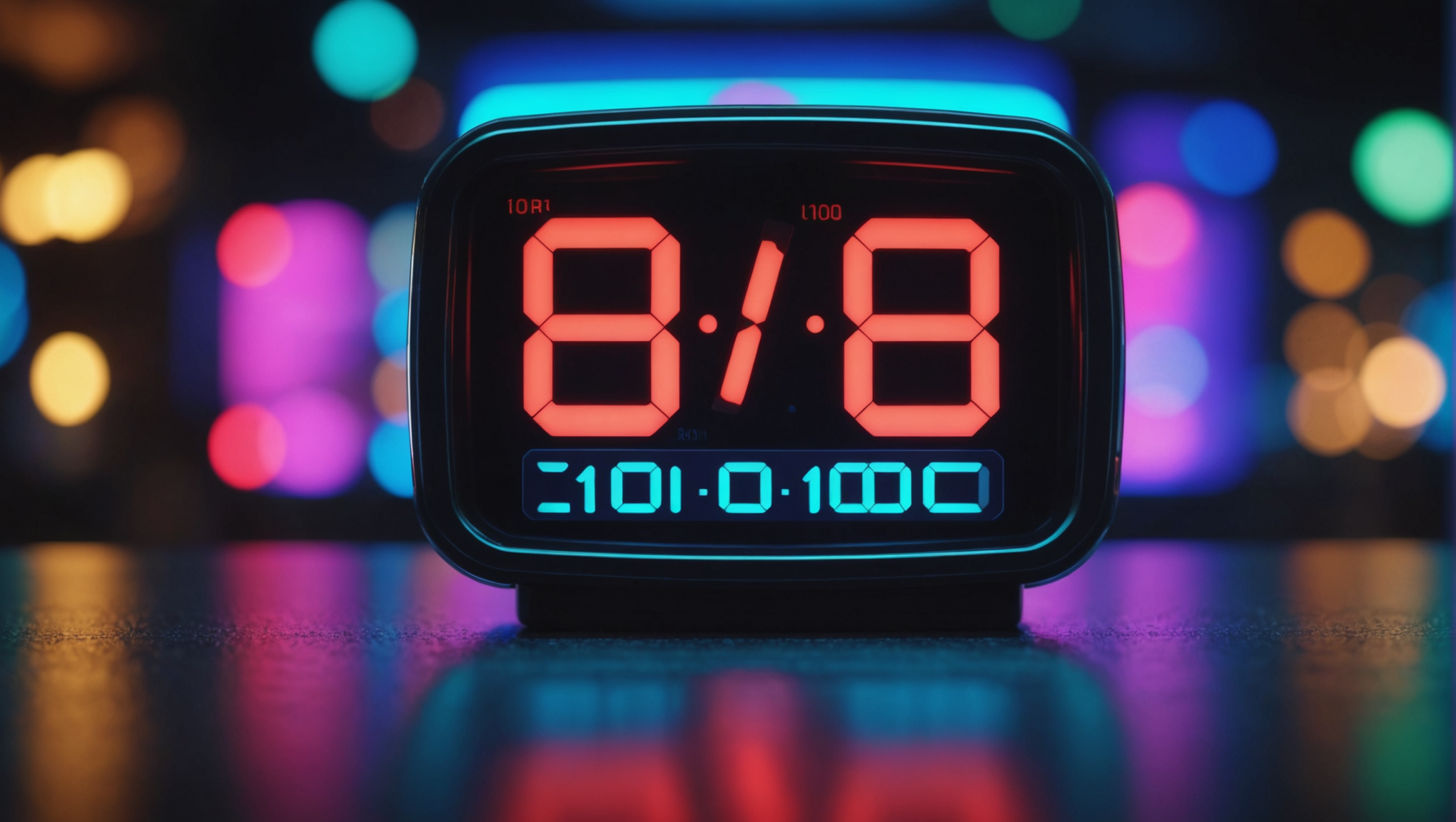
Property Observers in Swift
In Swift, property observers provide a powerful mechanism to observe and respond to changes in property values. They enable developers to execute custom code before or after a property’s value is set, offering a layer of control that can be invaluable in various scenarios. This functionality is particularly useful in situations where you need to perform additional validation, trigger UI updates, or synchronize data with other components of your application.
