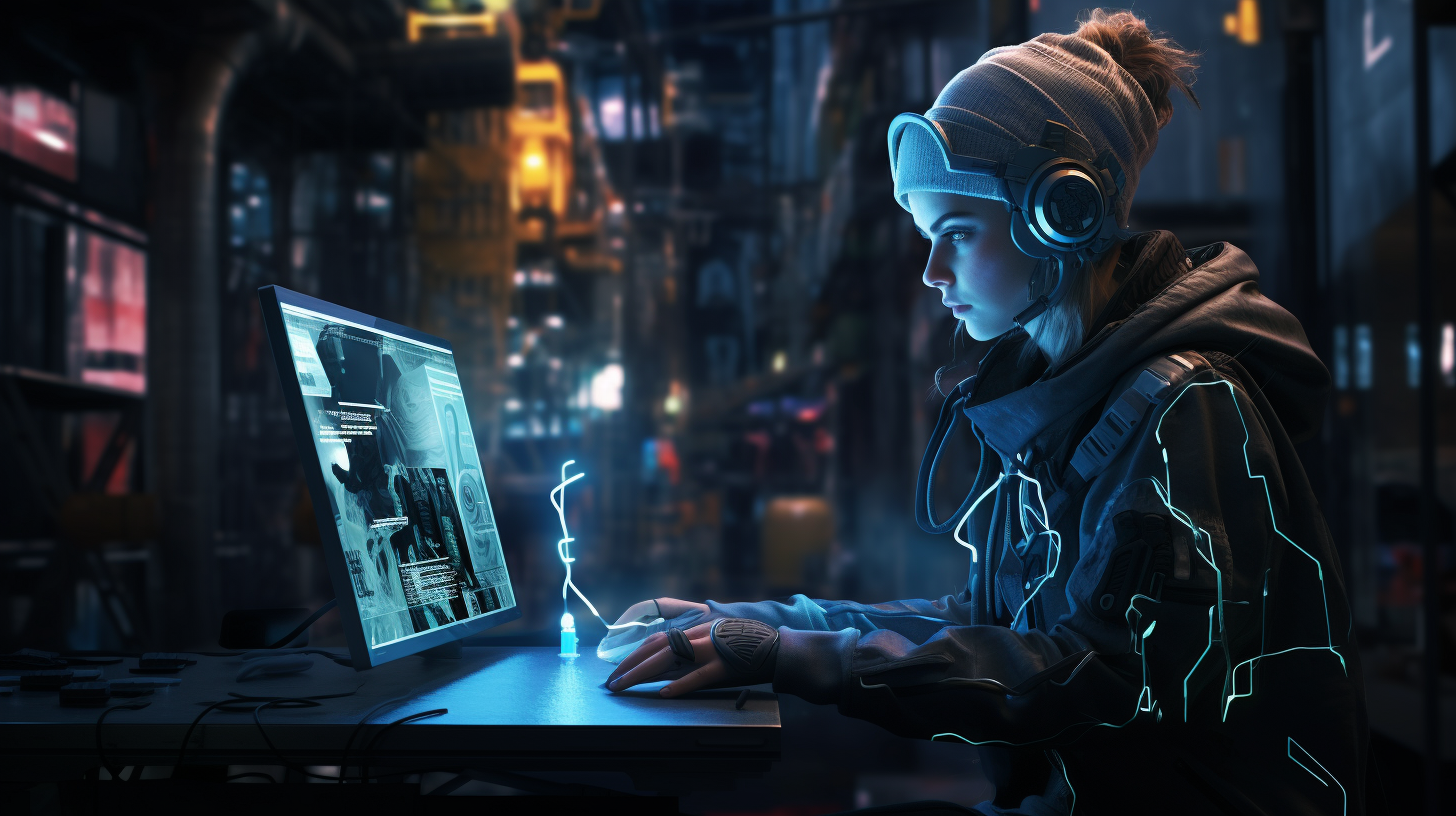
Python and Logistics: Streamlining Operations
In the bustling world of logistics, where every second counts and efficiency reigns supreme, Python emerges as a powerful ally. The versatility and ease of use that Python offers make it an unrivaled language in addressing the multifaceted challenges faced by modern logistics operations. From automating mundane tasks to performing complex data analyses, Python’s extensive libraries and frameworks provide the necessary tools to navigate the intricate web of supply chain management.
