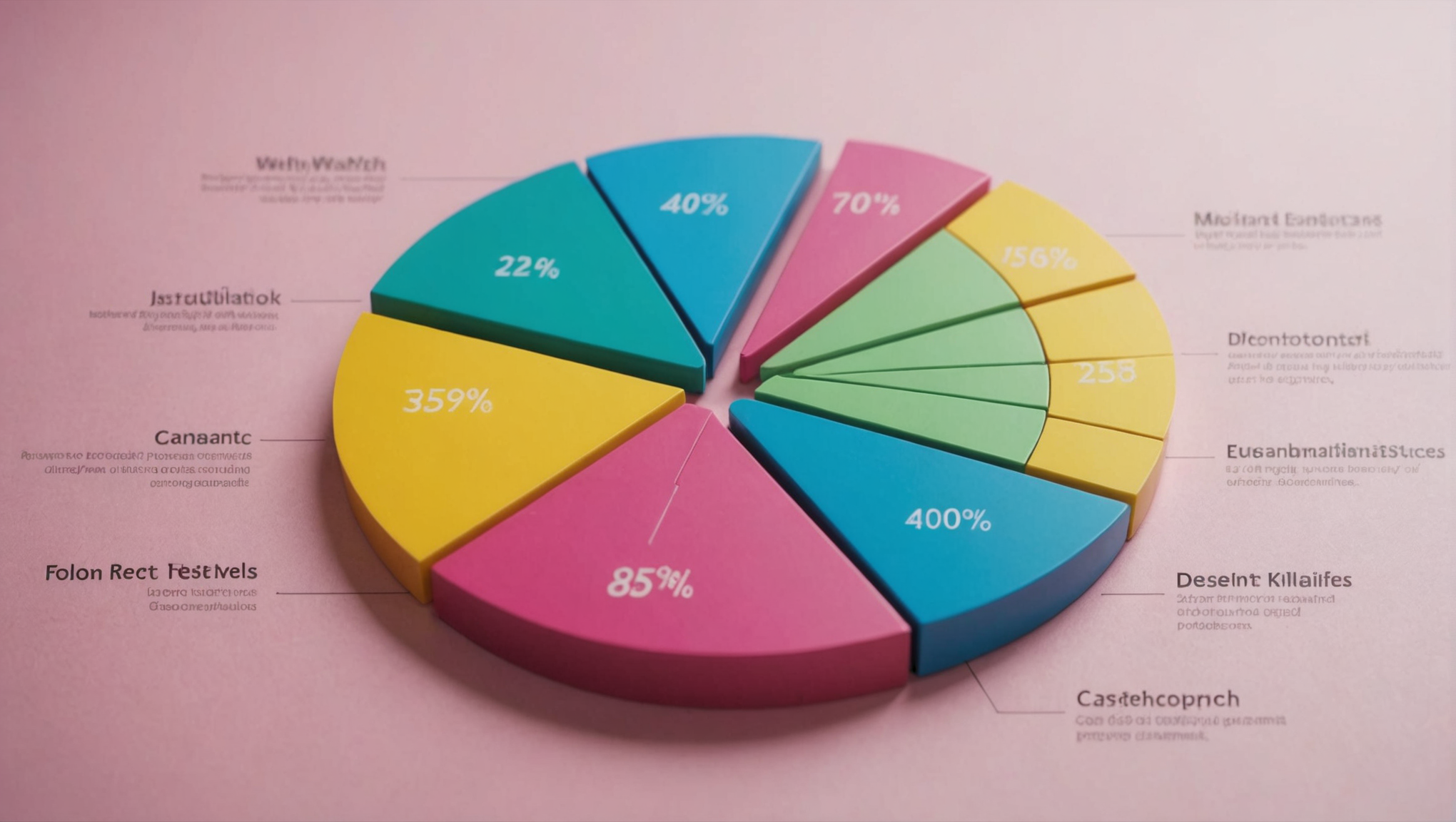
JavaScript for Environmental and Green Tech
In the delicate tapestry of our modern existence, where each thread of life interweaves with the fate of the planet, the art of visualization emerges as a profound means to comprehend the intricate patterns of environmental data. JavaScript, that versatile muse of the digital realm, offers a palette rich in colors and textures for rendering this complexity into forms that stir the soul and enlighten the mind.
