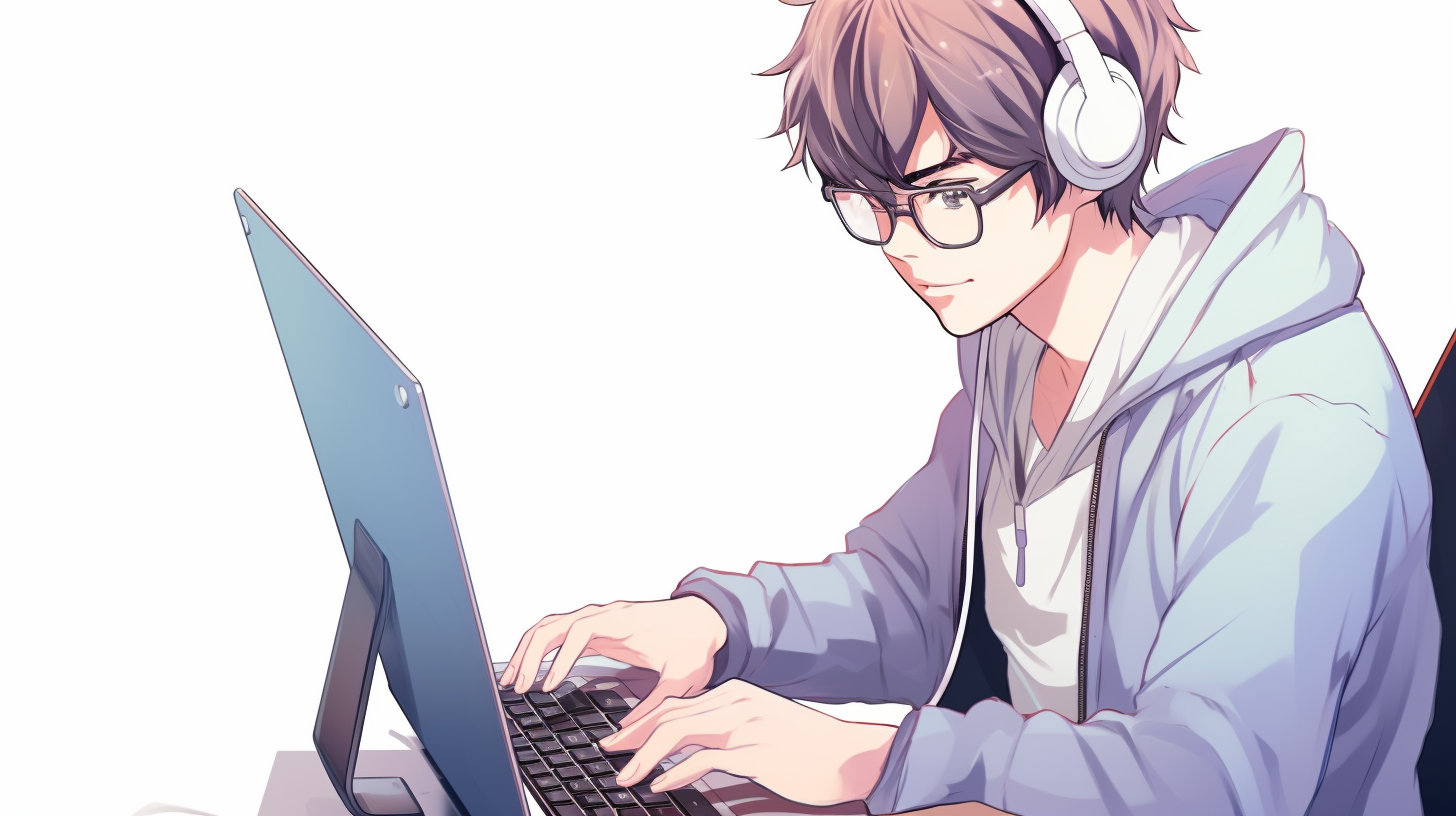
Python and Audio Processing: Basics
When delving into the world of audio processing in Python, it’s imperative to grasp the various audio formats available and the libraries that facilitate their manipulation. Audio formats can be broadly categorized into uncompressed and compressed types. Uncompressed formats, such as WAV and AIFF, store audio data in its raw form, making them ideal for high-quality applications. In contrast, compressed formats like MP3 and OGG use algorithms to reduce file size, which may sacrifice some audio fidelity but are more efficient for storage and streaming.
