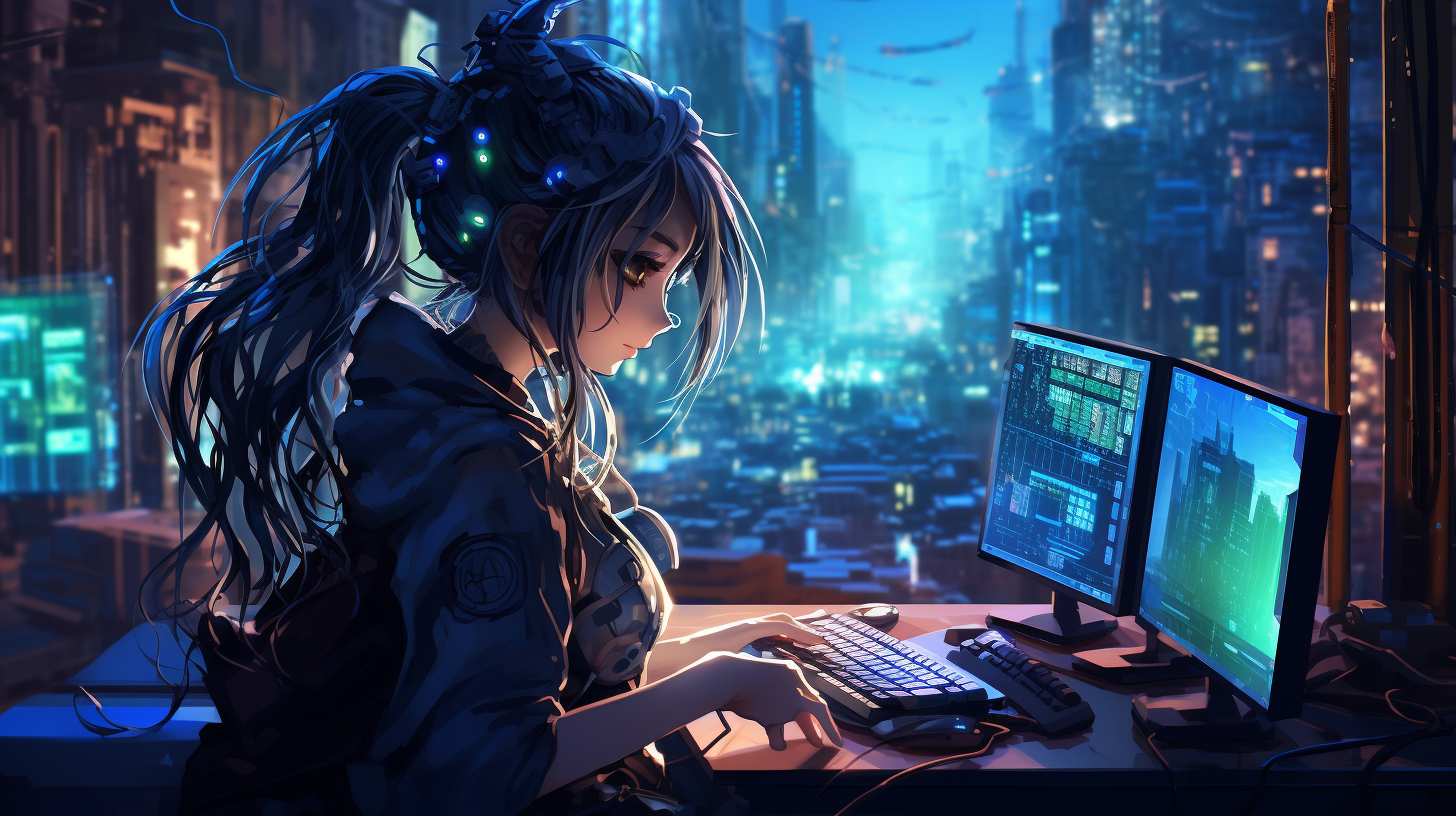
JavaScript Timers: SetTimeout and SetInterval
Within the scope of JavaScript programming, timers serve as an important mechanism for executing code at specified intervals or after a designated delay. Understanding how these timers function is essential for developing responsive and efficient applications. JavaScript provides two primary functions for timer management: setTimeout
and setInterval
.