To chart the ethereal dance of carbon emissions, one might employ a library such as D3.js, which allows the transformation of raw data into vivid, interactive graphics. Ponder a scenario where the cacophony of numbers is distilled into a gentle wave of a line chart, tracing the fluctuations of air quality across time. The code to bring this vision to fruition is both poetic and profound:
const data = [ { year: 2018, value: 20 }, { year: 2019, value: 25 }, { year: 2020, value: 15 }, { year: 2021, value: 30 }, { year: 2022, value: 10 } ]; const svg = d3.select("svg") .attr("width", 500) .attr("height", 300); const xScale = d3.scaleBand() .domain(data.map(d => d.year)) .range([0, 500]) .padding(0.1); const yScale = d3.scaleLinear() .domain([0, d3.max(data, d => d.value)]) .range([300, 0]); svg.selectAll(".bar") .data(data) .enter().append("rect") .attr("class", "bar") .attr("x", d => xScale(d.year)) .attr("y", d => yScale(d.value)) .attr("width", xScale.bandwidth()) .attr("height", d => 300 - yScale(d.value));
This snippet, etched in the ether of the web, conjures a visual narrative that beckons observers to think the implications of environmental stewardship. Each bar, a testament to the years that have passed, tells a story of progress and regression—a reminder of our collective responsibility.
As one delves deeper into the realm of JavaScript, one discovers the appeal of libraries such as Chart.js, which simplify the construction of various types of charts with elegance and ease. Imagine a pie chart that speaks in colors, illustrating the distribution of renewable energy sources in our society. The craft of this creation is equally compelling:
const ctx = document.getElementById('myPieChart').getContext('2d'); const myPieChart = new Chart(ctx, { type: 'pie', data: { labels: ['Solar', 'Wind', 'Hydro', 'Geothermal'], datasets: [{ label: 'Energy Sources', data: [30, 40, 20, 10], backgroundColor: ['#FF6384', '#36A2EB', '#FFCE56', '#4BC0C0'] }] }, options: { responsive: true } });
Each segment of the pie, each color, is imbued with significance, inviting the viewer to reflect on the balance of energy consumption and the potential for a future that embraces sustainability. Herein lies the power of JavaScript: to transform abstract data into visual experiences that resonate within the heart and mind.
But the journey does not end with mere visualization; it extends into the realm of interactivity, where the observer’s curiosity can lead to deeper understanding. The incorporation of tools such as Leaflet.js enables the mapping of environmental changes over geographical landscapes, revealing shifts that might otherwise remain hidden.
const map = L.map('map').setView([51.505, -0.09], 13); L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', { maxZoom: 19, }).addTo(map); const marker = L.marker([51.5, -0.09]).addTo(map); marker.bindPopup('A significant event in our environmental history.').openPopup();
In this tableau, the map becomes a canvas of awareness, each marker a beacon illuminating events that shape our ecological narrative. JavaScript, with its myriad capabilities, empowers developers not merely to observe, but to engage, to incite action, and to cultivate a deeper connection with the world around us.
Thus, the threads of data visualization weave a complex and beautiful fabric, one that harmonizes the relentless march of technology with the urgent call of environmental consciousness. It is through these visual articulations that we may hope to inspire change, invoking a collective sense of duty to nurture and protect our fragile planet.
Building Sustainable Applications with JavaScript
In the endeavor to build sustainable applications, JavaScript stands as a formidable ally, offering pathways through which the ethereal concept of sustainability can be transformed into tangible experiences that enrich our lives while honoring the delicate balance of nature. As the architect of such applications, one may find themselves conjuring the spirit of sustainability within the very structure of the code, crafting solutions that not only serve a purpose but also resonate with the ethos of care for our planet.
At the heart of this mission lies the ability to create applications that monitor and manage resources with precision and grace. Imagine, if you will, a web application that tracks energy consumption within a household, a guardian of efficiency that whispers insights into the daily habits of its users. JavaScript, with its responsive capabilities, allows for the seamless integration of APIs that communicate with smart devices, enabling the user to visualize their energy usage in real-time, thus fostering a consciousness that nurtures sustainability.
Here, we might employ a framework such as Express.js in conjunction with a database like MongoDB to manage our data, along with a front end that utilizes React or Vue.js to present the information in a compelling way. The following snippet illustrates a simple API endpoint that gathers energy usage data:
const express = require('express'); const mongoose = require('mongoose'); const app = express(); mongoose.connect('mongodb://localhost:27017/energyDB', { useNewUrlParser: true, useUnifiedTopology: true }); const energySchema = new mongoose.Schema({ device: String, usage: Number, timestamp: Date }); const EnergyUsage = mongoose.model('EnergyUsage', energySchema); app.get('/api/usage', async (req, res) => { const usageData = await EnergyUsage.find(); res.json(usageData); }); app.listen(3000, () => { console.log('Server is running on port 3000'); });
This code sets the stage for a symbiotic relationship between technology and sustainability, allowing users to reflect on their energy consumption patterns, to discern the impact of their daily choices. With every kilowatt saved, a step closer is taken toward a more sustainable future.
