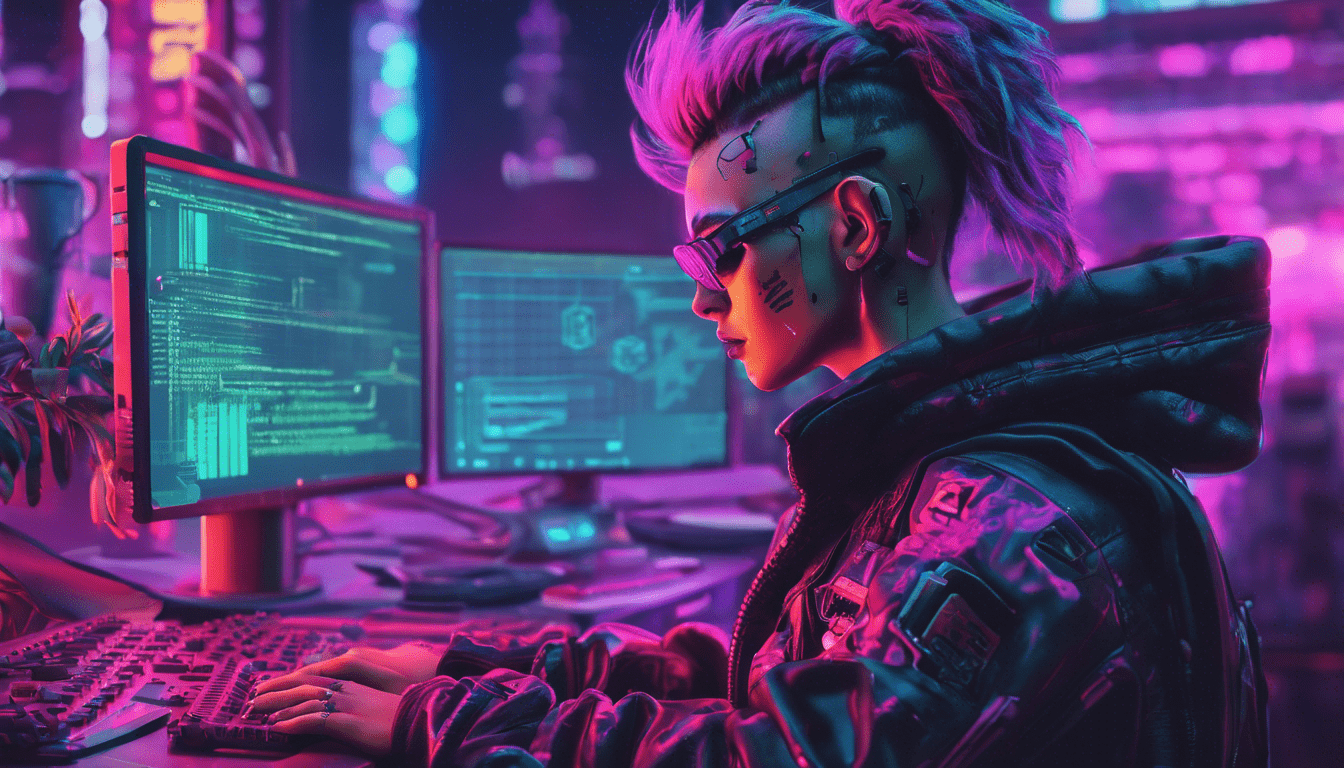
Python for Archaeology: Data Analysis and Visualization
Welcome to a beginner-friendly guide on using Python for data analysis and visualization in the field of archaeology. Python is a versatile programming language that offers powerful tools for working with historical data and creating compelling visualizations. In this article, we will explore key concepts, provide step-by-step examples, address common pitfalls, and recommend further learning resources.
Detailed Explanation of Concepts
Before we dive into the practical implementation, let’s explore some key concepts related to Python for archaeology data analysis and visualization.
1. Data Types: Python offers various data types such as strings, integers, floats, lists, and dictionaries. Understanding these data types is essential for organizing and manipulating archaeological data.
