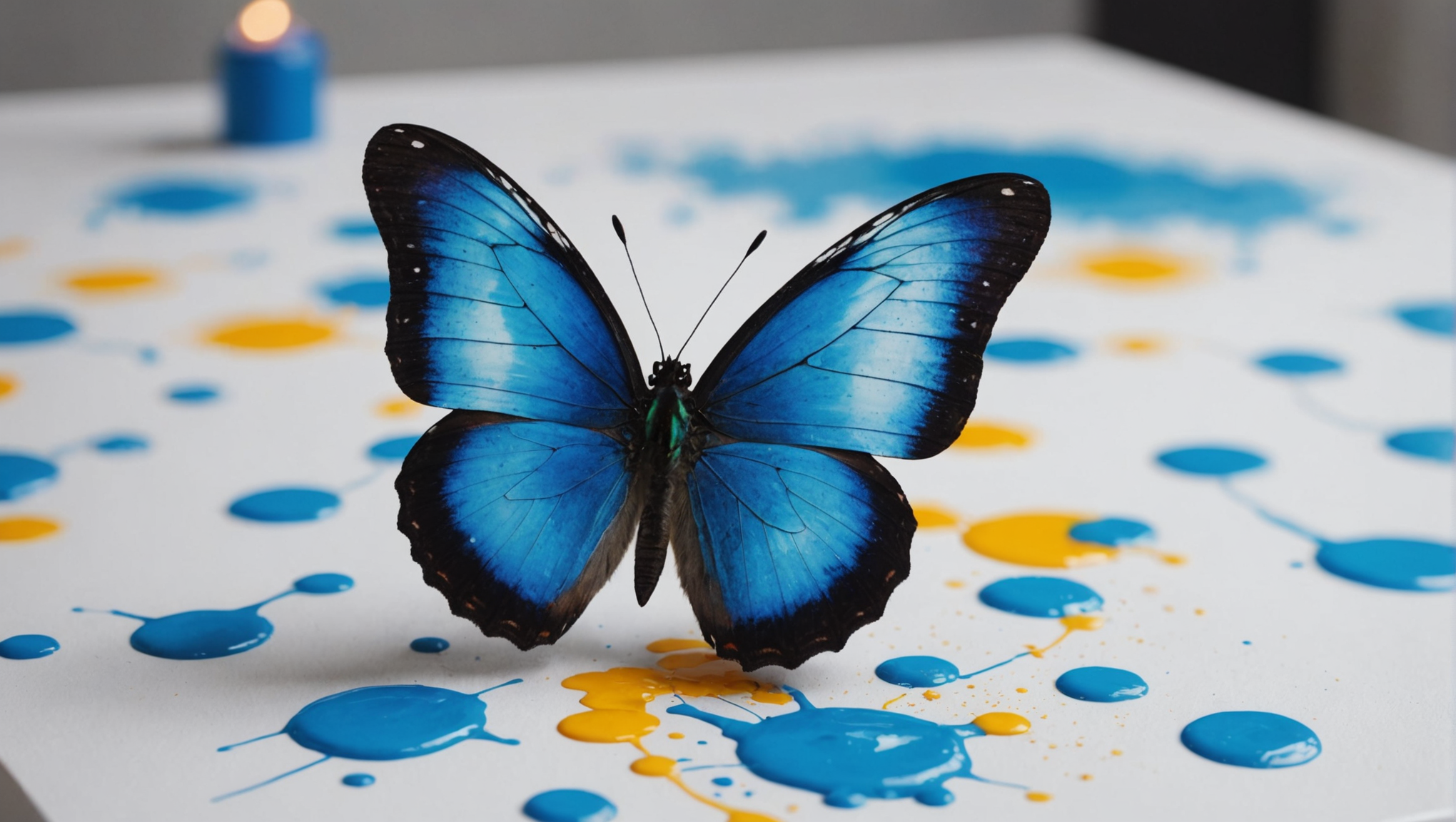
JavaScript for Photo Editing and Manipulation
The Canvas API is a powerful tool in HTML5 that allows developers to draw graphics via JavaScript. This capability is particularly useful for tasks such as photo editing and manipulation, where fine control over images is essential. Understanding the core principles of the Canvas API especially important for using its full potential.
