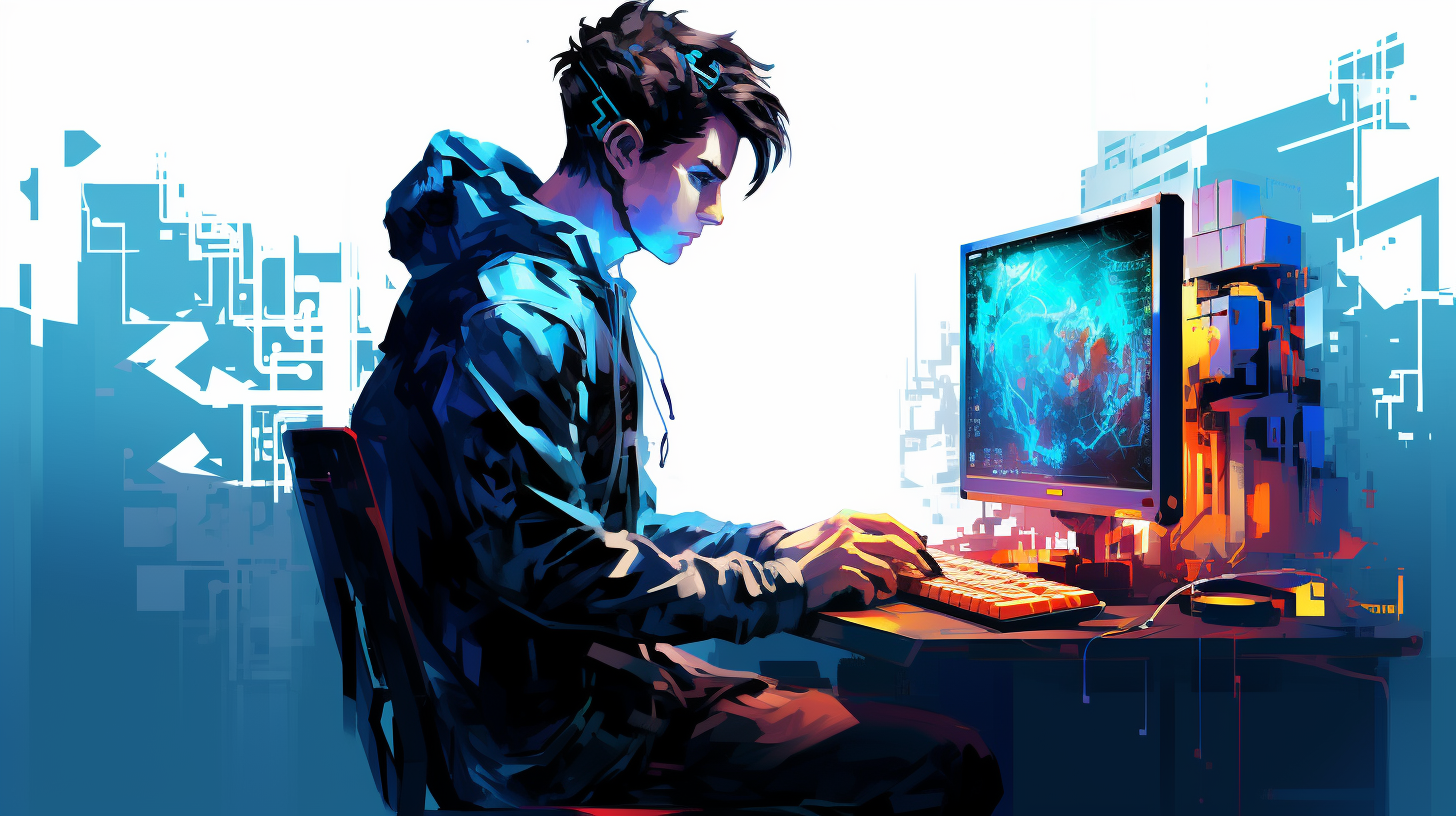
Python Dictionaries: Managing Key-Value Pairs
In Python, a dictionary is a versatile data structure that allows you to store and manage data in key-value pairs. This structure is akin to a real-world dictionary where each word (key) is associated with a definition (value). The beauty of dictionaries is that they enable fast access to data, which makes them invaluable for tasks that require frequent data retrieval.
