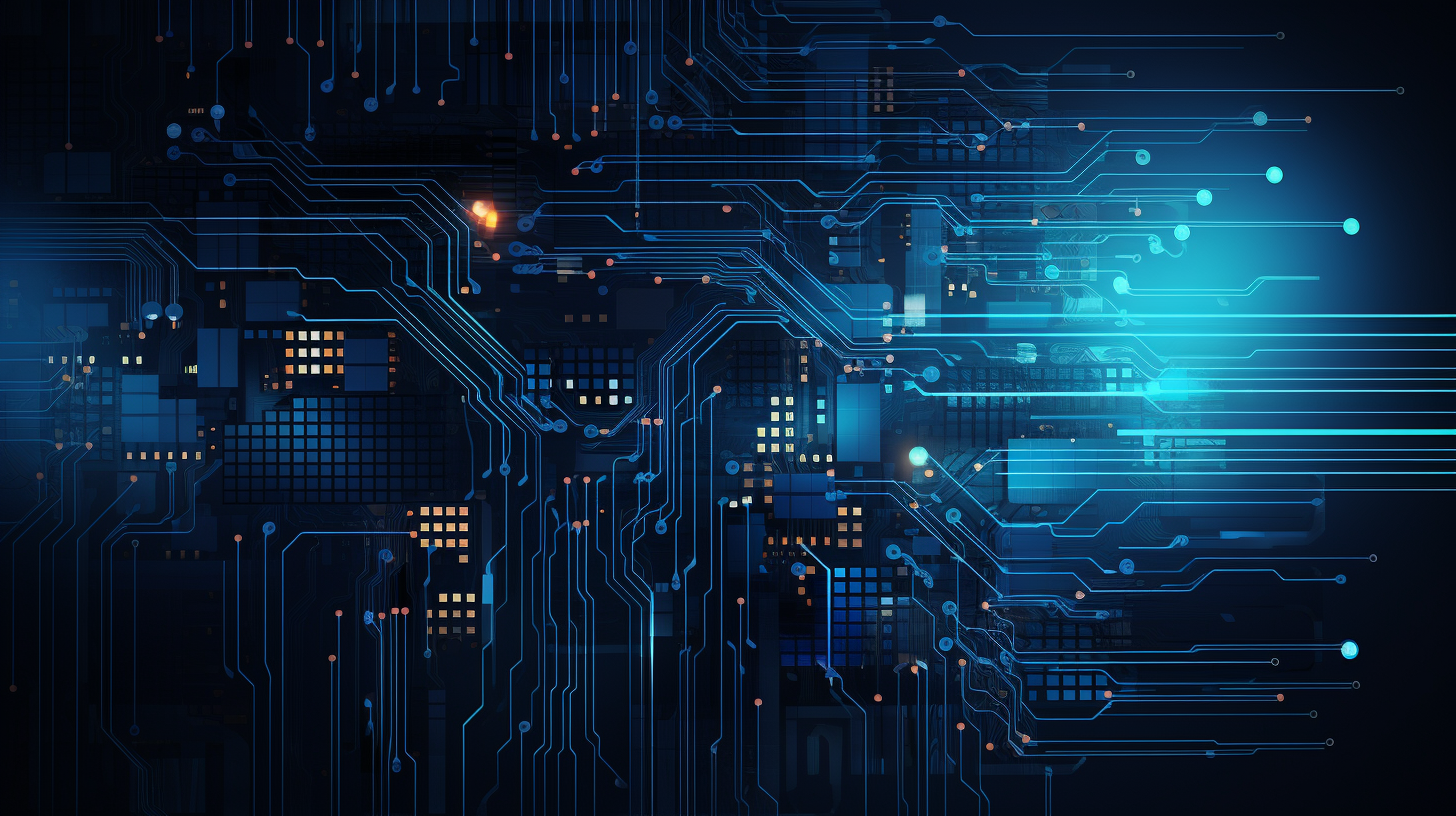
Python and Multithreading: Concurrent Execution
Multithreading in Python allows developers to run multiple threads (smaller units of a process) concurrently. This is particularly useful when dealing with I/O-bound tasks, where the program spends significant time waiting for external resources. By using threads, Python can continue executing other tasks while one thread is blocked waiting for I/O operations to complete, thereby improving performance and responsiveness.
In contrast to processes, threads within the same process share the same memory space, which means they can communicate with each other more easily. However, this shared environment also introduces potential issues regarding data consistency and thread safety.
Threads can be created using the threading module, which provides a higher-level interface for managing threads compared to the lower-level thread module. Each thread can run a specific function or method, allowing for a more modular approach to concurrent execution.
