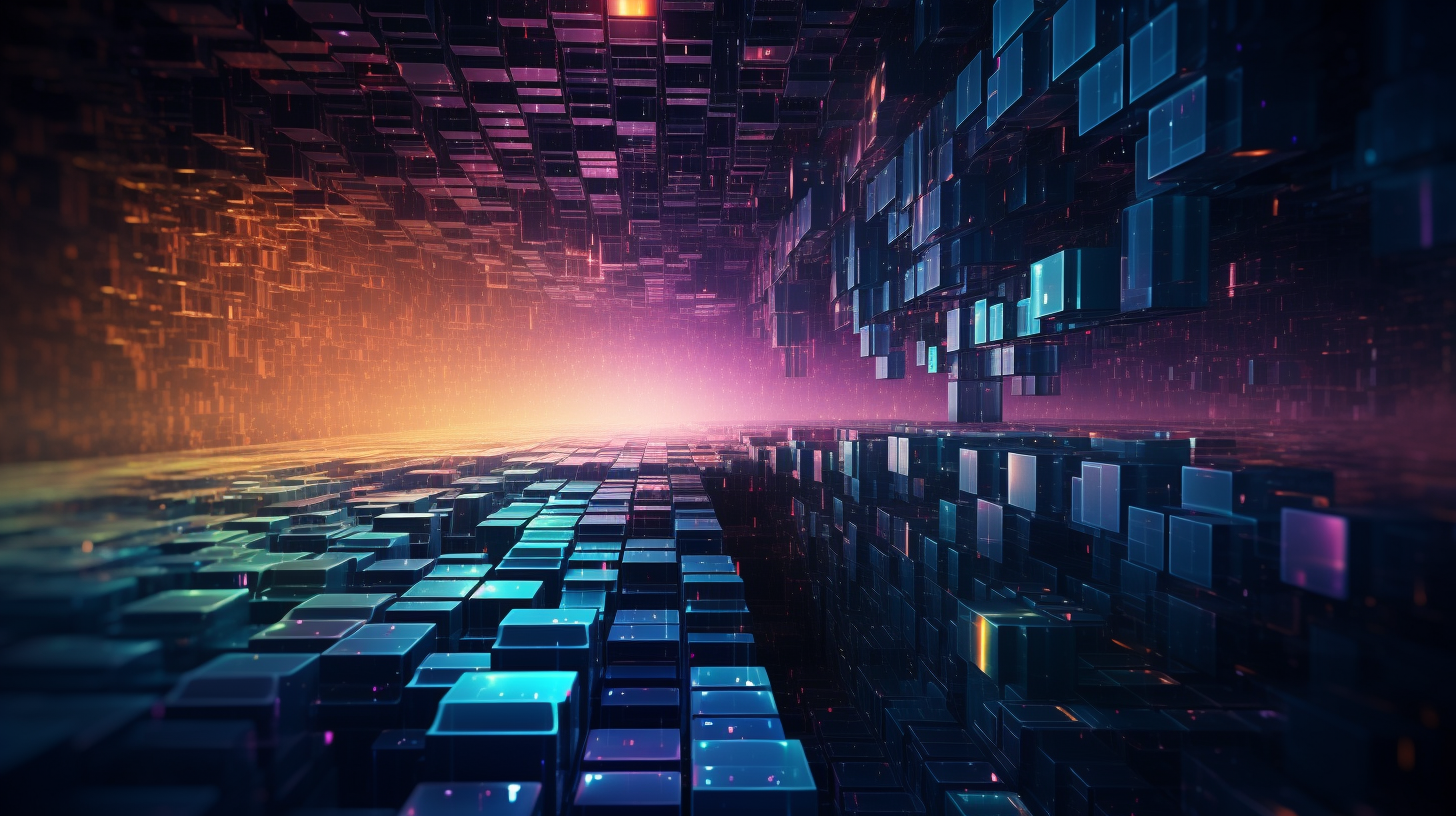
JavaScript for Mobile App Development
In the sphere of mobile app development, using JavaScript frameworks can significantly streamline the process, enhancing both productivity and performance. Several frameworks exist, each designed with different strengths tailored to mobile environments. Understanding these frameworks very important for selecting the right tools for your projects.
