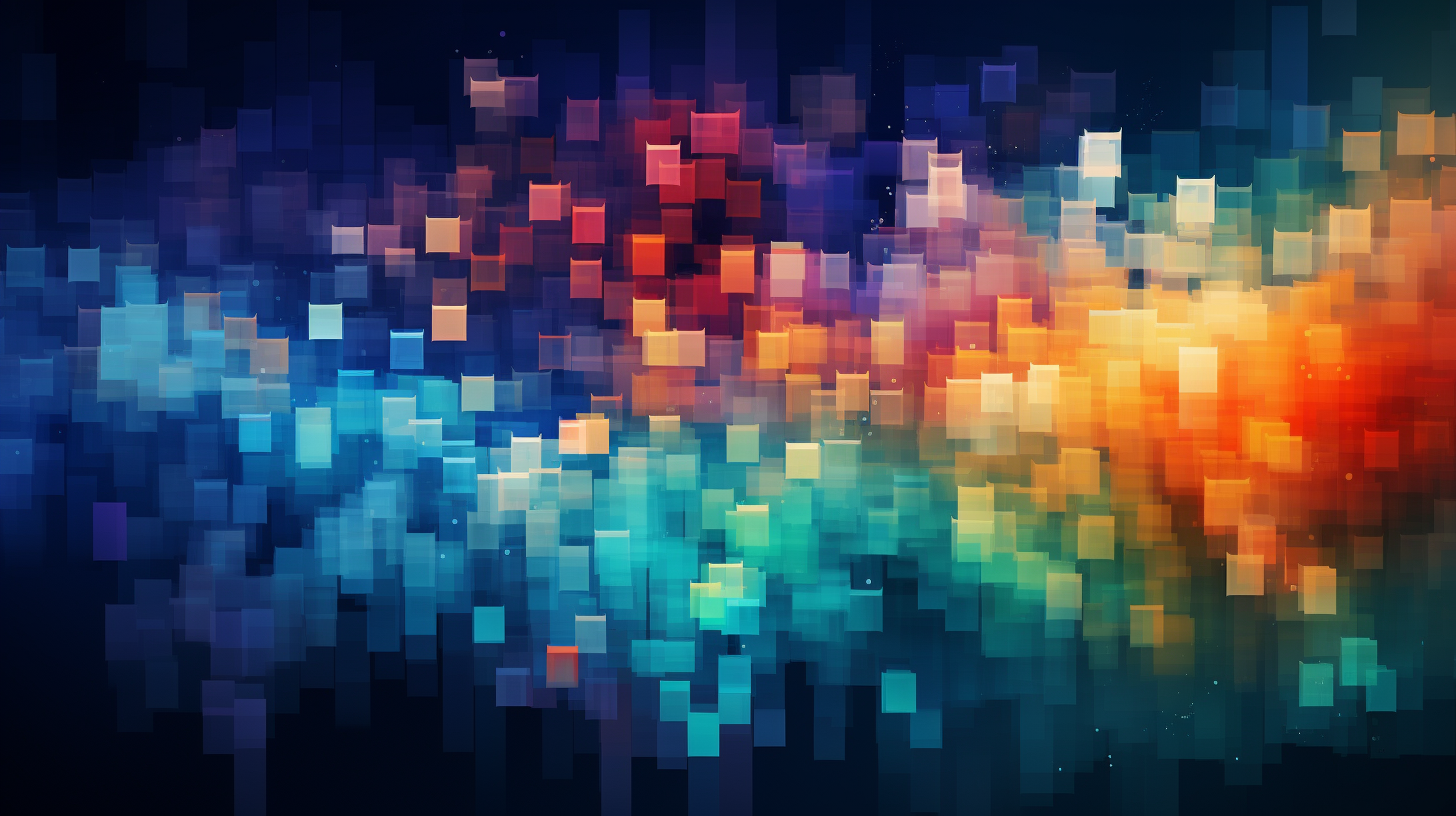
Python and Geospatial Data: Libraries and Tools
Geospatial data refers to information that is associated with a specific location on the Earth’s surface. In Python, understanding how to work with geospatial data is essential for various applications ranging from urban planning to environmental monitoring. This data can exist in multiple formats, such as vector data (points, lines, polygons) and raster data (grids, images). Each format has its own characteristics and use cases.