To illustrate the power of multithreading, think the following simple example where we create a couple of threads that print numbers with a delay:
import threading import time def print_numbers(): for i in range(5): time.sleep(1) print(i) thread1 = threading.Thread(target=print_numbers) thread2 = threading.Thread(target=print_numbers) thread1.start() thread2.start() thread1.join() thread2.join()
In this example, two threads are spawned to execute the print_numbers
function at once. Each thread will print numbers from 0 to 4, but due to the time.sleep(1)
call, they’ll print their numbers with a one-second delay. The output of this program will interleave the numbers from both threads, showcasing the concurrent execution.
It’s critical to understand the nature of your problem when deciding to use multithreading. While it can significantly improve performance for I/O-bound tasks, it may not yield the same benefits for CPU-bound tasks, particularly due to the Global Interpreter Lock (GIL), which limits the execution of multiple threads in CPU-intensive code.
Overall, multithreading is a powerful tool in Python’s arsenal, enabling developers to create responsive applications that efficiently manage multiple tasks at the same time. However, it requires careful consideration of thread management and synchronization to avoid common pitfalls associated with concurrent programming.
The Global Interpreter Lock (GIL)
The Global Interpreter Lock (GIL) is a mechanism that prevents multiple native threads from executing Python bytecodes at once. This lock is necessary because Python’s memory management is not thread-safe; it manages memory using reference counting and, in some cases, requires the GIL to ensure that only one thread modifies the memory at a time. The presence of the GIL means that even in a multithreaded application, only one thread can execute Python code at any given moment, thus limiting parallel execution of CPU-bound threads.
For I/O-bound tasks, the impact of the GIL is typically negligible as threads spend much of their time waiting for I/O operations to complete. However, when it comes to CPU-bound tasks—those that require heavy computation—the GIL can become a significant bottleneck. In such scenarios, even if multiple threads are available, only one can be executed at a time, which can result in the performance of the application suffering compared to a multiprocessing approach.
To demonstrate the effects of the GIL, ponder the following example that attempts to run two CPU-bound tasks in separate threads:
import threading import time def cpu_bound_task(): total = 0 for i in range(10**7): total += i return total # Creating threads thread1 = threading.Thread(target=cpu_bound_task) thread2 = threading.Thread(target=cpu_bound_task) start_time = time.time() thread1.start() thread2.start() thread1.join() thread2.join() end_time = time.time() print(f"Execution Time: {end_time - start_time} seconds")
In this example, both threads execute a CPU-bound task that sums a large range of numbers. However, due to the GIL, you may find that the total execution time does not significantly decrease when adding threads. In fact, when running this code, you might notice that the runtime is close to double that of a single thread executing the same task, as the threads are effectively competing for the GIL rather than executing in parallel.
For tasks that demand high computational power, Python provides alternatives like the multiprocessing module, which spawns separate processes instead of threads. Each process in Python has its own GIL and memory space, enabling true parallelism across CPU cores. This approach can lead to substantial performance gains in CPU-bound applications, as demonstrated in the following example:
from multiprocessing import Process import time def cpu_bound_task(): total = 0 for i in range(10**7): total += i return total # Creating processes process1 = Process(target=cpu_bound_task) process2 = Process(target=cpu_bound_task) start_time = time.time() process1.start() process2.start() process1.join() process2.join() end_time = time.time() print(f"Execution Time: {end_time - start_time} seconds")
This example uses the multiprocessing module, allowing both processes to run in parallel on separate cores, thus bypassing the GIL limitation. The result is that the total execution time is considerably reduced, showcasing how using multiple processes can effectively utilize the available CPU resources for demanding calculations.
While the GIL can be a limiting factor in Python’s multithreading capabilities, understanding its implications allows developers to make informed decisions about when to use threading or opt for multiprocessing instead. The key is to analyze the nature of the workload at hand—whether it is I/O-bound or CPU-bound—and choose the appropriate concurrency model accordingly.
Creating and Managing Threads
import threading import time def print_numbers(): for i in range(5): time.sleep(1) print(i) thread1 = threading.Thread(target=print_numbers) thread2 = threading.Thread(target=print_numbers) thread1.start() thread2.start() thread1.join() thread2.join()
Managing threads effectively requires understanding the lifecycle of a thread and the various states it can be in during its execution. In Python, a thread can be in one of several states: new, runnable, blocked, waiting, or terminated. This state management plays an important role in concurrent programming.
When you create a thread using `threading.Thread`, it starts in the ‘new’ state. Once you call the `start()` method, the thread transitions to the ‘runnable’ state and is eligible to be executed by the Python interpreter. However, if it tries to run while another thread holds the GIL or if it needs to wait for resources, it may enter the ‘blocked’ state, effectively pausing its execution until it can proceed.