One of the most notable frameworks is React Native. Developed by Facebook, it allows developers to build mobile applications using JavaScript and React. React Native enables the creation of truly native applications, meaning the components you use are compiled to native code rather than being rendered in a web view. This results in a performance boost, as the app benefits from native performance and UI elements.
import React from 'react'; import { View, Text, StyleSheet } from 'react-native'; const App = () => { return ( Hello, React Native! ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', backgroundColor: '#F5FCFF', }, text: { fontSize: 20, textAlign: 'center', }, }); export default App;
Another prominent framework is Ionic, which allows developers to create hybrid mobile applications using web technologies like HTML, CSS, and JavaScript. Ionic is particularly appealing for developers familiar with Angular or React, as it provides a rich set of pre-designed UI components that make building responsive applications more manageable.
import { Component } from '@angular/core'; @Component({ selector: 'app-home', template: ` Hello, Ionic!Welcome to your Ionic App!
`, }) export class HomePage {}
NativeScript is another framework worth mentioning. It enables building native mobile applications using Angular, Vue.js, or plain JavaScript. NativeScript provides the capability to call native APIs directly and access native UI components, granting a high level of flexibility and performance.
import { Frame } from '@nativescript/core'; export function navigatingTo(args) { const page = args.object; page.bindingContext = { title: 'Hello, NativeScript!' }; } export function goToNextPage() { Frame.topmost().navigate('next-page'); };
Lastly, Flutter deserves mention, even though it primarily uses Dart. However, it allows for embedding JavaScript through plugins, thus enabling web developers to utilize their existing skills while building cross-platform applications. Flutter’s architecture and performance characteristics make it an attractive solution for high-performance apps.
When tackling mobile app development with JavaScript, choosing the right framework can drastically influence the efficiency and quality of your work. Each framework comes with its own set of features and benefits, making it imperative to evaluate the specific needs of your project before deciding. The landscape is rich with options, allowing developers to pick the tools that best align with their project goals and personal skills.
Native vs. Hybrid Mobile Applications
In the sphere of mobile application development, the choice between native and hybrid applications often sparks considerable debate among developers. Each approach offers distinct advantages and drawbacks, significantly impacting the overall development process, user experience, and performance of the application.
Native applications are built specifically for a particular platform, such as iOS or Android, using platform-specific programming languages and tools. For instance, iOS applications are typically developed using Swift or Objective-C, while Android applications are often built in Java or Kotlin. This specialization allows native applications to take full advantage of the device’s hardware and software capabilities, leading to superior performance and a more seamless user experience.
One of the key benefits of native applications is their ability to provide a rich, responsive user interface that feels natural on the device. They have direct access to device features such as the camera, GPS, and push notifications, allowing for functionality this is tightly integrated with the operating system. For example, a simple native application for taking photos might look like this in Swift:
import UIKit class CameraViewController: UIViewController { let imagePicker = UIImagePickerController() override func viewDidLoad() { super.viewDidLoad() imagePicker.delegate = self imagePicker.sourceType = .camera } @IBAction func openCamera() { present(imagePicker, animated: true, completion: nil) } }
On the other hand, hybrid applications combine elements of both native and web applications. They are built using web technologies like HTML, CSS, and JavaScript, and are typically wrapped in a native shell, allowing them to be deployed across multiple platforms. Frameworks like Ionic, Cordova, and React Native facilitate this process, enabling developers to write code once and deploy it everywhere.