At its heart, the Canvas API uses a canvas element in HTML. This element acts as a drawable region defined in HTML code with JavaScript. You can create complex images, animations, and interactive graphics directly in the browser.
The first step in using the Canvas API is to create a canvas element within your HTML. Here’s a simple example:
<canvas id="myCanvas" width="500" height="400"></canvas>
Once you have your canvas in place, you can access it via JavaScript using the getContext method. This method returns a drawing context on the canvas, which provides methods and properties for drawing shapes, text, images, and other objects.
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d');
In this code snippet, we obtain the 2D context for our canvas, which allows us to draw in a two-dimensional space. With this context, you can begin performing various operations. For example, to draw a filled rectangle, you can use the fillRect method:
ctx.fillStyle = 'blue'; // Specify the fill color ctx.fillRect(20, 20, 150, 100); // Draw a rectangle at (20, 20) with width 150 and height 100
The Canvas API also supports image manipulation, which is vital for photo editing. You can load images and draw them onto the canvas using the drawImage method. Here’s how you can do that:
const img = new Image(); img.src = 'path/to/image.jpg'; // Specify the image source img.onload = function() { ctx.drawImage(img, 0, 0, canvas.width, canvas.height); // Draw the image on the canvas };
Additionally, the Canvas API allows you to manipulate pixel data directly. This manipulation can be done through the getImageData and putImageData methods, enabling you to work at the pixel level for advanced effects and alterations.
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height); // Perform operations on imageData.data array here ctx.putImageData(imageData, 0, 0); // Draw the data back onto the canvas
By mastering these fundamental elements of the Canvas API, you lay the groundwork for creating intricate and dynamic photo editing applications. The combination of drawing methods, image handling capabilities, and pixel manipulation provides a robust toolkit for developing creative solutions in JavaScript.
Image Manipulation Techniques
Image manipulation techniques in JavaScript using the Canvas API extend beyond simple drawing operations. By using pixel manipulation, blending modes, and transformation techniques, developers can create sophisticated photo editing features. These techniques are essential for enhancing images, applying effects, and creating custom filters.
One of the most powerful aspects of the Canvas API is the ability to manipulate individual pixels. When you obtain image data from the canvas using the getImageData
method, you receive an object that contains a one-dimensional array of pixel data. Each pixel is represented by four consecutive values corresponding to the red, green, blue, and alpha (transparency) channels.
For example, to invert the colors of an image, you could access and modify the data
array like this:
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height); const data = imageData.data; for (let i = 0; i < data.length; i += 4) { data[i] = 255 - data[i]; // Red data[i + 1] = 255 - data[i + 1]; // Green data[i + 2] = 255 - data[i + 2]; // Blue // Alpha remains unchanged } ctx.putImageData(imageData, 0, 0);
In this code, we iterate through each pixel in the image data, inverting the color values. This type of manipulation provides the basis for various artistic effects.
Another common technique is applying blending modes, which can create visually striking results by combining the colors of overlapping images. The canvas context allows you to use the globalCompositeOperation
property to set the blending mode before drawing shapes or images. Here’s an example of using the “multiply” blending mode:
ctx.globalCompositeOperation = 'multiply'; ctx.drawImage(img1, 0, 0, canvas.width, canvas.height); // Draw the first image ctx.drawImage(img2, 0, 0, canvas.width, canvas.height); // Draw the second image with the blending effect
This technique allows you to blend two images, resulting in a combined effect that can enhance the overall composition.
