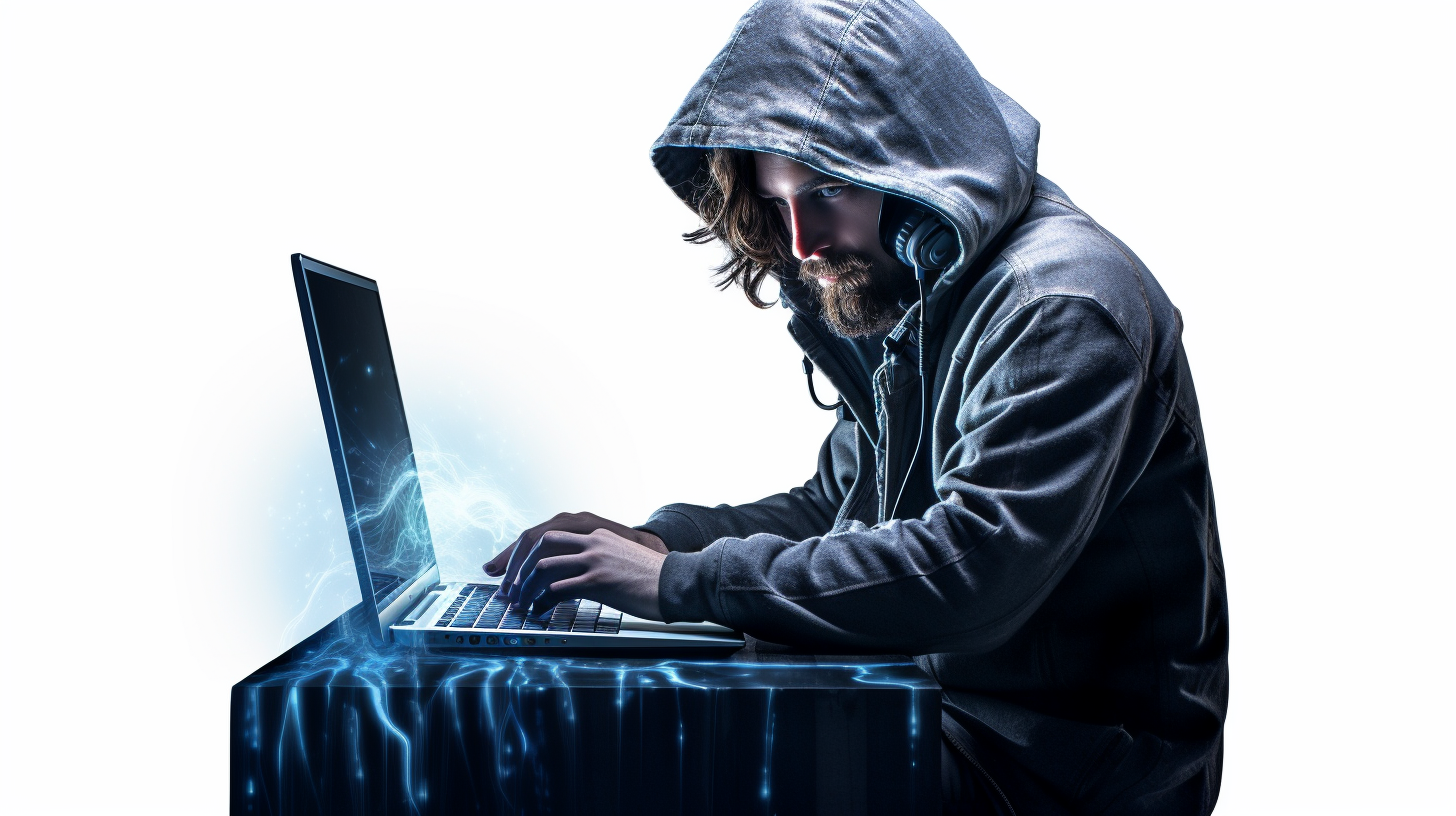
Python and Wildlife Conservation: Data Analysis
Data analysis serves as the backbone of modern wildlife conservation efforts, enabling researchers and conservationists to make informed decisions based on empirical evidence. The role of data analysis is multifaceted; it encompasses the collection, processing, and interpretation of vast amounts of biological and ecological data. With the advent of technology, the methodologies employed in data analysis have evolved significantly, allowing for more nuanced insights into wildlife populations, habitat conditions, and the impacts of human activities.
