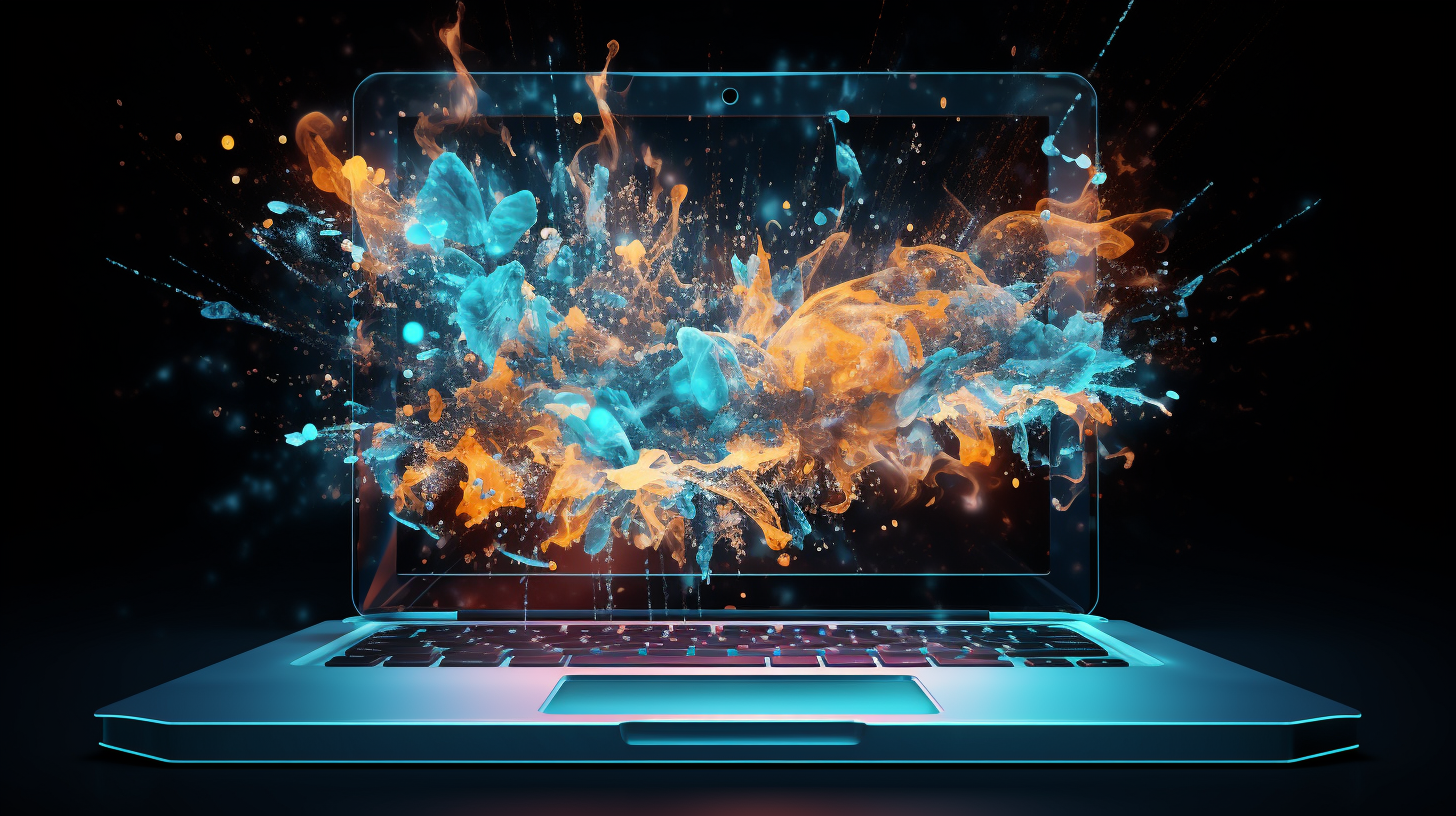
Python and Blockchain: Understanding the Basics
Blockchain technology represents a paradigm shift in how data is stored, managed, and transferred across networks. At its core, a blockchain is a decentralized ledger that records transactions across multiple computers in such a manner that the registered transactions cannot be altered retroactively. This ensures transparency and enhances security, as all participants in the network have access to the same data.
