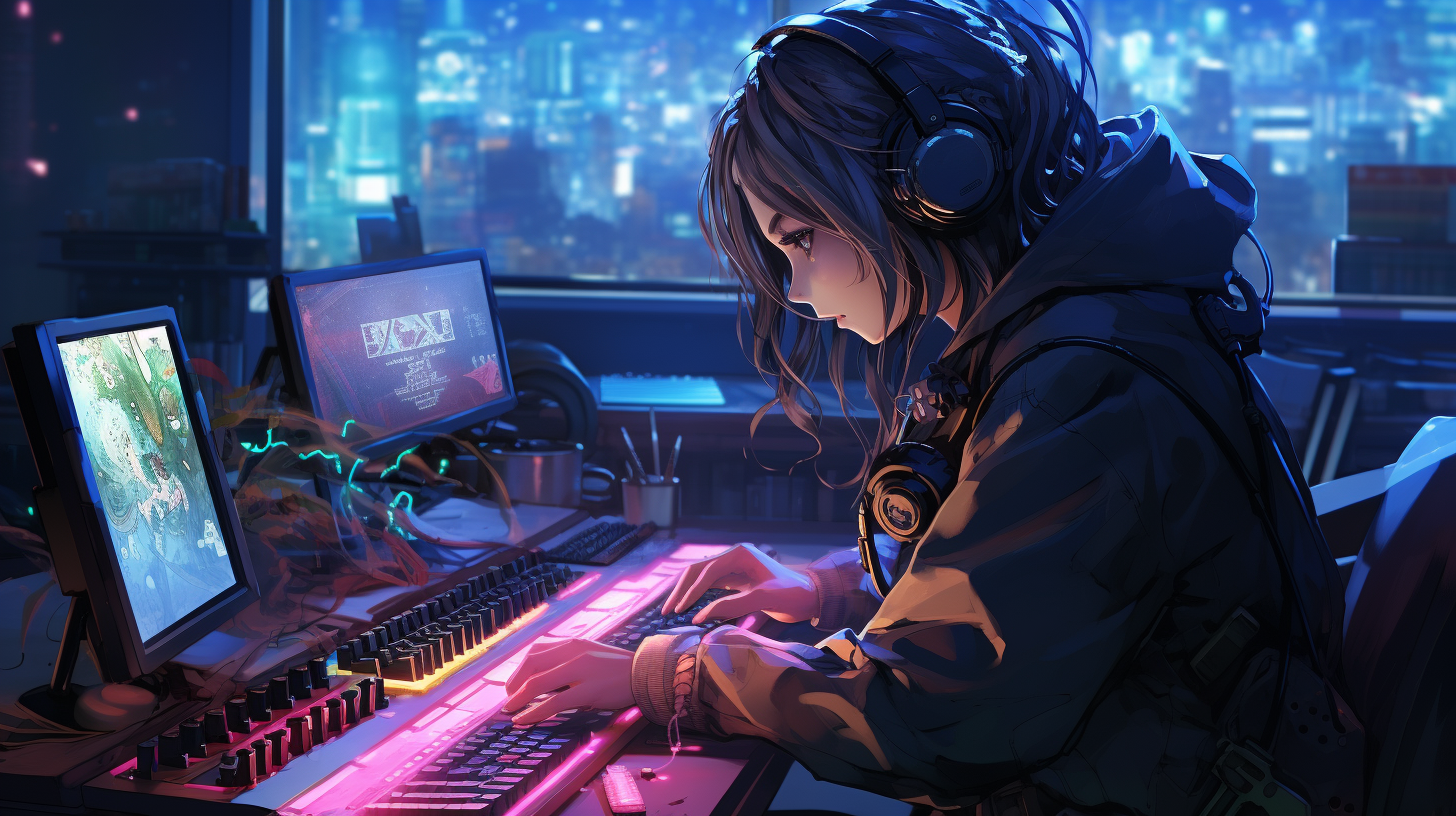
Python Decorators: Enhancing Functions
Python decorators are a powerful and expressive tool that allow you to modify the behavior of functions or methods in a clean and readable way. At their core, decorators are simply functions that take another function as an argument and extend or alter its behavior without permanently modifying the original function.
To understand decorators, it is essential to grasp the idea of higher-order functions, which are functions that can take other functions as arguments or return them as results. This feature of Python enables decorators to be elegantly implemented.
