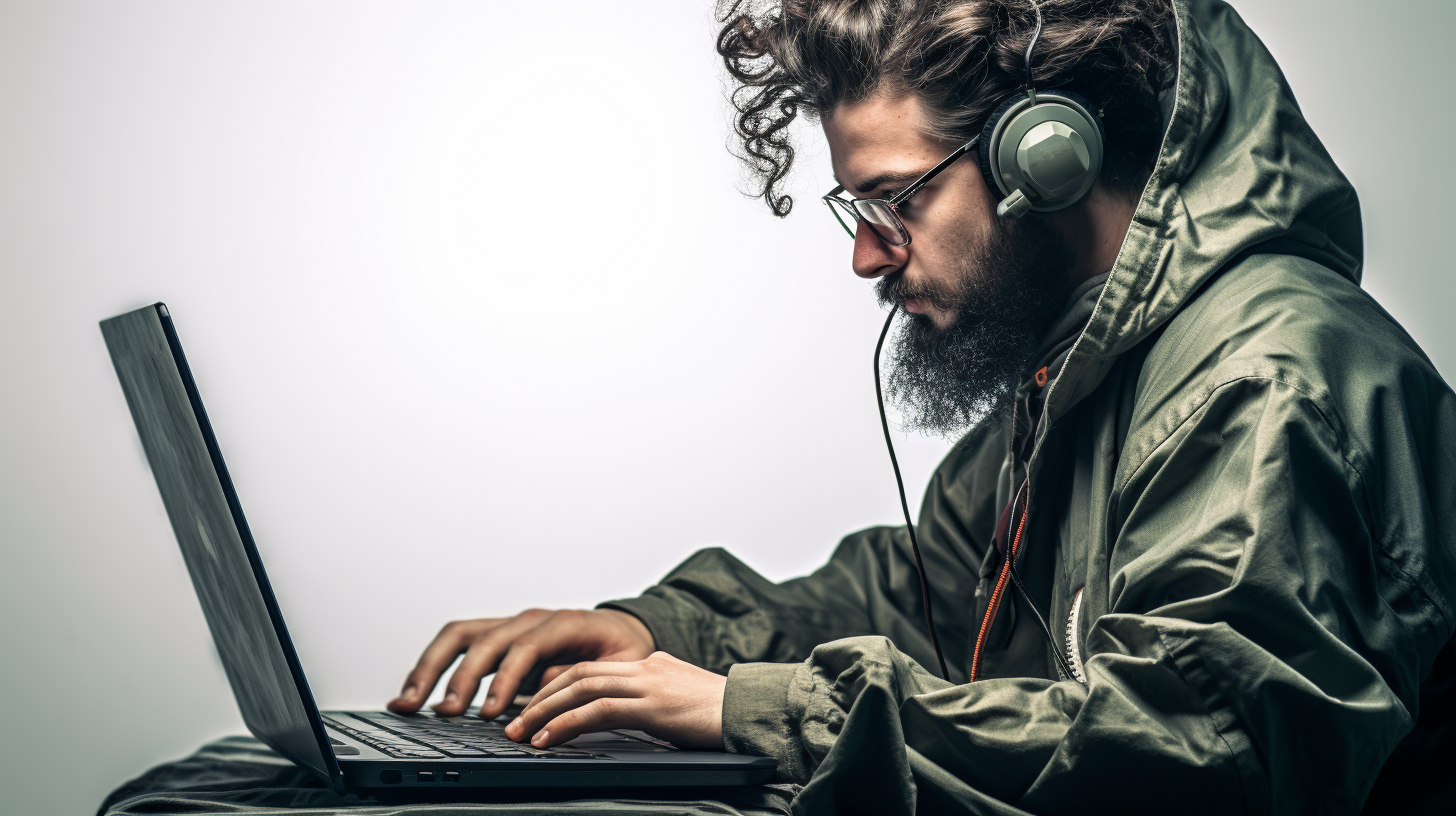
JavaScript for Project Management Tools
When constructing project management tools, using key JavaScript features can greatly enhance functionality and user experience. Understanding these features allows developers to craft applications that are not only robust but also responsive to user demands.
Asynchronous Programming is a vital aspect of JavaScript that enables non-blocking operations. That is particularly useful when handling multiple tasks such as fetching data from a server or managing user interactions simultaneously. Using Promises
and async/await
syntax can simplify the handling of asynchronous code, making it cleaner and more manageable.
async function fetchProjectData() { try { const response = await fetch('https://api.example.com/projects'); const data = await response.json(); console.log(data); } catch (error) { console.error('Error fetching project data:', error); } }
Dynamic DOM Manipulation is another critical feature that enhances interactivity. Using the Document Object Model (DOM), developers can create, delete, and modify elements on the fly, which is essential for applications that require real-time updates, such as task lists and status boards.
function addTask(task) { const taskList = document.getElementById('task-list'); const listItem = document.createElement('li'); listItem.textContent = task; taskList.appendChild(listItem); }
Event Handling in JavaScript allows applications to respond promptly to user actions, such as clicks or keyboard inputs. Defining custom event listeners can enable developers to build interactive and uncomplicated to manage interfaces, ensuring that Project Management Tools feel responsive and intuitive.
