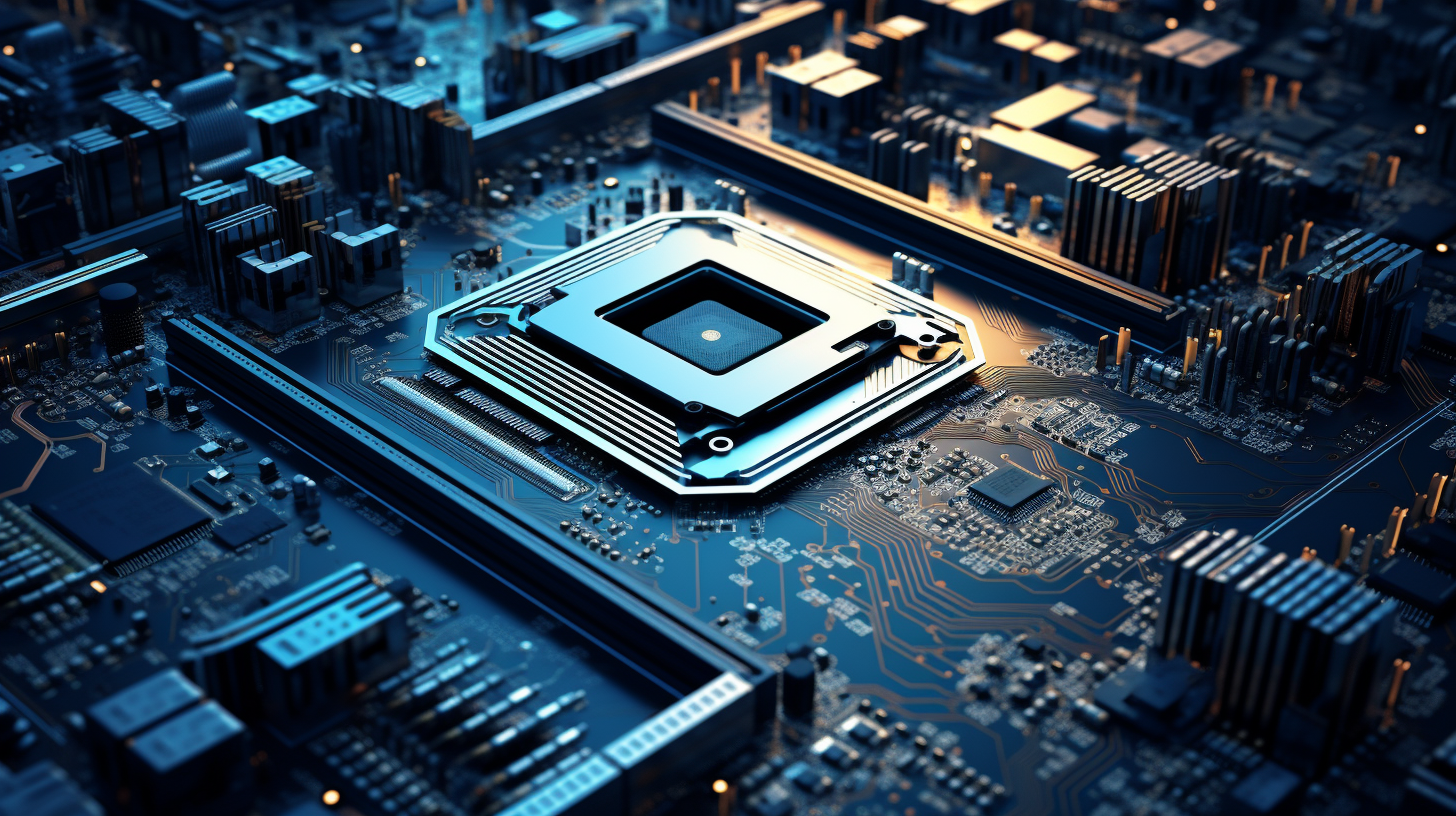
Python and Augmented Reality: An Overview
Augmented Reality (AR) bridges the gap between the physical and digital worlds, enhancing our perception of reality by overlaying digital content onto real-world environments. At its core, AR relies on advanced technologies such as computer vision, simultaneous localization and mapping (SLAM), and depth tracking to create immersive experiences that respond to user interactions in real-time.
To grasp the essence of AR, it is crucial to understand how it interacts with its environment. Through various devices—most commonly smartphones and smart glasses—AR applications utilize the camera and sensors to interpret the real world. Once the environment is understood, virtual objects can be rendered accordingly, creating the illusion that these objects coexist with physical surroundings.
