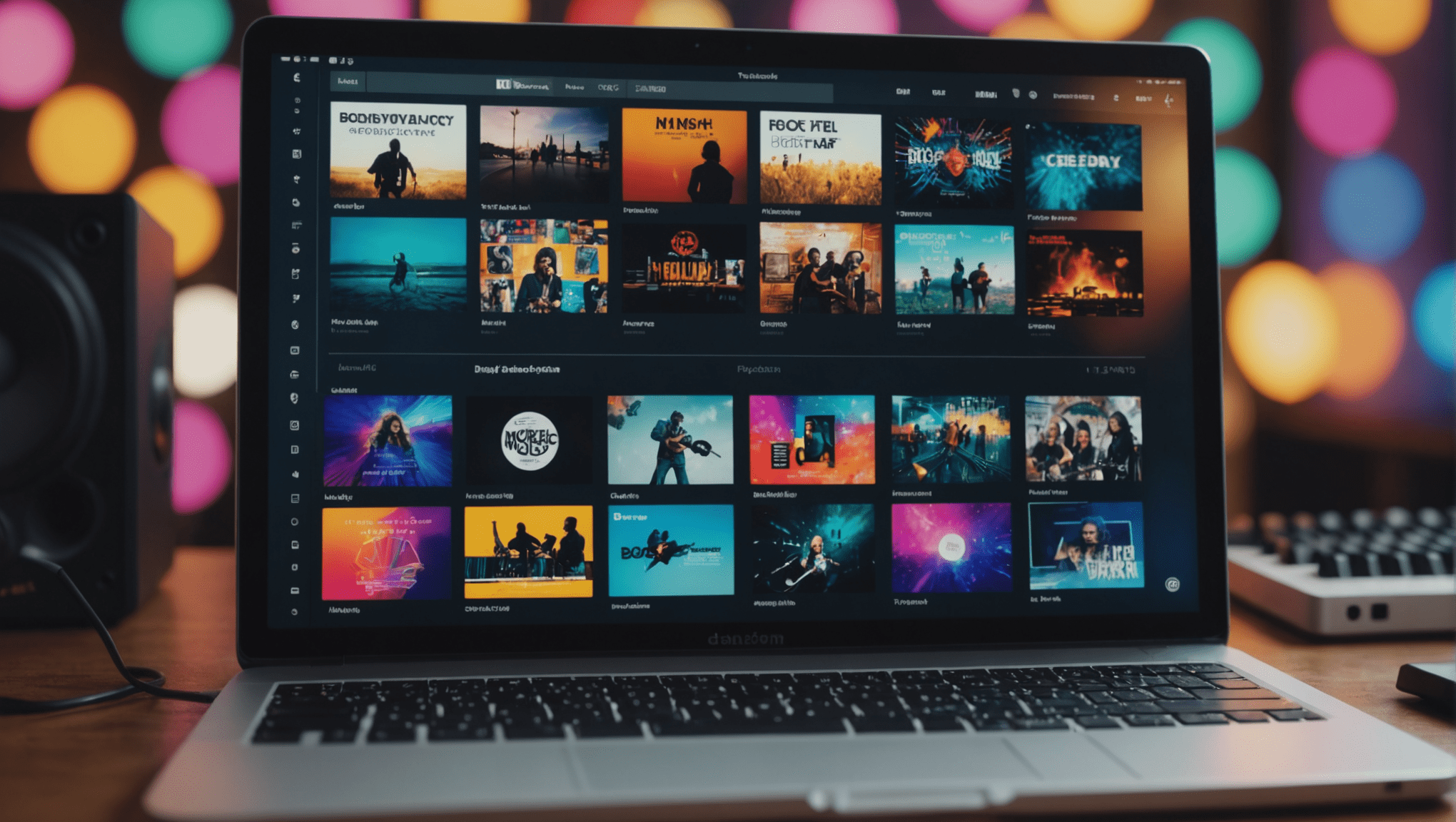
JavaScript for Music Streaming Services
In the ever-evolving world of music streaming services, where each note seems to dance upon the surface of digital waves, JavaScript emerges as a vital thread woven through the very fabric of user experience. With its elegance and versatility, JavaScript transforms static interfaces into dynamic realms, granting users an interactive canvas upon which they can paint their auditory journeys. Picture, if you will, a user browsing through an extensive library of melodies, each click producing not just a reaction, but a symphony of responses that resonate with the heart.