setTimeout allows you to schedule a single execution of a function after a specified delay, defined in milliseconds. That is particularly useful for creating pauses in execution or deferring tasks until a later time. Conversely, setInterval facilitates the repeated execution of a function at regular intervals, so that you can create recurring tasks or animations.
Both functions operate asynchronously, meaning that they do not block the execution of other code while waiting. That is aligned with JavaScript’s event-driven nature, ensuring that the user interface remains responsive while background tasks are processing.
The syntax for these functions is simpler, yet it opens the door to a plethora of programming possibilities:
setTimeout(function() { console.log('Executed after 2 seconds'); }, 2000); setInterval(function() { console.log('Executed every 1 second'); }, 1000);
When invoking these functions, the first argument is typically a callback function that contains the code you wish to execute. The second argument is the duration in milliseconds.
Timers also come equipped with the ability to manage their lifecycle. You can cancel a scheduled timeout with clearTimeout and stop an interval with clearInterval. This flexibility allows for dynamic control over your operation flow, especially in response to user interactions or other events within the application.
Understanding these foundational elements of JavaScript timers equips developers to utilize them effectively, ensuring that asynchronous tasks complement the overall user experience rather than detract from it.
Using setTimeout: Syntax and Examples
To harness the power of setTimeout
, it’s important to grasp its syntax and observe its practical applications. The basic structure of the setTimeout
function is as follows:
setTimeout(callback, delay);
In this syntax, callback
is the function that you want to execute after a delay, and delay
is the time to wait before executing the callback, specified in milliseconds. For example, if you want to execute a piece of code after 3 seconds, you would do the following:
setTimeout(function() { console.log('This message is displayed after 3 seconds'); }, 3000);
In this case, the message will be logged to the console after a 3-second pause. It’s worth noting that setTimeout
returns a timer ID, which can be useful for canceling the timeout later if necessary.
Here’s a more complex example that illustrates how setTimeout
can be effectively incorporated into a real-world scenario—let’s say you want to fetch user data from an API after a short delay:
function fetchUserData() { console.log('Fetching user data...'); // Simulate fetching data } setTimeout(fetchUserData, 2000); // Fetches user data after 2 seconds
This structure allows for a non-blocking delay, giving the impression of a responsive application. To cancel a timeout, you can store the timer ID returned by setTimeout
like so:
const timerId = setTimeout(() => { console.log('This will not run if cleared.'); }, 5000); // Cancel the timeout before it executes clearTimeout(timerId);
In this example, the code inside the setTimeout
will not execute because we have canceled it using clearTimeout
prior to the delay expiring.
Let’s explore a scenario where a delayed execution can enhance the user experience. Imagine a web application that prompts the user to take some action after a period of inactivity. You could implement this feature using setTimeout
:
let inactivityTimer; function resetTimer() { clearTimeout(inactivityTimer); inactivityTimer = setTimeout(() => { alert('You have been inactive for a while. Please check your tasks.'); }, 5000); } // Reset the timer on user actions window.onload = resetTimer; document.onmousemove = resetTimer; document.onkeypress = resetTimer;
This code sets up a timer that alerts the user after 5 seconds of inactivity. Every time an action is detected (like mouse movement or keystrokes), the timer resets, preventing the alert from appearing until there is genuine inactivity.
In summary, setTimeout
is a versatile tool in the JavaScript programmer’s toolkit. By understanding its syntax and exploring various examples, you can effectively leverage its capabilities to enhance interactivity and responsiveness in your applications.
