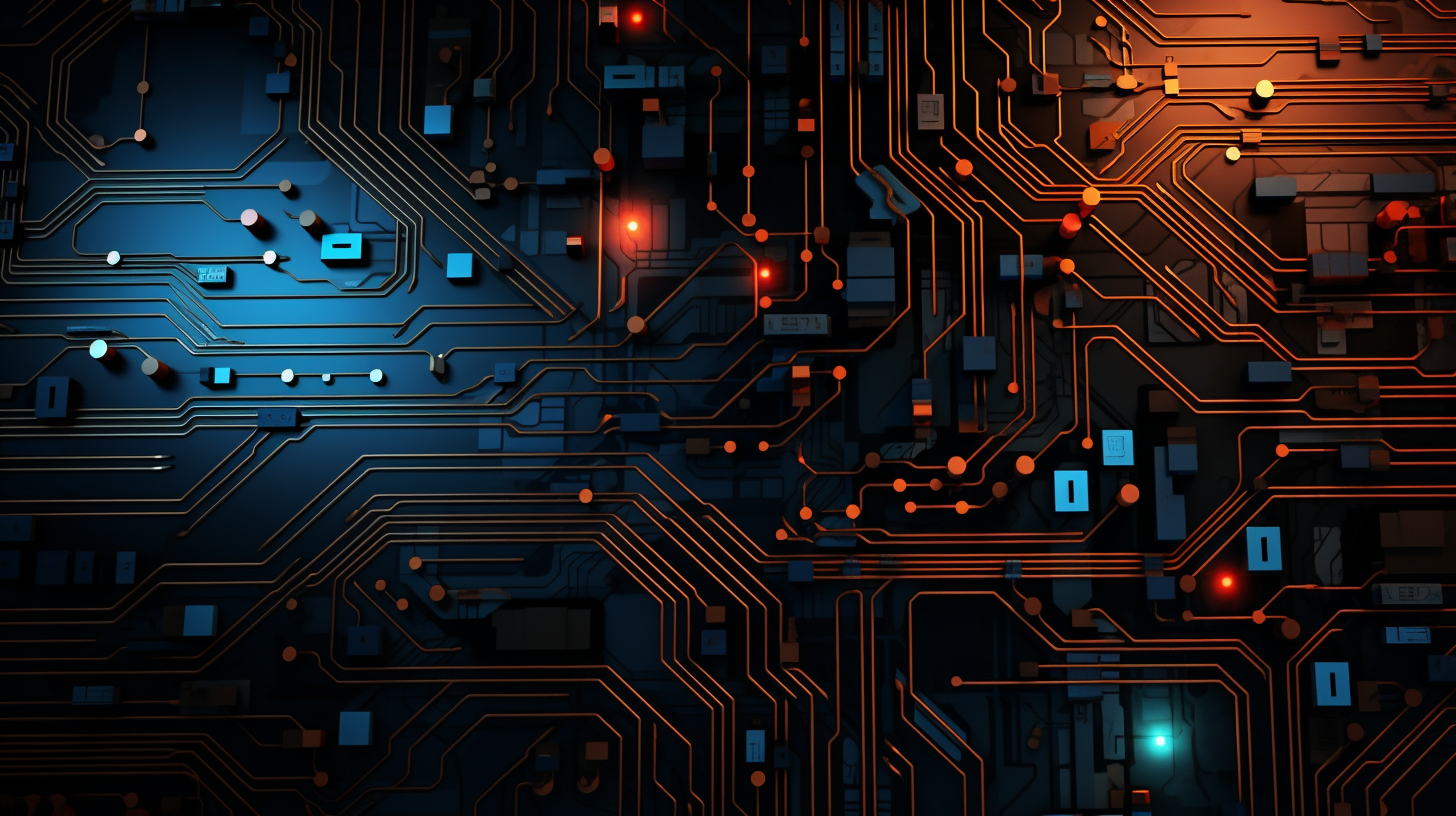
Looping in Java: For, While, Do-While
Looping constructs in Java are fundamental to controlling the flow of execution within a program. They allow developers to execute a block of code multiple times, which is particularly useful for tasks that require repetition, such as processing data, performing calculations, or iterating over collections. Understanding how different looping constructs work is essential for writing efficient and effective Java programs.