One primary aspect of data analysis in wildlife conservation is the ability to monitor species populations over time. By employing statistical models and algorithms, conservationists can track changes in species abundance and distribution, which very important for identifying trends that may indicate population declines or recoveries. For instance, using the Pandas library in Python, one can easily manipulate and analyze population data:
import pandas as pd # Load wildlife population data data = pd.read_csv('wildlife_population.csv') # Group by species and calculate mean population mean_population = data.groupby('species')['population'].mean() print(mean_population)
Furthermore, data analysis helps to evaluate the effectiveness of conservation strategies. By applying methods such as spatial analysis and predictive modeling, researchers can assess how various conservation initiatives influence wildlife habitats and populations. For example, using the Geopandas library allows for the analysis of geographic data related to animal movements and habitat use:
import geopandas as gpd # Load habitat data habitat = gpd.read_file('habitat_areas.shp') # Plot species distribution over habitat areas habitat.plot()
Additionally, advanced data analysis techniques such as machine learning have become invaluable tools in wildlife conservation. By using machine learning algorithms, conservationists can predict outcomes of various interventions or identify potential threats to wildlife. The following example demonstrates a simple implementation of a classification algorithm using the Scikit-learn library:
from sklearn.model_selection import train_test_split from sklearn.ensemble import RandomForestClassifier # Prepare dataset X = data[['feature1', 'feature2', 'feature3']] y = data['species_endangered'] # Split data into training and testing sets X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3) # Train Random Forest Classifier clf = RandomForestClassifier() clf.fit(X_train, y_train) # Evaluate model accuracy = clf.score(X_test, y_test) print(f'Model accuracy: {accuracy:.2f}')
Techniques for Collecting Wildlife Data
Collecting wildlife data is the first and most critical step in the conservation process. The techniques used for data collection can vary widely, ranging from traditional field surveys to sophisticated remote sensing technologies. Each method has its advantages and limitations, and often, a combination of techniques is employed to ensure comprehensive data gathering.
One common technique in wildlife data collection is the use of camera traps. These remote cameras automatically capture images when an animal passes by, providing invaluable information about species presence, behavior, and population density. The images collected can then be analyzed using Python libraries such as OpenCV for object detection or image classification. The following code snippet illustrates how to use OpenCV to count the number of times a specific animal appears in the captured images:
import cv2 import os # Path to the directory containing camera trap images image_folder = 'camera_trap_images/' animal_count = 0 # Load pre-trained model for animal detection model = cv2.CascadeClassifier('animal_cascade.xml') # Iterate through all images for filename in os.listdir(image_folder): if filename.endswith('.jpg'): image_path = os.path.join(image_folder, filename) img = cv2.imread(image_path) gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY) # Detect animals in the image animals = model.detectMultiScale(gray, 1.1, 4) animal_count += len(animals) print(f'Total number of animals detected: {animal_count}')
In addition to camera traps, field surveys are a hallmark of wildlife data collection. These surveys can involve direct observation, tracking, and capturing animals for physical examination. To analyze such field survey data, researchers often use Python’s Pandas library to manage and process large datasets effectively. The following example demonstrates how to load and summarize data collected from a field survey:
import pandas as pd # Load field survey data survey_data = pd.read_csv('field_survey_data.csv') # Summarize the data by species species_summary = survey_data.groupby('species')['count'].sum() print(species_summary)
Another innovative technique in wildlife data collection is the use of GPS collars. These collars provide real-time location data on animal movements, allowing researchers to analyze habitat use and migration patterns. Python can be leveraged to visualize this data using libraries like Matplotlib and Folium. The following example illustrates how to plot animal movement paths on a map:
import folium # Load GPS tracking data tracking_data = pd.read_csv('gps_tracking_data.csv') # Create a map centered around the first GPS coordinate map_center = [tracking_data['latitude'].mean(), tracking_data['longitude'].mean()] wildlife_map = folium.Map(location=map_center, zoom_start=10) # Plot the animal's movement path folium.PolyLine(locations=tracking_data[['latitude', 'longitude']].values, color='blue').add_to(wildlife_map) # Save the map to an HTML file wildlife_map.save('wildlife_tracking_map.html')
Moreover, advanced technologies such as drone surveys are revolutionizing wildlife data collection. Drones can cover vast areas quickly and capture high-resolution images, which can be analyzed to assess habitat conditions or conduct population counts. The integration of drone-collected data into conservation efforts can be facilitated via Python’s powerful data manipulation and analysis libraries.