To properly manage the lifecycle and interactions of threads, Python provides various methods and techniques:
1. **Joining Threads**: The `join()` method is a key aspect of thread management. It blocks the calling thread until the thread whose `join()` method is called is terminated. This is essential for ensuring that resources are properly cleaned up and that the main program waits for all threads to finish before proceeding.
2. **Daemon Threads**: By setting the `daemon` attribute to `True`, you can create threads that run in the background and do not prevent the program from exiting. Daemon threads are useful for tasks that should not block the termination of the program, such as logging or monitoring tasks. However, it’s important to remember that daemon threads are abruptly terminated when the program exits, so any necessary cleanup should be handled in non-daemon threads.
3. **Thread Lifespan**: The lifespan of a thread begins when it’s started and ends when its target function completes or when it’s terminated. Managing resources effectively within this lifespan is critical. Resources such as file handles or network connections should be released appropriately to prevent leaks.
To showcase how to use daemon threads, consider the following example:
import threading import time def background_task(): while True: print("Running in the background...") time.sleep(1) daemon_thread = threading.Thread(target=background_task) daemon_thread.daemon = True # Set the thread as a daemon daemon_thread.start() # Main thread sleeps for 5 seconds and then exits time.sleep(5)
In this code, the `background_task` function runs indefinitely, printing a message every second. However, since the thread is a daemon, it will not prevent the main program from exiting after 5 seconds. This demonstrates how daemon threads can be employed for background tasks that do not need to block the main application flow.
As you delve deeper into thread management, ponder the implications of thread safety. Since threads share the same memory space, accessing shared resources without proper synchronization can lead to race conditions or data corruption. In the next section, we will explore synchronization techniques to ensure that shared resources are accessed safely and consistently across threads.
Thread Synchronization Techniques
In a multithreaded environment, ensuring that threads operate without interfering with each other is critical. That is where thread synchronization techniques come into play. Synchronization allows us to control the access of multiple threads to shared resources, ensuring that data integrity is maintained. Failing to implement proper synchronization can lead to race conditions, deadlocks, and other unpredictable behaviors that can compromise the stability of an application.
Python provides several synchronization primitives in the `threading` module that can be effectively utilized to manage access to shared resources. The most common synchronization techniques include:
1. Locks
Locks are the simplest synchronization primitive. A lock allows only one thread to access a particular piece of code or resource at a time. When a thread acquires a lock, other threads that attempt to acquire the same lock will be blocked until the lock is released.
import threading # Shared resource counter = 0 lock = threading.Lock() def increment(): global counter for _ in range(100000): lock.acquire() # Acquire the lock counter += 1 # Critical section lock.release() # Release the lock threads = [] for _ in range(2): thread = threading.Thread(target=increment) threads.append(thread) thread.start() for thread in threads: thread.join() print(f"Final counter value: {counter}") # Should be 200000
In this example, two threads increment a shared counter. The lock ensures that only one thread can modify the counter at a time, thus preventing race conditions. Without the lock, the final counter value could be incorrect due to simultaneous modifications by both threads.
2. RLocks (Reentrant Locks)
An RLock (reentrant lock) allows a thread to acquire the lock multiple times without causing a deadlock. That is useful in scenarios where a thread might need to call a function that requires the same lock it already holds.
import threading # Using RLock rlock = threading.RLock() def recursive_increment(n): global counter if n > 0: rlock.acquire() counter += 1 recursive_increment(n - 1) rlock.release() # Reset counter counter = 0 thread = threading.Thread(target=recursive_increment, args=(5,)) thread.start() thread.join() print(f"Final counter value: {counter}") # Should be 5
Here, the `recursive_increment` function uses an RLock to safely increment the counter through recursive calls. The RLock allows the thread to re-enter the lock without deadlocking itself.
3. Condition Variables
Condition variables enable threads to wait for certain conditions to be met before they proceed. This is particularly useful when one thread needs to signal another that a resource is available.
import threading import time buffer = [] buffer_lock = threading.Lock() condition = threading.Condition(buffer_lock) def producer(): global buffer for i in range(5): time.sleep(1) # Simulate production time with condition: buffer.append(i) print(f"Produced {i}") condition.notify() # Notify a waiting consumer def consumer(): global buffer for _ in range(5): with condition: while not buffer: # Wait for an item to be available condition.wait() # Release lock and wait item = buffer.pop(0) print(f"Consumed {item}") producer_thread = threading.Thread(target=producer) consumer_thread = threading.Thread(target=consumer) producer_thread.start() consumer_thread.start() producer_thread.join() consumer_thread.join()
In this example, the producer generates items and notifies the consumer whenever an item is available. The consumer waits until there is something to consume before proceeding. This pattern effectively synchronizes the production and consumption of resources.