Java provides several types of loops, each suited for different scenarios. The most common looping constructs are:
- Best used when the number of iterations is known beforehand.
- Ideal for situations where the number of iterations is not known and depends on a condition.
- Similar to the while loop, but ensures that the code block is executed at least once.
When implementing loops, it’s crucial to understand the syntax and flow control of each construct. This allows for smooth execution and helps avoid common pitfalls such as infinite loops, which can occur if the loop’s termination condition is never met.
In Java, looping constructs are designed to be simple yet powerful. The for loop is particularly versatile, allowing developers to initialize a counter, check a condition, and increment or decrement the counter within a single line. Here’s a classic example of a for loop that sums the numbers from 1 to 10:
int sum = 0; for (int i = 1; i <= 10; i++) { sum += i; } System.out.println("Sum: " + sum);
The while loop, on the other hand, is suitable for scenarios where the loop must continue until a specific condition is met. This might be useful when reading data from a stream or waiting for user input:
Scanner scanner = new Scanner(System.in); String input; while (true) { System.out.print("Enter something (type 'exit' to quit): "); input = scanner.nextLine(); if ("exit".equalsIgnoreCase(input)) { break; } System.out.println("You entered: " + input); } scanner.close();
Lastly, the do-while loop guarantees that the loop’s body is executed at least once, regardless of the condition. This can be particularly useful when you need to prompt the user for input while ensuring the prompt appears at least a single time:
int number; do { System.out.print("Enter a positive number (negative to quit): "); number = scanner.nextInt(); } while (number >= 0); System.out.println("You entered a negative number. Exiting.");
Understanding when to use each of these constructs is critical for effective Java programming. As we delve deeper into the specifics of each loop type, we will explore their syntactical nuances and practical applications. Recognizing the right looping construct can lead to clearer, more maintainable code and ultimately enhance the performance of your applications.
For Loop: Syntax and Usage
int sum = 0; for (int i = 1; i <= 10; i++) { sum += i; } System.out.println("The sum of numbers from 1 to 10 is: " + sum);
In this example, the for loop initializes a variable `i` to 1 and continues to execute the block of code as long as `i` is less than or equal to 10. Each iteration increments `i` by 1, ultimately summing the integers from 1 to 10. The elegance of the for loop lies in its compact structure, which keeps all loop-related statements in one place, enhancing readability.
The general syntax of a for loop in Java is as follows:
for (initialization; termination; increment) { // code block to be executed }
– **Initialization**: This step is executed once at the start of the loop. Typically, it involves declaring and initializing a loop control variable.
– **Termination**: This condition is evaluated before each iteration, and the loop continues to run as long as it evaluates to true.
– **Increment**: This operation is executed at the end of each loop iteration, so that you can modify the loop control variable.
For loops can also be used to iterate over arrays or collections, making them incredibly useful in data processing. For instance, think an array of integers and the need to print each number:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { System.out.println("Number: " + numbers[i]); }
In this case, the loop initializes `i` to 0, and continues as long as `i` is less than the length of the array. Each iteration prints the current element of the array, showcasing the simplicity and efficiency of the for loop in traversing data structures.
One notable feature of the for loop in Java is that it can also be written in a more compact form when using the enhanced for loop (also known as the “for-each” loop). This form is particularly useful for iterating over collections or arrays without the need for an index variable:
for (int number : numbers) { System.out.println("Number: " + number); }
Here, the enhanced for loop automatically iterates through each element in the `numbers` array, making it easier to read and reducing the chance of errors associated with managing loop indices manually.
In summary, the for loop in Java is a powerful construct that provides clarity and efficiency when dealing with a known number of iterations or when processing collections. Understanding its syntax and applications allows developers to harness its full potential, enabling the creation of concise and effective loops in their Java programs.
While Loop: Syntax and Practical Applications
The while loop in Java is a powerful control structure that allows for repeated execution of a block of code as long as a specified condition remains true. Its syntax is simpler and consists of the keyword while
, followed by a condition enclosed in parentheses, and a block of code enclosed in braces. Here’s the basic syntax:
while (condition) { // code to be executed }
When using a while loop, the condition is evaluated before the execution of the loop’s body. If the condition evaluates to true
, the code inside the block executes. This continues until the condition evaluates to false
, at which point the loop terminates. This mechanism makes the while loop particularly suitable for scenarios where the number of iterations is not predetermined, such as reading data until the end of a file or waiting for user input.
