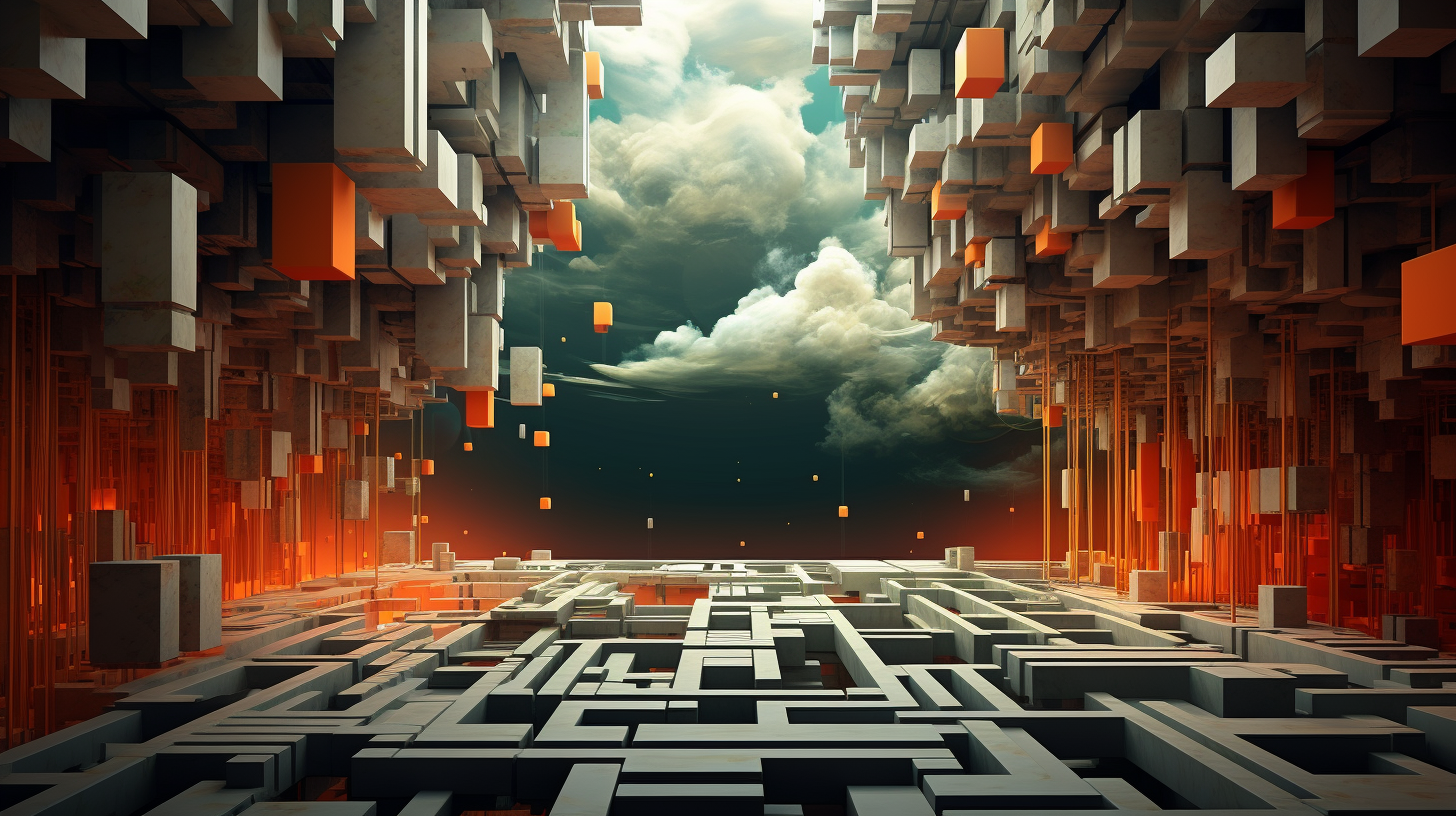
Managing Disk Space with Bash Scripts
Understanding disk usage is fundamental to managing a system’s resources effectively. In Linux and Unix-like operating systems, the disk space can be divided into various filesystem types, each with its own characteristics and optimal use cases. Filesystems such as ext4, XFS, and Btrfs are commonly used, each offering different features like journaling, snapshots, and scalability.
