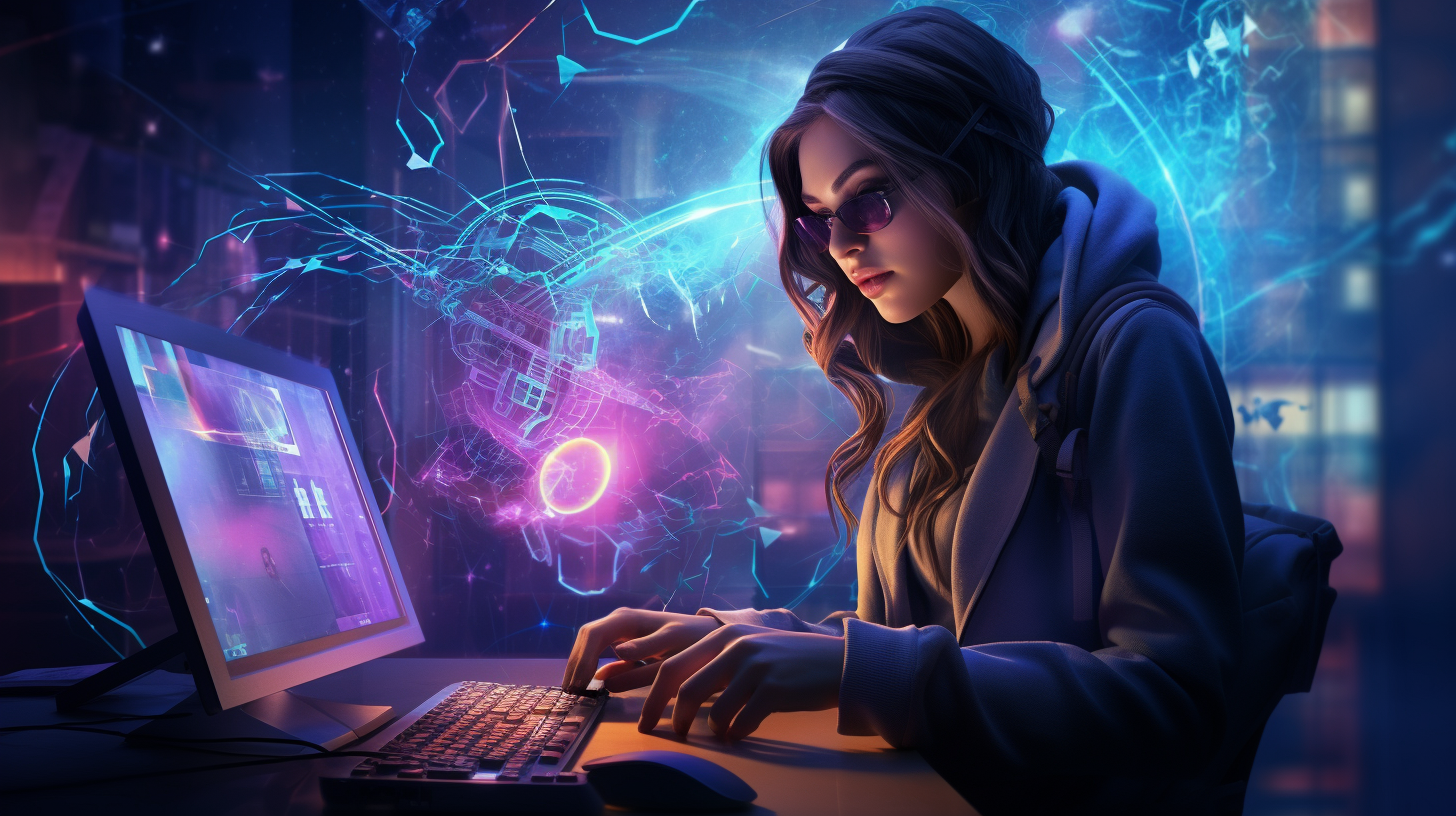
Python and Quantum Computing: An Introduction
Quantum computing represents a paradigm shift in the way we understand computation. At its core, it leverages the principles of quantum mechanics to process information in ways that classical computers cannot. To truly grasp the essence of quantum computing, one must first understand a few fundamental concepts: qubits, superposition, entanglement, and quantum gates.
Qubits are the basic units of quantum information, analogous to bits in classical computing. However, while a classical bit can exist in one of two states (0 or 1), a qubit can exist simultaneously in a combination of both states due to the principle of superposition. This essentially allows quantum computers to process a vast amount of possibilities at the same time.
