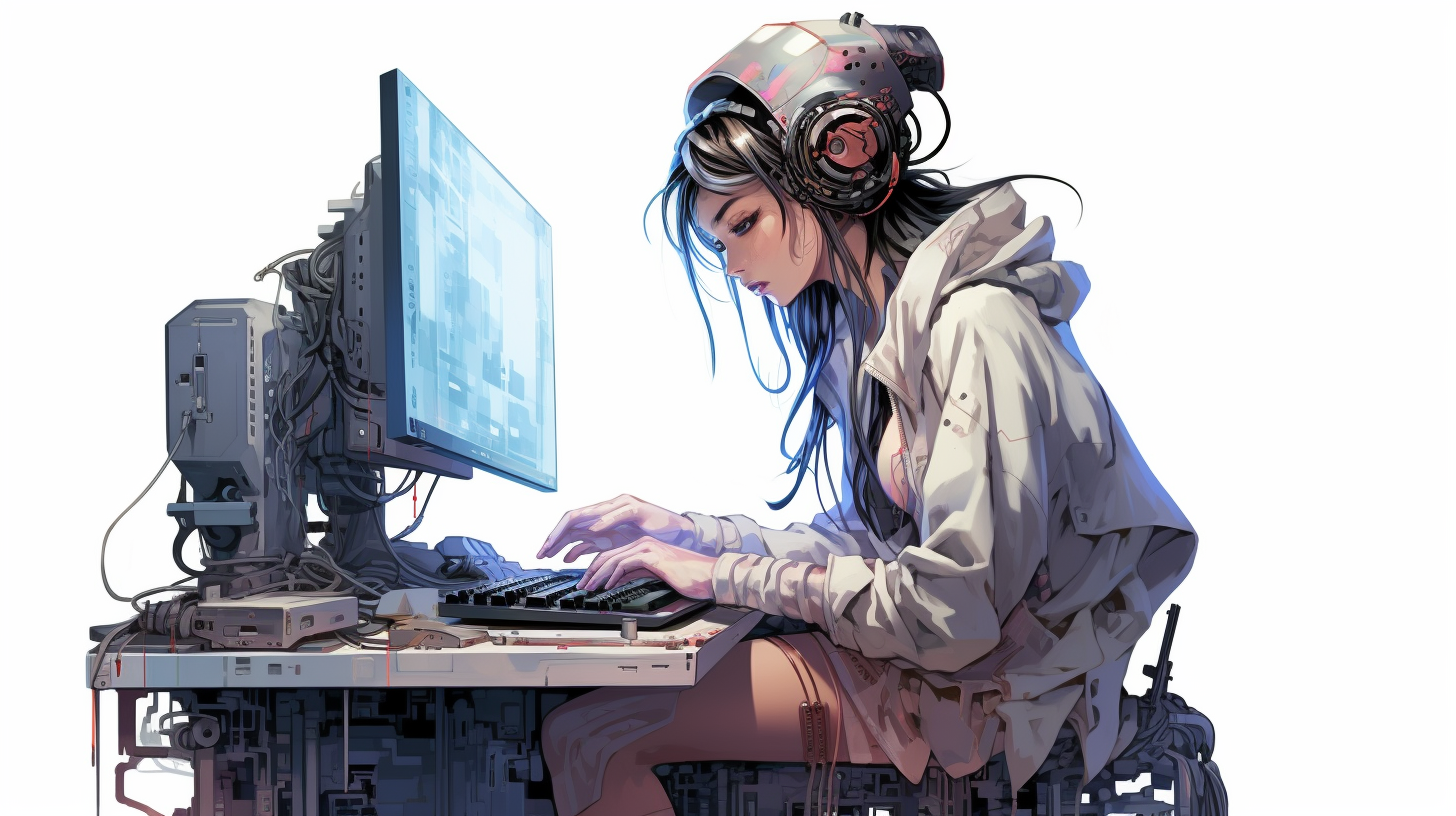
JavaScript Memory Management
JavaScript memory allocation is a fundamental concept that dictates how memory is managed during program execution. Understanding this process very important for developers aiming to write efficient and performant applications. In JavaScript, memory allocation is largely handled automatically, but knowing how it works can help you write better code and avoid common pitfalls.
