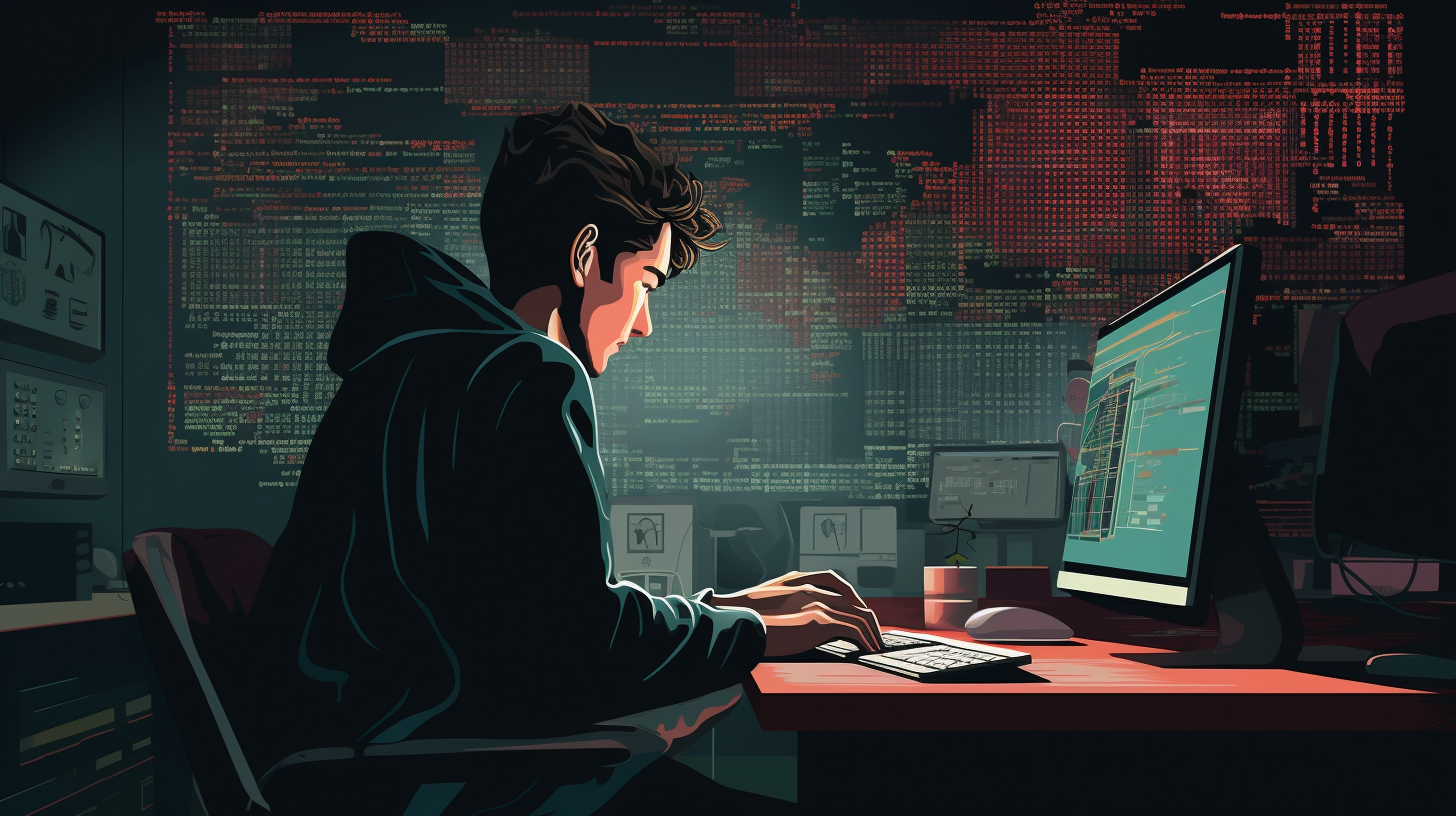
Memory Management Techniques in Bash
In Bash, memory allocation is generally abstracted away from the user, as it does not provide explicit memory management features like languages such as C or C++. However, understanding how Bash handles memory can help you write more efficient scripts that utilize system resources effectively.
When you start a Bash session, the shell allocates memory to store various types of data, including variables, functions, and commands. The size of this allocated memory is dependent on the complexity and number of the variables and structures you’re using. Bash primarily uses two types of data structures for memory allocation: scalar variables and arrays.
Scalar Variables: These are the simplest form of data storage in Bash. When you declare a scalar variable, Bash allocates enough memory to hold the contents of that variable. For example:
my_variable="Hello, World!"
In this case, Bash allocates memory to store the string “Hello, World!”. The amount of memory allocated is determined by the length of the string plus some overhead.
Arrays: Bash supports indexed arrays, which can hold multiple values under a single variable name. When you create an array, Bash allocates memory not only for the array itself but also for each of its elements. Here’s how you can declare and populate an array:
my_array=(one two three four five)
In this example, memory is allocated for the entire array, as well as for each of the individual string elements.
