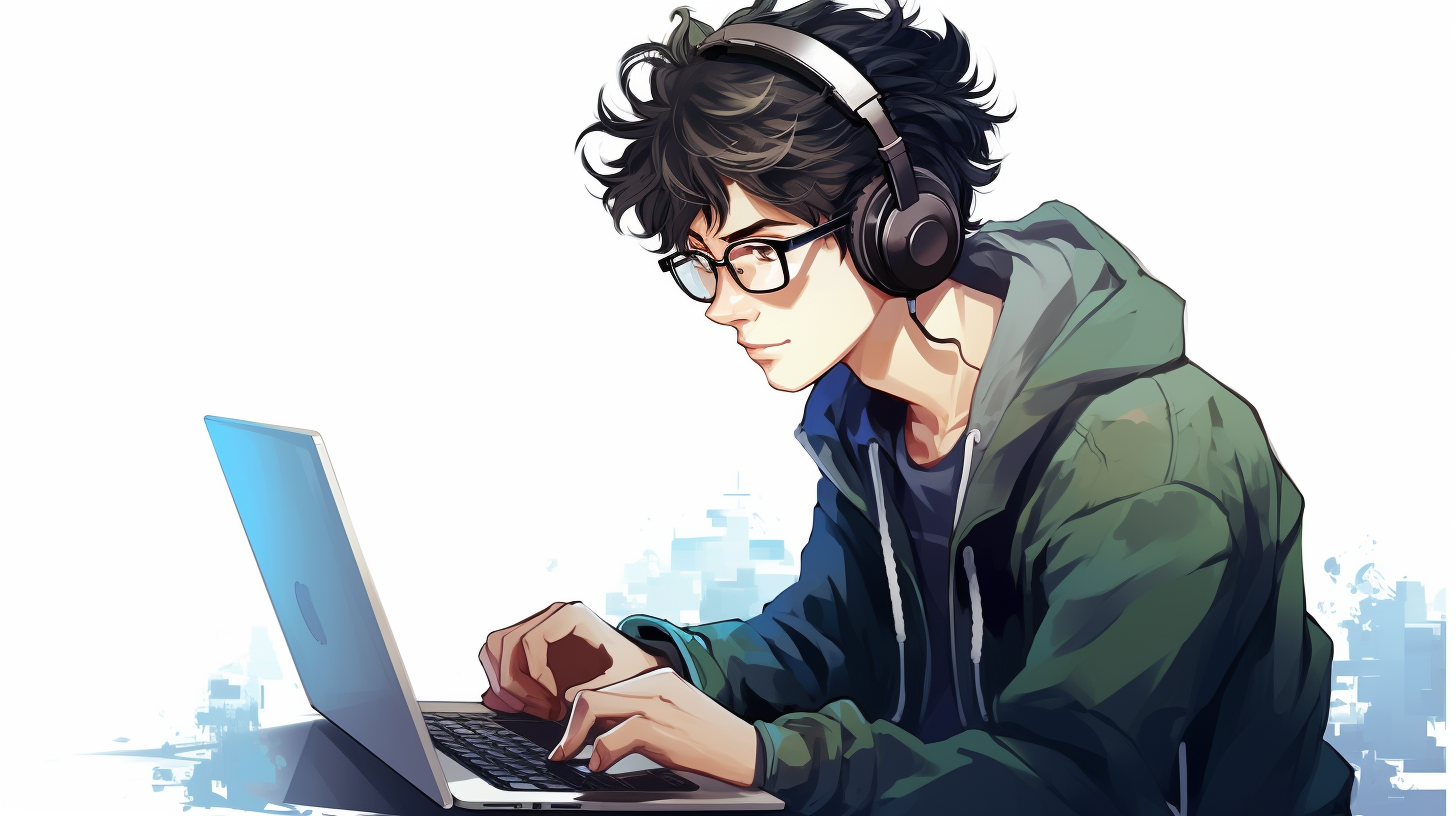
Parsing Logs with Bash
Log files serve as the backbone of system monitoring, capturing a detailed history of events that help diagnose issues and track system performance. Understanding the various formats of these log files is paramount for effective analysis. There are several common log file formats, each with its own structure and conventions. Familiarity with these formats will streamline your parsing efforts and enable you to extract meaningful insights from the noise.