Property observers are defined directly within a property declaration. Swift supports two types of property observers: willSet and didSet. The willSet
observer is called just before the value of the property is set, enabling you to access the new value before it is applied. Conversely, the didSet
observer is called immediately after the value is set, giving you access to the old value and so that you can perform actions based on the change.
To illustrate the concept, ponder the following example of a simple class that utilizes property observers:
class ScoreManager { var score: Int = 0 { willSet { print("The score is about to change from (score) to (newValue)") } didSet { print("The score has changed from (oldValue) to (score)") } } } let manager = ScoreManager() manager.score = 10 manager.score = 20
In this example, the ScoreManager
class has a property called score
. Whenever the value of score
is about to change, the willSet
observer triggers and prints a message indicating the impending change. After the change, the didSet
observer executes, printing the old and new values. This demonstrates how property observers can provide a clear and concise way to monitor property changes and execute code in response.
Property observers are not limited to stored properties; they can also be used with computed properties, but only in specific cases where you are defining a property that has a getter and a setter. However, it’s important to note that property observers cannot be used on lazy properties, as their initialization is deferred until they’re first accessed, which does not align with the timing requirements of property observers.
Types of Property Observers: WillSet and DidSet
The two types of property observers in Swift, willSet and didSet, serve distinct purposes and are utilized for different stages of property value changes. Understanding their specific functionalities can enhance how you manage state and behavior in your applications.
willSet is invoked just before the property value is changed. This observer gives you access to the new value this is about to be assigned, which you can reference using a special implicit parameter named newValue
. This feature is particularly useful when you want to perform actions based on the imminent change of a property, such as validating the new value or preparing other parts of your application to respond to this change.
On the other hand, didSet is called immediately after the property value has been changed. It provides access to the old value of the property through the implicit parameter named oldValue
. This is perfect for tasks that need to react to the completed change, such as updating user interfaces or triggering further processes that depend on the new property value.
To clarify the differences further, consider the following refined code example:
class TemperatureManager { var temperature: Double = 0.0 { willSet { print("Preparing to set temperature to (newValue)°C") } didSet { print("Temperature changed from (oldValue)°C to (temperature)°C") if temperature > 100.0 { print("Warning: Temperature exceeds safe limit!") } } } } let tempManager = TemperatureManager() tempManager.temperature = 25.0 tempManager.temperature = 105.0
In this example, the TemperatureManager
class includes a temperature
property with both willSet
and didSet
observers. When the temperature is about to change, willSet
outputs a message indicating the new temperature. After the change, didSet
provides a notification of the old and new temperature values and checks if the new temperature exceeds a safe limit, thus demonstrating how both observers work together to manage the property effectively.
Implementing these observers helps you maintain a clear flow of data and logic in your applications. By using the capabilities of willSet and didSet, you can create responsive and robust data models that adjust to changes in their properties naturally and efficiently.
Implementing Property Observers in Your Code
Implementing property observers in your Swift code is simpler and can significantly enhance the interactivity and responsiveness of your data models. To get started, you simply define the observers within the property declaration, which allows you to customize behavior for when the property’s value changes.
Here’s a deeper dive into practical implementation. Consider a scenario where you’re managing user settings for a game. You might want to adjust the gameplay dynamically based on user preferences. We can model this with a property observer:
class GameSettings { var soundEnabled: Bool = true { willSet { print("Sound is about to be (newValue ? "enabled" : "disabled")") } didSet { if soundEnabled { print("Sound has been enabled.") // Code to enable sound in the game } else { print("Sound has been disabled.") // Code to disable sound in the game } } } } let settings = GameSettings() settings.soundEnabled = false settings.soundEnabled = true
In this example, the GameSettings class has a property called soundEnabled. The willSet observer allows us to notify the user of the impending change, while the didSet observer contains the logic to actually enable or disable sound in the game. This encapsulates the behavior directly alongside the property definition, promoting maintainability and clarity.