One important aspect of memory allocation in Bash is dynamic resizing. While arrays can grow and shrink as needed, this process is managed internally by Bash. When you append elements to an array, Bash may need to allocate additional memory, which can lead to inefficiencies if done excessively.
Understanding the nuances of how Bash allocates memory can help you avoid common pitfalls. For instance, using too many large arrays or scalar variables can consume a significant amount of memory, leading to performance degradation. Keeping an eye on memory usage is essential, especially in scripts that may run for extended periods or handle large amounts of data.
Using Arrays for Efficient Data Storage
Using arrays in Bash can greatly enhance your script’s efficiency, especially when dealing with collections of data. Arrays allow you to store multiple values in a single variable, making it easier to manage and manipulate data without resorting to numerous scalar variables. This organization not only simplifies code readability but also optimizes memory usage by keeping related data together.
Bash supports indexed arrays, where each element is accessed using its numerical index, starting from zero. Here’s a concise example of creating and accessing an indexed array:
my_array=(apple banana cherry) echo ${my_array[1]} # Outputs: banana
In addition to indexed arrays, Bash also supports associative arrays, which are key-value pairs. Associative arrays can be particularly useful for creating more complex data structures where each value is associated with a unique key. To declare an associative array, you need to use the declare
command with the -A
option:
declare -A fruit_colors fruit_colors[apple]="red" fruit_colors[banana]="yellow" echo ${fruit_colors[banana]} # Outputs: yellow
When using arrays, it’s important to understand how Bash allocates memory for them. The memory size is dynamic, meaning as you add or remove elements, Bash will adjust the memory allocation accordingly. However, this dynamic resizing can lead to inefficiencies if you frequently modify the size of the array, especially in performance-critical scripts. It’s more efficient to predefine the size of an array if you know how many elements you’ll need, or to use a loop to process elements rather than appending them one by one.
To illustrate effective use of arrays, think a script that processes a list of filenames. Using an indexed array, you can store filenames and then iterate through them efficiently:
files=("file1.txt" "file2.txt" "file3.txt") for file in "${files[@]}"; do echo "Processing $file" done
When working with associative arrays, you can also iterate through keys and values in a simpler manner:
declare -A colors=( ["apple"]="red" ["banana"]="yellow" ) for fruit in "${!colors[@]}"; do echo "$fruit is ${colors[$fruit]}" done
Dynamic Memory Management with Associative Arrays
Dynamic memory management in Bash becomes particularly powerful with the use of associative arrays. These arrays enable the storage of complex data structures by associating unique keys with corresponding values, allowing for efficient data retrieval and manipulation. Associative arrays are essentially hash tables that simplify tasks where you need to map values to specific identifiers.
To declare an associative array in Bash, utilize the declare -A
command. Once declared, you can assign values to keys as follows:
declare -A user_info user_info[username]="john_doe" user_info[email]="[email protected]" user_info[age]=30
In this example, the key username
is associated with the value john_doe
, and similarly for email and age. This structure allows for a clear and organized representation of user-related data.
Accessing values stored in an associative array is simpler. For instance, if you want to retrieve the email associated with the user, you simply reference the key:
echo "Email: ${user_info[email]}"
This will output:
Email: [email protected]
One of the significant advantages of associative arrays is the ease with which you can manage data collections without relying on positional indices. This is particularly useful when the keys are more meaningful than numerical indexes, enhancing code readability and maintainability.
Moreover, when dealing with a large dataset, performance becomes a concern. Associative arrays excel at this by providing average-case constant time complexity for lookups, which can be a game changer in scripts that require frequent data access.
Consider a scenario where you need to count the occurrences of words in a text. An associative array can efficiently store each word as a key and count its occurrences as the value:
declare -A word_count for word in "hello" "world" "hello" "bash"; do ((word_count[$word]++)) done for word in "${!word_count[@]}"; do echo "$word: ${word_count[$word]}" done
This code snippet will output:
hello: 2 world: 1 bash: 1
Using associative arrays not only simplifies the code but also optimizes memory usage, as the data structure is dynamically allocated and deallocated based on usage. However, as with any dynamic memory structure, be mindful of the size and number of keys being stored. Excessive use of large associative arrays can still lead to performance issues.
Garbage Collection and Cleanup Strategies
Garbage collection in Bash is not an explicit operation as it is in languages like Java or Python, where memory management is automated and handled by the runtime. Instead, Bash relies on its own mechanisms for memory cleanup, which very important for maintaining efficiency, especially in long-running scripts or those that handle substantial amounts of data.
When variables or arrays go out of scope, or when a script finishes executing, Bash reclaims the memory allocated for them. However, it’s up to the programmer to manage resources wisely to prevent memory leaks, where unused variables still consume memory unnecessarily.