Each block in a blockchain contains a list of transactions, a timestamp, and a cryptographic hash of the previous block. This chaining of blocks is what gives the technology its name. The integrity of the blockchain is maintained through a consensus mechanism, which ensures that all nodes on the network agree on the current state of the ledger. Common consensus algorithms include Proof of Work (PoW) and Proof of Stake (PoS).
One of the foundational attributes of blockchain is its immutability. Once a block is added to the chain, altering its data without the consensus of the network becomes computationally impractical. This characteristic is achieved through cryptographic techniques, making it exceedingly difficult for malicious actors to tamper with the data.
Another critical component of blockchain technology is decentralization. Unlike traditional databases that rely on a central authority, blockchain distributes its data across a network of nodes. This not only enhances the security of the system but also fosters trust among participants, as no single entity has control over the entire network.
The usage of smart contracts further illustrates the potential of blockchain. Smart contracts are self-executing contracts with the terms of the agreement directly written into code. They run on the blockchain and automatically execute actions when predefined conditions are met, thereby reducing the need for intermediaries.
In Python, developers can interact with blockchain networks using several libraries and frameworks that facilitate the creation, management, and querying of blockchain data. For example, the web3.py library allows Python programs to communicate with Ethereum networks, enabling developers to create and manage smart contracts seamlessly.
from web3 import Web3 # Connect to an Ethereum node w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID')) # Check connection if w3.isConnected(): print("Connected to Ethereum Network") # Get the latest block number latest_block = w3.eth.blockNumber print(f"Latest Ethereum Block Number: {latest_block}")
This code snippet establishes a connection to the Ethereum blockchain using the Infura service, which provides a hassle-free way to interact with the Ethereum network. Once connected, it retrieves the latest block number, demonstrating how easy it is to access blockchain data using Python.
Understanding these fundamentals very important for anyone looking to dive deeper into the world of blockchain development. The technology not only supports cryptocurrencies but also has potential applications across various industries, from finance to supply chain management, making it an exciting field for developers to explore.
Key Concepts in Python Programming
To effectively harness the power of Python in the sphere of blockchain development, one must first grasp several key programming concepts that underpin the language itself. Python is known for its simplicity and readability, making it an ideal choice for both novice and advanced developers alike. Its dynamic typing and interpreted nature allow for rapid development, enabling developers to prototype and deploy applications swiftly.
Data Structures are fundamental in Python, allowing developers to store and manipulate data efficiently. Key structures include lists, tuples, dictionaries, and sets. For instance, dictionaries are particularly useful for representing blockchain transactions, as they can map transaction attributes to their respective values:
transaction = { "sender": "Alice", "recipient": "Bob", "amount": 50, "timestamp": "2023-10-05T12:00:00Z" }
This dictionary structure allows for easy access and modification of transaction data, which can be critical when developing smart contracts or blockchain applications.
Functions in Python serve as reusable blocks of code that can be executed when called. This modular approach not only promotes code reusability but also enhances readability. Consider creating a function that calculates the hash of a block, a critical operation in the blockchain process:
import hashlib def calculate_hash(block): block_string = str(block).encode() return hashlib.sha256(block_string).hexdigest() # Example block data block_data = { "index": 1, "previous_hash": "0", "transactions": [transaction] } # Calculating the hash of the block block_hash = calculate_hash(block_data) print(f"Block Hash: {block_hash}")
This function takes a block’s data, encodes it, and generates a SHA-256 hash, which very important for maintaining the integrity of the blockchain.
Object-Oriented Programming (OOP) is another essential concept within Python that allows developers to create classes and objects, encapsulating data and functionality. This approach can be particularly useful when designing blockchain nodes or smart contracts. Here’s a simple example of a blockchain class:
class Blockchain: def __init__(self): self.chain = [] self.current_transactions = [] def create_block(self, previous_hash): block = { "index": len(self.chain) + 1, "transactions": self.current_transactions, "previous_hash": previous_hash, } self.current_transactions = [] # Reset the transaction list self.chain.append(block) return block # Create a blockchain instance my_blockchain = Blockchain() first_block = my_blockchain.create_block(previous_hash="0") print(first_block)
In this example, the Blockchain
class initializes a chain and manages transactions, encapsulating the logic required to add new blocks to the blockchain. This structure is foundational for building more complex applications.