Another common use case is updating the state of a user interface when a property changes. Suppose you have a property that represents the player’s score in a game:
class Player { var score: Int = 0 { willSet { print("Score is about to change from (score) to (newValue)") } didSet { updateUI() } } private func updateUI() { print("Player's score is now (score)") // Code to refresh the UI with the new score } } let player = Player() player.score = 50 player.score = 100
Here, the Player class uses the score property to keep track of the player’s current score. The willSet observer informs us of the upcoming change, while the didSet observer immediately calls a method to update the user interface—ensuring that the UI stays in sync with the player’s score.
Overall, implementing property observers in Swift not only streamlines the state management within your classes but also provides a clear, expressive way to handle changes dynamically. This can lead to more responsive applications where the user interface and underlying logic are tightly integrated, all while maintaining clean and readable code.
Use Cases for Property Observers
Property observers can be incredibly useful in a variety of scenarios, enhancing the interactivity and responsiveness of your applications. Let’s explore some practical use cases where implementing property observers can significantly improve your code.
One of the most common use cases is managing application state based on user preferences. For example, consider a settings manager for a media application that needs to adjust its behavior when the user modifies preferences such as volume or playback speed. Using property observers can provide immediate feedback and trigger necessary updates without cluttering your main logic with checks scattered across your codebase.
class MediaSettings { var volume: Float = 0.5 { willSet { print("Volume will change from (volume) to (newValue)") } didSet { if volume > 1.0 { volume = 1.0 print("Volume set to maximum limit.") } print("Volume is now (volume)") // Code to adjust media player volume } } } let settings = MediaSettings() settings.volume = 0.7 settings.volume = 1.2
In this example, the `MediaSettings` class manages the volume level. The `willSet` observer informs the developer about the impending change, while the `didSet` observer ensures that the volume does not exceed its maximum limit, automatically adjusting it and maintaining a consistent state.
Another compelling use case is in data synchronization between different components of your application. Imagine a scenario where you have a model representing a user’s profile. Changes to specific attributes should trigger updates to related views or data structures. Here, property observers can streamline this synchronization process.
class UserProfile { var username: String = "" { didSet { print("Username updated to: (username)") notifyProfileUpdate() } } private func notifyProfileUpdate() { // Code to update UI or notify other components about the username change } } let userProfile = UserProfile() userProfile.username = "NewUser123"
In this `UserProfile` example, whenever the username changes, the `didSet` observer triggers a method to notify other parts of the application that depend on the username. This keeps your application dynamic and responsive to user input.
Property observers also play an important role in enforcing validation rules. For instance, when working with input fields in a form, you might want to ensure that a user’s age is always a positive number. Implementing property observers allows you to enforce these rules seamlessly.
class User { var age: Int = 0 { willSet { print("Attempting to set age to (newValue)") if newValue < 0 { print("Invalid age: Cannot be negative.") } } didSet { if age < 0 { age = 0 print("Age cannot be negative. Reset to 0.") } } } } let user = User() user.age = 25 user.age = -5
In the `User` class, both observers work in tandem to ensure that the age value is always valid. The `willSet` observer allows for immediate validation before the change occurs, while the `didSet` observer corrects invalid states if necessary, maintaining the integrity of the data model.
Finally, property observers are invaluable in scenarios where resources need to be allocated or released based on property changes. For instance, in a game, you might want to load specific assets when a player achieves a new level:
class GameLevel { var level: Int = 1 { didSet { print("Level changed from (oldValue) to (level)") loadAssetsForLevel(level) } } private func loadAssetsForLevel(_ level: Int) { // Code to load game assets for the current level } } let game = GameLevel() game.level = 2
In this `GameLevel` class, the `didSet` observer allows you to react immediately to a change in the level and handle resource loading efficiently. This encapsulates the logic elegantly, ensuring that your game responds dynamically to player progress.
By using property observers, developers can create more maintainable and responsive applications. The aforementioned scenarios demonstrate how property observers contribute to cleaner code and a better user experience by managing state transitions effectively. Whether it’s managing user settings, synchronizing data, enforcing validation, or allocating resources, property observers offer a robust solution for a range of programming challenges.
Limitations of Property Observers
While property observers in Swift provide a powerful tool for monitoring and responding to property changes, they come with certain limitations that developers need to be aware of. Understanding these constraints very important for making informed design choices in your applications.
Firstly, property observers can only be used with stored properties, not with computed properties. This means that if you need to perform actions based on the value of a property this is derived from other properties, you will not be able to attach observers directly to that computed property. Instead, you would need to manage the state changes manually or ponder restructuring your data model.