Transformations are another powerful set of techniques at your disposal. Using methods like scale()
, rotate()
, and translate()
, you can manipulate images and shapes in various ways. Here’s how to rotate an image around its center:
ctx.save(); // Save the current state ctx.translate(canvas.width / 2, canvas.height / 2); // Move the origin to the center ctx.rotate(Math.PI / 4); // Rotate 45 degrees ctx.drawImage(img, -img.width / 2, -img.height / 2); // Draw the image centered ctx.restore(); // Restore the original state
In this example, we save the current drawing state, translate the origin for rotation, and draw the image after applying the rotation. Finally, we restore the state to continue drawing without the transformation.
Through these image manipulation techniques—pixel manipulation, blending modes, and transformations—you can create a wide array of effects and enhancements suitable for any photo editing application. The power of the Canvas API, combined with JavaScript’s flexibility, opens up a world of creative possibilities that can elevate your projects to new heights.
Applying Filters and Effects
Applying filters and effects is a quintessential aspect of photo editing that can dramatically alter the visual aesthetics of an image. The Canvas API provides a robust framework for implementing a variety of filters and effects through pixel manipulation and the use of the ImageData object. By understanding how to harness these capabilities, developers can create rich, engaging image editing experiences directly in the browser.
One of the most simpler ways to apply filters is by manipulating the pixel data directly. You can achieve effects such as grayscale, sepia, and blur by adjusting the pixel values. For instance, to convert an image to grayscale, you can use the following code snippet:
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height); const data = imageData.data; for (let i = 0; i < data.length; i += 4) { const avg = (data[i] + data[i + 1] + data[i + 2]) / 3; // Average of red, green, blue data[i] = avg; // Red data[i + 1] = avg; // Green data[i + 2] = avg; // Blue // Alpha remains unchanged } ctx.putImageData(imageData, 0, 0);
This code takes the average of the red, green, and blue components for each pixel, resulting in a grayscale effect. Grayscale is a foundational filter, and understanding its implementation sets the stage for more complex effects.
Another popular filter is the sepia effect, which adds a warm tone to images, reminiscent of old photographs. Here’s how to apply a sepia filter using the same pixel manipulation technique:
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height); const data = imageData.data; for (let i = 0; i < data.length; i += 4) { const r = data[i]; const g = data[i + 1]; const b = data[i + 2]; data[i] = r * 0.393 + g * 0.769 + b * 0.189; // Red data[i + 1] = r * 0.349 + g * 0.686 + b * 0.168; // Green data[i + 2] = r * 0.272 + g * 0.534 + b * 0.131; // Blue // Alpha remains unchanged } ctx.putImageData(imageData, 0, 0);
In this example, we apply a color transformation based on the sepia algorithm, giving the image that nostalgic warmth. The manipulation of pixel values showcases the flexibility of the Canvas API for creating unique artistic effects.
Blurring is another common effect, usually accomplished through a convolution filter. By averaging pixel values in a defined area, you can create a softening effect. Here’s a simplified implementation of a basic box blur:
function boxBlur(imageData, width, height) { const data = imageData.data; const result = ctx.createImageData(width, height); const resultData = result.data; for (let y = 1; y < height - 1; y++) { for (let x = 1; x < width - 1; x++) { const idx = (y * width + x) * 4; const r = (data[idx - 4] + data[idx + 4] + data[idx - width * 4] + data[idx + width * 4]) / 4; const g = (data[idx - 3] + data[idx + 3] + data[idx - width * 4 + 1] + data[idx + width * 4 + 1]) / 4; const b = (data[idx - 2] + data[idx + 2] + data[idx - width * 4 + 2] + data[idx + width * 4 + 2]) / 4; resultData[idx] = r; resultData[idx + 1] = g; resultData[idx + 2] = b; resultData[idx + 3] = 255; // Full opacity } } return result; } const blurredImageData = boxBlur(imageData, canvas.width, canvas.height); ctx.putImageData(blurredImageData, 0, 0);
This box blur function creates a simple but effective blurring effect by averaging the color values of neighboring pixels. Although more advanced techniques exist, this example shows how the Canvas API can be used to implement basic filters efficiently.