Moreover, the appeal of gamification beckons, inviting developers to weave narratives that encourage users to engage with their environmental footprint. By employing libraries such as GreenSock for animations or incorporating scoring systems, one can transform mundane tasks into thrilling quests for sustainability. For instance, a rewards feature that celebrates users when they reduce their energy consumption can significantly enhance engagement:
function checkEnergySavings(currentUsage, previousUsage) { if (currentUsage < previousUsage) { alert('Congratulations! You have saved energy this month!'); } } checkEnergySavings(150, 200);
In this whimsical dance of code and purpose, the application not only serves as a tool but as a companion in the journey toward eco-consciousness, nurturing habits that may ripple through communities and beyond.
As we traverse the vibrant landscape of building sustainable applications, one must not overlook the profound potential inherent in the integration of educational resources. By using JavaScript’s capabilities to create interactive quizzes or informative modules, developers can empower users with knowledge, fostering a deeper understanding of environmental issues and the importance of sustainable practices. This approach can extend to integrating third-party libraries that facilitate educational content delivery, thus ensuring that the message of sustainability is not just heard, but felt:
const quizData = [ { question: 'What percentage of energy should come from renewable sources by 2030?', options: ['30%', '50%', '70%'], answer: '50%' } ]; function displayQuiz() { quizData.forEach((item) => { console.log(item.question); item.options.forEach(option => console.log(option)); }); } displayQuiz();
Herein lies the beauty of JavaScript—a tool not merely for crafting applications, but for shaping a narrative that intertwines technology with the urgent call for ecological mindfulness. The journey of creating sustainable applications is one steeped in creativity and innovation, inviting developers to become stewards of the environment through their craft. In this delicate dance, we find not just code, but a canvas upon which the future of our planet may be painted, one sustainable choice at a time.
Integrating IoT Solutions for Green Technology
As we venture into the harmonious confluence of technology and ecology, the integration of Internet of Things (IoT) solutions for green technology unfurls a tapestry of possibilities, each thread woven with intention, illuminating paths toward a more sustainable existence. JavaScript emerges as an important instrument in this symphony, orchestrating the communication between devices, sensors, and users, creating an ecosystem replete with data and automation that seeks to preserve the sanctity of our environment.
Imagine a world where every flicker of a light bulb or the gentle hum of a thermostat is monitored in real-time, where the ethereal lines of code translate into tangible outcomes that nurture our planet. In this context, we can employ frameworks such as Node.js to bridge the gap between hardware and software, empowering developers to construct applications that not only respond to user input but also adapt to environmental conditions. The following illustration captures the essence of this integration:
const express = require('express'); const mqtt = require('mqtt'); const app = express(); const client = mqtt.connect('mqtt://broker.hivemq.com'); client.on('connect', () => { console.log('Connected to MQTT broker'); client.subscribe('environment/temperature'); }); client.on('message', (topic, message) => { const temperature = message.toString(); console.log(`Current temperature: ${temperature}°C`); }); app.listen(3000, () => { console.log('Server listening on port 3000'); });
Here, the code forms a bridge, a conduit through which temperature data flows, transforming raw readings into insights that guide decision-making. Each message received from the MQTT broker serves not just as a number, but as a clarion call, urging us to respond with wisdom and care. This is the beauty of integrating IoT within the sphere of green technology—a dialogue between devices and the environment that enhances our understanding of energy consumption and resource management.
Moreover, the interplay of JavaScript with IoT devices fosters a deeper engagement with our surroundings. Ponder an application that allows users to control smart irrigation systems, ensuring that every drop of water is a testament to our commitment to conservation. By employing a combination of JavaScript and APIs, we can create a effortless to handle interface that communicates with these systems, optimizing water usage based on real-time data:
function adjustIrrigation(moistureLevel) { if (moistureLevel < 30) { console.log('Activating irrigation system'); // Code to activate irrigation } else { console.log('Soil moisture sufficient, irrigation not needed'); } } adjustIrrigation(25);
In this instance, the juxtaposition of data and action unfolds, as the moisture sensor whispers its secrets to the application, prompting a response that echoes our aspiration for efficiency and responsibility. Each decision made is informed by the environment, ensuring that the delicate balance of nature remains undisturbed.