When a JavaScript program runs, the engine allocates memory for variables, objects, and functions. This memory is essentially divided into two segments: the stack and the heap. The stack is a region of memory that stores static data and function call information, such as local variables, while the heap is a larger pool of memory used for dynamic allocation, primarily for objects and arrays.
When you declare a variable or create an object, memory allocation occurs:
let myNumber = 42; // Allocated on the stack let myObject = { name: "John", age: 30 }; // Allocated on the heap
In the example above, myNumber
is stored in the stack, while myObject
, being a more complex structure, is stored in the heap. The stack has a strict Last In, First Out (LIFO) structure, which makes it fast and efficient to manage. However, objects and arrays can grow in size dynamically, necessitating the use of the heap, which allows for more flexible memory allocation.
Memory allocation involves not just assigning a block of memory but also determining its type. JavaScript is a dynamically typed language, enabling you to change the type of a variable at any time. This flexibility, while convenient, can lead to complexities in memory management.
As you define and utilize more complex data structures, the JavaScript engine optimizes memory allocation and deallocation through various strategies. For example, when you create an array:
let myArray = [1, 2, 3, 4]; // Memory allocated for an array on the heap
The engine allocates sufficient memory based on the size of the array and its contents. However, when the array grows or shrinks, the engine must manage the resizing, which can involve allocating a new block of memory and copying existing data to it.
Understanding memory allocation in JavaScript is not merely academic; it has direct implications for performance. Inefficient memory usage can lead to slowdowns and, in severe cases, crashes. Developers should be mindful of how memory is allocated and released throughout the lifecycle of their applications.
By recognizing the differences between stack and heap allocation, and by paying close attention to the types and sizes of data structures you use, you can ensure that your JavaScript applications remain efficient and responsive.
Garbage Collection Mechanisms
Garbage collection in JavaScript is an automatic process that helps manage memory by reclaiming space that is no longer in use. Unlike languages that require explicit memory management, JavaScript abstracts this complexity, allowing developers to focus more on writing code rather than worrying about memory allocation and deallocation. However, understanding how garbage collection works is vital for optimizing application performance and avoiding memory-related issues.
The primary mechanism for garbage collection in JavaScript is known as reference counting. Essentially, every object in JavaScript has a reference count that tracks how many references exist to that object. When an object is created and assigned to a variable, its reference count increases. Conversely, when a reference to that object is removed or goes out of scope, the reference count decreases. Once the reference count hits zero, the JavaScript engine can safely reclaim that memory.
let myObject = { name: "Alice" }; // Reference count: 1 myObject = null; // Reference count: 0 (object can be garbage collected)
In this example, the object initially has a reference count of one when assigned to myObject
. Setting myObject
to null
decreases the reference count to zero, and the garbage collector can now reclaim the memory used by the object.
While reference counting is effective, it can lead to situations known as circular references. A circular reference occurs when two or more objects reference each other, creating a cycle that prevents their reference counts from ever reaching zero, even if they are no longer needed. To combat this issue, modern JavaScript engines use an additional mechanism known as mark-and-sweep.
In the mark-and-sweep algorithm, the garbage collector performs two main phases:
- The garbage collector traverses all live objects that are still reachable from the root objects (e.g., global variables and active function calls) and marks them as “alive.”
- After marking, the garbage collector scans through the memory heap, identifying all unmarked objects. These unmarked objects are considered unreachable and are thus eligible for garbage collection.
This approach effectively deals with circular references, as any object that is no longer accessible from the root will be marked for garbage collection, even if it references another dead object.
let objA = {}; let objB = { ref: objA }; objA.ref = objB; // Circular reference // If objA and objB go out of scope, they can still be collected using mark-and-sweep
Despite the advantages of automatic garbage collection, developers need to be aware of certain scenarios that can lead to memory leaks, where memory is not released back to the system. Common causes of memory leaks include:
- Global variables that remain in memory because they’re not properly dereferenced.
