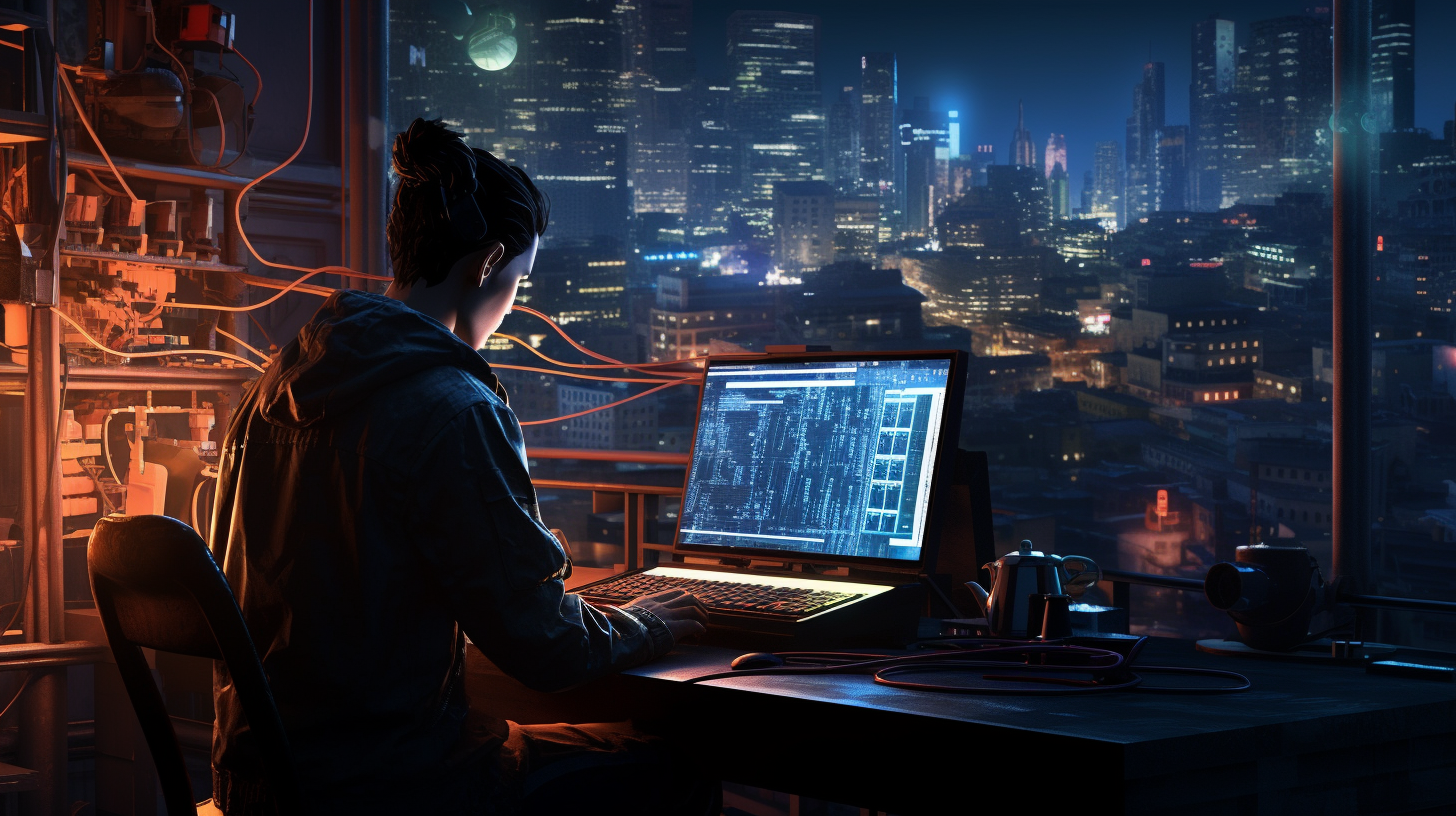
JavaScript Operators and Expressions
In JavaScript, operators are the foundational tools that allow developers to perform operations on variables and values. They’re essential for manipulating data, controlling the flow of a program, and facilitating interactions with user input and external resources.
Operators can be categorized based on their functionality, and understanding their roles is important for writing effective JavaScript code. The most common types of operators include arithmetic operators, comparison operators, logical operators, bitwise operators, assignment operators, and more.