One of the foundational technologies behind AR is computer vision, which enables computers to interpret and understand visual information from the world. Using techniques such as feature detection, object recognition, and image processing, AR systems can identify surfaces and objects, allowing for the accurate placement of digital content. For instance, the OpenCV library in Python provides powerful tools for image processing, making it a popular choice for developers working on AR applications.
import cv2 # Load an image image = cv2.imread('image.jpg') # Convert to grayscale gray_image = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY) # Detect edges using Canny edges = cv2.Canny(gray_image, 100, 200) # Display the original image and the edges cv2.imshow('Original Image', image) cv2.imshow('Edges', edges) cv2.waitKey(0) cv2.destroyAllWindows()
Another significant technology is SLAM, which allows AR systems to at once map an environment and track the location of a user or device within that environment. This technology especially important for applications requiring real-time response and interaction, as it enables the accurate overlay of digital content based on the user’s position and orientation. Implementations of SLAM in Python can leverage libraries like RTAB-Map and Open3D, which facilitate 3D mapping and visualization.
Depth tracking is also integral to AR experiences, enhancing the realism of virtual objects by providing information about their distance from the user. Devices equipped with depth sensors, like those found in modern smartphones and AR glasses, can calculate the spatial relationships between objects and the user, further enriching the interaction. Python developers utilize libraries such as PyTorch3D for rendering and manipulating 3D models, allowing for dynamic adjustments based on depth data.
In essence, the convergence of these technologies enables developers to create rich, interactive experiences that seamlessly integrate digital content with the physical world. As AR technology continues to evolve, the integration of Python libraries tailored for these functionalities will enhance accessibility and broaden the horizons of AR development, enabling creators to push the boundaries of innovation.
Python Libraries for Augmented Reality Development
Within the scope of Augmented Reality (AR) development using Python, several libraries stand out, each offering unique functionalities that cater to various aspects of AR. These libraries not only simplify the development process but also empower developers to harness the full potential of AR technologies.
OpenCV is one of the most widely utilized libraries for computer vision tasks in AR applications. Its extensive functionality allows developers to perform image processing, feature detection, and object recognition, forming the backbone of many AR experiences. With OpenCV, you can easily manipulate images and videos, detect faces, or even track objects in real-time.
import cv2 # Initialize webcam cap = cv2.VideoCapture(0) while True: # Capture frame-by-frame ret, frame = cap.read() # Convert to grayscale gray_frame = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY) # Display the resulting frame cv2.imshow('Video Feed', gray_frame) # Break the loop on 'q' key press if cv2.waitKey(1) & 0xFF == ord('q'): break # Release the capture cap.release() cv2.destroyAllWindows()
Another noteworthy library is ARKit, which, while primarily designed for iOS, can be accessed via Python using tools like PyObjC. ARKit leverages the device’s camera and motion sensors, offering robust capabilities for tracking and rendering AR content. For cross-platform development, the AR.js library provides a lightweight solution for creating AR applications that can be accessed through web browsers. Python can interface with AR.js through back-end frameworks, enabling developers to serve AR content seamlessly over the web.
For 3D modeling and rendering, Pygame and PyOpenGL are invaluable. Pygame can be used for developing 2D aspects of AR applications, while PyOpenGL allows for complex 3D graphics rendering, which is essential when dealing with immersive AR experiences. These libraries enable developers to create engaging environments and interactive elements, enhancing the user’s experience.
import pygame from pygame.locals import * from OpenGL.GL import * from OpenGL.GLU import * # Initialize Pygame pygame.init() display = (800, 600) pygame.display.set_mode(display, DOUBLEBUF | OPENGL) gluPerspective(45, (display[0] / display[1]), 0.1, 50.0) glTranslatef(0.0, 0.0, -5) while True: for event in pygame.event.get(): if event.type == QUIT or (event.type == KEYDOWN and event.key == K_ESCAPE): pygame.quit() glRotatef(1, 0, 1, 0) glClear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT) glBegin(GL_TRIANGLES) glColor3f(1, 0, 0) glVertex3f(-1, -1, 0) glColor3f(0, 1, 0) glVertex3f(1, -1, 0) glColor3f(0, 0, 1) glVertex3f(0, 1, 0) glEnd() pygame.display.flip() pygame.time.wait(10)
For developers focusing on spatial computing, Blender can also be controlled through Python for creating and exporting 3D models suitable for AR environments. The integration of Blender allows for highly customizable and visually appealing 3D assets that can be used in AR applications.
Lastly, TensorFlow and PyTorch can be leveraged for creating machine learning models that enhance AR applications through features like object recognition and environmental understanding. These frameworks enable developers to train models on datasets specific to their AR use cases, thereby improving the overall functionality and responsiveness of their AR experiences.
As the AR landscape continues to grow, the Python ecosystem will likely see further advancements and new libraries tailored for AR development. With these tools at their disposal, developers are well-equipped to push the limits of what is possible in augmented reality.
