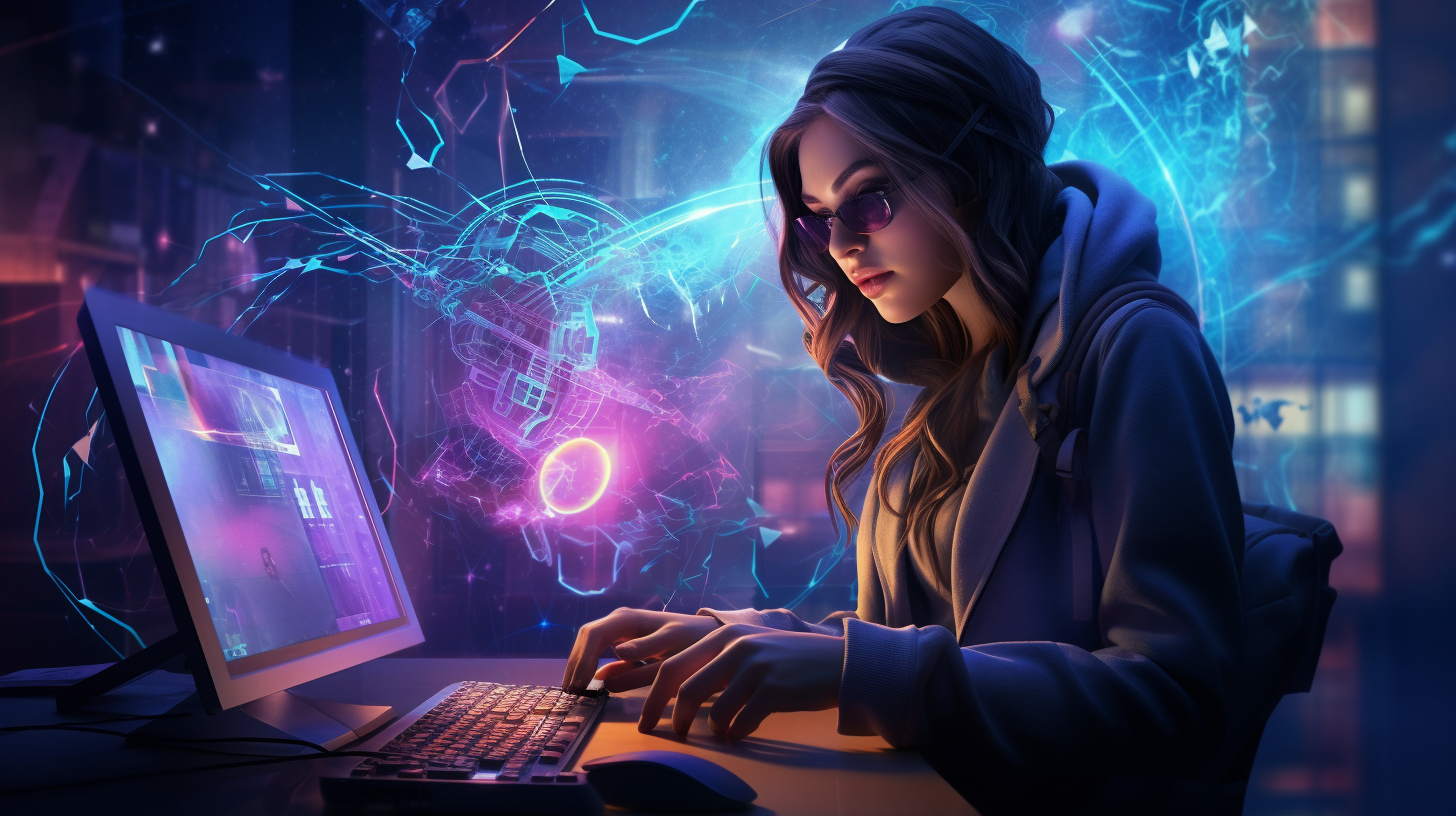
Python and Fashion: Analyzing Trends
In recent years, the intersection of data analysis and fashion has generated significant interest, driven by the increasing availability of data and the need for businesses to make data-informed decisions. As fashion evolves, understanding consumer behavior, preferences, and market dynamics has become paramount. Current trends highlight the importance of using data analytics to stay ahead in the competitive fashion industry.
