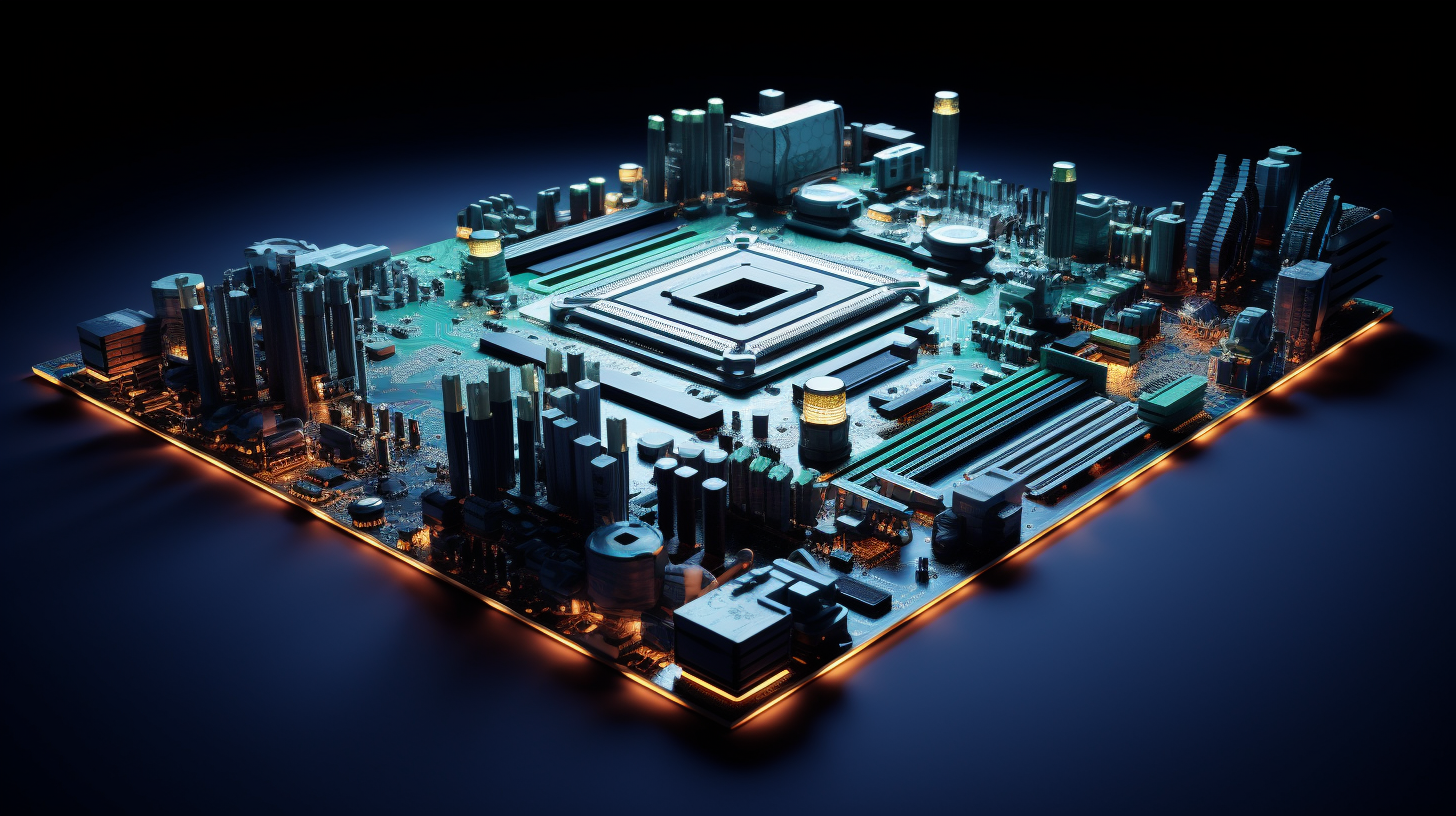
Python Classes and Objects: An Introduction
In Python, a class is a blueprint for creating objects. Objects are instances of classes and encapsulate data for the object along with methods to manipulate that data. This object-oriented approach allows for better organization of code and reusability, creating a more intuitive structure for programming.
To understand classes, ponder of them as templates. Just as you might have a blueprint for a house that specifies how many rooms, floors, and windows there are, a class defines the properties and behaviors of the objects you will create from it. This blueprint does not represent any particular house; rather, it describes the general concept of what a house can be.
In Python, defining a class starts with the class
keyword, followed by the class name and a colon. Class names typically use the CapWords convention. Here’s a simple example:
class Dog: pass
This class definition creates a new class called Dog. Currently, it doesn’t have any attributes or methods, but it sets the stage for what we can add later. You can ponder of this as having the framework of a house without yet adding any rooms or features.