Furthermore, the allure of real-time monitoring can extend to energy consumption in commercial buildings, where the collective wisdom of IoT devices coalesces into an overarching strategy for sustainability. JavaScript can play a pivotal role in creating dashboards that visualize energy usage, allowing building managers to glean insights that lead to actionable changes. The following snippet illustrates a simple means of fetching and displaying energy data:
fetch('http://api.energydata.com/building/usage') .then(response => response.json()) .then(data => { console.log(`Total energy usage: ${data.totalUsage} kWh`); });
This code encapsulates a moment of clarity, where raw energy metrics transform into narratives that can drive policies and behaviors toward sustainability. The act of monitoring and analyzing empowers us, offering a lens through which we can witness the impact of our actions on the greater ecosystem.
Thus, the integration of IoT solutions with JavaScript stands as a testament to human ingenuity, a dance of innovation that beckons us to embrace the future while honoring the past. These interconnected systems, rich with data and potential, remind us that every action taken within the digital realm can translate into meaningful outcomes for our planet. In this intricate web of technology and nature, we find the promise of a harmonious existence, where the threads of code intertwine with the very fabric of life itself.
Case Studies: Successful JavaScript Projects in Environmental Initiatives
In the realm of environmental initiatives, the marriage of JavaScript and creativity has birthed a plethora of projects that echo the urgent clarion call for ecological preservation. Each case study, a microcosm of innovation, stands as a testament to human ingenuity, illustrating how lines of code can translate into actions that reverberate through communities and ecosystems alike.
Consider the initiative of an open-source platform that harnesses the power of crowdsourced data to track urban air quality. Developers, inspired by the plight of communities affected by pollution, utilized JavaScript to create an application that captures real-time data from various sensors scattered throughout a city. By employing libraries such as Socket.IO for real-time communication, they fashioned a living, breathing map that pulsates with information, each data point a narrative of environmental health:
const io = require('socket.io')(3000); io.on('connection', (socket) => { console.log('New connection established'); socket.on('airQualityData', (data) => { console.log(`Air quality level in ${data.location}: ${data.level}`); // Code to update a database or user interface }); });
This snippet reveals the heartbeat of the application, a rhythm that resonates with the community’s desire for transparency and action. Each connection forged between sensor and server not only gathers data but cultivates a collective awareness, inspiring citizens to advocate for cleaner air and healthier living environments.
Another remarkable venture showcases the ingenuity of JavaScript in the realm of habitat conservation. A team of developers collaborated with environmentalists to create a web-based tool that visualizes deforestation trends across the globe. By employing the Leaflet.js library, they crafted an interactive map that chronicles the battle against habitat loss, inviting users to explore the data through a simple to operate interface:
const map = L.map('map').setView([0, 0], 2); L.tileLayer('https://{s}.tile.openstreetmap.org/{z}/{x}/{y}.png', { maxZoom: 19, }).addTo(map); fetch('/api/deforestation_data') .then(response => response.json()) .then(data => { data.forEach(entry => { L.marker([entry.latitude, entry.longitude]) .addTo(map) .bindPopup(`Year: ${entry.year}, Area: ${entry.area} km²`); }); });
Through this endeavor, the developers not only disseminate crucial information but also invoke a sense of urgency, urging viewers to reflect on their role in the preservation of the planet’s rich biodiversity. Each marker on the map serves as a reminder of the fragile ecosystems that hang in the balance, challenging observers to act with intention.
In yet another illuminating case, a project emerged that intertwines education and action through a gamified platform designed to engage youth in environmental stewardship. Developers leveraged JavaScript to create an interactive game where players earn rewards for completing tasks related to sustainability—be it recycling, conserving water, or reducing energy consumption. The code that orchestrates this engagement is both playful and purposeful:
let score = 0; function completeTask(task) { console.log(`Task Completed: ${task}`); score += 10; // Increment score for each task completed alert(`Your score: ${score}`); } completeTask('Recycled plastic bottles');
This playful approach transforms mundane actions into delightful experiences, fostering a culture of environmental awareness among the younger generation. The gamification of sustainability not only captivates but also cultivates habits that extend beyond the screen, weaving a fabric of consciousness that spans communities.
Thus, as we traverse the landscape of these case studies, each project unfurls like a blossom, rich with potential and purpose. JavaScript, in its elegance and versatility, emerges not merely as a tool for technical execution but as a catalyst for change—inviting us all to participate in the vital narrative of environmental preservation, urging us to weave our own threads into the intricate tapestry of sustainability.