Ponder the following simple example of a decorator:
def my_decorator(func): def wrapper(): print("Something is happening before the function is called.") func() print("Something is happening after the function is called.") return wrapper @my_decorator def say_hello(): print("Hello!") say_hello()
In this code snippet, we define a decorator called my_decorator, which takes a function func as an input. Inside my_decorator, we define a nested function wrapper that incorporates additional behavior before and after calling func. The @my_decorator syntax is syntactic sugar that allows us to apply the decorator to the say_hello function without explicitly calling it.
When we run say_hello(), the output will reveal the additional functionality provided by the decorator:
Something is happening before the function is called. Hello! Something is happening after the function is called.
This illustrates how decorators can be used to extend functionality in a clean, maintainable way. Instead of cluttering the core logic of say_hello with pre- and post-execution logic, the decorator cleanly encapsulates this behavior, making the code easier to read and manage.
Moreover, decorators can be stacked, meaning you can apply multiple decorators to a single function. This allows for even greater flexibility and modularity in your code, promoting the DRY (Don’t Repeat Yourself) principle.
Overall, by using the power of decorators, Python developers can write more modular, reusable, and clean code, all while enhancing functionality in an intuitive manner.
Types of Decorators: Function and Class
In the context of Python decorators, one can broadly categorize them into two types: function decorators and class decorators. Understanding the distinction between these two categories is fundamental to using decorators effectively in your Python applications.
Function Decorators
Function decorators are the most common type. As previously illustrated, they are functions that accept a function as an argument and return a new function that typically extends or modifies the behavior of the original function. The primary purpose of function decorators is to enhance functionality in a reusable manner while keeping the original function unchanged.
Consider a more elaborate example of a function decorator that measures the execution time of a function:
import time def time_decorator(func): def wrapper(*args, **kwargs): start_time = time.time() result = func(*args, **kwargs) end_time = time.time() print(f"{func.__name__} executed in {end_time - start_time:.6f} seconds") return result return wrapper @time_decorator def compute_sum(n): return sum(range(n)) compute_sum(1000000)
In this example, the time_decorator
measures the time it takes to execute the compute_sum
function. The wrapper
function captures the start and end times, calculates the difference, and prints it out, demonstrating how decorators can be leveraged for performance monitoring.
Class Decorators
On the other hand, class decorators operate at the class level. They can modify or enhance the behavior of classes, similar to how function decorators work at the function level. Class decorators take a class as an argument and return a new class or an instance of a class that usually extends the functionality of the original class.
Here’s an illustrative example of a class decorator that adds a method to a class:
def add_method(cls): cls.new_method = lambda self: "This is a new method added to the class." return cls @add_method class MyClass: def original_method(self): return "This is the original method." instance = MyClass() print(instance.original_method()) print(instance.new_method())
In this example, the decorator add_method
takes MyClass
as an argument and adds a new method called new_method
. When we create an instance of MyClass
, we can access both the original and the newly added method, showcasing the versatility of class decorators.
Both function and class decorators provide powerful tools for modifying behavior in a clean and maintainable way. By understanding these types, Python developers can apply decorators judiciously to improve their code structure, promote reuse, and encapsulate functionality effectively.
Creating Custom Decorators
def log_function_call(func): def wrapper(*args, **kwargs): print(f"Function '{func.__name__}' called with arguments: {args} and keyword arguments: {kwargs}") return func(*args, **kwargs) return wrapper @log_function_call def calculate_area(width, height): return width * height area = calculate_area(10, 5) print(f"Area: {area}")
Creating custom decorators is not just about wrapping functions; it is about designing reusable components that can be tailored to specific needs while preserving the integrity of the original function. Let’s delve deeper into the mechanics of custom decorators and explore their flexibility.
A decorator typically follows a pattern where it contains a wrapper function that performs some operation before or after calling the original function. This allows us to introduce additional behavior without modifying the original function’s code directly. The beauty of custom decorators lies in their ability to accept arguments as well, enabling even greater customization.
def repeat(num_times): def decorator_repeat(func): def wrapper(*args, **kwargs): for _ in range(num_times): result = func(*args, **kwargs) return result return wrapper return decorator_repeat @repeat(num_times=3) def greet(name): print(f"Hello, {name}!") greet("Alice")
In the example above, the repeat
function is a decorator factory, meaning it returns a decorator that, in turn, wraps the function. The num_times
parameter allows us to specify how many times the function should be executed. When we call greet
, it prints the greeting three times, demonstrating how decorators can be both powerful and adaptable.