Using setInterval: Syntax and Examples
setInterval(callback, delay);
setInterval(() => { console.log('This message is displayed every 2 seconds'); }, 2000);
The setInterval function operates on a simple premise: it repeatedly invokes a specified callback function at predetermined intervals defined in milliseconds. The essential structure of setInterval is similar to that of setTimeout, but with the key distinction that setInterval is designed for recurrent execution rather than a one-time delay.
Here’s a basic example that demonstrates how to use setInterval to log a message to the console every two seconds:
setInterval(() => { console.log('This message is displayed every 2 seconds'); }, 2000);
In this case, the callback function logs a message to the console every 2 seconds without ceasing until the interval is explicitly cleared. This allows developers to create animations, update user interfaces, or perform regular checks at defined intervals.
Ponder a scenario where you want to create a simple clock that updates every second. You can achieve this by using setInterval as follows:
function updateClock() { const now = new Date(); console.log(`Current time: ${now.toLocaleTimeString()}`); } setInterval(updateClock, 1000); // Updates every second
In this example, the `updateClock` function retrieves the current time and logs it to the console every second. This example illustrates the power of setInterval in creating dynamic updates that reflect real-time data.
However, it is important to be mindful of the potential pitfalls associated with setInterval. If the execution time of the callback exceeds the specified delay, it can lead to overlapping calls, which may cause performance issues or unexpected behaviors. For example:
let count = 0; setInterval(() => { console.log(`Count: ${count}`); count++; // Simulating a long-running operation if (count === 5) { // This will take more than the interval delay for (let i = 0; i < 1e9; i++) {} } }, 1000);
In this code, the loop simulates a long-running operation that could potentially cause the next execution of the setInterval callback to overlap, leading to multiple instances running concurrently. This is something to be avoided, as it can complicate the state and performance of your application.
To manage the lifecycle of an interval timer, you can capture the timer ID returned by setInterval. This allows you to stop the repeated execution when it’s no longer necessary:
const intervalId = setInterval(() => { console.log('This will run until cleared.'); }, 1000); // Stop the interval after 5 seconds setTimeout(() => { clearInterval(intervalId); console.log('Interval cleared.'); }, 5000);
In this code segment, the interval is set to log a message every second, but we use clearInterval to stop it after 5 seconds, preventing further execution of the callback.
A practical application of setInterval can be seen in scenarios requiring periodic checks, such as monitoring user activity. For instance, if you want to check the status of an application every five seconds, you can implement it as follows:
function checkStatus() { console.log('Checking application status...'); // Insert logic to check status here } const statusCheckInterval = setInterval(checkStatus, 5000); // Clear the interval when it is no longer needed // clearInterval(statusCheckInterval);
By borrowing from these examples and understanding the implications of using setInterval, developers can harness its capabilities for creating interactive, responsive applications that require timely updates without blocking the event loop. This balance of functionality and performance is a hallmark of well-crafted JavaScript programming, enabling applications to thrive in a dynamic user-driven environment.
Clearing Timers: clearTimeout and clearInterval
Within the scope of JavaScript, managing the lifecycle of timers is as crucial as creating them. When working with tasks that are delayed or repeated, there will invariably be scenarios where you need to halt the execution of a timer for various reasons—be it user interaction, a change in state, or simply optimizing performance. The functions clearTimeout and clearInterval provide the necessary control to stop these timers from executing their callbacks.
To stop a scheduled timeout, you utilize clearTimeout. This function takes a single argument, which is the timer ID returned by setTimeout. Once invoked, it cancels the timeout, preventing the specified callback from executing. Here’s an example:
const timerId = setTimeout(() => { console.log('This message will never be displayed.'); }, 3000); // Clearing the timeout before it executes clearTimeout(timerId);
In this snippet, the timeout is cleared before the 3-second delay elapses, meaning the callback is never executed.