One prevalent trend is the rise of social media analytics. Brands are now scrutinizing social media platforms to gauge consumer sentiment and identify emerging styles. By analyzing posts, likes, and shares, companies can effectively predict which trends will gain traction. This process often involves scraping data from platforms like Instagram and Twitter, followed by sentiment analysis to interpret the data.
import tweepy from textblob import TextBlob # Twitter API credentials consumer_key = 'your_consumer_key' consumer_secret = 'your_consumer_secret' access_token = 'your_access_token' access_token_secret = 'your_access_token_secret' # Setup tweepy to access Twitter API auth = tweepy.OAuthHandler(consumer_key, consumer_secret) auth.set_access_token(access_token, access_token_secret) api = tweepy.API(auth) # Function to fetch tweets and analyze sentiment def analyze_sentiment(query): tweets = api.search(query, count=100) tweet_data = [] for tweet in tweets: analysis = TextBlob(tweet.text) tweet_data.append((tweet.text, analysis.sentiment.polarity)) return tweet_data # Example usage result = analyze_sentiment('fashion trends') print(result)
Another impactful trend is the use of predictive analytics to forecast demand. Retailers are now employing machine learning algorithms to analyze historical sales data, which helps in inventory management and optimizing supply chains. By accurately predicting which items will sell well, companies can reduce overstock and minimize losses from unsold products.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression # Load sales data data = pd.read_csv('sales_data.csv') # Prepare data for modeling X = data[['previous_sales', 'season', 'price']] y = data['current_sales'] # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) # Train a linear regression model model = LinearRegression() model.fit(X_train, y_train) # Make predictions predictions = model.predict(X_test) print(predictions)
Furthermore, visualizing fashion data has become essential for extracting insights at a glance. Data visualization tools allow brands to present complex data in a more digestible format, making it easier for stakeholders to understand trends and make strategic decisions. Libraries such as Matplotlib and Seaborn in Python are frequently used to create aesthetically pleasing and informative visualizations.
import matplotlib.pyplot as plt import seaborn as sns # Example data for visualization data = {'Trend': ['Streetwear', 'Sustainable', 'Vintage', 'Luxury'], 'Popularity': [30, 50, 20, 40]} # Create a bar chart sns.barplot(x='Trend', y='Popularity', data=data) plt.title('Current Fashion Trends Popularity') plt.xlabel('Fashion Trends') plt.ylabel('Popularity (%)') plt.show()
As these trends continue to shape the fashion industry, the role of data analysis will only become more significant. By using the power of Python and its libraries, fashion brands can gain invaluable insights that drive innovation and consumer engagement.
Using Python Libraries for Fashion Insights
To effectively leverage Python for fashion insights, practitioners often rely on a variety of specialized libraries tailored to specific analytical needs. Each library offers unique functionalities that allow for deep dives into the multifaceted data generated by the fashion industry, enabling professionals to derive actionable insights. Let’s explore some of the most impactful libraries and their applications within the scope of fashion data analysis.
Pandas is an essential library for data manipulation and analysis. It provides powerful data structures, like DataFrames, which are perfect for handling complex datasets typical in fashion analytics. For instance, a fashion retailer can utilize Pandas to clean and preprocess sales data, enabling more accurate analysis of consumer preferences over time.
import pandas as pd # Load fashion sales data data = pd.read_csv('fashion_sales_data.csv') # Clean data by removing null values data.dropna(inplace=True) # Group by category to analyze sales trends category_sales = data.groupby('category')['sales'].sum().reset_index() # Display the sales trends by category print(category_sales)
NumPy complements Pandas by providing support for numerical operations, which is critical when performing statistical analyses on fashion datasets. For example, retailers can use NumPy to calculate statistical measures like averages and standard deviations to understand sales performance across different demographics.
import numpy as np # Calculate mean and standard deviation of sales mean_sales = np.mean(data['sales']) std_sales = np.std(data['sales']) print(f'Mean Sales: {mean_sales}, Standard Deviation: {std_sales}')
Scikit-learn is a robust library for machine learning, equipped with a plethora of algorithms for classification, regression, and clustering. Fashion brands can employ Scikit-learn to build models that predict future trends based on historical data. For instance, creating a clustering model can help identify distinct customer segments, allowing for targeted marketing strategies.
from sklearn.cluster import KMeans # Preparing data for clustering X = data[['age', 'spending_score']] # Implement KMeans clustering kmeans = KMeans(n_clusters=3) data['cluster'] = kmeans.fit_predict(X) # Display the first few rows of the clustered data print(data.head())
Visualization is another critical aspect, and libraries like Matplotlib and Seaborn facilitate the conversion of complex data into visual representations that are more accessible. For instance, a fashion brand can visualize customer demographics to tailor their marketing strategies effectively.
