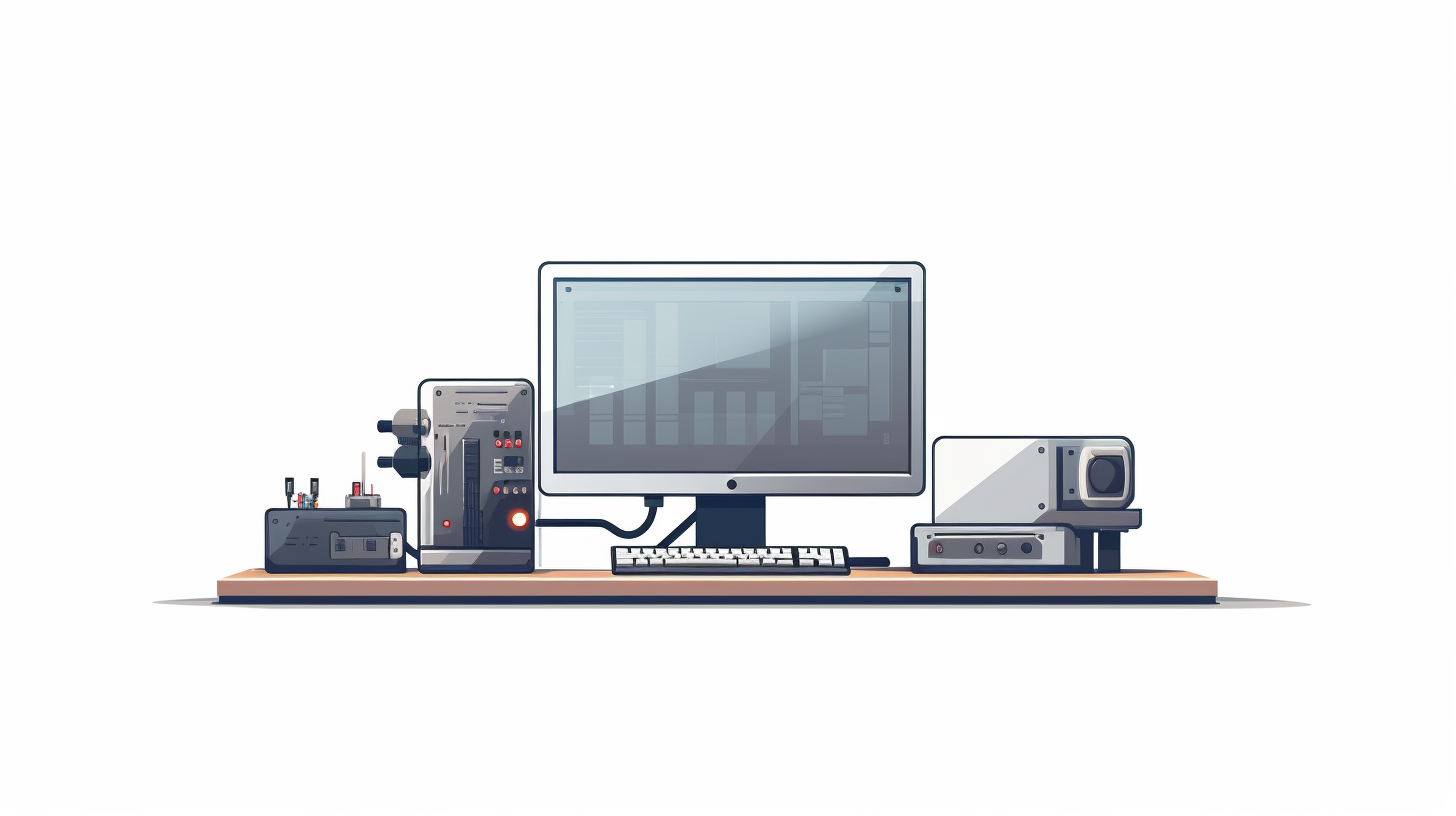
Protocols in Swift
In Swift, protocols serve as a powerful tool for defining a blueprint of methods, properties, and other requirements that suit a particular task or functionality. They play a critical role in achieving polymorphism and allowing for a high degree of flexibility in your code. Think of a protocol as a contract that any conforming type agrees to adhere to. This not only promotes code reusability but also provides a means to define shared interfaces across disparate types.