Another important aspect of custom decorators is preserving the metadata of the original function. By default, the wrapper function does not retain the original function’s name or docstring. To tackle this, Python provides the functools.wraps
decorator, which is essential for maintaining the original function’s identity within the wrapper. Here’s how you can use it:
from functools import wraps def logged(func): @wraps(func) def wrapper(*args, **kwargs): print(f"Logging: {func.__name__} was called") return func(*args, **kwargs) return wrapper @logged def multiply(x, y): """Multiplies two numbers.""" return x * y print(multiply(3, 4)) print(multiply.__name__) # This will correctly show 'multiply' print(multiply.__doc__) # This will show the docstring
By using functools.wraps
, the wrapper
function captures the original function’s name and docstring, ensuring that introspection remains accurate. This practice especially important for maintaining code clarity, especially when decorators are extensively used in larger codebases.
Creating custom decorators in Python is an art that combines flexibility, reusability, and clarity. Whether enhancing function behavior, adding logging details, or implementing complex logic, decorators provide a powerful mechanism to enrich your Python applications without compromising readability or maintainability.
Practical Applications of Decorators in Python
Practical applications of decorators in Python are vast and varied, showcasing their ability to add functionality without modifying the original code. They can be employed for various purposes, such as logging, enforcing access control, caching results, and even measuring execution time. By using decorators, developers can write cleaner and more organized code while maintaining the integrity of the underlying functions.
One common use case for decorators is logging function calls. That is particularly useful for debugging and monitoring the behavior of applications in production. By wrapping functions with logging decorators, developers can automatically track when and how functions are invoked, along with their arguments.
def log_execution(func): def wrapper(*args, **kwargs): print(f"Executing {func.__name__} with arguments: {args} and {kwargs}") return func(*args, **kwargs) return wrapper @log_execution def fetch_data(query): print(f"Fetching data for query: {query}") fetch_data("SELECT * FROM users")
In this example, the log_execution
decorator logs the function name and its arguments each time fetch_data
is called. This approach allows developers to gain insights into the function’s usage without scattering print statements throughout the code.
Another compelling application of decorators is access control in web applications. By creating a decorator that checks user permissions, developers can enforce security constraints without repeating the same logic in multiple endpoints.
def require_admin(func): def wrapper(user, *args, **kwargs): if not user.is_admin: raise PermissionError("User does not have admin privileges.") return func(user, *args, **kwargs) return wrapper @require_admin def delete_user(user, user_id): print(f"User {user_id} deleted by {user.name}") class User: def __init__(self, name, is_admin): self.name = name self.is_admin = is_admin admin_user = User("Alice", True) regular_user = User("Bob", False) delete_user(admin_user, 1) # This works delete_user(regular_user, 2) # This raises PermissionError
In this case, the require_admin
decorator checks if the user has administrative privileges before allowing the deletion of a user account. This encapsulation of logic ensures that the codebase remains clean while enforcing necessary security measures.
Caching results is another practical application of decorators, especially in scenarios where function calls are expensive in terms of computation or I/O operations. By caching the results of a function, subsequent calls with the same arguments can return quickly without recalculating the result.
def cache(func): memo = {} def wrapper(*args): if args in memo: return memo[args] result = func(*args) memo[args] = result return result return wrapper @cache def fibonacci(n): if n <= 1: return n return fibonacci(n - 1) + fibonacci(n - 2) print(fibonacci(30)) # Fast computation due to caching
The cache
decorator stores the results of previous calculations in a dictionary, allowing for rapid retrieval on subsequent calls with the same input. This strategy dramatically improves performance for computationally intensive algorithms like the Fibonacci sequence.
Lastly, decorators can also be used to measure performance metrics, allowing developers to optimize their code by identifying bottlenecks. By wrapping functions with a timing decorator, it becomes simpler to log execution times and identify which parts of the codebase require attention.
import time def time_it(func): def wrapper(*args, **kwargs): start_time = time.time() result = func(*args, **kwargs) end_time = time.time() print(f"{func.__name__} took {end_time - start_time:.4f} seconds") return result return wrapper @time_it def long_running_task(): time.sleep(2) # Simulating a long-running task long_running_task()
With the time_it
decorator, we can easily track how long long_running_task
takes to execute, providing valuable data for performance analysis.
Through these practical applications, decorators demonstrate their utility across various programming paradigms. By promoting code reuse, enhancing readability, and encapsulating behavior, Python decorators empower developers to build robust and maintainable applications with ease.