From the moment a user enters the domain of a music streaming service, JavaScript orchestrates a seamless ballet of functionality. Think the search functionality: as fingers glide over the keyboard, JavaScript captures each keystroke, sending queries to the server with a grace that belies the underlying complexity. That is achieved through techniques such as AJAX, allowing data to flow in real-time, thus enabling users to discover their favorite tracks without the clunky burden of page reloads.
fetch('/api/search?query=' + encodeURIComponent(userInput)) .then(response => response.json()) .then(data => { displayResults(data); }) .catch(error => console.error('Error fetching search results:', error));
Then, there is the enchanting ability to create personalized playlists. Here, JavaScript serves not merely as a tool but as an architect, building a structure that reflects the unique taste of each listener. Through the manipulation of arrays and objects, users can curate their sonic collections, dragging and dropping tracks with delightful ease. The dynamic updating of the user interface, as playlists are adjusted, is a testament to the prowess of JavaScript. Each interaction is met with immediate feedback, a gentle reminder that this digital space is alive and responsive.
function addTrackToPlaylist(trackId) { playlist.push(trackId); updatePlaylistUI(); }
Moreover, the integration of social features, a profound aspect of state-of-the-art streaming services, is made possible through JavaScript’s ability to handle asynchronous communication. Connections to social media platforms allow listeners to share their musical discoveries, creating a community that thrives on shared passions. The rhythmic pulse of notifications, likes, and comments brings a sense of camaraderie to the experience, each interaction a note in an ongoing composition of connectivity.
function shareTrack(trackId) { fetch('/api/share', { method: 'POST', body: JSON.stringify({ trackId }), headers: { 'Content-Type': 'application/json' } }) .then(response => response.json()) .then(data => console.log('Track shared:', data)) .catch(error => console.error('Error sharing track:', error)); }
With every keystroke and every interaction, JavaScript weaves a tapestry rich with functionality and engagement. It stands as the silent conductor of this orchestra, guiding the flow of music and the ebb of user interactions, ensuring that the experience is not merely transactional but deeply immersive. It invites users to explore a sea of sound, where each wave reflects their preferences, and every ripple speaks to the beauty of technology harmonizing with art.
Building Real-Time Features with WebSockets
But let us delve deeper into the realm of real-time features, where the magic of WebSockets emerges, shimmering like a beacon in the fog of digital isolation. Imagine a scenario where a user, engrossed in the rhythmic pulse of their chosen playlist, receives instantaneous updates about their friends’ listening habits—a notification that they have just discovered an obscure gem, a fleeting moment of shared experience that transforms solitary listening into a collective celebration. That’s the power of WebSockets, an elegant solution that allows for two-way communication between a client and server, allowing data to flow freely and continuously, unbound by the constraints of traditional HTTP requests.
Through the embrace of WebSockets, one can forge connections as vibrant as the notes cascading from a grand piano, where each message sent across this channel reverberates with the potential to enhance user interaction. Setting up a WebSocket connection begins with the simple act of establishing a link to the server, a handshake that promises a dialogue rich with possibilities. Here, JavaScript becomes the artist, deftly crafting these connections to ensure that the experience is fluid, responsive, and alive.
const socket = new WebSocket('wss://example.com/socket'); socket.onopen = function() { console.log('WebSocket connection established'); }; socket.onmessage = function(event) { const message = JSON.parse(event.data); displayNotification(message); }; socket.onclose = function() { console.log('WebSocket connection closed'); };
As the connection blossoms, the potential for real-time updates unfurls before us. Notifications about new releases, live chat with fellow listeners, and updates on concert tickets—all can be elegantly woven into the user experience. With every message received, the interface responds, blossoming with new information, creating a lively interaction that feels as spontaneous as a jazz improvisation. Herein lies the beauty of WebSockets; they allow for an immediate exchange, a flow of information that nurtures a sense of community among users, each notification a note in the grand symphony of shared musical experiences.