One of the most compelling features of Python is its rich ecosystem of libraries tailored for data manipulation and analysis. Libraries such as Pandas and NumPy allow logistics professionals to handle vast amounts of data with grace. With Pandas, for instance, one can freely import, clean, and manipulate datasets, which is essential for effective decision-making in logistics.
import pandas as pd # Loading a dataset data = pd.read_csv('supply_chain_data.csv') # Displaying the first few rows of the dataset print(data.head()) # Simple data manipulation example data['Delivery Time'] = data['Delivery Date'] - data['Order Date'] average_delivery_time = data['Delivery Time'].mean() print(f'Average Delivery Time: {average_delivery_time}')
Moreover, Python’s capabilities extend beyond data handling to include visualization. Libraries like Matplotlib and Seaborn can turn complex data points into intuitive visual representations, making it easier for logistics managers to comprehend trends and insights at a glance. This ability to visualize data effectively can mean the difference between reactive and proactive decision-making.
import matplotlib.pyplot as plt import seaborn as sns # Assuming 'Delivery Times' is a column in the DataFrame plt.figure(figsize=(10, 6)) sns.histplot(data['Delivery Time'], bins=30, kde=True) plt.title('Distribution of Delivery Times') plt.xlabel('Delivery Time (days)') plt.ylabel('Frequency') plt.show()
Beyond mere analysis and visualization, Python shines in automation. Logistics processes often involve repetitive tasks that can drain resources and time. With Python, one can write scripts that automate these processes, be it generating reports, sending notifications, or even retrieving and processing data from external APIs. This automation not only speeds up operations but also reduces the likelihood of human error.
import requests # Example: Fetching data from an API response = requests.get('https://api.example.com/logistics_data') logistics_data = response.json() # Processing and displaying a summary of the data print(f'Total Shipments: {len(logistics_data)}')
Furthermore, Python’s compatibility with various platforms allows it to integrate with existing logistics management systems, enhancing their functionality without requiring a complete overhaul. This flexibility is pivotal in a landscape where businesses often rely on a mix of legacy systems and modern solutions.
Ultimately, Python is more than just a programming language in the logistics sector; it is a transformative tool that empowers companies to streamline their operations, enhance their analytical capabilities, and adapt to the ever-evolving demands of the supply chain landscape. By using Python’s potential, businesses not only optimize their processes but also position themselves for sustainable growth in a competitive market.
Data Analysis for Supply Chain Optimization
In the sphere of supply chain optimization, data analysis is the cornerstone of informed decision-making. Python, with its robust libraries, facilitates deep insights into logistics data that can significantly enhance operational efficiency. By using Python’s capabilities, organizations can dissect complex datasets, uncovering patterns that inform strategies for cost reduction, service improvement, and risk management.
One of the primary steps in data analysis is data cleaning and preprocessing. Real-world datasets often come laden with inaccuracies and missing values, which can skew results if not addressed. Python’s Pandas library offers tools to seamlessly handle these issues. For example, using built-in functions, analysts can quickly identify and fill missing values, ensuring the integrity of the data before analysis begins.
import pandas as pd # Load the dataset data = pd.read_csv('logistics_data.csv') # Check for missing values missing_values = data.isnull().sum() print(f'Missing Values:n{missing_values}') # Fill missing values with the mean for numerical columns data.fillna(data.mean(), inplace=True)
Once the data is clean, exploratory data analysis (EDA) can commence. EDA is important for understanding the underlying trends and relationships in the data. Python provides various statistical tools and visualization libraries that allow analysts to create meaningful representations of the data. By identifying correlations between different variables—such as delivery times and transportation methods—organizations can optimize their supply chain operations.
import seaborn as sns import matplotlib.pyplot as plt # Create a correlation matrix correlation_matrix = data.corr() plt.figure(figsize=(10, 8)) sns.heatmap(correlation_matrix, annot=True, fmt='.2f', cmap='coolwarm') plt.title('Correlation Matrix') plt.show()
This visualization serves as a powerful tool for logistics professionals, allowing them to quickly grasp the relationships between variables. For instance, if the analysis reveals a strong negative correlation between delivery times and certain routes, it prompts further investigation into route optimization strategies.