For practical applications, ponder a scenario where we want to count down from a specified number to zero. A while loop can effectively handle this task, adjusting the counting variable within the loop body. Here is an example:
int count = 10; while (count > 0) { System.out.println("Countdown: " + count); count--; // Decrement the counter } System.out.println("Liftoff!");
In this example, the loop continues to execute as long as count
is greater than zero. Within the loop, we print the current value of count
and then decrement it, ensuring that the loop will eventually terminate when count
reaches zero.
Another common practical application for a while loop is processing input data. For instance, if we want to collect user input until an exit command is issued, we can utilize a while loop like so:
import java.util.Scanner; public class UserInput { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); String input; System.out.println("Enter commands (type 'exit' to quit):"); while (true) { input = scanner.nextLine(); if (input.equalsIgnoreCase("exit")) { break; // Exit the loop if the user types 'exit' } System.out.println("You entered: " + input); } scanner.close(); } }
In this example, we enter an infinite loop using while (true)
. The loop will continue until the user types “exit”, at which point we call break
to exit the loop. This pattern is common in applications that require continuous user input until a certain condition is met.
While loops can also lead to potential pitfalls, such as infinite loops, if the condition is not properly managed. It is essential to ensure that the terminating condition will eventually be met, either by modifying a variable used in the condition or by providing a break mechanism within the loop.
While loops are highly effective for scenarios where the number of iterations is not known in advance. They provide flexibility and can be employed in various situations, from countdowns to user input processing, making them a staple in the Java programmer’s toolkit.
Do-While Loop: Characteristics and Use Cases
The do-while loop in Java is a unique construct that guarantees a block of code will be executed at least once, regardless of whether the condition is true or false at the beginning. This characteristic sets it apart from the while loop, which checks the condition before executing the block of code. The syntax and flow of a do-while loop allow for scenarios where the initial execution of the code is necessary before any condition check is performed.
The do-while loop is structured as follows:
do { // Code to be executed } while (condition);
Here’s a simple yet effective example demonstrating the do-while loop in action, where we want to prompt the user at least once to enter a number:
import java.util.Scanner; public class DoWhileExample { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int number; do { System.out.print("Please enter a positive number (or -1 to exit): "); number = scanner.nextInt(); if (number != -1) { System.out.println("You entered: " + number); } } while (number != -1); System.out.println("Exiting the program."); scanner.close(); } }
In this example, the user is prompted to enter a positive number. The do-while loop ensures that the prompt is displayed to the user at least once. If the user enters -1, the loop terminates; otherwise, the entered number is printed, and the loop repeats. This pattern is particularly useful in user input scenarios, where you need to ensure the prompt is presented before checking for a termination condition.
Another practical use case for the do-while loop can be seen in menu-driven programs, where you want to display the menu to the user at least once and repeatedly until they choose to exit. Ponder the following example:
import java.util.Scanner; public class MenuDrivenExample { public static void main(String[] args) { Scanner scanner = new Scanner(System.in); int choice; do { System.out.println("Menu:"); System.out.println("1. Option 1"); System.out.println("2. Option 2"); System.out.println("3. Exit"); System.out.print("Enter your choice: "); choice = scanner.nextInt(); switch (choice) { case 1: System.out.println("You chose option 1."); break; case 2: System.out.println("You chose option 2."); break; case 3: System.out.println("Exiting the menu."); break; default: System.out.println("Invalid choice. Please try again."); } } while (choice != 3); scanner.close(); } }
In this menu-driven program, the menu is displayed to the user, and depending on their choice, different actions are taken. The do-while loop ensures that the menu is presented at least once, and it continues to display until the user selects the exit option. This design is not only uncomplicated to manage but also efficient in managing user interactions.
The do-while loop serves as a powerful tool in situations where executing the loop body at least once is a requirement. Its structure allows developers to create interactive programs and user-oriented functionalities that demand initial interaction before evaluating conditions for continuation or exit. Its versatility makes it a valuable addition to the Java programmer’s repertoire.
Comparing Looping Constructs: When to Use Each
When it comes to comparing the different looping constructs available in Java, the primary factor influencing your choice should be the specific requirements of the task at hand. Each loop type has its unique strengths and ideal use cases, which can significantly impact both the readability and performance of your code.