While hybrid apps benefit from a faster development cycle and reduced cost, they may not match the performance of native applications. They often rely on web views to render content, which can introduce latency and affect the app’s responsiveness. However, modern frameworks have made significant strides in closing this gap. For instance, a simple hybrid application might look like this when using Ionic:
import { Component } from '@angular/core'; @Component({ selector: 'app-home', template: ` Camera Open Camera ` }) export class HomePage { openCamera() { // Logic to open the camera } }
Ultimately, the choice between native and hybrid development hinges on various factors, including project requirements, timelines, and team expertise. Native applications are ideal for projects demanding high performance and access to device features, while hybrid applications are suitable for quicker development cycles and cross-platform deployment. As a developer, understanding these nuances allows you to make informed decisions that align with your project goals and user needs.
Building Responsive User Interfaces
Building responsive user interfaces in mobile app development is paramount, as users expect seamless and visually appealing experiences across various devices. JavaScript, combined with modern frameworks and libraries, provides powerful tools to create adaptive layouts that respond gracefully to different screen sizes and orientations.
One of the essential concepts in responsive design is the use of flexible layouts. CSS Flexbox and Grid are instrumental in achieving this flexibility. They allow developers to create layouts that can adjust dynamically based on the viewport size. For instance, using Flexbox, you can create a simple responsive card layout that rearranges based on the screen size:
.container { display: flex; flex-wrap: wrap; justify-content: space-around; } .card { flex: 0 1 300px; /* Flex-grow, flex-shrink, flex-basis */ margin: 10px; padding: 20px; background-color: #f8f9fa; border: 1px solid #ced4da; border-radius: 4px; }Card 1Card 2Card 3
CSS media queries further enhance responsiveness by allowing specific styles to be applied based on the device’s characteristics. For instance, you can adjust font sizes, margins, and visibility of elements depending on the screen width:
@media (max-width: 600px) { .card { flex: 0 1 100%; /* Full width on small screens */ } }
In addition to layout techniques, using JavaScript can also enhance user interfaces dynamically. For example, you can use event listeners to modify styles or classes in response to user interactions or screen size changes:
document.addEventListener('resize', () => { const cards = document.querySelectorAll('.card'); if (window.innerWidth { card.style.backgroundColor = '#e9ecef'; // Change color on small screens }); } else { cards.forEach(card => { card.style.backgroundColor = '#f8f9fa'; // Original color }); } });
Frameworks like React and Vue.js offer components and libraries that simplify responsive design further. For instance, libraries such as Material-UI or Bootstrap can provide pre-built responsive components that can be easily integrated into your applications. Here’s an example of a responsive button using React and Material-UI:
import React from 'react'; import Button from '@mui/material/Button'; const ResponsiveButton = () => { return ( ); }; export default ResponsiveButton;
By employing these techniques and tools, developers can create user interfaces that not only look great on all devices but also provide the functionality and performance that users expect. This adaptability is key with the current focus on mobile app development, where user experience is at the forefront of success.
Accessing Device Features with JavaScript
Accessing device features is one of the most powerful aspects of modern mobile app development with JavaScript. Whether you are developing a native or hybrid application, tapping into the hardware capabilities of the device can significantly enhance the functionality and user experience of your app. This capability allows developers to create applications that feel more integrated and responsive to the user’s context.
JavaScript frameworks, such as React Native and Ionic, provide APIs that allow you to access various device features like the camera, GPS, accelerometer, and more. These APIs enable you to build applications that leverage the full potential of mobile devices, letting you create experiences that go beyond static content.
For example, if you are using React Native, you can access the device’s camera using the `react-native-camera` library. Here’s a simple implementation that demonstrates how to capture a photo:
import React, { useRef } from 'react'; import { View, Button, Image } from 'react-native'; import { RNCamera } from 'react-native-camera'; const CameraComponent = () => { const cameraRef = useRef(null); const [imageUri, setImageUri] = React.useState(null); const takePicture = async () => { if (cameraRef.current) { const options = { quality: 0.5, base64: true }; const data = await cameraRef.current.takePictureAsync(options); setImageUri(data.uri); } }; return ( {imageUri && } ); }; export default CameraComponent;
This example illustrates how easy it’s to integrate camera functionality into a React Native application. The `RNCamera` component provides a simple interface for accessing the camera, while the `takePicture` function handles the capturing process, so that you can display the captured image immediately.