Each audio format has its own set of advantages. For example, while WAV files provide excellent sound quality, they occupy significantly more disk space compared to MP3 files. Therefore, the choice of format often boils down to the requirements of your specific project, such as quality, file size, and ease of use.
Python offers an array of libraries tailored for audio processing, each with its own strengths. The following are some of the most popular:
- That is a powerful library for music and audio analysis, providing functions to extract audio features, perform time stretching, pitch shifting, and much more. It’s particularly well-suited for processing music.
- Best known for its simplicity, Pydub allows you to easily manipulate audio files with minimal code. It supports many formats and makes tasks like slicing, concatenating, and applying effects simpler.
- This library provides an interface to read and write sound files in various formats, particularly focused on uncompressed files.
- A built-in library in Python for reading and writing WAV files. It’s less feature-rich than the others but is useful for basic operations.
- This library is essential for audio input and output. It allows you to play sounds, record audio from microphones, and capture sound data.
For instance, using Pydub, you can easily manipulate audio files with just a few lines of code. Here’s a simple example that demonstrates loading an audio file and playing it:
from pydub import AudioSegment from pydub.playback import play # Load an audio file audio = AudioSegment.from_file("example.mp3") # Play the audio play(audio)
Setting Up Your Python Environment for Audio Processing
To embark on your audio processing journey in Python, setting up your environment correctly is important. This setup involves installing the necessary libraries and ensuring that your system is configured to handle audio processing tasks efficiently. Below are detailed steps to get your Python environment ready.
First, ensure you have Python installed on your system. It is recommended to use Python 3.7 or newer, as many audio processing libraries have moved on from Python 2.x. You can download the latest version of Python from the official Python website.
Once Python is installed, you’ll want to manage your packages efficiently. One of the most popular tools for that is pip, which comes bundled with Python installations. You can verify that pip is installed by running the following command in your terminal or command prompt:
pip --version
If pip is installed, you’ll see its version number. If it’s not installed, follow the instructions on the Python website to set it up.
The next step involves creating a virtual environment. That is a best practice in Python development that allows you to manage dependencies for different projects separately without conflicts. You can create a virtual environment by navigating to your project directory and running the following command:
python -m venv audio_env
Activate the virtual environment with the appropriate command for your operating system:
# On Windows audio_envScriptsactivate # On macOS and Linux source audio_env/bin/activate
After activating your virtual environment, you can install the libraries essential for audio processing. For our purposes, you’ll want to install Librosa, Pydub, and PyAudio. You can do this with a single pip command:
pip install librosa pydub pyaudio
Depending on your operating system, you may need additional dependencies for PyAudio. For instance, on Windows, you might download a precompiled wheel file, while on macOS, you can install it via Homebrew:
brew install portaudio
With your libraries installed, you’re now equipped to start processing audio in Python. You can verify the installation by importing the libraries in a Python console or script:
import librosa import pydub import pyaudio print("Libraries loaded successfully!")
Loading and Playing Audio Files
Loading and playing audio files in Python is quite simpler, especially when you leverage the power of libraries like Pydub and Soundfile. These libraries abstract away much of the complexity involved in handling different audio formats, allowing you to focus on the audio processing tasks at hand.
To load an audio file, you first need to specify the file path and the format. Pydub makes this process easy. Here’s an example of how to load an audio file using Pydub:
from pydub import AudioSegment # Load an audio file audio = AudioSegment.from_file("example.mp3")
Once the audio file is loaded into an `AudioSegment` object, you can manipulate it in various ways. For instance, if you want to play the audio, you can utilize the playback feature from Pydub:
from pydub.playback import play # Play the audio play(audio)
In addition to Pydub, you might also want to explore Soundfile for low-level audio loading and playback. This library is particularly useful when working with uncompressed audio files like WAV. Here’s how to load and play a WAV file using Soundfile and PyAudio:
import soundfile as sf import pyaudio # Load the audio file data, samplerate = sf.read('example.wav') # Initialize PyAudio p = pyaudio.PyAudio() # Open a stream stream = p.open(format=pyaudio.paInt16, channels=1, rate=samplerate, output=True) # Play the audio stream.write(data.tobytes()) # Stop and close the stream stream.stop_stream() stream.close() p.terminate()
This example demonstrates how to load a WAV file with Soundfile and play it using PyAudio. The `sf.read` function loads the audio data and its sample rate, while PyAudio manages the playback. This combination gives you more control over audio playback compared to Pydub.