Key Characteristics of Python Dictionaries:
- Unlike lists, dictionaries maintain no specific order. The insertion order is preserved in Python 3.7 and later, but reliance on this behavior can lead to code that’s less readable and maintainable.
- Dictionaries are mutable, which means you can change them after creation. You can add, update, or remove key-value pairs at any time.
- Each value in a dictionary is accessed using its corresponding key, allowing for efficient retrieval.
- Dictionaries can grow and shrink in size as needed, accommodating the changes in the data being handled.
To create a dictionary in Python, you can use curly braces or the dict()
constructor. Here are two common methods:
# Using curly braces my_dict = {"name": "Alice", "age": 30, "city": "New York"} # Using the dict constructor my_dict = dict(name="Alice", age=30, city="New York")
In both examples, the keys are strings that describe the data, and the values are the associated data points, which can be of any data type, including lists or even other dictionaries.
One of the most powerful aspects of Python dictionaries is their ability to handle dynamic data structures. This flexibility allows for advanced use cases, such as creating multi-level dictionaries or using them to represent complex objects. For instance, you can nest dictionaries to represent a more complex data structure:
# Nested dictionary student_info = { "student1": {"name": "Alice", "age": 30, "grades": [90, 95, 85]}, "student2": {"name": "Bob", "age": 22, "grades": [88, 92, 90]}, }
This nesting capability is particularly useful for representing real-world entities where a single key-value pair is insufficient to encapsulate all relevant information. By using dictionaries, developers can build robust applications that require efficient data management and retrieval.
Creating and Initializing Dictionaries
To expand on the topic of creating and initializing dictionaries in Python, it is important to recognize the various ways you can populate a dictionary beyond the simpler examples previously mentioned. One particularly useful method is dictionary comprehension, which allows you to create dictionaries in a concise and expressive manner.
Dictionary comprehension utilizes a similar syntax to list comprehension, enabling the construction of a dictionary from an iterable in a single line of code. That is especially handy when you need to generate keys and values dynamically based on existing data. Here’s an example that demonstrates how to create a dictionary that maps numbers to their squares:
squares = {x: x**2 for x in range(1, 6)} print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
In this example, the dictionary comprehension iterates over a range of numbers from 1 to 5, creating a key-value pair for each number and its square. It’s a clear demonstration of how Python allows for elegant and efficient coding practices.
Another method to initialize a dictionary is to use the `fromkeys()` method, which is particularly useful when you want to create a dictionary with default values for multiple keys. The `fromkeys()` method takes a sequence (like a list or tuple) and a default value, populating the dictionary with keys from the sequence and the specified value. Here’s how it works:
keys = ['name', 'age', 'city'] default_value = None my_dict = dict.fromkeys(keys, default_value) print(my_dict) # Output: {'name': None, 'age': None, 'city': None}
In this instance, `my_dict` is initialized with keys from the `keys` list, and each key is assigned a default value of `None`. This method is useful in scenarios where you want to create a template for a dictionary without immediately populating all values.
For those who prefer more granular control, you can also initialize an empty dictionary and populate it iteratively. That’s done using assignment statements, which can be particularly useful when data is gathered from various sources or when complex logic is required to determine values:
my_dict = {} my_dict['name'] = 'Alice' my_dict['age'] = 30 my_dict['city'] = 'New York' print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
In this example, the dictionary is created empty, and individual key-value pairs are added one by one. This approach offers flexibility, especially when dealing with conditionally derived values.
