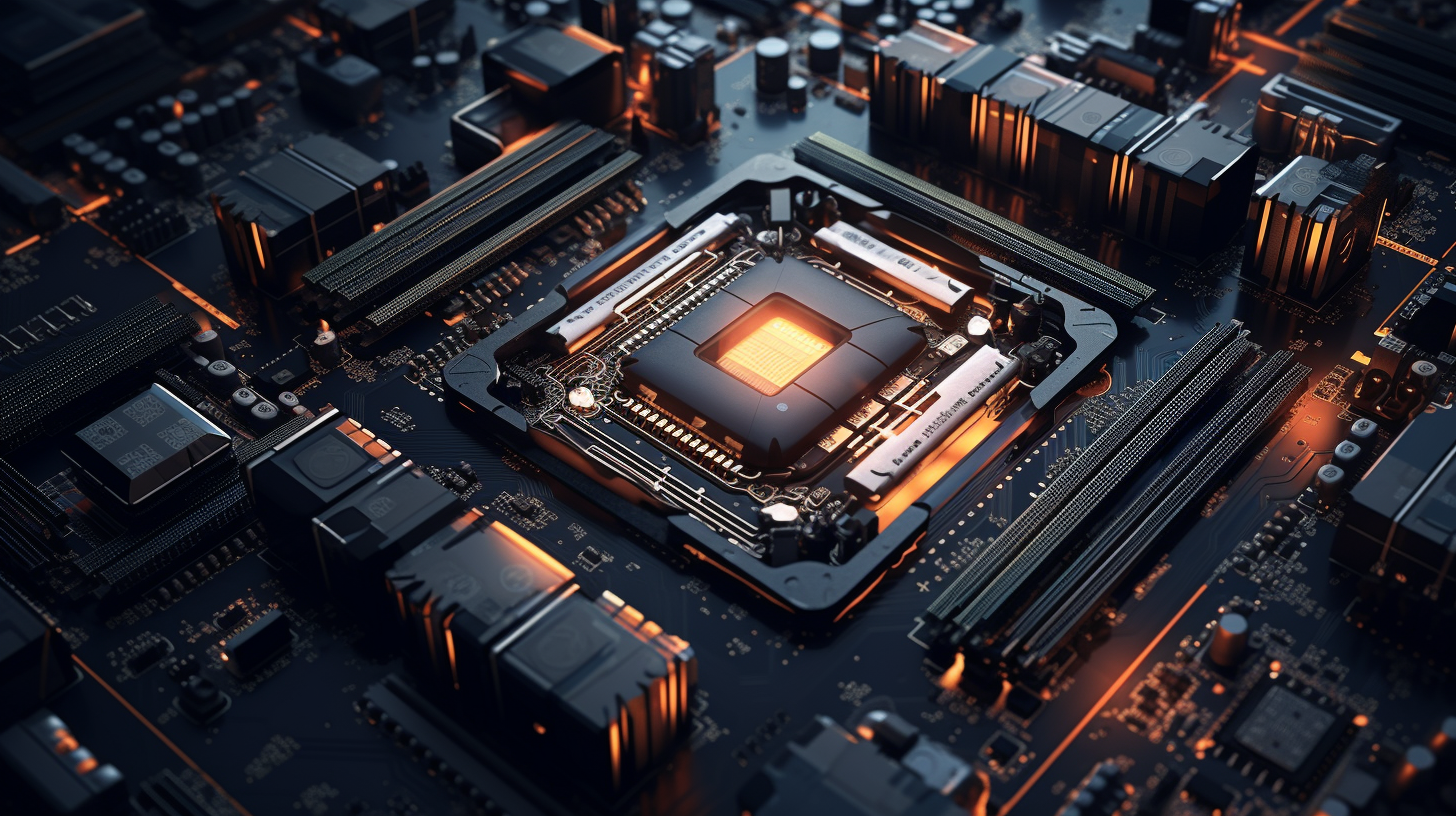
JavaScript for SEO Optimization
JavaScript has become an integral part of contemporary web development, but its role in SEO is often misunderstood. Search engines like Google have made significant strides in crawling and indexing JavaScript-heavy websites, yet there are nuances that developers must grasp to ensure optimal visibility in search results.
At its core, JavaScript enables dynamic content rendering, which enhances user experience by creating interactive elements. However, search engines primarily rely on HTML to index content. This means that if JavaScript is used to generate content after the initial page load, it may not be picked up by crawlers unless certain practices are followed.
A pivotal aspect of understanding JavaScript’s role in SEO is recognizing how search engines execute scripts. Googlebot, for example, can render JavaScript, but it does so in a two-step process: first, it fetches the HTML, and then it executes the JavaScript. This could lead to a delay in indexing or even missed content if it is conditionally displayed or loaded asynchronously.