Use Cases of Python in Augmented Reality Applications
Python has carved out a significant niche in the context of augmented reality (AR) applications, with its flexible syntax and extensive libraries enabling a broad range of use cases. From gaming to education, Python’s role in AR development is increasingly pivotal, allowing developers to create immersive experiences that engage users in novel ways.
One of the most compelling use cases for Python in AR is in the field of education. By overlaying digital content onto physical textbooks or educational materials, AR can transform traditional learning methods into interactive experiences. For instance, using libraries such as OpenCV alongside AR frameworks, developers can create applications that bring static images to life. Imagine a history textbook where students can point their device at a picture, triggering a 3D model of an ancient artifact to appear, complete with audio explanations and interactive quizzes.
import cv2 # Load the AR marker image marker_image = cv2.imread('marker.jpg') # Function to overlay 3D model on marker def display_3D_model(frame): # Assuming we have a function that renders our model # render_model will handle the 3D rendering logic rendered_model = render_model(frame) return rendered_model # Initialize webcam cap = cv2.VideoCapture(0) while True: ret, frame = cap.read() # Detect markers in the frame # if marker is detected, overlay the 3D model if detect_marker(frame, marker_image): frame = display_3D_model(frame) cv2.imshow('AR Application', frame) if cv2.waitKey(1) & 0xFF == ord('q'): break cap.release() cv2.destroyAllWindows()
In the gaming industry, Python’s versatility allows developers to create AR games that blend physical movement with digital interaction. Using libraries like Pygame in conjunction with AR frameworks, developers can design games that require players to move in the real world while interacting with virtual elements. For example, a scavenger hunt game could use GPS data to guide players to specific locations where they can discover virtual treasures, enhancing the gameplay experience with an element of physical exploration.
import pygame from pygame.locals import * # Initialize Pygame pygame.init() screen = pygame.display.set_mode((800, 600)) # Game loop running = True while running: for event in pygame.event.get(): if event.type == QUIT: running = False # Game logic for AR interactions goes here pygame.display.flip() pygame.quit()
Another interesting application of Python in AR is in remote collaboration. With an increasing need for virtual teamwork, applications that allow users to share their AR views can enhance communication and productivity. Developers can use Python to create tools that overlay annotations on shared views, enabling teams to collaborate in real-time on projects regardless of their physical location. This can be particularly valuable in fields such as architecture or engineering, where teams can visualize designs together and modify them on the fly.
Additionally, healthcare is reaping the benefits of AR technology powered by Python. Surgeons can use AR applications to visualize complex anatomical structures during procedures, improving precision and outcomes. By using Python’s machine learning libraries, such as TensorFlow, developers can create applications that offer predictive analytics, enhancing surgical planning with data-driven insights.
import tensorflow as tf # Load a pre-trained model for medical imaging model = tf.keras.models.load_model('medical_model.h5') # Function to predict conditions based on imaging data def predict_condition(image_data): processed_data = preprocess_image(image_data) prediction = model.predict(processed_data) return prediction # Example usage condition = predict_condition('scan_image.jpg') print(f'Predicted condition: {condition}
As AR technology continues to evolve, the possibilities for Python applications in this space are virtually limitless. Whether it is enhancing user engagement through interactive learning, creating compelling gaming experiences, facilitating remote collaboration, or reshaping healthcare practices, Python proves to be a powerful ally in the development of innovative AR solutions.
Challenges and Limitations of Using Python for AR
While Python offers a plethora of advantages for augmented reality (AR) development, it’s not without its challenges and limitations. Understanding these aspects especially important for developers eager to push the boundaries of what AR technologies can achieve.
One of the primary difficulties lies in Python’s performance characteristics. As an interpreted language, Python often falls short in terms of execution speed compared to compiled languages like C++ or Rust. AR applications typically require real-time processing, particularly in areas like computer vision and rendering, where latency can significantly impact user experience. For instance, when developing an AR application that needs to process video feeds in real-time, the delays attributable to Python’s interpreted nature can lead to a disjointed user experience.
import cv2 import time # Initialize webcam cap = cv2.VideoCapture(0) while True: start_time = time.time() # Start timing ret, frame = cap.read() # Simulate processing delay # In a real scenario, this would be image processing work time.sleep(0.05) # Simulating delay cv2.imshow('Video Feed', frame) if cv2.waitKey(1) & 0xFF == ord('q'): break # Check frame processing time processing_time = time.time() - start_time print(f'Frame processing time: {processing_time:.4f} seconds') cap.release() cv2.destroyAllWindows()
Another limitation is the ecosystem surrounding Python. While there are impressive libraries available, the maturity and depth of AR-specific frameworks do not match those of languages such as Swift or Java, which have dedicated environments and support through platforms like ARKit and ARCore, respectively. Developers may find themselves needing to bridge gaps between Python and these more established AR frameworks, which can complicate development workflows. For instance, integrating Python with ARKit requires additional layers of abstraction, which can introduce overhead and complexity to the project.
