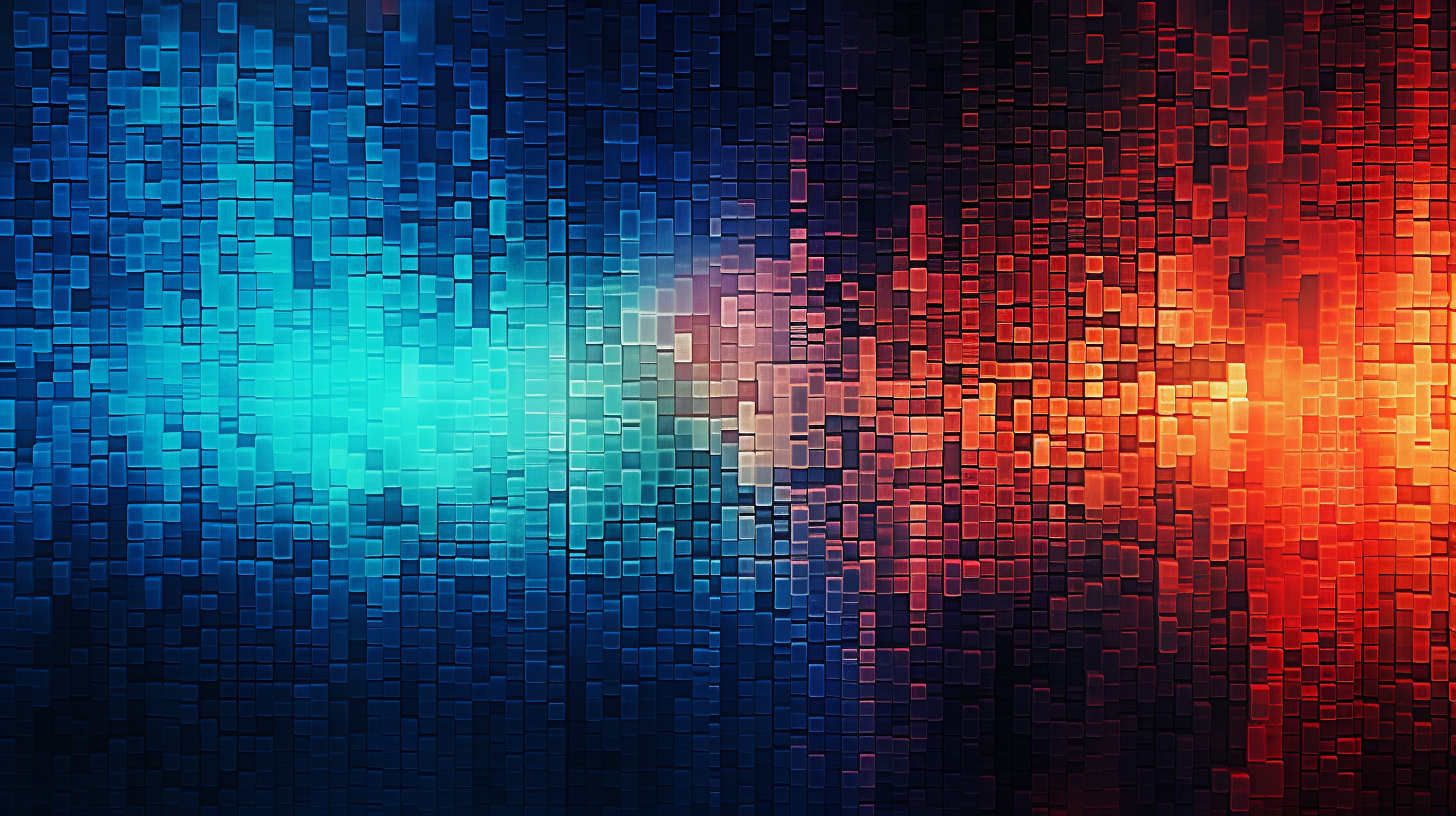
JavaScript Security Best Practices
JavaScript, while a powerful tool for enhancing user experience, harbors a number of vulnerabilities that can be exploited if not properly managed. Understanding these vulnerabilities is the first step in fortifying your applications against malicious attacks.
One of the most prevalent vulnerabilities in JavaScript is Cross-Site Scripting (XSS). This occurs when an attacker injects malicious scripts into web pages viewed by other users. For instance, if user input is not properly sanitized, attackers can manipulate the output to execute arbitrary JavaScript. Here’s a simple illustration:
const userInput = '<script>alert("XSS Attack!")</script>'; document.body.innerHTML += userInput;
In this case, the attacker’s script will execute, leading to potential data theft or session hijacking.