In hybrid applications developed with frameworks like Ionic, accessing device features is often done through plugins. For instance, if you want to access the device’s geolocation, you can use the `@ionic-native/geolocation` plugin. Here’s how you might retrieve the user’s current location:
import { Component } from '@angular/core'; import { Geolocation } from '@ionic-native/geolocation/ngx'; @Component({ selector: 'app-home', template: ` Geolocation Example Get Current LocationLatitude: {{ location.coords.latitude }}`, }) export class HomePage { location: any; constructor(private geolocation: Geolocation) {} getLocation() { this.geolocation.getCurrentPosition().then((resp) => { this.location = resp; }).catch((error) => { console.error('Error getting location', error); }); } }
Longitude: {{ location.coords.longitude }}
In this Ionic example, we leverage the Geolocation plugin to fetch the user’s current coordinates. The `getLocation` method invokes the `getCurrentPosition` function, which retrieves the location data and displays it on the screen. This integration showcases how hybrid apps can interact with native device features effectively.
Moreover, when accessing device features, it is crucial to handle permissions properly. Both native and hybrid apps require user consent to access certain features, such as the camera or location services. On Android, for instance, you must declare permissions in the `AndroidManifest.xml`, while in iOS, you specify them in the `Info.plist`. Failure to do so can lead to runtime exceptions or denial of access to essential features.
As you explore accessing device features in your JavaScript mobile applications, remember that the right framework and proper handling of permissions are vital for creating robust, user-friendly experiences that leverage the unique capabilities of mobile devices.
Performance Optimization Techniques
When it comes to performance optimization techniques in mobile app development using JavaScript, it is essential to focus on both the efficiency of the code and the overall user experience. Performance is a critical aspect that can significantly impact how users perceive and interact with your application. To achieve optimal performance, consider various strategies that include minimizing resource usage, optimizing rendering, and using caching.
One of the first steps in optimizing performance is to minimize the size of your JavaScript bundles. Tools like Webpack, Rollup, or Parcel can help you achieve this by allowing you to tree-shake unused code, bundle your application efficiently, and even serve minified files in production. Here’s an example of a Webpack configuration that optimizes your build for production:
module.exports = { mode: 'production', entry: './src/index.js', output: { filename: 'bundle.js', path: __dirname + '/dist', }, module: { rules: [ { test: /.js$/, exclude: /node_modules/, use: { loader: 'babel-loader', options: { presets: ['@babel/preset-env'], }, }, }, ], }, optimization: { minimize: true, }, };
Another effective measure is to debounce or throttle events, especially those that could fire rapidly, such as scrolling or resizing. Implementing these techniques can prevent your application from becoming overburdened by too many function calls in a short period. Here’s how you can create a simple debounce function:
function debounce(func, wait) { let timeout; return function executedFunction(...args) { const later = () => { clearTimeout(timeout); func(...args); }; clearTimeout(timeout); timeout = setTimeout(later, wait); }; };
Using the browser’s built-in capabilities can also yield significant performance benefits. For instance, to reduce layout thrashing, you should minimize DOM reads and writes. Batch your read and write operations together, as shown here:
function updateLayout() { const element = document.getElementById('myElement'); const width = element.offsetWidth; // Read const height = element.offsetHeight; // Read // Perform writes after reads element.style.width = `${width * 2}px`; element.style.height = `${height * 2}px`; }
Caching is another vital technique for enhancing performance. Use caching strategies to store frequently accessed data, thus reducing the number of network requests and speeding up load times. For example, you can implement caching in a service worker to cache assets and API responses:
self.addEventListener('install', (event) => { event.waitUntil( caches.open('my-cache-v1').then((cache) => { return cache.addAll([ '/', '/index.html', '/styles.css', '/script.js', ]); }) ); });
Moreover, using asynchronous programming with Promises or async/await helps keep your app responsive. By offloading heavy computations or network requests asynchronously, you can ensure that the main thread remains unblocked and the user interface remains smooth. For example:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error fetching data:', error); } }
Finally, performance profiling tools, such as Chrome DevTools or React Profiler, can provide insights into your application’s bottlenecks. Use these tools to monitor rendering times, evaluate memory usage, and identify long-running JavaScript operations. By analyzing these metrics, you can make informed decisions on where optimizations are most needed.
