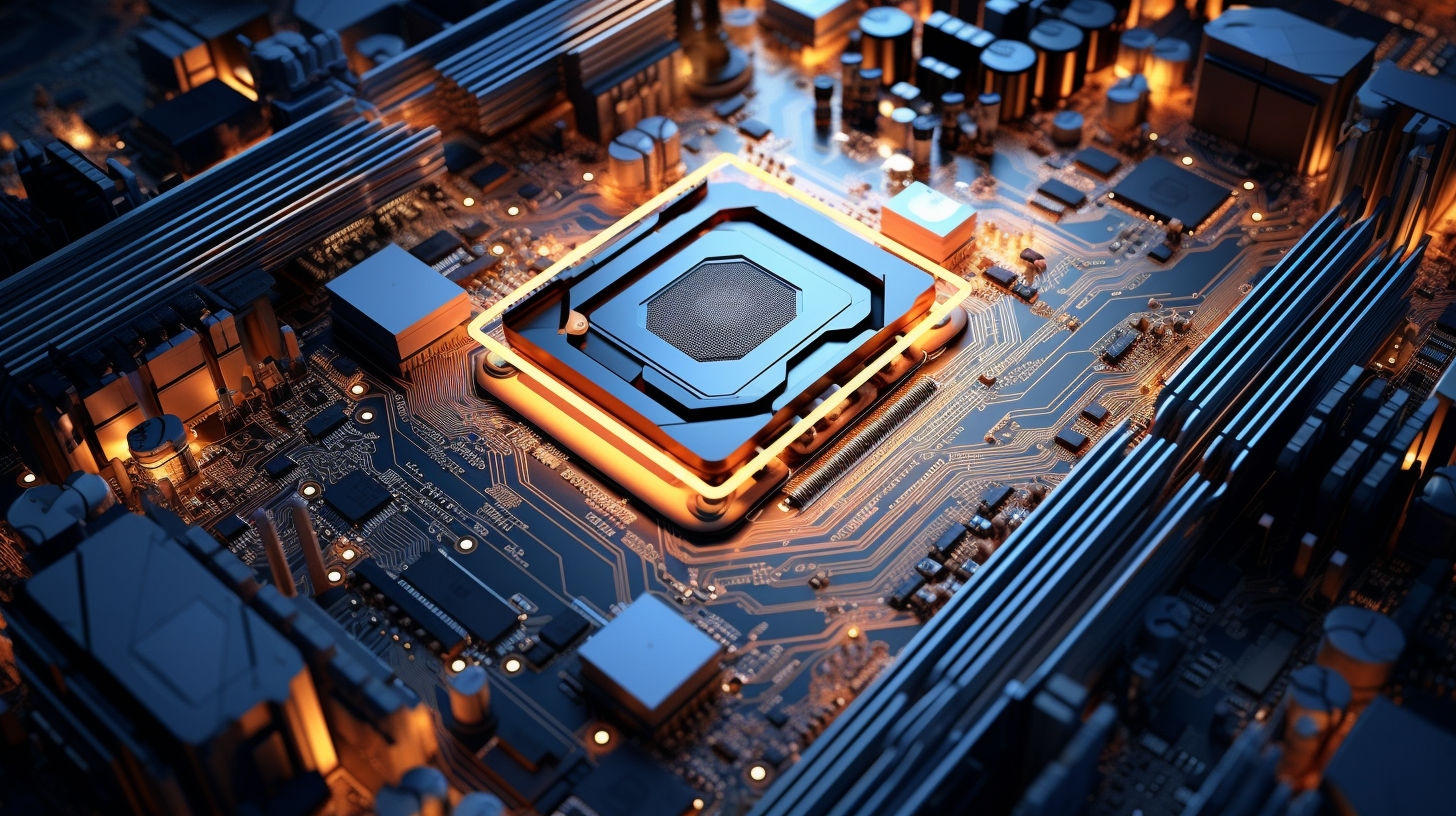
JavaScript for Sports and Fitness Tracking
JavaScript has emerged as a powerful tool in the context of sports and fitness applications, enabling developers to create dynamic, interactive experiences that engage users in their fitness journeys. With the increasing popularity of web-based applications and mobile platforms, JavaScript offers the versatility needed to build applications that track workouts, monitor progress, and provide personalized feedback.