Consider a single qubit, which can be represented mathematically as:
|ψ⟩ = α|0⟩ + β|1⟩
Here, |0⟩ and |1⟩ are the basis states, and α and β are complex numbers that define the probability amplitudes of those states. The probabilities of measuring the qubit in the |0⟩ or |1⟩ state are given by |α|² and |β|², respectively, with the normalization condition |α|² + |β|² = 1 ensuring that the total probability sums to 1.
Entanglement is another cornerstone of quantum mechanics that allows qubits to be correlated with one another, regardless of the distance separating them. When qubits become entangled, the state of one qubit instantly influences the state of another. This phenomenon is famously illustrated by the Einstein-Podolsky-Rosen (EPR) paradox.
Mathematically, if we have two qubits in an entangled state, their combined state can be expressed as:
|Φ⟩ = (|00⟩ + |11⟩) / √2
In this case, measuring one qubit will instantly determine the state of the other qubit, a property that classical physics cannot replicate.
Next, we have quantum gates, which are the building blocks of quantum circuits. Just as classical logic gates manipulate bits, quantum gates manipulate qubits through unitary transformations. Common quantum gates include:
- Creates superposition.
- Flips the state of a qubit.
- Flips the state of one qubit based on the state of another.
For instance, applying a Hadamard gate to a qubit initialized in the |0⟩ state would yield:
H|0⟩ = (|0⟩ + |1⟩) / √2
This transformation is critical for generating superposition, thus enabling quantum parallelism.
As we delve deeper into quantum computing, it is essential to appreciate these foundational principles. They’re not just theoretical musings; they are the bedrock upon which the towering capabilities of quantum computation are built. The ability to harness qubits, leverage superposition and entanglement, and manipulate states with quantum gates positions quantum computing as a powerful tool for solving problems that are currently intractable for classical systems.
Python Libraries for Quantum Computing
Among the most compelling aspects of quantum computing is the growing ecosystem of Python libraries designed to facilitate both experimentation and development in this emerging field. Python, with its simplicity and readability, has become the de facto language for quantum computing enthusiasts and professionals alike. Several libraries have emerged, each offering unique functionalities and catering to different needs within the quantum computational landscape.
Qiskit is one of the most prominent libraries, developed by IBM. It provides a comprehensive framework for writing quantum algorithms and running them on real quantum systems. With Qiskit, users can construct quantum circuits, simulate them, and execute them on actual IBM Quantum devices. The library is modular, consisting of several components, including:
- The foundation for creating and optimizing quantum circuits.
- A high-performance simulator for quantum circuits.
- Tools for quantum error correction and mitigation.
- A library for quantum algorithms, focused on applications in chemistry, optimization, and machine learning.
To illustrate Qiskit in action, think the following example that creates a simple quantum circuit with a Hadamard gate and a measurement:
from qiskit import QuantumCircuit, Aer, transpile, assemble, execute # Create a Quantum Circuit with 1 qubit qc = QuantumCircuit(1, 1) # Apply Hadamard gate qc.h(0) # Measurement qc.measure(0, 0) # Visualize the circuit print(qc.draw())
This code snippet initializes a quantum circuit with a single qubit, applies a Hadamard gate, and measures the qubit’s state. The resulting circuit diagram is an essential tool for visualizing the flow of quantum operations.
PyQuil, developed by Rigetti Computing, is another important library focused on quantum programming. It uses the Quantum Instruction Language (QIL) and provides a unique interface for quantum programming. PyQuil excels in creating quantum algorithms that are compatible with Rigetti’s quantum processors. Here’s how you can use PyQuil to set up a simple quantum circuit:
from pyquil import Program from pyquil.gates import H, MEASURE # Create a Quantum Program program = Program() # Apply Hadamard gate program += H(0) # Measurement ro = program.declare("ro", "BIT", 1) program += MEASURE(0, ro[0]) # Display the program print(program)
This snippet creates a quantum program similar to the Qiskit example, showcasing how to apply quantum gates and perform measurements using PyQuil.