To gauge how much disk space is being utilized, the command df
(disk filesystem) comes in handy. It provides a snapshot of the disk usage across mounted filesystems. A typical output of df -h
might look like this:
Filesystem Size Used Avail Use% Mounted on /dev/sda1 50G 20G 28G 43% / tmpfs 2.0G 0 2.0G 0% /dev/shm /dev/sdb1 100G 70G 25G 74% /data
The du
(disk usage) command helps in discovering how much space individual files and directories take up. This is particularly useful for identifying large files that may be consuming valuable disk resources. For example, running du -sh *
in a directory provides a summary of sizes of all items within that directory:
4.0K documents 20G movies 1.5G music
Understanding your filesystem type is equally critical, as it influences performance, reliability, and available features. For instance, ext4 is known for its stability and performance on traditional spinning drives, while XFS excels in handling large files and scalability, making it a favorite for high-performance servers.
Using the command lsblk
, you can also list all block devices, showing their mount points, sizes, and types:
NAME MAJ:MIN RM SIZE RO TYPE MOUNTPOINT sda 8:0 0 50G 0 disk └─sda1 8:1 0 50G 0 part / sdb 8:16 0 100G 0 disk └─sdb1 8:17 0 100G 0 part /data
With this knowledge, you can better manage disk space by combining these commands and understanding the underlying filesystem characteristics, allowing for optimized performance and efficiency.
Basic Bash Commands for Disk Management
When it comes to managing disk space effectively, familiarity with a few basic Bash commands is essential. These commands not only allow you to assess the current state of your filesystems, but also enable you to perform necessary maintenance tasks that can prevent space-related issues before they arise.
One of the first commands you should become comfortable with is df. This command displays the amount of disk space used and available on your mounted filesystems. The -h
option is particularly useful as it presents the sizes in a human-readable format, making it easier to comprehend at a glance. Here’s how you can use it:
df -h
This command will produce output similar to the following, providing a clear overview of your disk usage:
Filesystem Size Used Avail Use% Mounted on /dev/sda1 50G 20G 28G 43% / tmpfs 2.0G 0 2.0G 0% /dev/shm /dev/sdb1 100G 70G 25G 74% /data
Another invaluable command is du, which stands for disk usage. This command helps you identify how much space individual files and directories are consuming. To get a summary of sizes for all items in the current directory, you can execute:
du -sh *
The output will provide a concise breakdown of disk usage, helping you pinpoint which files or directories are taking up the most space:
4.0K documents 20G movies 1.5G music
For a more detailed view, you can drop the -s
option to see the size of each subdirectory and file recursively:
du -h
Recognizing the block devices on your system is equally important, and the lsblk command is your go-to for this information. It lists all available block devices, providing insight into their sizes, types, and mount points. That’s particularly helpful when you need to understand the structure of your storage. Execute this command as follows:
lsblk
The output will look something like this:
NAME MAJ:MIN RM SIZE RO TYPE MOUNTPOINT sda 8:0 0 50G 0 disk └─sda1 8:1 0 50G 0 part / sdb 8:16 0 100G 0 disk └─sdb1 8:17 0 100G 0 part /data
Combining these commands allows you to maintain a keen awareness of your disk space situation. Regularly checking your filesystem usage with df, assessing individual file sizes with du, and surveying your block devices using lsblk will arm you with the information needed to make informed decisions regarding your system’s disk management.
Automating Disk Cleanup with Scripts
Automating disk cleanup with Bash scripts can significantly streamline your system maintenance tasks, so that you can reclaim valuable disk space without manual intervention. The beauty of scripting lies in its ability to execute repetitive tasks efficiently, making it an essential tool for any system administrator or power user.
One common approach to automate disk cleanup is by scheduling scripts to find and remove temporary or unnecessary files. For instance, using the find command, you can locate files that haven’t been accessed for a specified number of days and delete them. Here’s a simple script that removes files older than 30 days from a designated directory:
#!/bin/bash # Directory to clean up CLEANUP_DIR="/path/to/directory" # Find and delete files older than 30 days find "$CLEANUP_DIR" -type f -mtime +30 -exec rm {} ; echo "Cleanup completed in $CLEANUP_DIR, removing files older than 30 days."
This script defines a variable for the directory you want to clean. The find command searches for files within that directory that have not been modified in the last 30 days, and the -exec option executes the rm command to delete them. By executing this script, you can effectively keep your directory tidy without the need for manual checks.
To ensure that your cleanup scripts run automatically at specific intervals, you can utilize cron jobs. A cron job is a time-based job scheduler in Unix-like operating systems that allows scripts to run at predetermined times or intervals. To set up a cron job for the cleanup script created above, you would first open the crontab file:
crontab -e
Then, you can add a line to the crontab to schedule the script to run daily at 2 AM:
0 2 * * * /path/to/your/cleanup_script.sh
This line specifies that the script will execute at 2:00 AM every day. The structure of the cron expression is simple: it consists of five fields representing minute, hour, day of the month, month, and day of the week, respectively.