Case Studies: Successful Conservation Efforts Using Python
In recent years, several case studies have highlighted the successful integration of Python in wildlife conservation efforts, showcasing how data analysis can lead to significant improvements in species protection and habitat preservation. One notable example is the African Wildlife Foundation’s initiative to monitor the populations of endangered elephants in Kenya. By employing Python for data analysis, conservationists were able to process large datasets derived from GPS collar tracking and aerial surveys. The analysis enabled them to identify critical habitats and migration corridors, which are essential for the elephants’ survival.
The implementation of Python’s libraries, such as Pandas for data manipulation and Matplotlib for data visualization, played an important role in synthesizing and interpreting this data. The following code snippet demonstrates how to analyze and visualize elephant movement patterns using GPS data:
import pandas as pd import matplotlib.pyplot as plt # Load GPS tracking data for elephants gps_data = pd.read_csv('elephant_gps_data.csv') # Convert timestamp to datetime gps_data['timestamp'] = pd.to_datetime(gps_data['timestamp']) # Filter data for a specific elephant elephant_id = 'E123' elephant_data = gps_data[gps_data['elephant_id'] == elephant_id] # Plot the movement path plt.figure(figsize=(10, 6)) plt.plot(elephant_data['longitude'], elephant_data['latitude'], marker='o', linestyle='-', color='green') plt.title(f'Movement Path of Elephant {elephant_id}') plt.xlabel('Longitude') plt.ylabel('Latitude') plt.grid() plt.show()
Another impactful case study involves the use of camera traps in the Amazon rainforest to study jaguar populations. Researchers leveraged Python to automate the classification of images captured by these traps, significantly reducing the time required for manual analysis. By using machine learning libraries such as TensorFlow, they developed a model that could identify and count jaguars accurately in the images. The following code snippet illustrates how to train a simple image classification model for jaguar detection:
import tensorflow as tf from tensorflow.keras.preprocessing.image import ImageDataGenerator # Prepare the dataset train_datagen = ImageDataGenerator( rescale=1./255, validation_split=0.2 ) train_gen = train_datagen.flow_from_directory( 'jaguars', target_size=(150, 150), batch_size=32, class_mode='binary', subset='training' ) validation_gen = train_datagen.flow_from_directory( 'jaguars', target_size=(150, 150), batch_size=32, class_mode='binary', subset='validation' ) # Build and train the model model = tf.keras.models.Sequential([ tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(150, 150, 3)), tf.keras.layers.MaxPooling2D(pool_size=(2, 2)), tf.keras.layers.Flatten(), tf.keras.layers.Dense(64, activation='relu'), tf.keras.layers.Dense(1, activation='sigmoid') ]) model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) model.fit(train_gen, validation_data=validation_gen, epochs=10)
These case studies exemplify how Python not only facilitates data collection and analysis but also enhances the effectiveness of conservation strategies through the integration of advanced computational techniques. The ability to analyze large datasets allows conservationists to make data-driven decisions that can lead to successful outcomes, such as improving species management and habitat restoration efforts.