Cirq, developed by Google, is another library worth noting. It’s designed to create, edit, and invoke Noisy Intermediate Scale Quantum (NISQ) circuits. Cirq focuses on the unique challenges posed by current quantum hardware, providing tools that allow developers to optimize their quantum circuits specifically for available quantum processors. Here’s a basic example of creating a circuit in Cirq:
import cirq # Create a qubit qubit = cirq.LineQubit(0) # Create a circuit circuit = cirq.Circuit() # Apply Hadamard gate circuit.append(cirq.H(qubit)) # Measurement circuit.append(cirq.measure(qubit, key='result')) # Print the circuit print(circuit)
In this example, a qubit is created, a Hadamard gate is applied, and the qubit is measured, demonstrating Cirq’s intuitive syntax for quantum circuit creation.
Strawberry Fields is a library specifically designed for quantum machine learning and photonic quantum computing. It allows users to define quantum neural networks and leverage quantum algorithms for machine learning tasks. Here’s a simple example of using Strawberry Fields to create a quantum circuit:
import strawberryfields as sf from strawberryfields.ops import * # Create a quantum program with 2 modes prog = sf.Program(2) with prog.context as q: # Apply a beam splitter BS(0.5) | (q[0], q[1]) # Measure the modes MeasureFock() | q # Print the program print(prog)
This code snippet showcases the application of a beam splitter in a photonic quantum circuit, highlighting Strawberry Fields’ focus on quantum optics.
These libraries reflect the rapid advancement and diversification in the quantum computing landscape. Each one empowers developers to harness the unique properties of quantum mechanics, enabling a new class of applications that were previously unimaginable. As Python continues to be the language of choice for quantum computing, these libraries will be pivotal in shaping the future of how we engage with quantum technologies.
Programming Quantum Algorithms with Python
Programming quantum algorithms with Python involves not only using the libraries available but also understanding how to conceptualize quantum algorithms themselves. Quantum algorithms differ significantly from classical ones, requiring a different mindset to develop and optimize solutions. The following discussion will focus on a few fundamental quantum algorithms and show how to implement them using Python.
One of the most famous quantum algorithms is Deutsch-Josza algorithm. This algorithm efficiently solves the problem of determining whether a given function is constant or balanced, achieving this with just one query to the function, in contrast to the classical approach, which may require up to half the possible inputs. The algorithm operates on a quantum state and uses superposition and interference to derive the result.
Let’s implement the Deutsch-Josza algorithm using Qiskit:
from qiskit import QuantumCircuit, Aer, execute def deutsch_jozsa(oracle): # Create a quantum circuit with 2 qubits and 1 classical bit circuit = QuantumCircuit(2, 1) # Initialize the second qubit to |1⟩ state circuit.x(1) # Apply Hadamard gates circuit.h(0) circuit.h(1) # Oracle function circuit += oracle # Apply Hadamard gate to the first qubit circuit.h(0) # Measurement circuit.measure(0, 0) return circuit # Example oracle for balanced function def balanced_oracle(circuit): circuit.cx(0, 1) # Example: flips the second qubit based on first qubit # Create the quantum circuit with the balanced oracle oracle_circuit = QuantumCircuit(2) balanced_oracle(oracle_circuit) # Run the Deutsch-Josza algorithm q_circuit = deutsch_jozsa(oracle_circuit) backend = Aer.get_backend('qasm_simulator') result = execute(q_circuit, backend, shots=1024).result() counts = result.get_counts() print("Result:", counts)
In this example, we define a function to generate the Deutsch-Josza circuit with an oracle. The oracle is composed of controlled operations that depend on the specific function being evaluated. After executing the algorithm, the output will indicate whether the function is constant or balanced based on the measurement of the first qubit.
Next, let’s explore Grover’s Search Algorithm, which is designed for searching unsorted databases with quadratic speedup compared to classical algorithms. The algorithm leverages the principles of superposition and interference to amplify the probability of the correct solution while diminishing the probabilities of incorrect ones.