Classes can also have attributes (variables that belong to the class) and methods (functions that belong to the class). Attributes hold data relevant to the class, while methods define behaviors that can be performed by instances of the class. To illustrate this, here’s how you might expand the Dog class to include an attribute and a method:
class Dog: def __init__(self, name): self.name = name # Attribute def bark(self): # Method return f"{self.name} says woof!"
In this enhanced version, when you create an instance of Dog, you can provide a name. The __init__
method is a special method called a constructor, which initializes the object’s state. The bark method lets the dog “speak” by returning a string that incorporates the dog’s name.
Classes in Python provide a powerful way to structure your code. They enable encapsulation, inheritance, and polymorphism, which are core principles of object-oriented programming. By using classes, you can create complex programs that are easier to manage, extend, and debug.
Creating Your First Class
To create your first class in Python, you’ll need to understand how to define attributes and methods that belong to that class. Attributes are the data that the objects of your class will hold, while methods are the functions that define the behaviors of those objects. Let’s dive deeper into the class definition and how to work with attributes and methods effectively.
When you define a class, the __init__
method plays an important role. This method is automatically called when a new object is created from the class, which will allow you to set initial values for the object’s attributes. Here’s an example that expands on the previous Dog class, adding more attributes and methods:
class Dog: def __init__(self, name, breed): self.name = name # Attribute: name self.breed = breed # Attribute: breed def bark(self): # Method: bark return f"{self.name} says woof!" def info(self): # Method: info return f"{self.name} is a {self.breed}."
In this version of the Dog class, we have added a new attribute, breed
, which allows us to create more descriptive Dog objects. The info
method provides a way to retrieve a formatted string that gives information about the dog. Now that we have a more detailed class, let’s see how to instantiate objects from this class:
my_dog = Dog("Buddy", "Golden Retriever") print(my_dog.bark()) # Output: Buddy says woof! print(my_dog.info()) # Output: Buddy is a Golden Retriever.
Here, we create an instance of Dog called my_dog
, passing in a name and breed. We then call the bark
and info
methods to see how our Dog object behaves. This encapsulation of attributes and methods is what makes classes in Python so powerful.
Classes can further be enhanced with more complex methods, properties, and even class-level attributes that apply to all instances of the class. The ability to organize and structure your code through classes leads to improved readability and maintainability.
Once you have a grasp of how to create classes, you’ll find that designing your programs using this object-oriented approach allows for elegant solutions to complex problems, thus embodying the true spirit of Python programming.
Working with Class Attributes and Methods
When working with classes in Python, understanding the distinction between instance attributes and class attributes especially important. Instance attributes are unique to each object created from the class, while class attributes are shared across all instances of that class. This distinction allows for a flexible design that can accommodate different behaviors and properties at both the instance and class levels.
Here’s how you can define class attributes in addition to instance attributes:
class Dog: species = "Canis familiaris" # Class attribute def __init__(self, name, breed): self.name = name # Instance attribute self.breed = breed # Instance attribute def bark(self): return f"{self.name} says woof!" def info(self): return f"{self.name} is a {self.breed} and belongs to the species {Dog.species}."
In this example, the species
attribute is a class attribute that all instances of Dog
share. Even when you create different dogs, they will all refer to the same species. Notice how we access the class attribute inside the info
method using Dog.species
. This approach maintains a clean separation between the data unique to each object and the data common to all objects of the class.
Let’s see how this works in practice:
my_dog = Dog("Buddy", "Golden Retriever") print(my_dog.bark()) # Output: Buddy says woof! print(my_dog.info()) # Output: Buddy is a Golden Retriever and belongs to the species Canis familiaris. another_dog = Dog("Max", "Bulldog") print(another_dog.info()) # Output: Max is a Bulldog and belongs to the species Canis familiaris.
Both my_dog
and another_dog
have their unique name
and breed
attributes, yet they share the same species
class attribute. This illustrates the benefit of class attributes: they allow you to manage shared data efficiently without redundancy.
Methods can also be defined at the class level—known as class methods—using the @classmethod
decorator. Class methods operate on the class itself rather than on instances, making them useful for factory methods that can return class instances based on certain conditions. Here’s how you would implement a class method:
class Dog: species = "Canis familiaris" def __init__(self, name, breed): self.name = name self.breed = breed @classmethod def create_puppy(cls, name): return cls(name, "Unknown Breed") def bark(self): return f"{self.name} says woof!" def info(self): return f"{self.name} is a {self.breed} and belongs to the species {Dog.species}."