By using JavaScript, developers can create real-time data visualizations that provide users with immediate insights into their performance. That is vital for athletes and fitness enthusiasts who seek to improve their routines, as immediate feedback can inform decisions and adjustments to workouts.
JavaScript’s ability to operate seamlessly across various devices is another significant advantage. Whether users access applications via desktop, tablet, or mobile, JavaScript ensures a consistent experience, making it easy to track activities on the go. This cross-device functionality especially important for fitness tracking, where users may want to log their activities from different locations or devices throughout the day.
Moreover, the non-blocking asynchronous nature of JavaScript allows for smooth user experiences. Applications can fetch data from servers or APIs without interrupting the user interface, enabling users to continue interacting with the app while their data updates in the background. This is particularly beneficial in fitness apps that might integrate with third-party services or databases to fetch workout plans, nutritional information, or community support.
Here’s an example of how to fetch user activity data from a hypothetical API using JavaScript:
fetch('https://api.fitnessapp.com/user/activity') .then(response => response.json()) .then(data => { console.log('User Activity:', data); }) .catch(error => { console.error('Error fetching activity data:', error); });
Incorporating geolocation features is another area where JavaScript shines. By using the Geolocation API, developers can offer location-based services, such as route tracking during runs or cycling sessions. This not only adds a layer of engagement but also helps users discover new routes and challenges based on their current location.
Furthermore, JavaScript enables the integration of gamification elements into fitness applications. By using libraries like Phaser, developers can create engaging challenges and competitions that motivate users to stay active. Implementing point systems, badges, and leaderboards can enhance user engagement and retention, fostering a sense of community among users.
Overall, JavaScript serves as a foundational technology in building robust sports and fitness applications, offering developers the tools necessary to create engaging, interactive, and effortless to handle experiences that meet the diverse needs of fitness enthusiasts.
Key Libraries and Frameworks for Tracking
As developers embark on creating sports and fitness applications, they can greatly enhance their projects by using key libraries and frameworks tailored for tracking. These tools not only streamline development but also provide built-in functionalities that cater specifically to the needs of fitness tracking.
One of the most notable libraries for building fitness applications is Chart.js. This open-source library facilitates the creation of interactive charts and graphs, allowing developers to visualize user data effectively. Whether it’s tracking progress over time or displaying workout intensity, Chart.js makes it easy to present data in a visually appealing manner. Its flexibility in chart types—such as line, bar, radar, and pie charts—ensures that developers can choose the best way to represent their data.
const ctx = document.getElementById('myChart').getContext('2d'); const myChart = new Chart(ctx, { type: 'line', data: { labels: ['January', 'February', 'March', 'April', 'May', 'June'], datasets: [{ label: 'Workout Duration (hours)', data: [1, 2, 3, 4, 5, 6], borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } } } });
In addition to Chart.js, the Moment.js library is invaluable for manipulating and displaying dates and times in fitness applications. Tracking workouts often involves significant temporal elements, such as session durations and rest periods. Moment.js simplifies these operations, making it simple to calculate durations or format dates for effortless to handle displays.
const startTime = moment('2023-01-01T10:00:00'); const endTime = moment('2023-01-01T11:30:00'); const duration = moment.duration(endTime.diff(startTime)); console.log(`Workout Duration: ${duration.hours()} hours and ${duration.minutes()} minutes`);
For developers looking to manage state efficiently in their applications, Redux or the context API of React can be invaluable. These tools help manage the app’s state, making it easier to track user input, workout history, and performance metrics. By organizing data in a predictable manner, developers can easily respond to user actions and optimize the overall user experience.
