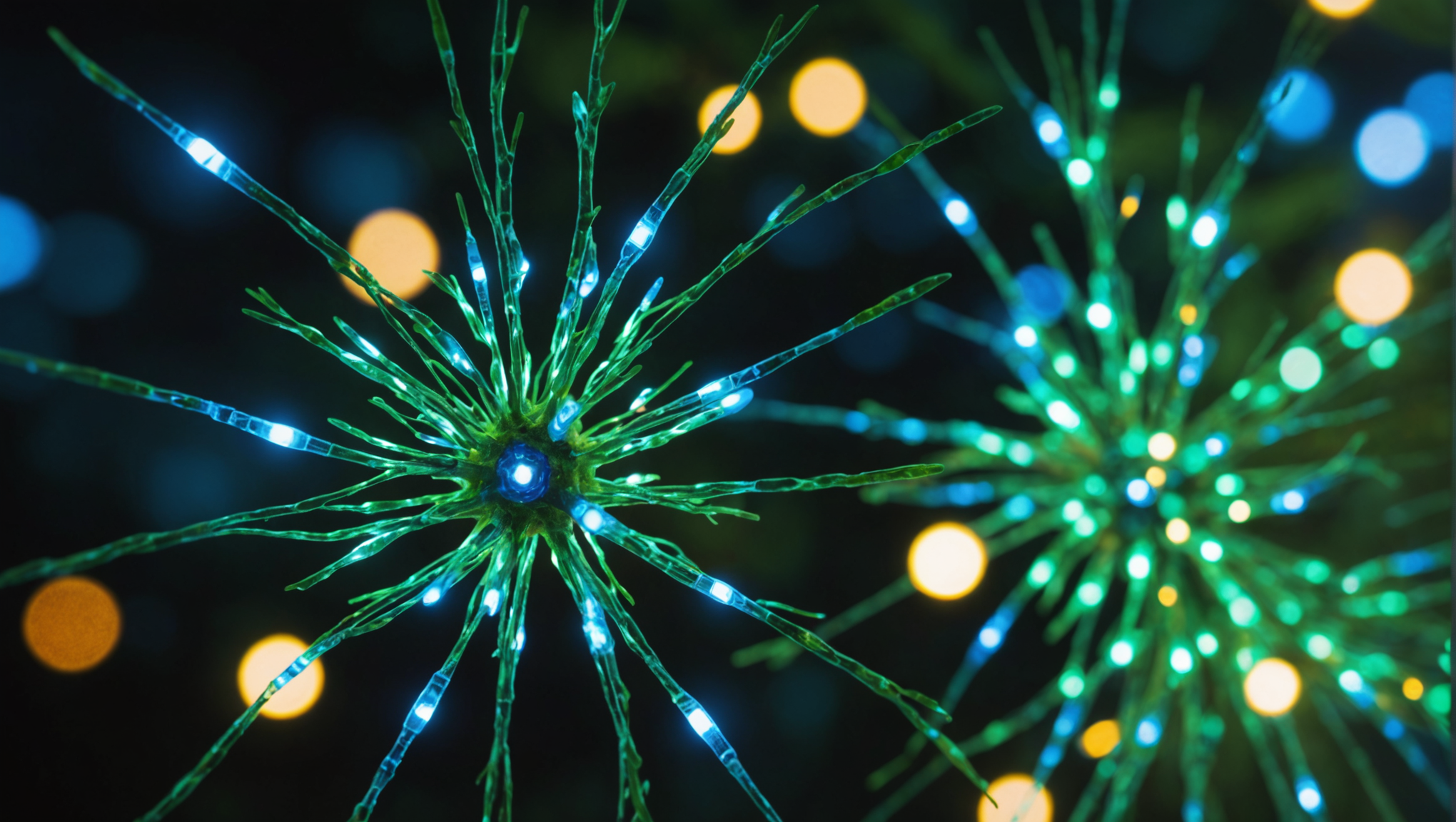
Optimizing SQL Queries with Indexes
Indexes are fundamental components of a database that significantly enhance the speed and efficiency of data retrieval operations. By creating a structured reference to the actual data stored in your tables, indexes allow the database engine to locate and access data without scanning every row. Understanding the various types of indexes and their benefits very important for optimizing SQL queries.