document.getElementById('submit-button').addEventListener('click', function() { const taskInput = document.getElementById('task-input'); addTask(taskInput.value); taskInput.value = ''; });
Data Storage capabilities through local storage or session storage provide a way to persist user data without needing a server-side database. This can be instrumental for tasks that require saving user preferences or session details, especially for applications that are expected to function offline or have varying connectivity.
function saveTask(task) { let tasks = JSON.parse(localStorage.getItem('tasks')) || []; tasks.push(task); localStorage.setItem('tasks', JSON.stringify(tasks)); }
Furthermore, using Modular Programming through ES6 modules can enhance the organization and maintainability of the project. This approach allows developers to compartmentalize code into reusable components, reducing redundancy and improving clarity when revisiting or refactoring code.
// task.js export function createTask(title) { return { title, completed: false }; } // main.js import { createTask } from './task.js'; const newTask = createTask('Learn JavaScript features');
Using these key JavaScript features not only streamlines the development of project management tools but also results in applications that deliver an exceptional user experience. By embracing asynchronous programming, dynamic DOM manipulation, efficient event handling, persistent data storage, and modular programming, developers can create powerful tools tailored to the intricate needs of project management.
Integrating APIs for Enhanced Project Functionality
Integrating APIs into project management tools can significantly enhance functionality and user experience. APIs (Application Programming Interfaces) allow developers to connect their applications with external services, enabling access to a wealth of data and features that can elevate the capabilities of the tool. By effectively using APIs, developers can implement functionalities such as task management, real-time updates, and data visualizations without having to build everything from scratch.
One common use of APIs in project management applications is to fetch project-related data from a backend service. By making asynchronous requests to the API, developers can retrieve real-time information, keeping users up-to-date with the latest changes. Here’s a simple example of how to fetch the details of a specific project using the Fetch API:
async function fetchProjectDetails(projectId) { try { const response = await fetch(`https://api.example.com/projects/${projectId}`); const project = await response.json(); displayProjectDetails(project); } catch (error) { console.error('Error fetching project details:', error); } }
Once the project data is fetched, it can be displayed on the user interface by dynamically manipulating the DOM. This not only keeps the information current but also enhances the overall user experience by making the interface responsive to the latest data.
Another powerful aspect of integrating APIs is the ability to interact with third-party services, such as task management, communication, or time tracking tools. For instance, integrating with a service like Slack can allow project managers to send notifications and updates directly to team members. This can be achieved with a simple POST request to the Slack API:
async function sendSlackNotification(message) { try { const response = await fetch('https://slack.com/api/chat.postMessage', { method: 'POST', headers: { 'Content-Type': 'application/json', 'Authorization': `Bearer ${SLACK_BOT_TOKEN}` }, body: JSON.stringify({ channel: '#project-updates', text: message }) }); const result = await response.json(); if (!result.ok) { throw new Error('Error sending message to Slack'); } } catch (error) { console.error('Error sending Slack notification:', error); } }
Additionally, APIs can provide access to analytics and reporting features that are crucial for project management. By integrating with analytics platforms, developers can fetch usage statistics or project performance metrics, allowing project managers to make informed decisions based on data-driven insights.
To effectively manage API responses and errors, implementing robust error handling is essential. This ensures that even if an API call fails, the application can gracefully handle the situation, providing feedback to users and maintaining functionality. For example, when fetching data, it is important to check for both network errors and application-level errors:
async function fetchDataWithErrorHandling(url) { try { const response = await fetch(url); if (!response.ok) { throw new Error(`HTTP error! status: ${response.status}`); } const data = await response.json(); return data; } catch (error) { console.error('Fetch error:', error); alert('An error occurred while fetching data. Please try again later.'); } }
Integrating APIs into project management tools opens up a high number of possibilities for enhancing functionality and user engagement. By using external services, developers can provide richer features, streamline workflows, and create a more collaborative environment for teams. The key lies in understanding the available APIs, effectively managing asynchronous operations, and implementing robust error handling to ensure a seamless user experience.
Building Real-Time Collaboration Tools with JavaScript
In the context of project management, building real-time collaboration tools is paramount. JavaScript offers a suite of capabilities that facilitate the creation of applications where multiple users can interact at the same time, making the experience seamless and dynamic. One of the cornerstones of real-time collaboration is the use of WebSockets, which enable bi-directional communication between the client and server. This allows for instantaneous updates and data sharing without the need for constant polling.
To establish a WebSocket connection, you typically start with the following code:
const socket = new WebSocket('wss://example.com/socket'); socket.onopen = function() { console.log('WebSocket connection established'); }; socket.onmessage = function(event) { const data = JSON.parse(event.data); updateUI(data); }; socket.onerror = function(error) { console.error('WebSocket error:', error); }; socket.onclose = function() { console.log('WebSocket connection closed'); };
Once connected, the application can send and receive messages in real-time. For instance, when a user adds a new task, the application can broadcast this task to all connected clients:
function addTask(task) { const taskData = JSON.stringify({ action: 'add', task }); socket.send(taskData); updateUI(task); }
When a task is added, every user connected to the WebSocket will receive this update, ensuring that all task lists are synchronized. The updateUI
function would be responsible for rendering the new task in the DOM, maintaining a coherent state across all users’ interfaces.