To harness this fluidity, think implementing features such as live chat or collaborative playlists, where users can see changes made by others in real-time, adding an element of excitement and unpredictability. Imagine the delight as friends collaborate on a playlist, each addition sparkled into existence without delay, a harmonious blend of tastes and preferences emerging before their eyes.
function sendMessage(message) { socket.send(JSON.stringify({ type: 'chat', content: message })); } socket.onmessage = function(event) { const message = JSON.parse(event.data); if (message.type === 'chat') { displayChatMessage(message.content); } };
Thus, the integration of WebSockets within the JavaScript ecosystem breathes life into the user experience, rendering it a vivid tapestry of interactions, informed by the immediacy of connection. The listener is no longer a solitary figure; they are part of a dynamic collective, each heartbeat echoing in the spaces between tracks, each message a reminder that music, at its core, is a communal experience, meant to be shared, reveled in, and celebrated.
As we navigate the undulating waves of this digital ocean, it becomes clear that with WebSockets, the potential for real-time features is not merely a technical innovation but a philosophical embrace of connectivity—an invitation to revel in the joy of sharing, of discovering, and of being together, even when miles apart. And within this ever-expanding universe of sound and silence, JavaScript stands resilient, a steadfast companion in the voyage of musical exploration.
Implementing Audio Playback with the Web Audio API
Yet, as we traverse this landscape of sound, a deeper layer of engagement awaits—one that transforms the mere act of listening into a visceral experience. The Web Audio API, a marvel of modern technology, unfurls its wings, presenting a canvas upon which JavaScript can paint the intricacies of audio playback. Imagine, if you will, a symphony of sound not just emitted through speakers, but crafted and sculpted, each note shaped by the hands of an artist wielding code.
The Web Audio API provides a suite of tools that allow developers to manipulate audio with precision and grace. It brings forth the ability to create complex audio graphs, where sounds are not merely played but orchestrated, layered, and intertwined. Here, JavaScript steps into the role of composer, guiding the flow of audio, adjusting volumes, applying effects, and even creating spatial audio experiences that transport listeners into the very heart of the music.
Think the simple yet profound act of loading and playing a track. The Web Audio API invites us to create an audio context, a sanctuary where sound exists and flourishes. To embark on this journey, we first establish an audio context:
const audioContext = new (window.AudioContext || window.webkitAudioContext)();
With this context, we can now summon an audio buffer, a space where our music resides, ready to be explored. Loading the audio file, we encapsulate the essence of the track, preparing it for playback:
function loadAudio(url) { return fetch(url) .then(response => response.arrayBuffer()) .then(data => audioContext.decodeAudioData(data)); }
As the audio buffer emerges from the depths of our code, we can summon it forth to dance upon the ears of our listeners. We create a source node, a conduit through which the sound flows, and connect it to the audio context, painting the air with our chosen melody:
function playTrack(buffer) { const source = audioContext.createBufferSource(); source.buffer = buffer; source.connect(audioContext.destination); source.start(0); }
But the Web Audio API offers more than mere playback; it invites us to manipulate sound with a suite of audio nodes. We can add effects, envelop our music in reverb, and shape it with filters, creating an auditory experience that resonates deeply within the soul. Imagine enhancing a track with a gain node to adjust its volume, allowing the listener to bask in the warmth of sound:
const gainNode = audioContext.createGain(); gainNode.gain.setValueAtTime(0.5, audioContext.currentTime); // Adjust the volume to 50% source.connect(gainNode); gainNode.connect(audioContext.destination);
Moreover, the API’s capabilities extend to the spatial realm, enabling us to position sounds in a three-dimensional space. With the creation of a spatial audio context, listeners can feel the distance and directionality of sound, immersing them in the music as if they were surrounded by an orchestra:
const panner = audioContext.createPanner(); panner.setPosition(0, 0, -1); // Position the sound directly in front of the listener source.connect(panner); panner.connect(audioContext.destination);
This ability to manipulate audio not only enhances the user experience but also engages the listener’s imagination, allowing them to visualize the soundscape as it envelops them. Each adjustment, each effect applied, becomes a brushstroke on the canvas of sound, a testament to the artistry that lies within JavaScript.