There are primarily two types of indexes: B-Tree Indexes and Hash Indexes.
- These are the most common type of index used in relational databases. They maintain a balanced tree structure that enables efficient searching, inserting, and deleting operations. B-Tree indexes work best for range queries and are perfect for columns with a wide variety of values.
- These indexes map keys to their corresponding values using a hash function. They provide fast access for equality comparisons but are not suitable for range queries. Hash indexes are best utilized in scenarios where exact matches are the primary requirement.
In addition to the types of indexes, understanding their benefits is equally important:
- By reducing the amount of data that needs to be scanned during query execution, indexes can drastically decrease response times. For example, instead of scanning an entire table, a query can quickly locate the indexed entries.
- Indexes can also facilitate faster sort operations. When a query requires ordered data, an index can provide the necessary order without additional sorting overhead.
- Fewer data reads from disk translate to less input/output overhead. This reduction in I/O can lead to improved performance, especially for large datasets.
- When joining tables, indexes on the join keys can significantly enhance performance by allowing the database to quickly find matching rows across tables.
Consider the following SQL command to create a B-Tree index on a ‘users’ table:
CREATE INDEX idx_users_email ON users(email);
This index will allow queries that filter or sort by the ’email’ column to execute more efficiently. By understanding the types and benefits of indexes, developers can make informed decisions to imropve the performance of their SQL queries.
Analyzing Query Performance
Once you have established the types and benefits of indexes, the next critical step is to analyze query performance. Query performance analysis very important for identifying bottlenecks and ensuring that your database operates at peak efficiency. It involves examining how queries execute and how indexes affect those executions.
To analyze query performance, you can make use of several tools and techniques provided by most relational database management systems (RDBMS). These tools help to generate execution plans, which reveal how the database engine intends to execute a query, including which indexes it uses and how data is accessed.
One of the simplest ways to analyze query performance is by using the EXPLAIN statement. By prefixing your SQL query with EXPLAIN, you can obtain insights into the query execution plan, including the chosen strategy for data retrieval, whether indexes are being utilized, and the estimated cost of operations.
EXPLAIN SELECT * FROM users WHERE email = '[email protected]';
The output from the EXPLAIN command will provide information such as the type of scan (e.g., index scan, full table scan), the number of rows examined, and the estimated cost of the operation. This information is invaluable for understanding how your queries interact with indexes and can highlight opportunities for optimization.
In addition to the EXPLAIN command, you can utilize the ANALYZE statement in some databases. This command gathers statistics about the distribution of data in your tables and provides the optimizer with the necessary information to make informed decisions about query execution plans.
ANALYZE users;
Moreover, many databases offer comprehensive performance monitoring tools that provide detailed insights into execution times, I/O statistics, and resource usage. These tools often include graphical interfaces that make it easier to visualize and interpret performance data.
Another useful practice is to monitor slow queries by enabling logging of slow query execution. This allows you to review queries that take longer than a specified threshold, which can help you identify problematic queries that may benefit from additional indexing or optimization efforts.
Ultimately, the goal of analyzing query performance is to strike a balance between query efficiency and resource consumption. By understanding how your SQL queries utilize indexes and where performance issues lie, you can make informed decisions on how to optimize your database schema, index design, and queries for the best possible performance.
Choosing the Right Index for Your Queries
Choosing the right index for your queries is a critical aspect of database optimization, as the effectiveness of an index can significantly influence query performance. The process involves analyzing the specific queries that will be executed against your database and understanding how different types of indexes can enhance their performance. The key is to align your indexing strategy with the queries you anticipate running, ensuring that you’re optimizing for the right use cases.