One of the most prevalent formats is the syslog format, often used by Unix-like systems. Syslog entries typically consist of a timestamp, hostname, service name, and the actual log message. An example entry might look like this:
Jan 12 05:30:01 hostname service_name: Log message here
Another common format is the Apache log format, which records web server activity. Each entry captures the client’s IP address, the timestamp of the request, the HTTP method, the requested URL, and the response status. An example entry may appear as follows:
127.0.0.1 - - [12/Jan/2023:05:30:01 +0000] "GET /index.html HTTP/1.1" 200 2326
Log files can also be in JSON format, particularly in modern applications. This format is structured and easy to parse, as it uses key-value pairs. Here’s a brief example:
{ "timestamp": "2023-01-12T05:30:01Z", "level": "info", "message": "Service started." }
Additionally, some systems generate logs in CSV format, where entries are separated by commas, making it suitable for spreadsheet applications. A CSV log entry might look like this:
timestamp,level,message 2023-01-12 05:30:01,info,Service started.
Recognizing these formats helps in crafting the appropriate parsing strategies. Each format may require distinct approaches when employing Bash, especially when it comes to extracting relevant fields or filtering specific entries. For instance, the structured nature of JSON files can be easily handled with tools like jq
, while CSV files can be processed using awk
or cut
.
Ultimately, the initial step in effective log parsing is a solid grasp of these formats. With this knowledge in hand, you can deploy Bash’s powerful text processing capabilities to sift through the clutter and pinpoint the data that matters most.
Basic Bash Commands for Log Analysis
When diving into the world of log analysis with Bash, mastering a few fundamental commands can significantly enhance your ability to extract pertinent information from log files. Bash provides a rich set of built-in commands that allow you to manipulate and analyze text data effectively.
One of the most frequently used commands is cat, which concatenates and displays file contents. This command allows you to quickly view the contents of a log file:
cat /var/log/syslog
While cat is great for quick views, it can become unwieldy with large files. To make the output more manageable, you can use less or more, which allow you to scroll through the file:
less /var/log/syslog
Another useful command is grep, which searches for specific patterns within text. This command excels at filtering log entries based on keywords, making it indispensable for log analysis. For instance, if you want to find all occurrences of the word “error” in a log file, you can execute:
grep "error" /var/log/syslog
To improve your search, grep offers options like -i for case-insensitive searches and -r for recursive searches through directories:
grep -i "error" /var/log/*
Additionally, the awk command is a powerful tool for processing and analyzing structured data. It allows you to extract specific fields from log entries, which is especially useful for log formats like CSV or space-separated values. For example, if you want to extract the timestamp and message from a custom log file, you can use:
awk '{print $1, $2, $3}' custom_log_file.log
The tail command is handy for monitoring logs in real-time, particularly with the -f option that outputs new entries as they are added. That’s particularly useful for tracking live events or errors:
tail -f /var/log/syslog
To summarize, using these basic Bash commands—cat, less, grep, awk, and tail—will empower you to sift through log files with precision and efficiency. As you become adept at combining these commands through pipes and redirections, you will unlock the full potential of Bash for log analysis, allowing you to focus on the critical data that informs your system’s performance and stability.
Using Regular Expressions for Pattern Matching
Regular expressions (regex) are a powerful tool in the Bash arsenal for pattern matching and text processing. They allow you to define complex search patterns that can match strings, extract data, and manipulate text in ways that simple string searches cannot. Understanding how to utilize regular expressions effectively can elevate your log parsing capabilities to new heights.