The for loop shines when you have a predetermined number of iterations. It’s a concise way to iterate over a range, making it perfect for simple counting tasks or iterating through arrays and collections. For instance, if you need to sum the first 100 integers, a for loop provides a clean solution:
int sum = 0; for (int i = 1; i <= 100; i++) { sum += i; } System.out.println("Sum of the first 100 integers is: " + sum);
On the other hand, the while loop comes into play when the number of iterations is not known beforehand. It is ideal for situations where you must continuously execute a block of code until a certain condition is met. That is particularly useful for scenarios such as waiting for a user input or processing data streams. Consider the following example where we read user input until a specific termination command is given:
import java.util.Scanner; Scanner scanner = new Scanner(System.in); String input; while (!input.equals("exit")) { System.out.print("Enter input (type 'exit' to quit): "); input = scanner.nextLine(); System.out.println("You entered: " + input); } scanner.close();
The do-while loop serves a distinct purpose by ensuring that the loop’s body is executed at least once, regardless of the condition. This makes it suitable for scenarios where user interaction is involved, and the prompt must be displayed to the user before checking for a valid response. The following example demonstrates a simple menu that continues to prompt the user for a choice until they decide to exit:
int choice; do { System.out.println("Menu:"); System.out.println("1. Option 1"); System.out.println("2. Option 2"); System.out.println("3. Exit"); System.out.print("Enter your choice: "); choice = scanner.nextInt(); } while (choice != 3);
In determining which loop to use, think the nature of your problem. If you need a loop that runs a specific number of times, the for loop is your best bet. If the loop’s continuation depends on dynamic conditions, a while loop is preferable. For interactive applications where at least one execution is required, the do-while loop is most appropriate.
Ultimately, the choice of looping constructs is not merely a matter of personal preference; it reflects a deeper understanding of the code’s logic, efficiency, and purpose. Selecting the right loop can lead to cleaner, more maintainable code, and improved performance in your Java applications.
Best Practices for Looping in Java
When it comes to best practices in looping within Java, there are several key considerations that can enhance both performance and readability of your code. By following these practices, you can mitigate common pitfalls and ensure that your loops are efficient and easy to understand.
1. Choose the Right Loop Type: The first step in optimizing your loops is to select the appropriate looping construct. Use a for loop when the number of iterations is known. On the other hand, if the number of iterations depends on a condition that may change over time, a while loop or do-while loop may be more suitable. For example, iterating through an array is best accomplished with a for loop:
int[] numbers = {1, 2, 3, 4, 5}; for (int i = 0; i < numbers.length; i++) { System.out.println(numbers[i]); }
2. Minimize Loop Overhead: In Java, a common practice is to minimize the work done in the loop condition. If you’re iterating over a collection, ponder caching the length or size before entering the loop. This prevents recalculating the size on each iteration, which can introduce unnecessary overhead:
List<String> list = new ArrayList<>(); // Add elements to the list int size = list.size(); // Cache the size for (int i = 0; i < size; i++) { System.out.println(list.get(i)); }
3. Avoid Infinite Loops: One of the most common pitfalls in looping is unintentionally creating an infinite loop. Always ensure that there is a clear and reachable exit condition for your loops. This can be done by carefully managing loop control variables and using break statements judiciously. For example:
int count = 10; while (count > 0) { System.out.println("Count: " + count); count--; // Ensure count is updated }
4. Use Enhanced For Loop for Collections: When working with collections or arrays, consider using the enhanced for loop (also known as the for-each loop). This construct simplifies syntax and reduces the chance of errors related to index management:
for (String item : list) { System.out.println(item); }
5. Keep Loop Bodies Simple: Try to keep the logic within the loop body as simpler as possible. Complex operations can make it difficult to understand the flow and intent of the loop. If a loop does too much, consider breaking it into smaller methods or functions to improve clarity:
for (int i = 0; i < n; i++) { processItem(i); if (shouldStop(i)) { break; } }
6. Profile and Optimize: If your application has performance concerns, use profiling tools to identify bottlenecks in your loops. Optimization should only be performed when necessary; often, clear and maintainable code is preferable to highly optimized code this is difficult to read.
By adhering to these best practices, you can write Java loops that are not only efficient but also maintainable. Thoughtful loop design promotes clearer code and reduces the likelihood of introducing errors, making your Java applications more robust and easier to understand.