To ensure effective cleanup, you can explicitly unset variables or arrays when they are no longer needed. That is particularly important in scripts that create a large number of temporary variables or handle large datasets. The unset
command is your friend here:
unset my_variable
For arrays, you can unset the entire array using:
unset my_array
In cases where you have multiple variables that need to be removed, you can also unset them in a single command:
unset var1 var2 var3
Another key aspect of memory management in Bash is the use of local and global variables. By defining variables as local within functions, you ensure they are only available within the function’s scope and automatically cleaned up when the function exits. This can significantly reduce memory usage in scripts that utilize functions extensively:
my_function() { local local_var="I'm local!" echo $local_var } my_function # local_var is not accessible here
In contrast, global variables persist throughout the script’s lifetime, which can lead to increased memory usage if not managed properly. Keeping track of your variable scope is essential for efficient memory management.
For long-running scripts, periodically using the declare -p
command to inspect variables can help you monitor what’s taking up memory. This way, you can decide if certain large variables can be unset or if data can be processed and discarded, thus freeing up resources:
declare -p my_large_array
Optimizing Memory Usage in Scripts
Optimizing memory usage in Bash scripts requires a keen understanding of how data is stored and accessed. One effective technique is to minimize the use of large global variables and frequently modify arrays, as these can lead to unnecessary memory consumption. Instead, localize data wherever possible. By using local variables within functions, you ensure that memory is freed once the function exits, reducing the overall memory footprint of your script.
Another optimization strategy is to limit the size of arrays. If you know the maximum size of an array in advance, it is beneficial to declare it with that size to avoid dynamic resizing, which is memory-intensive. For instance:
declare -a my_array my_array=($(seq 1 100)) # Pre-allocating an array initialized with 100 elements
Moreover, think using the `read` command with the `-a` option to populate an array from command output efficiently, which avoids intermediate variable creation that would consume additional memory:
read -a my_array <<< "$(ls -1)"
This command will read the output of `ls -1` directly into `my_array`, streamlining the process and minimizing memory overhead.
When manipulating data, especially in loops, it’s prudent to work with subscripts and avoid creating copies of large arrays. Instead of expanding arrays inside loops, consider using a single indexed variable to iterate, thereby conserving memory:
for i in "${!my_array[@]}"; do echo "Element $i: ${my_array[$i]}" done
Additionally, you can use process substitution or pipes to handle large datasets without loading everything into memory at once. This can be particularly useful when dealing with file processing, so that you can stream data through commands without needing to store it all in RAM:
while read line; do echo "Processing: $line" done < <(cat large_file.txt)
Finally, always keep an eye on your script’s memory usage. Use commands like `top`, `htop`, or `ps` to monitor how much memory your script consumes during execution. If you notice excessive memory usage, think profiling your script to diagnose bottlenecks.
Debugging Memory Issues in Bash Scripts
Debugging memory issues in Bash scripts can be a challenging endeavor, especially because Bash does not provide built-in tools for tracking memory usage like some other programming languages. However, there are methods and best practices you can employ to pinpoint and resolve memory-related problems.
One of the first steps in debugging memory issues is to use the set
command with the -x
option. This option enables a mode of the shell where all executed commands are printed to the terminal. It allows you to see the flow of execution and track down where memory usage might be spiking:
set -x # Your script here set +x
By examining the output, you can identify which commands are consuming significant resources. Look for large array declarations or nested function calls that may not be returning to the original state, thus retaining memory unnecessarily.
Another useful command is declare -p
, which allows you to inspect variables and their states at any point in your script. By inserting this command at strategic locations, you can monitor how variable contents and sizes change over time:
declare -p my_array
If your script manipulates large datasets, ponder using the printf
command to output the memory usage at various stages. Though Bash does not directly report memory usage, tracking the sizes of your data structures can provide insight into potential issues:
echo "Size of my_array: ${#my_array[@]}"
For scripts that run for long periods or handle substantial input/output operations, the top
command is invaluable. It provides a real-time view of system resource usage, enabling you to see which processes are consuming excessive memory. You can invoke it in a separate terminal while your script is running:
top
Memory leaks may also occur if you use global variables excessively. Keeping track of variable scope especially important. Use local variables in functions and ensure to unset any variables that are no longer required:
unset my_temp_var
For associative arrays, be mindful of how their size may grow. Each time you add a new key-value pair, Bash dynamically allocates memory. Use declare -p
to check the contents and size of associative arrays periodically to ensure they are not ballooning out of control:
declare -p my_associative_array
Finally, incorporate debugging functions into your scripts. These functions can summarize memory usage and provide insights into variable states at various execution points. Here’s an example of a simple debugging function:
debug_memory() { echo "Current memory usage:" declare -p | grep -E 'my_variable|my_array|my_associative_array' }
By calling debug_memory
at key points in your script, you can gather information about the current memory state without cluttering your main codebase.