Basic Audio Analysis Techniques
When it comes to audio processing, a fundamental aspect is the ability to analyze audio data to extract meaningful insights. Basic audio analysis techniques involve understanding the characteristics of the audio signal, such as its amplitude, frequency content, and temporal features. Libraries like Librosa provide a robust framework for performing these analyses with ease.
One of the first steps in audio analysis is to load your audio file into a format that can be manipulated. Using Librosa, you can easily load an audio file and retrieve both the audio time series and its sample rate. Here’s how you can do it:
import librosa # Load an audio file audio_file = 'example.wav' audio_data, sample_rate = librosa.load(audio_file, sr=None) # Display the sample rate and length of audio print(f'Sample Rate: {sample_rate}, Length: {len(audio_data)} samples')
Once the audio is loaded, you can perform various analyses. A common analysis technique is to compute the waveform, which represents the amplitude of the audio signal over time. This can be visualized using Matplotlib, providing a clear picture of how the audio evolves. Here’s how to visualize the waveform:
import matplotlib.pyplot as plt import numpy as np # Plot the waveform plt.figure(figsize=(12, 4)) plt.plot(np.linspace(0, len(audio_data) / sample_rate, num=len(audio_data)), audio_data) plt.title('Waveform') plt.xlabel('Time (s)') plt.ylabel('Amplitude') plt.xlim(0, len(audio_data) / sample_rate) plt.show()
Another powerful feature of Librosa is the ability to analyze the frequency content of audio through the Short-Time Fourier Transform (STFT). This technique breaks the audio signal into smaller segments and computes the Fourier Transform for each segment, resulting in a time-frequency representation known as a spectrogram.
Generating a spectrogram can be achieved with the following code:
# Compute the Short-Time Fourier Transform (STFT) stft = librosa.stft(audio_data) # Convert to amplitude (magnitude) spectrogram spectrogram = np.abs(stft) # Plot the spectrogram plt.figure(figsize=(12, 6)) librosa.display.specshow(librosa.amplitude_to_db(spectrogram, ref=np.max), sr=sample_rate, x_axis='time', y_axis='log') plt.colorbar(format='%+2.0f dB') plt.title('Spectrogram') plt.show()
By visualizing the spectrogram, you can observe how the frequency content of the audio signal changes over time, revealing insights about musical notes, spoken words, or other audio characteristics.
Furthermore, you might want to extract specific features from the audio signal, such as the Mel-frequency cepstral coefficients (MFCCs). MFCCs are widely used in audio processing, particularly in speech and music analysis, as they represent the short-term power spectrum of sound. Here’s how to compute and visualize MFCCs:
# Compute the MFCCs mfccs = librosa.feature.mfcc(y=audio_data, sr=sample_rate, n_mfcc=13) # Plot the MFCCs plt.figure(figsize=(12, 6)) librosa.display.specshow(mfccs, sr=sample_rate, x_axis='time') plt.colorbar() plt.title('MFCCs') plt.show()
Applying Audio Effects and Filters
In the context of audio processing, applying effects and filters can drastically alter the sound of an audio file, allowing for creative enhancements or corrective adjustments. Python’s rich set of libraries provides various tools to achieve these modifications, lending themselves to both simple and sophisticated audio effects. Here, we’ll explore how to utilize Pydub and SciPy for applying basic effects and filters.
Pydub simplifies the process of manipulating audio files, making it an excellent choice for applying basic effects. For instance, let’s think how to apply a fade-in and fade-out effect to an audio segment. Here’s a quick demonstration:
from pydub import AudioSegment # Load an audio file audio = AudioSegment.from_file("example.mp3") # Apply a fade-in effect of 2000 milliseconds (2 seconds) fade_in_audio = audio.fade_in(2000) # Apply a fade-out effect of 3000 milliseconds (3 seconds) fade_out_audio = fade_in_audio.fade_out(3000) # Export the modified audio fade_out_audio.export("modified_example.mp3", format="mp3")
In this code snippet, the `fade_in` and `fade_out` methods take the duration in milliseconds, allowing for smooth transitions in the audio playback.
