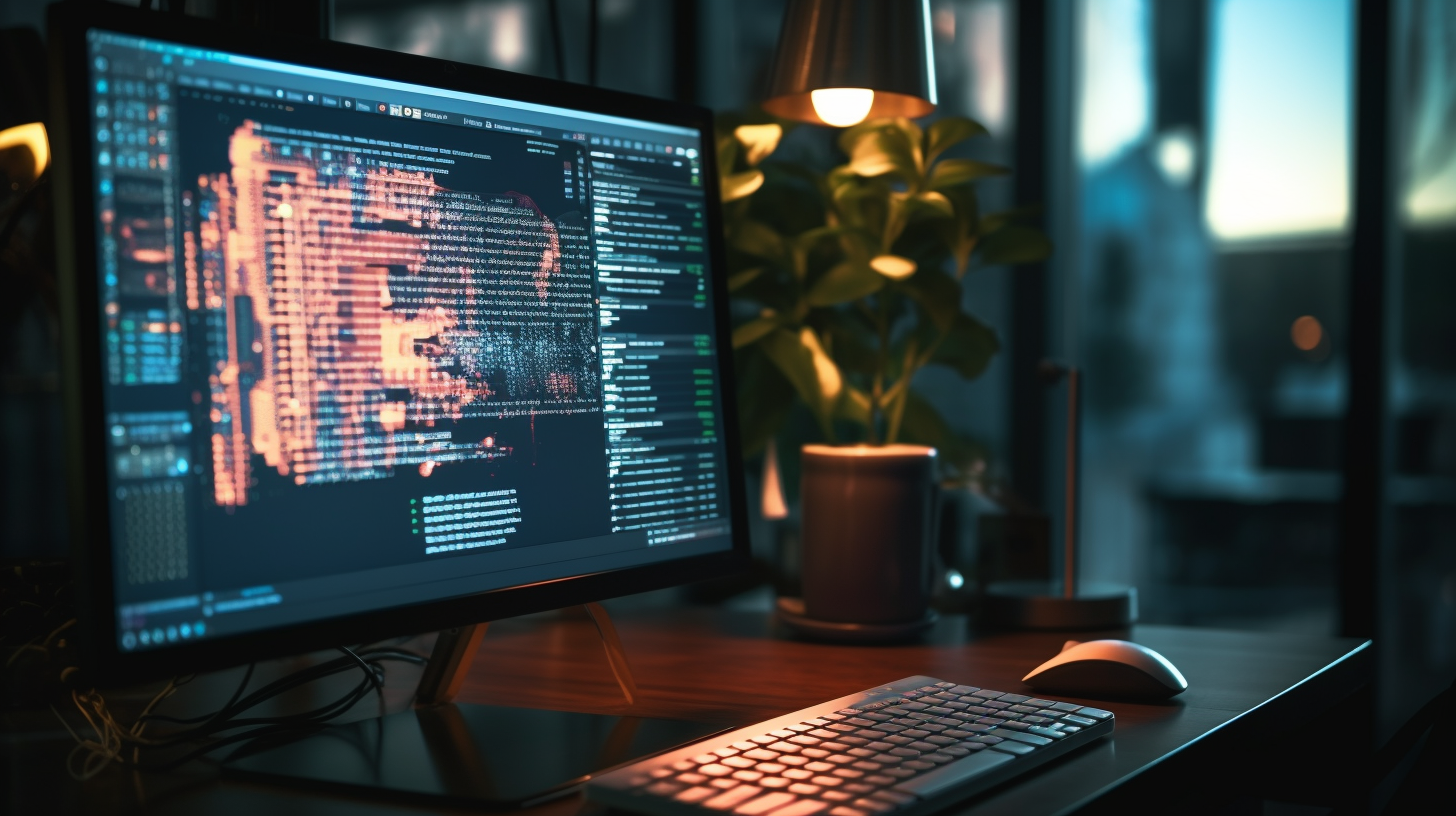
Python and Weather Forecasting: Data Analysis
Weather forecasting relies on a diverse array of data sources, each contributing vital information necessary for accurate predictions. Understanding these sources is critical, as they determine the quality and reliability of the forecasts generated. The primary types of data sources include satellite imagery, weather stations, radar systems, and global weather models.
