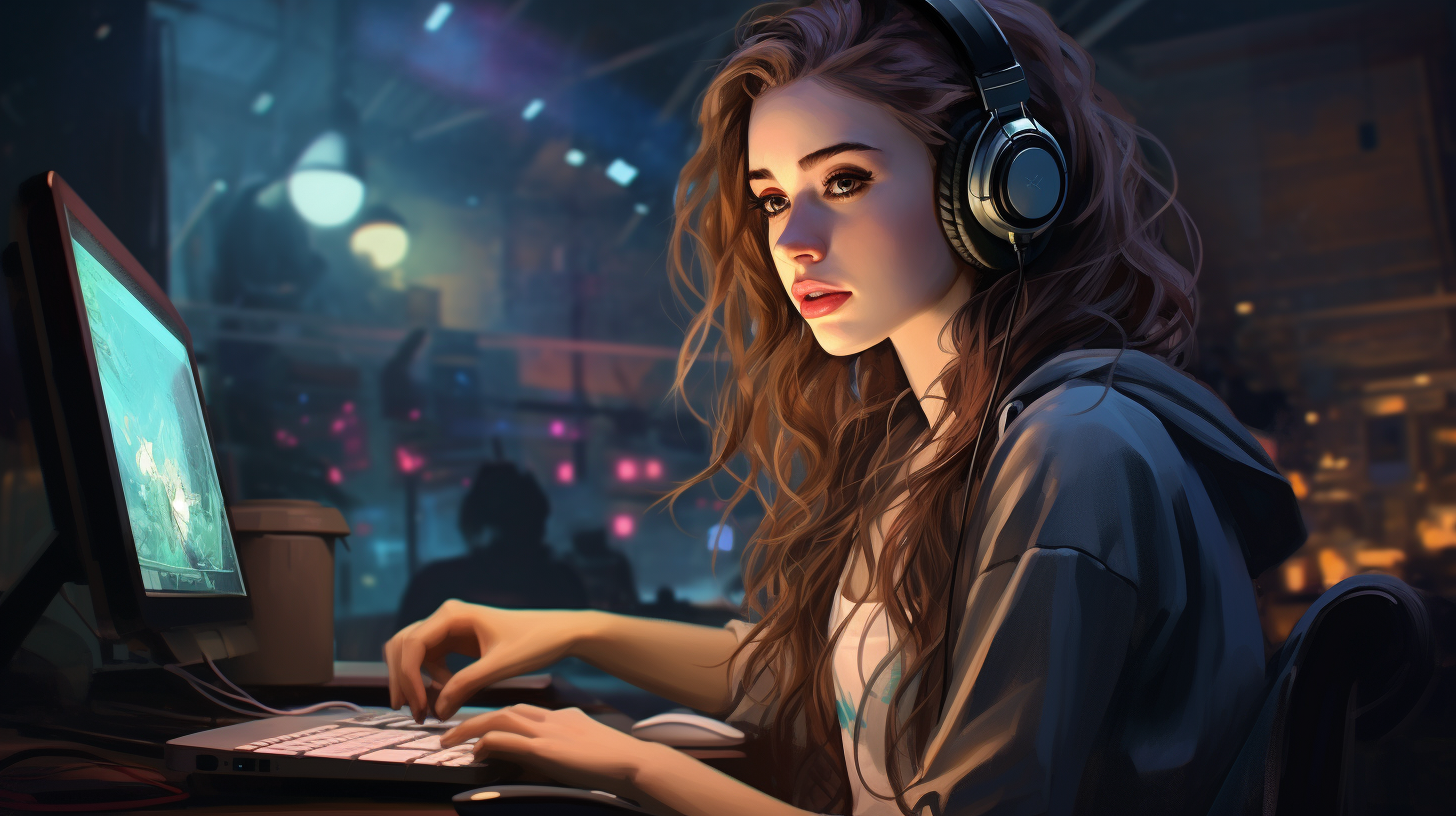
JavaScript for Interactive Art and Installations
JavaScript stands out as a powerful medium for artists and developers alike, enabling the creation of interactive experiences that captivate audiences. At its core, JavaScript offers a vibrant ecosystem for expressing creativity through code, transforming static visuals into dynamic displays that respond to user input.
One of the most alluring aspects of JavaScript is its ability to manipulate the Document Object Model (DOM) in real-time, allowing artists to create fluid animations and transformations that breathe life into their work. By using the capabilities of state-of-the-art web browsers, developers can forge intricate visual narratives that engage viewers on a deeper level.