4. Semaphores
Semaphores are used to control access to a shared resource through a counter. They allow a specified number of threads to access a particular resource concurrently. That is useful in scenarios where you want to limit the number of concurrent accesses to a resource.
import threading import time semaphore = threading.Semaphore(2) # Allow up to 2 threads def access_resource(thread_num): with semaphore: print(f"Thread {thread_num} is accessing the resource.") time.sleep(2) # Simulating resource access print(f"Thread {thread_num} is done.") threads = [] for i in range(5): thread = threading.Thread(target=access_resource, args=(i,)) threads.append(thread) thread.start() for thread in threads: thread.join()
In this case, the semaphore allows only two threads to access the resource concurrently, demonstrating how to control access while permitting some concurrency.
Each of these synchronization techniques has its specific use cases and implications. Choosing the right one depends on the nature of the tasks being performed and the shared resources accessed by your threads. Mastering these synchronization strategies can help you avoid the pitfalls of concurrent programming and write robust multithreaded applications.
Common Pitfalls and Best Practices
When engaging in multithreading, it’s essential to recognize that while threading can enhance responsiveness and performance, it also opens the door to various pitfalls that can undermine the benefits of concurrent execution. Below are some common pitfalls, along with best practices to mitigate them and ensure your multithreaded applications run smoothly.
1. Race Conditions
Race conditions occur when two or more threads attempt to modify shared data at the same time, leading to inconsistent or unexpected results. To prevent race conditions, it is crucial to use synchronization mechanisms such as locks or semaphores whenever threads are accessing shared resources. For example:
import threading # Shared data shared_counter = 0 lock = threading.Lock() def increment(): global shared_counter for _ in range(100000): with lock: shared_counter += 1 # Safe increment threads = [threading.Thread(target=increment) for _ in range(2)] for thread in threads: thread.start() for thread in threads: thread.join() print(f"Final counter value: {shared_counter}") # Should be 200000
Using the lock in the `increment` function ensures that only one thread modifies `shared_counter` at a time, thus safeguarding against race conditions.
2. Deadlocks
A deadlock occurs when two or more threads are waiting for each other to release resources, resulting in them all being blocked indefinitely. To avoid deadlocks, follow these best practices:
- Acquire locks in a consistent order across all threads.
- Implement timeouts when acquiring locks to break potential deadlocks.
Here’s an example demonstrating potential deadlock avoidance:
import threading lock1 = threading.Lock() lock2 = threading.Lock() def thread1_function(): with lock1: print("Thread 1 acquired lock 1") with lock2: print("Thread 1 acquired lock 2") def thread2_function(): with lock1: print("Thread 2 acquired lock 1") with lock2: print("Thread 2 acquired lock 2") t1 = threading.Thread(target=thread1_function) t2 = threading.Thread(target=thread2_function) t1.start() t2.start() t1.join() t2.join()
This code risks deadlock because each thread holds one lock and tries to acquire the other. To mitigate this, we could enforce a consistent lock acquisition order or use a timeout.
3. Starvation
Starvation happens when a thread is perpetually denied the resources it needs to execute. This can occur if higher-priority threads continuously consume available resources. To prevent starvation, ensure that your thread management strategy is fair. You could implement a scheduling mechanism that gives each thread a chance to execute, or use condition variables judiciously to manage access to resources better.
4. Resource Leakage
Resource leakage can occur when threads do not properly release resources like file handles or locks after use. This may lead to a gradual degradation in performance and eventual exhaustion of system resources. Always ensure that resources are released in a `finally` block or by using context managers (`with` statement) to guarantee that cleanup code runs, even if an exception occurs.
def safe_open_file(file_path): with open(file_path) as file: data = file.read() # Safe access to the file # Example of usage in a thread t = threading.Thread(target=safe_open_file, args=("myfile.txt",)) t.start() t.join()
This approach guarantees that the file is closed properly, avoiding leaks.
5. Overhead and Complexity
While threading can improve performance, it can also introduce complexity and overhead, particularly for small tasks. It is vital to evaluate whether the performance benefits of threading outweigh the additional complexity. For lightweight tasks, think using asynchronous programming with `asyncio` as an alternative.