Another common vulnerability is Cross-Site Request Forgery (CSRF). This type of attack tricks the victim into submitting a request that they did not intend to make. For example, if a user is logged into a banking site and visits a malicious site, that site could send a request to transfer funds from the user’s account. This can happen unnoticed if the request is crafted correctly:
// Example of a CSRF attack fetch('https://banking-site.com/transfer', { method: 'POST', credentials: 'include', body: JSON.stringify({ amount: 1000, to: 'malicious-account' }), headers: { 'Content-Type': 'application/json' } });
To counter CSRF, implementing anti-CSRF tokens is essential.
Additionally, Insecure Direct Object References (IDOR) can occur when an application uses user-supplied input to access objects directly without proper authorization checks. This could allow an attacker to access or manipulate data that they shouldn’t be able to. For example:
const userId = getParameterByName('userId'); // Get user ID from URL const userData = database.getUserData(userId); // Insecure access
Without adequate checks to ensure that the user has permission to access that specific user data, IDOR vulnerabilities can be exploited.
Finally, JavaScript Injection can occur when malicious JavaScript is injected into the client-side code. This often happens in web applications that take user input and execute it without proper validation. For example:
const code = userInput; // Unsanitized code from user eval(code); // Dangerous execution of injected code
Using eval()
or similar methods with unsanitized input is a grave mistake that can lead to severe security breaches.
Implementing Secure Coding Practices
Implementing secure coding practices is critical for safeguarding JavaScript applications from various vulnerabilities. Developers must adopt a defensive programming mindset, ensuring that the code not only functions as intended but also withstands potential attacks. Here are several best practices to follow.
1. Input Validation and Sanitization
One of the first lines of defense is to rigorously validate and sanitize all user inputs. This helps prevent security issues such as XSS and SQL injection. Inputs should be checked against a list of expected formats, and any input that does not conform should be rejected. For instance, a simple validation function could look like this:
function validateInput(input) { const regex = /^[a-zA-Z0-9]*$/; // Only allow alphanumeric characters return regex.test(input); } const userInput = 'malicious'; if (!validateInput(userInput)) { console.error('Invalid input detected!'); } else { // Safe to use }
In this example, the function ensures that only alphanumeric characters are allowed, thereby blocking potentially dangerous content.
2. Use of Secure Functions
Functions that evaluate or execute code dynamically, such as eval()
, should be avoided. Instead, developers should use safer alternatives whenever possible. For example, instead of using eval()
to parse JSON, utilize JSON.parse()
:
const jsonString = '{"name": "John", "age": 30}'; const userObject = JSON.parse(jsonString); // Safe parsing of JSON
Using secure functions helps prevent code injection attacks that can occur with unsafe evaluations.
3. Implementing Content Security Policy (CSP)
A strong Content Security Policy is an essential measure that adds an additional layer of security. CSP can help mitigate XSS attacks by controlling which resources can be loaded and executed in the browser. For example:
const csp = ` default-src 'self'; script-src 'self' https://trusted-source.com; img-src 'self' data:; `; document.head.appendChild(document.createElement('meta')).setAttribute('http-equiv', 'Content-Security-Policy'); document.head.lastChild.setAttribute('content', csp);
This policy restricts scripts to be loaded only from the same origin and a specified trusted source, minimizing the risk of malicious script execution.
4. Error Handling
Proper error handling especially important in securing applications. Instead of displaying raw error messages that may reveal sensitive information, developers should log errors on the server-side and show effortless to handle messages. Here’s an example:
try { // Risky operation const data = riskyFunction(); } catch (error) { console.error('An error occurred:', error); // Log error details for debugging alert('Something went wrong. Please try again later.'); // Generic user message }
This approach prevents attackers from gaining insights into the inner workings of the application through error messages.
5. Regular Security Audits
Finally, conducting regular security audits of your codebase is vital. Use automated tools and manual reviews to identify vulnerabilities. Libraries such as npm audit
can help detect known vulnerabilities in dependencies:
// Run this in your terminal npm audit
Protecting Against Client-Side Attacks
Client-side attacks pose significant threats to JavaScript applications, and recognizing how to protect your applications from these attacks is critical. One of the most effective defenses is implementing robust client-side validation techniques, but it’s important to remember that such measures should not be your only line of defense. Security must be layered, and proper measures should be taken on both the client and server sides.
Among the various client-side attacks, Cross-Site Scripting (XSS) remains a leading concern. To mitigate such attacks, it’s crucial to apply strict encoding practices to user inputs before displaying them in the DOM. A simple yet effective approach is to escape user-entered data, ensuring that any malicious scripts are not executed. Ponder the following example:
function escapeHtml(unsafe) { return unsafe .replace(/&/g, "&") .replace(//g, ">") .replace(/"/g, """) .replace(/'/g, "'"); } const userInput = 'alert("XSS Attack!");'; document.body.innerHTML += escapeHtml(userInput);
In this code, the escapeHtml function converts potentially dangerous characters into their corresponding HTML entities, effectively neutralizing any scripts. This ensures that XSS payloads do not execute when rendered in the browser.