Similarly, when dealing with intervals, clearInterval serves as the counterpart to setInterval. Just as with setTimeout, clearInterval takes the timer ID returned by setInterval and halts any further executions of the callback function. This is particularly valuable for managing resources and preventing unnecessary computations or network requests. Here’s a simpler example:
const intervalId = setInterval(() => { console.log('This will run every second.'); }, 1000); // Clearing the interval after 5 seconds setTimeout(() => { clearInterval(intervalId); console.log('Interval cleared.'); }, 5000);
In this case, the code sets up a recurring task that logs a message every second, but it is stopped after 5 seconds by invoking clearInterval. The flexibility to stop these timers enhances control over the execution flow of your application.
A practical application of clearing timers often involves responding to specific user actions. For instance, in a scenario where you want to delay a function until a user interacts with the page, you may want to clear both the timeout and interval timers when an action occurs. This ensures that the application behaves responsively without executing unnecessary code:
let inactivityTimer; function resetTimer() { clearTimeout(inactivityTimer); inactivityTimer = setTimeout(() => { alert('You have been inactive for a while.'); }, 5000); } document.onmousemove = resetTimer; document.onkeypress = resetTimer; // Simulating a periodic check const statusCheckInterval = setInterval(() => { console.log('Checking status...'); }, 2000); // Clearing both timers when the user logs out function userLogout() { clearTimeout(inactivityTimer); clearInterval(statusCheckInterval); console.log('Logged out, timers cleared.'); }
In this scenario, both the inactivity timer and the status check interval are cleared when the user logs out. This not only conserves resources but also ensures that your application remains agile and responsive to user-driven events.
In conclusion, mastering the use of clearTimeout and clearInterval is essential for any JavaScript developer. By effectively managing timer lifecycles, you can optimize your applications, maintain responsiveness, and create a smoother user experience. Understanding when and how to halt timer executions can profoundly impact the overall performance and reliability of your JavaScript code.
Common Use Cases for Timers
JavaScript timers, particularly setTimeout and setInterval, find their way into an array of real-world applications, tapping into the need for asynchronous operations to create experiences that are both engaging and efficient. The usage of timers extends far beyond simple logging to the console; they play pivotal roles in user interface interactions, data fetching, animations, and more.
One of the most common use cases for timers is in creating captivating animations. By using setInterval, developers can render frame updates at specified intervals, simulating motion. Here’s a practical example of how to utilize setInterval to animate an element’s position on the screen:
const box = document.getElementById('box'); let position = 0; const intervalId = setInterval(() => { if (position >= 400) { clearInterval(intervalId); // Stop the animation when reaching the limit } else { position++; // Increment position box.style.left = position + 'px'; // Update the position of the box } }, 10); // Move the box every 10 milliseconds
In this example, an element with the id ‘box’ is moved horizontally across the screen. Each interval, the box’s left position is incremented, and the animation continues until the box reaches a specified limit.
Timers are also instrumental in managing user interactions in applications. For instance, they can be employed to implement features that guide user behavior based on their actions or inactions. Ponder a scenario where users need encouragement to complete tasks; you could use setTimeout to display a reminder after a certain period of inactivity:
let reminderTimer; function startReminder() { reminderTimer = setTimeout(() => { alert('Don’t forget to complete your task!'); }, 30000); // Reminder after 30 seconds of inactivity } function resetReminder() { clearTimeout(reminderTimer); startReminder(); } // Attach resetReminder to user activity events document.onmousemove = resetReminder; document.onkeypress = resetReminder; startReminder(); // Start the reminder timer when the page loads
In this setup, the reminder is scheduled to trigger after 30 seconds of user inactivity. Each time a user interacts with the page, the timer resets. This proactive approach enhances user engagement by gently nudging them towards task completion.
Another prevalent use case involves periodic data fetching, particularly in applications that require real-time updates, such as chat applications or stock market tickers. You can utilize setInterval to regularly fetch data from a server. Here’s a simplified example:
function fetchData() { console.log('Fetching new data...'); // Insert your data-fetching logic here } const dataFetchInterval = setInterval(fetchData, 5000); // Fetch every 5 seconds
This example sets an interval to check for new data every 5 seconds, ensuring that the application remains updated without requiring user intervention.