Finally, the saturation effect is another intriguing filter that can be easily applied. You can increase or decrease the saturation by manipulating the pixel values accordingly. The following demonstrates saturating an image:
const imageData = ctx.getImageData(0, 0, canvas.width, canvas.height); const data = imageData.data; for (let i = 0; i < data.length; i += 4) { const avg = (data[i] + data[i + 1] + data[i + 2]) / 3; // Increase saturation by moving colors away from the average data[i] += (data[i] - avg) * 1.5; // Red data[i + 1] += (data[i + 1] - avg) * 1.5; // Green data[i + 2] += (data[i + 2] - avg) * 1.5; // Blue } ctx.putImageData(imageData, 0, 0);
In this example, we amplify the difference between each color channel and the average color, effectively increasing saturation. Adjusting the multiplier provides control over the intensity of the effect.
By using these techniques—grayscale, sepia, blurring, and saturation—you can effectively apply filters and effects to images on the canvas. The Canvas API’s ability to manipulate pixel data allows for a wide range of creative possibilities, enabling developers to craft sophisticated photo editing applications that can rival professional software directly in the browser.
Creating Custom Drawing Tools
Creating custom drawing tools is an exciting extension of the capabilities provided by the Canvas API. This section delves into developing drawing tools that allow users to create and manipulate graphics directly on the canvas. By providing intuitive controls and features, users can engage in artistic expression, resulting in unique creations.
To begin, we must set up event listeners to capture mouse movements and clicks. This enables us to track when the user is drawing on the canvas. Below is a basic implementation that allows users to draw freehand lines on the canvas:
const canvas = document.getElementById('myCanvas'); const ctx = canvas.getContext('2d'); let drawing = false; // Start drawing canvas.addEventListener('mousedown', (e) => { drawing = true; ctx.beginPath(); // Start a new path ctx.moveTo(e.offsetX, e.offsetY); // Move to the mouse position }); // Draw on the canvas canvas.addEventListener('mousemove', (e) => { if (drawing) { ctx.lineTo(e.offsetX, e.offsetY); // Draw a line to the current mouse position ctx.stroke(); // Render the path } }); // Stop drawing canvas.addEventListener('mouseup', () => { drawing = false; ctx.closePath(); // Close the path });
In this code, we initialize a drawing state and listen for mouse events. When the user presses down the mouse button, we start a new path at the current position. As the mouse moves, we draw lines connecting the points, and we stop drawing when the mouse button is released.
To enhance the user experience, we can add features such as color selection and line width adjustments. This allows users to customize their drawing tools to fit their creative needs. Here’s how we can implement these features:
const colorPicker = document.getElementById('colorPicker'); const lineWidthInput = document.getElementById('lineWidth'); colorPicker.addEventListener('change', (e) => { ctx.strokeStyle = e.target.value; // Update stroke color }); lineWidthInput.addEventListener('change', (e) => { ctx.lineWidth = e.target.value; // Update line width });
In this example, we assume there’s an HTML color input and a range input for line width. By listening to changes in these inputs, we update the drawing context’s stroke style and line width dynamically. This flexibility empowers users to express their creativity more freely.
Next, we can introduce additional features such as erasing and shapes. For instance, to implement an eraser tool, we can change the stroke style to match the canvas background color:
const eraserButton = document.getElementById('eraserButton'); eraserButton.addEventListener('click', () => { ctx.strokeStyle = '#FFFFFF'; // Assuming white is the canvas background ctx.lineWidth = 10; // Set a thicker line for erasing });
With this code, once the user clicks the eraser button, it alters the stroke color to white, effectively allowing the user to “erase” their drawings. The line width can also be adjusted for more precise erasing.