Think the creation of a simple interactive canvas. Here, the canvas
element combined with JavaScript can serve as a blank slate for artistic expression:
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); canvas.width = window.innerWidth; canvas.height = window.innerHeight; let particles = []; function createParticle(x, y) { particles.push({ x: x, y: y, size: Math.random() * 5 + 1 }); } function drawParticles() { ctx.clearRect(0, 0, canvas.width, canvas.height); particles.forEach(particle => { ctx.beginPath(); ctx.arc(particle.x, particle.y, particle.size, 0, Math.PI * 2); ctx.fillStyle = 'rgba(255, 255, 255, 0.8)'; ctx.fill(); }); } canvas.addEventListener('click', (event) => { createParticle(event.clientX, event.clientY); }); function animate() { drawParticles(); requestAnimationFrame(animate); } animate();
This code snippet sets up a canvas where clicking creates particles that scatter across the screen. It demonstrates the immediacy with which an artist can iterate upon their ideas, adding layers of complexity and interactivity. Through each click, the canvas evolves, embodying the spirit of spontaneity this is often essential in artistic creation.
Moreover, JavaScript’s asynchronous capabilities allow for seamless integration of multimedia elements, such as sound and video, further enriching the interactive experience. By communicating with APIs, artists can pull in external data to inform their visuals, creating installations that not only react to user behavior but also to the world around them.
Tools like the Web Audio API extend the auditory potential of interactive art, enabling soundscapes that respond to visual stimuli. Imagine visual elements pulsing in sync with sound, creating a holistic experience that envelops the audience. Such interactions can be crafted through simpler JavaScript, allowing for experimentation and improvisation without the constraints typically associated with traditional art forms.
Ultimately, the creative potential of JavaScript lies in its flexibility and accessibility. As developers explore its depths, they uncover novel ways to communicate ideas and evoke emotions, turning code into a canvas and bringing imagination to life in thrilling new fashions.
Key Libraries and Frameworks for Interactive Art
When delving into the world of interactive art, it becomes evident that using established libraries and frameworks can significantly enhance the creative process. These tools provide robust functionalities, allowing artists to focus on their vision rather than the intricacies of low-level programming. A few standout libraries and frameworks that have made a mark in this domain include p5.js, Three.js, and Paper.js, each catering to different aspects of interactive and visual art.
p5.js is particularly beloved within the creative coding community for its effortless to handle syntax and comprehensive set of features. It was designed with artists in mind and offers a friendly interface that simplifies the process of drawing and animation. With p5.js, artists can create captivating visual experiences by writing simpler code that feels almost like a natural extension of their artistic instincts. Below is a basic example demonstrating how to create a dynamic sketch using p5.js:
function setup() { createCanvas(windowWidth, windowHeight); background(0); } function draw() { noStroke(); fill(random(255), random(255), random(255), 150); ellipse(random(width), random(height), random(10, 50)); }
In this snippet, the setup
function prepares the canvas, while the draw
function continuously generates random colored circles on the screen. The randomization adds a layer of unpredictability, making each viewing experience unique.
Three.js, on the other hand, is a powerful library specifically tailored for creating 3D graphics in the browser. It abstracts away many of the complexities involved in WebGL programming, making it accessible for artists who want to explore three-dimensional spaces. With Three.js, artists can build immersive environments that invite users to interact with intricate models and scenes. Here’s a minimal example of setting up a 3D cube:
const scene = new THREE.Scene(); const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000); const renderer = new THREE.WebGLRenderer(); renderer.setSize(window.innerWidth, window.innerHeight); document.body.appendChild(renderer.domElement); const geometry = new THREE.BoxGeometry(); const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 }); const cube = new THREE.Mesh(geometry, material); scene.add(cube); camera.position.z = 5; function animate() { requestAnimationFrame(animate); cube.rotation.x += 0.01; cube.rotation.y += 0.01; renderer.render(scene, camera); } animate();
This example illustrates how to create a spinning cube using Three.js. The simplicity of the code belies the complexity of the visual experience, as users can navigate around the cube, enhancing the interactive element of the artwork.