Satellite Imagery plays an important role in monitoring weather patterns over vast areas. Satellites equipped with advanced sensors capture images of cloud formations, temperature variations, and atmospheric moisture content. These images are analyzed to track storm systems and predict severe weather events.
In contrast, data from Weather Stations provides localized readings. These stations, often operated by governmental and private organizations, measure temperature, humidity, wind speed, and precipitation levels. Aggregating this data allows meteorologists to understand current conditions and make short-term forecasts.
Radar Systems enhance weather forecasting by detecting precipitation and its intensity in real-time. Doppler radar, in particular, is vital for tracking thunderstorms and tornadoes. By measuring the change in frequency of reflected waves, radar can provide insights into storm movement and strength.
Finally, Global Weather Models utilize numerical simulations to forecast future weather patterns based on current conditions. These models assimilate vast amounts of data from various sources, including satellites and weather stations, to generate forecasts that can predict weather over days or even weeks. The output of these models is essential for long-range forecasting.
These various data sources are often integrated into a single framework for analysis. Using Python’s powerful libraries, we can pull in data from these different channels and prepare it for further analysis. Below is a simple example of how to retrieve and visualize weather data from an API:
import requests import matplotlib.pyplot as plt # Example: Fetching weather data from a public API API_URL = "http://api.openweathermap.org/data/2.5/weather" API_KEY = "your_api_key" CITY = "London" response = requests.get(API_URL, params={"q": CITY, "appid": API_KEY}) data = response.json() # Extracting relevant information temperature = data['main']['temp'] humidity = data['main']['humidity'] weather_description = data['weather'][0]['description'] # Visualizing the data plt.bar(['Temperature', 'Humidity'], [temperature, humidity]) plt.title(f"Current Weather in {CITY}: {weather_description}") plt.show()
Incorporating data from these sources into your forecasting models is a fundamental step toward improving prediction accuracy. As we delve deeper into the techniques and methodologies of weather forecasting, understanding the nuances of these data sources will be key to using their full potential.
Data Collection Techniques for Weather Forecasting
When it comes to weather forecasting, the methods of data collection are as varied as the data sources themselves. Each technique comes with its own set of strengths and weaknesses, influencing the reliability and timeliness of the forecasts. Here, we will explore some of the most prominent data collection techniques used in the field of weather forecasting.
One prevalent technique is the use of Remote Sensing. This encompasses the collection of data from a distance, particularly through satellites and aerial drones. Remote sensing allows meteorologists to gather atmospheric data over large geographic areas without the need for physical weather stations. For example, satellite data can measure cloud cover and sea surface temperatures, which are critical for understanding larger weather systems. Python can be used to process and analyze this data effectively.
import numpy as np import matplotlib.pyplot as plt from netCDF4 import Dataset # Load satellite data (NetCDF format) data = Dataset('satellite_data.nc') # Extract relevant variables temp = data.variables['temperature'][:] latitude = data.variables['latitude'][:] longitude = data.variables['longitude'][:] # Plotting the temperature data plt.figure(figsize=(10, 6)) plt.contourf(longitude, latitude, temp[0, :, :], cmap='coolwarm') plt.colorbar(label='Temperature (K)') plt.title('Satellite Measured Temperature') plt.xlabel('Longitude') plt.ylabel('Latitude') plt.show()
Another key technique is the deployment of Automated Weather Stations (AWS). These stations collect data continuously and can provide real-time information on temperature, humidity, wind speed, and precipitation. They’re especially useful in remote areas where human observation is limited. Data from AWS especially important for short-term forecasting and can be easily ingested into Python for analysis and visualization.