Creating shapes can also be a fun addition. Users might want to draw rectangles, circles, or other predefined shapes. Here’s a simple implementation of drawing rectangles:
let startX, startY; canvas.addEventListener('mousedown', (e) => { drawing = true; startX = e.offsetX; startY = e.offsetY; }); canvas.addEventListener('mousemove', (e) => { if (drawing) { const width = e.offsetX - startX; const height = e.offsetY - startY; ctx.clearRect(0, 0, canvas.width, canvas.height); // Clear to redraw ctx.strokeRect(startX, startY, width, height); // Draw rectangle } }); canvas.addEventListener('mouseup', () => { drawing = false; });
In this snippet, we record the starting coordinates when the mouse button is pressed. As the mouse moves, we calculate the width and height of the rectangle and redraw it. This method provides immediate feedback, enhancing the user’s drawing experience.
By building upon these foundational tools, you can expand your canvas application into a versatile drawing program. Custom drawing tools, equipped with options for different colors, line widths, erasing, and shape drawing, provide a framework for users to explore their creativity. The Canvas API, combined with JavaScript’s flexibility, allows developers to create engaging and interactive applications that delight users and elevate their creative endeavors.
Integrating External Libraries for Advanced Editing
Integrating external libraries can significantly enhance the capabilities of your photo editing application, providing advanced features that simplify complex tasks or enable functionalities beyond the native capabilities of the Canvas API. Libraries often deliver a wealth of pre-built functions, making it easier to implement features like filters, transformations, and even user interface components.
One of the most popular libraries for image manipulation in JavaScript is Fabric.js. This framework simplifies working with the Canvas API by providing an object-oriented approach to drawing and manipulating images. With Fabric.js, you can easily create, group, and manipulate objects on the canvas. Here’s a quick example of how to set it up:
const canvas = new fabric.Canvas('myCanvas'); // Add an image to the canvas fabric.Image.fromURL('path/to/image.jpg', function(img) { img.scale(0.5); // Scale the image canvas.add(img); // Add image to the Fabric canvas });
In this snippet, we initialize a Fabric.js canvas, load an image, and then scale and add it to the canvas. Fabric.js provides methods for easy handling of images, shapes, and text, enabling seamless integration of complex features.
Another powerful library worth exploring is PixiJS, which is particularly well-suited for creating interactive graphics. While it’s primarily used for WebGL rendering, PixiJS can perform 2D image manipulation with impressive performance. Below is an example of how to use PixiJS to manipulate an image:
const app = new PIXI.Application({ width: 800, height: 600 }); document.body.appendChild(app.view); PIXI.Loader.shared.add('image', 'path/to/image.jpg').load((loader, resources) => { const sprite = new PIXI.Sprite(resources.image.texture); sprite.scale.set(0.5, 0.5); // Scale the image app.stage.addChild(sprite); // Add the sprite to the stage });
In this example, we create a new PixiJS application, load an image, and then add it as a sprite to the application’s stage. PixiJS not only handles image manipulation but also optimizes rendering, making it an excellent choice for applications requiring high-performance graphics.
Additionally, you might ponder libraries like p5.js for creative coding, which abstracts many complexities of the Canvas API while providing powerful functions for image manipulation and drawing. For instance, p5.js simplifies drawing and applying effects:
function setup() { createCanvas(500, 400); loadImage('path/to/image.jpg', img => { image(img, 0, 0); // Display image filter(GRAY); // Apply grayscale filter }); }
In the p5.js example, we load an image and apply a grayscale filter with a simple function call. Libraries like p5.js focus on making creative coding accessible, encouraging experimentation with images and animations.
Lastly, integrating user interface libraries, such as React or Vue.js, can allow you to build a robust front end for your photo editing application. These frameworks facilitate the creation of dynamic, stateful components that can respond to user interactions, enabling a more seamless editing experience. When combined with the Canvas API, these frameworks serve as the backbone for delivering a rich user interface.
Using external libraries can significantly enhance your photo editing application. By providing advanced functionalities, performance optimizations, and easier integrations, libraries like Fabric.js, PixiJS, and p5.js empower developers to create sophisticated and interactive applications efficiently. Using these tools allows you to focus on the creative aspects of your application, transforming ideas into a vibrant digital reality.