Another common attack vector is Man-in-the-Middle (MitM) attacks, where attackers intercept communications between the user and the server. To protect against this, always enforce HTTPS on all connections. Using secure protocols not only encrypts the data in transit but also helps ensure that connections are made to legitimate sites. Here’s how you might enforce HTTPS in your application:
if (location.protocol !== 'https:') { window.location.href = 'https://' + window.location.host + window.location.pathname; }
This snippet redirects users to the HTTPS version of the site if they attempt to access it via an unsecured HTTP connection.
Moreover, it is essential to be cautious with third-party libraries and scripts. When integrating external resources, always verify their integrity by using Subresource Integrity (SRI). This feature allows browsers to check that fetched resources are delivered without unexpected manipulation. Here’s an example of how SRI can be used:
By including the integrity attribute, you ensure that the resource has not been tampered with during transmission.
Finally, always keep your dependencies up to date. Regularly review your package.json file and run audits to detect vulnerabilities in third-party libraries. Use tools such as npm audit or yarn audit, which provide detailed reports about the security status of the packages you are using:
npm audit
This command identifies known vulnerabilities and provides guidance on how to address them, ensuring that your application remains secure against evolving threats.
Using Security Tools and Libraries
When it comes to bolstering JavaScript security, using security tools and libraries is imperative. These resources can significantly enhance the protective measures in place, allowing developers to focus on building robust applications while relying on established frameworks and libraries to mitigate vulnerabilities. Using these tools not only streamlines development processes but also enforces best practices that might otherwise be overlooked.
One of the most effective tools for safeguarding JavaScript applications is the use of libraries specifically designed for secure coding practices. For instance, DOMPurify is a popular library for sanitizing HTML and preventing XSS attacks. It effectively cleans user input, ensuring that any potentially harmful content is neutralized before it can be rendered in the DOM. Here’s an example of how to use DOMPurify:
// Assume userInput contains potentially dangerous HTML const userInput = '<script>alert("XSS Attack!")</script>'; // Purify the user input const cleanHTML = DOMPurify.sanitize(userInput); // Safely insert cleanHTML into the DOM document.body.innerHTML += cleanHTML;
This snippet demonstrates how DOMPurify effectively removes any scripts from the user input, ensuring that the application remains secure. By integrating such libraries, developers can considerably lower the risk of XSS vulnerabilities.
Another critical aspect of JavaScript security is the use of security linters and static analysis tools. Tools like ESLint with security-focused plugins can help you identify security flaws in your code before it is deployed. For example, the eslint-plugin-security plugin can flag potentially dangerous patterns in your JavaScript code, such as the use of eval() or unsanitized user inputs. Here’s how to set it up:
// Install ESLint and the security plugin npm install eslint eslint-plugin-security --save-dev // Example ESLint configuration { "env": { "browser": true, "es6": true }, "extends": "eslint:recommended", "plugins": [ "security" ], "rules": { "security/detect-eval-with-expression": "error" } }
This setup ensures that any usage of eval() will be flagged during development, prompting developers to find safer alternatives. Using such tools fosters a culture of security awareness among developers.
In addition to linters, automated vulnerability scanners play an essential role in maintaining code security. For instance, Snyk can be used to identify and fix vulnerabilities in your dependencies. It scans your project for known vulnerabilities in packages and suggests fixes or upgrades. Running Snyk is straightforward:
// Install Snyk globally npm install -g snyk // Run Snyk to test for vulnerabilities snyk test
This command analyzes your project and provides a report on any security risks, which will allow you to address them proactively. Regularly incorporating such tools into your development workflow can help maintain a strong security posture.
Furthermore, employing Content Security Policy (CSP) tools can enhance your defenses against XSS. Libraries like csp-html-webpack-plugin can be integrated into your build process to automatically generate and manage CSP headers. Here’s an example of how to configure it with Webpack:
const CspHtmlWebpackPlugin = require('csp-html-webpack-plugin'); const cspPlugin = new CspHtmlWebpackPlugin({ 'default-src': "'self'", 'script-src': ["'self'", 'https://trusted-source.com'], 'img-src': ["'self'", 'data:'] }); // Add the plugin to your Webpack configuration module.exports = { plugins: [ cspPlugin ] };
By using CSP through this plugin, you can enforce strict resource loading policies within your application, further mitigating XSS risks.