Moreover, using real-time databases like Firebase can further simplify the implementation of collaboration tools. Firebase provides a simpler API for integrating real-time data synchronization, allowing developers to focus on building features rather than worrying about the complexities of backend infrastructure.
const db = firebase.database(); function listenForTasks() { db.ref('tasks').on('value', (snapshot) => { const tasks = snapshot.val(); renderTasks(tasks); }); }
The listenForTasks
function subscribes to changes in the tasks node. Whenever a task is added, modified, or deleted, Firebase automatically sends the updated list to all clients listening on that node. This approach ensures that all users see the same view without having to refresh or fetch data manually.
In addition to task management, real-time collaboration tools can include features such as live chat, document editing, or shared whiteboards. Adding a chat feature can be as simple as sending and receiving messages through the established WebSocket connection:
function sendMessage(msg) { const messageData = JSON.stringify({ action: 'chat', message: msg }); socket.send(messageData); } socket.onmessage = function(event) { const data = JSON.parse(event.data); if (data.action === 'chat') { displayChatMessage(data.message); } };
Here, the application not only handles task updates but also integrates a chat functionality, enhancing the collaborative experience. By sending a structured message, users can engage in discussions regarding tasks, streamlining communication within the project management tool.
Real-time collaboration requires a robust architecture that can handle multiple connections and data integrity. Implementing optimistic UI updates, where the user interface reflects changes immediately while awaiting confirmation from the server, can significantly enhance the user experience. This technique gives users the impression of instantaneous feedback, keeping them engaged and productive.
Building real-time collaboration tools with JavaScript is not just about connecting users; it’s about creating an interactive, fluid environment that fosters teamwork. By using WebSockets, real-time databases, and effective UI practices, developers can craft project management applications that transform how teams collaborate and manage their workflows.
Using JavaScript Libraries for Improved User Experience
Using various JavaScript libraries can significantly improve the user experience in project management tools by providing pre-built components, enhancing interactivity, and simplifying complex tasks. Libraries such as jQuery, React, and D3.js offer developers the ability to create more dynamic and responsive applications, allowing them to focus on core functionalities rather than reinventing the wheel.
jQuery, despite being older than many state-of-the-art libraries, remains a powerful tool for DOM manipulation and event handling. It simplifies tasks such as animations, AJAX requests, and cross-browser compatibility. For instance, using jQuery to create a smooth user experience when adding tasks or updating project statuses can be achieved with minimal code:
$('#add-task-button').click(function() { const task = $('#task-input').val(); $('#task-list').append(`
In this snippet, jQuery is used to handle the click event on the “Add Task” button, retrieving the task input and appending it to the task list with ease, showcasing how the library can enhance user interactions without cumbersome syntax.
React, on the other hand, is a library designed for building user interfaces, particularly suited for applications that require a dynamic and state-driven approach. By using React, developers can create reusable components that manage their own state, streamlining the development process and making the application more maintainable. Here’s a simple example demonstrating a task list component:
import React, { useState } from 'react'; function TaskList() { const [tasks, setTasks] = useState([]); const addTask = (task) => { setTasks([...tasks, task]); }; return (); }{tasks.map((task, index) =>
- {task}
)}
This React component maintains its own state for tasks and dynamically updates the UI as tasks are added. The use of JSX allows for a clean and descriptive way to define the UI structure, making it easier to visualize the component’s functionality.
When it comes to data visualization, D3.js is a powerhouse for creating dynamic and interactive data-driven graphics. In project management, visualizing project progress and team performance can be incredibly beneficial. Here’s a basic example of using D3.js to create a simple bar chart representing task completion:
const data = [ { task: 'Task 1', progress: 75 }, { task: 'Task 2', progress: 50 }, { task: 'Task 3', progress: 100 } ]; const svg = d3.select('svg'); const barHeight = 20; svg.selectAll('rect') .data(data) .enter() .append('rect') .attr('width', d => d.progress) .attr('height', barHeight) .attr('y', (d, i) => i * (barHeight + 5));
This snippet selects an SVG element and appends rectangles for each task’s progress, creating a visual representation of completion rates. Such visualizations can help teams quickly assess project status at a glance.
In addition to these libraries, using frameworks like Bootstrap for styling can enhance the overall aesthetic and usability of the application. By implementing responsive grids and components, developers can ensure that the project management tool is accessible across devices, improving the user experience for teams on-the-go.
By integrating these JavaScript libraries into project management tools, developers can create applications that are not only functional but also visually appealing and easy to use. The combined power of jQuery, React, D3.js, and CSS frameworks can turn a basic tool into a sophisticated platform that meets the nuanced needs of project management.