Paper.js is another noteworthy framework, primarily focused on vector graphics. It allows for precise control over shapes and paths, making it perfect for artists who wish to create intricate designs. Paper.js introduces a powerful object model that simplifies working with vector graphics through easy-to-use APIs. Below is a basic example of creating a simple graphic:
paper.setup('myCanvas'); const circle = new Path.Circle({ center: view.center, radius: 50, }); circle.fillColor = 'red'; const text = new PointText({ content: 'Hello, Paper.js!', point: [50, 50], fillColor: 'white', fontSize: 20, });
In this snippet, we create a red circle and overlay a text element in a simple yet effective manner. Paper.js enables detailed manipulation of graphics, allowing artists to express their vision through powerful vector tools.
The breadth of libraries available in the JavaScript ecosystem empowers artists to explore diverse styles and methodologies within interactive art. By using these frameworks, creators can shift their focus from the technical challenges to the artistic expression itself, tapping into a rich vein of creative potential that JavaScript unlocks.
Techniques for Engaging User Interaction
Engaging user interaction is a cornerstone of creating memorable interactive art experiences. Techniques for achieving this engagement can vary significantly, but they often revolve around understanding user behavior and designing responsive elements that cater to it. JavaScript, with its robust event handling capabilities, enables creators to craft experiences that resonate with audiences on multiple sensory levels.
One of the most fundamental interactions artists can implement is the mouse movement or hover effect. By responding to the cursor’s position, artworks can create a sense of discovery and excitement. A simple example involves changing colors or shapes based on where the user moves their mouse:
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); canvas.width = window.innerWidth; canvas.height = window.innerHeight; canvas.addEventListener('mousemove', (event) => { ctx.clearRect(0, 0, canvas.width, canvas.height); const x = event.clientX; const y = event.clientY; ctx.fillStyle = `hsl(${(x / canvas.width) * 360}, 100%, 50%)`; ctx.beginPath(); ctx.arc(x, y, 50, 0, Math.PI * 2); ctx.fill(); });
In this example, as the user moves their mouse across the canvas, a circle follows their cursor, changing its color based on the horizontal position. This real-time response not only keeps the user engaged but also transforms the act of moving the mouse into a participatory artistic experience.
Another effective technique for user interaction is using keyboard events to alter the artwork dynamically. By listening for specific key presses, an artist can introduce layers of complexity to their installation. Here’s a simple illustration:
document.addEventListener('keydown', (event) => { if (event.key === 'r') { ctx.fillStyle = 'red'; } else if (event.key === 'g') { ctx.fillStyle = 'green'; } else if (event.key === 'b') { ctx.fillStyle = 'blue'; } });
In this snippet, pressing different keys changes the color of the artwork. This interaction not only engages the user actively but also allows them to influence the outcome of the art in a playful manner. Such familiarity with keyboard controls can create a deeper connection between the user and the artwork, as they feel a sense of ownership over the experience.
Touch events are equally vital, especially in mobile contexts. As more users engage with art installations through touch devices, incorporating touch interactions can significantly enhance the experience. Here’s an example illustrating how to respond to touch events:
canvas.addEventListener('touchstart', (event) => { const touch = event.touches[0]; createParticle(touch.clientX, touch.clientY); });
This code allows users to create particles by tapping on the screen, making the artwork accessible to a broader audience. By considering touch interactions, artists can ensure that their installations are inclusive and engaging across various devices.
Using sound is another powerful way to heighten user engagement. Integrating the Web Audio API allows for creating rich auditory landscapes that can respond to visual changes, enhancing the immersive quality of the piece. For instance, users could trigger different sounds by interacting with visual elements:
const audioContext = new (window.AudioContext || window.webkitAudioContext)(); function playSound(frequency) { const oscillator = audioContext.createOscillator(); oscillator.type = 'sine'; oscillator.frequency.setValueAtTime(frequency, audioContext.currentTime); oscillator.connect(audioContext.destination); oscillator.start(); oscillator.stop(audioContext.currentTime + 0.5); } canvas.addEventListener('click', (event) => { const frequency = Math.random() * 1000; playSound(frequency); });
In this scenario, every click generates a sound at a random frequency, creating an auditory feedback loop that encourages users to explore the piece further. The combination of visual and auditory elements amplifies the interactivity, making the experience more captivating.
Ultimately, the techniques for engaging user interaction are as varied as the artists creating them. Through a blend of visual stimuli, responsive mechanics, and sensory feedback, JavaScript enables a dynamic interplay between the audience and the artwork. By embracing these techniques, artists can forge deeper connections with their viewers, transforming passive observation into active participation.
