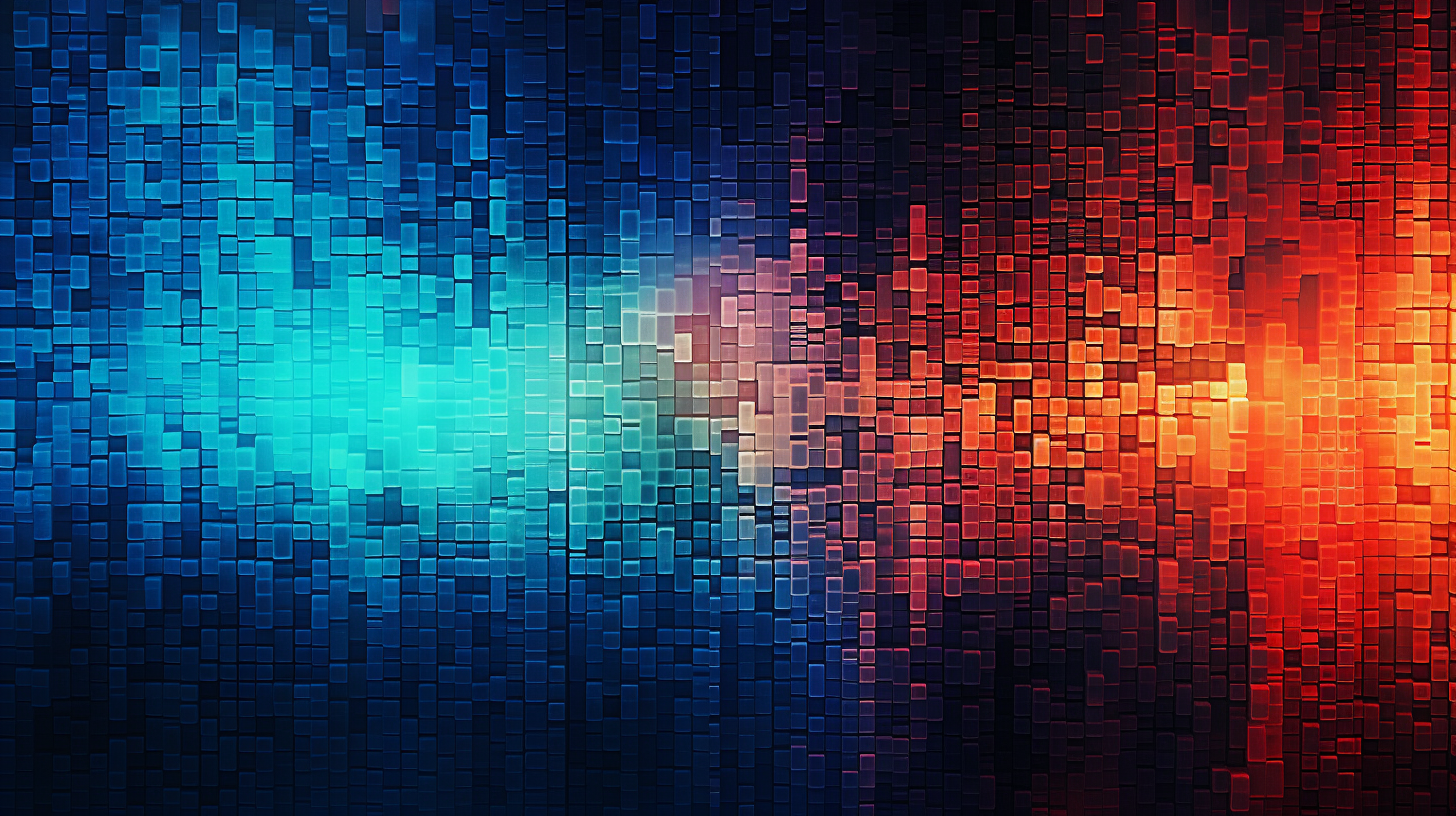
Python Conditional Statements Explained
Conditional statements are a fundamental aspect of programming in Python, enabling developers to direct the flow of execution based on specific conditions. At their core, conditional statements allow a program to make decisions: if a condition evaluates to true, a block of code will execute; if it evaluates to false, that block will be skipped. This ability to branch logic is what makes programming dynamic and powerful.
