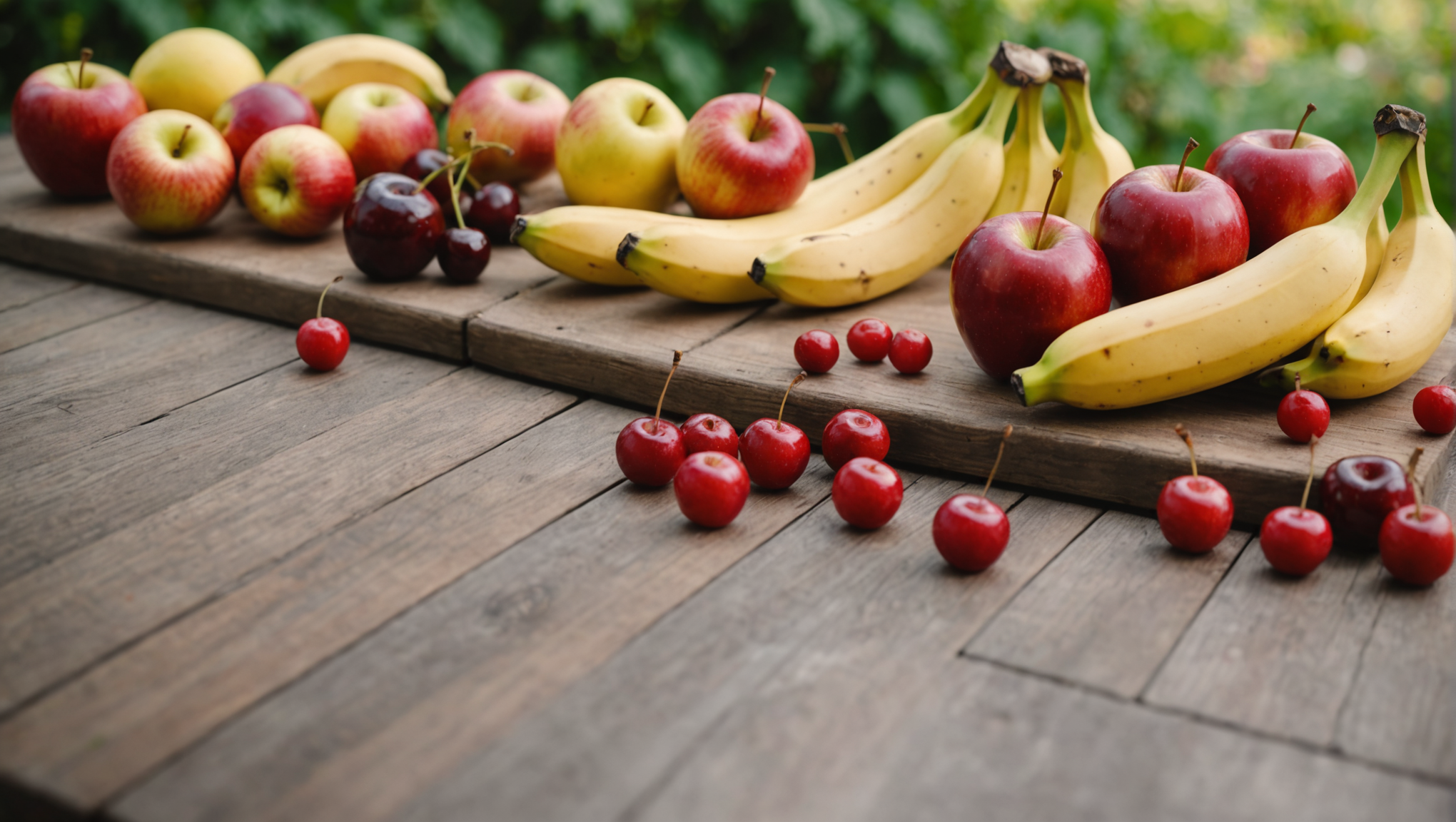
Looping in JavaScript: For, While, and More
The for loop is one of the most commonly used looping constructs in JavaScript. It provides a compact way to iterate through a block of code a specific number of times. The basic syntax of a for loop consists of three parts: initialization, condition, and increment/decrement.
for (let i = 0; i < 5; i++) { console.log(i); }
In this example, the loop initializes a variable i
to 0
, checks if i
is less than 5
, and increments i
by 1
after each iteration. The output of this code will be:
0 1 2 3 4
For loops are particularly useful when you know in advance how many times you want to execute a statement or a block of statements. Here are a few common use cases for for loops:
- You can use a for loop to access each element in an array.
- For loops are perfect for counting operations, such as generating a list of numbers.
- When you need to repeat code a specific number of times, for loops provide a clean syntax.
Here’s an example of using a for loop to iterate over an array:
const fruits = ['apple', 'banana', 'cherry']; for (let i = 0; i < fruits.length; i++) { console.log(fruits[i]); }
This code will output:
apple banana cherry
The for loop can also be customized with different initialization and increment expressions. For instance, you can decrement the loop variable or define multiple variables in the initialization:
for (let i = 5; i >= 0; i--) { console.log(i); }
This will count down from 5
to 0
, producing the following output:
5 4 3 2 1 0
The for loop is a powerful and flexible tool for developers, making it an essential part of any JavaScript programmer’s toolkit.
Mastering While and Do-While Loops
While loops are another fundamental looping construct in JavaScript, providing a method to execute a block of code repeatedly as long as a specified condition evaluates to true. The syntax of a while loop is straightforward:
while (condition) { // code to be executed }
In this structure, the condition is evaluated before each iteration. If it returns true, the code block inside the while loop executes. If it returns false, the execution of the loop stops. Here’s a simple example of a while loop:
let count = 0; while (count < 5) { console.log(count); count++; }
This code initializes a variable count
to 0 and uses a while loop to print the value of count
as long as it is less than 5. The output will be:
0 1 2 3 4
While loops are particularly useful when the number of iterations is not known beforehand and is determined by dynamic conditions. Here are some common use cases for while loops:
- Reading data until a certain condition is met, such as processing input until the user enters a specific command.