Bash provides tight integration with tools like grep
, sed
, and awk
, all of which support regex. The basic syntax of a regular expression involves constructing patterns using literal characters and special symbols. For example, the dot (.) matches any single character, while the asterisk (*) matches zero or more occurrences of the preceding element. To search for any log entry that contains the word “failed” followed by any characters, you can leverage the following grep
command:
grep "failed.*" /var/log/syslog
This command will return lines from the syslog that contain the term “failed” anywhere in the entry, followed by any subsequent characters. The power of regex extends further with the use of anchors like caret (^) and dollar sign ($), which denote the start and end of a line, respectively. For instance, if you want to find lines that start with a specific timestamp format, you could use:
grep "^Jan 12" /var/log/syslog
In this example, only lines beginning with “Jan 12” will be matched. Additionally, regex character classes allow you to specify a set of characters to match. For example, using brackets to match any digit, you might search for entries that indicate an error code:
grep "[0-9][0-9][0-9]" /var/log/syslog
This command captures any three-digit number, which may represent error codes in your logs.
For more complex scenarios, sed
can be employed not only for searching but also for replacing text based on regex patterns. Suppose you want to anonymize IP addresses in your logs. Using sed
, you can apply a regex pattern to replace all occurrences of an IP address with a placeholder:
sed 's/[0-9]{1,3}(.[0-9]{1,3}){3}/XXX.XXX.XXX.XXX/g' access.log
This command identifies IP addresses in the format of four octets (0-255) and replaces them with “XXX.XXX.XXX.XXX” to maintain privacy in the log output.
Another tool at your disposal is awk
, which also supports regex for pattern matching within fields. For example, you can filter rows in a CSV log file where the level is “error” like this:
awk -F, '$2 ~ /error/' log.csv
This command sets the field separator to a comma and matches lines where the second field (level) contains the word “error”.
Using the power of regular expressions in Bash allows you to perform intricate searches and transformations on your log data, streamlining the process of extracting relevant information. By mastering regex, you can refine your log analysis workflows, allowing you to quickly pinpoint issues and gain deeper insights into system behavior.
Filtering and Extracting Relevant Data
Once you’re features an solid understanding of log file formats and basic Bash commands, the next step is to filter and extract the relevant data. This stage is critical as it allows you to hone in on specific entries that matter most to your analysis, ultimately leading to actionable insights. Filtering involves narrowing down log entries based on certain criteria, while extraction focuses on pulling out specific pieces of information from those entries.
At the heart of this process are commands like grep
and awk
, which offer powerful capabilities for filtering and extracting data based on patterns and field specifications.
To filter log entries effectively, you might start with grep
to identify lines of interest. For instance, suppose you are monitoring an application and want to find all occurrences of “warning” in your logs. You can run:
grep "warning" /var/log/app.log
This command quickly displays all lines that contain the word “warning,” which will allow you to sift through the noise. However, the functionality of grep
extends beyond simple searches. You can combine it with other commands for more complex queries. For example, if you want to exclude certain terms, you can use the -v
option:
grep -v "info" /var/log/app.log | grep "warning"
This line retrieves all “warning” entries while filtering out any “info” logs, giving you a cleaner, more relevant output.
Moving on to extraction, awk
shines when you need to pull specific fields from structured log data. Let’s say you have an Apache log file and you want to extract only the IP address and status code from each entry. You would use:
awk '{print $1, $9}' access.log
In this command, $1
denotes the first field (the IP address) and $9
refers to the ninth field (the status code). This approach is especially effective in CSV logs, where you can specify the delimiter using the -F
option:
awk -F, '{print $1, $3}' log.csv
This extracts the first and third fields, assuming they contain the timestamp and message respectively.
Combining filtering and extraction boosts your efficiency significantly. For instance, if you want to find all entries related to “failed login attempts” in a log file and only extract the timestamp and the corresponding message, your command might look like this:
grep "failed login" auth.log | awk '{print $1, $2, $3, $9}'
In this example, grep
isolates the relevant log entries, while awk
extracts the specific fields that provide you with actionable insights.
Furthermore, you might find yourself needing to format the output for better readability. For instance, if you want to format the extracted details into a more structured format, you can use awk
like this:
grep "failed login" auth.log | awk '{printf "Date: %s %s, Message: %sn", $1, $2, $9}'
This command will output the date and message in a clean format, making it easier to scan through the results.