With this create_puppy
method, you can easily create a new instance of Dog
without specifying the breed:
puppy = Dog.create_puppy("Charlie") print(puppy.info()) # Output: Charlie is a Unknown Breed and belongs to the species Canis familiaris.
This approach encapsulates the creation logic within the class itself, promoting maintainability and clarity in your code. By using both instance attributes and class attributes, as well as methods, you can build robust and flexible class designs that enhance your Python programming experience.
Instantiating Objects
Instantiating objects in Python is the process of creating an instance of a class. Once a class is defined, you can create multiple objects from it, each with its own unique state based on the attributes defined in the class. This allows for the modeling of real-world entities in a way this is both intuitive and efficient.
To instantiate an object, you simply call the class as if it were a function, passing any required parameters that correspond to the class’s constructor (the __init__
method). For example, using the Dog class defined earlier, you can create instances like this:
my_dog = Dog("Buddy", "Golden Retriever") your_dog = Dog("Max", "Bulldog")
Here, my_dog
and your_dog
are separate instances of the Dog class. Each instance maintains its own state, reflected in its attributes. Let’s look at how we can interact with these objects:
print(my_dog.bark()) # Output: Buddy says woof! print(your_dog.info()) # Output: Max is a Bulldog and belongs to the species Canis familiaris.
In the code snippet above, each object can call its methods independently. The bark
method returns a message unique to the my_dog
instance, while the info
method provides details about your_dog
. This illustrates the encapsulated nature of each object, where methods operate on the instance’s attributes.
Furthermore, instantiation can be used to create collections of objects. For example, if you wanted to manage a group of dogs, you could store them in a list:
dogs = [Dog("Buddy", "Golden Retriever"), Dog("Max", "Bulldog"), Dog("Charlie", "Beagle")] for dog in dogs: print(dog.info())
This loop iterates through the list of Dog instances, calling the info
method on each one, which will allow you to easily manage and interact with multiple instances.
Instantiating objects is a foundational aspect of working with classes in Python. It enables the creation of distinct entities derived from a common blueprint, each capable of independent behavior and state. This not only enhances code modularity but also aligns closely with real-world modeling, making your programs more relatable and simpler to organize.
Inheritance and Polymorphism in Python
Inheritance and polymorphism are two fundamental concepts in object-oriented programming that allow for more flexible and reusable code. Inheritance enables a new class to inherit attributes and methods from an existing class, promoting code reuse and establishing a natural hierarchy between classes. Polymorphism, on the other hand, allows different classes to be treated as instances of the same class through a shared interface, even if the underlying implementations are different.
To illustrate inheritance, let’s build upon our Dog class and create a new class called Puppy. This subclass will inherit from the Dog class, meaning it will have access to the attributes and methods defined in Dog:
class Dog: species = "Canis familiaris" def __init__(self, name, breed): self.name = name self.breed = breed def bark(self): return f"{self.name} says woof!" def info(self): return f"{self.name} is a {self.breed} and belongs to the species {Dog.species}." class Puppy(Dog): def __init__(self, name): super().__init__(name, "Puppy") # Inherits from Dog and sets breed to 'Puppy' def play(self): return f"{self.name} is playing!" puppy = Puppy("Charlie") print(puppy.bark()) # Output: Charlie says woof! print(puppy.info()) # Output: Charlie is a Puppy and belongs to the species Canis familiaris. print(puppy.play()) # Output: Charlie is playing!
In this example, the Puppy class inherits from the Dog class. In the constructor of the Puppy class, we call super().__init__(name, "Puppy")
to initialize the inherited attributes. This means that every Puppy instance can use the methods defined in Dog, such as bark
and info
, while also introducing its unique behavior through the play
method.
Now let’s move on to polymorphism. In Python, polymorphism allows methods to be called on objects of different classes, as long as they share the same method name. That’s particularly useful in scenarios where you want to handle objects from different classes through a common interface.
Let’s define another class, Cat, which also has a bark
method, but it will behave differently:
class Cat: def __init__(self, name): self.name = name def bark(self): return f"{self.name} says meow!" # A function that demonstrates polymorphism def animal_sound(animal): print(animal.bark()) dog = Dog("Buddy", "Golden Retriever") cat = Cat("Luna") animal_sound(dog) # Output: Buddy says woof! animal_sound(cat) # Output: Luna says meow!
In this example, the animal_sound function takes an animal
argument and calls the bark
method on it. Both Dog and Cat have a bark
method, allowing this function to work with instances of both classes seamlessly. This illustrates how polymorphism can simplify code and provide greater flexibility.
By using inheritance and polymorphism in your Python classes, you can create a more organized and efficient codebase. These principles allow you to build complex systems that are easier to maintain and extend, ultimately leading to a more powerful object-oriented design.