Moreover, Python’s extensive libraries and frameworks further enhance its capabilities in blockchain development. Libraries such as Flask
for web applications and pycryptodome
for cryptographic operations provide developers with a robust toolkit for building decentralized applications.
As one explores the landscape of blockchain development, it becomes evident that a solid understanding of Python programming concepts is not just beneficial but essential. Mastery of these concepts sets the groundwork for diving into more advanced topics, such as integrating with blockchain APIs, developing smart contracts, and deploying decentralized applications.
Integrating Python with Blockchain Platforms
Integrating Python with blockchain platforms opens a world of possibilities for developers wishing to leverage the capabilities of both technologies. With the right libraries and frameworks, Python can act as a bridge to various blockchain networks, allowing for the development of decentralized applications (dApps), smart contracts, and more.
One of the most popular blockchain platforms that can be integrated with Python is Ethereum. The web3.py library, as previously mentioned, is a powerful tool that enables developers to interact with the Ethereum blockchain. This library allows for the management of wallets, deployment of smart contracts, and execution of transactions with ease. Below is a demonstration of how to deploy a simple smart contract using web3.py:
from web3 import Web3 # Connect to an Ethereum node w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID')) # Check connection if w3.isConnected(): print("Connected to Ethereum Network") # Sample Solidity contract code contract_source_code = ''' pragma solidity ^0.8.0; contract SimpleStorage { uint256 storedData; function set(uint256 x) public { storedData = x; } function get() public view returns (uint256) { return storedData; } } ''' # Compile the contract using a Solidity compiler (this could be done using solcx library) from solcx import compile_source compiled_sol = compile_source(contract_source_code) contract_id, contract_interface = compiled_sol.popitem() # Get the contract's ABI and bytecode abi = contract_interface['abi'] bytecode = contract_interface['bin'] # Create a contract instance SimpleStorage = w3.eth.contract(abi=abi, bytecode=bytecode) # Set up the transaction tx_hash = SimpleStorage.constructor().transact({'from': w3.eth.accounts[0]}) receipt = w3.eth.waitForTransactionReceipt(tx_hash) print(f"Contract deployed at address: {receipt.contractAddress}")
This code snippet first establishes a connection to the Ethereum network, then defines a simple Solidity smart contract that can store and retrieve a number. It compiles the contract, creates a transaction to deploy it, and finally produces the address where the contract is deployed. This is a prime example of how Python can facilitate interactions with blockchain platforms.
Another powerful integration point for Python in the blockchain space is with Hyperledger Fabric. Hyperledger Fabric is a permissioned blockchain framework this is designed for use in enterprise settings. Python developers can use the Hyperledger Fabric SDK for Python, which provides functionalities for managing network interactions and smart contract executions.
from hfc.fabric import Client # Create a client instance fabric_client = Client(net_profile="network.json") # Define the channel and chaincode channel = fabric_client.get_channel('mychannel') chaincode = 'mychaincode' # Invoke a chaincode function response = channel.upgrade_chaincode(chaincode, '1.0', 'init', [ 'arg1', 'arg2' ]) print(f"Chaincode upgraded with response: {response}")
In this example, the Hyperledger Fabric SDK for Python is utilized to connect to a defined network profile and interact with a specific channel and chaincode (smart contract). The upgrade_chaincode function is invoked to upgrade a chaincode, demonstrating how Python can be used to manage and interact with enterprise-level blockchain solutions.
Through these integrations, Python developers are not limited to just Ethereum or Hyperledger; many other platforms such as EOS, NEO, and Corda also provide APIs that can be accessed using Python. The flexibility and simplicity of Python make it an excellent choice for developers looking to harness the power of blockchain technology.
The integration of Python with blockchain platforms is not just about accessing blockchain data; it involves creating complex applications that can handle transactions, execute smart contracts, and manage the intricacies of decentralized networks. With Python’s extensive libraries and the ever-evolving blockchain ecosystem, developers have the tools they need to push the boundaries of what’s possible in this exciting field.
Real-World Applications of Python in Blockchain Development
As we delve into the real-world applications of Python in blockchain development, it becomes abundantly clear that this combination has sparked innovation across diverse sectors. From financial services to healthcare and supply chain management, Python’s versatility and ease of use enable developers to create robust solutions that leverage the unique capabilities of blockchain technology.