JavaScript is a dynamically typed language, which means that variables can hold values of different types at different times. This flexibility allows for a wide range of operations to be performed on various data types, including numbers, strings, and objects.
Arithmetic operators are used for mathematical operations. For example:
let sum = 5 + 10; // 15 let product = 5 * 10; // 50 let difference = 10 - 5; // 5 let quotient = 10 / 2; // 5
Comparison operators, on the other hand, are utilized to compare two values and return a boolean result. For instance:
let isEqual = (5 === 5); // true let isGreater = (10 > 5); // true let isLessOrEqual = (5 <= 5); // true
Logical operators are critical for combining multiple boolean expressions. They include AND (&&), OR (||), and NOT (!):
let a = true; let b = false; let result = a && b; // false result = a || b; // true result = !a; // false
Assignment operators are used to assign values to variables. They can also be combined with arithmetic operations, such as with the += operator:
let count = 0; count += 5; // count is now 5
Understanding the nuances of these operators, including their types and how they interact with different data types, forms the backbone of effective programming in JavaScript. With knowledge of operators, you can control the logic of your applications and create more dynamic and responsive web experiences.
Types of JavaScript Operators
<= 10); // true
Logical operators are vital for combining multiple boolean expressions. They include AND (&&), OR (||), and NOT (!). These operators allow for more complex decision-making processes in your code. For example:
let isTrue = true && false; // false let isEither = true || false; // true let isNotTrue = !true; // false
Bitwise operators perform operations on the binary representations of integers. They’re less commonly used in high-level programming but can be incredibly powerful when manipulating data at a low level. The bitwise operators include AND (&), OR (|), XOR (^), NOT (~), and bit shifts (<>). For example:
let bitwiseAnd = 5 & 3; // 1 (binary: 0101 & 0011 = 0001) let bitwiseOr = 5 | 3; // 7 (binary: 0101 | 0011 = 0111) let bitwiseNot = ~5; // -6 (binary: ~0101 = 1010) let leftShift = 5 << 1; // 10 (binary: 0101 << 1 = 1010)
Assignment operators are used to assign values to variables, and they can also include arithmetic operations. The most basic assignment operator is the equal sign (=), but JavaScript provides shorthand operators for convenience, such as +=, -=, *=, and /=. For instance:
let value = 10; value += 5; // value is now 15 value *= 2; // value is now 30
Additionally, JavaScript features the ternary operator, which is a shorthand for if-else conditions. It evaluates a condition and returns one of two values based on whether the condition is true or false. This can be extremely useful for concise code. For example:
let age = 18; let canVote = (age >= 18) ? "Yes" : "No"; // "Yes"
Lastly, JavaScript also includes the typeof operator, which allows you to determine the type of a variable or an expression. That’s particularly useful for debugging and ensuring that variables hold the expected data types.
let name = "Alice"; console.log(typeof name); // "string"
Understanding these various types of operators and their functionalities is key to writing efficient and effective JavaScript code. As you delve deeper into your JavaScript journey, the efficient use of these operators will help you to manipulate data and control program flow with precision.
Operator Precedence and Associativity
Understanding operator precedence and associativity is critical for writing correct and efficient JavaScript code. Operator precedence determines the order in which different operators are evaluated in an expression. If two operators have the same precedence, associativity decides the order of evaluation for these operators.
In JavaScript, operators are not all created equal; they have different levels of precedence. For example, multiplication and division have higher precedence than addition and subtraction. This means that in an expression like 5 + 10 * 2
, the multiplication will be performed first, resulting in 5 + 20
and ultimately yielding 25
rather than 30
.
let result = 5 + 10 * 2; // result is 25
To clarify this concept, ponder the following example that illustrates how operator precedence works:
let a = 10; let b = 5; let c = 2; // Here, multiplication has higher precedence than addition let result = a + b * c; // result is 20, because 5 * 2 is evaluated first
When multiple operators with the same precedence appear in an expression, associativity comes into play. JavaScript uses left-to-right associativity for most operators, which means that expressions are evaluated from the left side to the right. For example:
let x = 5 - 2 + 1; // evaluated as (5 - 2) + 1, result is 4
However, some operators have right-to-left associativity, such as the assignment operator (=
) and the exponentiation operator (**
). For instance:
let a, b, c; a = b = c = 10; // all variables a, b, and c will be assigned the value 10
In this case, the assignment is performed starting from the rightmost variable, c, and works its way leftward. Understanding the rules governing operator precedence and associativity enables developers to predict how expressions will be evaluated and avoid common pitfalls that can lead to unexpected results.