Accessing and Modifying Values
Accessing values in a Python dictionary is a simpler task that’s both efficient and intuitive. By using the keys associated with each value, you can quickly retrieve or manipulate the data within the dictionary. The most common method for accessing a value is by using square brackets with the corresponding key. Here’s a simple example:
my_dict = {"name": "Alice", "age": 30, "city": "New York"} print(my_dict["name"]) # Output: Alice
In this case, the value associated with the key “name” is accessed directly, resulting in the output “Alice”. However, it’s essential to think scenarios where the key might not exist in the dictionary. Attempting to access a non-existent key raises a KeyError. To handle such situations gracefully, you can use the get() method, which allows you to specify a default value to return if the key is absent:
age = my_dict.get("age", "Not Found") print(age) # Output: 30 salary = my_dict.get("salary", "Not Found") print(salary) # Output: Not Found
In this example, trying to access the “salary” key, which doesn’t exist in the dictionary, returns “Not Found” instead of raising an error. This method enhances the robustness of your code by preventing unexpected crashes and allowing for smoother user experiences.
Modifying values in a dictionary is equally simpler. You can update an existing key’s value simply by assigning a new value to that key:
my_dict["age"] = 31 print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'city': 'New York'}
Here, the age of “Alice” is updated from 30 to 31. If you attempt to update a key that does not exist, Python will add that key-value pair to the dictionary:
my_dict["salary"] = 70000 print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'city': 'New York', 'salary': 70000}
This dynamic nature of dictionaries allows for fluid data manipulation, making them suitable for a multitude of applications. Another way to modify dictionaries is through the update() method, which merges another dictionary or an iterable of key-value pairs into the existing dictionary:
updates = {"city": "San Francisco", "country": "USA"} my_dict.update(updates) print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'city': 'San Francisco', 'salary': 70000, 'country': 'USA'}
Using the update() method, the existing key “city” is updated, and a new key “country” is added seamlessly. This method is particularly useful for batch updating or enriching dictionaries with additional data.
Finally, if you find the need to remove a key-value pair, you can do so using the del statement or the pop() method. The del statement removes the entry without returning its value:
del my_dict["city"] print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'salary': 70000, 'country': 'USA'}
In contrast, the pop() method removes the specified key and returns its associated value:
salary = my_dict.pop("salary") print(salary) # Output: 70000 print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'country': 'USA'}
In this instance, the “salary” key is removed, and its value is returned, showcasing a handy way to access the data being removed in one operation.
Common Dictionary Methods
When it comes to Python dictionaries, understanding the common methods available to manipulate them is essential for using their full potential. These methods provide an array of functionalities that allow developers to perform various operations with ease and efficiency. Here, we will delve into some of the most frequently used dictionary methods, showcasing their usage with practical examples.
1. get()
The get()
method is important for safely accessing values in a dictionary. Unlike direct key access, which can raise a KeyError
if the key is not found, get()
allows you to specify a default return value. This feature enhances the robustness of your code by preventing unexpected crashes.
my_dict = {"name": "Alice", "age": 30} # Accessing existing key name = my_dict.get("name", "Unknown") print(name) # Output: Alice # Accessing a non-existent key with a default value salary = my_dict.get("salary", "Not Found") print(salary) # Output: Not Found
2. keys(), values(), and items()
These three methods allow you to retrieve the keys, values, and key-value pairs from a dictionary, respectively. They return view objects that dynamically reflect changes in the dictionary.
my_dict = {"name": "Alice", "age": 30, "city": "New York"} # Getting keys keys = my_dict.keys() print(keys) # Output: dict_keys(['name', 'age', 'city']) # Getting values values = my_dict.values() print(values) # Output: dict_values(['Alice', 30, 'New York']) # Getting key-value pairs items = my_dict.items() print(items) # Output: dict_items([('name', 'Alice'), ('age', 30), ('city', 'New York')])
3. update()
The update()
method allows you to merge another dictionary or an iterable of key-value pairs into the existing dictionary. This method is invaluable for batch updates and combining data from multiple sources.
my_dict = {"name": "Alice", "age": 30} updates = {"city": "New York", "age": 31} my_dict.update(updates) print(my_dict) # Output: {'name': 'Alice', 'age': 31, 'city': 'New York'}
4. pop()
Removing items from a dictionary can be accomplished using the pop()
method, which not only deletes the specified key but also returns its value. This method is particularly useful when you need to access the value being removed.