Vector data represents discrete objects and their attributes, while raster data represents continuous data across a geographical area. The representation of geospatial data allows us to store multi-dimensional information, which can be crucial for analysis and visualization.
One of the foundational aspects of working with geospatial data in Python is understanding coordinate reference systems (CRS). The CRS defines how the two-dimensional, projected map in your GIS relates to real places on the earth. Many geospatial libraries make it easier to manage these systems, allowing for transformations between different coordinate systems.
To illustrate the concept, let’s use the geopandas
library, which extends the functionality of Pandas to allow spatial operations on geometric types. Below is an example of how to read a shapefile and explore its basic properties:
import geopandas as gpd # Load a shapefile gdf = gpd.read_file('path/to/your/shapefile.shp') # Display the first few rows of the GeoDataFrame print(gdf.head()) # Display the coordinate reference system (CRS) print(gdf.crs)
In this example, we use the read_file
method to load a shapefile which contains vector data. The GeoDataFrame
behaves similarly to a traditional DataFrame but includes a geometry column that contains shapes. Understanding these fundamental components is important for geospatial data manipulation.
Furthermore, the analysis of geospatial data often necessitates performing spatial operations. You might want to calculate distances, intersections, or areas. The shapely
library complements geopandas
by allowing for advanced geometric manipulations. For example, to calculate the area of geometries in the GeoDataFrame:
# Calculate area of geometries gdf['area'] = gdf.geometry.area # Display the area of the first few geometries print(gdf[['geometry', 'area']].head())
Understanding geospatial data in Python also involves knowledge of underlying data formats such as GeoJSON, KML, and various raster formats, which can be handled effectively using libraries like rasterio
and fiona
. These libraries provide the necessary tools to read, write, and manipulate geospatial data files, thus creating a robust ecosystem for geospatial analysis.
Key Libraries for Geospatial Analysis
The world of geospatial analysis in Python is richly populated with a variety of powerful libraries designed to simplify the handling and processing of geospatial data. Each library serves a unique purpose and can be combined to create a comprehensive workflow that tackles complex geospatial challenges.
One of the key libraries is Geopandas, which builds on the capabilities of Pandas to facilitate spatial data manipulation. It allows you to visualize and analyze geospatial data in a familiar DataFrame format. Geopandas supports a variety of geospatial operations, such as merging, spatial indexing, and plotting. With Geopandas, you can easily manipulate geometries and perform operations like buffering and spatial joins.
import geopandas as gpd # Load a GeoDataFrame gdf1 = gpd.read_file('path/to/first_shapefile.shp') gdf2 = gpd.read_file('path/to/second_shapefile.shp') # Perform a spatial join between two GeoDataFrames joined_gdf = gpd.sjoin(gdf1, gdf2, how="inner", op="intersects") # Display the results print(joined_gdf.head())
Another noteworthy library is Shapely, which provides geometric objects and operations. Shapely allows for the creation of complex geometries and the execution of geometric operations like union, intersection, and difference. This library is essential for tasks that require precise geometric calculations.
from shapely.geometry import Point, Polygon # Create a Point and a Polygon point = Point(1, 1) polygon = Polygon([(0, 0), (2, 0), (2, 2), (0, 2)]) # Check if the point is within the polygon is_within = point.within(polygon) print(f'Point is within polygon: {is_within}') # Output: True
For raster data manipulation, Rasterio is the go-to library. It’s designed to read and write raster datasets and provides an interface for performing operations like cropping, masking, and transforming raster data. Rasterio works seamlessly with NumPy arrays, allowing for efficient data manipulation and analysis.