Protocols can be adopted by classes, structures, and enumerations, enabling a diverse range of types to implement shared functionality. By using protocols, developers can create a more modular codebase, making it easier to manage complexity as systems grow. For instance, ponder a scenario where you have different types of vehicles, each with its own implementation of how to start. Here, you can define a protocol that specifies a `start` method.
protocol Startable { func start() }
Now, any type that conforms to this protocol must implement the `start()` method. Let’s see how we can have different vehicles conform to this protocol, each providing its own implementation.
class Car: Startable { func start() { print("Car engine starting...") } } class Motorcycle: Startable { func start() { print("Motorcycle revving up...") } }
In this way, the `Car` and `Motorcycle` classes both adhere to the `Startable` protocol, ensuring that they provide their own versions of the `start` method. When you create instances of these classes and call the `start()` method, the unique behavior of each type is executed.
let myCar = Car() myCar.start() // Outputs: Car engine starting... let myMotorcycle = Motorcycle() myMotorcycle.start() // Outputs: Motorcycle revving up...
This example exemplifies how protocols facilitate polymorphism. You can write functions that operate on protocol types, allowing for functions to accept any object that conforms to the `Startable` protocol, regardless of its underlying type.
func startVehicle(_ vehicle: Startable) { vehicle.start() } startVehicle(myCar) // Outputs: Car engine starting... startVehicle(myMotorcycle) // Outputs: Motorcycle revving up...
With this approach, you can abstract away the specifics of each vehicle type and work with them in a uniform manner. The elegance of protocols in Swift lies not just in their ability to define interfaces but also in their capacity to enable a clean, organized architecture that fosters collaboration and code maintainability.
Defining and Implementing Protocols
Defining a protocol is a simpler process, but implementing it can introduce some nuances worth exploring. When a type adopts a protocol, it’s essential to ensure that all requirements specified in the protocol are met. This can include methods, properties, and even initializers. The implementation of a protocol is a commitment to uphold its contract; neglecting to satisfy these requirements will result in a compile-time error. This mechanism not only enforces the structure of your code but also adds a layer of safety.
Here’s a more detailed look at how to define a protocol with various requirements:
protocol Vehicle { var numberOfWheels: Int { get } func start() func stop() }
In this example, the `Vehicle` protocol specifies a property `numberOfWheels` and two methods: `start()` and `stop()`. Any type that conforms to this protocol will need to provide an implementation for these requirements.
Now, let’s see how different types can conform to the `Vehicle` protocol by providing implementations for the required methods and properties:
class Car: Vehicle { var numberOfWheels: Int { return 4 } func start() { print("Car engine starting...") } func stop() { print("Car engine stopping...") } } class Bicycle: Vehicle { var numberOfWheels: Int { return 2 } func start() { print("Bicycle is ready to ride...") } func stop() { print("Bicycle is stopping...") } }
In this case, both `Car` and `Bicycle` conform to the `Vehicle` protocol by implementing the required `numberOfWheels` property, as well as the `start()` and `stop()` methods. Each type provides its unique behavior while fulfilling the obligations set by the protocol.
When working with these types, you can take advantage of polymorphism to treat them uniformly. For instance, you can create an array of `Vehicle` types and iterate through them, invoking the same methods:
let vehicles: [Vehicle] = [Car(), Bicycle()] for vehicle in vehicles { print("Number of wheels: (vehicle.numberOfWheels)") vehicle.start() vehicle.stop() }
This code snippet demonstrates how the protocol allows for a heterogeneous collection of types that can be treated uniformly. The `for` loop iterates through each vehicle, printing the number of wheels and invoking the `start()` and `stop()` methods, showcasing the power of abstraction and code reuse that protocols enable.
Implementing protocols also allows you to take advantage of protocol extensions, which provide default implementations of methods and properties. This feature can be particularly useful for reducing code duplication across multiple conforming types. For instance, you might want to provide a default implementation for the `stop()` method that can be shared among all vehicles:
extension Vehicle { func stop() { print("Vehicle is stopping...") } }
With this extension, any type that conforms to the `Vehicle` protocol will automatically inherit the default implementation of `stop()`, unless it provides its own. This capability not only fosters code reuse but also allows for a more streamlined implementation process, reducing the overhead of implementing common behavior across various types.
Protocol Inheritance and Composition
Protocol inheritance and composition in Swift adds an elegant layer to how types can share and extend functionality. This allows protocols to inherit from other protocols, enabling a hierarchy that can define shared requirements across multiple protocols. The concept of protocol composition lets you combine multiple protocols to create a more complex type that adheres to several interfaces concurrently.