import { createStore } from 'redux'; const initialState = { workouts: [] }; const workoutReducer = (state = initialState, action) => { switch (action.type) { case 'ADD_WORKOUT': return { ...state, workouts: [...state.workouts, action.payload] }; default: return state; } }; const store = createStore(workoutReducer);
Moreover, for those working with real-time data, Socket.IO enables real-time, bidirectional communication between web clients and servers. This capability is particularly useful in fitness applications where users might want to engage in live competitions or share achievements in real time with their communities.
const socket = io('https://myfitnessapp.com'); socket.on('connect', () => { console.log('Connected to server!'); }); socket.on('newWorkout', (data) => { console.log('New workout added:', data); });
Finally, for those who wish to integrate with external APIs to fetch data regarding nutrition, workouts, or even community forums, libraries such as Axios can simplify these HTTP requests. Axios provides a promise-based API that simplifies the process of making asynchronous HTTP requests, which can be crucial for dynamically fetching user data and responding to user needs promptly.
axios.get('https://api.fitnessapp.com/user/nutrition') .then(response => { console.log('Nutritional Data:', response.data); }) .catch(error => { console.error('Error fetching nutritional data:', error); });
By using these libraries and frameworks, developers can focus on crafting rich user experiences while efficiently managing the complexities of data tracking in sports and fitness applications. As the ecosystem continues to evolve, keeping abreast of these tools will empower developers to create even more engaging and effective fitness applications.
Building User Interfaces for Activity Monitoring
Building user interfaces for activity monitoring in sports and fitness applications requires a thoughtful approach to design and usability. Developers need to create interfaces that are not only functional but also intuitive, allowing users to navigate simply through their fitness data. JavaScript plays an important role in this process, offering a plethora of tools and frameworks that facilitate the development of dynamic and responsive user interfaces.
One of the fundamental aspects of UI design in fitness applications is the incorporation of uncomplicated to manage dashboards. A dashboard serves as the command center for users, providing a quick overview of their activity statistics, workout history, and upcoming goals. To create an engaging dashboard using JavaScript, developers can utilize libraries like React.js, which allow for the building of interactive components that can update in real time as users log their activities.
import React, { useState, useEffect } from 'react'; const Dashboard = () => { const [activityData, setActivityData] = useState([]); useEffect(() => { // Fetch user activity data fetch('https://api.fitnessapp.com/user/activity') .then(response => response.json()) .then(data => setActivityData(data)); }, []); return (); }; export default Dashboard;Your Activity Dashboard
{activityData.map(activity => (
- {activity.type}: {activity.duration} minutes
))}
Another vital component is ensuring that users can easily log their workouts. A well-designed logging interface should be simpler and quick to use, allowing users to input their activity details without unnecessary complexity. Using JavaScript’s Event Handling capabilities, developers can create forms that validate user input in real time, enhancing the user experience by providing immediate feedback on any errors.
const WorkoutForm = () => { const [activity, setActivity] = useState(''); const [duration, setDuration] = useState(''); const handleSubmit = (e) => { e.preventDefault(); if (activity && duration) { // Code to submit the workout data console.log('Workout logged:', { activity, duration }); } else { alert('Please fill in all fields!'); } }; return ( setActivity(e.target.value)} /> setDuration(e.target.value)} /> ); };
Moreover, integrating visual elements such as charts and graphs into the user interface is essential for helping users understand their progress at a glance. By using libraries like Chart.js or D3.js, developers can create compelling visualizations that track changes over time. These visual elements can be embedded directly into the dashboard, providing users with an engaging way to view their fitness journey.
const ProgressChart = ({ data }) => { const ctx = React.useRef(null); useEffect(() => { const myChart = new Chart(ctx.current, { type: 'bar', data: { labels: data.labels, datasets: [{ label: 'Weekly Workout Duration', data: data.values, backgroundColor: 'rgba(75, 192, 192, 0.2)', borderColor: 'rgba(75, 192, 192, 1)', borderWidth: 1 }] }, options: { scales: { y: { beginAtZero: true } } } }); return () => myChart.destroy(); }, [data]); return ; };
To enhance user engagement, developers can also incorporate social features, enabling users to share their achievements or compete with friends. Using WebSocket technology with frameworks like Socket.IO can facilitate real-time updates to the user interface, allowing for instant feedback when friends log activities or reach milestones.