Here’s how to implement Grover’s algorithm in Qiskit:
from qiskit import QuantumCircuit, Aer, execute def grovers_algorithm(num_qubits, marked_element): # Create a quantum circuit circuit = QuantumCircuit(num_qubits) # Apply Hadamard gates to all qubits circuit.h(range(num_qubits)) # Grover iterations for _ in range(int((3.14/4) * (2**(num_qubits/2))**0.5)): # Oracle: flip the state corresponding to marked element circuit.x(marked_element) circuit.h(marked_element) circuit.cz(0, marked_element) circuit.h(marked_element) circuit.x(marked_element) # Diffusion operator circuit.h(range(num_qubits)) circuit.x(range(num_qubits)) circuit.h(num_qubits - 1) circuit.cx(range(num_qubits - 1), range(num_qubits)) circuit.h(num_qubits - 1) circuit.x(range(num_qubits)) circuit.h(range(num_qubits)) # Measurement circuit.measure_all() return circuit # Example: Search for element |11⟩ in a 2-qubit system grover_circuit = grovers_algorithm(2, 1) backend = Aer.get_backend('qasm_simulator') result = execute(grover_circuit, backend, shots=1024).result() counts = result.get_counts() print("Result:", counts)
In this implementation, we create a quantum circuit for Grover’s algorithm, applying Hadamard gates to initialize the qubits in equal superposition. The algorithm iteratively applies the oracle and the diffusion operator to amplify the probability of the marked state (in this case, |11⟩). Finally, we measure all qubits to determine the output.
These examples illustrate the power of quantum algorithms and their implementation in Python using libraries like Qiskit. As quantum computing continues to evolve, the development of more sophisticated quantum algorithms will enable us to tackle complex problems across various domains, from cryptography to optimization and beyond. Understanding how to program these algorithms is important for using their potential and pushing the boundaries of computational capabilities.
Practical Applications of Quantum Computing in Python
As we continue to explore the practical applications of quantum computing in Python, it becomes evident that the potential for innovation is vast and varied. One of the most compelling areas is optimization, where quantum algorithms can outperform classical counterparts in finding solutions to complex problems.
One application of quantum algorithms lies in the sphere of finance, particularly in portfolio optimization. Classical methods often rely on approximations and heuristics, which can be time-consuming and inefficient when dealing with large datasets. In contrast, quantum annealing—a technique that utilizes quantum mechanics to find the global minimum of a cost function—can potentially sidestep these limitations.
For instance, using D-Wave’s Ocean SDK, a Python library tailored for quantum annealing, we can define a simple portfolio optimization problem. The objective is to maximize returns while minimizing risk, modeled as a quadratic objective function.
from dwave.system import EmbeddingComposite, DWaveSampler import numpy as np # Define the covariance matrix and expected returns covariance_matrix = np.array([[0.1, 0.02], [0.02, 0.1]]) returns = np.array([0.1, 0.05]) # Create a quadratic objective function Q = {('a', 'a'): covariance_matrix[0, 0], ('b', 'b'): covariance_matrix[1, 1], ('a', 'b'): covariance_matrix[0, 1]} # Solve using D-Wave's quantum annealer sampler = EmbeddingComposite(DWaveSampler()) response = sampler.sample_ising(Q, num_reads=100) # Process results best_solution = response.first.sample print("Best solution:", best_solution)
In this example, we’ve defined a covariance matrix and a vector of expected returns, creating a quadratic objective function suited for our optimization problem. By using D-Wave’s quantum annealer, we can efficiently search for an optimal allocation of assets.
Another area where quantum computing is making waves is in drug discovery. Quantum simulations can model molecular interactions at an unprecedented level of detail, potentially revolutionizing how new pharmaceuticals are developed. The Variational Quantum Eigensolver (VQE) algorithm is particularly suited for this task, as it can approximate the ground state energy of a quantum system, offering insights into molecular structures.
Using Qiskit, we can implement a basic version of the VQE algorithm to simulate a simple molecule, such as hydrogen:
from qiskit import Aer from qiskit.circuit.library import EfficientSU2 from qiskit.algorithms import VQE from qiskit.primitives import Sampler from qiskit.quantum_info import Pauli # Define the Hamiltonian for the hydrogen molecule hamiltonian = Pauli.from_list([('ZZ', 0.5)]) # Initialize a quantum circuit ansatz = EfficientSU2(num_qubits=2, entanglement='linear') # Set up the VQE algorithm vqe = VQE(ansatz, optimizer='SLSQP', initial_point=[0.01, 0.01]) # Execute the algorithm on a simulator backend = Aer.get_backend('aer_simulator') sampler = Sampler(backend) result = vqe.compute_minimum_eigenvalue(hamiltonian, sampler) print("Computed ground state energy:", result.eigenvalue.real)
This code snippet outlines how to set up a VQE for the hydrogen molecule. By defining the Hamiltonian, selecting an ansatz, and then executing the VQE on a quantum simulator, we can derive valuable insights into molecular properties that are crucial for drug discovery.