6. Testing and Debugging
Testing multithreaded applications can be significantly more challenging than single-threaded ones due to their non-deterministic behavior. Incorporate extensive logging and consider using thread-safe data structures when collecting metrics or logs. Additionally, employing tools such as thread sanitizers can help identify concurrency-related issues during development.
By being mindful of these common pitfalls and implementing best practices, developers can harness the power of multithreading in Python while minimizing the risks associated with concurrent programming. As with any advanced feature, a cautious approach, combined with thorough testing, will pave the way for robust and efficient applications.
Use Cases for Multithreading in Python
Use cases for multithreading in Python are abundant and varied, reflecting the diverse nature of tasks that developers encounter in real-world applications. While multithreading excels in scenarios that involve I/O-bound tasks, it also finds its place in other areas where responsiveness and concurrent execution are paramount. Below are several compelling use cases for multithreading in Python:
1. Web Scraping
Web scraping is a classic example of an I/O-bound task, where the application spends a significant amount of time waiting for responses from web servers. By employing multithreading, developers can make multiple requests at once, drastically reducing the overall time required to gather data from various web pages.
import requests import threading urls = [ 'https://example.com/page1', 'https://example.com/page2', 'https://example.com/page3' ] def fetch_url(url): response = requests.get(url) print(f"Fetched {url} with status code {response.status_code}") threads = [] for url in urls: thread = threading.Thread(target=fetch_url, args=(url,)) threads.append(thread) thread.start() for thread in threads: thread.join()
In this example, multiple threads are spawned to fetch web pages, allowing the application to process multiple responses simultaneously while waiting for network calls to complete.
2. GUI Applications
Graphical User Interface (GUI) applications benefit from multithreading as they require a responsive interface while performing background tasks. Long-running operations, such as file uploads or data processing, can be executed in separate threads to prevent the GUI from freezing, thus providing a smoother user experience.
import tkinter as tk import threading import time def long_running_task(): time.sleep(5) # Simulate a long task print("Task completed!") def start_task(): thread = threading.Thread(target=long_running_task) thread.start() root = tk.Tk() button = tk.Button(root, text="Start Task", command=start_task) button.pack() root.mainloop()
In this GUI example, clicking the button starts a long-running task in a separate thread, allowing the user to interact with the GUI without interruption.
3. Network Services
In server applications, such as web servers or chat applications, multithreading is vital for handling multiple client connections simultaneously. Each client connection can be managed in a separate thread, enabling the server to serve multiple clients without blocking.
import socket import threading def handle_client(client_socket): request = client_socket.recv(1024) print(f"Received: {request.decode()}") client_socket.send(b"ACK") client_socket.close() server = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server.bind(('0.0.0.0', 9999)) server.listen(5) print("Listening on port 9999") while True: client_sock, addr = server.accept() print(f"Accepted connection from {addr}") client_handler = threading.Thread(target=handle_client, args=(client_sock,)) client_handler.start()
This example demonstrates a simple TCP server that uses threads to handle each client connection, allowing the server to accept new connections while processing existing ones.
4. Data Processing
In scenarios involving data processing, such as parsing large files or performing bulk operations on databases, multithreading can help improve performance by processing chunks of data simultaneously. This aspect is especially advantageous when the processing involves I/O operations like database queries.
import threading data_chunks = [range(10000), range(10000, 20000), range(20000, 30000)] def process_chunk(chunk): result = sum(chunk) # Simulate data processing print(f"Processed chunk with result: {result}") threads = [] for chunk in data_chunks: thread = threading.Thread(target=process_chunk, args=(chunk,)) threads.append(thread) thread.start() for thread in threads: thread.join()
In this example, each chunk of data is processed in its own thread, allowing for concurrent execution, which can significantly speed up processing time compared to a single-threaded approach.
5. Real-Time Data Monitoring
Applications that require real-time monitoring of data streams, such as stock price tickers or sensor data, can greatly benefit from multithreading. By employing threads to poll for updates while at the same time updating the display, developers can ensure that the interface remains responsive and up-to-date.
import time import random import threading def monitor_data(): while True: data = random.random() # Simulate data fetching print(f"New data: {data}") time.sleep(1) monitor_thread = threading.Thread(target=monitor_data) monitor_thread.start() # Main thread can perform other tasks while True: pass # Simulate other tasks
In this real-time monitoring example, a thread continually fetches and prints new data, showcasing how multithreading can enhance the performance of applications that require constant updates.
These examples illustrate the versatility and effectiveness of multithreading in Python for various use cases. Whether the task is I/O-bound, requires responsiveness, or involves concurrent processing, using threads can lead to more efficient and responsive applications. Understanding the context and nature of your workload, however, remains crucial in determining the appropriate use of multithreading in your Python projects.