In addition to traditional analysis, Python can also facilitate advanced analytics techniques such as clustering and regression analysis. Clustering algorithms like K-Means can segment customers based on order patterns, enabling personalized logistics strategies that enhance customer satisfaction. Regression analysis, on the other hand, allows organizations to forecast demand based on historical data, a critical function in inventory management.
from sklearn.cluster import KMeans # Assuming 'Order Quantity' and 'Delivery Time' are relevant features features = data[['Order Quantity', 'Delivery Time']] kmeans = KMeans(n_clusters=3) data['Cluster'] = kmeans.fit_predict(features) # Visualize the clusters plt.scatter(data['Order Quantity'], data['Delivery Time'], c=data['Cluster'], cmap='viridis') plt.title('Customer Clustering') plt.xlabel('Order Quantity') plt.ylabel('Delivery Time') plt.show()
By clustering customers, logistics firms can tailor their services, ensuring that they meet diverse customer needs while optimizing resource allocation. Furthermore, predictive modeling, facilitated by libraries like Scikit-learn, allows for proactive decision-making by anticipating future trends based on historical data.
Ultimately, data analysis in logistics, powered by Python, transforms raw data into actionable insights. This analytical rigor informs everything from inventory levels to supplier partnerships, laying the groundwork for a responsive and efficient supply chain. As logistics continues to evolve, the capacity to analyze and act on data effectively will define leading organizations in the industry.
Automating Inventory Management with Python
In the intricate dance of inventory management, automation plays a pivotal role in ensuring that logistics operations run smoothly and efficiently. Python, with its robust capabilities, provides a powerful means to automate various aspects of inventory management, reducing human error and freeing up valuable resources for more strategic activities.
One of the primary tasks in inventory management is tracking stock levels. By employing Python scripts, businesses can maintain real-time visibility of inventory, enabling them to react swiftly to fluctuations in demand. Using a combination of Python’s libraries, such as Pandas and SQLite, one can create a simple yet effective inventory tracking system that automatically updates stock levels.
import pandas as pd import sqlite3 # Create a connection to the SQLite database conn = sqlite3.connect('inventory.db') # Load existing inventory data inventory_data = pd.read_sql_query('SELECT * FROM inventory', conn) # Function to update inventory def update_inventory(item_id, quantity_sold): global inventory_data inventory_data.loc[inventory_data['ItemID'] == item_id, 'StockLevel'] -= quantity_sold # Update the database inventory_data.to_sql('inventory', conn, if_exists='replace', index=False) # Example update update_inventory(101, 3) print(inventory_data)
This script connects to an SQLite database storing inventory data, allowing for seamless updates to stock levels. By automating this process, businesses can ensure that their inventory records are always accurate, thereby preventing stockouts or overstock situations that can be costly.
Another crucial component of inventory management automation is the generation of stock reports. Regular reporting is vital for understanding stock movement, identifying slow-moving items, and making informed purchasing decisions. By using Python, businesses can automate the generation of these reports, saving time and providing insights that drive better inventory practices.
def generate_stock_report(): report = inventory_data[['ItemID', 'ItemName', 'StockLevel', 'ReorderLevel']] low_stock_items = report[report['StockLevel'] < report['ReorderLevel']] return low_stock_items # Generate and display low stock report low_stock_report = generate_stock_report() print("Low Stock Items:n", low_stock_report)
This function extracts relevant information from the inventory dataset, highlighting items that require reordering. Automating the reporting process not only enhances operational efficiency but also empowers teams to make timely decisions that can mitigate risks associated with stock management.