In this realm, JavaScript stands not merely as a language, but as a conduit for creativity, a means to transform auditory experiences into something magical and profound. The Web Audio API, with its expansive capabilities, opens doors to innovation and exploration, inviting developers to craft unique sound experiences that resonate with users long after the final note has faded into silence. Each interaction becomes a part of a larger composition, where music is not just heard but felt, an echo that lingers in the heart and mind, a reminder of the beauty that lies at the intersection of technology and art.
User Authentication and Subscription Management
In the delicate dance of music streaming services, where each user seeks a unique harmony within a vast landscape of sound, the role of user authentication and subscription management is paramount. Here, JavaScript unfurls its wings, embracing the intricacies of identity and access, ensuring that each listener’s experience is tailored, secure, and seamless. Imagine a world where the digital gates to an expansive library of melodies swing open with a mere whisper of a password, where the listener’s identity is carefully curated, allowing them to explore the vast oceans of sound without fear or hesitation.
At the heart of this orchestration lies the login process, an important prelude that establishes the connection between user and service. JavaScript stands ready to facilitate this connection, transforming what could be a mundane task into a serene ritual. The act of logging in is not merely a transaction; it is an invitation into a world of personalized experiences, where each user feels recognized and valued. Ponder the elegant simplicity of an AJAX request that captures the essence of the user’s credentials, sending them forth to the server in search of validation.
function loginUser(username, password) { fetch('/api/login', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ username, password }) }) .then(response => response.json()) .then(data => { if (data.success) { storeUserSession(data.token); redirectToDashboard(); } else { displayError(data.message); } }) .catch(error => console.error('Error during login:', error)); }
Upon the successful validation of these credentials, a token is bestowed upon the user, an emblem of their identity within this vibrant ecosystem. This token serves as a key, unlocking personalized playlists, favorite tracks, and curated recommendations. Here, JavaScript intricately weaves session management into the fabric of the application, ensuring that each visit feels like a homecoming, familiar and welcoming.
function storeUserSession(token) { localStorage.setItem('authToken', token); }
Yet, the journey of personalized exploration does not end with mere authentication. Subscription management emerges, a harmonious extension of the user’s experience, where choices abound—free tiers, premium memberships, each offering a distinct journey through the auditory landscape. JavaScript takes on the role of a guide, presenting users with seamless options to upgrade, manage, or cancel their subscriptions, all the while ensuring their movements through the application feel fluid and instinctive.
function updateSubscription(plan) { fetch('/api/update-subscription', { method: 'POST', headers: { 'Authorization': 'Bearer ' + localStorage.getItem('authToken'), 'Content-Type': 'application/json' }, body: JSON.stringify({ subscriptionPlan: plan }) }) .then(response => response.json()) .then(data => { if (data.success) { displaySuccess(data.message); } else { displayError(data.message); } }) .catch(error => console.error('Error updating subscription:', error)); }
In this vast tapestry of sound, the balance between security and accessibility very important. JavaScript empowers developers to implement robust security measures, ensuring that users feel safe as they navigate their musical journeys. The beauty of hashing passwords, the elegance of secure token storage, and the vigilance of session timeouts intertwine to create a fortified sanctuary for users, safeguarding their identities amid the ever-expanding universe of streaming services.
Within this realm, the notion of user authentication transcends mere verification; it becomes a dance of trust and engagement. As users explore their preferences, their playlists bloom like wildflowers in spring, each choice a reflection of their individual tastes, nurtured by a system that understands and anticipates their desires. JavaScript, functioning as the invisible hand, guides this process with grace, ensuring that every user feels seen, heard, and valued in this harmonious confluence of technology and art.
Thus, as the user embarks on their journey through the vast expanse of music, they do so with the knowledge that they are not just another voice lost in the cacophony, but rather a cherished participant in an ongoing symphony—a melody this is uniquely their own, woven into the fabric of a collective experience, thanks to the elegant orchestration of JavaScript within the realm of user authentication and subscription management.
