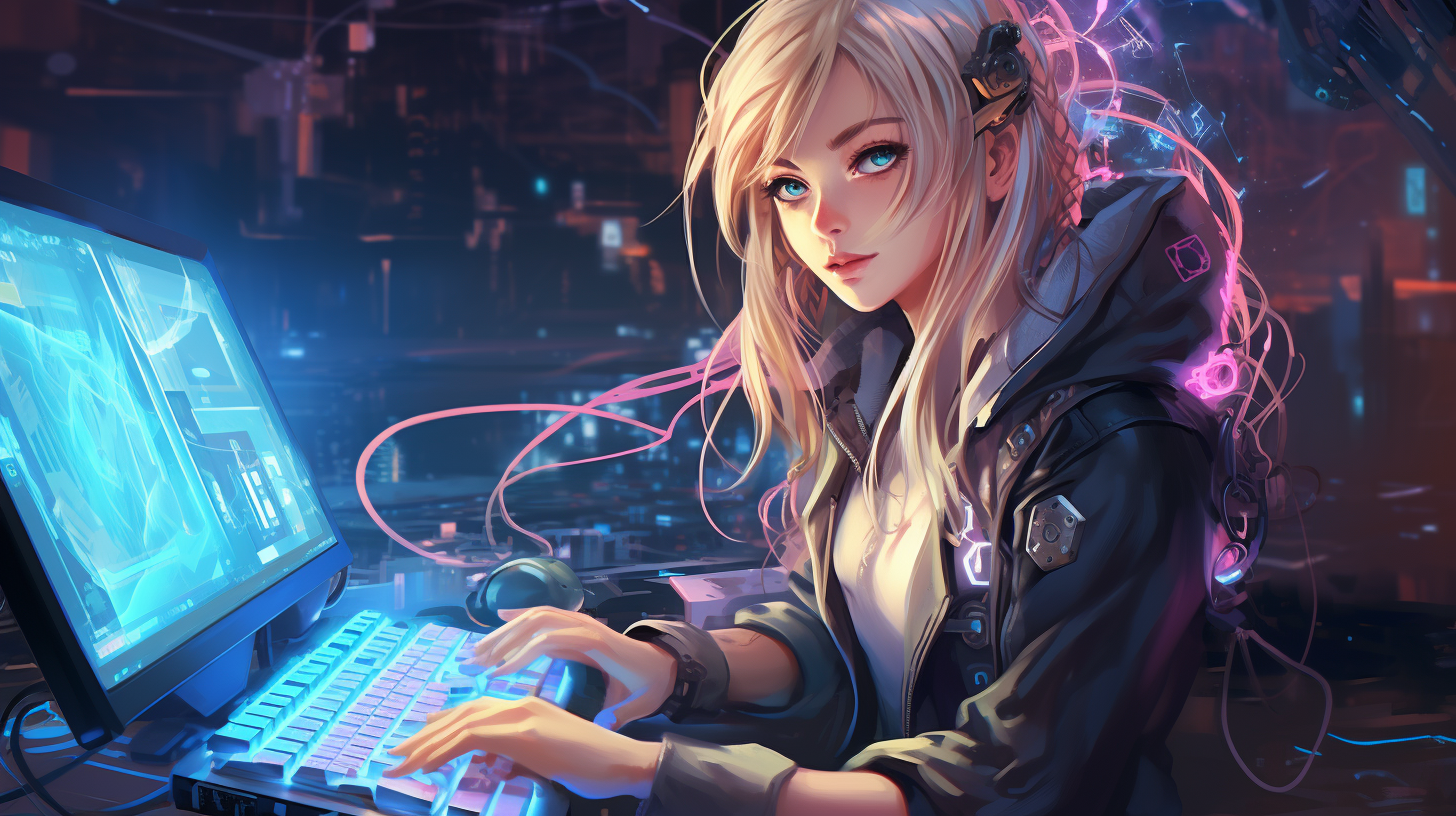
Python and Legal Tech: Innovations and Applications
Python has emerged as a pivotal programming language in the sphere of legal technology, distinguished by its simplicity and versatility. This dynamic language facilitates the development of tools that streamline tedious legal processes, enabling legal professionals to focus on more complex tasks. One of the primary advantages of Python is its extensive library ecosystem, which provides pre-built functions and modules that cater to various legal tech applications.