- Event listeners that are not removed, keeping references to DOM elements and preventing their garbage collection.
- Closures that inadvertently retain references to outer function scopes, preventing those scopes from being collected.
By understanding these garbage collection mechanisms and their intricacies, JavaScript developers can write more efficient code and ensure that their applications consume memory wisely, ultimately leading to better performance and user experiences.
Memory Leaks and Their Causes
Memory leaks in JavaScript occur when the application retains references to objects that are no longer needed, preventing the garbage collector from reclaiming that memory. These leaks can lead to increased memory consumption, slower performance, and ultimately, application crashes. Recognizing the common causes of memory leaks is essential for maintaining efficient memory management in your JavaScript applications.
One of the primary sources of memory leaks stems from global variables. When variables are declared in the global scope, they remain in memory as long as the application is running. If they are not explicitly dereferenced or removed, these variables can accumulate, leading to unnecessary memory usage.
var globalVar = "I'm global!"; // This global variable persists in memory
Another common issue arises from event listeners. When an event listener is attached to a DOM element and that element is later removed from the DOM, the reference to the listener can still linger, preventing the garbage collector from cleaning up the associated memory. It is crucial to remove any event listeners that are no longer needed to avoid this situation.
function handleClick() { console.log("Clicked!"); } const button = document.getElementById("myButton"); button.addEventListener("click", handleClick); // Later, if button is removed from the DOM, remember to remove the event listener button.removeEventListener("click", handleClick); // Prevent memory leak
Closures, while powerful for encapsulating variables, can also inadvertently lead to memory leaks. A closure retains references to its outer function’s variables. If the closure is long-lived and the outer function’s variables are not dereferenced, those variables will occupy memory even when they’re no longer needed.
function createClosure() { let bigData = new Array(1000000).fill("Memory Leak!"); return function() { console.log(bigData); }; } const myClosure = createClosure(); // bigData remains in memory due to closure // If myClosure is not used anymore, bigData can still leak memory
Moreover, using certain data structures, such as caches or maps, without a proper eviction strategy can lead to memory leaks. If you store references in these structures without a mechanism to remove unused items, your application can consume more memory than it should.
const cache = new Map(); function cacheResult(key, value) { cache.set(key, value); } // If old entries are not removed, they will persist in memory
To avoid memory leaks, developers should cultivate a habit of closely monitoring their code for these common pitfalls. Embracing best practices, such as proper dereferencing of variables, removing event listeners, and managing closures efficiently, can significantly reduce the likelihood of memory leaks. Additionally, using tools like memory profiling can assist in identifying and resolving memory-related issues within your applications.
Best Practices for Efficient Memory Management
Effective memory management in JavaScript is not just a technical necessity; it’s an art that can greatly influence the performance and responsiveness of your applications. As you strive to write more efficient JavaScript, it’s essential to adopt a set of best practices that can help mitigate memory issues and optimize resource usage.
1. Minimize Global Variables: Global variables persist throughout the lifecycle of your application and can lead to memory leaks if not carefully managed. Always prefer local variables within functions or modules. This practice not only reduces memory consumption but also minimizes potential naming conflicts.
let myLocalVar = "I'm local!"; // Scoped to this block only
2. Use let
and const
: One of the key features introduced in ES6 is the block-scoped let
and const
keywords. By using these, you can limit the scope of your variables, reducing the chance of unintentional memory retention from unwanted references.
{ let blockScopedVar = "I exist only here!"; } // blockScopedVar is not accessible here
3. Dereference Unused Variables: As soon as you’re done using a variable, dereference it by setting it to null
. That is particularly important for objects or arrays that might retain large amounts of data. Clearing references helps the garbage collector reclaim memory faster.
let myObject = { data: "Large data" }; // Do something with myObject myObject = null; // Dereference when done
4. Remove Event Listeners: Anytime you attach an event listener to an element, ensure to remove it when it’s no longer needed. Failing to do so can lead to memory leaks as the listener maintains a reference to the DOM element, preventing its garbage collection.
const button = document.getElementById("myButton"); function handleClick() { console.log("Button clicked!"); } button.addEventListener("click", handleClick); // Later, when the button is removed: button.removeEventListener("click", handleClick); // Clean up!