import rasterio # Open a raster file with rasterio.open('path/to/raster.tif') as src: # Read the data into a NumPy array raster_data = src.read(1) # Read the first band # Display raster data dimensions print(raster_data.shape)
Additionally, Fiona is a library that serves as a lightweight way to handle file formats and metadata in geospatial data. It allows for reading and writing spatial data in various formats, facilitating workflows that require interoperability between different geospatial data formats.
import fiona # Read a shapefile using Fiona with fiona.open('path/to/shapefile.shp') as src: # Print the schema and crs info print(src.schema) print(src.crs)
Finally, to enhance the visualization capabilities of your geospatial data, libraries like Matplotlib and Folium can be employed. Matplotlib is excellent for creating static plots, while Folium makes web mapping simpler, allowing for interactive maps to visualize geospatial data effectively.
import folium # Create a map centered at a specific latitude and longitude m = folium.Map(location=[45.5236, -122.6750], zoom_start=13) # Add a marker folium.Marker([45.5236, -122.6750], tooltip='Click for more info').add_to(m) # Save map to an HTML file m.save('map.html')
The Python ecosystem provides a suite of libraries tailored for geospatial analysis, each contributing to a robust framework for processing and visualizing geospatial data. Mastery of these tools very important for any geospatial analyst looking to leverage the power of Python in their projects.
Data Visualization Techniques for Geospatial Data
Data visualization techniques are fundamental when working with geospatial data in Python. The ability to visually represent geographical information not only aids in understanding complex datasets but also facilitates communication of insights to stakeholders. In Python, there are several libraries designed to visualize geospatial data effectively, each with its own strengths and specializations.
Matplotlib is one of the most widely used libraries for data visualization in Python, and it can be extended to visualize geospatial data. When combined with GeoPandas, you can create informative visualizations of geographical shapes and associated attributes directly from GeoDataFrames. Here’s an example demonstrating how to plot a GeoDataFrame:
import geopandas as gpd import matplotlib.pyplot as plt # Load a GeoDataFrame gdf = gpd.read_file('path/to/your/shapefile.shp') # Plot the GeoDataFrame gdf.plot(column='attribute_name', cmap='viridis', legend=True) plt.title('Geospatial Data Visualization') plt.xlabel('Longitude') plt.ylabel('Latitude') plt.show()
In this example, we load a GeoDataFrame from a shapefile and use the plot method to visualize the geometries colored by a specified attribute. The cmap
parameter allows you to choose a color map, and the legend
parameter enables a legend for better interpretability.
For more interactive visualizations, Folium is an excellent tool that leverages the Leaflet.js library. Folium allows you to create interactive maps easily, which can display markers, choropleth maps, and other layers that can be manipulated by end users. Here’s an example of how to create a simple interactive map with Folium:
import folium # Create a base map m = folium.Map(location=[45.5236, -122.6750], zoom_start=13) # Add a circle marker folium.CircleMarker(location=[45.5236, -122.6750], radius=50, color='blue', fill=True).add_to(m) # Add a choropleth layer folium.Choropleth( geo_data='path/to/geojson_file.geojson', data=gdf, columns=['attribute_name', 'value'], key_on='feature.properties.attribute_name', fill_color='YlGn', fill_opacity=0.6, line_opacity=0.2, ).add_to(m) # Save the map to an HTML file m.save('map.html')
In this snippet, we create a map centered at given coordinates and add a circle marker. The choropleth layer visualizes data from a GeoJSON file, allowing users to see variations across a geographical area based on attribute values. This interactive approach not only enhances user engagement but also provides a more informative visual experience.