import pandas as pd # Example: Load AWS data aws_data = pd.read_csv('aws_data.csv') # Display first few rows of the dataset print(aws_data.head()) # Plotting temperature over time plt.plot(aws_data['timestamp'], aws_data['temperature'], label='Temperature (°C)') plt.xlabel('Time') plt.ylabel('Temperature (°C)') plt.title('Temperature Readings from AWS') plt.legend() plt.show()
In addition to AWS, Radiosondes are deployed via weather balloons to collect data from the atmosphere at various altitudes. These instruments measure temperature, pressure, and humidity as they ascend through the atmosphere. The data collected provides insights into atmospheric profiles necessary for weather prediction models.
The integration of all these data collection techniques into a unified framework is essential for effective weather forecasting. Python, with its extensive libraries for data manipulation and analysis, serves as an ideal platform for handling and analyzing this diverse data. Through techniques such as data fusion, we can merge data from multiple sources, improving the accuracy and reliability of forecasts.
As we move forward into more advanced methods of forecasting, we must ponder how to best utilize these data collection techniques to improve our predictive models. The interplay between technology and meteorology continues to evolve, offering exciting possibilities for improving our understanding of weather phenomena.
Exploratory Data Analysis in Weather Prediction
Exploratory Data Analysis (EDA) is an important step in the weather prediction workflow, as it allows meteorologists and data scientists to understand the characteristics of the data they’re working with. EDA provides insights into the patterns, trends, and anomalies present in weather data, which can significantly influence the development of forecasting models. By employing various visualization techniques and statistical analyses, we can delve deeper into the data before applying machine learning algorithms.
One of the first steps in EDA is to visualize the distribution of key weather variables such as temperature, humidity, and precipitation. This can help identify any outliers or unusual patterns that may need special attention in subsequent analyses. Using libraries such as Matplotlib and Seaborn in Python, we can create informative visualizations that reveal the underlying structure of the data.
import pandas as pd import seaborn as sns import matplotlib.pyplot as plt # Load weather data weather_data = pd.read_csv('weather_data.csv') # Visualizing temperature distribution plt.figure(figsize=(10, 6)) sns.histplot(weather_data['temperature'], bins=30, kde=True) plt.title('Temperature Distribution') plt.xlabel('Temperature (°C)') plt.ylabel('Frequency') plt.show()
Beyond visualizing individual variables, examining relationships between different weather parameters is essential. Correlation analysis can help determine how different variables interact with one another, providing insights that can inform model selection. For instance, temperature and humidity are often correlated, and understanding this relationship can be beneficial when predicting weather phenomena.
# Correlation matrix correlation_matrix = weather_data.corr() # Plotting the correlation heatmap plt.figure(figsize=(10, 8)) sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm') plt.title('Correlation Matrix of Weather Variables') plt.show()
Time series analysis also plays a pivotal role in EDA for weather forecasting. Since weather data is inherently temporal, analyzing trends over time can provide valuable insights into seasonal patterns and unusual weather events. Techniques such as moving averages and seasonal decomposition can help highlight these trends.
# Time series visualization weather_data['timestamp'] = pd.to_datetime(weather_data['timestamp']) weather_data.set_index('timestamp', inplace=True) plt.figure(figsize=(12, 6)) plt.plot(weather_data['temperature'], label='Temperature (°C)') plt.title('Temperature Over Time') plt.xlabel('Date') plt.ylabel('Temperature (°C)') plt.legend() plt.show()
Furthermore, identifying and addressing missing values is critical in EDA, as they can skew the results of our analysis and subsequent forecasts. Various imputation techniques can be applied to fill these gaps, or we may choose to drop incomplete records altogether, depending on their significance.