my_dict = {"name": "Alice", "age": 30, "city": "New York"} # Popping an existing key age = my_dict.pop("age") print(age) # Output: 30 print(my_dict) # Output: {'name': 'Alice', 'city': 'New York'} # Popping a non-existent key with a default value city = my_dict.pop("country", "Not Found") print(city) # Output: Not Found
5. clear()
If you wish to empty a dictionary, the clear()
method will remove all items within it, leaving you with an empty dictionary.
my_dict = {"name": "Alice", "age": 30} my_dict.clear() print(my_dict) # Output: {}
6. setdefault()
The setdefault()
method is a convenient way to retrieve a value for a specified key while also setting a default value if that key does not exist. This method allows for streamlined data retrieval and initialization in a single operation.
my_dict = {"name": "Alice"} # Retrieving the value for an existing key age = my_dict.setdefault("age", 30) print(age) # Output: 30 print(my_dict) # Output: {'name': 'Alice', 'age': 30} # Setting a default for a non-existing key city = my_dict.setdefault("city", "New York") print(city) # Output: New York print(my_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}
Best Practices for Using Dictionaries
When it comes to using dictionaries in Python, adhering to best practices can significantly enhance the clarity, efficiency, and maintainability of your code. Here are several strategies that can help you wield dictionaries more effectively:
1. Key Uniqueness: Ensure that keys in a dictionary are unique. That’s fundamental since each key maps to a single value. If you attempt to insert a duplicate key, the old value will be overwritten without warning.
my_dict = {"name": "Alice", "age": 30, "name": "Bob"} # Only 'name' will be 'Bob' print(my_dict) # Output: {'name': 'Bob', 'age': 30}
2. Choosing Hashable Keys: When selecting keys, make sure they’re hashable, which typically means using immutable types like strings, numbers, or tuples. Lists and other mutable types cannot serve as dictionary keys.
my_dict = {["key1"]: "value1"} # This will raise a TypeError.
3. Use the get() Method for Access: Always prefer the get() method over direct key access when retrieving values. This practice prevents your program from crashing due to KeyErrors and allows for smoother data handling.
value = my_dict.get("non_existent_key", "Default Value") # Safe retrieval print(value) # Output: Default Value
4. Default Values with setdefault(): When initializing keys that may not exist yet, the setdefault() method is invaluable. This method not only retrieves the value but also initializes it if absent, which is particularly useful in counting or aggregating scenarios.
counts = {} for item in ["apple", "banana", "apple"]: counts.setdefault(item, 0) # Initialize if absent counts[item] += 1 print(counts) # Output: {'apple': 2, 'banana': 1}
5. Avoid Mutable Values: If possible, avoid using mutable objects (like lists or dictionaries) as values in a dictionary. This can lead to unexpected behavior when the value is modified, as all references to that value will reflect the change, potentially causing hard-to-trace bugs.
my_dict = {"key": []} my_dict["key"].append("item") # Affects all references to my_dict["key"] print(my_dict) # Output: {'key': ['item']}
6. Dictionary Comprehensions: Utilize dictionary comprehensions for concise and readable dictionary creation. This feature facilitates the generation of dictionaries from iterables in a single expression, enhancing both brevity and clarity.
squares = {x: x**2 for x in range(1, 6)} print(squares) # Output: {1: 1, 2: 4, 3: 9, 4: 16, 5: 25}
7. Documentation and Comments: Always document your dictionaries. Brief comments about the purpose of the keys and values can be extremely helpful for anyone reading your code (including your future self). Clear variable names and docstrings can prevent confusion and misinterpretation.
# User details dictionary user_info = { "username": "johndoe", # Unique identifier for the user "email": "[email protected]", # User's email address }
8. Ponder Alternative Structures: While dictionaries are powerful, ponder if a different data structure might better serve your needs. For example, if order matters, `collections.OrderedDict` (older Python versions) or using lists of tuples can sometimes be more appropriate.