However, while timers are powerful, they should be used judiciously to avoid performance pitfalls. Overusing setInterval can lead to resource exhaustion if not managed correctly, especially when the execution time of the callback exceeds the interval duration. Therefore, it’s essential to assess the necessity of each timer and to clear them when they’re no longer needed.
By recognizing and implementing these common use cases of timers, developers can craft applications that not only meet functional requirements but also provide an enhanced user experience. The ability to utilize setTimeout and setInterval effectively contributes to the creation of responsive, interactive, and easy to use web applications.
Best Practices for Using Timers in JavaScript
When using timers in JavaScript, adhering to best practices is paramount to ensure that your code remains efficient, maintainable, and responsive. While setTimeout and setInterval are powerful tools, their misuse can lead to performance degradation and bugs that are often hard to diagnose. Below are several guidelines and practices that can help refine your implementation of these timer functions.
1. Prefer setTimeout over setInterval for Recurring Tasks
While setInterval seems like a simpler choice for tasks that need to recur, using it can lead to complications if the execution time of the callback exceeds the defined interval. This can result in overlapping function calls, which may create unintended side effects. Instead, consider using setTimeout to create a recursive function that schedules the next execution only after the current one has completed:
function repeatTask() { console.log('Task executed'); // Perform the task here // Schedule the next execution setTimeout(repeatTask, 1000); // Adjust the delay as necessary } // Start the task setTimeout(repeatTask, 1000); // Initial delay
This method ensures that each execution finishes before the next one starts, eliminating potential overlaps.
2. Clear Unnecessary Timers
It’s crucial to clear timers when they’re no longer needed. This not only prevents functions from executing at inappropriate times but also helps to conserve resources. For instance, if a user navigates away from a component that was using setInterval, ensure you call clearInterval:
const intervalId = setInterval(() => { console.log('This will run every second.'); }, 1000); // Clear when component unmounts or when it is no longer needed clearInterval(intervalId);
This practice is especially vital in single-page applications where components may be dynamically added and removed.
3. Avoid Long Running Functions in Callbacks
As discussed previously, long-running functions can disrupt the timing mechanism and lead to performance issues. Always ensure that the code inside your timer callbacks executes quickly. If a task is complex and requires significant processing, ponder breaking it into smaller chunks or using asynchronous techniques to spread out the workload:
function processInChunks(data) { // Process a chunk of data for (let i = 0; i processInChunks(data), 0); } }
This strategy keeps the application responsive while still accomplishing the necessary tasks.
4. Utilize Debouncing and Throttling
When dealing with events that can trigger timers frequently (like scrolling or resizing), employing debouncing and throttling techniques can significantly enhance performance. Debouncing ensures that a function only executes after a specified time has elapsed since the last call, while throttling limits the execution to once per specified interval:
function debounce(func, delay) { let timeoutId; return function(...args) { clearTimeout(timeoutId); timeoutId = setTimeout(() => { func.apply(this, args); }, delay); }; } const handleResize = debounce(() => { console.log('Window resized'); }, 300); window.addEventListener('resize', handleResize);
This technique is invaluable when optimizing performance for events that can occur in rapid succession.
5. Be Mindful of the Context
When passing functions as callbacks, the context (i.e., `this`) may not always point to what you expect. That’s particularly important when using methods that belong to an object. Use arrow functions or bind methods to ensure that the correct context is maintained:
class Timer { constructor() { this.count = 0; } start() { setInterval(() => { this.count++; console.log(this.count); }, 1000); } } const timer = new Timer(); timer.start();
This ensures that `this` inside the setInterval callback refers to the Timer instance.
By adhering to these best practices, you can harness the true power of JavaScript timers while avoiding common pitfalls. These principles will not only help in optimizing performance but also in creating a codebase that is cleaner, more maintainable, and easier to debug.