To better demonstrate how JavaScript affects SEO, think the following example where we dynamically load content based on user interaction:
document.getElementById('loadMore').addEventListener('click', function() { fetch('/load-more-content') .then(response => response.text()) .then(html => { document.getElementById('content').innerHTML += html; }); });
In this code snippet, new content is added only when the user clicks a button. While this enhances user engagement, the dynamically loaded content may not be indexed unless specific strategies are employed, such as implementing server-side rendering (SSR) or ensuring that the content is available in the initial HTML response.
Another consideration is the use of progressive enhancement and graceful degradation. By starting with a basic HTML structure this is fully crawlable, you can layer on JavaScript functionality without sacrificing accessibility or SEO. This method not only ensures that your content is indexed but also provides a fallback for users with JavaScript disabled.
Ultimately, understanding how JavaScript interacts with search engine algorithms especially important for developers who want to leverage its capabilities without compromising their site’s search visibility. By adhering to best practices and being mindful of how content is presented, it is possible to harness the power of JavaScript while still optimizing for search engines effectively.
Best Practices for SEO-Friendly JavaScript
To further enhance the SEO-friendliness of your JavaScript, consider implementing several best practices that align with both user experience and search engine requirements. One of the most effective strategies is to utilize server-side rendering (SSR). By generating HTML content on the server before it’s sent to the client, you ensure that search engines can easily crawl and index your content without waiting for JavaScript execution. This approach can significantly improve the visibility of your site in search results.
Here’s a simplified example of how you might implement server-side rendering with Node.js and Express:
const express = require('express'); const app = express(); app.get('/', (req, res) => { // Simulating data fetching const pageTitle = 'My SEO-Friendly Page'; const content = 'This is content rendered on the server.
'; // Send a fully rendered HTML response res.send(`${pageTitle} ${content} `); }); app.listen(3000, () => { console.log('Server is running on http://localhost:3000'); });
In addition to SSR, consider using the History API to manage URL states without full page reloads. This allows you to create a seamless user experience while ensuring that each state is properly indexed. By using the History API, you can push new states with meaningful URLs that search engines can crawl effectively.
document.getElementById('loadMore').addEventListener('click', function() { fetch('/load-more-content') .then(response => response.text()) .then(html => { document.getElementById('content').innerHTML += html; // Update the URL without reloading the page history.pushState({ page: "load-more" }, "Load More", "/load-more"); }); });
Moreover, it’s crucial to ensure that your JavaScript does not create unnecessary redirects or errors, which can be detrimental to SEO. Validate your JavaScript to prevent broken links, incorrect paths, or malformed URLs. Regularly testing with tools like Google’s Mobile-Friendly Test or the URL Inspection Tool can help identify issues early on.
Another impactful practice is to use structured data. Implementing schema markup within your JavaScript can enhance the way search engines interpret your content. By providing additional context about your pages, structured data can lead to rich snippets in search results, improving click-through rates.
const structuredData = { "@context": "https://schema.org", "@type": "Article", "headline": "Best Practices for SEO-Friendly JavaScript", "datePublished": "2023-10-01", "author": { "@type": "Person", "name": "Your Name" } }; const script = document.createElement('script'); script.type = 'application/ld+json'; script.text = JSON.stringify(structuredData); document.head.appendChild(script);
Finally, always ensure to keep performance in mind. Minifying your JavaScript files and using tools like lazy loading for images and videos can lead to faster page load times, which is a critical ranking factor for SEO. Regularly audit your site’s performance using tools such as Google Lighthouse to identify and rectify any issues.
Optimizing Page Load Speed with JavaScript
When it comes to page load speed, JavaScript can be both a powerful ally and a potential hurdle. To harness its benefits while minimizing its drawbacks, developers must adopt strategies that contribute to faster load times without compromising functionality or user experience.
One effective approach is to defer or asynchronously load non-essential JavaScript files. By doing so, you instruct the browser to load essential resources first, enhancing the perceived performance of the website. This technique allows the main content to render quickly, giving users access to information without delays. Here’s how you can implement this:
In this example, the “defer” attribute ensures that the script will only execute after the document has been fully parsed, preventing it from blocking the rendering of the page.
In addition to deferring scripts, consider optimizing your JavaScript by implementing code splitting. This strategy involves breaking up your JavaScript into smaller, manageable chunks that can be loaded on demand. This not only reduces the initial loading time but also ensures that users are only downloading the code necessary for their current interaction. Here’s a basic illustration using dynamic imports:
document.getElementById('loadFeature').addEventListener('click', function() { import('./feature.js') .then(module => { module.init(); // Initialize the feature }); });
By using dynamic imports, the feature is only loaded when the user interacts with the interface, improving the load speed for users who may not need that functionality immediately.