Another interesting effect you can easily apply using Pydub is the change in volume. This can be achieved using the `+` and `-` operators to increase or decrease the volume by a specified number of decibels. Here’s how you can do that:
# Increase volume by 6 dB louder_audio = audio + 6 # Decrease volume by 10 dB quieter_audio = audio - 10 # Export the modified audio louder_audio.export("louder_example.mp3", format="mp3") quieter_audio.export("quieter_example.mp3", format="mp3")
For more advanced filtering, the SciPy library comes into play. It provides capabilities for applying digital filters to audio data. Let’s say you want to apply a low-pass Butterworth filter. Here’s how you can do that:
import numpy as np import soundfile as sf from scipy.signal import butter, lfilter # Define a function to create a Butterworth low-pass filter def butter_lowpass(cutoff, fs, order=5): nyq = 0.5 * fs normal_cutoff = cutoff / nyq b, a = butter(order, normal_cutoff, btype='low', analog=False) return b, a # Apply the filter to the audio data def lowpass_filter(data, cutoff, fs, order=5): b, a = butter_lowpass(cutoff, fs, order=order) y = lfilter(b, a, data) return y # Load the audio file data, samplerate = sf.read('example.wav') # Apply a low-pass filter with a cutoff frequency of 1kHz filtered_data = lowpass_filter(data, cutoff=1000.0, fs=samplerate) # Save the filtered audio sf.write('filtered_example.wav', filtered_data, samplerate)
This example demonstrates the process of defining a Butterworth filter and applying it to the audio signal. The `butter` function computes the filter coefficients, while `lfilter` applies the filter to the audio data, effectively reducing high-frequency noise.
Saving and Exporting Processed Audio
Once you have applied your desired audio effects and alterations, the next critical step is to save and export the processed audio. This allows you to retain your modifications for future use or share them with others. Python provides various libraries that make saving audio files simpler, supporting multiple formats such as WAV, MP3, and OGG. Here, we will explore how to effectively save your processed audio using both Pydub and Soundfile.
Using Pydub is particularly convenient for exporting audio after applying effects. The library simplifies the process of writing audio back to disk. For example, after applying audio effects, you can export your modified audio file with just a few lines of code. Here’s how you can do it:
from pydub import AudioSegment # Load an audio file audio = AudioSegment.from_file("example.mp3") # Apply effects (e.g., fade-in and fade-out) processed_audio = audio.fade_in(2000).fade_out(3000) # Export the modified audio processed_audio.export("modified_example.mp3", format="mp3")
In this snippet, after loading and processing the audio, the `export` method is invoked to save the audio. The `format` parameter specifies the desired audio format, which can be adjusted based on your requirements.
On the other hand, if you are working with uncompressed audio formats, Soundfile is a robust choice for saving processed audio data. Here’s how you can save a WAV file using Soundfile:
import soundfile as sf # Assume 'filtered_data' is the processed audio array and 'samplerate' is its sample rate # Save the filtered audio sf.write('filtered_example.wav', filtered_data, samplerate)
This example highlights the use of the `sf.write` function to save audio data to a WAV file, providing the processed data and its sampling rate. Soundfile handles the file writing efficiently, ensuring that your audio is saved accurately without loss of fidelity.
When exporting audio, it’s essential to ensure that the format chosen is compatible with your intended use case. For instance, while WAV files retain top notch, they can be significantly larger in size compared to MP3 files, which are more suitable for web applications and streaming due to their compressed nature. Always think the trade-off between audio quality and file size when selecting an export format for your processed audio.
Additionally, you may want to include metadata in your exported audio files. Pydub allows for this through the use of the `tags` parameter in the `export` method, enabling you to add details such as artist name or album title. Here’s an example of how to include metadata:
processed_audio.export("modified_with_tags.mp3", format="mp3", tags={"artist": "Your Name", "album": "Your Album"})