For instance, Python’s rich selection of libraries—such as Flask for web development and Pandas for data manipulation—allows developers to create robust applications efficiently. This capacity for rapid development is important in the legal field, where the demand for innovative solutions often outpaces the traditional methods that have long been in place.
Moreover, Python’s strong community support and well-documented resources make it easier for legal tech startups and established firms alike to adopt and integrate this language into their technology stacks. By using Python, legal technology developers can build applications that automate document management, enhance client relationship management, and facilitate case tracking, ultimately transforming how legal services are delivered.
Ponder a scenario where a law firm seeks to automate the generation of legal documents. Using Python, a developer can craft a simple script that takes input data and fills in a template, significantly reducing the time spent on these repetitive tasks.
from docx import Document def create_legal_document(client_name, case_details): doc = Document() doc.add_heading('Legal Document', level=1) doc.add_paragraph(f'Client Name: {client_name}') doc.add_paragraph(f'Case Details: {case_details}') doc.save(f'{client_name}_legal_document.docx') # Example usage create_legal_document('Luke Douglas', 'Breach of Contract')
This simple function illustrates the ease with which Python can be utilized to automate the otherwise cumbersome task of document creation. By using the power of Python, legal practitioners not only gain efficiency but also improve accuracy, thereby minimizing the potential for human error.
As the legal technology landscape continues to evolve, the role of Python will undoubtedly grow more significant. Its ability to adapt and integrate with other technologies ensures that it remains at the forefront of innovations aimed at enhancing legal processes and delivering value to clients.
Automation of Legal Processes through Python
Automation takes many forms within the legal industry, allowing firms to streamline workflows and reduce overhead costs. Beyond simple document generation, Python can be employed in various automation scenarios, such as managing client communications, tracking billable hours, and even conducting legal research. The automation of these processes not only accelerates workflows but also allows legal professionals to allocate their time and resources more effectively, focusing on high-value activities.
One of the critical areas where Python shines is in the automation of client communications. For instance, using libraries like smtplib and email , legal tech developers can create scripts to automatically send personalized emails to clients. This functionality can be particularly useful for sending reminders about upcoming court dates or deadlines.
import smtplib from email.mime.text import MIMEText def send_reminder_email(client_email, court_date): msg = MIMEText(f'Reminder: Your court date is on {court_date}.') msg['Subject'] = 'Court Date Reminder' msg['From'] = '[email protected]' msg['To'] = client_email with smtplib.SMTP('smtp.example.com') as server: server.login('your_username', 'your_password') server.send_message(msg) # Example usage send_reminder_email('[email protected]', '2023-10-30')
This code snippet demonstrates how easily Python can automate the process of sending reminder emails, enabling firms to maintain communication with clients without manual intervention. The automation not only saves time but also enhances client satisfaction by ensuring that clients receive timely notifications.
Another area where Python excels is in time tracking and billing automation. By integrating with project management APIs, developers can create systems that log billable hours automatically based on tasks completed. This capability can significantly reduce inaccuracies in billing and improve transparency between the legal firm and its clients.
import requests from datetime import datetime def log_billable_hours(task_name, hours, client_id): url = 'https://api.time-tracking.com/log' data = { 'client_id': client_id, 'task_name': task_name, 'hours': hours, 'date': datetime.now().isoformat() } response = requests.post(url, json=data) return response.status_code # Example usage log_billable_hours('Research Case Law', 3, 'client123')
In this example, a simple function logs billable hours to a time-tracking API, ensuring that all work is accounted for accurately. Automating this process not only minimizes errors but also provides a clear record of how legal resources are allocated, which can be invaluable during audits or client reviews.