# Checking for missing values missing_values = weather_data.isnull().sum() # Filling missing values with the mean weather_data.fillna(weather_data.mean(), inplace=True) print("Missing values after imputation:n", weather_data.isnull().sum())
As we engage with the data through EDA, we cultivate a holistic understanding of the various factors at play in weather phenomena. This foundational knowledge equips us to build more robust machine learning models that leverage these insights for better forecasting accuracy. The interplay between EDA and subsequent modeling phases ensures that our predictions are informed by a thorough analysis of the data landscape.
Implementing Machine Learning Models for Forecasting
Implementing machine learning models for weather forecasting is a sophisticated endeavor that marries statistical principles with computational techniques. As we transition from exploratory data analysis to model building, the goal is to improve our predictive capabilities using the patterns and insights we’ve uncovered. The choice of machine learning algorithms is critical, as it can significantly influence the accuracy and reliability of our forecasts.
One commonly used approach in weather forecasting is regression analysis, particularly when predicting continuous variables such as temperature or precipitation levels. Linear regression serves as a good starting point, but more complex models such as decision trees, random forests, and gradient boosting often yield superior results due to their ability to capture nonlinear relationships in the data.
For instance, let’s ponder implementing a Random Forest model to predict temperature based on various weather features. First, we need to prepare our dataset by splitting it into training and testing subsets, ensuring that our model can be evaluated effectively.
import pandas as pd from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestRegressor from sklearn.metrics import mean_squared_error # Load the weather data weather_data = pd.read_csv('weather_data.csv') # Features and target variable X = weather_data[['humidity', 'pressure', 'wind_speed']] # Example features y = weather_data['temperature'] # Target variable # Split the dataset X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Initialize and train the Random Forest model model = RandomForestRegressor(n_estimators=100, random_state=42) model.fit(X_train, y_train) # Make predictions y_pred = model.predict(X_test) # Evaluate the model mse = mean_squared_error(y_test, y_pred) print(f'Mean Squared Error: {mse:.2f}')
In the above code, we utilize the Random Forest regressor, a powerful ensemble learning technique that reduces the risk of overfitting by combining predictions from multiple decision trees. By evaluating the model’s performance with metrics such as mean squared error (MSE), we can gauge how well our model is likely to perform on unseen data.
Beyond regression models, we can also explore classification algorithms when predicting discrete weather events, such as whether it will rain or not. Here, logistic regression, support vector machines, or neural networks might be employed. The choice of algorithm will depend on the nature of the target variable and the complexity of the relationship between features and outcomes.
For example, let’s implement a logistic regression model to forecast the likelihood of rain based on humidity and temperature:
from sklearn.linear_model import LogisticRegression from sklearn.metrics import accuracy_score, confusion_matrix # Creating a binary target variable for rain (1 if rain, 0 if no rain) weather_data['rain'] = (weather_data['precipitation'] > 0).astype(int) # Features and target variable X_class = weather_data[['humidity', 'temperature']] # Example features for classification y_class = weather_data['rain'] # Target variable # Split the dataset X_train_class, X_test_class, y_train_class, y_test_class = train_test_split(X_class, y_class, test_size=0.2, random_state=42) # Initialize and train the logistic regression model logistic_model = LogisticRegression() logistic_model.fit(X_train_class, y_train_class) # Make predictions y_pred_class = logistic_model.predict(X_test_class) # Evaluate the model accuracy = accuracy_score(y_test_class, y_pred_class) conf_matrix = confusion_matrix(y_test_class, y_pred_class) print(f'Accuracy: {accuracy:.2f}') print(f'Confusion Matrix:n{conf_matrix}')
By using logistic regression, we can assess the probability of rain based on the defined features. The accuracy score provides insight into how well our model performs, while the confusion matrix allows us to visualize the performance in terms of true positives, true negatives, false positives, and false negatives.
Moreover, hyperparameter tuning plays a pivotal role in optimizing model performance. Techniques such as grid search or randomized search can be employed to systematically select the best parameters for our models.