When determining the appropriate index types, think the nature of your queries. For instance, if your database frequently executes equality searches, a Hash index may be appropriate. Conversely, for queries that involve range searches or sorting, a B-Tree index is more suitable. In many cases, composite indexes—indexes that cover multiple columns—can provide additional performance benefits for queries that filter or sort based on multiple attributes.
Let’s illustrate this with an example. Suppose you have a ‘products’ table with the following structure:
CREATE TABLE products ( id INT PRIMARY KEY, name VARCHAR(255), category_id INT, price DECIMAL(10, 2), created_at TIMESTAMP );
Now, if you frequently run queries like:
SELECT * FROM products WHERE category_id = 3 AND price < 20.00 ORDER BY created_at DESC;
You might choose to create a composite index on the ‘category_id’ and ‘price’ columns, as well as an additional index on ‘created_at’ for sorting:
CREATE INDEX idx_products_category_price ON products(category_id, price); CREATE INDEX idx_products_created_at ON products(created_at);
This dual-indexing strategy allows the database engine to quickly filter products by category and price, and efficiently sort the results by creation date, improving overall query performance.
Another important consideration is index cardinality—the uniqueness of the index values. High cardinality indexes (where the indexed column has a large number of unique values) are typically more effective than low cardinality indexes. For example, indexing a column like ’email’ in a ‘users’ table, which is unique to each user, can dramatically improve query performance, while indexing a boolean column would yield less benefit due to its limited value range.
Additionally, the frequency of updates, inserts, and deletes on your indexed columns must be considered. Indexes can slow down these operations due to the overhead of maintaining the index structure. In cases where write performance is critical, you may need to be selective about which indexes to keep. It may be beneficial to run performance tests to determine the impact of each index on both read and write operations.
Ultimately, the goal of choosing the right index is to evaluate how your data will be queried and to create indexes that cater directly to those patterns. By carefully analyzing your query patterns, considering the data distribution and nature of your operations, you can create a tailored indexing strategy that optimizes performance while minimizing overhead.
Best Practices for Index Maintenance
Maintaining indexes especially important for preserving the performance benefits they provide. Over time, as data in your database changes—through updates, inserts, and deletions—indexes can become fragmented or outdated. This degradation can lead to increased query execution times, negating the performance gains that indexes are supposed to offer. Therefore, adopting best practices for index maintenance is essential for ensuring that your database continues to operate efficiently.
Regularly Rebuild or Reorganize Indexes
One of the most effective ways to maintain the health of your indexes is through regular rebuilding or reorganizing. Rebuilding an index creates a new index structure from scratch, which can eliminate fragmentation and reorganize the data into a contiguous structure. Reorganizing an index, on the other hand, is a lighter operation that simply defragments the existing index structure without requiring additional disk space. The choice between these actions often depends on the level of fragmentation.
Most RDBMS platforms provide automated tools for monitoring index fragmentation. In SQL Server, for example, you can use the following SQL command to check the fragmentation level of your indexes:
SELECT OBJECT_NAME(object_id) AS TableName, name AS IndexName, index_id, avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL) WHERE avg_fragmentation_in_percent > 10; -- Threshold of 10%
If fragmentation is significant, you can rebuild the index using the following command:
ALTER INDEX idx_users_email ON users REBUILD;
Update Statistics
Another critical aspect of index maintenance is keeping statistics up to date. Database optimizers rely on statistical information about data distribution to make informed decisions regarding query execution plans. Outdated statistics can lead the optimizer to choose inefficient execution paths, resulting in slower query performance. Most RDBMS systems provide commands to update statistics automatically or manually.
In PostgreSQL, for example, you can update statistics for a specific table with the following command:
ANALYZE users;
This command recalibrates the statistics based on the current data distribution within the ‘users’ table, allowing the optimizer to make better decisions when executing queries.