Furthermore, Python’s ability to integrate with web frameworks allows businesses to create simple to operate dashboards for inventory monitoring. Using libraries like Flask or Django, developers can build applications that provide real-time insights into stock levels, order statuses, and supplier information. Such dashboards can be pivotal in enabling logistics teams to stay ahead of inventory challenges.
from flask import Flask, render_template app = Flask(__name__) @app.route('/inventory') def inventory_dashboard(): inventory_data = pd.read_sql_query('SELECT * FROM inventory', conn) return render_template('dashboard.html', tables=[inventory_data.to_html(classes='data')], titles=inventory_data.columns.values) if __name__ == '__main__': app.run(debug=True)
In this example, a simple Flask application serves an inventory dashboard, showcasing the data in a structured format. The ability to visualize inventory data through these dashboards allows stakeholders to quickly assess their inventory situation and take proactive steps as needed.
Moreover, as market demands fluctuate unpredictably, Python’s automation capabilities extend to managing reorder points and automating purchase orders. By setting thresholds for each item, logistics managers can automate the process of generating purchase orders when stock levels dip below a specified point, ensuring that replenishment occurs without manual intervention.
def reorder_items(): reorder_items = inventory_data[inventory_data['StockLevel'] < inventory_data['ReorderLevel']] for index, row in reorder_items.iterrows(): print(f"Reorder item: {row['ItemName']} - Quantity: {row['ReorderLevel'] - row['StockLevel']}") # Trigger the reorder function reorder_items()
This function assesses the inventory data and identifies items that need to be reordered, effectively automating a critical aspect of inventory management. By implementing such automation, businesses can not only save time but also ensure that they meet customer demands without interruptions.
The automation of inventory management through Python is not merely a convenience; it is a necessity in the modern logistics landscape. By streamlining these processes, organizations can achieve greater accuracy, enhance responsiveness, and ultimately improve their operational efficiency. The ability to automate tasks allows logistics professionals to focus on strategic planning and continuous improvement, thereby solidifying their competitive edge in an increasingly complex marketplace.
Real-time Tracking and Monitoring Solutions
In the sphere of logistics, where timely decisions can mean the difference between success and failure, real-time tracking and monitoring solutions are essential. Python, with its extensive libraries and frameworks, provides the means to implement these solutions effectively. The capability to track shipments and inventory in real-time allows logistics professionals to respond promptly to any issues that may arise during the supply chain process.
One of the foundational steps in establishing a real-time tracking system is the integration of GPS data. Python’s ability to handle APIs and process data from various sources can be leveraged to obtain real-time location information of shipments. For instance, using the popular requests
library, logistics companies can pull GPS data from a shipment tracking API and process it accordingly.
import requests # Fetching real-time location data from an API def fetch_tracking_data(tracking_number): url = f'https://api.example.com/track/{tracking_number}' response = requests.get(url) if response.status_code == 200: return response.json() else: print('Error fetching data:', response.status_code) return None # Example usage tracking_info = fetch_tracking_data('123456789') print(tracking_info)
This simple function demonstrates how to access real-time tracking information by querying an external API. The result can include precise location data, estimated arrival times, and more, enabling logistics managers to stay informed about their shipments.
Once the tracking data is obtained, it is vital to monitor and visualize this information effectively. Python’s Matplotlib
and Folium
libraries can be employed to create interactive maps that display the current locations of shipments. Being able to visualize data on a map not only enhances understanding but also aids in communication with stakeholders.
import folium def plot_shipment_location(lat, lon): # Create a map centered at the shipment's location shipment_map = folium.Map(location=[lat, lon], zoom_start=12) # Add a marker for the shipment folium.Marker([lat, lon], popup='Shipment Location').add_to(shipment_map) # Save the map to an HTML file shipment_map.save('shipment_location.html') # Example usage plot_shipment_location(tracking_info['location']['latitude'], tracking_info['location']['longitude'])
The plot_shipment_location
function creates a map with a marker indicating the current location of a shipment. This dynamic visual representation can be invaluable during meetings or reports, giving everyone a clear picture of the logistical landscape.
Moreover, real-time monitoring extends beyond just visualizing locations. It encompasses alerting systems that notify logistics managers about potential delays or anomalies. Python’s ability to handle data streams and implement logic for conditions allows for such automation. For instance, if a shipment is delayed beyond a certain threshold, the system can trigger an alert.
def check_delivery_status(shipment): if shipment['status'] == 'in transit' and shipment['estimated_arrival'] < current_time: send_alert(shipment['tracking_number']) def send_alert(tracking_number): print(f'Alert: Shipment {tracking_number} is delayed!') # Example usage current_time = '2023-10-15T12:00:00' check_delivery_status(tracking_info)
In this example, the check_delivery_status
function checks the status of a shipment and sends an alert if the estimated arrival time has passed. This proactive approach enables logistics teams to address issues before they escalate, thereby enhancing customer satisfaction and operational efficiency.