Moreover, the collaboration between conservation organizations, data scientists, and software engineers has resulted in the development of innovative tools that streamline data collection and analysis processes. For instance, the Wildbook project uses Python to develop a citizen science platform where volunteers can contribute to wildlife monitoring by submitting photos of animals. The platform employs image recognition algorithms to match submitted photos to existing databases, greatly expanding the reach of wildlife tracking initiatives.
Future Trends in Data-Driven Wildlife Conservation
The future of data-driven wildlife conservation is poised to be transformative, fueled by advancements in technology and methodologies that enable more precise and efficient data analysis. As we venture further into the age of big data, conservation efforts will increasingly rely on sophisticated algorithms, machine learning, and artificial intelligence to process vast amounts of ecological information. This shift not only enhances our understanding of wildlife dynamics but also improves the effectiveness of conservation interventions.
One significant trend is the increasing use of remote sensing technologies. Satellite imagery and aerial surveys, combined with Python’s powerful data processing capabilities, will allow conservationists to monitor habitats on a macro scale. For example, the integration of remote sensing data with machine learning models can help identify habitat changes over time due to climate change or human encroachment. The following code snippet illustrates how to use the Rasterio library to analyze satellite imagery for land cover classification:
import rasterio import numpy as np import matplotlib.pyplot as plt # Load satellite image with rasterio.open('satellite_image.tif') as src: image = src.read(1) # Display the image plt.imshow(image, cmap='gray') plt.title('Satellite Image') plt.colorbar() plt.show() # Example of thresholding for land cover classification land_cover = np.where(image > 100, 1, 0) # Simple thresholding for vegetation plt.imshow(land_cover, cmap='green') plt.title('Land Cover Classification') plt.show()
Moreover, the rise of citizen science platforms, supported by Python-based applications, empowers the public to contribute to wildlife monitoring efforts. This democratization of data collection not only increases the volume of data but also enhances community engagement in conservation. Tools such as mobile applications for reporting sightings play a critical role in gathering real-time data from diverse locations. The following code snippet demonstrates how to create a simple web application using Flask to report wildlife sightings:
from flask import Flask, request, jsonify app = Flask(__name__) # Store sightings in-memory (for example purposes) sightings = [] @app.route('/report', methods=['POST']) def report_sighting(): data = request.json sightings.append(data) return jsonify({'status': 'success', 'message': 'Sighting reported!'}), 201 if __name__ == '__main__': app.run(debug=True)
Furthermore, the integration of artificial intelligence into wildlife conservation is expected to revolutionize the way we analyze and predict wildlife behavior. Deep learning models can be trained on vast datasets to recognize patterns in animal movements and interactions, providing insights that were previously unreachable. For instance, convolutional neural networks (CNNs) can be employed to analyze images captured from camera traps, allowing for more efficient species identification and population estimates. The following example illustrates how to implement a CNN for wildlife image classification:
import tensorflow as tf from tensorflow.keras.models import Sequential from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense # Define the CNN model model = Sequential([ Conv2D(32, (3, 3), activation='relu', input_shape=(150, 150, 3)), MaxPooling2D(pool_size=(2, 2)), Conv2D(64, (3, 3), activation='relu'), MaxPooling2D(pool_size=(2, 2)), Flatten(), Dense(128, activation='relu'), Dense(1, activation='sigmoid') ]) # Compile the model model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Assume we have prepared training and validation datasets model.fit(train_data, validation_data=validation_data, epochs=10)
Lastly, the importance of collaborative efforts among conservationists, technologists, and data scientists cannot be overstated. Partnerships will lead to the development of new tools and frameworks that enhance data analysis capabilities while ensuring that conservation strategies are grounded in scientific rigor. This collaboration is important for creating adaptive management strategies that can respond to emerging threats and changing ecological conditions.