Another technique for visualizing geospatial data is to use Plotly, which provides extensive support for interactive plots, including geographical visualizations. Plotly allows you to create sophisticated visualizations with minimal code. Here’s an example of using Plotly for a scatter map:
import plotly.express as px # Create a scatter map fig = px.scatter_geo(gdf, lat='latitude_column', lon='longitude_column', color='attribute_name', hover_name='hover_info_column', size='size_column', projection='natural earth') fig.update_layout(title='Geospatial Scatter Map') fig.show()
In this example, we use scatter_geo
from Plotly Express to create a scatter map where points are plotted based on latitude and longitude data. Various attributes are included to enrich the visualization, such as hover information and size scaling based on another attribute. This interactivity allows users to engage with the data more dynamically.
Choosing the right visualization technique depends on the specific requirements of your data and the insights you wish to convey. With the wealth of libraries available, Python provides powerful tools to bring geospatial data to life, enabling analysts to uncover patterns, trends, and insights effectively.
Working with Geographic Information Systems (GIS)
When working with Geographic Information Systems (GIS) in Python, the integration of geospatial data manipulation and analysis tools is paramount. GIS platforms are designed to capture, store, manipulate, analyze, manage, and present spatial or geographic data. In Python, several libraries provide the necessary functionality to work with GIS data effectively, enabling users to perform spatial queries, analyze relationships, and visualize data.
One of the most powerful libraries in this domain is Geopandas, which allows for easy manipulation of geospatial data in a Pandas-like DataFrame. Geopandas extends the functionalities of traditional Pandas by incorporating geometric data types, making it possible to conduct spatial operations seamlessly. For example, you can easily read, write, and manipulate common vector formats such as shapefiles and GeoJSON.
import geopandas as gpd # Load a shapefile into a GeoDataFrame gdf = gpd.read_file('path/to/your/shapefile.shp') # Display basic information about the GeoDataFrame print(gdf.info())
In addition to data loading, Geopandas facilitates spatial indexing, allowing for efficient querying of spatial data. For instance, you can filter a GeoDataFrame to include only those geometries that fall within a specific bounding box, which is particularly useful for preprocessing data before analysis.
# Define a bounding box bbox = gdf.total_bounds # [minx, miny, maxx, maxy] # Filter the GeoDataFrame based on the bounding box filtered_gdf = gdf.cx[bbox[0]:bbox[2], bbox[1]:bbox[3]] # Display the filtered GeoDataFrame print(filtered_gdf.head())
Another essential library for GIS work is Shapely, which provides a set of geometric operations that can be applied to the geometries within a GeoDataFrame. For example, calculating the intersection of two spatial features can help determine shared areas:
from shapely.geometry import Point, Polygon # Define two geometries poly = Polygon([(0, 0), (1, 1), (1, 0), (0, 0)]) point = Point(0.5, 0.5) # Check for intersection intersection = poly.intersection(point) print(f'Intersection: {intersection}') # Output: POINT (0.5 0.5)
Spatial joins are another critical operation in GIS analysis. Using Geopandas’ spatial join function, you can merge two GeoDataFrames based on their geometries, thus enriching your data with relevant information from other datasets:
# Load a second GeoDataFrame gdf2 = gpd.read_file('path/to/second_shapefile.shp') # Perform a spatial join joined_gdf = gpd.sjoin(gdf, gdf2, how="inner", op="intersects") # Display the results print(joined_gdf.head())
Moreover, for raster data analysis, the Rasterio library is instrumental. It provides an interface for reading and writing raster datasets, enabling users to manipulate pixel data efficiently. Rasterio complements Geopandas and Shapely when dealing with different types of geospatial data:
import rasterio # Open a raster file with rasterio.open('path/to/raster.tif') as src: raster_data = src.read(1) # Read the first band # Display raster data dimensions print(f'Raster dimensions: {raster_data.shape}') # Provides dimensions of the raster
To visualize geospatial data effectively, integration with libraries such as Matplotlib and Folium can enhance the presentation of spatial analysis results. Folium, in particular, allows for the creation of interactive maps that can be embedded in web applications, providing a dynamic way to explore GIS data.
import folium # Create an interactive map m = folium.Map(location=[45.5236, -122.6750], zoom_start=13) # Add a marker to the map folium.Marker([45.5236, -122.6750], tooltip='Oregon').add_to(m) # Save the map to an HTML file m.save('map.html')
This combination of libraries and tools empowers users to perform comprehensive GIS analyses in Python, allowing for sophisticated data manipulation, analysis, and visualization. The ability to work with both vector and raster types, coupled with the capacity for spatial operations and effortless to handle visualizations, makes Python a formidable choice for GIS applications.