As we delve deeper into the implementation of machine learning models for weather forecasting, it becomes evident that the synergy between data collection, preprocessing, feature engineering, and model selection is what ultimately drives the success of our predictions. By using the right algorithms and methodologies, we can significantly enhance the accuracy and reliability of weather forecasts, paving the way for more informed decision-making in various sectors impacted by weather conditions.
Evaluating and Visualizing Forecast Results
Once we have built our machine learning models for weather forecasting, the next critical step is evaluating and visualizing the forecast results. This stage not only assesses the model’s performance but also helps in understanding the predictions in a tangible manner. By employing a variety of evaluation metrics and visualization techniques, we can gain insights into the reliability of our forecasts and identify areas for improvement.
For regression models, metrics such as Mean Absolute Error (MAE), Mean Squared Error (MSE), and R-squared are commonly used to quantify the accuracy of predictions. These metrics provide a numerical representation of how close the predicted values are to the actual values. Let’s calculate these metrics for our Random Forest model used in the previous section:
from sklearn.metrics import mean_absolute_error, r2_score # Calculate evaluation metrics mae = mean_absolute_error(y_test, y_pred) r2 = r2_score(y_test, y_pred) print(f'Mean Absolute Error: {mae:.2f}') print(f'R-squared: {r2:.2f}')
In addition to numerical metrics, visualizing the predictions can provide an intuitive understanding of the model’s performance. One effective way to visualize the forecast results is by plotting the predicted values against the actual values. A scatter plot can help reveal how well the model is performing across different ranges of the target variable:
import matplotlib.pyplot as plt # Scatter plot of actual vs predicted values plt.figure(figsize=(10, 6)) plt.scatter(y_test, y_pred, alpha=0.6) plt.plot([y_test.min(), y_test.max()], [y_test.min(), y_test.max()], 'r--', lw=2) # Line of perfect prediction plt.title('Actual vs Predicted Temperature') plt.xlabel('Actual Temperature (°C)') plt.ylabel('Predicted Temperature (°C)') plt.grid() plt.show()
For classification models, accuracy alone may not be sufficient to evaluate performance, especially in cases of imbalanced datasets. Here, metrics such as precision, recall, F1-score, and the ROC-AUC curve come into play. These metrics help assess not just the overall accuracy but also the model’s ability to correctly classify positive instances (e.g., predicting rain accurately):
from sklearn.metrics import classification_report, roc_auc_score # Generate classification report report = classification_report(y_test_class, y_pred_class) roc_auc = roc_auc_score(y_test_class, logistic_model.predict_proba(X_test_class)[:, 1]) print("Classification Report:n", report) print(f'ROC-AUC Score: {roc_auc:.2f}')
Visualizing the confusion matrix can also provide a clear perspective on the performance of a classification model. It presents the number of true and false predictions in a tabular format, making it easier to understand where the model is excelling and where it might be falling short:
import seaborn as sns from sklearn.metrics import confusion_matrix # Confusion matrix visualization conf_matrix = confusion_matrix(y_test_class, y_pred_class) plt.figure(figsize=(8, 6)) sns.heatmap(conf_matrix, annot=True, fmt='d', cmap='Blues', xticklabels=['No Rain', 'Rain'], yticklabels=['No Rain', 'Rain']) plt.title('Confusion Matrix') plt.ylabel('Actual') plt.xlabel('Predicted') plt.show()
Visualizations play a pivotal role in conveying the performance of the forecasting models. By employing these techniques, meteorologists can communicate complex results in an understandable manner, which is especially crucial when informing stakeholders or the public about potential weather events.
Ultimately, the evaluation and visualization of forecast results are integral components of the weather prediction workflow. These steps not only validate the effectiveness of the models but also foster an environment of continuous improvement and refinement. By understanding the strengths and weaknesses of our forecasting models, we can make informed adjustments to enhance their predictive capabilities, ensuring that we stay ahead in the ever-evolving field of weather forecasting.