import matplotlib.pyplot as plt import seaborn as sns # Sample demographic data demographics = {'Age Group': ['18-24', '25-34', '35-44', '45-54'], 'Percentage': [25, 35, 20, 20]} demo_df = pd.DataFrame(demographics) # Create a pie chart to visualize customer demographics plt.pie(demo_df['Percentage'], labels=demo_df['Age Group'], autopct='%1.1f%%') plt.title('Customer Demographics by Age Group') plt.show()
Moreover, using Natural Language Processing (NLP) libraries such as NLTK or spaCy can provide insights from textual data, which is increasingly relevant in analyzing fashion blogs, reviews, and social media. By employing sentiment analysis, brands can better understand consumer opinions and sentiments regarding their products.
from nltk.sentiment import SentimentIntensityAnalyzer # Initialize the sentiment intensity analyzer sia = SentimentIntensityAnalyzer() # Example reviews reviews = ["I absolutely love this new collection!", "The quality is terrible, I'm disappointed."] # Analyze sentiment for review in reviews: score = sia.polarity_scores(review) print(f'Review: {review} - Sentiment Score: {score}
)
By integrating these libraries into their workflows, fashion brands can develop a comprehensive understanding of market trends and consumer behavior. The ability to manipulate, analyze, and visualize data empowers brands to make informed decisions that align with consumer preferences and industry trends.
Case Studies: Successful Applications of Python in Fashion
Examining case studies where Python has been effectively utilized in the fashion industry reveals several successful applications that underscore its transformative potential. One notable example is how leading fashion retailers have adopted machine learning for trend forecasting. By analyzing vast datasets that include past sales, social media interactions, and consumer behavior, brands can predict future demand for specific styles, colors, and designs.
A well-documented case is that of a global fashion retailer that implemented a machine learning model using Scikit-learn to enhance its inventory management system. This retailer analyzed historical sales data and external factors like weather patterns and cultural events to develop a comprehensive forecasting model. The model utilized a combination of regression and classification algorithms to provide insights into which products were likely to perform well in upcoming seasons.
from sklearn.ensemble import RandomForestRegressor # Historical sales data loaded into a DataFrame data = pd.read_csv('historical_sales_data.csv') # Features for the model: past sales, promotions, and seasonality X = data[['past_sales', 'promotion_level', 'season']] y = data['future_sales'] # Train-test split X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2) # Train the Random Forest model model = RandomForestRegressor(n_estimators=100) model.fit(X_train, y_train) # Predictions for future sales predictions = model.predict(X_test) print(predictions)
This approach not only minimized overstock situations but also improved the accuracy of inventory levels across different store locations. By forecasting demand based on a sophisticated analysis, the retailer could adjust its supply chain strategy in real time, thus enhancing profitability.
Another illustrative case involves a luxury fashion brand that sought to leverage consumer sentiment from social media to tailor its marketing strategies. By employing NLP techniques with Python’s NLTK library, the brand analyzed thousands of tweets, Instagram posts, and online reviews. This deep dive into consumer sentiment allowed the brand to identify key influences on buying behavior and preferences.
from nltk.sentiment import SentimentIntensityAnalyzer # Collecting data from social media (simulated here) social_media_posts = [ "I'm obsessed with this new handbag!", "Not worth the price, really disappointed.", "The new collection is breathtaking!" ] # Sentiment analysis on social media posts sia = SentimentIntensityAnalyzer() sentiment_scores = [sia.polarity_scores(post) for post in social_media_posts] # Display the sentiment scores for post, score in zip(social_media_posts, sentiment_scores): print(f'Post: "{post}" - Sentiment Score: {score}')
With the insights gained, the brand was able to adjust its marketing campaigns to emphasize aspects that resonated positively with consumers while addressing criticisms in their product lines. This strategic responsiveness not only strengthened their market position but also fostered a deeper engagement with their customer base.
Furthermore, data visualization played an important role in these case studies. By employing Matplotlib and Seaborn, brands visualized complex datasets to extract trends and consumer insights. For example, one company created a series of visual dashboards that presented sales performance across different regions, helping decision-makers quickly identify emerging trends.
import seaborn as sns # Sample sales data sales_data = pd.DataFrame({ 'Region': ['North', 'South', 'East', 'West'], 'Sales': [200, 300, 150, 400] }) # Create a barplot to visualize sales by region sns.barplot(x='Region', y='Sales', data=sales_data) plt.title('Sales Performance by Region') plt.xlabel('Region') plt.ylabel('Sales ($)') plt.show()
This visualization allowed stakeholders to pinpoint regions with underperforming sales, leading to targeted marketing efforts and product adjustments based on local preferences.