const FriendsActivity = () => { const [activities, setActivities] = useState([]); useEffect(() => { const socket = io('https://myfitnessapp.com'); socket.on('newActivity', (data) => { setActivities(prevActivities => [...prevActivities, data]); }); }, []); return (); };Friends' Activities
{activities.map(activity => (
- {activity.user}: {activity.description}
))}
By combining these elements—intuitive dashboards, easy to use logging forms, dynamic visualizations, and real-time social interactions—developers can create rich, engaging user interfaces that not only motivate users but also facilitate seamless tracking of their fitness activities. The JavaScript ecosystem provides the tools and frameworks necessary to bring these design concepts to life, ensuring that users have the best possible experience on their fitness journey.
Data Analysis and Visualization Techniques
Within the scope of sports and fitness applications, data analysis and visualization techniques are paramount to transforming raw data into actionable insights. The ability to interpret complex datasets effectively can greatly enhance a user’s understanding of their performance and progress. JavaScript, with its extensive libraries and capabilities, offers powerful tools for both data manipulation and visual representation.
Data analysis begins with the collection of metrics that matter most to users—such as workout duration, heart rate, calories burned, and distance traveled. Once this data is collected, it can be processed and analyzed using JavaScript to identify trends, patterns, and anomalies. For example, using the reduce method in JavaScript can allow developers to calculate averages or totals from an array of activity data.
const activities = [ { duration: 30, calories: 300 }, { duration: 45, calories: 500 }, { duration: 60, calories: 600 } ]; const totalCalories = activities.reduce((sum, activity) => sum + activity.calories, 0); console.log(`Total Calories Burned: ${totalCalories}`);
Once analysis is complete, the next step is visualization. Visual representation of data can make it easier for users to grasp their fitness journey at a glance. Libraries such as Chart.js or D3.js provide developers with the necessary tools to create visually appealing and informative charts. These visualizations can range from simple bar charts showing workout frequency to complex scatter plots displaying performance trends over time.
For instance, using Chart.js to create a line chart that tracks a user’s running distance over several weeks can provide immediate visual feedback regarding their progress. This not only helps users stay motivated but also facilitates goal setting based on past performances.
const ctx = document.getElementById('myLineChart').getContext('2d'); const myLineChart = new Chart(ctx, { type: 'line', data: { labels: ['Week 1', 'Week 2', 'Week 3', 'Week 4'], datasets: [{ label: 'Running Distance (km)', data: [5, 8, 10, 12], borderColor: 'rgba(75, 192, 192, 1)', fill: false }] }, options: { scales: { y: { beginAtZero: true } } } });
Moreover, JavaScript allows for dynamic updates to these visualizations, making it possible for users to see their progress in real-time as they log new activities or workouts. Using the WebSocket API in conjunction with data visualization libraries enables developers to implement real-time data feeds. For instance, athletes can view their live performance stats during training sessions, providing instant feedback that can help them adjust their efforts on the fly.
const socket = io('https://myfitnessapp.com'); socket.on('activityUpdate', (data) => { myLineChart.data.datasets[0].data.push(data.distance); myLineChart.update(); });
Beyond mere visualization, the integration of machine learning algorithms can further enhance the analysis capabilities of fitness applications. By employing libraries like TensorFlow.js, developers can analyze user data to offer personalized recommendations, suggest workouts based on performance trends, or even predict future achievements based on historical data.
import * as tf from '@tensorflow/tfjs'; const inputs = tf.tensor2d([[30], [45], [60]], [3, 1]); // Example workout durations const outputs = tf.tensor2d([[300], [500], [600]], [3, 1]); // Corresponding calories burned const model = tf.sequential(); model.add(tf.layers.dense({inputShape: [1], units: 1})); model.compile({loss: 'meanSquaredError', optimizer: 'sgd'}); // Model training and prediction would follow here
Ultimately, the combination of analysis and visualization in JavaScript applications fosters a greater understanding of fitness data, empowering users to take control of their health and wellness journeys. By using the power of JavaScript and its associated libraries, developers can create sophisticated tools that not only track but also enhance the user experience in sports and fitness tracking applications.