Moreover, resource constraints on mobile devices pose a significant challenge. AR applications often demand substantial computing power and memory, especially when rendering complex 3D models or processing extensive datasets. Python’s memory management, while convenient, can lead to inefficiencies when handling large-scale AR applications, potentially leading to crashes or performance degradation on devices with limited resources.
Licensing and distribution can also be a hurdle. Many Python libraries rely on open-source licenses, which may not be suitable for commercial applications due to compliance and distribution restrictions. This can limit a developer’s ability to deploy AR solutions on a larger scale or necessitate costly legal consultations to navigate licensing agreements.
Lastly, the community and support for AR development in Python may not be as robust as for other languages. While the community is active, the specialized nature of AR development poses challenges in finding dedicated forums or resources tailored specifically for Python AR developers. This can make troubleshooting and knowledge sharing more difficult, potentially slowing down the development process.
While Python stands as a versatile tool in the AR developer’s arsenal, the challenges it presents—performance issues, ecosystem limitations, resource constraints, licensing hurdles, and community support—are critical factors to think. Developers must weigh these challenges against the benefits of using Python, ensuring they deploy the right tools and strategies to create effective AR experiences.
Future Trends in Python and Augmented Reality Integration
As the landscape of augmented reality (AR) continues to expand, so too does the potential for Python to play a pivotal role in its evolution. The integration of Python with AR is projected to deepen, influenced by several key trends that are shaping the future of both technologies. These trends reflect advancements in hardware, software, and the growing adoption of AR across various industries.
One of the most significant trends is the increasing accessibility of AR hardware. As devices equipped with advanced sensors and cameras become more ubiquitous—ranging from smartphones to specialized AR glasses—developers will have more opportunities to leverage Python in creating sophisticated AR applications. This accessibility will not only democratize AR development but will also inspire a new wave of innovative applications, particularly in education, gaming, and remote collaboration.
Another trend is the rising demand for cross-platform AR solutions. Businesses increasingly seek to deploy AR applications that function seamlessly across various operating systems and devices. Python’s flexibility and the availability of frameworks that facilitate cross-platform development will enable developers to create applications that reach broader audiences without sacrificing functionality. For instance, using tools like Flask combined with AR.js can allow Python developers to create web-based AR applications, making AR content more accessible to users regardless of their device.
Moreover, the integration of artificial intelligence (AI) and machine learning (ML) with AR is set to improve the capabilities of applications. Python, being a dominant language in the AI field, will naturally play a critical role in this convergence. The ability to incorporate ML models into AR applications can provide features like real-time object recognition, gesture detection, and personalized experiences based on user interactions. Python frameworks such as TensorFlow and PyTorch will continue to be instrumental in this evolution, allowing developers to build intelligent AR systems that respond dynamically to their environments.
import tensorflow as tf # Load a pre-trained model for object detection model = tf.keras.models.load_model('object_detection_model.h5') # Function to detect objects in an image def detect_objects(image): processed_image = preprocess_image(image) predictions = model.predict(processed_image) return predictions # Example usage with an AR application detected_objects = detect_objects('scene_image.jpg') print(f'Detected objects: {detected_objects}')
Furthermore, advancements in cloud computing and edge processing will significantly impact AR development. By offloading complex processing tasks to the cloud, developers can leverage Python to create more resource-efficient AR applications. This shift will enable devices with limited computing power to run sophisticated AR experiences, as data can be processed remotely and streamed back to the device. Python’s compatibility with cloud frameworks ensures that developers can easily integrate these capabilities into their AR applications.
As Python libraries continue to evolve, we can expect to see more specialized tools emerging for AR development. Libraries that streamline the integration of AR features, enhance rendering capabilities, or improve interaction design will likely be developed as the demand for AR solutions grows. The community-driven nature of Python will play a vital role in fostering innovation, as developers share their solutions and build upon each other’s work.
Finally, the societal impact of AR technology cannot be overlooked. As AR becomes more ingrained in daily life, ethical considerations surrounding privacy, data security, and user consent will gain prominence. Python developers will need to navigate these challenges responsibly, ensuring that the applications they create are not only innovative but also respect user rights and promote positive user experiences.
The future of Python in augmented reality integration is bright, driven by trends in hardware accessibility, cross-platform solutions, AI and ML capabilities, cloud computing, the emergence of new libraries, and societal considerations. As these trends unfold, Python developers will be uniquely positioned to craft the next generation of AR experiences, pushing the boundaries of what is possible in this exciting field.