These case studies showcase how Python’s capabilities in data analysis, machine learning, and visualization not only drive efficiency but also foster innovation in the fashion industry. The ability to extract and interpret data effectively enables brands to respond to market demands swiftly, thereby securing an advantage in a rapidly changing landscape.
Future Directions: Python’s Role in Fashion Innovation
As fashion continues to evolve, the role of Python in driving innovation is set to expand even further. Emerging technologies such as artificial intelligence and machine learning are increasingly integrated into the fashion industry, providing new avenues for enhancing consumer experiences, optimizing operations, and pioneering creative designs.
One exciting direction is the application of AI-driven design tools that leverage generative algorithms. By using Python’s capabilities along with libraries like TensorFlow or PyTorch, designers can create unique garment patterns based on existing styles or even generate entirely new concepts. For example, a fashion brand could feed a neural network a dataset of historical designs to guide the algorithm in creating innovative yet marketable clothing items.
import tensorflow as tf from tensorflow import keras from tensorflow.keras import layers # Load and preprocess fashion dataset fashion_data = keras.datasets.fashion_mnist (train_images, train_labels), (test_images, test_labels) = fashion_data.load_data() # Normalize the images train_images = train_images / 255.0 test_images = test_images / 255.0 # Build a simple neural network model = keras.Sequential([ layers.Flatten(input_shape=(28, 28)), layers.Dense(128, activation='relu'), layers.Dense(10, activation='softmax') ]) # Compile and train the model model.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy']) model.fit(train_images, train_labels, epochs=5) # Evaluate the model test_loss, test_acc = model.evaluate(test_images, test_labels, verbose=2) print('nTest accuracy:', test_acc)
Moreover, augmented reality (AR) and virtual reality (VR) are also poised to reshape the fashion landscape. Using Python with libraries like OpenCV, brands can create immersive shopping experiences that allow customers to visualize clothing on themselves without physically trying them on. This not only enhances the shopping experience but also reduces return rates, a significant issue within the industry.
import cv2 # Load the image of the customer customer_image = cv2.imread('customer.jpg') # Load the garment overlay image garment_overlay = cv2.imread('garment.png') # Function to apply the garment overlay on the customer's image def apply_garment_overlay(customer_img, garment_img, position): # Resize garment to fit the target position garment_resized = cv2.resize(garment_img, (100, 100)) # Example size customer_img[position[1]:position[1]+100, position[0]:position[0]+100] = garment_resized return customer_img # Example usage position = (50, 50) # Define the position on the customer's image final_image = apply_garment_overlay(customer_image, garment_overlay, position) # Display the final image cv2.imshow('Fashion Overlay', final_image) cv2.waitKey(0) cv2.destroyAllWindows()
Additionally, blockchain technology is gaining traction in the fashion industry for supply chain transparency and authenticity verification. Python can facilitate the development of decentralized applications (dApps) that track the provenance of materials, ensuring ethical sourcing and production practices. This not only builds consumer trust but also aligns with the growing demand for sustainability in fashion.
# Pseudocode for a simple blockchain implementation class Block: def __init__(self, index, previous_hash, timestamp, data, hash): self.index = index self.previous_hash = previous_hash self.timestamp = timestamp self.data = data self.hash = hash def create_genesis_block(): return Block(0, "0", "01/01/2023", "Genesis Block", "hash") def create_new_block(previous_block, data): index = previous_block.index + 1 timestamp = "now" # Simplified for demonstration hash = "new_hash" # Typically calculated using SHA256 return Block(index, previous_block.hash, timestamp, data, hash) # Example usage genesis_block = create_genesis_block() new_block = create_new_block(genesis_block, "Fashion Item Data") print(f"New Block Hash: {new_block.hash}")
To wrap it up, the future of fashion innovation is ripe with possibilities as Python continues to play a pivotal role in bridging technology and creativity. By embracing advancements in AI, AR/VR, and blockchain, the fashion industry can not only enhance operational efficiency but also deliver captivating consumer experiences that resonate with modern values and expectations. In this digital age, the synergy between technology and fashion will undoubtedly pave the way for the next wave of groundbreaking transformations.