In Python, conditional statements are primarily implemented using the if, elif, and else constructs. Understanding how these statements operate especially important for creating algorithms that can adapt to varying inputs and situations.
When executing conditional statements, Python evaluates the expression right after the if keyword. Depending on whether this expression is true or false, the program will either execute the associated block of code or move to the next condition, if one exists. The basic syntax looks like this:
if condition: # execute this block if condition is true elif another_condition: # execute this block if the previous condition was false and this one is true else: # execute this block if all previous conditions were false
This simple structure allows developers to handle multiple scenarios cleanly and efficiently. Think the following example, which checks the temperature and assigns clothing recommendations:
temperature = 30 if temperature > 25: print("It's warm outside, wear shorts.") elif temperature > 15: print("The weather is mild, a light jacket will do.") else: print("It's chilly, better wear a coat.")
In this example, based on the value of temperature, the program decides what clothing is appropriate. As the temperature changes, different print statements are triggered, demonstrating how conditional statements can be utilized to create responsive and adaptable programs.
Understanding this basic mechanism allows for more complex logic to be built around it, including nested conditional statements, which can lead to more intricate decision-making processes in your code.
The Syntax of If, Elif, and Else
The syntax for conditional statements in Python is both simpler and powerful, enabling developers to craft intricate decision-making algorithms with ease. The primary keywords involved in this process—if, elif, and else—are used to control the flow based on the conditions provided.
At the heart of conditional syntax is the if statement, which checks a condition and executes the following block of code if that condition is true. Following an if statement, you can include one or more elif statements to check subsequent conditions. The else statement acts as a catch-all, executing its block of code only if all preceding conditions are false.
Here’s a closer look at the syntax:
if condition1: # Block of code to execute if condition1 is true elif condition2: # Block of code to execute if condition1 is false and condition2 is true elif condition3: # Additional checks as needed else: # Block of code to execute if all previous conditions are false
This structure allows for clear and organized decision-making in your code. Consider the following example:
age = 20 if age < 13: print("You are a child.") elif age < 20: print("You are a teenager.") else: print("You are an adult.")
In this scenario, the program evaluates the variable age against several conditions. Depending on the value of age, the output will differ, illustrating how conditional logic can reflect different states or classifications in your program.
Importantly, the conditions can be any valid expression that evaluates to a boolean (True or False). These expressions can include comparisons, logical operations, or even function calls that return a boolean value. This flexibility allows for a wide range of applications in programming.
For instance, you can combine conditions using logical operators such as and, or, and not, allowing for more nuanced decision-making. Think the following example that incorporates logical operators:
temperature = 20 is_raining = False if temperature > 25 and not is_raining: print("It's a great day for the beach!") elif temperature > 15 and is_raining: print("It's mild but raining; take an umbrella.") else: print("Stay indoors; it is either too cold or raining.")
In this code, both the temperature and the weather condition (is_raining) are evaluated to determine the appropriate response. The use of logical operators expands the potential for making complex decisions based on multiple factors.
By mastering the syntax of if, elif, and else, along with the use of logical operators, you will be able to create programs that can respond dynamically to a variety of conditions, making your code not just functional but also intelligent in its decision-making capabilities.
Nested Conditional Statements
Nested conditional statements are a powerful tool in Python that allow you to further refine decision-making processes in your applications. By embedding one or more conditional statements within another, you can create a hierarchy of checks, enabling your program to evaluate multiple layers of conditions before arriving at a conclusion. That’s particularly useful when the outcome depends not only on a primary condition but also on additional criteria.
The syntax for nested conditional statements mirrors that of the basic conditional constructs, but with an added layer of indentation to denote the inner blocks. The structure typically looks like this:
if condition1: # Block to execute if condition1 is true if condition2: # Block to execute if condition2 is also true else: # Block to execute if condition2 is false else: # Block to execute if condition1 is false
To illustrate this concept, ponder an example where we assess a student’s performance based on their score:
score = 85 if score >= 60: print("You passed!") if score >= 90: print("Excellent job!") elif score >= 75: print("Good job!") else: print("You did well, but there's room for improvement.") else: print("You failed. Please try again.")