Another useful cleanup strategy could involve cleaning up logs. System logs can grow large over time, consuming disk space. Here’s a script that compresses log files older than 7 days in a specified log directory:
#!/bin/bash # Log directory LOG_DIR="/var/log/myapp" # Compress log files older than 7 days find "$LOG_DIR" -name "*.log" -type f -mtime +7 -exec gzip {} ; echo "Old log files compressed in $LOG_DIR."
This script uses a similar approach to the previous one, finding log files with a .log extension that are older than 7 days and compressing them using gzip. This not only saves space but also helps in organizing log data more efficiently.
By implementing these automated scripts and scheduling them with cron jobs, you can maintain a cleaner disk environment with minimal effort. The combination of smart scripting and scheduling is a powerful method for effective disk space management, which will allow you to focus on other important tasks while the system takes care of its own housekeeping.
Monitoring Disk Space with Cron Jobs
Monitoring disk space effectively is essential for ensuring that your system runs smoothly and without interruption. One of the most efficient ways to keep an eye on your disk usage is by using cron jobs. These scheduled tasks can automate the monitoring process, so that you can receive timely alerts or take action before running out of space. The idea is to create scripts that check disk usage at regular intervals and notify you if certain thresholds are crossed.
To get started, you can create a simple Bash script that checks the available disk space and sends an alert if the available space falls below a specified percentage. Here’s a basic example of such a script:
#!/bin/bash # Set the threshold percentage THRESHOLD=10 # Get the available space percentage AVAILABLE_SPACE=$(df / | grep / | awk '{ print $5 }' | sed 's/%//g') # Check if available space is below the threshold if [ "$AVAILABLE_SPACE" -gt "$THRESHOLD" ]; then echo "Warning: Available disk space is critically low at ${AVAILABLE_SPACE}%!" # You can add additional actions here, like sending an email else echo "Disk space is within acceptable limits at ${AVAILABLE_SPACE}%." fi
This script first defines a threshold percentage for alerting. It then uses the df command to find out the available space on the root filesystem. If the available space exceeds the threshold, a warning message is displayed. You can extend this script by integrating commands to send email notifications or log the output to a file, providing you with more visibility into your disk space status.
Once you have your monitoring script ready, you can set it to run at desired intervals using cron jobs. To schedule the script to run every hour, you would edit your crontab file:
crontab -e
Then, add the following line to schedule your disk space monitoring script:
0 * * * * /path/to/your/disk_space_check.sh
This cron expression means that the script will execute at the top of every hour. With this setup, you’ll receive regular updates about your disk space, so that you can take action before space issues escalate.
In addition to basic monitoring, you can enhance your scripts to check multiple filesystems, log the output to a file, or even trigger cleanup scripts when the threshold is crossed. Here’s an extended version that checks multiple mounted filesystems:
#!/bin/bash # Set the threshold percentage THRESHOLD=10 # Check each mounted filesystem for FILESYSTEM in $(df -h | awk 'NR>1 {print $1}'); do AVAILABLE_SPACE=$(df $FILESYSTEM | grep $FILESYSTEM | awk '{ print $5 }' | sed 's/%//g') if [ "$AVAILABLE_SPACE" -gt "$THRESHOLD" ]; then echo "Warning: Available disk space on $FILESYSTEM is critically low at ${AVAILABLE_SPACE}%!" else echo "Disk space on $FILESYSTEM is within acceptable limits at ${AVAILABLE_SPACE}%." fi done
This script iterates through each mounted filesystem, checking its available space and alerting you if any of them fall below the designated threshold. By implementing such comprehensive monitoring using cron jobs and Bash scripts, you can proactively manage your disk space, avoid critical failures, and keep your system performing optimally.
Handling Large Files and Directories
When dealing with large files and directories, a systematic approach is necessary to manage disk space effectively. Large files can quickly consume available storage and lead to performance degradation if not properly handled. To tackle this issue, you can utilize a combination of Bash commands and scripts that help identify, manage, and sometimes even automate the handling of these sizable entities.
Initially, it’s crucial to identify which files or directories are taking up significant amounts of space. The du
command is your ally here, so that you can assess disk usage and target large files or directories accordingly. For instance, executing the following command will help you find the largest directories within your current working directory:
du -h --max-depth=1 | sort -hr
This command will output the sizes of all directories up to one level deep, sorted in human-readable format. The largest directories will appear at the top, enabling you to pinpoint where the bulk of your disk space is being consumed.
To drill down further into a specific directory, you can modify the depth parameter. For instance, if you suspect that a certain directory contains large files, you can run:
du -h /path/to/directory --max-depth=1 | sort -hr
This will show you the sizes of subdirectories within the specified directory, giving you insight into where to focus your cleanup efforts.