Implementing these performance optimization techniques will enhance the responsiveness and efficiency of your JavaScript mobile applications, ultimately leading to a better experience for users. Remember that performance is an ongoing process; as your application evolves, continuously monitor and optimize its performance to meet user expectations.
Testing and Debugging Mobile JavaScript Applications
Testing and debugging mobile JavaScript applications is a critical phase in the development lifecycle, directly influencing the overall quality of the final product. With the complexities of mobile environments, ensuring that your application runs smoothly across various devices and operating systems can be quite challenging. Employing robust testing methodologies and debugging strategies aids in identifying issues early, thereby enhancing the user experience and maintaining high app performance.
One of the fundamental practices in testing mobile applications is unit testing. This involves testing individual components or functions within your application for correctness. JavaScript developers frequently use testing frameworks like Jest, Mocha, or Jasmine to facilitate unit testing. For instance, a simple unit test using Jest might look like this:
test('adds 1 + 2 to equal 3', () => { expect(1 + 2).toBe(3); });
In the context of mobile app development, you may want to test specific functions that handle data processing or UI updates. Ensuring these functions behave as expected especially important. In addition to unit testing, integration testing is also important, as it evaluates how well different parts of your application work together. This can help catch issues that arise when multiple components interact.
When moving beyond unit tests, automated testing frameworks can further streamline the process. Tools such as Appium, Detox, or Cypress allow developers to automate the testing of user interactions in a mobile app. For example, using Appium, you can write tests that simulate user actions, such as tapping buttons or entering text:
const { Builder, By } = require('selenium-webdriver'); (async function example() { let driver = await new Builder().forBrowser('chrome').build(); try { await driver.get('http://localhost:3000'); // Navigate to the app await driver.findElement(By.id('myButton')).click(); // Simulate a button click const result = await driver.findElement(By.id('result')).getText(); console.log(result); // Log the result } finally { await driver.quit(); } })();
Debugging tools play a pivotal role in troubleshooting and fixing issues that arise during development. Mobile frameworks typically come with built-in debugging tools. For example, React Native offers the React Developer Tools, which provide insights into component hierarchies and state management, enabling developers to trace issues effectively.
Furthermore, browser developer tools can be utilized for debugging hybrid applications. With Chrome or Firefox, you can inspect elements, monitor network requests, and view console logs—all essential for diagnosing problems within your app. For example, using the console to log a variable’s state can help determine where the error lies:
console.log('Current state:', this.state);
Using source maps is another crucial technique for debugging JavaScript applications. Source maps allow you to see the original source code while debugging minified code, making it easier to identify where things went wrong. That’s particularly useful in production environments, where the source code may be compressed for performance.
In addition, using error tracking services like Sentry or Bugsnag can help monitor your app in real-time. These tools capture errors as they occur in users’ devices, providing valuable context like stack traces and user interactions leading up to the error. This information can significantly expedite the debugging process by pinpointing the exact conditions under which an issue arises.
Finally, user feedback is an invaluable resource in the testing and debugging process. Encourage users to report bugs or issues within the app. Implementing a feedback system can provide insights into how the application performs in the real world and highlight areas that need improvement.
Testing and debugging mobile JavaScript applications require a multifaceted approach that encompasses unit tests, automation, effective debugging tools, and real-time monitoring. By implementing these strategies, you can ensure a higher-quality application that performs reliably across a diverse range of devices and scenarios.