Optimizing Performance for Real-Time Editing
Optimizing performance for real-time editing in a photo editing application especially important for ensuring a smooth and responsive user experience. The Canvas API provides a powerful foundation, but achieving real-time performance requires strategic approaches to resource management, rendering techniques, and event handling. By implementing these techniques, developers can significantly enhance the usability and effectiveness of their applications.
One primary strategy is to minimize the number of times the canvas is redrawn. When performing complex operations or effects, it’s essential to avoid unnecessary rendering. Instead of redrawing the entire canvas on every change, consider using techniques like dirty rectangles, where only the areas that need updating are redrawn. This can drastically reduce the workload on the rendering engine.
// Pseudo-code for dirty rectangles optimization function update() { const dirtyRect = getDirtyRectangle(); // Calculate the area that needs updating ctx.clearRect(dirtyRect.x, dirtyRect.y, dirtyRect.width, dirtyRect.height); // Clear only the dirty rectangle ctx.drawImage(yourImage, 0, 0); // Redraw the image or affected elements }
Another effective technique is to leverage off-screen canvases. By using an additional canvas as a buffer, you can perform transformations and filters on the off-screen canvas first. Once the processing is complete, the final result can be drawn onto the visible canvas in one go. This approach minimizes the flickering and performance hits associated with rendering multiple operations directly onto the visible canvas.
// Off-screen canvas example const offScreenCanvas = document.createElement('canvas'); const offScreenCtx = offScreenCanvas.getContext('2d'); function applyFilters() { offScreenCtx.drawImage(originalImage, 0, 0); // Draw original image to off-screen canvas // Apply filter operations const imageData = offScreenCtx.getImageData(0, 0, offScreenCanvas.width, offScreenCanvas.height); // Manipulate pixels offScreenCtx.putImageData(imageData, 0, 0); // Draw back to off-screen canvas ctx.drawImage(offScreenCanvas, 0, 0); // Finally draw to visible canvas }
Moreover, consider throttling or debouncing event handlers when responding to user inputs like mouse movements or touch events. Instead of processing every single event, which can lead to performance bottlenecks in real-time applications, you can limit how often your handler is called. That is especially important when users are painting or dragging to edit images.
// Throttling function example function throttle(fn, wait) { let lastTime = 0; return function(...args) { const now = Date.now(); if (now - lastTime >= wait) { lastTime = now; fn.apply(this, args); } }; } // Using the throttled function on mousemove canvas.addEventListener('mousemove', throttle((e) => { drawAt(e.offsetX, e.offsetY); }, 100)); // Adjust wait time as needed
Memory management is another critical aspect that influences performance. When working with images and pixel data, ensure that you release any resources that are no longer needed. For instance, if you’re dynamically creating images or canvases, remove references to them when they are no longer in use, allowing the garbage collector to reclaim memory.
Lastly, ponder using Web Workers to offload heavy computations from the main thread. Image processing can be resource-intensive, and by moving these tasks to a Web Worker, you can keep the main UI thread responsive. This approach allows for complex calculations to occur without interrupting the user interface, enhancing the overall performance of your application.
// Example of using Web Workers const worker = new Worker('imageProcessor.js'); worker.onmessage = function(e) { const processedImageData = e.data; ctx.putImageData(processedImageData, 0, 0); // Draw processed data back to canvas }; // Post data to worker for processing worker.postMessage(originalImageData);
By implementing these performance optimization techniques—minimizing redraws, using off-screen canvases, throttling event handlers, managing memory effectively, and using Web Workers—you can enhance the efficiency of your photo editing application. This ensures that users enjoy a fluid, responsive experience even when applying complex effects or editing high-resolution images. The Canvas API, combined with these strategies, empowers developers to create sophisticated and performant photo editing tools that cater to the needs of modern users.