Ultimately, the applications of quantum computing in Python are not limited to finance or drug discovery. Industries such as logistics, artificial intelligence, and cybersecurity are beginning to harness quantum algorithms to tackle their own sets of challenges. As the technology matures and the Python libraries continue to evolve, we can expect a surge in innovative solutions that leverage the unique capabilities of quantum mechanics.
Future Trends in Quantum Computing and Python Development
As we look towards the future of quantum computing and its integration with Python development, several trends are emerging that promise to reshape the landscape of computing as we know it. The intersection between quantum technology and Python programming is becoming increasingly significant, driven by the rapid advancements in quantum hardware and the growing accessibility of quantum programming frameworks.
One noticeable trend is the shift towards hybrid quantum-classical algorithms. These algorithms leverage the strengths of both quantum and classical computing to deliver superior performance on specific tasks. For instance, in optimization problems, classical algorithms can handle certain components more efficiently, while quantum systems can explore the solution space more effectively. The Variational Quantum Eigensolver (VQE) is a prime example, using classical optimization to adjust parameters in a quantum circuit. This blending of paradigms allows developers to maximize computational efficiency and tackle complex problems that were previously intractable.
from qiskit import Aer from qiskit.circuit.library import EfficientSU2 from qiskit.algorithms import VQE from qiskit.primitives import Sampler from qiskit.quantum_info import Pauli # Set up a Hamiltonian for the optimization problem hamiltonian = Pauli.from_list([('ZZ', 0.5)]) # Initialize the ansatz circuit ansatz = EfficientSU2(num_qubits=2, entanglement='linear') # Configure VQE with a chosen optimizer vqe = VQE(ansatz, optimizer='SLSQP', initial_point=[0.01, 0.01]) # Execute on a quantum simulator backend = Aer.get_backend('aer_simulator') sampler = Sampler(backend) result = vqe.compute_minimum_eigenvalue(hamiltonian, sampler) print("Computed ground state energy:", result.eigenvalue.real)
Another trend gaining traction is the expansion of quantum cloud computing. As quantum processors become available through the cloud, developers are provided unprecedented access to advanced quantum resources without needing to invest in expensive hardware. Companies like IBM and D-Wave are already offering cloud-based quantum computing services, enabling developers to run quantum algorithms on real quantum hardware right from their Python environments. This democratization of quantum computing resources is important for fostering innovation and accelerating research in the field.
Moreover, the community around quantum computing is rapidly growing. Open-source initiatives and collaborative platforms are emerging, allowing developers to contribute to and benefit from collective advancements. The development of libraries such as Qiskit, Cirq, and PyQuil illustrates this trend, as they pool resources from academia and industry to create powerful tools that simplify the complexities of quantum programming. As more professionals enter the field, the depth and breadth of knowledge will expand, leading to new techniques and applications that push the boundaries of what quantum computing can achieve.
Furthermore, as the demand for quantum-native applications increases, there is a parallel rise in the need for educational resources and training programs. Initiatives aimed at upskilling the workforce in quantum programming are becoming common, with universities and online platforms offering courses in quantum algorithms, quantum mechanics fundamentals, and practical programming with Python. This focus on education is essential for preparing the next generation of developers who will drive innovation in quantum computing.
Lastly, the integration of machine learning techniques with quantum computing is poised to revolutionize sectors like artificial intelligence. Quantum machine learning algorithms are expected to outperform their classical counterparts in processing and analyzing large datasets. As researchers dive deeper into this hybrid field, we can anticipate the emergence of more sophisticated models that take advantage of quantum speedups. For example, using quantum systems to enhance neural network training will likely lead to breakthroughs in fields ranging from personalized medicine to autonomous systems.
import numpy as np from qiskit import QuantumCircuit, Aer, transpile, assemble, execute # Example quantum feature map for machine learning def create_feature_map(data): circuit = QuantumCircuit(len(data)) for i, x in enumerate(data): if x == 1: circuit.x(i) return circuit # Sample data data = np.array([1, 0, 1]) feature_map = create_feature_map(data) # Visualization of the feature map circuit print(feature_map.draw())
The future of quantum computing and Python development is bright and full of potential. The trends we are witnessing today indicate a dynamic interplay between quantum technology and software development that will lead to groundbreaking innovations across various domains. As we continue to explore these frontiers, Python stands as a critical bridge, connecting developers with the powerful capabilities of quantum computing and enabling a new era of discovery and advancement.