Monitor Index Usage
Understanding how indexes are being used is another key aspect of maintenance. It is important to periodically review whether your indexes are still beneficial or if they have become redundant. Unused indexes can consume valuable resources and slow down write operations since every insert or update must also modify the indexes associated with a table.
In SQL Server, you can use the following query to find unused indexes:
SELECT OBJECT_NAME(s.object_id) AS TableName, i.name AS IndexName, s.user_seeks, s.user_scans, s.user_lookups, s.user_updates FROM sys.index_usage_stats AS s JOIN sys.indexes AS i ON s.object_id = i.object_id AND s.index_id = i.index_id WHERE i.is_primary_key = 0 AND i.is_unique = 0 ORDER BY s.user_seeks + s.user_scans + s.user_lookups DESC;
After identifying unused indexes, you can drop them with the following command:
DROP INDEX idx_users_email ON users;
Set a Maintenance Schedule
Establishing a regular maintenance schedule for your indexes can help automate these processes. Many RDBMS platforms enable you to create maintenance plans or scripts that can perform operations such as index rebuilding, updating statistics, and monitoring index usage at specified intervals. By integrating these operations into your database maintenance routine, you can proactively manage index health and ensure optimal performance.
Effective index maintenance involves a combination of rebuilding or reorganizing indexes, updating statistics, monitoring index usage, and implementing a structured maintenance schedule. By adhering to these best practices, you can help ensure that your database continues to perform efficiently, even as the underlying data changes over time.
Common Indexing Mistakes to Avoid
When working with indexes, it’s essential to be aware of common mistakes that can hinder their effectiveness and ultimately degrade database performance. Even the most well-intentioned database optimizations can backfire if these pitfalls are not carefully navigated.
1. Over-Indexing: One of the most frequent mistakes is creating too many indexes on a table. While indexes can significantly speed up read operations, they also introduce overhead for write operations such as INSERT, UPDATE, and DELETE. Each time a row is modified, all associated indexes must be updated, which can lead to significant performance degradation. It’s crucial to strike a balance between having sufficient indexes to optimize query performance and minimizing the overhead imposed on write operations.
2. Indexing Columns with Low Cardinality: Indexing columns that have low cardinality (i.e., a limited number of unique values) often provides little to no benefit. For instance, indexing a boolean column, which can only take two values (true or false), is unlikely to improve query performance significantly. Instead, focus on indexing columns that are frequently used in WHERE clauses and have a high degree of uniqueness to ensure that the index provides maximum benefit.
3. Neglecting Composite Indexes: Many developers overlook the power of composite indexes—indexes that cover multiple columns. When a query filters on multiple columns, a composite index can dramatically improve performance. Failure to implement composite indexes when appropriate can lead to suboptimal query execution plans and slower response times. For example, think a query:
SELECT * FROM orders WHERE customer_id = 42 AND order_date > '2023-01-01';
Creating a composite index on both columns would be beneficial:
CREATE INDEX idx_orders_customer_date ON orders(customer_id, order_date);
4. Ignoring Query Patterns: Another common mistake is failing to understand the specific query patterns that drive your application. Indexes should align with how data is accessed. For example, if you frequently query a ‘products’ table based on the ‘category_id’ and ‘price’, but your indexes are primarily focused on ‘product_name’, you are misaligning your indexing strategy. Regularly reviewing your query logs can help identify the most common queries and inform your indexing strategy accordingly.
5. Inadequate Monitoring and Maintenance: After creating indexes, many developers neglect ongoing monitoring and maintenance. Index fragmentation can occur over time, which can negatively impact performance. Regularly monitoring index usage and fragmentation levels and scheduling maintenance operations, such as index rebuilds or reorganizations, can prevent performance issues caused by degraded indexes.