# Example of using different data types in Python # String artifact_name = 'Amphora' # Integer artifact_age = 2000 # Float artifact_weight = 12.5 # List excavation_years = [2018, 2019, 2020] # Dictionary artifact_details = { 'name': 'Amphora', 'age': 2000, 'weight': 12.5 }
2. Data Cleaning and Preparation: Archaeological data often require cleaning and preparation before analysis. Python provides libraries like Pandas that offer powerful tools for cleaning, transforming, and organizing data.
import pandas as pd # Load archaeological data from a CSV file archaeology_data = pd.read_csv('archaeology_data.csv') # Drop missing values archaeology_data = archaeology_data.dropna() # Filter data based on specific criteria filtered_data = archaeology_data[archaeology_data['age'] > 1000] # Convert data types filtered_data['age'] = filtered_data['age'].astype(int) # Select specific columns selected_columns = filtered_data[['name', 'age']]
3. Data Analysis: With cleaned and prepared data, Python offers a wide range of libraries for statistical analysis. NumPy and SciPy provide tools for mathematical operations, while scikit-learn facilitates machine learning tasks.
import numpy as np # Calculate mean age mean_age = np.mean(filtered_data['age']) # Calculate standard deviation std_deviation = np.std(filtered_data['age'])
4. Data Visualization: Python has popular visualization libraries such as Matplotlib and Seaborn that enable the creation of captivating visualizations to represent archaeological findings.
import matplotlib.pyplot as plt # Create a histogram of artifact ages plt.hist(filtered_data['age'], bins=10, alpha=0.5) plt.xlabel('Age (in years)') plt.ylabel('Count') plt.title('Distribution of Artifact Ages') plt.show()
Step-by-Step Guide
Now that we understand the key concepts, let’s walk through a step-by-step guide on implementing data analysis and visualization in Python for archaeology:
- Visit the official Python website (https://www.python.org) and download the latest version of Python. Install it on your computer.
- Install Required Libraries: Open your command prompt or terminal and use the following commands to install the necessary libraries:
pip install pandas numpy matplotlib
- Prepare the Data: Ensure you have an archaeological dataset in a suitable format like CSV. Load the dataset using Pandas and perform necessary data cleaning and preparation steps.
import pandas as pd # Load archaeological data from a CSV file archaeology_data = pd.read_csv('archaeology_data.csv') # Perform data cleaning steps # Perform data preparation steps
- Analyze the Data: Utilize the powerful tools provided by libraries like NumPy and SciPy to perform exploratory data analysis and statistical calculations.
import numpy as np # Perform data analysis operations # Perform statistical calculations
- Visualize the Data: Use Matplotlib or other visualization libraries to create meaningful visual representations of your archaeological findings.
import matplotlib.pyplot as plt # Create visualizations
Common Pitfalls and Troubleshooting Tips
As a newbie in Python for archaeology, you may encounter some common pitfalls. Here are a few troubleshooting tips to help you overcome them:
- Ensure that your archaeological dataset is in a compatible format, such as CSV, and matches the expected structure.
- Make sure the libraries you’re using are compatible with the Python version you have installed. Verify library versions and consult the official documentation for any issues.
- Handle missing data appropriately by using techniques like dropping rows with missing values or imputing missing values with suitable strategies.
Further Learning Resources
To further enhance your skills in Python for archaeology, ponder exploring these resources:
- By Wes McKinney – A comprehensive book that introduces data analysis using Python and Pandas.
- An online learning platform with interactive Python courses for data analysis and visualization.
- A platform that hosts machine learning competitions and provides datasets for practice. Explore archaeological datasets and learn from other professionals’ code.
Congratulations on embarking on your journey into Python for archaeology data analysis and visualization. Understanding key concepts, following a step-by-step guide, troubleshooting common issues, and continuously learning from additional resources will help you become proficient in using Python for your archaeological projects. With Python’s powerful tools at your disposal, you can make meaningful discoveries and present them visually to engage and inform others in the field of archaeology.