Optimizing Performance for Large Audio Libraries
In the realm of music streaming services, where vast libraries brim with melodies waiting to be unearthed, the need for optimization becomes a clarion call, resonating through the corridors of technology and art. Here, JavaScript, with its nimble fingers, dances deftly to the rhythm of efficiency, weaving intricate patterns that enhance performance, ensuring that each note is delivered without delay, each interaction met with a swift response. Imagine, if you will, a user scrolling through an expansive collection of tracks, each click eliciting a quick and delightful reaction—a testament to the art of optimization.
As we delve into the world of large audio libraries, we encounter challenges that echo like the distant sound of a symphony tuning before the grand performance. The sheer volume of data, the multitude of audio files, and the intricate relationships between them create a complex web that demands careful management. Here, JavaScript steps forth as both conductor and composer, orchestrating a seamless experience that transcends the limitations of traditional approaches.
One of the first notes in this symphony of optimization is the art of lazy loading. Rather than overwhelming the user with an avalanche of data at the same time, JavaScript allows us to load audio files incrementally, freeing the user from the burden of long wait times. Picture a user browsing through a list of albums; as they scroll, only the tracks in view are fetched from the server, while those just beyond the horizon remain in the shadows, waiting for their moment to shine.
const loadTracks = (startIndex, endIndex) => { fetch(`/api/tracks?start=${startIndex}&end=${endIndex}`) .then(response => response.json()) .then(tracks => { displayTracks(tracks); }) .catch(error => console.error('Error loading tracks:', error)); }; // Event listener for scrolling window.addEventListener('scroll', () => { if (isNearBottom()) { loadTracks(currentTrackIndex, currentTrackIndex + 20); currentTrackIndex += 20; } });
In addition to lazy loading, caching strategies emerge as a vital instrument in this optimization ensemble. By storing frequently accessed audio files in memory, JavaScript ensures that subsequent requests are met with the swiftness of a maestro’s baton. This preloading acts as a reservoir of sound, ready to be summoned at a moment’s notice, creating an illusion of responsiveness that captivates the user’s senses.
const cache = new Map(); const fetchTrack = (trackId) => { if (cache.has(trackId)) { return Promise.resolve(cache.get(trackId)); } return fetch(`/api/track/${trackId}`) .then(response => response.json()) .then(track => { cache.set(trackId, track); return track; }) .catch(error => console.error('Error fetching track:', error)); };
As the user navigates through their auditory journey, the importance of minimizing data payloads cannot be overstated. JavaScript enables developers to compress audio files, ensuring that the size of each track does not become a cumbersome anchor weighing down the experience. Techniques such as transcoding audio to more efficient formats allow for rapid delivery without compromising sound quality, thus striking a delicate balance between fidelity and performance.
const compressAudio = (audioFile) => { // Hypothetical function to compress audio return compressToFormat(audioFile, 'mp3', { quality: 0.8 }); };
In this delicate choreography of data, the asynchronous nature of JavaScript plays a pivotal role. By employing promises and async functions, the loading of audio becomes a harmonious process, where the user interface remains fluid and responsive, even as data flows in and out of the application. Each interaction, whether it be a search or a playlist update, unfolds without disruption, creating a fluid experience akin to a flowing river.
async function loadAndPlayTrack(trackId) { try { const track = await fetchTrack(trackId); playTrack(track.buffer); } catch (error) { console.error('Error loading and playing track:', error); } }
As we navigate the labyrinth of audio libraries, the significance of user experience becomes increasingly pronounced. JavaScript, with its versatile toolkit, allows us to craft interfaces that not only engage the eyes but also delight the ears. By combining visual cues, such as loading indicators, with the auditory experience, developers can create a symphony of interaction that resonates deeply with users, transforming each click into a note in a grand composition of sound.
Thus, in the delicate balance of performance optimization, JavaScript emerges not merely as a programming language but as an eloquent facilitator of experiences where users can explore vast libraries of music with ease and joy. Each optimization, each line of code, contributes to a greater ensemble, creating a space where the beauty of sound and the artistry of technology intertwine, echoing in the hearts of listeners as they embark on their unique auditory journeys.