Integrating Python with Remote Sensing Data
Integrating Python with remote sensing data opens a world of possibilities for analyzing and interpreting images captured from satellites and aerial platforms. Remote sensing data is often represented in raster format, which contains pixel values that correspond to measurements of the Earth’s surface, such as temperature, vegetation indices, or land cover classifications. With Python, you can harness powerful libraries to process, analyze, and visualize this data effectively.
One of the key libraries for working with raster data in Python is Rasterio. Rasterio provides tools for reading and writing raster datasets, allowing you to manipulate pixel data with ease. It integrates seamlessly with NumPy, allowing for efficient data handling. Below is an example showing how to read a raster file and access its metadata:
import rasterio # Open a raster file with rasterio.open('path/to/remote_sensing_image.tif') as src: # Read the data into a NumPy array raster_data = src.read(1) # Read the first band # Access metadata print(f'Raster dimensions: {src.width} x {src.height}') print(f'Coordinate reference system: {src.crs}') print(f'Bounds: {src.bounds}') # Print the geographical bounds of the image
Once the data is loaded, you can perform various analyses, such as calculating vegetation indices. One common index is the Normalized Difference Vegetation Index (NDVI), which is calculated using the near-infrared (NIR) and red bands of the image. This index is useful for assessing vegetation health. Here’s how you can compute NDVI:
import numpy as np # Assuming you have read the NIR and Red bands with rasterio.open('path/to/nir_band.tif') as nir_src: nir = nir_src.read(1) with rasterio.open('path/to/red_band.tif') as red_src: red = red_src.read(1) # Calculate NDVI ndvi = (nir - red) / (nir + red) # Save the NDVI result to a new raster file with rasterio.open('path/to/ndvi_result.tif', 'w', driver='GTiff', height=ndvi.shape[0], width=ndvi.shape[1], count=1, dtype=ndvi.dtype, crs=nir_src.crs, transform=nir_src.transform) as dst: dst.write(ndvi, 1)
In addition to Rasterio, the GDAL library is another powerful tool for handling remote sensing data. GDAL (Geospatial Data Abstraction Library) can read various raster and vector formats, and it’s often used for preprocessing steps such as reprojecting or resampling images. Here’s an example of using GDAL to open a raster file and perform a basic operation:
from osgeo import gdal # Open a raster file using GDAL dataset = gdal.Open('path/to/remote_sensing_image.tif') band = dataset.GetRasterBand(1) # Get the first band # Read the band into a NumPy array data = band.ReadAsArray() # Perform a simple operation, e.g., calculating the mean value mean_value = np.mean(data) print(f'Mean value of the raster band: {mean_value}')
For visualization, combining raster data with geospatial libraries such as Matplotlib and Folium allows for effective representation of the analyzed data. Here’s how to visualize the NDVI output using Matplotlib:
import matplotlib.pyplot as plt # Visualize the NDVI plt.imshow(ndvi, cmap='RdYlGn') # Use a color map that represents vegetation plt.colorbar(label='NDVI Value') plt.title('Normalized Difference Vegetation Index (NDVI)') plt.xlabel('Pixel Column') plt.ylabel('Pixel Row') plt.show()
Furthermore, if you want to create an interactive map to display remote sensing results, Folium is an excellent option. You can overlay raster data on a Leaflet map, enhancing the depth of analysis:
import folium from folium.raster_layers import ImageOverlay # Create a base map m = folium.Map(location=[latitude, longitude], zoom_start=10) # Add NDVI overlay using ImageOverlay ndvi_image = 'path/to/ndvi_result.tif' image_overlay = ImageOverlay(image=ndvi_image, bounds=[[south, west], [north, east]], opacity=0.6) image_overlay.add_to(m) # Save the map to an HTML file m.save('ndvi_map.html')
By integrating Python with remote sensing data, analysts can leverage these libraries to conduct complex spatial analyses, visualize results effectively, and derive meaningful insights from large datasets. The ability to manipulate and interpret remote sensing data especially important for applications in agriculture, environmental monitoring, and urban planning, making Python an invaluable tool in the geospatial analyst’s toolkit.