As the logistics landscape continues to evolve, the need for real-time tracking and monitoring solutions powered by Python is more critical than ever. By employing these tools, organizations can not only improve their operational responsiveness but also build a more resilient and transparent supply chain. The ability to react swiftly to real-time data transforms logistics from a reactive process into a proactive strategy, enabling companies to reduce costs, enhance service levels, and maintain a competitive edge.
Predictive Analytics for Demand Forecasting
Predictive analytics has emerged as a transformative element in logistics, allowing organizations to forecast demand, optimize inventory, and enhance overall efficiency. Python, with its powerful data manipulation libraries and machine learning capabilities, plays a pivotal role in implementing predictive analytics for demand forecasting. By using historical data, businesses can create models that predict future demand patterns, thereby enabling them to make informed decisions.
At the heart of predictive analytics is the process of data preparation. This involves gathering historical sales data, external factors such as seasonality, promotions, and market trends. Python’s Pandas library is invaluable here, allowing for seamless data extraction, cleaning, and transformation. Once the data is prepared, the next step involves choosing the right predictive model.
One of the simplest yet effective models for demand forecasting is the linear regression model. This model can capture the relationship between the independent variables (like time, marketing spend, etc.) and the dependent variable (demand). Using Scikit-learn, a popular machine learning library in Python, we can implement this model with ease.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn.metrics import mean_squared_error # Load historical data data = pd.read_csv('historical_sales_data.csv') # Prepare features and target variable X = data[['Marketing_Spend', 'Seasonality_Index']] y = data['Demand'] # Split the data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the model model = LinearRegression() model.fit(X_train, y_train) # Predict on the test set y_pred = model.predict(X_test) # Evaluate the model mse = mean_squared_error(y_test, y_pred) print(f'Mean Squared Error: {mse}')
In this code snippet, we begin by importing the required libraries and loading historical sales data. We prepare the features (such as marketing spend and seasonality index) alongside the target variable (demand). After splitting the data into training and testing sets, we initialize a linear regression model and fit it to our training data. Finally, we evaluate the model’s performance using the mean squared error metric, which helps us understand how well our model is predicting demand.
Once a predictive model is established, it’s critical to fine-tune it through a process known as hyperparameter optimization. This involves adjusting model parameters to enhance accuracy. Python’s Scikit-learn library offers tools like GridSearchCV, which can automate the search for optimal parameters, enhancing model performance.
from sklearn.model_selection import GridSearchCV # Define the model and parameters for grid search model = LinearRegression() params = {'fit_intercept': [True, False], 'normalize': [True, False]} grid_search = GridSearchCV(model, params, cv=5) # Fit the model to the training data grid_search.fit(X_train, y_train) # Print the best parameters found print(f'Best Parameters: {grid_search.best_params_}')
After identifying the optimal parameters, the model can be retrained to ensure that it’s in its best form for making predictions. With a well-tuned model, logistics companies can forecast demand with greater certainty, allowing them to align their inventory levels more closely with expected sales.
In addition to linear regression, Python offers a plethora of advanced algorithms for demand forecasting, including decision trees, random forests, and neural networks. Each of these methods can capture different patterns in the data, and Python’s libraries such as TensorFlow and Keras empower organizations to implement these complex models effectively.
from tensorflow import keras from tensorflow.keras import layers # Build a simple neural network model model = keras.Sequential([ layers.Dense(64, activation='relu', input_shape=(X_train.shape[1],)), layers.Dense(64, activation='relu'), layers.Dense(1) # Output layer for regression ]) # Compile the model model.compile(optimizer='adam', loss='mean_squared_error') # Train the model model.fit(X_train, y_train, epochs=50, validation_split=0.2)
This code illustrates how to construct a basic neural network for demand forecasting. The model consists of two hidden layers with 64 neurons each, followed by an output layer tailored for regression tasks. By training this model on the prepared dataset, logistics companies can leverage deep learning to improve their demand forecasts significantly.