Integrating Wearable Devices with JavaScript
Integrating wearable devices with JavaScript opens up a realm of possibilities, allowing developers to create seamless experiences that enhance user engagement and tracking accuracy. Wearable technology, such as fitness trackers and smartwatches, has become increasingly popular in the sports and fitness industry, providing users with real-time data about their activities, heart rates, and overall health metrics. By using JavaScript, developers can access and manipulate this data to build applications that offer personalized insights and feedback.
One of the most common ways to integrate wearables is through the use of APIs provided by manufacturers. Many wearable devices come equipped with their own SDKs (Software Development Kits) and APIs that allow developers to retrieve data such as step count, heart rate, and sleep patterns. For instance, developers can use the Fitbit API to access a user’s activity data directly from their Fitbit device.
fetch('https://api.fitbit.com/1/user/-/activities/date/today.json', { method: 'GET', headers: { 'Authorization': 'Bearer ACCESS_TOKEN' } }) .then(response => response.json()) .then(data => { console.log('Fitbit Activity Data:', data); }) .catch(error => { console.error('Error fetching Fitbit data:', error); });
The example above demonstrates how to perform a simple GET request to retrieve a user’s daily activity data from Fitbit. The returned data can include a variety of metrics, such as total steps taken, calories burned, and active minutes. By processing this data, developers can present insights that are tailored to the user’s fitness level and goals.
Furthermore, Web Bluetooth API is another powerful tool that enables web applications to communicate with Bluetooth-enabled devices directly. This allows developers to create applications that can connect to a wearable device in real-time, enabling them to receive live updates about the user’s activities.
navigator.bluetooth.requestDevice({ filters: [{ services: ['heart_rate'] }] }) .then(device => { console.log('Connected to device:', device.name); return device.gatt.connect(); }) .then(server => { console.log('Connected to GATT Server'); return server.getPrimaryService('heart_rate'); }) .then(service => { return service.getCharacteristic('heart_rate_measurement'); }) .then(characteristic => { characteristic.startNotifications().then(() => { console.log('Notifications started'); characteristic.addEventListener('characteristicvaluechanged', handleHeartRateChanged); }); }) .catch(error => { console.error('Error connecting to device:', error); }); function handleHeartRateChanged(event) { const value = event.target.value; const heartRate = value.getUint8(1); // Assuming heart rate is at index 1 console.log('Current Heart Rate:', heartRate); }
In this example, we request a Bluetooth device that provides heart rate data, connect to its GATT server, and subscribe to notifications for heart rate changes. This real-time data can be incredibly useful during workouts, allowing users to monitor their heart rate on the fly, adjust their intensity, and make informed decisions about their training.
Moreover, integrating wearables with JavaScript allows for enriching user experiences through gamification. By tracking metrics from wearables, developers can implement challenges, leaderboards, and social sharing features that encourage users to remain active and engaged. For example, users could compete in weekly step challenges based on data pulled from their wearable devices, fostering a sense of community and friendly competition.
const socket = io('https://myfitnessapp.com'); socket.on('stepChallengeUpdate', (data) => { updateLeaderboard(data); }); function updateLeaderboard(data) { // Logic to update the leaderboard based on step counts from wearables console.log('Updated Leaderboard:', data); }
This integration not only enhances user motivation but also solidifies the link between technology and personal fitness goals. By creating a platform this is responsive to users’ real-time data, developers can deliver a more personalized and impactful fitness tracking experience.
As wearables continue to evolve, the opportunities to innovate in the fitness space with JavaScript will only expand. Whether through direct API access or Bluetooth communication, integrating wearable devices effectively can lead to the development of sophisticated applications that empower users to take charge of their health and fitness journeys.