6. Not Using Database Features: Most modern RDBMS come equipped with powerful tools to help manage indexes and optimize performance. Failing to utilize features such as automated index tuning, statistics gathering, and query optimization can lead to missed opportunities for performance improvements. Take advantage of these built-in features to keep your database performing optimally.
7. Not Testing Index Changes: Finally, it’s crucial to test the impact of any index changes in a controlled environment before applying them to production. Changes that seem beneficial in theory may not yield the desired results due to unforeseen interactions with existing queries or application logic. Using a staging environment to benchmark performance before and after index changes can help mitigate potential risks.
By being aware of these common indexing mistakes and taking proactive steps to avoid them, you can ensure that your indexing strategy remains effective and continues to provide the performance improvements you seek. Remember, the goal of indexing is not merely to add speed but to do so in a way that complements your overall database architecture and query patterns.
Case Studies: Real-World Index Optimization Examples
In the realm of database optimization, real-world case studies often serve as guiding lights, illuminating the paths taken by various organizations to improve their SQL query performance through effective index utilization. Let’s delve into a few illustrative examples that highlight the practical application of indexing strategies and their tangible benefits.
One prominent case involved an e-commerce website that experienced sluggish performance during peak shopping periods. The development team analyzed their SQL queries, discovering that searches on product names and categories were taking an excessive amount of time. The team decided to implement a composite index on the ‘products’ table, which included both the ‘category_id’ and ‘product_name’ columns. The SQL command executed was:
CREATE INDEX idx_products_category_name ON products(category_id, product_name);
This strategic move allowed the database engine to efficiently filter products based on category and subsequently narrow down the results by product name. Following the implementation of this index, query performance improved markedly, reducing response times from several seconds to milliseconds, even during high traffic.
In another case, a financial institution faced challenges with their reporting queries, particularly those that involved date ranges across massive datasets. The SQL queries were taking an exorbitant amount of time to execute, leading to delays in generating crucial reports. After conducting a thorough analysis, the team opted to create an index on the ‘transactions’ table aimed at enhancing the performance of the date filtering:
CREATE INDEX idx_transactions_date ON transactions(transaction_date);
This index significantly reduced the number of rows scanned during queries, enhancing the efficacy of date-based searches. The result was a dramatic cut in report generation times from minutes to seconds, empowering the organization to make timely decisions based on up-to-date data.
Moreover, a healthcare provider’s database faced performance issues due to extensive JOIN operations across multiple tables. The team recognized the need for optimized JOIN performance and created indexes on the foreign key columns involved in these joins. For example, they implemented:
CREATE INDEX idx_patients_doctor_id ON patients(doctor_id);
CREATE INDEX idx_appointments_patient_id ON appointments(patient_id);
This strategic indexing not only expedited the JOIN operations but also improved the overall response time of queries that involved retrieving patient and appointment records. Post-implementation, the healthcare provider noticed a substantial increase in the speed of their data retrieval processes, which was critical in providing prompt patient care.
Lastly, consider the case of a SaaS company that encountered performance degradation due to a rapidly growing user base. With frequent updates and queries executed on the ‘users’ table, the need for efficient management of user data became paramount. The team took a proactive approach by adopting a strategy of regularly monitoring index usage and implementing maintenance routines. They began by evaluating the index fragmentation levels using:
SELECT OBJECT_NAME(object_id) AS TableName, name AS IndexName, index_id, avg_fragmentation_in_percent FROM sys.dm_db_index_physical_stats(DB_ID(), NULL, NULL, NULL, NULL);
After identifying high fragmentation levels, they routinely rebuilt the indexes to maintain optimal performance. This continuous monitoring and maintenance ensured that their database could handle high read and write operations without significant performance dips.
These case studies illustrate the critical role that indexes play in optimizing SQL query performance across various industries. Through targeted indexing strategies, organizations can achieve remarkable improvements in data retrieval efficiency, which in turn supports better decision-making and enhances overall operational effectiveness.