In the financial sector, for instance, many organizations utilize Python to develop trading platforms that interact with blockchain networks. The ability to write scripts that handle real-time data feeds and execute trades through smart contracts is essential for firms looking to capitalize on market opportunities. Here’s a simple example demonstrating how to interact with a cryptocurrency exchange API using Python:
import requests # Fetch data from the cryptocurrency exchange API response = requests.get('https://api.coingecko.com/api/v3/simple/price?ids=bitcoin&vs_currencies=usd') data = response.json() print(f"Current Bitcoin Price: ${data['bitcoin']['usd']}
”
This snippet fetches the current price of Bitcoin in USD, showcasing how Python can be used to retrieve and manipulate real-time cryptocurrency data, which is a foundational aspect of many trading applications.
Healthcare is another field where blockchain applications are gaining traction, particularly in managing patient records and ensuring data integrity. Python is often employed to create secure applications that allow for the creation, sharing, and storage of health records on a blockchain while maintaining patient privacy. Below is an example of defining a patient record as a transaction in a blockchain:
patient_record = { "patient_id": "12345", "name": "Nick Johnson", "medical_history": [ {"date": "2023-01-20", "condition": "Flu", "treatment": "Rest and fluids"}, {"date": "2023-05-15", "condition": "Check-up", "treatment": "Routine examination"} ], "timestamp": "2023-10-05T12:00:00Z" } # Function to add patient record to the blockchain def add_patient_record(blockchain, record): blockchain.create_transaction(record) blockchain.add_to_chain() # Assuming blockchain is an instance of Blockchain class add_patient_record(my_blockchain, patient_record)
This code defines a patient record and includes a function to add it to a blockchain. In practice, this would involve hashing this record and creating a transaction to ensure that the data is immutable and secure.
Within the scope of supply chain management, Python’s ability to manage complex data structures makes it an excellent choice for developing solutions that track goods from origin to end consumer on a blockchain. By providing transparency and traceability, businesses can ensure the authenticity of their products. For example, think the following approach to log supply chain events:
supply_chain_event = { "product_id": "XYZ789", "status": "Shipped", "location": "Warehouse A", "timestamp": "2023-10-05T12:01:00Z" } # Function to log the event def log_supply_chain_event(blockchain, event): blockchain.create_transaction(event) blockchain.add_to_chain() # Log the supply chain event log_supply_chain_event(my_blockchain, supply_chain_event)
This snippet illustrates how supply chain events can be recorded on a blockchain, providing stakeholders with real-time updates about the status and location of products. This level of visibility very important for maintaining trust and efficiency in supply chains.
Moreover, the integration of Python with machine learning libraries can enhance blockchain applications. Predictive analytics can be applied to transaction data to identify patterns and potential security threats, allowing organizations to proactively address issues before they escalate. For instance, by using Python’s scikit-learn library, developers can build models that analyze transaction trends and detect anomalies.
from sklearn.ensemble import IsolationForest import numpy as np # Sample transaction amounts transactions = np.array([[100], [150], [200], [300], [500], [700], [1000]]) # Train the model model = IsolationForest() model.fit(transactions) # Predict anomalies predictions = model.predict(transactions) print("Anomaly Predictions:", predictions)
In this example, the Isolation Forest algorithm is used to identify unusual transaction amounts, which could indicate fraudulent activity. Python’s integration capabilities facilitate the blending of blockchain with advanced data analytics for enhanced security and operational efficiency.
As these examples illustrate, the synergy between Python and blockchain technology fosters a plethora of real-world applications that not only solve existing problems but also pave the way for innovative solutions across various industries. Whether it’s enabling secure financial transactions, managing healthcare data, tracking product authenticity in supply chains, or enhancing security through data analysis, Python remains a vital tool for developers navigating the blockchain landscape.
Future Trends: Python and the Evolving Blockchain Landscape
As we look forward into the evolving landscape of blockchain technology, it’s clear that Python will continue to play a pivotal role in this dynamic field. The trends emerging today indicate that the integration of Python with blockchain will become even more sophisticated, driven by advances in technology and the increasing demand for decentralized applications.
One significant trend is the rise of decentralized finance (DeFi) platforms, which aim to recreate traditional financial systems—such as lending, borrowing, and trading—in a decentralized manner. Python’s role in this ecosystem cannot be understated, as developers leverage its simplicity and robust libraries to create and manage complex smart contracts. The development of Python-based frameworks that simplify interaction with DeFi protocols is already underway, which will enable even novice developers to participate in this space.