Another consideration is to optimize your JavaScript performance through efficient coding practices. Minimize the use of global variables and avoid excessive DOM manipulations, which can slow down rendering. Instead, batch your DOM updates to reduce reflows and repaints:
const list = document.getElementById('myList'); const items = ['Item 1', 'Item 2', 'Item 3']; const fragment = document.createDocumentFragment(); items.forEach(item => { const li = document.createElement('li'); li.textContent = item; fragment.appendChild(li); }); list.appendChild(fragment);
This approach appends multiple items to the list in one go, minimizing the performance hit from frequent updates to the DOM.
Using a content delivery network (CDN) for hosting your JavaScript files can further boost performance. CDNs deliver content from servers that are geographically closer to the user, reducing latency and speeding up load times. When combined with strategies like compression and caching, the impact on page load speed can be dramatically improved.
Finally, be mindful of the impact of third-party libraries and plugins. While they can provide useful functionality, they also contribute to load times. Analyze the necessity of each library and think alternatives that are lighter and more efficient. If you must use them, load them asynchronously to mitigate their effect on the initial rendering of your page:
const script = document.createElement('script'); script.src = 'https://example.com/library.js'; script.async = true; document.head.appendChild(script);
By carefully managing the loading and execution of JavaScript, developers can significantly enhance page load speeds, leading to better user experiences and improved SEO outcomes. Ultimately, the goal is to strike a balance between interactivity and performance, ensuring that JavaScript serves as a tool for enhancement rather than a barrier to efficiency.
Common JavaScript SEO Pitfalls to Avoid
While JavaScript can enhance your website’s interactivity, it can also introduce several pitfalls that may negatively impact your SEO efforts. Identifying and avoiding these common traps is essential for maintaining the visibility of your site in search engine results.
One of the most significant issues arises with the improper handling of content loading. As previously mentioned, if your JavaScript is responsible for loading critical content only after the initial page load, search engines may not index it. That is particularly problematic for content this is essential for understanding the purpose of the page or that contributes to ranking. To mitigate this risk, think implementing a fallback static version of your content, ensuring it remains accessible even if JavaScript fails to execute.
document.addEventListener('DOMContentLoaded', function() { // Check if JavaScript is enabled if (typeof fetch === 'function') { fetch('/dynamic-content') .then(response => response.text()) .then(html => { document.getElementById('content').innerHTML = html; }); } else { // Fallback content for SEO document.getElementById('content').innerHTML = 'This is the static fallback content for SEO.
'; } });
Another common pitfall is the over-reliance on client-side routing without proper handling for SEO. Single Page Applications (SPAs) often utilize frameworks that manipulate the URL and content dynamically. When done improperly, this can lead to scenarios where search engines index non-canonical URLs or miss out on important routes entirely. Hence, ensure that your application responds correctly to different URL paths and returns the appropriate content based on the request. Implementing server-side redirects or using pushState properly within the History API can drastically improve this situation.
window.onpopstate = function(event) { if (event.state) { loadPageContent(event.state.page); } }; function loadPageContent(page) { fetch(page) .then(response => response.text()) .then(html => { document.getElementById('content').innerHTML = html; history.pushState({ page: page }, '', page); }); }
Moreover, using excessive redirections in your JavaScript can create a convoluted path for search engine crawlers. Each redirect adds latency, and if not managed correctly, they can lead to “redirect chains” that frustrate users and search engines alike. To combat this, limit the use of redirects and ensure that they’re direct and efficient. Testing your site with tools like Screaming Frog can help identify such issues.
In addition to these challenges, there are also performance-related pitfalls associated with JavaScript. Large libraries or poorly optimized scripts can lead to prolonged load times, which can have a negative impact on your SEO ranking. Conducting regular audits of your JavaScript files, removing unused code, and using techniques like tree-shaking can substantially improve load performance.
const unusedFunction = () => { console.log('This function is not used anywhere!'); }; // Example of tree-shaking by removing unused code in a module bundler import { usedFunction } from './utils'; // Only the usedFunction will be included in the final bundle
Lastly, neglecting to test your JavaScript in different environments can result in errors or misconfigurations that harm your SEO. Ensure that your site is functional on various browsers and devices, and utilize tools like Google Search Console to monitor how your content is being crawled and indexed. By proactively addressing these common pitfalls, you can leverage JavaScript effectively while safeguarding your site’s SEO integrity.