Ultimately, the implementation of predictive analytics for demand forecasting through Python not only enhances operational efficiencies but also empowers logistics firms to make proactive decisions. By anticipating customer demand, businesses can optimize inventory levels, minimize waste, and ensure that they are prepared for fluctuations in the market, thus remaining competitive in a rapidly changing landscape.
Integrating Python with Logistics Management Systems
Integrating Python with logistics management systems can significantly enhance operational efficiency and responsiveness. The flexibility of Python allows it to seamlessly communicate with various logistics platforms, whether they are cloud-based solutions or on-premise systems. By using Python, organizations can develop applications that automate processes, analyze data, and integrate disparate systems, ultimately creating a cohesive logistics management environment.
A foundational aspect of this integration is the ability to interact with APIs provided by logistics management systems. Many modern logistics solutions expose RESTful APIs that allow external applications to interact with their data and functionalities. Python’s requests library makes it simpler to query these APIs and retrieve or send data as needed. This capability is pivotal for maintaining up-to-date information across systems.
import requests # Function to fetch order details from a logistics management system API def fetch_order_details(order_id): url = f'https://api.logisticsystem.com/orders/{order_id}' headers = {'Authorization': 'Bearer YOUR_API_TOKEN'} response = requests.get(url, headers=headers) if response.status_code == 200: return response.json() else: print('Error fetching order details:', response.status_code) return None # Example usage order_details = fetch_order_details('ORD123456') print(order_details)
This example illustrates how to fetch order details from a logistics management system’s API. By automating such data retrieval processes, logistics managers can access real-time information without manual intervention, allowing for quicker decision-making.
Another critical aspect of integrating Python into logistics management systems is data synchronization. Python’s ability to handle data manipulation and transformation with libraries like Pandas can facilitate the synchronization of data between different systems. For instance, after fetching data from one system, it may need to be transformed and pushed to another system to ensure consistency.
import pandas as pd # Example function to synchronize data between two systems def synchronize_data(source_data): # Transform data as required transformed_data = pd.DataFrame(source_data['items']) # Push transformed data to another system url = 'https://api.anotherlogisticsystem.com/update_inventory' response = requests.post(url, json=transformed_data.to_dict(orient='records'), headers={'Authorization': 'Bearer YOUR_API_TOKEN'}) if response.status_code == 200: print('Data synchronized successfully!') else: print('Error during synchronization:', response.status_code) # Example source data source_data = {'items': [{'item_id': 101, 'quantity': 50}, {'item_id': 102, 'quantity': 30}]} synchronize_data(source_data)
In this case, the synchronize_data function takes JSON data from a source system, transforms it into a suitable format using Pandas, and then posts it to another logistics system. This type of integration ensures that all systems are aligned, which is essential for maintaining visibility and control over logistics operations.
Furthermore, Python’s capabilities extend to the development of custom dashboards and reporting tools that pull data from various logistics management systems. By using libraries like Dash or Flask, developers can create interactive web applications that visualize key performance indicators (KPIs) and operational metrics. This real-time reporting capability enhances decision-making by providing stakeholders with the insights they need to monitor performance and identify areas for improvement.
from flask import Flask, render_template import pandas as pd app = Flask(__name__) @app.route('/dashboard') def dashboard(): # Fetch data from logistics system API orders_data = fetch_order_details('ORD123456') return render_template('dashboard.html', orders=orders_data['items']) if __name__ == '__main__': app.run(debug=True)
The above Flask application demonstrates how to create a simple web dashboard that displays order data fetched from a logistics management system. By integrating data retrieval and web frameworks, logistics organizations can provide stakeholders with easy access to essential information, enhancing transparency and accountability.
Ultimately, the integration of Python with logistics management systems is about creating an ecosystem that promotes efficiency, data-driven decision-making, and improved service delivery. By using Python’s extensive libraries for data manipulation, automation, and visualization, organizations can build a logistics infrastructure that not only meets current demands but also adapts to future challenges.