5. Watch for Closures: While closures are powerful tools for encapsulation, they can also lead to memory leaks if they retain references to unnecessary outer variables. Be cautious and ensure that the variables captured by closures are essential.
function createClosure() { let largeData = new Array(1000000).fill("Retain me!"); return function() { console.log(largeData); }; } const myClosure = createClosure(); // largeData remains in scope
6. Use Weak References: If you need to maintain a reference to an object without preventing garbage collection, think using WeakMap
or WeakSet
. These data structures allow you to reference objects without keeping them alive, significantly reducing memory overhead.
let weakMap = new WeakMap(); let obj = {}; weakMap.set(obj, "value"); // obj can be garbage collected if no other references exist
7. Regular Profiling: Regularly profile your application using built-in browser developer tools or third-party libraries. Monitoring memory usage can help identify hotspots and potential leaks before they become significant issues, allowing for timely optimization.
8. Optimize Data Structures: Choose the appropriate data structure for your needs. Using arrays for random access when objects would suffice can lead to unnecessary memory usage. Think the nature of your data and the operations you need to perform on it when choosing how to store it.
let array = [1, 2, 3, 4]; // Use when order matters let obj = { one: 1, two: 2 }; // Use when key-value pairs are needed
By adhering to these best practices, developers can significantly improve the efficiency of their JavaScript applications. With a mindful approach to memory management, you can create responsive, high-performing applications that provide a better user experience while minimizing resource waste.
Tools and Techniques for Memory Profiling
Memory profiling is an important aspect of JavaScript development that enables developers to identify memory usage patterns and detect potential issues such as leaks or inefficient memory utilization. By employing the right tools and techniques, you can gain valuable insights into how your application utilizes memory, so that you can make informed optimizations.
One of the most common tools for memory profiling is the built-in developer tools available in modern web browsers. For instance, Google Chrome offers a comprehensive set of performance profiling tools. To access these tools, open Chrome DevTools (by pressing F12 or right-clicking on the page and selecting “Inspect”), then navigate to the “Performance” or “Memory” tab. From there, you can capture snapshots of memory usage at different points in time, which will allow you to analyze the allocation patterns of objects and identify any that are unexpectedly retained in memory.
// Example of taking a heap snapshot in Chrome DevTools // Open the Memory tab and click on "Take Snapshot" to analyze memory allocation.
The “Heap Snapshot” feature of Chrome DevTools is particularly useful. It provides a detailed view of memory usage, showing you the types and sizes of objects in memory, as well as their reference chains. By comparing multiple snapshots taken at different times, you can identify which objects are not being released and track down the sources of potential memory leaks.
Another powerful feature is the “Timeline” view, which allows you to record JavaScript execution and memory allocation over time. This way, you can see how memory consumption changes during specific interactions or operations within your application.
For example:
// Start recording memory usage performance.mark("start"); // Execute memory-intensive code here performance.mark("end"); // Measure time taken performance.measure("Memory usage", "start", "end");
Moreover, third-party libraries like memwatch and node-memwatch can be utilized in Node.js applications to monitor memory usage and detect leaks programmatically. These libraries can provide notifications when memory usage exceeds a certain threshold or when potential leaks are detected, thereby allowing for proactive management.
In addition to these tools, adopting a systematic approach to memory profiling is beneficial. Start by creating a baseline snapshot of your application’s memory when it’s in a stable state. Then, execute common user interactions or workflows while capturing memory snapshots. Analyzing these snapshots can reveal patterns of memory usage and help identify specific functions or components that may be causing excessive memory consumption.
Finally, remember to test your application under different scenarios, including varying loads and usage patterns. This will help you understand how memory allocation behaves under stress and can spotlight areas that need optimization.
By using these tools and techniques for memory profiling, you can not only identify memory issues effectively but also enhance the overall performance and reliability of your JavaScript applications. It’s an ongoing process that requires diligence, but the results—robust, efficient, and high-performing applications—are well worth the effort.