Furthermore, Python can facilitate legal research through automation. By using libraries such as Beautiful Soup and Scrapy , developers can create web scrapers that retrieve and compile relevant case law or legal articles from various online resources. This capability can significantly enhance the efficiency of legal research, allowing attorneys to access pertinent information quickly.
import requests from bs4 import BeautifulSoup def scrape_case_law(url): response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') cases = soup.find_all(class_='case-entry') return [case.text for case in cases] # Example usage case_law_entries = scrape_case_law('https://www.caselawwebsite.com') print(case_law_entries)
This script retrieves case law entries from a specified URL, demonstrating how Python can be employed to automate the gathering of legal information. With such automation, legal professionals can stay updated with the latest developments in their areas of practice, empowering them to provide better-informed advice to clients.
As the legal sector continues its digital transformation, the role of Python in automating legal processes will only increase. By embracing this technology, legal practitioners can not only enhance their practice efficiency but also provide superior service to their clients.
Data Analysis and Visualization for Legal Insights
Data analysis and visualization are indispensable components of legal technology, enabling law firms and legal professionals to derive actionable insights from vast amounts of data. The legal field generates a staggering volume of information, from case law to client records, and Python offers powerful tools to analyze and visualize this data effectively. By using libraries such as Pandas, NumPy, and Matplotlib, legal tech developers can transform raw data into meaningful representations that can guide decision-making and strategy.
One of the primary functions of data analysis in legal tech is to examine trends within case outcomes, helping firms identify patterns in litigation or settlement results. For instance, a law firm might want to analyze how often certain types of cases are won in specific jurisdictions. By using Python, they can easily pull data from their case management systems, clean it, and perform insightful analyses.
import pandas as pd def analyze_case_outcomes(file_path): df = pd.read_csv(file_path) win_rate = df.groupby('jurisdiction')['outcome'].value_counts(normalize=True).unstack() return win_rate # Example usage win_rates = analyze_case_outcomes('case_data.csv') print(win_rates)
This function reads a CSV file containing case data, calculates the win rates by jurisdiction, and presents the results in a structured format. Such analysis can help law firms refine their strategies by identifying jurisdictions where they may perform better or need improvement.
Visualization further enhances the analysis by allowing legal professionals to see the data in a more digestible format. With Python’s Matplotlib and Seaborn libraries, firms can create visual representations of their analytical findings, making it easier to communicate insights to stakeholders.
import matplotlib.pyplot as plt import seaborn as sns def plot_win_rates(win_rates): win_rates.plot(kind='bar', stacked=True) plt.title('Win Rates by Jurisdiction') plt.xlabel('Jurisdiction') plt.ylabel('Win Rate') plt.legend(title='Outcome') plt.show() # Example usage plot_win_rates(win_rates)
This code snippet generates a bar chart that illustrates win rates across different jurisdictions, allowing firms to visualize their performance effectively. Such visualizations can be pivotal during strategic meetings or presentations, providing clear insights that guide future actions.
Moreover, Python can be leveraged to analyze client data, such as billing history and satisfaction metrics, to determine which factors contribute to successful client relationships. By employing techniques like customer segmentation or sentiment analysis, firms can tailor their services to meet client needs more effectively.
from sklearn.cluster import KMeans def segment_clients(data): kmeans = KMeans(n_clusters=3) data['segment'] = kmeans.fit_predict(data[['billing_amount', 'satisfaction_score']]) return data # Example usage client_data = pd.DataFrame({ 'billing_amount': [1000, 1500, 2000, 2500, 3000], 'satisfaction_score': [5, 4, 3, 4, 5] }) segmented_clients = segment_clients(client_data) print(segmented_clients)
This function applies K-means clustering to categorize clients based on their billing amounts and satisfaction scores. By identifying distinct client segments, firms can develop specialized marketing strategies and improve client engagement.
In addition to these applications, advanced visualization libraries like Plotly can further enhance the interactivity of data presentations, allowing legal professionals to explore datasets dynamically. With such capabilities, legal teams can create dashboards that provide real-time insights into their operations, improving transparency and facilitating data-driven decision-making.
import plotly.express as px def create_interactive_dashboard(data): fig = px.scatter(data, x='billing_amount', y='satisfaction_score', color='segment', title='Client Segmentation Dashboard') fig.show() # Example usage create_interactive_dashboard(segmented_clients)
This code snippet creates an interactive scatter plot using Plotly, enabling users to visualize and explore the relationship between billing amounts and satisfaction scores across different client segments. The flexibility of such visual tools allows legal practitioners to gain insights tailored to their specific inquiries.