Once you identify large files, you have several options for managing them. If certain large files are no longer needed, you can delete them using the rm
command. However, it’s always good practice to review files before removal. You might also want to move less frequently accessed large files to a different storage medium or archive them. For archiving, you can compress these files using tools like gzip
or bzip2
. Here’s an example of how to compress a file:
gzip largefile.txt
This command will create a compressed version of the file named largefile.txt.gz
, effectively reducing the amount of occupied disk space. Once you confirm that you no longer need the original file, it can be deleted to free up space.
Managing large directories may involve similar strategies, but it’s often more efficient to create a script that automates the process of identifying and archiving or deleting large files. Below is a simple example of a script that finds and compresses files larger than a specified size, for instance, 100MB:
#!/bin/bash # Directory to search for large files SEARCH_DIR="/path/to/search" # Minimum file size to consider (in bytes) MIN_SIZE=100000000 # 100MB # Find and compress files larger than MIN_SIZE find "$SEARCH_DIR" -type f -size +$MIN_SIZEc -exec gzip {} ; echo "Files larger than 100MB have been compressed in $SEARCH_DIR."
This script utilizes the find
command to locate files that exceed 100MB and compresses them with gzip
. You can adjust the MIN_SIZE
variable to suit your specific needs.
In some cases, it may be beneficial to establish criteria for retaining or deleting large files. For instance, you might decide to keep files that have been modified within the last month or files that belong to certain types. Implementing these business rules can enhance the efficiency of your disk management strategy.
Handling large files and directories involves not just identification but also taking appropriate actions based on your system’s requirements. Whether it’s compressing, archiving, or deleting, the key lies in maintaining a proactive approach to disk management with the assistance of Bash scripting and command-line utilities.
Best Practices for Disk Space Management
When it comes to managing disk space, following best practices can make all the difference. These practices can help you maintain a clean, efficient, and well-organized system, ensuring that you have sufficient disk space for your applications and services to operate smoothly. Below are some key best practices to consider:
1. Regular Monitoring
Establish a routine for checking disk space usage. Using commands like df -h
and du -sh *
will give you immediate insights into how much space is utilized and what is consuming it. You might want to set a reminder to run these commands weekly or implement cron jobs to automate these checks, logging the output to track changes over time.
2. Automated Cleanup
Incorporate automation into your disk management strategy. Scripts that delete or compress old files based on certain criteria (such as age or file type) are invaluable. Automating these tasks reduces the likelihood of human error and ensures that you are consistently managing your disk space. For example, consider setting up a cron job that runs a cleanup script weekly:
0 2 * * 0 /path/to/your/cleanup_script.sh
This schedules the cleanup script to run every Sunday at 2 AM, ensuring that your system is regularly maintained.
3. Utilize Disk Quotas
Implementing disk quotas can be an effective way to manage disk space, especially on multi-user systems. Quotas allow you to limit the amount of disk space a user or group can consume. You can set quotas using the edquota
command, which helps prevent individual users from monopolizing resources:
edquota -u username
This command opens the quota settings for the specified user in a text editor, where you can define their limits.
4. Clean Up Temporary Files
Temporary files can accumulate quickly and consume significant disk space. Implement regular clean-up routines for directories like /tmp
and /var/tmp
, which can contain outdated and unnecessary files. You can create a script to remove files older than a certain number of days:
find /tmp -type f -mtime +7 -exec rm {} ;
This command removes files in /tmp
that haven’t been modified in the last 7 days, keeping your temporary storage clean.
5. Archive Old Data
Instead of deleting old files, think archiving them. Use compression tools like tar
and gzip
to create archives of infrequently accessed data. This not only saves space but also keeps your directory structures tidy:
tar -czf archive.tar.gz /path/to/old_data
This command packages the specified directory into a compressed archive, preserving the original files while freeing up space.
6. Stay Informed About Filesystem Usage
Stay informed on filesystem types and their behavior. For example, ext4 is commonly used, but it may not be the best choice for every application. Understanding the strengths and weaknesses of different filesystems can help you make informed decisions about data placement and performance optimization.
7. Document Your Processes
Finally, document your disk management processes. Whether you’re implementing automated scripts or setting up user quotas, having clear documentation helps ensure consistency and facilitates troubleshooting should any issues arise. Keeping a log of changes and practices also aids in continuity when team members change or new staff come aboard.
By integrating these best practices into your disk space management strategy, you can maintain a healthy filesystem, optimize performance, and ensure that your system runs efficiently without the headaches that come from poor disk management.