To see a more comprehensive list of operator precedence in JavaScript, think the following ordering:
// 1. Parentheses () // 2. Exponentiation (**) // 3. Unary operators (+, -, ++, --) // 4. Multiplication, Division, and Modulus (*, /, %) // 5. Addition and Subtraction (+, -) // 6. Bitwise shifts (<>, >>>) // 7. Relational operators (<, , >=) // 8. Equality operators (==, !=, ===, !==) // 9. Bitwise AND (&) // 10. Bitwise XOR (^) // 11. Bitwise OR (|) // 12. Logical AND (&&) // 13. Logical OR (||) // 14. Conditional (ternary) operator (condition ? expr1 : expr2) // 15. Assignment operators (=, +=, -=, etc.) // 16. Comma (,)
By being aware of these rules, you can write more efficient and less error-prone JavaScript code, ensuring that your expressions yield the expected results. Understanding operator precedence and associativity is essential for mastering JavaScript and elevating your programming skills.
Expressions and Their Evaluation
Expressions in JavaScript are fundamental constructs that evaluate to a value. An expression can be as simple as a single value or variable, or it can be a more complex combination of variables, operators, and function calls. The evaluation of an expression involves interpreting the components of the expression according to the rules of JavaScript, ultimately yielding a result.
Consider the following simple expression:
let a = 5; let b = 10; let result = a + b; // result is 15
In this case, the expression a + b
is evaluated by adding the values of a
and b
, resulting in 15
.
Expressions can also be more intricate, involving various operators and function calls. For example:
let x = 3; let y = 4; let z = Math.max(x, y) * 2; // z is 8
Here, the expression Math.max(x, y) * 2
first evaluates the function Math.max(x, y)
to find the maximum value between x
and y
, which is 4
. Then it multiplies that result by 2
, yielding 8
.
It’s also essential to understand how expressions can be nested. You can create expressions that make use of other expressions:
let a = 1; let b = 2; let c = 3; let result = a + b * c; // result is 7
In this example, the expression b * c
is evaluated first due to operator precedence, resulting in 6
. The outer expression then adds a
, resulting in 7
.
JavaScript allows for expressions to be grouped using parentheses, which can be a powerful way to control evaluation order:
let result = (a + b) * c; // result is 9
In this case, the expression (a + b)
is evaluated first, yielding 3
, which is then multiplied by c
, resulting in 9
.
Expressions also play a key role in control structures, such as conditionals and loops. For example:
let score = 85; if (score >= 75) { console.log('You passed!'); }
Here, the expression score >= 75
is evaluated to determine if the condition is true, allowing the subsequent block of code to execute.
Expressions are the building blocks of JavaScript programming. They can be simple or complex, involve different types of operations, and can be nested within one another. Mastering the evaluation of expressions will enhance your ability to write efficient and effective JavaScript code.
Common Use Cases and Examples
When working with JavaScript, understanding the common use cases of operators and expressions very important for writing effective code. Operators can streamline various tasks, ranging from calculations to control flow, and knowing how to employ them correctly can significantly enhance your programming efficiency.
One of the most prevalent use cases for operators is in performing calculations using arithmetic operators. For example, when calculating the total price of items in a shopping cart, you might use the following code:
let item1 = 20.99; let item2 = 15.49; let total = item1 + item2; // total is 36.48
In this scenario, the addition operator (+) aggregates the prices of two items simply. This demonstrates how arithmetic operators are vital in handling numerical data efficiently.
Comparison operators frequently come into play when validating user input or comparing values. For instance, ensuring that a user’s age meets a minimum requirement before granting access to specific content can be achieved like this:
let userAge = 18; let minimumAge = 21; if (userAge >= minimumAge) { console.log('Access granted.'); } else { console.log('Access denied.'); }
Here, the greater than or equal to operator (>=) compares the user’s age against the minimum age requirement, providing a clear control flow based on the result of that comparison.
Logical operators also find their place in complex conditional statements. Ponder validating a user’s credentials, where both username and password must meet certain conditions:
let username = 'admin'; let password = '1234'; if (username === 'admin' && password === '1234') { console.log('Login successful.'); } else { console.log('Login failed.'); }
In this example, the logical AND operator (&&) ensures that both conditions are satisfied before permitting login. This illustrates how logical operators can manage multiple conditions, streamlining decision-making in your code.