Best Practices for Geospatial Data Management and Processing
When it comes to managing and processing geospatial data, following best practices is paramount to ensure data integrity, efficiency, and reproducibility in your analyses. The complexities of geospatial data, with its varying formats and structures, necessitate a disciplined approach to data management. Here are some best practices to think when working with geospatial data in Python:
1. Data Organization: Keeping your data organized is vital. Use a consistent directory structure to separate raw data, processed data, and output files. This practice not only aids in efficient data handling but also enhances reproducibility. For instance, you might create directories such as data/raw
, data/processed
, and outputs/maps
.
2. Use of Version Control: Employ version control systems like Git to track changes to your scripts and data processing workflows. Versioning your datasets can be particularly useful when dealing with iterative analyses. This setup allows you to revert back to earlier states if necessary, providing a safety net for your work.
3. Metadata Management: Maintain comprehensive metadata for your datasets. Metadata should include information about the source of the data, collection methods, date of acquisition, and any preprocessing steps taken. The pandas
library can help in creating DataFrames to store and manage metadata effectively:
import pandas as pd # Create a DataFrame to store metadata metadata = { 'filename': ['shapefile.shp', 'raster.tif'], 'source': ['OpenStreetMap', 'Sentinel-2'], 'acquisition_date': ['2023-01-15', '2023-03-20'], 'description': ['Road network data', 'Satellite imagery'] } metadata_df = pd.DataFrame(metadata) print(metadata_df)
4. Data Format Standardization: Standardize the formats of your geospatial data. Convert files to widely accepted formats (like GeoJSON for vector data and GeoTIFF for raster data) to ensure compatibility across different libraries and tools. This practice minimizes issues related to format discrepancies.
5. Efficient Data Handling: When working with large geospatial datasets, loading entire files into memory can be inefficient. Utilize libraries like Dask
to manage large datasets in chunks, or use GeoPandas
‘ capabilities to perform operations directly on disk when possible.
6. Spatial Indexing: Implement spatial indexing for faster spatial queries. Libraries like GeoPandas
automatically handle indexing for geometries, which can drastically increase the speed of spatial operations. Here’s an example of how to set up a spatial index:
import geopandas as gpd # Load a GeoDataFrame gdf = gpd.read_file('path/to/shapefile.shp') # Create a spatial index gdf.sindex # Access the spatial index for efficient spatial queries
7. Regular Backups: Regularly back up your data, especially when working with critical datasets. Utilize cloud storage solutions or external drives to store copies of your work. This redundancy protects against data loss due to accidental deletions or hardware failures.
8. Documentation and Comments: Document your code thoroughly. Use comments to explain the purpose of sections of code, especially complex operations. Documentation not only aids your future self but also makes it easier for collaborators to understand your workflow.
9. Automation of Workflows: Automate repetitive tasks using scripts. Libraries such as Make
or Snakemake
can help design reproducible workflows. Automation reduces the risk of human error and increases efficiency when processing and analyzing data.
10. Testing and Validation: Implement testing frameworks to validate your code and ensure that your data processing scripts function as intended. Use libraries like pytest
to conduct unit tests on your functions, ensuring your analyses yield expected results.
By adhering to these best practices in geospatial data management and processing, you can enhance the quality and reliability of your analyses. Python’s rich ecosystem of libraries facilitates these practices, so that you can focus on deriving insights from your geospatial datasets.