By using the power of data analysis and visualization with Python, law firms and legal professionals can unlock significant value from their data. This capability not only leads to more informed decision-making but also enhances their ability to serve clients effectively, ultimately driving better outcomes in a highly competitive legal landscape.
Machine Learning Applications in Legal Tech
Machine learning is rapidly becoming a cornerstone in the development of legal technology, offering transformative capabilities that enhance efficiency, accuracy, and insight. Python, with its rich ecosystem of libraries and frameworks, is perfectly suited for implementing machine learning solutions in the legal sector. From predicting case outcomes to automating contract analysis, the applications of machine learning in legal tech are vast and impactful.
One of the most prominent applications of machine learning in the legal field is in predictive analytics. By analyzing historical case data, machine learning models can provide insights into the likelihood of success for various legal strategies. This predictive capability allows lawyers to make more informed decisions about which cases to take and how to approach them.
For instance, a law firm might employ logistic regression or decision tree algorithms to predict the outcome of cases based on various features such as jurisdiction, type of case, and previous rulings. The following Python code illustrates how to implement a simple logistic regression model using the scikit-learn
library:
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.linear_model import LogisticRegression from sklearn.metrics import accuracy_score # Load historical case data data = pd.read_csv('case_outcomes.csv') # Features and target variable X = data[['jurisdiction', 'case_type', 'previous_outcomes']] y = data['outcome'] # 1 for win, 0 for lose # Preprocessing: Convert categorical variables to numerical X = pd.get_dummies(X, columns=['jurisdiction', 'case_type'], drop_first=True) # Split the data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the model model = LogisticRegression() model.fit(X_train, y_train) # Make predictions y_pred = model.predict(X_test) accuracy = accuracy_score(y_test, y_pred) print(f'Model Accuracy: {accuracy:.2f}')
This example demonstrates how to preprocess data, train a logistic regression model, and evaluate its accuracy. By incorporating machine learning into their workflows, legal professionals can harness the power of data-driven predictions, thereby enhancing their strategic planning.
Another fascinating application of machine learning in legal tech is contract analysis. Legal documents often contain dense and complex language, making it challenging for attorneys to quickly extract relevant information. Using natural language processing (NLP) techniques, developers can build tools that automatically review contracts and flag important clauses, compliance issues, or risks.
The following Python code showcases how to use NLP for extracting entities from contract text using the spaCy
library:
import spacy # Load the NLP model nlp = spacy.load('en_core_web_sm') def extract_entities(contract_text): doc = nlp(contract_text) entities = [(entity.text, entity.label_) for entity in doc.ents] return entities # Example usage contract_text = "This contract is made between Frank McKinnon and Acme Corp. Effective date is January 1st, 2023." entities = extract_entities(contract_text) print(entities)
This code snippet processes a sample contract and extracts named entities such as persons and organizations. By automating the review process, legal professionals can save countless hours and mitigate the risk of overlooking critical information.
Machine learning also plays a vital role in e-discovery, where vast amounts of electronic data must be sifted to find relevant documents for litigation. Techniques such as clustering and classification can be employed to group documents by relevance or to identify privileged materials. The following example illustrates how to use K-means clustering for document categorization:
from sklearn.feature_extraction.text import TfidfVectorizer from sklearn.cluster import KMeans def cluster_documents(documents): vectorizer = TfidfVectorizer() X = vectorizer.fit_transform(documents) # Fit the K-means model kmeans = KMeans(n_clusters=5) # Assuming 5 clusters kmeans.fit(X) return kmeans.labels_ # Example usage documents = [ "Document about contract law.", "Analysis of tort cases.", "Guide to corporate law compliance.", "Litigation strategies for civil cases.", "Overview of criminal law procedures." ] labels = cluster_documents(documents) print(labels)
This function converts a list of documents into a TF-IDF matrix and applies K-means clustering to categorize them. Such methodologies can dramatically streamline the e-discovery process, significantly reducing the time and effort required to find pertinent information.