Moreover, the increasing popularity of non-fungible tokens (NFTs) has opened new avenues for Python developers. NFTs, which have taken the art and gaming industries by storm, require a blend of blockchain technology with uncomplicated to manage applications. Libraries such as Flask or Django can be utilized alongside web3.py to create intuitive user interfaces for NFT marketplaces. Ponder the following code snippet demonstrating how to mint an NFT using Python on Ethereum:
from web3 import Web3 from solcx import compile_source # Connect to Ethereum w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/YOUR_INFURA_PROJECT_ID')) # Sample NFT contract code nft_source_code = ''' pragma solidity ^0.8.0; contract SimpleNFT { mapping(uint256 => address) public tokenOwners; uint256 public totalSupply; function mint() public { tokenOwners[totalSupply] = msg.sender; totalSupply++; } } ''' # Compile the NFT contract compiled_nft = compile_source(nft_source_code) nft_contract_id, nft_contract_interface = compiled_nft.popitem() nft_abi = nft_contract_interface['abi'] nft_bytecode = nft_contract_interface['bin'] # Create a contract instance SimpleNFT = w3.eth.contract(abi=nft_abi, bytecode=nft_bytecode) # Mint an NFT tx_hash = SimpleNFT.constructor().transact({'from': w3.eth.accounts[0]}) receipt = w3.eth.waitForTransactionReceipt(tx_hash) print(f"NFT minted and contract deployed at address: {receipt.contractAddress}")
This code snippet illustrates the minting process of an NFT on the Ethereum blockchain. As the DeFi and NFT markets grow, the demand for Python developers proficient in these areas will surge, providing ample opportunities for those with the right skills.
Another trend poised to shape the future of Python and blockchain is the integration of artificial intelligence (AI) and machine learning (ML) with decentralized applications. By using Python’s extensive AI and ML libraries, developers can create intelligent applications that analyze blockchain data in real-time. For instance, predictive analytics could be applied to transaction patterns to enhance security measures or improve user experience within dApps. Below is a simple example of using Python’s TensorFlow library to predict transaction trends:
import tensorflow as tf import numpy as np # Sample historical transaction data historical_data = np.array([100, 150, 200, 300, 500]) # Prepare the model model = tf.keras.Sequential() model.add(tf.keras.layers.Dense(10, activation='relu', input_shape=(1,))) model.add(tf.keras.layers.Dense(1)) model.compile(optimizer='adam', loss='mean_squared_error') # Train the model model.fit(historical_data[:-1], historical_data[1:], epochs=100) # Make a prediction prediction = model.predict(np.array([[historical_data[-1]]])) print(f"Predicted next transaction amount: {prediction[0][0]}")
In this example, a simple neural network is trained on historical transaction data to predict future transaction amounts. The intersection of AI, ML, and blockchain has the potential to revolutionize data analysis and decision-making processes within the blockchain realm.
Additionally, as blockchain technology matures, there is an increasing need for regulatory compliance and security. Python developers must stay ahead of the curve by incorporating best practices in security and data protection into their applications. This includes implementing robust cryptographic measures and ensuring that smart contracts are free from vulnerabilities. Libraries such as PyCryptodome can be instrumental in achieving these goals:
from Crypto.PublicKey import RSA from Crypto.Cipher import PKCS1_OAEP # Generate a public/private key pair key = RSA.generate(2048) public_key = key.publickey() # Encrypt a message message = b'Hello, secure blockchain!' cipher = PKCS1_OAEP.new(public_key) encrypted_message = cipher.encrypt(message) print("Encrypted Message:", encrypted_message)
In this snippet, we generate a public/private key pair and encrypt a message, demonstrating the importance of cryptographic techniques in securing blockchain applications. As security concerns grow, the demand for Python developers with expertise in secure coding practices will only increase.
To wrap it up, the future trends surrounding Python and the evolving blockchain landscape are vibrant and full of promise. The interplay between these technologies will continue to reshape industries, creating new opportunities for developers to harness their skills in innovative ways. Whether it is through the development of DeFi platforms, the minting of NFTs, or the integration of AI and ML, Python will remain a cornerstone of blockchain development, enabling solutions that drive progress and transformation in this exciting domain.