- Polling for a condition to change, like waiting for a user to take action or something in a game to change state.
- Implementing algorithms that require dynamic iteration based on the values being processed.
In contrast to while loops, the do-while loop guarantees that the code inside the loop will execute at least once, regardless of whether the condition is true or false. The syntax of a do-while loop is as follows:
do { // code to be executed } while (condition);
Here’s how it works: the code block is executed first, and then the condition is checked. If the condition is true, the loop continues; if false, the loop exits. Below is an example of a do-while loop:
let count = 0; do { console.log(count); count++; } while (count < 5);
This code will produce the same output as the previous while loop example:
0 1 2 3 4
In this case, even if count
were initialized to a value greater than or equal to 5, the code inside the do-while loop would still execute once before checking the condition. This characteristic makes do-while loops particularly useful in scenarios such as:
- Prompting users for input where you want to ensure that the prompt appears at least once.
- Executing a block of code where the initial conditions require at least one execution.
- Implementing certain algorithms where the first iteration must occur before the conditions can be checked.
When using while and do-while loops, it is crucial to ensure that the condition will eventually become false; otherwise, you risk creating an infinite loop, which can freeze or crash your application. This can be avoided by carefully managing the loop variables, ensuring that they will reach a terminating condition.
Advanced Looping Techniques: For…of and For…in
JavaScript also provides advanced looping techniques that allow for more flexibility in iterating over collections and objects: the for…of and for…in loops. These constructs simplify the process of working with iterable objects such as arrays, strings, and more.
The for…of loop is specifically designed to loop over iterable objects. It provides a clean and concise syntax for iterating through the values of an iterable, which makes it particularly useful for arrays and other collections. The syntax is as follows:
for (const element of iterable) { // code to be executed }
Here’s an example of using a for…of loop to iterate over an array:
const colors = ['red', 'green', 'blue']; for (const color of colors) { console.log(color); }
This code will output:
red green blue
The for…of loop is ideal when you need to access the values of an iterable without needing the index. It is important to note that this loop will not work with objects that are not iterable, such as plain objects without extensions.
On the other hand, the for…in loop is designed for iterating over the properties of an object. This loop enumerates over the keys (or property names) of the object, providing a way to access both the keys and their corresponding values. The syntax is as follows:
for (const key in object) { // code to be executed }
Below is an example of using a for…in loop to iterate over the properties of an object:
const person = { name: 'Alice', age: 30, city: 'New York' }; for (const key in person) { console.log(key + ': ' + person[key]); }
The output of this code will be:
name: Alice age: 30 city: New York
While the for…in loop is useful for objects, it is important to be cautious when using it with arrays, as it will iterate over all enumerable properties, which can lead to unexpected results if the array has been extended with custom properties.
Here are some key considerations when using for…of and for…in loops:
- Best suited for iterating over values in iterable objects (arrays, strings, etc.).
- Best suited for iterating over the properties of an object, but avoid using it for arrays if you need to preserve order.
- Use for…of when you need the values from an array or other iterable, and use for…in when you need the keys of an object.
Both constructs enhance your ability to work with different types of data in JavaScript, making it easier to write clear and effective code.
Performance Considerations and Best Practices for Looping
When it comes to performance considerations in JavaScript, the type of loop you choose can have a significant impact on your application, especially when dealing with large datasets or high-frequency iterations. Here are some key factors to think when optimizing loop performance:
- Generally,
for
loops are preferred for numeric iterations due to their simplicity and performance. They’re often faster thanwhile
anddo-while
loops due to the way JavaScript engines optimize these structures. - Declaring loop variables outside of the loop can sometimes lead to faster execution times since the engine doesn’t have to repeatedly allocate memory for new variables. Using
let
orconst
can also influence performance as they have block-level scope, which can be beneficial in specific contexts.
For example, modifying the previous for loop to define the loop variable outside can yield better performance:
let i; for (i = 0; i < 1000000; i++) { // Some operation }
Minimizing Operations Inside the Loop: It’s a good practice to minimize the number of operations performed inside the loop. If you have operations that can be done outside the loop, move them out. Here’s an example of a loop that does unnecessary calculations inside:
let arrayLength = array.length; for (let i = 0; i < array.length; i++) { // Accessing array length repeatedly can lead to performance issues console.log(array[i]); }
Instead, declare the length outside the loop:
let arrayLength = array.length; for (let i = 0; i < arrayLength; i++) { console.log(array[i]); }
Avoiding Side Effects Inside Loops: If the loop involves asynchronous operations, it is important to structure your code effectively to avoid side effects that can lead to unexpected behavior and performance hiccups. Keeping the looping logic simple and synchronous can help maintain performance.
Using Break and Continue Wisely: The break
and continue
statements can enhance performance by stopping the execution of the loop early or skipping iterations, as needed. However, excessive use of these statements can lead to code that’s harder to read and maintain.
for (let i = 0; i < 100; i++) { if (i > 50) break; // Exit the loop early if condition is met console.log(i); }
Using Efficient Data Structures: The choice of data structure can significantly affect performance. For example, when iterating over large datasets, using typed arrays can greatly improve performance compared to regular arrays.
Profiling Your Code: Always profile your loops to see where the bottlenecks are. Tools like Chrome’s DevTools can help you analyze performance and find out which parts of your looping logic may be slowing down your application.
By considering these performance aspects and implementing best practices, you can write more efficient JavaScript code that runs smoothly even in demanding scenarios.