As machine learning continues to evolve, its potential applications in legal tech will expand, necessitating a skilled workforce adept in both law and technology. Python’s accessibility and the growing array of machine learning libraries position it as an ideal choice for legal tech developers looking to innovate and improve legal services. By using machine learning, law firms can not only enhance their operational efficiency but also provide better outcomes for their clients, ultimately reshaping the landscape of legal practice.
Future Trends: Python’s Impact on the Legal Industry
Looking ahead, the future of Python in the legal industry appears promising, with several key trends likely to influence how legal professionals operate and deliver services. As law firms and legal departments increasingly recognize the value of advanced technologies, Python’s role is expected to expand, driven by its adaptability and the ease with which it integrates with other systems.
One significant trend is the continued adoption of automation tools powered by Python. As legal professionals become more accustomed to using technology, the demand for solutions that can automate repetitive tasks will grow. This includes not only document generation and client communications but also more complex workflows such as case management and compliance tracking. Python’s libraries, like Celery for task queue management and SQLAlchemy for database interactions, will facilitate the creation of robust automation frameworks that can improve efficiency across various legal operations.
Furthermore, the integration of artificial intelligence into legal tech solutions is set to intensify. Python has become the de facto language for AI, thanks to its extensive libraries such as TensorFlow and PyTorch. Legal professionals will increasingly rely on machine learning algorithms to analyze case data, predict outcomes, and assist in legal research. The ability to build sophisticated models that learn from historical data will empower firms to make data-driven decisions, leading to improved case strategies and client outcomes.
Additionally, the emergence of legal analytics will reshape how firms approach litigation and settlement strategies. By using Python’s data analysis capabilities, firms can uncover insights from large datasets and historical case law. Legal analytics tools will facilitate trend analysis, helping firms identify patterns in litigation success rates, settlement amounts, and even judge behavior. This data-driven approach will enable lawyers to tailor their strategies based on empirical evidence rather than intuition alone.
import pandas as pd # Function to analyze litigation trends def analyze_litigation_trends(file_path): df = pd.read_csv(file_path) trends = df.groupby('case_type')['outcome'].value_counts(normalize=True).unstack() return trends # Example usage litigation_trends = analyze_litigation_trends('litigation_data.csv') print(litigation_trends)
This example demonstrates how firms can analyze litigation trends by case type, helping them make informed decisions about which cases to pursue based on historical outcomes.
Another area where Python’s impact will be felt is in compliance and regulatory technology. As regulations become increasingly complex, legal tech solutions will need to evolve to ensure firms adhere to compliance mandates. Python can facilitate the development of compliance monitoring tools that automatically assess regulatory requirements against current practices, thereby mitigating risks associated with non-compliance. Tools using Python libraries like Beautiful Soup and requests can easily scrape regulatory updates, providing firms with real-time insights into compliance changes.
import requests from bs4 import BeautifulSoup def scrape_regulatory_updates(url): response = requests.get(url) soup = BeautifulSoup(response.text, 'html.parser') updates = soup.find_all(class_='update-entry') return [update.text for update in updates] # Example usage updates = scrape_regulatory_updates('https://www.regulatorywebsite.com/updates') print(updates)
As firms seek to stay ahead of regulatory changes, Python will play an important role in developing tools that streamline compliance efforts and ensure timely responses to new legislation.
Moreover, the legal tech landscape will increasingly embrace cloud-based solutions, and Python’s compatibility with cloud platforms makes it an ideal choice for developing scalable applications. The shift toward cloud computing allows firms to deliver services more flexibly while reducing infrastructure costs. Python frameworks such as Django and Flask enable developers to create powerful web applications that can be deployed in the cloud, ensuring that legal professionals have access to critical tools and data from anywhere.
Lastly, the rise of remote work, accelerated by global events, will continue to influence legal practice. Python’s capabilities in building collaborative platforms will allow legal teams to work effectively in a distributed environment. Tools that facilitate virtual collaboration, secure document sharing, and real-time communication will empower legal professionals to maintain productivity regardless of their physical location.
The integration of Python into the legal industry is set to deepen as trends such as automation, AI, legal analytics, compliance technology, and cloud computing gain traction. By using Python’s versatility and power, legal professionals will be better equipped to navigate the complexities of modern legal practice, ultimately enhancing their ability to serve clients and adapt to an ever-evolving landscape.