When defining a protocol that inherits from another protocol, the new protocol will inherit all the requirements of its superclass protocol. That is akin to class inheritance but specifically tailored for protocols. Let’s illustrate this with an example.
protocol Drivable { func drive() } protocol ElectricVehicle: Drivable { var batteryLevel: Int { get } func charge() }
In this case, the `ElectricVehicle` protocol inherits from the `Drivable` protocol, which means any type conforming to `ElectricVehicle` must implement both the `drive()` method as well as satisfy the requirements of `batteryLevel` and `charge()` method. Let’s see how a specific type can conform to this protocol.
class Tesla: ElectricVehicle { var batteryLevel: Int = 100 func drive() { print("Driving the Tesla...") } func charge() { print("Charging the Tesla...") } }
Here, the `Tesla` class conforms to the `ElectricVehicle` protocol, which inherently requires an implementation of the `drive()` method from `Drivable`. Thus, the `Tesla` class must provide concrete implementations for both `drive()` and `charge()` methods, along with the `batteryLevel` property.
When you use the `Tesla` instance, you can utilize it wherever an `ElectricVehicle` or `Drivable` is expected:
func testDrive(vehicle: Drivable) { vehicle.drive() } let myTesla = Tesla() testDrive(vehicle: myTesla) // Outputs: Driving the Tesla...
On the other hand, protocol composition allows you to define a new protocol that requires adherence to multiple protocols at the same time. That’s done using the `&` operator. Think this scenario:
protocol Flyable { func fly() } func operate(vehicle: Drivable & Flyable) { vehicle.drive() vehicle.fly() } class FlyingCar: Drivable, Flyable { func drive() { print("Driving the flying car...") } func fly() { print("Flying the flying car...") } }
In this example, the `operate` function accepts any type that conforms to both `Drivable` and `Flyable`, allowing for a versatile function that can drive and fly vehicles. The `FlyingCar` class provides implementations for both protocols, showcasing how protocol composition can facilitate more complex behaviors in a consistent manner.
let myFlyingCar = FlyingCar() operate(vehicle: myFlyingCar) // Outputs: // Driving the flying car... // Flying the flying car...
By employing protocol inheritance and composition, you can craft flexible and reusable code in Swift this is both powerful and maintainable. It allows developers to create a hierarchy of protocols, facilitating a more organized approach to defining shared functionalities across disparate types, while also enabling the combination of behaviors in a concise and expressive manner.
Using Protocols with Generics and Associated Types
protocol JSONRepresentable { func toJSON() -> String } protocol User: JSONRepresentable { var username: String { get } var email: String { get } } extension User { func toJSON() -> String { return """ { "username": "(username)", "email": "(email)" } """ } } struct Admin: User { var username: String var email: String // Additional properties specific to Admin var adminLevel: Int func describe() -> String { return "Admin (username) with level (adminLevel)" } } struct RegularUser: User { var username: String var email: String // Additional properties specific to a regular user var subscriptionType: String func describe() -> String { return "Regular user (username) with a (subscriptionType) subscription" } } let admin = Admin(username: "AdminUser", email: "[email protected]", adminLevel: 1) let regularUser = RegularUser(username: "RegularUser", email: "[email protected]", subscriptionType: "Basic") print(admin.toJSON()) print(regularUser.toJSON())
In the example above, we define a `JSONRepresentable` protocol that requires a `toJSON()` method, which converts conforming types into a JSON format. The `User` protocol inherits from `JSONRepresentable`, thereby requiring the `username` and `email` properties, along with the `toJSON()` method.
By using protocol extensions, we provide a default implementation of `toJSON()` for the `User` protocol. This means any type that conforms to `User` automatically gains the capability to convert itself to JSON. The `Admin` and `RegularUser` structs then conform to `User`, implementing required properties and adding their unique behavior.
When instances of `Admin` and `RegularUser` are created, they can utilize the `toJSON()` method seamlessly, showcasing how protocols can be extended and provide default behavior while still allowing for specialization in individual types.
The power of using protocols with generics and associated types becomes apparent when creating more complex data structures. Consider a scenario where you need to create a generic repository that can store various types of users and manage their persistence.
protocol Storable { associatedtype Item func save(item: Item) func fetch() -> Item? } struct UserRepository: Storable { private var users: [T] = [] func save(item: T) { users.append(item) } func fetch() -> T? { return users.first } } let userRepository = UserRepository() userRepository.save(item: admin) if let fetchedAdmin = userRepository.fetch() { print(fetchedAdmin.toJSON()) // Outputs the JSON representation of the admin }
Here, the `Storable` protocol introduces an associated type, allowing us to define a generic type `Item` that can vary based on the conforming type. The `UserRepository` struct implements the `Storable` protocol and can operate with any type that conforms to `User`, providing a flexible storage mechanism.
This construction showcases the synergy between protocols, generics, and associated types, enabling developers to create abstractions that are not only powerful but also adaptable to various use cases. By defining clear contracts through protocols and using the type safety of Swift’s generics, you can maintain a clean architecture while enhancing the reusability and composability of your code.