Another significant limitation is that property observers are not available for lazy properties. Lazy properties are initialized only the first time they are accessed, which means that the timing of their initialization does not align with the purpose of property observers. If you attempt to add observers to a lazy property, you will encounter a compilation error, as the language does not support this feature.
Additionally, property observers cannot be used with properties whose types are defined as optional. When a property is declared as optional, Swift does not allow you to define observers because the explicit setting of the value is not guaranteed. You must handle optionality using different patterns, such as using a computed property or handling states explicitly through methods.
Moreover, while property observers allow for notifications on value changes, they do not provide a simpler means to prevent recursive updates. If the code inside a didSet observer modifies the property being observed, it can lead to unexpected behaviors and infinite loops. Developers must take care to avoid such situations, ensuring that the logic within these observers does not inadvertently trigger further updates.
Lastly, when property observers are used in conjunction with reference types, the implications on memory management should be considered. Observers can create strong references to the properties they monitor, which could lead to retain cycles if not managed properly, especially in scenarios involving closures.
To illustrate some of these limitations, think the following examples:
class User { var name: String { didSet { print("Name changed to (name)") } } init(name: String) { self.name = name } } // This will not compile // class User { // lazy var age: Int = 0 { // didSet { // print("Age changed to (age)") // } // } // } // This will not compile // class User { // var age: Int? { // didSet { // print("Age changed to (age)") // } // } // } let user = User(name: "Alice") user.name = "Bob" // Attempting to use observers on lazy or optional properties will result in compilation errors.
While property observers enhance the responsiveness and interactivity of Swift applications, developers need to navigate their limitations carefully. By understanding where property observers can and cannot be applied, you can design more robust and effective data models while avoiding common pitfalls associated with misuse.
Best Practices for Using Property Observers
When using property observers in Swift, adhering to best practices can significantly enhance code maintainability, clarity, and performance. Here are several recommendations to ensure you leverage property observers effectively in your applications.
First and foremost, keep the logic within your property observers concise. Property observers should primarily focus on responding to changes rather than executing complex business logic. Overly complicated code within these observers can lead to difficulties in debugging and understanding the flow of your application. Aim to offload any non-trivial logic to separate methods or functions.
For instance, rather than including extensive conditionals within a `didSet` observer, think refactoring the logic into a dedicated method:
class UserProfile { var age: Int = 0 { didSet { validateAge() } } private func validateAge() { if age < 0 { age = 0 print("Age cannot be negative. Reset to 0.") } } }
This structure not only makes your property observer cleaner and easier to read, but it also simplifies unit testing for the validation logic.
Another best practice is to avoid side effects within your observers. Side effects can lead to unpredictable behavior, especially when the same property is observed in multiple places. Instead, ensure that your observers are purely responsive. For example, use `didSet` to notify other components or trigger events rather than modifying other properties directly:
class Game { var score: Int = 0 { didSet { notifyScoreChange() } } private func notifyScoreChange() { print("Score updated to (score)") // Notify observers or update UI here } }
Additionally, consider the potential performance implications of using property observers. Since observers are called every time the property is changed, using them excessively in performance-critical areas can lead to overhead. Profile your application to ensure that the use of property observers does not introduce bottlenecks, especially in tight loops or frequently called methods.
Furthermore, take care when using property observers with reference types. If an observer captures `self` within a closure, it can create strong reference cycles. To avoid this, use weak references when necessary, particularly in cases where the observer may outlive the object it observes.
class ViewModel { var name: String = "" { didSet { updateUI() } } private func updateUI() { // Capture self weakly to prevent retain cycles let updateUIClosure: () -> Void = { [weak self] in guard let self = self else { return } // Update UI using self.name } updateUIClosure() } }
Finally, document your property observers clearly. Explain the purpose of each observer, any side effects it may have, and the expected behavior. This documentation will be invaluable for future maintainers of the codebase, ensuring that the intention behind the observers is clear and understood.
By adhering to these best practices, you can effectively use property observers to create responsive, maintainable, and efficient Swift applications. Such an approach not only enhances code quality but also improves the overall developer experience, making it easier to navigate and extend your code in the future.