As you delve deeper into log analysis, the ability to efficiently filter and extract relevant data will prove invaluable. By mastering the art of using grep
and awk
together, you can tailor your log analysis workflows to suit your specific needs, ultimately so that you can focus on the issues that require your immediate attention.
Automating Log Parsing with Bash Scripts
#!/bin/bash # Example log parsing script LOG_FILE="/var/log/app.log" # Filter for warning entries and extract relevant fields grep "warning" $LOG_FILE | awk '{print $1, $2, $9}'
Automating log parsing with Bash scripts brings efficiency and consistency to your log analysis workflows. By wrapping the commands you’ve learned into reusable scripts, you can streamline repetitive tasks and ensure that critical information is processed consistently, without the need for manual intervention.
To begin, consider a simple Bash script that automates the task of filtering and extracting data from a log file. Here’s a basic structure for a log parsing script:
#!/bin/bash # Define the log file to parse LOG_FILE="/var/log/syslog" # Check if the log file exists if [[ ! -f $LOG_FILE ]]; then echo "Log file does not exist!" exit 1 fi # Process the log file to find errors and output relevant fields grep "error" $LOG_FILE | awk '{print $1, $2, $3, $9}' > errors.log echo "Extracted error logs to errors.log"
In this script, we first define the log file to be parsed. It’s essential to include a check to confirm that the file exists, which helps prevent errors when the script runs. The core functionality utilizes grep
to filter entries that contain the word “error,” and then awk
extracts specific fields (in this case, the date, time, and message) to an output file called errors.log
.
Once you have the basic script working, you can expand its functionality by adding command-line arguments. This allows users to specify different log files or search terms at runtime. For instance, you can modify the script to accept a search pattern and a log file as inputs:
#!/bin/bash # Check for required arguments if [ "$#" -ne 2 ]; then echo "Usage: $0 <search_pattern> <log_file>" exit 1 fi SEARCH_PATTERN=$1 LOG_FILE=$2 # Check if the log file exists if [[ ! -f $LOG_FILE ]]; then echo "Log file does not exist!" exit 1 fi # Process the log file grep "$SEARCH_PATTERN" $LOG_FILE | awk '{print $1, $2, $3, $9}' > output.log echo "Extracted logs containing '$SEARCH_PATTERN' to output.log"
In this enhanced version, the script checks for the correct number of arguments and assigns them to variables. This level of flexibility makes the script far more powerful, as it can be reused for different scenarios without hardcoding values.
Moreover, you can schedule your log parsing scripts using cron
jobs. This allows for automated, periodic analysis of log files without manual oversight. For example, if you want to run your log parsing script every hour, you can add a cron
entry like this:
0 * * * * /path/to/your/script.sh "error" "/var/log/syslog"
This entry ensures that the script runs at the start of every hour, checking for new errors and storing the results, allowing your log analysis to keep pace with real-time data.
Finally, incorporating error handling and logging within your scripts will enhance their robustness. You could implement logging for script successes and failures, enabling you to track the performance of your automated tasks more effectively:
#!/bin/bash LOG_FILE="/var/log/app.log" OUTPUT_FILE="output.log" # Error log function log_error() { echo "$(date +"%Y-%m-%d %H:%M:%S") - ERROR: $1" >> error.log } # Main processing function process_logs() { if [[ ! -f $LOG_FILE ]]; then log_error "Log file does not exist!" exit 1 fi grep "error" $LOG_FILE | awk '{print $1, $2, $3, $9}' > $OUTPUT_FILE echo "Extracted error logs to $OUTPUT_FILE" } process_logs
In this script, an error logging function captures any issues encountered during execution and appends them to an error.log
file. This practice is invaluable for diagnosing problems in automation scripts and maintaining a clear overview of their operational status.
By employing these automation techniques, you not only enhance your efficiency but also create a framework for consistent log analysis that adapts to your ongoing needs. With a few lines of Bash code, you can transform a tedious manual process into a reliable and repeatable workflow, freeing you to focus on analysis rather than data extraction.