Case Studies: Successful Interactive Art Installations
let installations = [ { title: "The Obliteration Room", artist: "Yayoi Kusama", description: "An immersive environment where visitors cover a completely white room with colorful dot stickers, transforming it into a vibrant installation.", interaction: "Visitors interact by placing stickers on surfaces, which changes the overall aesthetic and feel of the space." }, { title: "Interactive Wall", artist: "Random International", description: "A wall that responds to the presence of users, creating dynamic visual patterns based on their movements.", interaction: "The installation tracks movements and generates visual representations that reflect the audience's interaction in real time." }, { title: "The Night Cafe", artist: "Teo Yu Siang", description: "A virtual reality rendition of Vincent van Gogh's painting, offering an interactive experience within the iconic setting.", interaction: "Users can walk through the space and interact with various elements, bringing the artwork to life in a new and engaging way." } ]; installations.forEach(installation => { console.log(`${installation.title} by ${installation.artist}: ${installation.description}`); console.log(`Interaction: ${installation.interaction}`); });
In the context of interactive art installations, case studies provide rich insights into how artists leverage JavaScript and technology to improve audience engagement. One notable example is “The Obliteration Room” by Yayoi Kusama. This immersive environment starts as a completely white room, inviting visitors to cover its surfaces with colorful dot stickers. As individuals participate, the stark contrast of the initial white space transforms into a vibrant explosion of color, showcasing the collective input of the audience. The simple act of placing stickers becomes a profound interaction with the artwork, making each visit unique and personal.
Another compelling case study is “Interactive Wall” by Random International. This installation employs advanced tracking technology to monitor user movements, allowing the wall to respond dynamically with captivating visual patterns. Each interaction creates a feedback loop where the audience’s actions influence the artwork in real time. The interplay between the viewer and the installation blurs the lines between creation and observation, fostering a deeper connection to the art.
Additionally, “The Night Cafe,” crafted by Teo Yu Siang, offers a mesmerizing virtual reality experience based on Vincent van Gogh’s iconic painting. Users navigate through a three-dimensional recreation of the café, interacting with elements that bring the artwork to life. This case study exemplifies how JavaScript can transcend traditional boundaries, inviting participants to step inside a painting and engage with it on a personal level through exploration and interaction.
These installations illustrate the power of JavaScript in crafting experiences that resonate with audiences. By integrating user participation into the fabric of the artwork, artists can evoke emotions, provoke thoughts, and create lasting memories. The potential for innovation in interactive art is limitless, as each case study demonstrates the unique ability of technology to enhance human creativity and engagement.
const installations = [ { title: "Digital Rain", artist: "Rafael Lozano-Hemmer", description: "An interactive installation where users can manipulate virtual rain falling on a screen, altering its speed and direction.", interaction: "Participants control the rain through gestures, creating an intimate relationship with the digital environment." }, { title: "The Singularity", artist: "Diana Thater", description: "Using projectors and sensors, this installation immerses users in a shifting landscape of light and color.", interaction: "As viewers move through the space, they influence the projection, making each experience unique." }, { title: "Submergence", artist: "Squidsoup", description: "A multi-sensory installation featuring thousands of suspended lights that respond to visitors' movements.", interaction: "As users walk through the space, they illuminate lights, creating a personalized light experience." } ]; installations.forEach(installation => { console.log(`${installation.title} by ${installation.artist}: ${installation.description}`); console.log(`Interaction: ${installation.interaction}`); });
Another fascinating case is “Digital Rain” by Rafael Lozano-Hemmer, where users manipulate virtual rain on a screen, affecting its speed and direction through gestures. This installation showcases how JavaScript can facilitate interactive experiences that allow users to exert control over the artwork, fostering a sense of agency and involvement.
Similarly, “The Singularity” by Diana Thater employs projectors and sensors to create an immersive environment that reacts to user movements. Each viewer’s interaction alters the projection, resulting in a dynamic and personalized experience. This exemplifies the capacity of technology to create a responsive art form that evolves with its audience.
Lastly, “Submergence” by Squidsoup captivates with its array of suspended lights that respond to visitors’ movements. As users navigate through the space, they trigger an intricate dance of illumination, creating an enchanting atmosphere that can be uniquely experienced by each individual. This installation illustrates how interactivity can elevate the sensory experience, merging sight with participation in a harmonious dialogue.
These diverse case studies underscore the transformative potential of JavaScript in the context of interactive art. By intricately weaving user engagement into the artistic narrative, installations can transcend traditional forms, inviting audiences to become co-creators in a shared experience. Each interaction leaves an imprint, turning passive spectators into active participants in the unfolding story of the artwork.