In this example, the outer conditional statement checks whether the score is 60 or higher. If so, it then proceeds to a nested check that categorizes the performance further based on the score’s value. This layering of conditions allows for a nuanced response to the student’s performance, enabling more detailed feedback.
When using nested conditionals, clarity is paramount. As complexity increases, so does the risk of introducing logical errors or making the code difficult to read. It is advisable to keep nesting to a reasonable level and ponder alternatives, such as functions or dictionaries, when the logic becomes convoluted.
Here’s another example that highlights the use of nested conditionals in a real-world context, such as determining the type of product discount based on the customer’s status:
customer_status = "VIP" purchase_amount = 150 if customer_status == "VIP": discount = 20 if purchase_amount > 100: discount += 10 # Additional discount for large purchases print(f"You receive a {discount}% discount!") elif customer_status == "Regular": discount = 10 print(f"You receive a {discount}% discount!") else: print("No discounts available.")
In this scenario, the program first checks the customer’s status. If the customer is a VIP, it then checks how much they spent to potentially increase their discount. Here, nested conditionals facilitate a tailored response that accommodates different customer segments and purchasing behaviors.
By mastering nested conditional statements, you can enhance your programs’ ability to handle complex decision-making processes, ensuring that they respond accurately to a wide range of scenarios. This skill is a hallmark of proficient Python programming, allowing you to craft solutions that are both flexible and powerful.
Common Use Cases for Conditional Statements
Conditional statements in Python are not just limited to the basic checks we’ve examined—they can be utilized in a variety of practical scenarios to streamline processes, enhance user interaction, and make applications more responsive. Understanding the common use cases for conditional statements can significantly elevate your programming prowess.
One of the most prevalent applications of conditional statements is in user input validation. When developing applications that rely on user data, ensuring that this data meets specific criteria is paramount. For example, think a scenario where a program collects a user’s age and ensures it falls within a valid range:
age = int(input("Please enter your age: ")) if age < 0: print("Age cannot be negative.") elif age < 18: print("You are a minor.") elif age < 65: print("You are an adult.") else: print("You are a senior citizen.")
In this snippet, conditional statements are used to categorize ages into different groups, providing appropriate feedback to the user based on their input. This approach not only enhances user experience but also safeguards your application from processing invalid data.
Another valuable use case for conditional statements is in configuring application settings or behaviors based on environment variables. For instance, consider a web application that needs to adjust its logging level based on the execution environment (development or production):
import os environment = os.getenv("APP_ENV", "development") # Default to development if environment == "production": log_level = "ERROR" elif environment == "staging": log_level = "WARNING" else: log_level = "DEBUG" print(f"Logging level set to: {log_level}")
This example showcases how conditional statements can be leveraged to adapt application behavior dynamically based on the context in which it is running, thereby enhancing both performance and security.
Conditional statements are also extensively used in implementing game logic. For example, consider a simple game where a player’s score determines their progression:
score = 450 if score >= 500: print("You've reached the highest level!") elif score >= 250: print("You're doing great, keep it up!") else: print("You need to score higher to progress.")
In this scenario, the player’s score dictates the narrative, allowing for dynamic game progression based on their performance. This responsiveness is central to creating engaging gaming experiences.
Moreover, conditional statements can be employed in financial applications to evaluate transactions based on user profiles. For instance, ponder a banking application that applies different interest rates based on account types:
account_type = "savings" balance = 1000 if account_type == "savings": interest_rate = 0.05 elif account_type == "checking": interest_rate = 0.01 else: interest_rate = 0.0 interest_earned = balance * interest_rate print(f"Interest earned: ${interest_earned:.2f}")
Here, the interest rate is determined based on the type of account, illustrating how conditional statements can help perform calculations that vary depending on user-specific criteria.
The versatility of conditional statements in Python opens up a plethora of opportunities for developers. Whether validating input, adjusting application settings, enhancing game logic, or calculating financial metrics, these statements serve as the backbone of responsive programming. By mastering their use in various contexts, you will be well-equipped to handle a wide range of programming challenges with finesse and adaptability.