Additionally, assignment operators are commonly used for updating values in loops or functions. For instance, when calculating a running total in an iterative process, you can utilize the shorthand assignment operator:
let runningTotal = 0; let items = [10, 20, 30]; items.forEach(item => { runningTotal += item; // updates runningTotal }); console.log(runningTotal); // outputs 60
This demonstrates how the += operator efficiently updates the `runningTotal` within the loop, showcasing the practicality of assignment operators in repetitive tasks.
In more advanced scenarios, the conditional (ternary) operator can condense logic into a single expression for better readability. For example, assigning a discount based on a user’s membership status can be succinctly expressed as:
let isMember = true; let discount = isMember ? 0.1 : 0; console.log(`Your discount is ${discount * 100}%`); // outputs "Your discount is 10%"
Here, the ternary operator evaluates the `isMember` condition, ultimately simplifying the assignment of the `discount` value based on membership status.
Operators and expressions in JavaScript provide powerful and efficient means to perform a wide range of tasks. From basic mathematical calculations to complex logical conditions, mastering their common use cases enables developers to write clean, effective, and maintainable code. Using the flexibility and capabilities of JavaScript operators opens up a world of possibilities in programming, making it an invaluable skill for any developer.
Related Posts
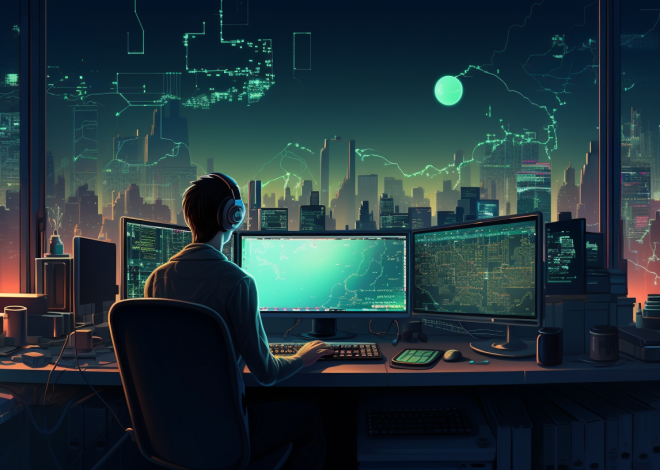
SQL for Retail Data Management
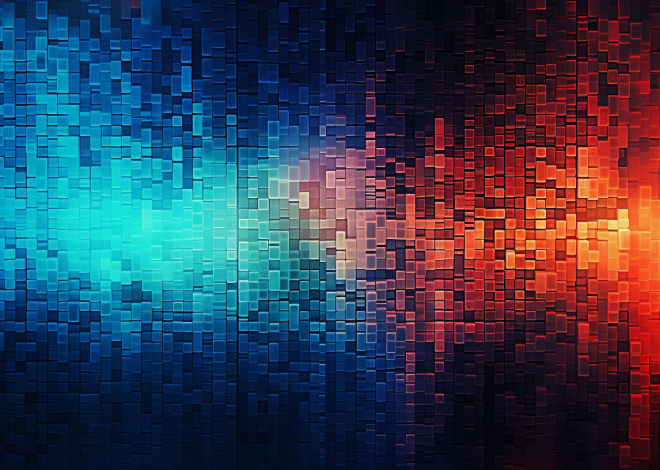
Swift and AVKit
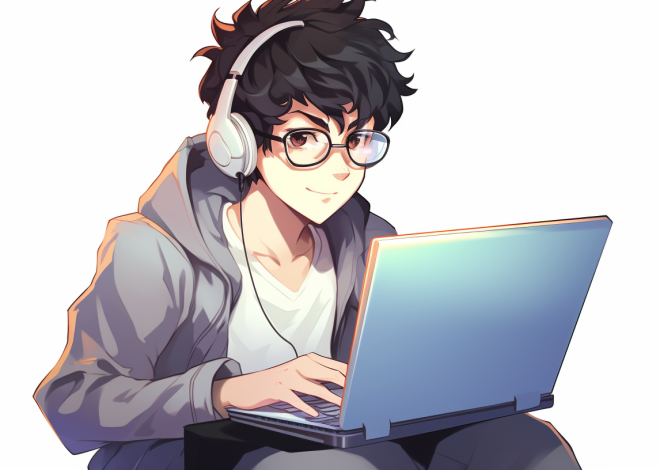